AJAX - Post to MVC Controller method without reloading page
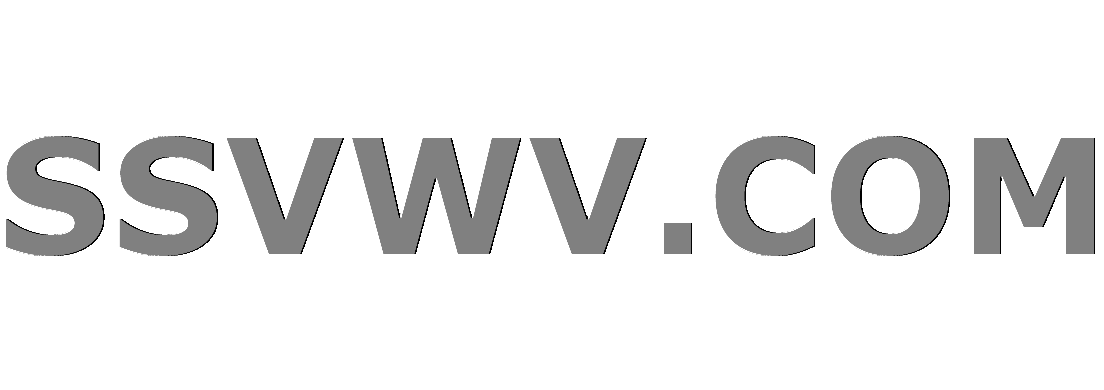
Multi tool use
I created an MVC View page where, when a user clicks a button, a Controller method is called. The method returns a text expression. This expression is then displayed in a pop-up message.
The pop-up functionality works when the button is clicked, however, it has an unwanted side effect of causing the page to reload. Is there a way to prevent this?
Here is the HTML which renders the button:
<button id="btnListGroups" title="List the groups the selected service ID is a member of">List Groups</button>
Here is the JavaScript:
$(function () {
$("#btnListGroups").click(function () {
var val = $("#ddlServiceID").val();
$.post("/Home/GetGroups", { serviceID: val }, function (data, textStatus)
{
alert(data);
});
});
})
And finally, here is the Controller method:
public partial class HomeController : Controller
{
public MvcHtmlString GetGroups(string serviceID)
{
ADHandler adHandler = new ADHandler();
string groups = adHandler.GetGroupMemberships(serviceID);
return new MvcHtmlString(groups);
}
}
javascript c# jquery ajax asp.net-mvc
add a comment |
I created an MVC View page where, when a user clicks a button, a Controller method is called. The method returns a text expression. This expression is then displayed in a pop-up message.
The pop-up functionality works when the button is clicked, however, it has an unwanted side effect of causing the page to reload. Is there a way to prevent this?
Here is the HTML which renders the button:
<button id="btnListGroups" title="List the groups the selected service ID is a member of">List Groups</button>
Here is the JavaScript:
$(function () {
$("#btnListGroups").click(function () {
var val = $("#ddlServiceID").val();
$.post("/Home/GetGroups", { serviceID: val }, function (data, textStatus)
{
alert(data);
});
});
})
And finally, here is the Controller method:
public partial class HomeController : Controller
{
public MvcHtmlString GetGroups(string serviceID)
{
ADHandler adHandler = new ADHandler();
string groups = adHandler.GetGroupMemberships(serviceID);
return new MvcHtmlString(groups);
}
}
javascript c# jquery ajax asp.net-mvc
2
This shouldn't be happening from a basic button click unless it is connected to a form. Did you try adding event.preventDefault(); to the js function?
– Sean Leroy
Nov 15 '18 at 18:04
is your button inside a form ? usepreventDefault
– Shyju
Nov 15 '18 at 18:09
@SeanLeroy - Thanks! Yes, the button exists within a form block. adding event.preventDefault(); to the .click function resolved the issue.
– Mike Bruno
Nov 15 '18 at 18:16
Don't forget to install query.unobtrusive-ajax package from nuget manager in your project.
– OfficalMesut
Nov 16 '18 at 14:14
add a comment |
I created an MVC View page where, when a user clicks a button, a Controller method is called. The method returns a text expression. This expression is then displayed in a pop-up message.
The pop-up functionality works when the button is clicked, however, it has an unwanted side effect of causing the page to reload. Is there a way to prevent this?
Here is the HTML which renders the button:
<button id="btnListGroups" title="List the groups the selected service ID is a member of">List Groups</button>
Here is the JavaScript:
$(function () {
$("#btnListGroups").click(function () {
var val = $("#ddlServiceID").val();
$.post("/Home/GetGroups", { serviceID: val }, function (data, textStatus)
{
alert(data);
});
});
})
And finally, here is the Controller method:
public partial class HomeController : Controller
{
public MvcHtmlString GetGroups(string serviceID)
{
ADHandler adHandler = new ADHandler();
string groups = adHandler.GetGroupMemberships(serviceID);
return new MvcHtmlString(groups);
}
}
javascript c# jquery ajax asp.net-mvc
I created an MVC View page where, when a user clicks a button, a Controller method is called. The method returns a text expression. This expression is then displayed in a pop-up message.
The pop-up functionality works when the button is clicked, however, it has an unwanted side effect of causing the page to reload. Is there a way to prevent this?
Here is the HTML which renders the button:
<button id="btnListGroups" title="List the groups the selected service ID is a member of">List Groups</button>
Here is the JavaScript:
$(function () {
$("#btnListGroups").click(function () {
var val = $("#ddlServiceID").val();
$.post("/Home/GetGroups", { serviceID: val }, function (data, textStatus)
{
alert(data);
});
});
})
And finally, here is the Controller method:
public partial class HomeController : Controller
{
public MvcHtmlString GetGroups(string serviceID)
{
ADHandler adHandler = new ADHandler();
string groups = adHandler.GetGroupMemberships(serviceID);
return new MvcHtmlString(groups);
}
}
<button id="btnListGroups" title="List the groups the selected service ID is a member of">List Groups</button>
<button id="btnListGroups" title="List the groups the selected service ID is a member of">List Groups</button>
$(function () {
$("#btnListGroups").click(function () {
var val = $("#ddlServiceID").val();
$.post("/Home/GetGroups", { serviceID: val }, function (data, textStatus)
{
alert(data);
});
});
})
$(function () {
$("#btnListGroups").click(function () {
var val = $("#ddlServiceID").val();
$.post("/Home/GetGroups", { serviceID: val }, function (data, textStatus)
{
alert(data);
});
});
})
javascript c# jquery ajax asp.net-mvc
javascript c# jquery ajax asp.net-mvc
asked Nov 15 '18 at 17:45
Mike BrunoMike Bruno
7214
7214
2
This shouldn't be happening from a basic button click unless it is connected to a form. Did you try adding event.preventDefault(); to the js function?
– Sean Leroy
Nov 15 '18 at 18:04
is your button inside a form ? usepreventDefault
– Shyju
Nov 15 '18 at 18:09
@SeanLeroy - Thanks! Yes, the button exists within a form block. adding event.preventDefault(); to the .click function resolved the issue.
– Mike Bruno
Nov 15 '18 at 18:16
Don't forget to install query.unobtrusive-ajax package from nuget manager in your project.
– OfficalMesut
Nov 16 '18 at 14:14
add a comment |
2
This shouldn't be happening from a basic button click unless it is connected to a form. Did you try adding event.preventDefault(); to the js function?
– Sean Leroy
Nov 15 '18 at 18:04
is your button inside a form ? usepreventDefault
– Shyju
Nov 15 '18 at 18:09
@SeanLeroy - Thanks! Yes, the button exists within a form block. adding event.preventDefault(); to the .click function resolved the issue.
– Mike Bruno
Nov 15 '18 at 18:16
Don't forget to install query.unobtrusive-ajax package from nuget manager in your project.
– OfficalMesut
Nov 16 '18 at 14:14
2
2
This shouldn't be happening from a basic button click unless it is connected to a form. Did you try adding event.preventDefault(); to the js function?
– Sean Leroy
Nov 15 '18 at 18:04
This shouldn't be happening from a basic button click unless it is connected to a form. Did you try adding event.preventDefault(); to the js function?
– Sean Leroy
Nov 15 '18 at 18:04
is your button inside a form ? use
preventDefault
– Shyju
Nov 15 '18 at 18:09
is your button inside a form ? use
preventDefault
– Shyju
Nov 15 '18 at 18:09
@SeanLeroy - Thanks! Yes, the button exists within a form block. adding event.preventDefault(); to the .click function resolved the issue.
– Mike Bruno
Nov 15 '18 at 18:16
@SeanLeroy - Thanks! Yes, the button exists within a form block. adding event.preventDefault(); to the .click function resolved the issue.
– Mike Bruno
Nov 15 '18 at 18:16
Don't forget to install query.unobtrusive-ajax package from nuget manager in your project.
– OfficalMesut
Nov 16 '18 at 14:14
Don't forget to install query.unobtrusive-ajax package from nuget manager in your project.
– OfficalMesut
Nov 16 '18 at 14:14
add a comment |
1 Answer
1
active
oldest
votes
The page reload was occurring because the button exists within an HTML Form. By default, the "type" attribute of HTML buttons is "submit" unless otherwise specified. Since I hadn't specified a "type" for my button, clicking the button was being interpreted as as a form submit.
To resolve this, I set the type attribute for my button as "button"
<button type="button" id="btnListGroups" title="List the groups the selected service ID is a member of">List Groups</button>
4
Also,<button />
default value for "type" is submit; if you define a button as<button type="button" .. />
it also won't postback.
– Brian Mains
Nov 15 '18 at 18:52
2
@MikeBruno +1 to Brian's solution as it is a bit cleaner in my opinion
– GregH
Nov 15 '18 at 20:02
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325187%2fajax-post-to-mvc-controller-method-without-reloading-page%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The page reload was occurring because the button exists within an HTML Form. By default, the "type" attribute of HTML buttons is "submit" unless otherwise specified. Since I hadn't specified a "type" for my button, clicking the button was being interpreted as as a form submit.
To resolve this, I set the type attribute for my button as "button"
<button type="button" id="btnListGroups" title="List the groups the selected service ID is a member of">List Groups</button>
4
Also,<button />
default value for "type" is submit; if you define a button as<button type="button" .. />
it also won't postback.
– Brian Mains
Nov 15 '18 at 18:52
2
@MikeBruno +1 to Brian's solution as it is a bit cleaner in my opinion
– GregH
Nov 15 '18 at 20:02
add a comment |
The page reload was occurring because the button exists within an HTML Form. By default, the "type" attribute of HTML buttons is "submit" unless otherwise specified. Since I hadn't specified a "type" for my button, clicking the button was being interpreted as as a form submit.
To resolve this, I set the type attribute for my button as "button"
<button type="button" id="btnListGroups" title="List the groups the selected service ID is a member of">List Groups</button>
4
Also,<button />
default value for "type" is submit; if you define a button as<button type="button" .. />
it also won't postback.
– Brian Mains
Nov 15 '18 at 18:52
2
@MikeBruno +1 to Brian's solution as it is a bit cleaner in my opinion
– GregH
Nov 15 '18 at 20:02
add a comment |
The page reload was occurring because the button exists within an HTML Form. By default, the "type" attribute of HTML buttons is "submit" unless otherwise specified. Since I hadn't specified a "type" for my button, clicking the button was being interpreted as as a form submit.
To resolve this, I set the type attribute for my button as "button"
<button type="button" id="btnListGroups" title="List the groups the selected service ID is a member of">List Groups</button>
The page reload was occurring because the button exists within an HTML Form. By default, the "type" attribute of HTML buttons is "submit" unless otherwise specified. Since I hadn't specified a "type" for my button, clicking the button was being interpreted as as a form submit.
To resolve this, I set the type attribute for my button as "button"
<button type="button" id="btnListGroups" title="List the groups the selected service ID is a member of">List Groups</button>
<button type="button" id="btnListGroups" title="List the groups the selected service ID is a member of">List Groups</button>
<button type="button" id="btnListGroups" title="List the groups the selected service ID is a member of">List Groups</button>
edited Nov 16 '18 at 14:11
answered Nov 15 '18 at 18:21
Mike BrunoMike Bruno
7214
7214
4
Also,<button />
default value for "type" is submit; if you define a button as<button type="button" .. />
it also won't postback.
– Brian Mains
Nov 15 '18 at 18:52
2
@MikeBruno +1 to Brian's solution as it is a bit cleaner in my opinion
– GregH
Nov 15 '18 at 20:02
add a comment |
4
Also,<button />
default value for "type" is submit; if you define a button as<button type="button" .. />
it also won't postback.
– Brian Mains
Nov 15 '18 at 18:52
2
@MikeBruno +1 to Brian's solution as it is a bit cleaner in my opinion
– GregH
Nov 15 '18 at 20:02
4
4
Also,
<button />
default value for "type" is submit; if you define a button as <button type="button" .. />
it also won't postback.– Brian Mains
Nov 15 '18 at 18:52
Also,
<button />
default value for "type" is submit; if you define a button as <button type="button" .. />
it also won't postback.– Brian Mains
Nov 15 '18 at 18:52
2
2
@MikeBruno +1 to Brian's solution as it is a bit cleaner in my opinion
– GregH
Nov 15 '18 at 20:02
@MikeBruno +1 to Brian's solution as it is a bit cleaner in my opinion
– GregH
Nov 15 '18 at 20:02
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325187%2fajax-post-to-mvc-controller-method-without-reloading-page%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Lt,OAaH FOtyfbcUKnziIff5cvq6s6Dn o7qByliORWCt1L9U2Narvw,3Gs EAcsC0 XoVeTZVmMWZh4PJwuOQXTOGelnuCqXcNa wvjr5
2
This shouldn't be happening from a basic button click unless it is connected to a form. Did you try adding event.preventDefault(); to the js function?
– Sean Leroy
Nov 15 '18 at 18:04
is your button inside a form ? use
preventDefault
– Shyju
Nov 15 '18 at 18:09
@SeanLeroy - Thanks! Yes, the button exists within a form block. adding event.preventDefault(); to the .click function resolved the issue.
– Mike Bruno
Nov 15 '18 at 18:16
Don't forget to install query.unobtrusive-ajax package from nuget manager in your project.
– OfficalMesut
Nov 16 '18 at 14:14