Child value = null
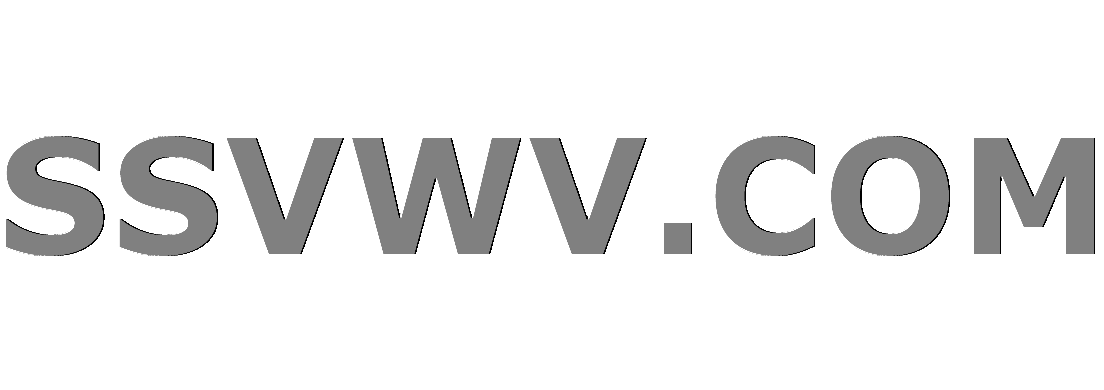
Multi tool use
I have a database that looks like this:
database
|
places
|
user1
| |___indoors: "yes"
| |___outdoors: "yes"
|
user2
|___indoors: "yes"
|___outdoors: "no"
When I do the following, I get all users places information as a single object (converted to String) with no problems:
ValueEventListener placesListener = new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
String locations = dataSnapshot.toString(); // I get the entire tree as String
}
}
//this is how I set the listener to changes
DatabaseReference database = FirebaseDatabase.getInstance().getReference();
DatabaseReference reference = database.child("places");
reference.addValueEventListener(placesListener);
But, when I want only the indoors
children, I get DataSnapshot {key = indoors, value = null}
.
This is how I try to get only the indoors
keys and values (almost the same, only adding extra .child("indoors")
:
ValueEventListener placesListener = new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
String indoors = dataSnapshot.child("indoors").toString(); // I get value = null
}
}
// listener remains the same

add a comment |
I have a database that looks like this:
database
|
places
|
user1
| |___indoors: "yes"
| |___outdoors: "yes"
|
user2
|___indoors: "yes"
|___outdoors: "no"
When I do the following, I get all users places information as a single object (converted to String) with no problems:
ValueEventListener placesListener = new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
String locations = dataSnapshot.toString(); // I get the entire tree as String
}
}
//this is how I set the listener to changes
DatabaseReference database = FirebaseDatabase.getInstance().getReference();
DatabaseReference reference = database.child("places");
reference.addValueEventListener(placesListener);
But, when I want only the indoors
children, I get DataSnapshot {key = indoors, value = null}
.
This is how I try to get only the indoors
keys and values (almost the same, only adding extra .child("indoors")
:
ValueEventListener placesListener = new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
String indoors = dataSnapshot.child("indoors").toString(); // I get value = null
}
}
// listener remains the same

add a comment |
I have a database that looks like this:
database
|
places
|
user1
| |___indoors: "yes"
| |___outdoors: "yes"
|
user2
|___indoors: "yes"
|___outdoors: "no"
When I do the following, I get all users places information as a single object (converted to String) with no problems:
ValueEventListener placesListener = new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
String locations = dataSnapshot.toString(); // I get the entire tree as String
}
}
//this is how I set the listener to changes
DatabaseReference database = FirebaseDatabase.getInstance().getReference();
DatabaseReference reference = database.child("places");
reference.addValueEventListener(placesListener);
But, when I want only the indoors
children, I get DataSnapshot {key = indoors, value = null}
.
This is how I try to get only the indoors
keys and values (almost the same, only adding extra .child("indoors")
:
ValueEventListener placesListener = new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
String indoors = dataSnapshot.child("indoors").toString(); // I get value = null
}
}
// listener remains the same

I have a database that looks like this:
database
|
places
|
user1
| |___indoors: "yes"
| |___outdoors: "yes"
|
user2
|___indoors: "yes"
|___outdoors: "no"
When I do the following, I get all users places information as a single object (converted to String) with no problems:
ValueEventListener placesListener = new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
String locations = dataSnapshot.toString(); // I get the entire tree as String
}
}
//this is how I set the listener to changes
DatabaseReference database = FirebaseDatabase.getInstance().getReference();
DatabaseReference reference = database.child("places");
reference.addValueEventListener(placesListener);
But, when I want only the indoors
children, I get DataSnapshot {key = indoors, value = null}
.
This is how I try to get only the indoors
keys and values (almost the same, only adding extra .child("indoors")
:
ValueEventListener placesListener = new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
String indoors = dataSnapshot.child("indoors").toString(); // I get value = null
}
}
// listener remains the same


edited Nov 15 '18 at 9:54


Grimthorr
4,45442235
4,45442235
asked Nov 15 '18 at 9:03
TTnoteTTnote
969
969
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
The data contained in a DataSnapshot
is relative, like a JSON tree. From the documentation on DataSnapshot#child()
:
Get a DataSnapshot for the location at the specified relative path. The relative path can either be a simple child key (e.g. 'fred') or a deeper slash-separated path (e.g. 'fred/name/first'). If the child location has no data, an empty DataSnapshot is returned.
In your example, there is no child named indoors
directly under the places
node. Instead, you have 2 children named user1
and user2
which each have a child named indoors
. Therefore, in order to access one of the indoors
children, you'll need to do something like:
dataSnapshot.child("user1").child("indoors").toString();
Alternatively, you can iterate through the children using:
for (DataSnapshot childSnapshot : dataSnapshot.getChildren()) {
Log.d(childSnapshot.getKey() + ": indoors=" + childSnapshot.child("indoors").toString());
}
Thank you. By the way, is there a way to retrieve data from the Firebase database NOT in real time? I just want a specific value but get it now, and not in real time
– TTnote
Nov 15 '18 at 9:56
Glad to help. Do you mean synchronously? Not exactly, as this isn't a supported feature of Firebase, but there are options, see Is it possible to synchronously load data from Firebase? for more details.
– Grimthorr
Nov 15 '18 at 9:59
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53315757%2fchild-value-null%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The data contained in a DataSnapshot
is relative, like a JSON tree. From the documentation on DataSnapshot#child()
:
Get a DataSnapshot for the location at the specified relative path. The relative path can either be a simple child key (e.g. 'fred') or a deeper slash-separated path (e.g. 'fred/name/first'). If the child location has no data, an empty DataSnapshot is returned.
In your example, there is no child named indoors
directly under the places
node. Instead, you have 2 children named user1
and user2
which each have a child named indoors
. Therefore, in order to access one of the indoors
children, you'll need to do something like:
dataSnapshot.child("user1").child("indoors").toString();
Alternatively, you can iterate through the children using:
for (DataSnapshot childSnapshot : dataSnapshot.getChildren()) {
Log.d(childSnapshot.getKey() + ": indoors=" + childSnapshot.child("indoors").toString());
}
Thank you. By the way, is there a way to retrieve data from the Firebase database NOT in real time? I just want a specific value but get it now, and not in real time
– TTnote
Nov 15 '18 at 9:56
Glad to help. Do you mean synchronously? Not exactly, as this isn't a supported feature of Firebase, but there are options, see Is it possible to synchronously load data from Firebase? for more details.
– Grimthorr
Nov 15 '18 at 9:59
add a comment |
The data contained in a DataSnapshot
is relative, like a JSON tree. From the documentation on DataSnapshot#child()
:
Get a DataSnapshot for the location at the specified relative path. The relative path can either be a simple child key (e.g. 'fred') or a deeper slash-separated path (e.g. 'fred/name/first'). If the child location has no data, an empty DataSnapshot is returned.
In your example, there is no child named indoors
directly under the places
node. Instead, you have 2 children named user1
and user2
which each have a child named indoors
. Therefore, in order to access one of the indoors
children, you'll need to do something like:
dataSnapshot.child("user1").child("indoors").toString();
Alternatively, you can iterate through the children using:
for (DataSnapshot childSnapshot : dataSnapshot.getChildren()) {
Log.d(childSnapshot.getKey() + ": indoors=" + childSnapshot.child("indoors").toString());
}
Thank you. By the way, is there a way to retrieve data from the Firebase database NOT in real time? I just want a specific value but get it now, and not in real time
– TTnote
Nov 15 '18 at 9:56
Glad to help. Do you mean synchronously? Not exactly, as this isn't a supported feature of Firebase, but there are options, see Is it possible to synchronously load data from Firebase? for more details.
– Grimthorr
Nov 15 '18 at 9:59
add a comment |
The data contained in a DataSnapshot
is relative, like a JSON tree. From the documentation on DataSnapshot#child()
:
Get a DataSnapshot for the location at the specified relative path. The relative path can either be a simple child key (e.g. 'fred') or a deeper slash-separated path (e.g. 'fred/name/first'). If the child location has no data, an empty DataSnapshot is returned.
In your example, there is no child named indoors
directly under the places
node. Instead, you have 2 children named user1
and user2
which each have a child named indoors
. Therefore, in order to access one of the indoors
children, you'll need to do something like:
dataSnapshot.child("user1").child("indoors").toString();
Alternatively, you can iterate through the children using:
for (DataSnapshot childSnapshot : dataSnapshot.getChildren()) {
Log.d(childSnapshot.getKey() + ": indoors=" + childSnapshot.child("indoors").toString());
}
The data contained in a DataSnapshot
is relative, like a JSON tree. From the documentation on DataSnapshot#child()
:
Get a DataSnapshot for the location at the specified relative path. The relative path can either be a simple child key (e.g. 'fred') or a deeper slash-separated path (e.g. 'fred/name/first'). If the child location has no data, an empty DataSnapshot is returned.
In your example, there is no child named indoors
directly under the places
node. Instead, you have 2 children named user1
and user2
which each have a child named indoors
. Therefore, in order to access one of the indoors
children, you'll need to do something like:
dataSnapshot.child("user1").child("indoors").toString();
Alternatively, you can iterate through the children using:
for (DataSnapshot childSnapshot : dataSnapshot.getChildren()) {
Log.d(childSnapshot.getKey() + ": indoors=" + childSnapshot.child("indoors").toString());
}
answered Nov 15 '18 at 9:51


GrimthorrGrimthorr
4,45442235
4,45442235
Thank you. By the way, is there a way to retrieve data from the Firebase database NOT in real time? I just want a specific value but get it now, and not in real time
– TTnote
Nov 15 '18 at 9:56
Glad to help. Do you mean synchronously? Not exactly, as this isn't a supported feature of Firebase, but there are options, see Is it possible to synchronously load data from Firebase? for more details.
– Grimthorr
Nov 15 '18 at 9:59
add a comment |
Thank you. By the way, is there a way to retrieve data from the Firebase database NOT in real time? I just want a specific value but get it now, and not in real time
– TTnote
Nov 15 '18 at 9:56
Glad to help. Do you mean synchronously? Not exactly, as this isn't a supported feature of Firebase, but there are options, see Is it possible to synchronously load data from Firebase? for more details.
– Grimthorr
Nov 15 '18 at 9:59
Thank you. By the way, is there a way to retrieve data from the Firebase database NOT in real time? I just want a specific value but get it now, and not in real time
– TTnote
Nov 15 '18 at 9:56
Thank you. By the way, is there a way to retrieve data from the Firebase database NOT in real time? I just want a specific value but get it now, and not in real time
– TTnote
Nov 15 '18 at 9:56
Glad to help. Do you mean synchronously? Not exactly, as this isn't a supported feature of Firebase, but there are options, see Is it possible to synchronously load data from Firebase? for more details.
– Grimthorr
Nov 15 '18 at 9:59
Glad to help. Do you mean synchronously? Not exactly, as this isn't a supported feature of Firebase, but there are options, see Is it possible to synchronously load data from Firebase? for more details.
– Grimthorr
Nov 15 '18 at 9:59
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53315757%2fchild-value-null%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6oK8ItO2uC,NJS qFbeU4,4zef13MjgyRUakAVe,MOCxsHH 8