Tiles for a BufferedImage in Java, any resolution
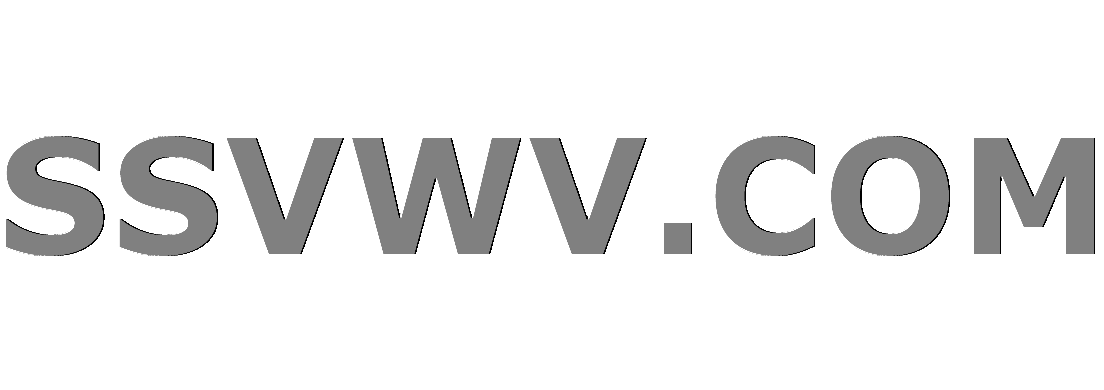
Multi tool use
I have a class to separate and join image in tiles. It works fine when the sides of the tile correspond to the dimensions of the image, i.e. height 250, tile height 25. But when it's not it doesn't create smaller tiles at the borders as it should.
Where would be the problem to properly create the border tiles smaller than the rest?
Constructor:
public EdgeBufferedImage(BufferedImage image, int w, int h){
this.sourceImg = image;
this.setCol((int)Math.ceil(image.getWidth()/(double)w));
this.setRow((int)Math.ceil(image.getHeight()/(double)h));
this.setWidth(image.getWidth());
this.setHeight(image.getHeight());
this.setTilew(w);
this.setTileh(h);
this.setMatImg(new BufferedImage[row][col]);
}
Methods:
Image tiling
public void createSmallImages() {
int rows = getRow();
int columns = getCol();
int smallWidth = getTilew();
int smallHeight = getTileh();
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) {
if (j == columns - 1) smallWidth = getWidth() - (getTilew() * j);
if (i == rows - 1) smallHeight = getHeight() - (getTileh() * i);
matImg[i][j] = getSourceImg().getSubimage(j * smallWidth, i
* smallHeight, smallWidth, smallHeight);
}
smallWidth = getTilew();
smallHeight = getTileh();
}
}
Image joining
public void joinTiles(){
int rows = getRow();
int columns = getCol();
int smallWidth = getTilew();
int smallHeight = getTileh();
BufferedImage comb = new BufferedImage(getWidth(), getHeight(), BufferedImage.TYPE_INT_RGB);
Graphics2D g = (Graphics2D) comb.getGraphics();
g.setColor(Color.RED);
for (int row = 0; row < rows; row++){
for (int col = 0; col < columns; col++){
BufferedImage piece = getMatImg()[row][col];
if (col == columns - 1) smallWidth = getWidth() - (getTilew() * col);
if (row == rows - 1) smallHeight = getHeight() - (getTileh() * row);
g.drawImage(piece, col * smallWidth, row * smallHeight, smallWidth, smallHeight, null);
g.drawRect(col * smallWidth, row * smallHeight, smallWidth, smallHeight);
}
smallWidth = getTilew();
smallHeight = getTileh();
}
g.dispose();
setSourceImg(comb);
}
Original image is 512*512
java image awt bufferedimage
add a comment |
I have a class to separate and join image in tiles. It works fine when the sides of the tile correspond to the dimensions of the image, i.e. height 250, tile height 25. But when it's not it doesn't create smaller tiles at the borders as it should.
Where would be the problem to properly create the border tiles smaller than the rest?
Constructor:
public EdgeBufferedImage(BufferedImage image, int w, int h){
this.sourceImg = image;
this.setCol((int)Math.ceil(image.getWidth()/(double)w));
this.setRow((int)Math.ceil(image.getHeight()/(double)h));
this.setWidth(image.getWidth());
this.setHeight(image.getHeight());
this.setTilew(w);
this.setTileh(h);
this.setMatImg(new BufferedImage[row][col]);
}
Methods:
Image tiling
public void createSmallImages() {
int rows = getRow();
int columns = getCol();
int smallWidth = getTilew();
int smallHeight = getTileh();
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) {
if (j == columns - 1) smallWidth = getWidth() - (getTilew() * j);
if (i == rows - 1) smallHeight = getHeight() - (getTileh() * i);
matImg[i][j] = getSourceImg().getSubimage(j * smallWidth, i
* smallHeight, smallWidth, smallHeight);
}
smallWidth = getTilew();
smallHeight = getTileh();
}
}
Image joining
public void joinTiles(){
int rows = getRow();
int columns = getCol();
int smallWidth = getTilew();
int smallHeight = getTileh();
BufferedImage comb = new BufferedImage(getWidth(), getHeight(), BufferedImage.TYPE_INT_RGB);
Graphics2D g = (Graphics2D) comb.getGraphics();
g.setColor(Color.RED);
for (int row = 0; row < rows; row++){
for (int col = 0; col < columns; col++){
BufferedImage piece = getMatImg()[row][col];
if (col == columns - 1) smallWidth = getWidth() - (getTilew() * col);
if (row == rows - 1) smallHeight = getHeight() - (getTileh() * row);
g.drawImage(piece, col * smallWidth, row * smallHeight, smallWidth, smallHeight, null);
g.drawRect(col * smallWidth, row * smallHeight, smallWidth, smallHeight);
}
smallWidth = getTilew();
smallHeight = getTileh();
}
g.dispose();
setSourceImg(comb);
}
Original image is 512*512
java image awt bufferedimage
If you add print statements or break points to your code, can you verify that the correct values are passed toBufferedImage#getSubimage(x, y, w, h)
?
– MTCoster
Nov 15 '18 at 17:55
1
Now that I look closely, when I change to the smaller width/height, I still multiply it by the row/col position. So I should first multiply the original value and add it the pad, correct?
– Santiago Rosales
Nov 15 '18 at 17:59
1) For better help sooner, post a Minimal, Complete, and Verifiable example or Short, Self Contained, Correct Example. 2) One way to get image(s) for an example is to hot link to images seen in this Q&A. E.G. This answer hot links to an image embedded in this question.
– Andrew Thompson
Nov 15 '18 at 23:37
add a comment |
I have a class to separate and join image in tiles. It works fine when the sides of the tile correspond to the dimensions of the image, i.e. height 250, tile height 25. But when it's not it doesn't create smaller tiles at the borders as it should.
Where would be the problem to properly create the border tiles smaller than the rest?
Constructor:
public EdgeBufferedImage(BufferedImage image, int w, int h){
this.sourceImg = image;
this.setCol((int)Math.ceil(image.getWidth()/(double)w));
this.setRow((int)Math.ceil(image.getHeight()/(double)h));
this.setWidth(image.getWidth());
this.setHeight(image.getHeight());
this.setTilew(w);
this.setTileh(h);
this.setMatImg(new BufferedImage[row][col]);
}
Methods:
Image tiling
public void createSmallImages() {
int rows = getRow();
int columns = getCol();
int smallWidth = getTilew();
int smallHeight = getTileh();
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) {
if (j == columns - 1) smallWidth = getWidth() - (getTilew() * j);
if (i == rows - 1) smallHeight = getHeight() - (getTileh() * i);
matImg[i][j] = getSourceImg().getSubimage(j * smallWidth, i
* smallHeight, smallWidth, smallHeight);
}
smallWidth = getTilew();
smallHeight = getTileh();
}
}
Image joining
public void joinTiles(){
int rows = getRow();
int columns = getCol();
int smallWidth = getTilew();
int smallHeight = getTileh();
BufferedImage comb = new BufferedImage(getWidth(), getHeight(), BufferedImage.TYPE_INT_RGB);
Graphics2D g = (Graphics2D) comb.getGraphics();
g.setColor(Color.RED);
for (int row = 0; row < rows; row++){
for (int col = 0; col < columns; col++){
BufferedImage piece = getMatImg()[row][col];
if (col == columns - 1) smallWidth = getWidth() - (getTilew() * col);
if (row == rows - 1) smallHeight = getHeight() - (getTileh() * row);
g.drawImage(piece, col * smallWidth, row * smallHeight, smallWidth, smallHeight, null);
g.drawRect(col * smallWidth, row * smallHeight, smallWidth, smallHeight);
}
smallWidth = getTilew();
smallHeight = getTileh();
}
g.dispose();
setSourceImg(comb);
}
Original image is 512*512
java image awt bufferedimage
I have a class to separate and join image in tiles. It works fine when the sides of the tile correspond to the dimensions of the image, i.e. height 250, tile height 25. But when it's not it doesn't create smaller tiles at the borders as it should.
Where would be the problem to properly create the border tiles smaller than the rest?
Constructor:
public EdgeBufferedImage(BufferedImage image, int w, int h){
this.sourceImg = image;
this.setCol((int)Math.ceil(image.getWidth()/(double)w));
this.setRow((int)Math.ceil(image.getHeight()/(double)h));
this.setWidth(image.getWidth());
this.setHeight(image.getHeight());
this.setTilew(w);
this.setTileh(h);
this.setMatImg(new BufferedImage[row][col]);
}
Methods:
Image tiling
public void createSmallImages() {
int rows = getRow();
int columns = getCol();
int smallWidth = getTilew();
int smallHeight = getTileh();
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) {
if (j == columns - 1) smallWidth = getWidth() - (getTilew() * j);
if (i == rows - 1) smallHeight = getHeight() - (getTileh() * i);
matImg[i][j] = getSourceImg().getSubimage(j * smallWidth, i
* smallHeight, smallWidth, smallHeight);
}
smallWidth = getTilew();
smallHeight = getTileh();
}
}
Image joining
public void joinTiles(){
int rows = getRow();
int columns = getCol();
int smallWidth = getTilew();
int smallHeight = getTileh();
BufferedImage comb = new BufferedImage(getWidth(), getHeight(), BufferedImage.TYPE_INT_RGB);
Graphics2D g = (Graphics2D) comb.getGraphics();
g.setColor(Color.RED);
for (int row = 0; row < rows; row++){
for (int col = 0; col < columns; col++){
BufferedImage piece = getMatImg()[row][col];
if (col == columns - 1) smallWidth = getWidth() - (getTilew() * col);
if (row == rows - 1) smallHeight = getHeight() - (getTileh() * row);
g.drawImage(piece, col * smallWidth, row * smallHeight, smallWidth, smallHeight, null);
g.drawRect(col * smallWidth, row * smallHeight, smallWidth, smallHeight);
}
smallWidth = getTilew();
smallHeight = getTileh();
}
g.dispose();
setSourceImg(comb);
}
Original image is 512*512
java image awt bufferedimage
java image awt bufferedimage
edited Nov 15 '18 at 23:37


Andrew Thompson
154k28163345
154k28163345
asked Nov 15 '18 at 17:43


Santiago RosalesSantiago Rosales
296
296
If you add print statements or break points to your code, can you verify that the correct values are passed toBufferedImage#getSubimage(x, y, w, h)
?
– MTCoster
Nov 15 '18 at 17:55
1
Now that I look closely, when I change to the smaller width/height, I still multiply it by the row/col position. So I should first multiply the original value and add it the pad, correct?
– Santiago Rosales
Nov 15 '18 at 17:59
1) For better help sooner, post a Minimal, Complete, and Verifiable example or Short, Self Contained, Correct Example. 2) One way to get image(s) for an example is to hot link to images seen in this Q&A. E.G. This answer hot links to an image embedded in this question.
– Andrew Thompson
Nov 15 '18 at 23:37
add a comment |
If you add print statements or break points to your code, can you verify that the correct values are passed toBufferedImage#getSubimage(x, y, w, h)
?
– MTCoster
Nov 15 '18 at 17:55
1
Now that I look closely, when I change to the smaller width/height, I still multiply it by the row/col position. So I should first multiply the original value and add it the pad, correct?
– Santiago Rosales
Nov 15 '18 at 17:59
1) For better help sooner, post a Minimal, Complete, and Verifiable example or Short, Self Contained, Correct Example. 2) One way to get image(s) for an example is to hot link to images seen in this Q&A. E.G. This answer hot links to an image embedded in this question.
– Andrew Thompson
Nov 15 '18 at 23:37
If you add print statements or break points to your code, can you verify that the correct values are passed to
BufferedImage#getSubimage(x, y, w, h)
?– MTCoster
Nov 15 '18 at 17:55
If you add print statements or break points to your code, can you verify that the correct values are passed to
BufferedImage#getSubimage(x, y, w, h)
?– MTCoster
Nov 15 '18 at 17:55
1
1
Now that I look closely, when I change to the smaller width/height, I still multiply it by the row/col position. So I should first multiply the original value and add it the pad, correct?
– Santiago Rosales
Nov 15 '18 at 17:59
Now that I look closely, when I change to the smaller width/height, I still multiply it by the row/col position. So I should first multiply the original value and add it the pad, correct?
– Santiago Rosales
Nov 15 '18 at 17:59
1) For better help sooner, post a Minimal, Complete, and Verifiable example or Short, Self Contained, Correct Example. 2) One way to get image(s) for an example is to hot link to images seen in this Q&A. E.G. This answer hot links to an image embedded in this question.
– Andrew Thompson
Nov 15 '18 at 23:37
1) For better help sooner, post a Minimal, Complete, and Verifiable example or Short, Self Contained, Correct Example. 2) One way to get image(s) for an example is to hot link to images seen in this Q&A. E.G. This answer hot links to an image embedded in this question.
– Andrew Thompson
Nov 15 '18 at 23:37
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325165%2ftiles-for-a-bufferedimage-in-java-any-resolution%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325165%2ftiles-for-a-bufferedimage-in-java-any-resolution%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
h S,DC3iDh6g,JE
If you add print statements or break points to your code, can you verify that the correct values are passed to
BufferedImage#getSubimage(x, y, w, h)
?– MTCoster
Nov 15 '18 at 17:55
1
Now that I look closely, when I change to the smaller width/height, I still multiply it by the row/col position. So I should first multiply the original value and add it the pad, correct?
– Santiago Rosales
Nov 15 '18 at 17:59
1) For better help sooner, post a Minimal, Complete, and Verifiable example or Short, Self Contained, Correct Example. 2) One way to get image(s) for an example is to hot link to images seen in this Q&A. E.G. This answer hot links to an image embedded in this question.
– Andrew Thompson
Nov 15 '18 at 23:37