add active/disable to child element on click
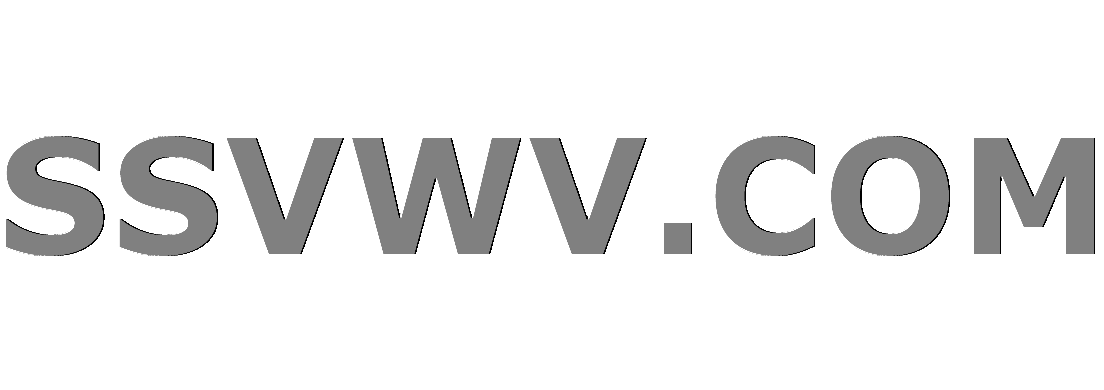
Multi tool use
I'm working on this simple selection that add 'active' class to the clicked element, and add 'disable' class to the element at opposite container.
My problem is how can move the active/disabled
class from .col-md-5ths
to their child element .num
.
kindly check code.
Thanks.
$('.numChoice > .col-md-5ths').click(function() {
if (!$(this).hasClass('disabled')) {
var otherSide = $(this).parent().hasClass('first-row') ? '.second-row' : '.first-row';
var otherElement = $(otherSide).children().removeClass('disabled').eq($(this).index());
$(this).toggleClass('active').siblings().removeClass('active');
$(this).hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass(
'disabled');
$(this).hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass(
'active');
} else {
$(this).removeClass('disabled');
var otherSide = $(this).parent().hasClass('first-row') ? '.second-row' : '.first-row';
var otherElement = $(otherSide).children().removeClass('disabled').eq($(this).index());
$(this).toggleClass('active').siblings().removeClass('active');
$(this).hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass(
'disabled');
$(this).hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass(
'active');
}
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.col-md-5ths.active{
color: green;
}
.col-md-5ths.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
javascript jquery
add a comment |
I'm working on this simple selection that add 'active' class to the clicked element, and add 'disable' class to the element at opposite container.
My problem is how can move the active/disabled
class from .col-md-5ths
to their child element .num
.
kindly check code.
Thanks.
$('.numChoice > .col-md-5ths').click(function() {
if (!$(this).hasClass('disabled')) {
var otherSide = $(this).parent().hasClass('first-row') ? '.second-row' : '.first-row';
var otherElement = $(otherSide).children().removeClass('disabled').eq($(this).index());
$(this).toggleClass('active').siblings().removeClass('active');
$(this).hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass(
'disabled');
$(this).hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass(
'active');
} else {
$(this).removeClass('disabled');
var otherSide = $(this).parent().hasClass('first-row') ? '.second-row' : '.first-row';
var otherElement = $(otherSide).children().removeClass('disabled').eq($(this).index());
$(this).toggleClass('active').siblings().removeClass('active');
$(this).hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass(
'disabled');
$(this).hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass(
'active');
}
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.col-md-5ths.active{
color: green;
}
.col-md-5ths.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
javascript jquery
1
Not sure what you mean. You want to click the .num and change the parent class? Use.closest
. Also DRY - don't repeat yourself. You could use a toggleClass to not have to repeat the code.
– mplungjan
Nov 15 '18 at 8:59
You are abusing ternaries. Just useotherElement.toggleClass(active)
– mplungjan
Nov 15 '18 at 9:01
@mplungjan yes sir I'd like to add the active/disabled class to the.num
– user123
Nov 15 '18 at 9:03
add a comment |
I'm working on this simple selection that add 'active' class to the clicked element, and add 'disable' class to the element at opposite container.
My problem is how can move the active/disabled
class from .col-md-5ths
to their child element .num
.
kindly check code.
Thanks.
$('.numChoice > .col-md-5ths').click(function() {
if (!$(this).hasClass('disabled')) {
var otherSide = $(this).parent().hasClass('first-row') ? '.second-row' : '.first-row';
var otherElement = $(otherSide).children().removeClass('disabled').eq($(this).index());
$(this).toggleClass('active').siblings().removeClass('active');
$(this).hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass(
'disabled');
$(this).hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass(
'active');
} else {
$(this).removeClass('disabled');
var otherSide = $(this).parent().hasClass('first-row') ? '.second-row' : '.first-row';
var otherElement = $(otherSide).children().removeClass('disabled').eq($(this).index());
$(this).toggleClass('active').siblings().removeClass('active');
$(this).hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass(
'disabled');
$(this).hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass(
'active');
}
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.col-md-5ths.active{
color: green;
}
.col-md-5ths.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
javascript jquery
I'm working on this simple selection that add 'active' class to the clicked element, and add 'disable' class to the element at opposite container.
My problem is how can move the active/disabled
class from .col-md-5ths
to their child element .num
.
kindly check code.
Thanks.
$('.numChoice > .col-md-5ths').click(function() {
if (!$(this).hasClass('disabled')) {
var otherSide = $(this).parent().hasClass('first-row') ? '.second-row' : '.first-row';
var otherElement = $(otherSide).children().removeClass('disabled').eq($(this).index());
$(this).toggleClass('active').siblings().removeClass('active');
$(this).hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass(
'disabled');
$(this).hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass(
'active');
} else {
$(this).removeClass('disabled');
var otherSide = $(this).parent().hasClass('first-row') ? '.second-row' : '.first-row';
var otherElement = $(otherSide).children().removeClass('disabled').eq($(this).index());
$(this).toggleClass('active').siblings().removeClass('active');
$(this).hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass(
'disabled');
$(this).hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass(
'active');
}
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.col-md-5ths.active{
color: green;
}
.col-md-5ths.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
$('.numChoice > .col-md-5ths').click(function() {
if (!$(this).hasClass('disabled')) {
var otherSide = $(this).parent().hasClass('first-row') ? '.second-row' : '.first-row';
var otherElement = $(otherSide).children().removeClass('disabled').eq($(this).index());
$(this).toggleClass('active').siblings().removeClass('active');
$(this).hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass(
'disabled');
$(this).hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass(
'active');
} else {
$(this).removeClass('disabled');
var otherSide = $(this).parent().hasClass('first-row') ? '.second-row' : '.first-row';
var otherElement = $(otherSide).children().removeClass('disabled').eq($(this).index());
$(this).toggleClass('active').siblings().removeClass('active');
$(this).hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass(
'disabled');
$(this).hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass(
'active');
}
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.col-md-5ths.active{
color: green;
}
.col-md-5ths.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
$('.numChoice > .col-md-5ths').click(function() {
if (!$(this).hasClass('disabled')) {
var otherSide = $(this).parent().hasClass('first-row') ? '.second-row' : '.first-row';
var otherElement = $(otherSide).children().removeClass('disabled').eq($(this).index());
$(this).toggleClass('active').siblings().removeClass('active');
$(this).hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass(
'disabled');
$(this).hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass(
'active');
} else {
$(this).removeClass('disabled');
var otherSide = $(this).parent().hasClass('first-row') ? '.second-row' : '.first-row';
var otherElement = $(otherSide).children().removeClass('disabled').eq($(this).index());
$(this).toggleClass('active').siblings().removeClass('active');
$(this).hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass(
'disabled');
$(this).hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass(
'active');
}
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.col-md-5ths.active{
color: green;
}
.col-md-5ths.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
javascript jquery
javascript jquery
edited Nov 15 '18 at 9:07
user123
asked Nov 15 '18 at 8:55
user123user123
9614
9614
1
Not sure what you mean. You want to click the .num and change the parent class? Use.closest
. Also DRY - don't repeat yourself. You could use a toggleClass to not have to repeat the code.
– mplungjan
Nov 15 '18 at 8:59
You are abusing ternaries. Just useotherElement.toggleClass(active)
– mplungjan
Nov 15 '18 at 9:01
@mplungjan yes sir I'd like to add the active/disabled class to the.num
– user123
Nov 15 '18 at 9:03
add a comment |
1
Not sure what you mean. You want to click the .num and change the parent class? Use.closest
. Also DRY - don't repeat yourself. You could use a toggleClass to not have to repeat the code.
– mplungjan
Nov 15 '18 at 8:59
You are abusing ternaries. Just useotherElement.toggleClass(active)
– mplungjan
Nov 15 '18 at 9:01
@mplungjan yes sir I'd like to add the active/disabled class to the.num
– user123
Nov 15 '18 at 9:03
1
1
Not sure what you mean. You want to click the .num and change the parent class? Use
.closest
. Also DRY - don't repeat yourself. You could use a toggleClass to not have to repeat the code.– mplungjan
Nov 15 '18 at 8:59
Not sure what you mean. You want to click the .num and change the parent class? Use
.closest
. Also DRY - don't repeat yourself. You could use a toggleClass to not have to repeat the code.– mplungjan
Nov 15 '18 at 8:59
You are abusing ternaries. Just use
otherElement.toggleClass(active)
– mplungjan
Nov 15 '18 at 9:01
You are abusing ternaries. Just use
otherElement.toggleClass(active)
– mplungjan
Nov 15 '18 at 9:01
@mplungjan yes sir I'd like to add the active/disabled class to the
.num
– user123
Nov 15 '18 at 9:03
@mplungjan yes sir I'd like to add the active/disabled class to the
.num
– user123
Nov 15 '18 at 9:03
add a comment |
4 Answers
4
active
oldest
votes
Here is a shorter version
$('.numChoice > .col-md-5ths > .num').on("click", function(e) {
e.preventDefault(); // stop the link
var $this = $(this);
if ($this.is(".disabled")) return; // assuming you cannot click on a disabled
$this.toggleClass('active');
var act = $this.is(".active");
var idx = $this.parent().index();
var $otherSide = $this.closest(".numChoice").siblings(); // plural but only one
var $otherElement = $otherSide.find(".num").eq(idx);
$otherElement.toggleClass('disabled', act);
});
.numChoice {
display: block;
}
.num {
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.num.active {
color: green;
}
.num.disabled {
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
the first version is correct sir
– user123
Nov 15 '18 at 9:30
Please see update - I think this is a better version
– mplungjan
Nov 16 '18 at 6:20
ok sir, thank you
– user123
Nov 16 '18 at 6:21
add a comment |
You can can achieve that in a more simplified way like the following:
$('.numChoice .num').click(function() {
$('.num').removeClass('active').removeClass('disabled');
var parent = $('.first-row').has(this).length == 0?'.first-row' : '.second-row';
var idx = $(this).parent().index();
$(this).toggleClass('active');
$(parent+' .num').eq(idx).toggleClass('disabled');
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.active{
color: green;
}
.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
add a comment |
You can do it like this...
$('.numChoice > .col-md-5ths').click(function(index,el) {
var thisind = $(this).index() + 1;
if($(this).parent().hasClass('first-row')){
$(this).addClass('active');
$(this).siblings().removeClass('active');
$('.second-row .col-md-5ths').removeClass('disabled');
$('.second-row .col-md-5ths:nth-child('+thisind+')').addClass('disabled');
}
else {
$(this).addClass('disabled');
$(this).siblings().removeClass('disabled');
$('.first-row .col-md-5ths:nth-child('+thisind+')').addClass('disabled');
}
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.col-md-5ths.active{
color: green;
}
.col-md-5ths.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
add a comment |
I created a sample fiddle for you
$('.numChoice > .col-md-5ths').click(function() {
var otherSide = $(this).parent().hasClass('first-row') ? '.second-row' : '.first-row';
var otherElement = $(otherSide).children().removeClass('disabled').eq($(this).index());
var $thisNum = $(this).children();
if (!$(this).hasClass('disabled')) {
$thisNum.toggleClass('active').siblings().removeClass('active');
$thisNum.hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass('disabled');
$thisNum.hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass('active');
} else {
$(this).removeClass('disabled');
$thisNum.toggleClass('active').siblings().removeClass('active');
$thisNum.hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass('disabled');
$thisNum.hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass('active');
}
});
thank you sir, almost there, when I click on the element it adds theactive
class to.num
, this is correct. On the other container, thedisabled
class should be on the.num
class too.
– user123
Nov 15 '18 at 9:21
DRY: Don't repeat yourself
– mplungjan
Nov 15 '18 at 9:41
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53315612%2fadd-active-disable-to-child-element-on-click%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
Here is a shorter version
$('.numChoice > .col-md-5ths > .num').on("click", function(e) {
e.preventDefault(); // stop the link
var $this = $(this);
if ($this.is(".disabled")) return; // assuming you cannot click on a disabled
$this.toggleClass('active');
var act = $this.is(".active");
var idx = $this.parent().index();
var $otherSide = $this.closest(".numChoice").siblings(); // plural but only one
var $otherElement = $otherSide.find(".num").eq(idx);
$otherElement.toggleClass('disabled', act);
});
.numChoice {
display: block;
}
.num {
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.num.active {
color: green;
}
.num.disabled {
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
the first version is correct sir
– user123
Nov 15 '18 at 9:30
Please see update - I think this is a better version
– mplungjan
Nov 16 '18 at 6:20
ok sir, thank you
– user123
Nov 16 '18 at 6:21
add a comment |
Here is a shorter version
$('.numChoice > .col-md-5ths > .num').on("click", function(e) {
e.preventDefault(); // stop the link
var $this = $(this);
if ($this.is(".disabled")) return; // assuming you cannot click on a disabled
$this.toggleClass('active');
var act = $this.is(".active");
var idx = $this.parent().index();
var $otherSide = $this.closest(".numChoice").siblings(); // plural but only one
var $otherElement = $otherSide.find(".num").eq(idx);
$otherElement.toggleClass('disabled', act);
});
.numChoice {
display: block;
}
.num {
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.num.active {
color: green;
}
.num.disabled {
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
the first version is correct sir
– user123
Nov 15 '18 at 9:30
Please see update - I think this is a better version
– mplungjan
Nov 16 '18 at 6:20
ok sir, thank you
– user123
Nov 16 '18 at 6:21
add a comment |
Here is a shorter version
$('.numChoice > .col-md-5ths > .num').on("click", function(e) {
e.preventDefault(); // stop the link
var $this = $(this);
if ($this.is(".disabled")) return; // assuming you cannot click on a disabled
$this.toggleClass('active');
var act = $this.is(".active");
var idx = $this.parent().index();
var $otherSide = $this.closest(".numChoice").siblings(); // plural but only one
var $otherElement = $otherSide.find(".num").eq(idx);
$otherElement.toggleClass('disabled', act);
});
.numChoice {
display: block;
}
.num {
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.num.active {
color: green;
}
.num.disabled {
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
Here is a shorter version
$('.numChoice > .col-md-5ths > .num').on("click", function(e) {
e.preventDefault(); // stop the link
var $this = $(this);
if ($this.is(".disabled")) return; // assuming you cannot click on a disabled
$this.toggleClass('active');
var act = $this.is(".active");
var idx = $this.parent().index();
var $otherSide = $this.closest(".numChoice").siblings(); // plural but only one
var $otherElement = $otherSide.find(".num").eq(idx);
$otherElement.toggleClass('disabled', act);
});
.numChoice {
display: block;
}
.num {
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.num.active {
color: green;
}
.num.disabled {
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
$('.numChoice > .col-md-5ths > .num').on("click", function(e) {
e.preventDefault(); // stop the link
var $this = $(this);
if ($this.is(".disabled")) return; // assuming you cannot click on a disabled
$this.toggleClass('active');
var act = $this.is(".active");
var idx = $this.parent().index();
var $otherSide = $this.closest(".numChoice").siblings(); // plural but only one
var $otherElement = $otherSide.find(".num").eq(idx);
$otherElement.toggleClass('disabled', act);
});
.numChoice {
display: block;
}
.num {
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.num.active {
color: green;
}
.num.disabled {
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
$('.numChoice > .col-md-5ths > .num').on("click", function(e) {
e.preventDefault(); // stop the link
var $this = $(this);
if ($this.is(".disabled")) return; // assuming you cannot click on a disabled
$this.toggleClass('active');
var act = $this.is(".active");
var idx = $this.parent().index();
var $otherSide = $this.closest(".numChoice").siblings(); // plural but only one
var $otherElement = $otherSide.find(".num").eq(idx);
$otherElement.toggleClass('disabled', act);
});
.numChoice {
display: block;
}
.num {
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.num.active {
color: green;
}
.num.disabled {
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
edited Nov 16 '18 at 6:19
answered Nov 15 '18 at 9:25
mplungjanmplungjan
88.8k22126182
88.8k22126182
the first version is correct sir
– user123
Nov 15 '18 at 9:30
Please see update - I think this is a better version
– mplungjan
Nov 16 '18 at 6:20
ok sir, thank you
– user123
Nov 16 '18 at 6:21
add a comment |
the first version is correct sir
– user123
Nov 15 '18 at 9:30
Please see update - I think this is a better version
– mplungjan
Nov 16 '18 at 6:20
ok sir, thank you
– user123
Nov 16 '18 at 6:21
the first version is correct sir
– user123
Nov 15 '18 at 9:30
the first version is correct sir
– user123
Nov 15 '18 at 9:30
Please see update - I think this is a better version
– mplungjan
Nov 16 '18 at 6:20
Please see update - I think this is a better version
– mplungjan
Nov 16 '18 at 6:20
ok sir, thank you
– user123
Nov 16 '18 at 6:21
ok sir, thank you
– user123
Nov 16 '18 at 6:21
add a comment |
You can can achieve that in a more simplified way like the following:
$('.numChoice .num').click(function() {
$('.num').removeClass('active').removeClass('disabled');
var parent = $('.first-row').has(this).length == 0?'.first-row' : '.second-row';
var idx = $(this).parent().index();
$(this).toggleClass('active');
$(parent+' .num').eq(idx).toggleClass('disabled');
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.active{
color: green;
}
.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
add a comment |
You can can achieve that in a more simplified way like the following:
$('.numChoice .num').click(function() {
$('.num').removeClass('active').removeClass('disabled');
var parent = $('.first-row').has(this).length == 0?'.first-row' : '.second-row';
var idx = $(this).parent().index();
$(this).toggleClass('active');
$(parent+' .num').eq(idx).toggleClass('disabled');
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.active{
color: green;
}
.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
add a comment |
You can can achieve that in a more simplified way like the following:
$('.numChoice .num').click(function() {
$('.num').removeClass('active').removeClass('disabled');
var parent = $('.first-row').has(this).length == 0?'.first-row' : '.second-row';
var idx = $(this).parent().index();
$(this).toggleClass('active');
$(parent+' .num').eq(idx).toggleClass('disabled');
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.active{
color: green;
}
.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
You can can achieve that in a more simplified way like the following:
$('.numChoice .num').click(function() {
$('.num').removeClass('active').removeClass('disabled');
var parent = $('.first-row').has(this).length == 0?'.first-row' : '.second-row';
var idx = $(this).parent().index();
$(this).toggleClass('active');
$(parent+' .num').eq(idx).toggleClass('disabled');
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.active{
color: green;
}
.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
$('.numChoice .num').click(function() {
$('.num').removeClass('active').removeClass('disabled');
var parent = $('.first-row').has(this).length == 0?'.first-row' : '.second-row';
var idx = $(this).parent().index();
$(this).toggleClass('active');
$(parent+' .num').eq(idx).toggleClass('disabled');
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.active{
color: green;
}
.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
$('.numChoice .num').click(function() {
$('.num').removeClass('active').removeClass('disabled');
var parent = $('.first-row').has(this).length == 0?'.first-row' : '.second-row';
var idx = $(this).parent().index();
$(this).toggleClass('active');
$(parent+' .num').eq(idx).toggleClass('disabled');
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.active{
color: green;
}
.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
edited Nov 15 '18 at 10:02
answered Nov 15 '18 at 9:44
MamunMamun
28.3k71830
28.3k71830
add a comment |
add a comment |
You can do it like this...
$('.numChoice > .col-md-5ths').click(function(index,el) {
var thisind = $(this).index() + 1;
if($(this).parent().hasClass('first-row')){
$(this).addClass('active');
$(this).siblings().removeClass('active');
$('.second-row .col-md-5ths').removeClass('disabled');
$('.second-row .col-md-5ths:nth-child('+thisind+')').addClass('disabled');
}
else {
$(this).addClass('disabled');
$(this).siblings().removeClass('disabled');
$('.first-row .col-md-5ths:nth-child('+thisind+')').addClass('disabled');
}
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.col-md-5ths.active{
color: green;
}
.col-md-5ths.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
add a comment |
You can do it like this...
$('.numChoice > .col-md-5ths').click(function(index,el) {
var thisind = $(this).index() + 1;
if($(this).parent().hasClass('first-row')){
$(this).addClass('active');
$(this).siblings().removeClass('active');
$('.second-row .col-md-5ths').removeClass('disabled');
$('.second-row .col-md-5ths:nth-child('+thisind+')').addClass('disabled');
}
else {
$(this).addClass('disabled');
$(this).siblings().removeClass('disabled');
$('.first-row .col-md-5ths:nth-child('+thisind+')').addClass('disabled');
}
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.col-md-5ths.active{
color: green;
}
.col-md-5ths.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
add a comment |
You can do it like this...
$('.numChoice > .col-md-5ths').click(function(index,el) {
var thisind = $(this).index() + 1;
if($(this).parent().hasClass('first-row')){
$(this).addClass('active');
$(this).siblings().removeClass('active');
$('.second-row .col-md-5ths').removeClass('disabled');
$('.second-row .col-md-5ths:nth-child('+thisind+')').addClass('disabled');
}
else {
$(this).addClass('disabled');
$(this).siblings().removeClass('disabled');
$('.first-row .col-md-5ths:nth-child('+thisind+')').addClass('disabled');
}
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.col-md-5ths.active{
color: green;
}
.col-md-5ths.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
You can do it like this...
$('.numChoice > .col-md-5ths').click(function(index,el) {
var thisind = $(this).index() + 1;
if($(this).parent().hasClass('first-row')){
$(this).addClass('active');
$(this).siblings().removeClass('active');
$('.second-row .col-md-5ths').removeClass('disabled');
$('.second-row .col-md-5ths:nth-child('+thisind+')').addClass('disabled');
}
else {
$(this).addClass('disabled');
$(this).siblings().removeClass('disabled');
$('.first-row .col-md-5ths:nth-child('+thisind+')').addClass('disabled');
}
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.col-md-5ths.active{
color: green;
}
.col-md-5ths.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
$('.numChoice > .col-md-5ths').click(function(index,el) {
var thisind = $(this).index() + 1;
if($(this).parent().hasClass('first-row')){
$(this).addClass('active');
$(this).siblings().removeClass('active');
$('.second-row .col-md-5ths').removeClass('disabled');
$('.second-row .col-md-5ths:nth-child('+thisind+')').addClass('disabled');
}
else {
$(this).addClass('disabled');
$(this).siblings().removeClass('disabled');
$('.first-row .col-md-5ths:nth-child('+thisind+')').addClass('disabled');
}
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.col-md-5ths.active{
color: green;
}
.col-md-5ths.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
$('.numChoice > .col-md-5ths').click(function(index,el) {
var thisind = $(this).index() + 1;
if($(this).parent().hasClass('first-row')){
$(this).addClass('active');
$(this).siblings().removeClass('active');
$('.second-row .col-md-5ths').removeClass('disabled');
$('.second-row .col-md-5ths:nth-child('+thisind+')').addClass('disabled');
}
else {
$(this).addClass('disabled');
$(this).siblings().removeClass('disabled');
$('.first-row .col-md-5ths:nth-child('+thisind+')').addClass('disabled');
}
});
.numChoice{
display: block;
}
.num{
width: 20px;
height: 20px;
background-color: #DDD;
text-align: center;
float: left;
cursor: pointer;
}
.col-md-5ths.active{
color: green;
}
.col-md-5ths.disabled{
color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="container">
<div class="numChoice first-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
<br><br>
<div class="numChoice second-row">
<div class="col-md-5ths">
<div class="num">1</div>
</div>
<div class="col-md-5ths">
<div class="num">2</div>
</div>
<div class="col-md-5ths">
<div class="num">3</div>
</div>
<div class="col-md-5ths">
<div class="num">4</div>
</div>
<div class="col-md-5ths">
<div class="num">5</div>
</div>
</div>
</div>
answered Nov 15 '18 at 9:10


ElusiveCoderElusiveCoder
1,2001218
1,2001218
add a comment |
add a comment |
I created a sample fiddle for you
$('.numChoice > .col-md-5ths').click(function() {
var otherSide = $(this).parent().hasClass('first-row') ? '.second-row' : '.first-row';
var otherElement = $(otherSide).children().removeClass('disabled').eq($(this).index());
var $thisNum = $(this).children();
if (!$(this).hasClass('disabled')) {
$thisNum.toggleClass('active').siblings().removeClass('active');
$thisNum.hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass('disabled');
$thisNum.hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass('active');
} else {
$(this).removeClass('disabled');
$thisNum.toggleClass('active').siblings().removeClass('active');
$thisNum.hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass('disabled');
$thisNum.hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass('active');
}
});
thank you sir, almost there, when I click on the element it adds theactive
class to.num
, this is correct. On the other container, thedisabled
class should be on the.num
class too.
– user123
Nov 15 '18 at 9:21
DRY: Don't repeat yourself
– mplungjan
Nov 15 '18 at 9:41
add a comment |
I created a sample fiddle for you
$('.numChoice > .col-md-5ths').click(function() {
var otherSide = $(this).parent().hasClass('first-row') ? '.second-row' : '.first-row';
var otherElement = $(otherSide).children().removeClass('disabled').eq($(this).index());
var $thisNum = $(this).children();
if (!$(this).hasClass('disabled')) {
$thisNum.toggleClass('active').siblings().removeClass('active');
$thisNum.hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass('disabled');
$thisNum.hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass('active');
} else {
$(this).removeClass('disabled');
$thisNum.toggleClass('active').siblings().removeClass('active');
$thisNum.hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass('disabled');
$thisNum.hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass('active');
}
});
thank you sir, almost there, when I click on the element it adds theactive
class to.num
, this is correct. On the other container, thedisabled
class should be on the.num
class too.
– user123
Nov 15 '18 at 9:21
DRY: Don't repeat yourself
– mplungjan
Nov 15 '18 at 9:41
add a comment |
I created a sample fiddle for you
$('.numChoice > .col-md-5ths').click(function() {
var otherSide = $(this).parent().hasClass('first-row') ? '.second-row' : '.first-row';
var otherElement = $(otherSide).children().removeClass('disabled').eq($(this).index());
var $thisNum = $(this).children();
if (!$(this).hasClass('disabled')) {
$thisNum.toggleClass('active').siblings().removeClass('active');
$thisNum.hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass('disabled');
$thisNum.hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass('active');
} else {
$(this).removeClass('disabled');
$thisNum.toggleClass('active').siblings().removeClass('active');
$thisNum.hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass('disabled');
$thisNum.hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass('active');
}
});
I created a sample fiddle for you
$('.numChoice > .col-md-5ths').click(function() {
var otherSide = $(this).parent().hasClass('first-row') ? '.second-row' : '.first-row';
var otherElement = $(otherSide).children().removeClass('disabled').eq($(this).index());
var $thisNum = $(this).children();
if (!$(this).hasClass('disabled')) {
$thisNum.toggleClass('active').siblings().removeClass('active');
$thisNum.hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass('disabled');
$thisNum.hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass('active');
} else {
$(this).removeClass('disabled');
$thisNum.toggleClass('active').siblings().removeClass('active');
$thisNum.hasClass('active') ? otherElement.addClass('disabled') : otherElement.removeClass('disabled');
$thisNum.hasClass('disabled') ? otherElement.removeClass('active') : otherElement.removeClass('active');
}
});
answered Nov 15 '18 at 9:13
dganencodganenco
2217
2217
thank you sir, almost there, when I click on the element it adds theactive
class to.num
, this is correct. On the other container, thedisabled
class should be on the.num
class too.
– user123
Nov 15 '18 at 9:21
DRY: Don't repeat yourself
– mplungjan
Nov 15 '18 at 9:41
add a comment |
thank you sir, almost there, when I click on the element it adds theactive
class to.num
, this is correct. On the other container, thedisabled
class should be on the.num
class too.
– user123
Nov 15 '18 at 9:21
DRY: Don't repeat yourself
– mplungjan
Nov 15 '18 at 9:41
thank you sir, almost there, when I click on the element it adds the
active
class to .num
, this is correct. On the other container, the disabled
class should be on the .num
class too.– user123
Nov 15 '18 at 9:21
thank you sir, almost there, when I click on the element it adds the
active
class to .num
, this is correct. On the other container, the disabled
class should be on the .num
class too.– user123
Nov 15 '18 at 9:21
DRY: Don't repeat yourself
– mplungjan
Nov 15 '18 at 9:41
DRY: Don't repeat yourself
– mplungjan
Nov 15 '18 at 9:41
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53315612%2fadd-active-disable-to-child-element-on-click%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
jpQOVjrq9IxAZrXLyS85TzlmmiqN,rIDCvQer0Xvh2uW5n 4Y
1
Not sure what you mean. You want to click the .num and change the parent class? Use
.closest
. Also DRY - don't repeat yourself. You could use a toggleClass to not have to repeat the code.– mplungjan
Nov 15 '18 at 8:59
You are abusing ternaries. Just use
otherElement.toggleClass(active)
– mplungjan
Nov 15 '18 at 9:01
@mplungjan yes sir I'd like to add the active/disabled class to the
.num
– user123
Nov 15 '18 at 9:03