how to combine php array of object by unique id?
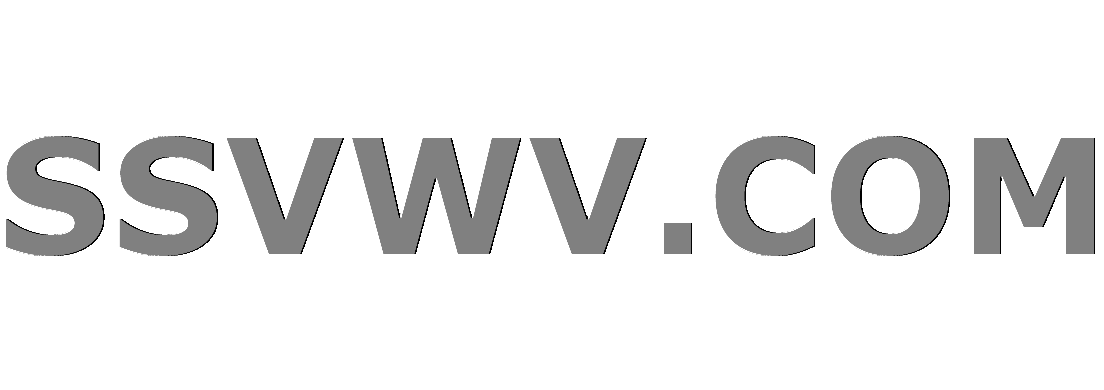
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I have these 2 array.
First array is from user input $cart
:
array(3) {
[0]=>
array(3) {
["id"]=>"3"
["weight"]=>"20"
["percentage"]=>"80"
}
[1]=>
array(3) {
["id"]=>"1"
["weight"]=>"50"
["percentage"]=>"80"
}
[2]=>
array(3) {
["id"]=>"2"
["weight"]=>"40"
["percentage"]=>"80"
}
}
and second array, I do a database SELECT id, stock WHERE id IN (3,1,2)
, resulting $db_item
array(3) {
[0]=>
array(2) {
["id"]=>"1"
["stock"]=>"9539.00"
}
[1]=>
array(2) {
["id"]=>"2"
["stock"]=>"9468.00"
}
[2]=>
array(2) {
["id"]=>"3"
["stock"]=>"9295.00"
}
}
I want to add the stock attribute in second array to first array.
This is what I tried, and it works, but I don't think it is necessary to have foreach
, array_filter
, and array_column
:
foreach ($cart as $key => $cart_item) {
$item = array_filter($db_item, function($item) use ($cart_item) {
return $item['id'] === $cart_item['id'];
});
$cart[$key]['stock'] = array_column($item, 'stock')[0];
}
anyone has better idea how to optimize this?
EDIT: following Mohammad's answer, I can use more attribute in second array
$keys = ;
foreach ($arr2 as $item) {
$keys[$item['id']] = array(
'attr1' => $item['attr1'],
'attr2' => $item['attr2'],
// and so on
);
}
$newArr = array_map(function($item) use($keys){
$item['attr1'] = $keys[$item['id']]['attr1'];
$item['attr2'] = $keys[$item['id']]['attr2'];
// and so on
return $item;
}, $arr1);
EDIT2: found out that we can simplify the foreach loop with just a single line using array_column
.
$keys = array_column($arr2, null, 'id');
$newArr = array_map(function($item) use($keys){
$item['attr1'] = $keys[$item['id']]['attr1'];
$item['attr2'] = $keys[$item['id']]['attr2'];
// and so on
return $item;
}, $arr1);
php arrays multidimensional-array
|
show 2 more comments
I have these 2 array.
First array is from user input $cart
:
array(3) {
[0]=>
array(3) {
["id"]=>"3"
["weight"]=>"20"
["percentage"]=>"80"
}
[1]=>
array(3) {
["id"]=>"1"
["weight"]=>"50"
["percentage"]=>"80"
}
[2]=>
array(3) {
["id"]=>"2"
["weight"]=>"40"
["percentage"]=>"80"
}
}
and second array, I do a database SELECT id, stock WHERE id IN (3,1,2)
, resulting $db_item
array(3) {
[0]=>
array(2) {
["id"]=>"1"
["stock"]=>"9539.00"
}
[1]=>
array(2) {
["id"]=>"2"
["stock"]=>"9468.00"
}
[2]=>
array(2) {
["id"]=>"3"
["stock"]=>"9295.00"
}
}
I want to add the stock attribute in second array to first array.
This is what I tried, and it works, but I don't think it is necessary to have foreach
, array_filter
, and array_column
:
foreach ($cart as $key => $cart_item) {
$item = array_filter($db_item, function($item) use ($cart_item) {
return $item['id'] === $cart_item['id'];
});
$cart[$key]['stock'] = array_column($item, 'stock')[0];
}
anyone has better idea how to optimize this?
EDIT: following Mohammad's answer, I can use more attribute in second array
$keys = ;
foreach ($arr2 as $item) {
$keys[$item['id']] = array(
'attr1' => $item['attr1'],
'attr2' => $item['attr2'],
// and so on
);
}
$newArr = array_map(function($item) use($keys){
$item['attr1'] = $keys[$item['id']]['attr1'];
$item['attr2'] = $keys[$item['id']]['attr2'];
// and so on
return $item;
}, $arr1);
EDIT2: found out that we can simplify the foreach loop with just a single line using array_column
.
$keys = array_column($arr2, null, 'id');
$newArr = array_map(function($item) use($keys){
$item['attr1'] = $keys[$item['id']]['attr1'];
$item['attr2'] = $keys[$item['id']]['attr2'];
// and so on
return $item;
}, $arr1);
php arrays multidimensional-array
Why not get all the required data in a single query. If you show us the 2 queries, someone will tell you how to get all the info in one query
– RiggsFolly
Nov 16 '18 at 13:36
@RiggsFolly but first array is from user input, not from the query
– Christhofer Natalius
Nov 16 '18 at 13:36
Ah, good point :) Missed that :) :(
– RiggsFolly
Nov 16 '18 at 13:37
2
Funny thing, I have something of this nature in a cart system, but I just used two foreach... which is most likely the same performance as array_filter, since it rolls over the array just the same. We are talking mini-milli-seconds, so you probably are ok with what you have... unless you just want to make it more readable for anyone coming along your code in the future.
– IncredibleHat
Nov 16 '18 at 13:40
1
In programming, one of the best ways to optimize array lookups is the use of hash tables. PHP's associative arrays are an easy to use form of this. However, when dealing with only a few elements, I think any optimization is overoptimization.
– Devon
Nov 16 '18 at 13:47
|
show 2 more comments
I have these 2 array.
First array is from user input $cart
:
array(3) {
[0]=>
array(3) {
["id"]=>"3"
["weight"]=>"20"
["percentage"]=>"80"
}
[1]=>
array(3) {
["id"]=>"1"
["weight"]=>"50"
["percentage"]=>"80"
}
[2]=>
array(3) {
["id"]=>"2"
["weight"]=>"40"
["percentage"]=>"80"
}
}
and second array, I do a database SELECT id, stock WHERE id IN (3,1,2)
, resulting $db_item
array(3) {
[0]=>
array(2) {
["id"]=>"1"
["stock"]=>"9539.00"
}
[1]=>
array(2) {
["id"]=>"2"
["stock"]=>"9468.00"
}
[2]=>
array(2) {
["id"]=>"3"
["stock"]=>"9295.00"
}
}
I want to add the stock attribute in second array to first array.
This is what I tried, and it works, but I don't think it is necessary to have foreach
, array_filter
, and array_column
:
foreach ($cart as $key => $cart_item) {
$item = array_filter($db_item, function($item) use ($cart_item) {
return $item['id'] === $cart_item['id'];
});
$cart[$key]['stock'] = array_column($item, 'stock')[0];
}
anyone has better idea how to optimize this?
EDIT: following Mohammad's answer, I can use more attribute in second array
$keys = ;
foreach ($arr2 as $item) {
$keys[$item['id']] = array(
'attr1' => $item['attr1'],
'attr2' => $item['attr2'],
// and so on
);
}
$newArr = array_map(function($item) use($keys){
$item['attr1'] = $keys[$item['id']]['attr1'];
$item['attr2'] = $keys[$item['id']]['attr2'];
// and so on
return $item;
}, $arr1);
EDIT2: found out that we can simplify the foreach loop with just a single line using array_column
.
$keys = array_column($arr2, null, 'id');
$newArr = array_map(function($item) use($keys){
$item['attr1'] = $keys[$item['id']]['attr1'];
$item['attr2'] = $keys[$item['id']]['attr2'];
// and so on
return $item;
}, $arr1);
php arrays multidimensional-array
I have these 2 array.
First array is from user input $cart
:
array(3) {
[0]=>
array(3) {
["id"]=>"3"
["weight"]=>"20"
["percentage"]=>"80"
}
[1]=>
array(3) {
["id"]=>"1"
["weight"]=>"50"
["percentage"]=>"80"
}
[2]=>
array(3) {
["id"]=>"2"
["weight"]=>"40"
["percentage"]=>"80"
}
}
and second array, I do a database SELECT id, stock WHERE id IN (3,1,2)
, resulting $db_item
array(3) {
[0]=>
array(2) {
["id"]=>"1"
["stock"]=>"9539.00"
}
[1]=>
array(2) {
["id"]=>"2"
["stock"]=>"9468.00"
}
[2]=>
array(2) {
["id"]=>"3"
["stock"]=>"9295.00"
}
}
I want to add the stock attribute in second array to first array.
This is what I tried, and it works, but I don't think it is necessary to have foreach
, array_filter
, and array_column
:
foreach ($cart as $key => $cart_item) {
$item = array_filter($db_item, function($item) use ($cart_item) {
return $item['id'] === $cart_item['id'];
});
$cart[$key]['stock'] = array_column($item, 'stock')[0];
}
anyone has better idea how to optimize this?
EDIT: following Mohammad's answer, I can use more attribute in second array
$keys = ;
foreach ($arr2 as $item) {
$keys[$item['id']] = array(
'attr1' => $item['attr1'],
'attr2' => $item['attr2'],
// and so on
);
}
$newArr = array_map(function($item) use($keys){
$item['attr1'] = $keys[$item['id']]['attr1'];
$item['attr2'] = $keys[$item['id']]['attr2'];
// and so on
return $item;
}, $arr1);
EDIT2: found out that we can simplify the foreach loop with just a single line using array_column
.
$keys = array_column($arr2, null, 'id');
$newArr = array_map(function($item) use($keys){
$item['attr1'] = $keys[$item['id']]['attr1'];
$item['attr2'] = $keys[$item['id']]['attr2'];
// and so on
return $item;
}, $arr1);
php arrays multidimensional-array
php arrays multidimensional-array
edited Feb 6 at 4:41
Christhofer Natalius
asked Nov 16 '18 at 13:34
Christhofer NataliusChristhofer Natalius
835822
835822
Why not get all the required data in a single query. If you show us the 2 queries, someone will tell you how to get all the info in one query
– RiggsFolly
Nov 16 '18 at 13:36
@RiggsFolly but first array is from user input, not from the query
– Christhofer Natalius
Nov 16 '18 at 13:36
Ah, good point :) Missed that :) :(
– RiggsFolly
Nov 16 '18 at 13:37
2
Funny thing, I have something of this nature in a cart system, but I just used two foreach... which is most likely the same performance as array_filter, since it rolls over the array just the same. We are talking mini-milli-seconds, so you probably are ok with what you have... unless you just want to make it more readable for anyone coming along your code in the future.
– IncredibleHat
Nov 16 '18 at 13:40
1
In programming, one of the best ways to optimize array lookups is the use of hash tables. PHP's associative arrays are an easy to use form of this. However, when dealing with only a few elements, I think any optimization is overoptimization.
– Devon
Nov 16 '18 at 13:47
|
show 2 more comments
Why not get all the required data in a single query. If you show us the 2 queries, someone will tell you how to get all the info in one query
– RiggsFolly
Nov 16 '18 at 13:36
@RiggsFolly but first array is from user input, not from the query
– Christhofer Natalius
Nov 16 '18 at 13:36
Ah, good point :) Missed that :) :(
– RiggsFolly
Nov 16 '18 at 13:37
2
Funny thing, I have something of this nature in a cart system, but I just used two foreach... which is most likely the same performance as array_filter, since it rolls over the array just the same. We are talking mini-milli-seconds, so you probably are ok with what you have... unless you just want to make it more readable for anyone coming along your code in the future.
– IncredibleHat
Nov 16 '18 at 13:40
1
In programming, one of the best ways to optimize array lookups is the use of hash tables. PHP's associative arrays are an easy to use form of this. However, when dealing with only a few elements, I think any optimization is overoptimization.
– Devon
Nov 16 '18 at 13:47
Why not get all the required data in a single query. If you show us the 2 queries, someone will tell you how to get all the info in one query
– RiggsFolly
Nov 16 '18 at 13:36
Why not get all the required data in a single query. If you show us the 2 queries, someone will tell you how to get all the info in one query
– RiggsFolly
Nov 16 '18 at 13:36
@RiggsFolly but first array is from user input, not from the query
– Christhofer Natalius
Nov 16 '18 at 13:36
@RiggsFolly but first array is from user input, not from the query
– Christhofer Natalius
Nov 16 '18 at 13:36
Ah, good point :) Missed that :) :(
– RiggsFolly
Nov 16 '18 at 13:37
Ah, good point :) Missed that :) :(
– RiggsFolly
Nov 16 '18 at 13:37
2
2
Funny thing, I have something of this nature in a cart system, but I just used two foreach... which is most likely the same performance as array_filter, since it rolls over the array just the same. We are talking mini-milli-seconds, so you probably are ok with what you have... unless you just want to make it more readable for anyone coming along your code in the future.
– IncredibleHat
Nov 16 '18 at 13:40
Funny thing, I have something of this nature in a cart system, but I just used two foreach... which is most likely the same performance as array_filter, since it rolls over the array just the same. We are talking mini-milli-seconds, so you probably are ok with what you have... unless you just want to make it more readable for anyone coming along your code in the future.
– IncredibleHat
Nov 16 '18 at 13:40
1
1
In programming, one of the best ways to optimize array lookups is the use of hash tables. PHP's associative arrays are an easy to use form of this. However, when dealing with only a few elements, I think any optimization is overoptimization.
– Devon
Nov 16 '18 at 13:47
In programming, one of the best ways to optimize array lookups is the use of hash tables. PHP's associative arrays are an easy to use form of this. However, when dealing with only a few elements, I think any optimization is overoptimization.
– Devon
Nov 16 '18 at 13:47
|
show 2 more comments
2 Answers
2
active
oldest
votes
Use combination of array_flip()
and array_column()
to create array contain id
and index of second array.
Then use array_map()
to add new key stock
to first array.
$keys = array_flip(array_column($arr2, 'id'));
$newArr = array_map(function($item) use($keys, $arr2){
$item['stock'] = $arr2[$keys[$item['id']]]['stock'];
return $item;
}, $arr1);
Check result in demo
Also you can use foreach
instead of array_flip()
$keys = ;
foreach ($arr2 as $item)
$keys[$item['id']] = $item['stock'];
$newArr = array_map(function($item) use($keys){
$item['stock'] = $keys[$item['id']];
return $item;
}, $arr1);
Check result in demo
this is the first time I see the use of array_flip. Nice idea btw, it is 2 lines shorter. But isn't doing array_flip + array_column + array_map is also doing 3 loops just like my solution above? I like your second solution, gonna change my code to use yours.
– Christhofer Natalius
Nov 16 '18 at 14:02
add a comment |
This can help too, one array_column + one array_map :
$arr2=array_column($arr2,'stock','id');
$arr1=array_map(function($val)use($arr2){$val['stock']=$arr2[$val['id']];return $val;},$arr1);
Oh nice, didn't know about array_column third parameter before.
– Christhofer Natalius
Nov 16 '18 at 14:24
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53338918%2fhow-to-combine-php-array-of-object-by-unique-id%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Use combination of array_flip()
and array_column()
to create array contain id
and index of second array.
Then use array_map()
to add new key stock
to first array.
$keys = array_flip(array_column($arr2, 'id'));
$newArr = array_map(function($item) use($keys, $arr2){
$item['stock'] = $arr2[$keys[$item['id']]]['stock'];
return $item;
}, $arr1);
Check result in demo
Also you can use foreach
instead of array_flip()
$keys = ;
foreach ($arr2 as $item)
$keys[$item['id']] = $item['stock'];
$newArr = array_map(function($item) use($keys){
$item['stock'] = $keys[$item['id']];
return $item;
}, $arr1);
Check result in demo
this is the first time I see the use of array_flip. Nice idea btw, it is 2 lines shorter. But isn't doing array_flip + array_column + array_map is also doing 3 loops just like my solution above? I like your second solution, gonna change my code to use yours.
– Christhofer Natalius
Nov 16 '18 at 14:02
add a comment |
Use combination of array_flip()
and array_column()
to create array contain id
and index of second array.
Then use array_map()
to add new key stock
to first array.
$keys = array_flip(array_column($arr2, 'id'));
$newArr = array_map(function($item) use($keys, $arr2){
$item['stock'] = $arr2[$keys[$item['id']]]['stock'];
return $item;
}, $arr1);
Check result in demo
Also you can use foreach
instead of array_flip()
$keys = ;
foreach ($arr2 as $item)
$keys[$item['id']] = $item['stock'];
$newArr = array_map(function($item) use($keys){
$item['stock'] = $keys[$item['id']];
return $item;
}, $arr1);
Check result in demo
this is the first time I see the use of array_flip. Nice idea btw, it is 2 lines shorter. But isn't doing array_flip + array_column + array_map is also doing 3 loops just like my solution above? I like your second solution, gonna change my code to use yours.
– Christhofer Natalius
Nov 16 '18 at 14:02
add a comment |
Use combination of array_flip()
and array_column()
to create array contain id
and index of second array.
Then use array_map()
to add new key stock
to first array.
$keys = array_flip(array_column($arr2, 'id'));
$newArr = array_map(function($item) use($keys, $arr2){
$item['stock'] = $arr2[$keys[$item['id']]]['stock'];
return $item;
}, $arr1);
Check result in demo
Also you can use foreach
instead of array_flip()
$keys = ;
foreach ($arr2 as $item)
$keys[$item['id']] = $item['stock'];
$newArr = array_map(function($item) use($keys){
$item['stock'] = $keys[$item['id']];
return $item;
}, $arr1);
Check result in demo
Use combination of array_flip()
and array_column()
to create array contain id
and index of second array.
Then use array_map()
to add new key stock
to first array.
$keys = array_flip(array_column($arr2, 'id'));
$newArr = array_map(function($item) use($keys, $arr2){
$item['stock'] = $arr2[$keys[$item['id']]]['stock'];
return $item;
}, $arr1);
Check result in demo
Also you can use foreach
instead of array_flip()
$keys = ;
foreach ($arr2 as $item)
$keys[$item['id']] = $item['stock'];
$newArr = array_map(function($item) use($keys){
$item['stock'] = $keys[$item['id']];
return $item;
}, $arr1);
Check result in demo
edited Nov 16 '18 at 13:56
answered Nov 16 '18 at 13:48
MohammadMohammad
16k123766
16k123766
this is the first time I see the use of array_flip. Nice idea btw, it is 2 lines shorter. But isn't doing array_flip + array_column + array_map is also doing 3 loops just like my solution above? I like your second solution, gonna change my code to use yours.
– Christhofer Natalius
Nov 16 '18 at 14:02
add a comment |
this is the first time I see the use of array_flip. Nice idea btw, it is 2 lines shorter. But isn't doing array_flip + array_column + array_map is also doing 3 loops just like my solution above? I like your second solution, gonna change my code to use yours.
– Christhofer Natalius
Nov 16 '18 at 14:02
this is the first time I see the use of array_flip. Nice idea btw, it is 2 lines shorter. But isn't doing array_flip + array_column + array_map is also doing 3 loops just like my solution above? I like your second solution, gonna change my code to use yours.
– Christhofer Natalius
Nov 16 '18 at 14:02
this is the first time I see the use of array_flip. Nice idea btw, it is 2 lines shorter. But isn't doing array_flip + array_column + array_map is also doing 3 loops just like my solution above? I like your second solution, gonna change my code to use yours.
– Christhofer Natalius
Nov 16 '18 at 14:02
add a comment |
This can help too, one array_column + one array_map :
$arr2=array_column($arr2,'stock','id');
$arr1=array_map(function($val)use($arr2){$val['stock']=$arr2[$val['id']];return $val;},$arr1);
Oh nice, didn't know about array_column third parameter before.
– Christhofer Natalius
Nov 16 '18 at 14:24
add a comment |
This can help too, one array_column + one array_map :
$arr2=array_column($arr2,'stock','id');
$arr1=array_map(function($val)use($arr2){$val['stock']=$arr2[$val['id']];return $val;},$arr1);
Oh nice, didn't know about array_column third parameter before.
– Christhofer Natalius
Nov 16 '18 at 14:24
add a comment |
This can help too, one array_column + one array_map :
$arr2=array_column($arr2,'stock','id');
$arr1=array_map(function($val)use($arr2){$val['stock']=$arr2[$val['id']];return $val;},$arr1);
This can help too, one array_column + one array_map :
$arr2=array_column($arr2,'stock','id');
$arr1=array_map(function($val)use($arr2){$val['stock']=$arr2[$val['id']];return $val;},$arr1);
answered Nov 16 '18 at 14:08


ElementaryElementary
1,3351216
1,3351216
Oh nice, didn't know about array_column third parameter before.
– Christhofer Natalius
Nov 16 '18 at 14:24
add a comment |
Oh nice, didn't know about array_column third parameter before.
– Christhofer Natalius
Nov 16 '18 at 14:24
Oh nice, didn't know about array_column third parameter before.
– Christhofer Natalius
Nov 16 '18 at 14:24
Oh nice, didn't know about array_column third parameter before.
– Christhofer Natalius
Nov 16 '18 at 14:24
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53338918%2fhow-to-combine-php-array-of-object-by-unique-id%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qGs,62H pYZlTtzb0YQ7F3atYXrbKg1,ki71tO
Why not get all the required data in a single query. If you show us the 2 queries, someone will tell you how to get all the info in one query
– RiggsFolly
Nov 16 '18 at 13:36
@RiggsFolly but first array is from user input, not from the query
– Christhofer Natalius
Nov 16 '18 at 13:36
Ah, good point :) Missed that :) :(
– RiggsFolly
Nov 16 '18 at 13:37
2
Funny thing, I have something of this nature in a cart system, but I just used two foreach... which is most likely the same performance as array_filter, since it rolls over the array just the same. We are talking mini-milli-seconds, so you probably are ok with what you have... unless you just want to make it more readable for anyone coming along your code in the future.
– IncredibleHat
Nov 16 '18 at 13:40
1
In programming, one of the best ways to optimize array lookups is the use of hash tables. PHP's associative arrays are an easy to use form of this. However, when dealing with only a few elements, I think any optimization is overoptimization.
– Devon
Nov 16 '18 at 13:47