how to get return value from pytest fixture in a function so I don't need to call this function with...
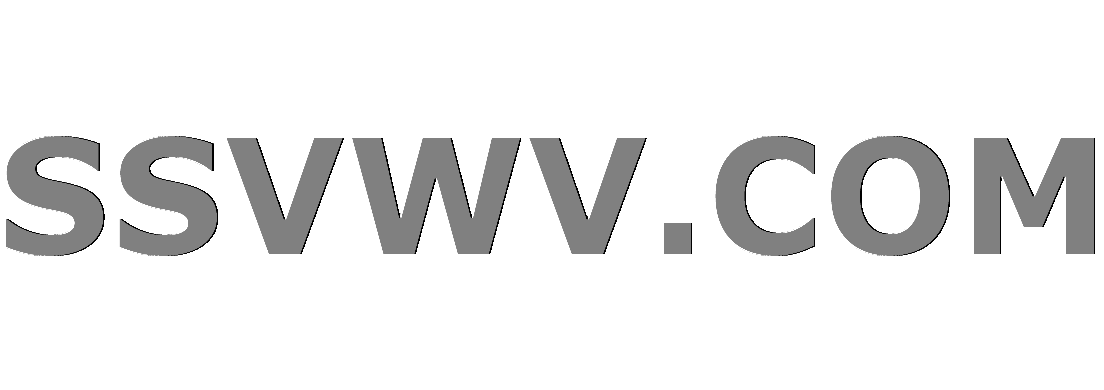
Multi tool use
I have the following fixture in conftest.py that returns an environment settings dictionary like user, password etc:
@pytest.fixture
def envparams(request):
env = request.config.getoption("--env")
return env_params[env]
Then I have module like:
def request_master_url(envparams):
cje_master_url = envparams['url']+'/'+test_master
cje_user = envparams['user']
cje_pass = envparams['password']
local = testinfra.get_host("ssh://localhost")
results = local.command(
'curl -L -I --user '+cje_user+':'
+ cje_pass+' '+cje_master_url+'| grep HTTP
|tail -1').stdout
if '200 OK' in results:
return True
else:
return False
and a test that uses this module like:
def test_cje_high_availability(envparams, env_option, script_loc):
workstation = testinfra.get_host('ssh://'+testinfra_hosts[0])
if not security.request_master_url(envparams):
print(test_master+' - is not availablen')
create_team_master(test_master, envparams, script_loc)
Can I get rid of the envparams parameter from module function somehow so I can call it without additional parameter?
like:
security.request_master_url(envparams)
I only need to setup this fixture once in a session. I tried to use:
@pytest.mark.usefixtures('envparams')
def request_master_url():
But, I am not sure how to get values returned from this fixture.
python pytest fixtures
add a comment |
I have the following fixture in conftest.py that returns an environment settings dictionary like user, password etc:
@pytest.fixture
def envparams(request):
env = request.config.getoption("--env")
return env_params[env]
Then I have module like:
def request_master_url(envparams):
cje_master_url = envparams['url']+'/'+test_master
cje_user = envparams['user']
cje_pass = envparams['password']
local = testinfra.get_host("ssh://localhost")
results = local.command(
'curl -L -I --user '+cje_user+':'
+ cje_pass+' '+cje_master_url+'| grep HTTP
|tail -1').stdout
if '200 OK' in results:
return True
else:
return False
and a test that uses this module like:
def test_cje_high_availability(envparams, env_option, script_loc):
workstation = testinfra.get_host('ssh://'+testinfra_hosts[0])
if not security.request_master_url(envparams):
print(test_master+' - is not availablen')
create_team_master(test_master, envparams, script_loc)
Can I get rid of the envparams parameter from module function somehow so I can call it without additional parameter?
like:
security.request_master_url(envparams)
I only need to setup this fixture once in a session. I tried to use:
@pytest.mark.usefixtures('envparams')
def request_master_url():
But, I am not sure how to get values returned from this fixture.
python pytest fixtures
Why not making a fixture out ofrequest_master_url
?
– hoefling
Nov 16 '18 at 9:33
it's some solution, but I have many more functions like this one that are also using envparams fixture. I think it would be perhaps, better to create a global parameter in conftest.py and set it to envparams fixture for a session. I'll just read that from my module functions and no additional parameter would be needed to call from test functions.
– Krzysztof
Nov 19 '18 at 9:29
Thing is, you can't applypytest
machinery to normal functions per se. Ifrequest_master_url
should be a function and is used in tests only, you can get the env viapytest.config.getoption('--env')
in the function directly, so you don't need to pass theenvparams
argument. Is this suitable to your needs?
– hoefling
Nov 19 '18 at 9:46
yes, this was very helpfull actually. Implemented today.
– Krzysztof
Dec 6 '18 at 15:59
add a comment |
I have the following fixture in conftest.py that returns an environment settings dictionary like user, password etc:
@pytest.fixture
def envparams(request):
env = request.config.getoption("--env")
return env_params[env]
Then I have module like:
def request_master_url(envparams):
cje_master_url = envparams['url']+'/'+test_master
cje_user = envparams['user']
cje_pass = envparams['password']
local = testinfra.get_host("ssh://localhost")
results = local.command(
'curl -L -I --user '+cje_user+':'
+ cje_pass+' '+cje_master_url+'| grep HTTP
|tail -1').stdout
if '200 OK' in results:
return True
else:
return False
and a test that uses this module like:
def test_cje_high_availability(envparams, env_option, script_loc):
workstation = testinfra.get_host('ssh://'+testinfra_hosts[0])
if not security.request_master_url(envparams):
print(test_master+' - is not availablen')
create_team_master(test_master, envparams, script_loc)
Can I get rid of the envparams parameter from module function somehow so I can call it without additional parameter?
like:
security.request_master_url(envparams)
I only need to setup this fixture once in a session. I tried to use:
@pytest.mark.usefixtures('envparams')
def request_master_url():
But, I am not sure how to get values returned from this fixture.
python pytest fixtures
I have the following fixture in conftest.py that returns an environment settings dictionary like user, password etc:
@pytest.fixture
def envparams(request):
env = request.config.getoption("--env")
return env_params[env]
Then I have module like:
def request_master_url(envparams):
cje_master_url = envparams['url']+'/'+test_master
cje_user = envparams['user']
cje_pass = envparams['password']
local = testinfra.get_host("ssh://localhost")
results = local.command(
'curl -L -I --user '+cje_user+':'
+ cje_pass+' '+cje_master_url+'| grep HTTP
|tail -1').stdout
if '200 OK' in results:
return True
else:
return False
and a test that uses this module like:
def test_cje_high_availability(envparams, env_option, script_loc):
workstation = testinfra.get_host('ssh://'+testinfra_hosts[0])
if not security.request_master_url(envparams):
print(test_master+' - is not availablen')
create_team_master(test_master, envparams, script_loc)
Can I get rid of the envparams parameter from module function somehow so I can call it without additional parameter?
like:
security.request_master_url(envparams)
I only need to setup this fixture once in a session. I tried to use:
@pytest.mark.usefixtures('envparams')
def request_master_url():
But, I am not sure how to get values returned from this fixture.
python pytest fixtures
python pytest fixtures
asked Nov 15 '18 at 17:54
KrzysztofKrzysztof
735
735
Why not making a fixture out ofrequest_master_url
?
– hoefling
Nov 16 '18 at 9:33
it's some solution, but I have many more functions like this one that are also using envparams fixture. I think it would be perhaps, better to create a global parameter in conftest.py and set it to envparams fixture for a session. I'll just read that from my module functions and no additional parameter would be needed to call from test functions.
– Krzysztof
Nov 19 '18 at 9:29
Thing is, you can't applypytest
machinery to normal functions per se. Ifrequest_master_url
should be a function and is used in tests only, you can get the env viapytest.config.getoption('--env')
in the function directly, so you don't need to pass theenvparams
argument. Is this suitable to your needs?
– hoefling
Nov 19 '18 at 9:46
yes, this was very helpfull actually. Implemented today.
– Krzysztof
Dec 6 '18 at 15:59
add a comment |
Why not making a fixture out ofrequest_master_url
?
– hoefling
Nov 16 '18 at 9:33
it's some solution, but I have many more functions like this one that are also using envparams fixture. I think it would be perhaps, better to create a global parameter in conftest.py and set it to envparams fixture for a session. I'll just read that from my module functions and no additional parameter would be needed to call from test functions.
– Krzysztof
Nov 19 '18 at 9:29
Thing is, you can't applypytest
machinery to normal functions per se. Ifrequest_master_url
should be a function and is used in tests only, you can get the env viapytest.config.getoption('--env')
in the function directly, so you don't need to pass theenvparams
argument. Is this suitable to your needs?
– hoefling
Nov 19 '18 at 9:46
yes, this was very helpfull actually. Implemented today.
– Krzysztof
Dec 6 '18 at 15:59
Why not making a fixture out of
request_master_url
?– hoefling
Nov 16 '18 at 9:33
Why not making a fixture out of
request_master_url
?– hoefling
Nov 16 '18 at 9:33
it's some solution, but I have many more functions like this one that are also using envparams fixture. I think it would be perhaps, better to create a global parameter in conftest.py and set it to envparams fixture for a session. I'll just read that from my module functions and no additional parameter would be needed to call from test functions.
– Krzysztof
Nov 19 '18 at 9:29
it's some solution, but I have many more functions like this one that are also using envparams fixture. I think it would be perhaps, better to create a global parameter in conftest.py and set it to envparams fixture for a session. I'll just read that from my module functions and no additional parameter would be needed to call from test functions.
– Krzysztof
Nov 19 '18 at 9:29
Thing is, you can't apply
pytest
machinery to normal functions per se. If request_master_url
should be a function and is used in tests only, you can get the env via pytest.config.getoption('--env')
in the function directly, so you don't need to pass the envparams
argument. Is this suitable to your needs?– hoefling
Nov 19 '18 at 9:46
Thing is, you can't apply
pytest
machinery to normal functions per se. If request_master_url
should be a function and is used in tests only, you can get the env via pytest.config.getoption('--env')
in the function directly, so you don't need to pass the envparams
argument. Is this suitable to your needs?– hoefling
Nov 19 '18 at 9:46
yes, this was very helpfull actually. Implemented today.
– Krzysztof
Dec 6 '18 at 15:59
yes, this was very helpfull actually. Implemented today.
– Krzysztof
Dec 6 '18 at 15:59
add a comment |
1 Answer
1
active
oldest
votes
Well, I've done as hoefling suggested.
Created small function in conftest.py:
def get_env_params():
env_name = pytest.config.getoption("--env")
return env_params[env_name]
and call it from my module functions where needed. Example function looks like below:
def request_master_url(team_id):
envparams = get_env_params()
cje_master_url = envparams['url']+'/'+team_id
cje_user = envparams['user']
cje_pass = envparams['password']
local = testinfra.get_host("ssh://localhost")
results = local.command(
'curl -L -I --user '+cje_user+':'
+ cje_pass+' '+cje_master_url+'| grep HTTP
|tail -1').stdout
if '200 OK' in results:
return True
else:
return False
removed unnecessary fixture from many more functions and was able to clean my code a lot. Thanks!
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325313%2fhow-to-get-return-value-from-pytest-fixture-in-a-function-so-i-dont-need-to-cal%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Well, I've done as hoefling suggested.
Created small function in conftest.py:
def get_env_params():
env_name = pytest.config.getoption("--env")
return env_params[env_name]
and call it from my module functions where needed. Example function looks like below:
def request_master_url(team_id):
envparams = get_env_params()
cje_master_url = envparams['url']+'/'+team_id
cje_user = envparams['user']
cje_pass = envparams['password']
local = testinfra.get_host("ssh://localhost")
results = local.command(
'curl -L -I --user '+cje_user+':'
+ cje_pass+' '+cje_master_url+'| grep HTTP
|tail -1').stdout
if '200 OK' in results:
return True
else:
return False
removed unnecessary fixture from many more functions and was able to clean my code a lot. Thanks!
add a comment |
Well, I've done as hoefling suggested.
Created small function in conftest.py:
def get_env_params():
env_name = pytest.config.getoption("--env")
return env_params[env_name]
and call it from my module functions where needed. Example function looks like below:
def request_master_url(team_id):
envparams = get_env_params()
cje_master_url = envparams['url']+'/'+team_id
cje_user = envparams['user']
cje_pass = envparams['password']
local = testinfra.get_host("ssh://localhost")
results = local.command(
'curl -L -I --user '+cje_user+':'
+ cje_pass+' '+cje_master_url+'| grep HTTP
|tail -1').stdout
if '200 OK' in results:
return True
else:
return False
removed unnecessary fixture from many more functions and was able to clean my code a lot. Thanks!
add a comment |
Well, I've done as hoefling suggested.
Created small function in conftest.py:
def get_env_params():
env_name = pytest.config.getoption("--env")
return env_params[env_name]
and call it from my module functions where needed. Example function looks like below:
def request_master_url(team_id):
envparams = get_env_params()
cje_master_url = envparams['url']+'/'+team_id
cje_user = envparams['user']
cje_pass = envparams['password']
local = testinfra.get_host("ssh://localhost")
results = local.command(
'curl -L -I --user '+cje_user+':'
+ cje_pass+' '+cje_master_url+'| grep HTTP
|tail -1').stdout
if '200 OK' in results:
return True
else:
return False
removed unnecessary fixture from many more functions and was able to clean my code a lot. Thanks!
Well, I've done as hoefling suggested.
Created small function in conftest.py:
def get_env_params():
env_name = pytest.config.getoption("--env")
return env_params[env_name]
and call it from my module functions where needed. Example function looks like below:
def request_master_url(team_id):
envparams = get_env_params()
cje_master_url = envparams['url']+'/'+team_id
cje_user = envparams['user']
cje_pass = envparams['password']
local = testinfra.get_host("ssh://localhost")
results = local.command(
'curl -L -I --user '+cje_user+':'
+ cje_pass+' '+cje_master_url+'| grep HTTP
|tail -1').stdout
if '200 OK' in results:
return True
else:
return False
removed unnecessary fixture from many more functions and was able to clean my code a lot. Thanks!
answered Dec 6 '18 at 15:56
KrzysztofKrzysztof
735
735
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325313%2fhow-to-get-return-value-from-pytest-fixture-in-a-function-so-i-dont-need-to-cal%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
yegTrBto,1E,2 FJYy,5NoSN4XUcO,seD,9Txwyb802oe h r0n,2
Why not making a fixture out of
request_master_url
?– hoefling
Nov 16 '18 at 9:33
it's some solution, but I have many more functions like this one that are also using envparams fixture. I think it would be perhaps, better to create a global parameter in conftest.py and set it to envparams fixture for a session. I'll just read that from my module functions and no additional parameter would be needed to call from test functions.
– Krzysztof
Nov 19 '18 at 9:29
Thing is, you can't apply
pytest
machinery to normal functions per se. Ifrequest_master_url
should be a function and is used in tests only, you can get the env viapytest.config.getoption('--env')
in the function directly, so you don't need to pass theenvparams
argument. Is this suitable to your needs?– hoefling
Nov 19 '18 at 9:46
yes, this was very helpfull actually. Implemented today.
– Krzysztof
Dec 6 '18 at 15:59