Possible to build a string using shorthand conditionals like this?
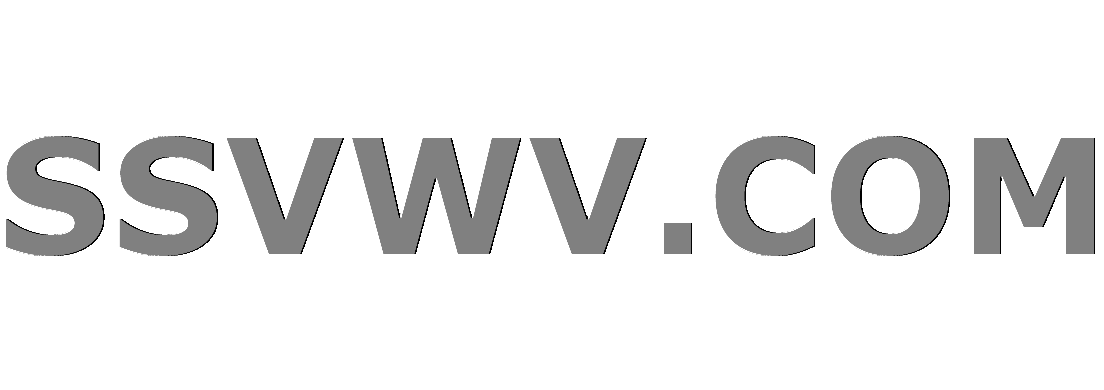
Multi tool use
I am trying to add some parameters to my URL based on dropdown selections, I want to keep the code as short and sweet as possible so I'm trying to build a string for the parameters that leaves out any variables that are blank so they do not get appended to the URL string. Below is what I've tried:
$(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = (product === '') ? '' : 'product=' + product + '&' + (size === '') ? '' : 'size=' + size + '&' + (color === '') ? '' : 'color=' + color + '&' + (custom === '') ? '' : 'custom=' + custom;
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
The expected output of urlParams
is:
product=shirt&size=large&color=blue
Unfortunately this returns an empty string. Is it possible to build the parameters like this? Or is there some better way to accomplish this?
javascript conditional-operator
add a comment |
I am trying to add some parameters to my URL based on dropdown selections, I want to keep the code as short and sweet as possible so I'm trying to build a string for the parameters that leaves out any variables that are blank so they do not get appended to the URL string. Below is what I've tried:
$(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = (product === '') ? '' : 'product=' + product + '&' + (size === '') ? '' : 'size=' + size + '&' + (color === '') ? '' : 'color=' + color + '&' + (custom === '') ? '' : 'custom=' + custom;
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
The expected output of urlParams
is:
product=shirt&size=large&color=blue
Unfortunately this returns an empty string. Is it possible to build the parameters like this? Or is there some better way to accomplish this?
javascript conditional-operator
add a comment |
I am trying to add some parameters to my URL based on dropdown selections, I want to keep the code as short and sweet as possible so I'm trying to build a string for the parameters that leaves out any variables that are blank so they do not get appended to the URL string. Below is what I've tried:
$(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = (product === '') ? '' : 'product=' + product + '&' + (size === '') ? '' : 'size=' + size + '&' + (color === '') ? '' : 'color=' + color + '&' + (custom === '') ? '' : 'custom=' + custom;
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
The expected output of urlParams
is:
product=shirt&size=large&color=blue
Unfortunately this returns an empty string. Is it possible to build the parameters like this? Or is there some better way to accomplish this?
javascript conditional-operator
I am trying to add some parameters to my URL based on dropdown selections, I want to keep the code as short and sweet as possible so I'm trying to build a string for the parameters that leaves out any variables that are blank so they do not get appended to the URL string. Below is what I've tried:
$(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = (product === '') ? '' : 'product=' + product + '&' + (size === '') ? '' : 'size=' + size + '&' + (color === '') ? '' : 'color=' + color + '&' + (custom === '') ? '' : 'custom=' + custom;
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
The expected output of urlParams
is:
product=shirt&size=large&color=blue
Unfortunately this returns an empty string. Is it possible to build the parameters like this? Or is there some better way to accomplish this?
$(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = (product === '') ? '' : 'product=' + product + '&' + (size === '') ? '' : 'size=' + size + '&' + (color === '') ? '' : 'color=' + color + '&' + (custom === '') ? '' : 'custom=' + custom;
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
$(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = (product === '') ? '' : 'product=' + product + '&' + (size === '') ? '' : 'size=' + size + '&' + (color === '') ? '' : 'color=' + color + '&' + (custom === '') ? '' : 'custom=' + custom;
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
javascript conditional-operator
javascript conditional-operator
asked Nov 15 '18 at 17:48
user13286user13286
77751847
77751847
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
Parantheses matter!
The problem is, you aren't looking into the older ones. The custom === ""
gets truthy and then your whole condition is collapsed. Better way to do is:
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = ((product === '') ? '' : 'product=' + product) + '&' + ((size === '') ? '' : 'size=' + size) + '&' + ((color === '') ? '' : 'color=' + color) + '&' + ((custom === '') ? '' : 'custom=' + custom);
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
Now you could see that there are &
s. A better version will be:
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = ((product === '') ? '' : 'product=' + product) + '&' + ((size === '') ? '' : 'size=' + size) + '&' + ((color === '') ? '' : 'color=' + color) + '&' + ((custom === '') ? '' : 'custom=' + custom);
urlParams = urlParams.replace(/^&+|&+$/g, '');
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
The best would be using arrays and .join()
s.
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = [
((product === '') ? '' : 'product=' + product),
((size === '') ? '' : 'size=' + size),
((color === '') ? '' : 'color=' + color),
((custom === '') ? '' : 'custom=' + custom)
];
urlParams = urlParams.join("&").replace(/^&+|&+$/g, '');
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
Thank you very much for explaining different solutions. I agree that using.join()
s is probably my best bet.
– user13286
Nov 15 '18 at 18:03
@user13286 You are welcome. I am glad that I am able to help you understand something new.:)
– Praveen Kumar Purushothaman
Nov 15 '18 at 18:04
add a comment |
You could check the value and take a logical AND for the formatted string.
var urlParams = (product && 'product=' + product + '&') +
(size && 'size=' + size + '&') +
(color && 'color=' + color + '&') +
(custom && 'custom=' + custom);
Anmother approach would be to use an object, filter truthy values and build formatted string with a template string.
function getString(object) {
return Object
.entries(object)
.filter(([, v]) => v)
.map(([k, v]) => `${k}=${v}`)
.join('&');
}
var product = 'foo',
size = '42',
color = '',
data = { product, size, color };
console.log(getString(data))
Thank you for these solutions, the filter approach is very interesting.
– user13286
Nov 15 '18 at 18:05
This is nice. Too complex for new people to understand.:(
– Praveen Kumar Purushothaman
Nov 15 '18 at 18:05
add a comment |
const params = {
product: 'shirt',
size: 'large',
color: '',
custom: null
}
const valid = p => k => typeof p [k] === 'string' && p [k].length > 0
let queryString = Object.keys (params).filter (valid (params)).map (k => `${k}=${params[k]}`).join ('&')
console.log (queryString)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325222%2fpossible-to-build-a-string-using-shorthand-conditionals-like-this%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Parantheses matter!
The problem is, you aren't looking into the older ones. The custom === ""
gets truthy and then your whole condition is collapsed. Better way to do is:
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = ((product === '') ? '' : 'product=' + product) + '&' + ((size === '') ? '' : 'size=' + size) + '&' + ((color === '') ? '' : 'color=' + color) + '&' + ((custom === '') ? '' : 'custom=' + custom);
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
Now you could see that there are &
s. A better version will be:
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = ((product === '') ? '' : 'product=' + product) + '&' + ((size === '') ? '' : 'size=' + size) + '&' + ((color === '') ? '' : 'color=' + color) + '&' + ((custom === '') ? '' : 'custom=' + custom);
urlParams = urlParams.replace(/^&+|&+$/g, '');
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
The best would be using arrays and .join()
s.
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = [
((product === '') ? '' : 'product=' + product),
((size === '') ? '' : 'size=' + size),
((color === '') ? '' : 'color=' + color),
((custom === '') ? '' : 'custom=' + custom)
];
urlParams = urlParams.join("&").replace(/^&+|&+$/g, '');
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
Thank you very much for explaining different solutions. I agree that using.join()
s is probably my best bet.
– user13286
Nov 15 '18 at 18:03
@user13286 You are welcome. I am glad that I am able to help you understand something new.:)
– Praveen Kumar Purushothaman
Nov 15 '18 at 18:04
add a comment |
Parantheses matter!
The problem is, you aren't looking into the older ones. The custom === ""
gets truthy and then your whole condition is collapsed. Better way to do is:
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = ((product === '') ? '' : 'product=' + product) + '&' + ((size === '') ? '' : 'size=' + size) + '&' + ((color === '') ? '' : 'color=' + color) + '&' + ((custom === '') ? '' : 'custom=' + custom);
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
Now you could see that there are &
s. A better version will be:
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = ((product === '') ? '' : 'product=' + product) + '&' + ((size === '') ? '' : 'size=' + size) + '&' + ((color === '') ? '' : 'color=' + color) + '&' + ((custom === '') ? '' : 'custom=' + custom);
urlParams = urlParams.replace(/^&+|&+$/g, '');
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
The best would be using arrays and .join()
s.
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = [
((product === '') ? '' : 'product=' + product),
((size === '') ? '' : 'size=' + size),
((color === '') ? '' : 'color=' + color),
((custom === '') ? '' : 'custom=' + custom)
];
urlParams = urlParams.join("&").replace(/^&+|&+$/g, '');
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
Thank you very much for explaining different solutions. I agree that using.join()
s is probably my best bet.
– user13286
Nov 15 '18 at 18:03
@user13286 You are welcome. I am glad that I am able to help you understand something new.:)
– Praveen Kumar Purushothaman
Nov 15 '18 at 18:04
add a comment |
Parantheses matter!
The problem is, you aren't looking into the older ones. The custom === ""
gets truthy and then your whole condition is collapsed. Better way to do is:
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = ((product === '') ? '' : 'product=' + product) + '&' + ((size === '') ? '' : 'size=' + size) + '&' + ((color === '') ? '' : 'color=' + color) + '&' + ((custom === '') ? '' : 'custom=' + custom);
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
Now you could see that there are &
s. A better version will be:
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = ((product === '') ? '' : 'product=' + product) + '&' + ((size === '') ? '' : 'size=' + size) + '&' + ((color === '') ? '' : 'color=' + color) + '&' + ((custom === '') ? '' : 'custom=' + custom);
urlParams = urlParams.replace(/^&+|&+$/g, '');
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
The best would be using arrays and .join()
s.
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = [
((product === '') ? '' : 'product=' + product),
((size === '') ? '' : 'size=' + size),
((color === '') ? '' : 'color=' + color),
((custom === '') ? '' : 'custom=' + custom)
];
urlParams = urlParams.join("&").replace(/^&+|&+$/g, '');
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
Parantheses matter!
The problem is, you aren't looking into the older ones. The custom === ""
gets truthy and then your whole condition is collapsed. Better way to do is:
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = ((product === '') ? '' : 'product=' + product) + '&' + ((size === '') ? '' : 'size=' + size) + '&' + ((color === '') ? '' : 'color=' + color) + '&' + ((custom === '') ? '' : 'custom=' + custom);
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
Now you could see that there are &
s. A better version will be:
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = ((product === '') ? '' : 'product=' + product) + '&' + ((size === '') ? '' : 'size=' + size) + '&' + ((color === '') ? '' : 'color=' + color) + '&' + ((custom === '') ? '' : 'custom=' + custom);
urlParams = urlParams.replace(/^&+|&+$/g, '');
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
The best would be using arrays and .join()
s.
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = [
((product === '') ? '' : 'product=' + product),
((size === '') ? '' : 'size=' + size),
((color === '') ? '' : 'color=' + color),
((custom === '') ? '' : 'custom=' + custom)
];
urlParams = urlParams.join("&").replace(/^&+|&+$/g, '');
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = ((product === '') ? '' : 'product=' + product) + '&' + ((size === '') ? '' : 'size=' + size) + '&' + ((color === '') ? '' : 'color=' + color) + '&' + ((custom === '') ? '' : 'custom=' + custom);
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = ((product === '') ? '' : 'product=' + product) + '&' + ((size === '') ? '' : 'size=' + size) + '&' + ((color === '') ? '' : 'color=' + color) + '&' + ((custom === '') ? '' : 'custom=' + custom);
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = ((product === '') ? '' : 'product=' + product) + '&' + ((size === '') ? '' : 'size=' + size) + '&' + ((color === '') ? '' : 'color=' + color) + '&' + ((custom === '') ? '' : 'custom=' + custom);
urlParams = urlParams.replace(/^&+|&+$/g, '');
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = ((product === '') ? '' : 'product=' + product) + '&' + ((size === '') ? '' : 'size=' + size) + '&' + ((color === '') ? '' : 'color=' + color) + '&' + ((custom === '') ? '' : 'custom=' + custom);
urlParams = urlParams.replace(/^&+|&+$/g, '');
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = [
((product === '') ? '' : 'product=' + product),
((size === '') ? '' : 'size=' + size),
((color === '') ? '' : 'color=' + color),
((custom === '') ? '' : 'custom=' + custom)
];
urlParams = urlParams.join("&").replace(/^&+|&+$/g, '');
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
(function() {
var product = 'shirt',
size = 'large',
color = 'blue',
custom = '';
var urlParams = [
((product === '') ? '' : 'product=' + product),
((size === '') ? '' : 'size=' + size),
((color === '') ? '' : 'color=' + color),
((custom === '') ? '' : 'custom=' + custom)
];
urlParams = urlParams.join("&").replace(/^&+|&+$/g, '');
console.log(urlParams);
// Go to results page
// location.href = 'results?' + urlParams;
})();
answered Nov 15 '18 at 17:51
Praveen Kumar PurushothamanPraveen Kumar Purushothaman
134k23139188
134k23139188
Thank you very much for explaining different solutions. I agree that using.join()
s is probably my best bet.
– user13286
Nov 15 '18 at 18:03
@user13286 You are welcome. I am glad that I am able to help you understand something new.:)
– Praveen Kumar Purushothaman
Nov 15 '18 at 18:04
add a comment |
Thank you very much for explaining different solutions. I agree that using.join()
s is probably my best bet.
– user13286
Nov 15 '18 at 18:03
@user13286 You are welcome. I am glad that I am able to help you understand something new.:)
– Praveen Kumar Purushothaman
Nov 15 '18 at 18:04
Thank you very much for explaining different solutions. I agree that using
.join()
s is probably my best bet.– user13286
Nov 15 '18 at 18:03
Thank you very much for explaining different solutions. I agree that using
.join()
s is probably my best bet.– user13286
Nov 15 '18 at 18:03
@user13286 You are welcome. I am glad that I am able to help you understand something new.
:)
– Praveen Kumar Purushothaman
Nov 15 '18 at 18:04
@user13286 You are welcome. I am glad that I am able to help you understand something new.
:)
– Praveen Kumar Purushothaman
Nov 15 '18 at 18:04
add a comment |
You could check the value and take a logical AND for the formatted string.
var urlParams = (product && 'product=' + product + '&') +
(size && 'size=' + size + '&') +
(color && 'color=' + color + '&') +
(custom && 'custom=' + custom);
Anmother approach would be to use an object, filter truthy values and build formatted string with a template string.
function getString(object) {
return Object
.entries(object)
.filter(([, v]) => v)
.map(([k, v]) => `${k}=${v}`)
.join('&');
}
var product = 'foo',
size = '42',
color = '',
data = { product, size, color };
console.log(getString(data))
Thank you for these solutions, the filter approach is very interesting.
– user13286
Nov 15 '18 at 18:05
This is nice. Too complex for new people to understand.:(
– Praveen Kumar Purushothaman
Nov 15 '18 at 18:05
add a comment |
You could check the value and take a logical AND for the formatted string.
var urlParams = (product && 'product=' + product + '&') +
(size && 'size=' + size + '&') +
(color && 'color=' + color + '&') +
(custom && 'custom=' + custom);
Anmother approach would be to use an object, filter truthy values and build formatted string with a template string.
function getString(object) {
return Object
.entries(object)
.filter(([, v]) => v)
.map(([k, v]) => `${k}=${v}`)
.join('&');
}
var product = 'foo',
size = '42',
color = '',
data = { product, size, color };
console.log(getString(data))
Thank you for these solutions, the filter approach is very interesting.
– user13286
Nov 15 '18 at 18:05
This is nice. Too complex for new people to understand.:(
– Praveen Kumar Purushothaman
Nov 15 '18 at 18:05
add a comment |
You could check the value and take a logical AND for the formatted string.
var urlParams = (product && 'product=' + product + '&') +
(size && 'size=' + size + '&') +
(color && 'color=' + color + '&') +
(custom && 'custom=' + custom);
Anmother approach would be to use an object, filter truthy values and build formatted string with a template string.
function getString(object) {
return Object
.entries(object)
.filter(([, v]) => v)
.map(([k, v]) => `${k}=${v}`)
.join('&');
}
var product = 'foo',
size = '42',
color = '',
data = { product, size, color };
console.log(getString(data))
You could check the value and take a logical AND for the formatted string.
var urlParams = (product && 'product=' + product + '&') +
(size && 'size=' + size + '&') +
(color && 'color=' + color + '&') +
(custom && 'custom=' + custom);
Anmother approach would be to use an object, filter truthy values and build formatted string with a template string.
function getString(object) {
return Object
.entries(object)
.filter(([, v]) => v)
.map(([k, v]) => `${k}=${v}`)
.join('&');
}
var product = 'foo',
size = '42',
color = '',
data = { product, size, color };
console.log(getString(data))
function getString(object) {
return Object
.entries(object)
.filter(([, v]) => v)
.map(([k, v]) => `${k}=${v}`)
.join('&');
}
var product = 'foo',
size = '42',
color = '',
data = { product, size, color };
console.log(getString(data))
function getString(object) {
return Object
.entries(object)
.filter(([, v]) => v)
.map(([k, v]) => `${k}=${v}`)
.join('&');
}
var product = 'foo',
size = '42',
color = '',
data = { product, size, color };
console.log(getString(data))
edited Nov 15 '18 at 18:03
answered Nov 15 '18 at 17:54


Nina ScholzNina Scholz
191k15102175
191k15102175
Thank you for these solutions, the filter approach is very interesting.
– user13286
Nov 15 '18 at 18:05
This is nice. Too complex for new people to understand.:(
– Praveen Kumar Purushothaman
Nov 15 '18 at 18:05
add a comment |
Thank you for these solutions, the filter approach is very interesting.
– user13286
Nov 15 '18 at 18:05
This is nice. Too complex for new people to understand.:(
– Praveen Kumar Purushothaman
Nov 15 '18 at 18:05
Thank you for these solutions, the filter approach is very interesting.
– user13286
Nov 15 '18 at 18:05
Thank you for these solutions, the filter approach is very interesting.
– user13286
Nov 15 '18 at 18:05
This is nice. Too complex for new people to understand.
:(
– Praveen Kumar Purushothaman
Nov 15 '18 at 18:05
This is nice. Too complex for new people to understand.
:(
– Praveen Kumar Purushothaman
Nov 15 '18 at 18:05
add a comment |
const params = {
product: 'shirt',
size: 'large',
color: '',
custom: null
}
const valid = p => k => typeof p [k] === 'string' && p [k].length > 0
let queryString = Object.keys (params).filter (valid (params)).map (k => `${k}=${params[k]}`).join ('&')
console.log (queryString)
add a comment |
const params = {
product: 'shirt',
size: 'large',
color: '',
custom: null
}
const valid = p => k => typeof p [k] === 'string' && p [k].length > 0
let queryString = Object.keys (params).filter (valid (params)).map (k => `${k}=${params[k]}`).join ('&')
console.log (queryString)
add a comment |
const params = {
product: 'shirt',
size: 'large',
color: '',
custom: null
}
const valid = p => k => typeof p [k] === 'string' && p [k].length > 0
let queryString = Object.keys (params).filter (valid (params)).map (k => `${k}=${params[k]}`).join ('&')
console.log (queryString)
const params = {
product: 'shirt',
size: 'large',
color: '',
custom: null
}
const valid = p => k => typeof p [k] === 'string' && p [k].length > 0
let queryString = Object.keys (params).filter (valid (params)).map (k => `${k}=${params[k]}`).join ('&')
console.log (queryString)
const params = {
product: 'shirt',
size: 'large',
color: '',
custom: null
}
const valid = p => k => typeof p [k] === 'string' && p [k].length > 0
let queryString = Object.keys (params).filter (valid (params)).map (k => `${k}=${params[k]}`).join ('&')
console.log (queryString)
const params = {
product: 'shirt',
size: 'large',
color: '',
custom: null
}
const valid = p => k => typeof p [k] === 'string' && p [k].length > 0
let queryString = Object.keys (params).filter (valid (params)).map (k => `${k}=${params[k]}`).join ('&')
console.log (queryString)
answered Nov 15 '18 at 18:22
leninelleninel
17914
17914
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325222%2fpossible-to-build-a-string-using-shorthand-conditionals-like-this%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
24XUKbJeJQVFwl2e4,UUyAAe0oNxnohPh6cGG1fmh9jBs71lBAqWp hm2Qc