Why can a function take (int *&) as a parameter? [duplicate]
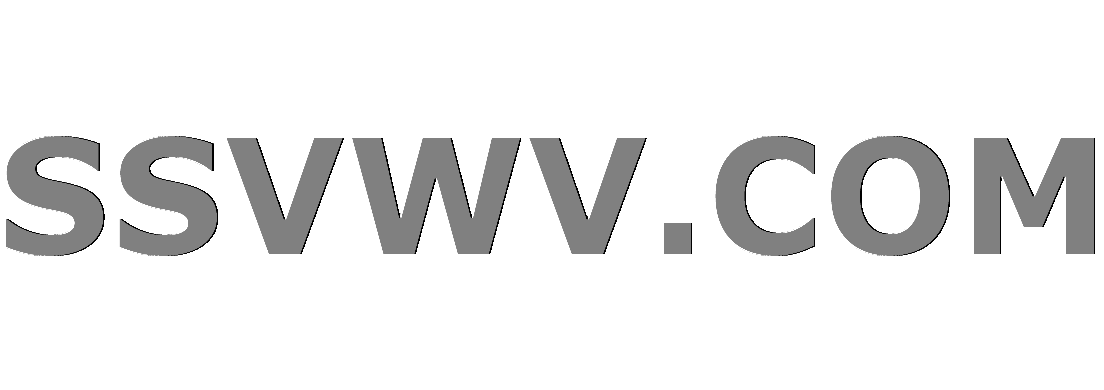
Multi tool use
This question already has an answer here:
Difference between pointer to a reference and reference to a pointer
6 answers
Following is a code to split an array. The
first output array will contain the input array entries in between the given two indices and the second
output array will contain the rest of the entries.
void splitArray(int *arr, int size, int ind1, int ind2,
int *&first, int &firstSize, int *&second, int &secondSize){
firstSize = ind2 - ind1 + 1;
secondSize = size - firstSize;
first = new int[firstSize];
second = new int[secondSize];
for (int i = 0; i < ind1; i++)
second[i] = arr[i];
for (int i = ind1; i <= ind2; i++)
first[i - ind1] = arr[i];
for (int i = ind2 + 1; i < size; i++)
second[i - firstSize] = arr[i];
}
The thing I can understand why it has parameters like int *&first
and int *&second
, arent they are same as just int first
and int second
but in this code they are used as pointers to dynamic arrays.
c++ arrays pointers
marked as duplicate by kabanus, DevSolar
StackExchange.ready(function() {
if (StackExchange.options.isMobile) return;
$('.dupe-hammer-message-hover:not(.hover-bound)').each(function() {
var $hover = $(this).addClass('hover-bound'),
$msg = $hover.siblings('.dupe-hammer-message');
$hover.hover(
function() {
$hover.showInfoMessage('', {
messageElement: $msg.clone().show(),
transient: false,
position: { my: 'bottom left', at: 'top center', offsetTop: -7 },
dismissable: false,
relativeToBody: true
});
},
function() {
StackExchange.helpers.removeMessages();
}
);
});
});
Nov 15 '18 at 9:05
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
add a comment |
This question already has an answer here:
Difference between pointer to a reference and reference to a pointer
6 answers
Following is a code to split an array. The
first output array will contain the input array entries in between the given two indices and the second
output array will contain the rest of the entries.
void splitArray(int *arr, int size, int ind1, int ind2,
int *&first, int &firstSize, int *&second, int &secondSize){
firstSize = ind2 - ind1 + 1;
secondSize = size - firstSize;
first = new int[firstSize];
second = new int[secondSize];
for (int i = 0; i < ind1; i++)
second[i] = arr[i];
for (int i = ind1; i <= ind2; i++)
first[i - ind1] = arr[i];
for (int i = ind2 + 1; i < size; i++)
second[i - firstSize] = arr[i];
}
The thing I can understand why it has parameters like int *&first
and int *&second
, arent they are same as just int first
and int second
but in this code they are used as pointers to dynamic arrays.
c++ arrays pointers
marked as duplicate by kabanus, DevSolar
StackExchange.ready(function() {
if (StackExchange.options.isMobile) return;
$('.dupe-hammer-message-hover:not(.hover-bound)').each(function() {
var $hover = $(this).addClass('hover-bound'),
$msg = $hover.siblings('.dupe-hammer-message');
$hover.hover(
function() {
$hover.showInfoMessage('', {
messageElement: $msg.clone().show(),
transient: false,
position: { my: 'bottom left', at: 'top center', offsetTop: -7 },
dismissable: false,
relativeToBody: true
});
},
function() {
StackExchange.helpers.removeMessages();
}
);
});
});
Nov 15 '18 at 9:05
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
6
No, they aren't -int*&
is a reference to a pointer toint
.
– UnholySheep
Nov 15 '18 at 8:57
Here,&
means "This is a C++ reference", not "take the address of". They use the same symbol for two different things which are only slightly more related to each other than they are to "bitwise and".
– Daniel H
Nov 15 '18 at 9:06
*&first
would be the same asfirst
if they were expressions and*
and&
were operators, but this is a declaration so*
and&
are not operators but parts of the type.
– molbdnilo
Nov 15 '18 at 9:19
add a comment |
This question already has an answer here:
Difference between pointer to a reference and reference to a pointer
6 answers
Following is a code to split an array. The
first output array will contain the input array entries in between the given two indices and the second
output array will contain the rest of the entries.
void splitArray(int *arr, int size, int ind1, int ind2,
int *&first, int &firstSize, int *&second, int &secondSize){
firstSize = ind2 - ind1 + 1;
secondSize = size - firstSize;
first = new int[firstSize];
second = new int[secondSize];
for (int i = 0; i < ind1; i++)
second[i] = arr[i];
for (int i = ind1; i <= ind2; i++)
first[i - ind1] = arr[i];
for (int i = ind2 + 1; i < size; i++)
second[i - firstSize] = arr[i];
}
The thing I can understand why it has parameters like int *&first
and int *&second
, arent they are same as just int first
and int second
but in this code they are used as pointers to dynamic arrays.
c++ arrays pointers
This question already has an answer here:
Difference between pointer to a reference and reference to a pointer
6 answers
Following is a code to split an array. The
first output array will contain the input array entries in between the given two indices and the second
output array will contain the rest of the entries.
void splitArray(int *arr, int size, int ind1, int ind2,
int *&first, int &firstSize, int *&second, int &secondSize){
firstSize = ind2 - ind1 + 1;
secondSize = size - firstSize;
first = new int[firstSize];
second = new int[secondSize];
for (int i = 0; i < ind1; i++)
second[i] = arr[i];
for (int i = ind1; i <= ind2; i++)
first[i - ind1] = arr[i];
for (int i = ind2 + 1; i < size; i++)
second[i - firstSize] = arr[i];
}
The thing I can understand why it has parameters like int *&first
and int *&second
, arent they are same as just int first
and int second
but in this code they are used as pointers to dynamic arrays.
This question already has an answer here:
Difference between pointer to a reference and reference to a pointer
6 answers
c++ arrays pointers
c++ arrays pointers
asked Nov 15 '18 at 8:55
Crazy_Boy53Crazy_Boy53
916
916
marked as duplicate by kabanus, DevSolar
StackExchange.ready(function() {
if (StackExchange.options.isMobile) return;
$('.dupe-hammer-message-hover:not(.hover-bound)').each(function() {
var $hover = $(this).addClass('hover-bound'),
$msg = $hover.siblings('.dupe-hammer-message');
$hover.hover(
function() {
$hover.showInfoMessage('', {
messageElement: $msg.clone().show(),
transient: false,
position: { my: 'bottom left', at: 'top center', offsetTop: -7 },
dismissable: false,
relativeToBody: true
});
},
function() {
StackExchange.helpers.removeMessages();
}
);
});
});
Nov 15 '18 at 9:05
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
marked as duplicate by kabanus, DevSolar
StackExchange.ready(function() {
if (StackExchange.options.isMobile) return;
$('.dupe-hammer-message-hover:not(.hover-bound)').each(function() {
var $hover = $(this).addClass('hover-bound'),
$msg = $hover.siblings('.dupe-hammer-message');
$hover.hover(
function() {
$hover.showInfoMessage('', {
messageElement: $msg.clone().show(),
transient: false,
position: { my: 'bottom left', at: 'top center', offsetTop: -7 },
dismissable: false,
relativeToBody: true
});
},
function() {
StackExchange.helpers.removeMessages();
}
);
});
});
Nov 15 '18 at 9:05
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
6
No, they aren't -int*&
is a reference to a pointer toint
.
– UnholySheep
Nov 15 '18 at 8:57
Here,&
means "This is a C++ reference", not "take the address of". They use the same symbol for two different things which are only slightly more related to each other than they are to "bitwise and".
– Daniel H
Nov 15 '18 at 9:06
*&first
would be the same asfirst
if they were expressions and*
and&
were operators, but this is a declaration so*
and&
are not operators but parts of the type.
– molbdnilo
Nov 15 '18 at 9:19
add a comment |
6
No, they aren't -int*&
is a reference to a pointer toint
.
– UnholySheep
Nov 15 '18 at 8:57
Here,&
means "This is a C++ reference", not "take the address of". They use the same symbol for two different things which are only slightly more related to each other than they are to "bitwise and".
– Daniel H
Nov 15 '18 at 9:06
*&first
would be the same asfirst
if they were expressions and*
and&
were operators, but this is a declaration so*
and&
are not operators but parts of the type.
– molbdnilo
Nov 15 '18 at 9:19
6
6
No, they aren't -
int*&
is a reference to a pointer to int
.– UnholySheep
Nov 15 '18 at 8:57
No, they aren't -
int*&
is a reference to a pointer to int
.– UnholySheep
Nov 15 '18 at 8:57
Here,
&
means "This is a C++ reference", not "take the address of". They use the same symbol for two different things which are only slightly more related to each other than they are to "bitwise and".– Daniel H
Nov 15 '18 at 9:06
Here,
&
means "This is a C++ reference", not "take the address of". They use the same symbol for two different things which are only slightly more related to each other than they are to "bitwise and".– Daniel H
Nov 15 '18 at 9:06
*&first
would be the same as first
if they were expressions and *
and &
were operators, but this is a declaration so *
and &
are not operators but parts of the type.– molbdnilo
Nov 15 '18 at 9:19
*&first
would be the same as first
if they were expressions and *
and &
were operators, but this is a declaration so *
and &
are not operators but parts of the type.– molbdnilo
Nov 15 '18 at 9:19
add a comment |
1 Answer
1
active
oldest
votes
T *&foo
declares a reference to a pointer to T
. Don't confuse the ampersand in declarations and definitions with the address-of operator.
References to pointers are used if the called function needs to be able to modify the value of the pointer passed to it:
void bar(int *&ptr) {
ptr = 42; // change the value of the pointer (pseudo ... 42 is most likely
*ptr = 42; // change the value of the pointee not a valid pointer value)
}
// ...
int *foo = nullptr;
bar(foo);
// foo == 42;
Hint: Read types from right to left: T *&
--> &* T
--> reference (&
) to pointer (*
) to type T
.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
T *&foo
declares a reference to a pointer to T
. Don't confuse the ampersand in declarations and definitions with the address-of operator.
References to pointers are used if the called function needs to be able to modify the value of the pointer passed to it:
void bar(int *&ptr) {
ptr = 42; // change the value of the pointer (pseudo ... 42 is most likely
*ptr = 42; // change the value of the pointee not a valid pointer value)
}
// ...
int *foo = nullptr;
bar(foo);
// foo == 42;
Hint: Read types from right to left: T *&
--> &* T
--> reference (&
) to pointer (*
) to type T
.
add a comment |
T *&foo
declares a reference to a pointer to T
. Don't confuse the ampersand in declarations and definitions with the address-of operator.
References to pointers are used if the called function needs to be able to modify the value of the pointer passed to it:
void bar(int *&ptr) {
ptr = 42; // change the value of the pointer (pseudo ... 42 is most likely
*ptr = 42; // change the value of the pointee not a valid pointer value)
}
// ...
int *foo = nullptr;
bar(foo);
// foo == 42;
Hint: Read types from right to left: T *&
--> &* T
--> reference (&
) to pointer (*
) to type T
.
add a comment |
T *&foo
declares a reference to a pointer to T
. Don't confuse the ampersand in declarations and definitions with the address-of operator.
References to pointers are used if the called function needs to be able to modify the value of the pointer passed to it:
void bar(int *&ptr) {
ptr = 42; // change the value of the pointer (pseudo ... 42 is most likely
*ptr = 42; // change the value of the pointee not a valid pointer value)
}
// ...
int *foo = nullptr;
bar(foo);
// foo == 42;
Hint: Read types from right to left: T *&
--> &* T
--> reference (&
) to pointer (*
) to type T
.
T *&foo
declares a reference to a pointer to T
. Don't confuse the ampersand in declarations and definitions with the address-of operator.
References to pointers are used if the called function needs to be able to modify the value of the pointer passed to it:
void bar(int *&ptr) {
ptr = 42; // change the value of the pointer (pseudo ... 42 is most likely
*ptr = 42; // change the value of the pointee not a valid pointer value)
}
// ...
int *foo = nullptr;
bar(foo);
// foo == 42;
Hint: Read types from right to left: T *&
--> &* T
--> reference (&
) to pointer (*
) to type T
.
edited Nov 15 '18 at 9:03
answered Nov 15 '18 at 8:58


SwordfishSwordfish
10.2k11436
10.2k11436
add a comment |
add a comment |
hJ5YGkK eX7xG
6
No, they aren't -
int*&
is a reference to a pointer toint
.– UnholySheep
Nov 15 '18 at 8:57
Here,
&
means "This is a C++ reference", not "take the address of". They use the same symbol for two different things which are only slightly more related to each other than they are to "bitwise and".– Daniel H
Nov 15 '18 at 9:06
*&first
would be the same asfirst
if they were expressions and*
and&
were operators, but this is a declaration so*
and&
are not operators but parts of the type.– molbdnilo
Nov 15 '18 at 9:19