Embedding Relations In Results
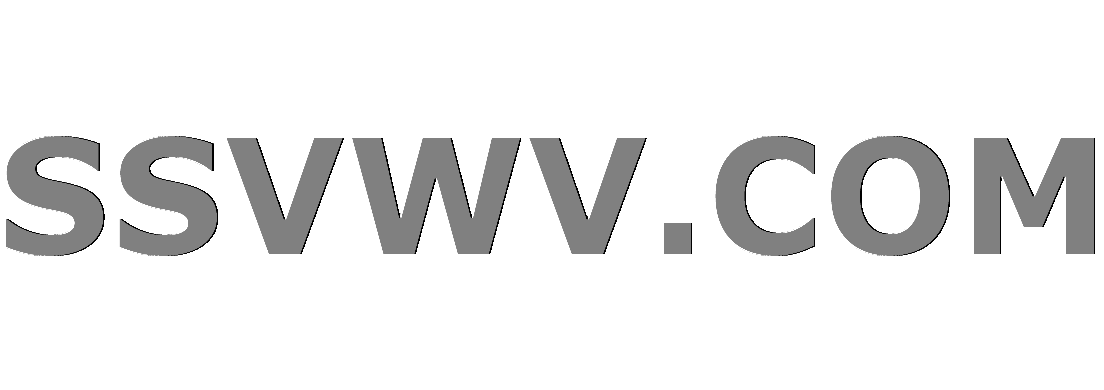
Multi tool use
I'm using API-Platform to deliver content via API. I have a Potential relationship between users and participants (not all users will have participants but all participants will have at least one user). My main goal is to embed the relationship's User data in the result set of Participants as that result will be consumed by a data table and it would be more efficient to have that data already present within the result opposed to performing an additional request for the data.
e.g.:
{
"@context": "/api/contexts/Participants",
"@id": "/api/participants",
"@type": "hydra:Collection",
"hydra:member": [
{
"@id": "/api/participants/1",
"@type": "Participants",
"id": 1,
"name": "Jeffrey Jones",
"users": [
{
"@id": "/api/users/1",
"@type": "User",
"name": "Jenny Jones"
},
{
"@id": "/api/users/2",
"@type": "User",
"name": "Jessie Jones"
}
]
}
],
"hydra:totalItems": 1
}
However, I'm not sure if this is possible. I have looked at https://api-platform.com/docs/core/serialization#embedding-relations but I am not certain it would work for multiple result sets as the example is one book to one author. However, my scenario is one participant to multiple users.
Also (and I may need to go about this in a more direct manner), I'm using a joining table so that I can assign additional metadata to the relationship. So... participants > joint table (containing additional data) > users (and vice versa). Again, I may need to consider having a direct relationship between participants and users and then using a ParticipantUserMeta table to hold the additional metadata. However, at the moment, I'm leaning towards the join table containing the association as well as the additional metadata.
Here are the basics of my entities (most unnecessary data omitted):
User:
/**
* @ApiResource
* ...
*/
class User implements UserInterface, Serializable
{
/**
* @var int
*
* @ORMId
* @ORMGeneratedValue
* @ORMColumn(type="integer")
*/
private $id;
/**
* @var string
* @ORMColumn(type="string")
* @AssertNotBlank()
*/
private $name = '';
/**
* @ORMOneToMany(targetEntity="AppEntityParticipantRel", mappedBy="user")
*/
private $participants;
public function __construct()
{
$this->participants = new ArrayCollection();
}
public function getId(): int
{
return $this->id;
}
public function getName(): string
{
return $this->name;
}
/**
* @return Collection|ParticipantRel
*/
public function getParticipants(): Collection
{
return $this->participants;
}
}
ParticipantRel:
/**
* @ApiResource
* ...
*/
class ParticipantRel
{
/**
* @var int The Participant Id
*
* @ORMId
* @ORMGeneratedValue
* @ORMColumn(type="integer")
*/
private $id;
/**
* @var int
*
* @ORMColumn(type="boolean")
*/
private $primary_contact;
/**
* @var string Relationship notes
*
* @ORMColumn(type="text", nullable=true)
*/
private $notes;
/**
* @ORMManyToOne(targetEntity="AppEntityParticipants", inversedBy="users")
* @ORMJoinColumn(nullable=false)
*/
private $participant;
/**
* @ORMManyToOne(targetEntity="AppEntityUser", inversedBy="participants")
* @ORMJoinColumn(nullable=false)
*/
private $user;
public function getId (): int
{
return $this->id;
}
public function getPrimaryContact(): ?bool
{
return $this->primary_contact;
}
public function getNotes(): ?string
{
return $this->notes;
}
public function getParticipant(): ?Participants
{
return $this->participant;
}
public function getUser(): ?User
{
return $this->user;
}
}
Participants
/**
* @ApiResource
* ...
*/
class Participants
{
/**
* @var int The Participant Id
*
* @ORMId
* @ORMGeneratedValue
* @ORMColumn(type="integer")
*/
private $id;
/**
* @var string Participant's first name
*
* @ORMColumn(name="name")
* @AssertNotBlank
*/
public $name;
/**
* @ORMOneToMany(targetEntity="AppEntityParticipantRel", mappedBy="participant")
*/
private $users;
public function __construct() {
$this->users = new ArrayCollection();
}
public function getId (): int
{
return $this->id;
}
public function getName(): ?string
{
return $this->name;
}
/**
* @return Collection|ParticipantRel
*/
public function getUsers(): Collection
{
return $this->users;
}
}
My question: Is what I'm attempting possible within an entity and if so, what am I missing? I have researched this a lot before coming here but haven't come up w/ any solution as most of the solutions I see involve a Twig tpl but I'm simply sending the data via api-platform. Any positive direction would be greatly appreciated.
doctrine-orm symfony4 api-platform.com
add a comment |
I'm using API-Platform to deliver content via API. I have a Potential relationship between users and participants (not all users will have participants but all participants will have at least one user). My main goal is to embed the relationship's User data in the result set of Participants as that result will be consumed by a data table and it would be more efficient to have that data already present within the result opposed to performing an additional request for the data.
e.g.:
{
"@context": "/api/contexts/Participants",
"@id": "/api/participants",
"@type": "hydra:Collection",
"hydra:member": [
{
"@id": "/api/participants/1",
"@type": "Participants",
"id": 1,
"name": "Jeffrey Jones",
"users": [
{
"@id": "/api/users/1",
"@type": "User",
"name": "Jenny Jones"
},
{
"@id": "/api/users/2",
"@type": "User",
"name": "Jessie Jones"
}
]
}
],
"hydra:totalItems": 1
}
However, I'm not sure if this is possible. I have looked at https://api-platform.com/docs/core/serialization#embedding-relations but I am not certain it would work for multiple result sets as the example is one book to one author. However, my scenario is one participant to multiple users.
Also (and I may need to go about this in a more direct manner), I'm using a joining table so that I can assign additional metadata to the relationship. So... participants > joint table (containing additional data) > users (and vice versa). Again, I may need to consider having a direct relationship between participants and users and then using a ParticipantUserMeta table to hold the additional metadata. However, at the moment, I'm leaning towards the join table containing the association as well as the additional metadata.
Here are the basics of my entities (most unnecessary data omitted):
User:
/**
* @ApiResource
* ...
*/
class User implements UserInterface, Serializable
{
/**
* @var int
*
* @ORMId
* @ORMGeneratedValue
* @ORMColumn(type="integer")
*/
private $id;
/**
* @var string
* @ORMColumn(type="string")
* @AssertNotBlank()
*/
private $name = '';
/**
* @ORMOneToMany(targetEntity="AppEntityParticipantRel", mappedBy="user")
*/
private $participants;
public function __construct()
{
$this->participants = new ArrayCollection();
}
public function getId(): int
{
return $this->id;
}
public function getName(): string
{
return $this->name;
}
/**
* @return Collection|ParticipantRel
*/
public function getParticipants(): Collection
{
return $this->participants;
}
}
ParticipantRel:
/**
* @ApiResource
* ...
*/
class ParticipantRel
{
/**
* @var int The Participant Id
*
* @ORMId
* @ORMGeneratedValue
* @ORMColumn(type="integer")
*/
private $id;
/**
* @var int
*
* @ORMColumn(type="boolean")
*/
private $primary_contact;
/**
* @var string Relationship notes
*
* @ORMColumn(type="text", nullable=true)
*/
private $notes;
/**
* @ORMManyToOne(targetEntity="AppEntityParticipants", inversedBy="users")
* @ORMJoinColumn(nullable=false)
*/
private $participant;
/**
* @ORMManyToOne(targetEntity="AppEntityUser", inversedBy="participants")
* @ORMJoinColumn(nullable=false)
*/
private $user;
public function getId (): int
{
return $this->id;
}
public function getPrimaryContact(): ?bool
{
return $this->primary_contact;
}
public function getNotes(): ?string
{
return $this->notes;
}
public function getParticipant(): ?Participants
{
return $this->participant;
}
public function getUser(): ?User
{
return $this->user;
}
}
Participants
/**
* @ApiResource
* ...
*/
class Participants
{
/**
* @var int The Participant Id
*
* @ORMId
* @ORMGeneratedValue
* @ORMColumn(type="integer")
*/
private $id;
/**
* @var string Participant's first name
*
* @ORMColumn(name="name")
* @AssertNotBlank
*/
public $name;
/**
* @ORMOneToMany(targetEntity="AppEntityParticipantRel", mappedBy="participant")
*/
private $users;
public function __construct() {
$this->users = new ArrayCollection();
}
public function getId (): int
{
return $this->id;
}
public function getName(): ?string
{
return $this->name;
}
/**
* @return Collection|ParticipantRel
*/
public function getUsers(): Collection
{
return $this->users;
}
}
My question: Is what I'm attempting possible within an entity and if so, what am I missing? I have researched this a lot before coming here but haven't come up w/ any solution as most of the solutions I see involve a Twig tpl but I'm simply sending the data via api-platform. Any positive direction would be greatly appreciated.
doctrine-orm symfony4 api-platform.com
add a comment |
I'm using API-Platform to deliver content via API. I have a Potential relationship between users and participants (not all users will have participants but all participants will have at least one user). My main goal is to embed the relationship's User data in the result set of Participants as that result will be consumed by a data table and it would be more efficient to have that data already present within the result opposed to performing an additional request for the data.
e.g.:
{
"@context": "/api/contexts/Participants",
"@id": "/api/participants",
"@type": "hydra:Collection",
"hydra:member": [
{
"@id": "/api/participants/1",
"@type": "Participants",
"id": 1,
"name": "Jeffrey Jones",
"users": [
{
"@id": "/api/users/1",
"@type": "User",
"name": "Jenny Jones"
},
{
"@id": "/api/users/2",
"@type": "User",
"name": "Jessie Jones"
}
]
}
],
"hydra:totalItems": 1
}
However, I'm not sure if this is possible. I have looked at https://api-platform.com/docs/core/serialization#embedding-relations but I am not certain it would work for multiple result sets as the example is one book to one author. However, my scenario is one participant to multiple users.
Also (and I may need to go about this in a more direct manner), I'm using a joining table so that I can assign additional metadata to the relationship. So... participants > joint table (containing additional data) > users (and vice versa). Again, I may need to consider having a direct relationship between participants and users and then using a ParticipantUserMeta table to hold the additional metadata. However, at the moment, I'm leaning towards the join table containing the association as well as the additional metadata.
Here are the basics of my entities (most unnecessary data omitted):
User:
/**
* @ApiResource
* ...
*/
class User implements UserInterface, Serializable
{
/**
* @var int
*
* @ORMId
* @ORMGeneratedValue
* @ORMColumn(type="integer")
*/
private $id;
/**
* @var string
* @ORMColumn(type="string")
* @AssertNotBlank()
*/
private $name = '';
/**
* @ORMOneToMany(targetEntity="AppEntityParticipantRel", mappedBy="user")
*/
private $participants;
public function __construct()
{
$this->participants = new ArrayCollection();
}
public function getId(): int
{
return $this->id;
}
public function getName(): string
{
return $this->name;
}
/**
* @return Collection|ParticipantRel
*/
public function getParticipants(): Collection
{
return $this->participants;
}
}
ParticipantRel:
/**
* @ApiResource
* ...
*/
class ParticipantRel
{
/**
* @var int The Participant Id
*
* @ORMId
* @ORMGeneratedValue
* @ORMColumn(type="integer")
*/
private $id;
/**
* @var int
*
* @ORMColumn(type="boolean")
*/
private $primary_contact;
/**
* @var string Relationship notes
*
* @ORMColumn(type="text", nullable=true)
*/
private $notes;
/**
* @ORMManyToOne(targetEntity="AppEntityParticipants", inversedBy="users")
* @ORMJoinColumn(nullable=false)
*/
private $participant;
/**
* @ORMManyToOne(targetEntity="AppEntityUser", inversedBy="participants")
* @ORMJoinColumn(nullable=false)
*/
private $user;
public function getId (): int
{
return $this->id;
}
public function getPrimaryContact(): ?bool
{
return $this->primary_contact;
}
public function getNotes(): ?string
{
return $this->notes;
}
public function getParticipant(): ?Participants
{
return $this->participant;
}
public function getUser(): ?User
{
return $this->user;
}
}
Participants
/**
* @ApiResource
* ...
*/
class Participants
{
/**
* @var int The Participant Id
*
* @ORMId
* @ORMGeneratedValue
* @ORMColumn(type="integer")
*/
private $id;
/**
* @var string Participant's first name
*
* @ORMColumn(name="name")
* @AssertNotBlank
*/
public $name;
/**
* @ORMOneToMany(targetEntity="AppEntityParticipantRel", mappedBy="participant")
*/
private $users;
public function __construct() {
$this->users = new ArrayCollection();
}
public function getId (): int
{
return $this->id;
}
public function getName(): ?string
{
return $this->name;
}
/**
* @return Collection|ParticipantRel
*/
public function getUsers(): Collection
{
return $this->users;
}
}
My question: Is what I'm attempting possible within an entity and if so, what am I missing? I have researched this a lot before coming here but haven't come up w/ any solution as most of the solutions I see involve a Twig tpl but I'm simply sending the data via api-platform. Any positive direction would be greatly appreciated.
doctrine-orm symfony4 api-platform.com
I'm using API-Platform to deliver content via API. I have a Potential relationship between users and participants (not all users will have participants but all participants will have at least one user). My main goal is to embed the relationship's User data in the result set of Participants as that result will be consumed by a data table and it would be more efficient to have that data already present within the result opposed to performing an additional request for the data.
e.g.:
{
"@context": "/api/contexts/Participants",
"@id": "/api/participants",
"@type": "hydra:Collection",
"hydra:member": [
{
"@id": "/api/participants/1",
"@type": "Participants",
"id": 1,
"name": "Jeffrey Jones",
"users": [
{
"@id": "/api/users/1",
"@type": "User",
"name": "Jenny Jones"
},
{
"@id": "/api/users/2",
"@type": "User",
"name": "Jessie Jones"
}
]
}
],
"hydra:totalItems": 1
}
However, I'm not sure if this is possible. I have looked at https://api-platform.com/docs/core/serialization#embedding-relations but I am not certain it would work for multiple result sets as the example is one book to one author. However, my scenario is one participant to multiple users.
Also (and I may need to go about this in a more direct manner), I'm using a joining table so that I can assign additional metadata to the relationship. So... participants > joint table (containing additional data) > users (and vice versa). Again, I may need to consider having a direct relationship between participants and users and then using a ParticipantUserMeta table to hold the additional metadata. However, at the moment, I'm leaning towards the join table containing the association as well as the additional metadata.
Here are the basics of my entities (most unnecessary data omitted):
User:
/**
* @ApiResource
* ...
*/
class User implements UserInterface, Serializable
{
/**
* @var int
*
* @ORMId
* @ORMGeneratedValue
* @ORMColumn(type="integer")
*/
private $id;
/**
* @var string
* @ORMColumn(type="string")
* @AssertNotBlank()
*/
private $name = '';
/**
* @ORMOneToMany(targetEntity="AppEntityParticipantRel", mappedBy="user")
*/
private $participants;
public function __construct()
{
$this->participants = new ArrayCollection();
}
public function getId(): int
{
return $this->id;
}
public function getName(): string
{
return $this->name;
}
/**
* @return Collection|ParticipantRel
*/
public function getParticipants(): Collection
{
return $this->participants;
}
}
ParticipantRel:
/**
* @ApiResource
* ...
*/
class ParticipantRel
{
/**
* @var int The Participant Id
*
* @ORMId
* @ORMGeneratedValue
* @ORMColumn(type="integer")
*/
private $id;
/**
* @var int
*
* @ORMColumn(type="boolean")
*/
private $primary_contact;
/**
* @var string Relationship notes
*
* @ORMColumn(type="text", nullable=true)
*/
private $notes;
/**
* @ORMManyToOne(targetEntity="AppEntityParticipants", inversedBy="users")
* @ORMJoinColumn(nullable=false)
*/
private $participant;
/**
* @ORMManyToOne(targetEntity="AppEntityUser", inversedBy="participants")
* @ORMJoinColumn(nullable=false)
*/
private $user;
public function getId (): int
{
return $this->id;
}
public function getPrimaryContact(): ?bool
{
return $this->primary_contact;
}
public function getNotes(): ?string
{
return $this->notes;
}
public function getParticipant(): ?Participants
{
return $this->participant;
}
public function getUser(): ?User
{
return $this->user;
}
}
Participants
/**
* @ApiResource
* ...
*/
class Participants
{
/**
* @var int The Participant Id
*
* @ORMId
* @ORMGeneratedValue
* @ORMColumn(type="integer")
*/
private $id;
/**
* @var string Participant's first name
*
* @ORMColumn(name="name")
* @AssertNotBlank
*/
public $name;
/**
* @ORMOneToMany(targetEntity="AppEntityParticipantRel", mappedBy="participant")
*/
private $users;
public function __construct() {
$this->users = new ArrayCollection();
}
public function getId (): int
{
return $this->id;
}
public function getName(): ?string
{
return $this->name;
}
/**
* @return Collection|ParticipantRel
*/
public function getUsers(): Collection
{
return $this->users;
}
}
My question: Is what I'm attempting possible within an entity and if so, what am I missing? I have researched this a lot before coming here but haven't come up w/ any solution as most of the solutions I see involve a Twig tpl but I'm simply sending the data via api-platform. Any positive direction would be greatly appreciated.
doctrine-orm symfony4 api-platform.com
doctrine-orm symfony4 api-platform.com
edited Nov 15 '18 at 19:10
user2220551
asked Nov 15 '18 at 17:54
user2220551user2220551
86
86
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
So, it turns out that I just needed to experiment more w/ the Groups option (https://api-platform.com/docs/core/serialization#embedding-relations). Associating all related fields w/ the relative groups on all relative enities did end up returning the results in the desired format.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325318%2fembedding-relations-in-results%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
So, it turns out that I just needed to experiment more w/ the Groups option (https://api-platform.com/docs/core/serialization#embedding-relations). Associating all related fields w/ the relative groups on all relative enities did end up returning the results in the desired format.
add a comment |
So, it turns out that I just needed to experiment more w/ the Groups option (https://api-platform.com/docs/core/serialization#embedding-relations). Associating all related fields w/ the relative groups on all relative enities did end up returning the results in the desired format.
add a comment |
So, it turns out that I just needed to experiment more w/ the Groups option (https://api-platform.com/docs/core/serialization#embedding-relations). Associating all related fields w/ the relative groups on all relative enities did end up returning the results in the desired format.
So, it turns out that I just needed to experiment more w/ the Groups option (https://api-platform.com/docs/core/serialization#embedding-relations). Associating all related fields w/ the relative groups on all relative enities did end up returning the results in the desired format.
edited Nov 29 '18 at 21:06
answered Nov 18 '18 at 15:33
user2220551user2220551
86
86
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325318%2fembedding-relations-in-results%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0r28GLTzx43ldaVxH8,NOMnk7XBvKxlYU6GJOBN,EYDVQATD RjJFPghay0Fz