Sorting names by their high scores
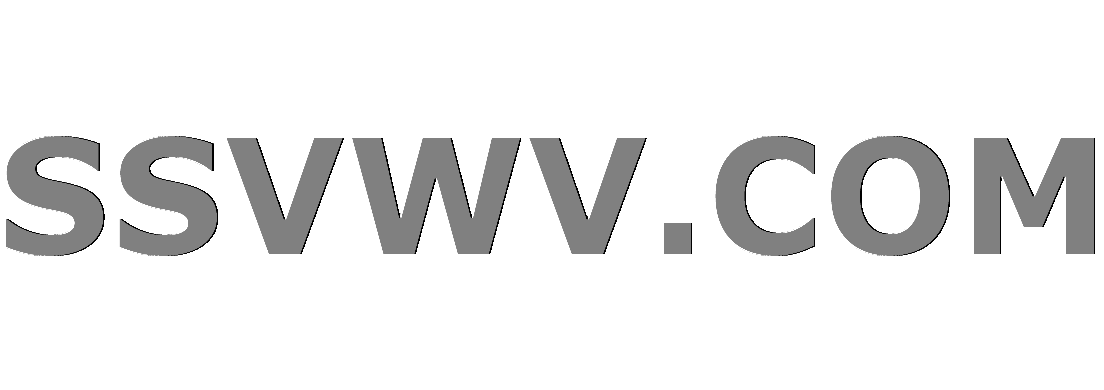
Multi tool use
I want to sort a list of names by their score.
What I have so far is
file = open("scores.txt", 'r')
for line in file:
name = line.strip()
print(name)
file.close()
I'm unsure how to sort them.
Here's the file contents:
Matthew, 13
Luke, 6
John, 3
Bobba, 4
What I want the output to be:
John 3
Bobba 4
Luke 6
Matthew 13
Can anyone help?
python sorting python-3.x
add a comment |
I want to sort a list of names by their score.
What I have so far is
file = open("scores.txt", 'r')
for line in file:
name = line.strip()
print(name)
file.close()
I'm unsure how to sort them.
Here's the file contents:
Matthew, 13
Luke, 6
John, 3
Bobba, 4
What I want the output to be:
John 3
Bobba 4
Luke 6
Matthew 13
Can anyone help?
python sorting python-3.x
add a comment |
I want to sort a list of names by their score.
What I have so far is
file = open("scores.txt", 'r')
for line in file:
name = line.strip()
print(name)
file.close()
I'm unsure how to sort them.
Here's the file contents:
Matthew, 13
Luke, 6
John, 3
Bobba, 4
What I want the output to be:
John 3
Bobba 4
Luke 6
Matthew 13
Can anyone help?
python sorting python-3.x
I want to sort a list of names by their score.
What I have so far is
file = open("scores.txt", 'r')
for line in file:
name = line.strip()
print(name)
file.close()
I'm unsure how to sort them.
Here's the file contents:
Matthew, 13
Luke, 6
John, 3
Bobba, 4
What I want the output to be:
John 3
Bobba 4
Luke 6
Matthew 13
Can anyone help?
python sorting python-3.x
python sorting python-3.x
edited May 19 '15 at 11:39
SuperBiasedMan
7,28083256
7,28083256
asked Jul 13 '13 at 17:14
user2365677user2365677
1815
1815
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
you can use the .split(',')
method to split a line into its separate parts, then use int()
to convert the score to a number. The .sort()
method sorts a list in place, and the key
tells it what to sort by.
scores =
with open("scores.txt") as f:
for line in f:
name, score = line.split(',')
score = int(score)
scores.append((name, score))
scores.sort(key=lambda s: s[1])
for name, score in scores:
print(name, score)
This will give you a list of tuples containing (name, score) pairs in sorted order. If you want to print them out with a comma in between them (to keep it consistent) change the print to print(name, score, sep=', ')
The reading of the input file can also be expressed as one (big) line
with open("scores.txt") as f:
scores = [(name, int(score)) for name, score in (line.split(',') for line in f)]
A brief explanation of the key=
:
a lambda function is an anonymous function, that is, a function without a name. You generally use these when you need a function only for a small operation. .sort
has an optional key
keyword argument that takes a function and uses the return of that function in sorting the objects.
So this lambda
could also be written as
def ret_score(pair):
return pair[1]
And you could then write .sort(key=ret_score)
but since we dont really need that function for anything else, its not necessary to declare it. The lambda syntax is
lambda <arguments> : <return value>
So this lambda takes a pair, and returns the second element in it. You can save a lambda
and use it like a regular function if you wish.
>>> square = lambda x: x**2 # takes x, returns x squared
>>> square(3)
9
>>> square(6)
36
Is there a way to make it so in prints out Vertically?Like- John 3 Bobba 4 Luke 6 Matthew 13
– user2365677
Jul 13 '13 at 17:28
@user2365677 yes I just added that
– Ryan Haining
Jul 13 '13 at 17:29
Just for information sake, ".sort(key=lambda s: s[1])" i know of the .sort, what does lambda mean? It does work, its what i wanted and thank you :)
– user2365677
Jul 13 '13 at 17:31
@user2365677 I've added a quick explanation oflambda
but it can be a strange thing to understand
– Ryan Haining
Jul 13 '13 at 17:38
AH, thank you very Much :)
– user2365677
Jul 13 '13 at 17:40
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f17632428%2fsorting-names-by-their-high-scores%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
you can use the .split(',')
method to split a line into its separate parts, then use int()
to convert the score to a number. The .sort()
method sorts a list in place, and the key
tells it what to sort by.
scores =
with open("scores.txt") as f:
for line in f:
name, score = line.split(',')
score = int(score)
scores.append((name, score))
scores.sort(key=lambda s: s[1])
for name, score in scores:
print(name, score)
This will give you a list of tuples containing (name, score) pairs in sorted order. If you want to print them out with a comma in between them (to keep it consistent) change the print to print(name, score, sep=', ')
The reading of the input file can also be expressed as one (big) line
with open("scores.txt") as f:
scores = [(name, int(score)) for name, score in (line.split(',') for line in f)]
A brief explanation of the key=
:
a lambda function is an anonymous function, that is, a function without a name. You generally use these when you need a function only for a small operation. .sort
has an optional key
keyword argument that takes a function and uses the return of that function in sorting the objects.
So this lambda
could also be written as
def ret_score(pair):
return pair[1]
And you could then write .sort(key=ret_score)
but since we dont really need that function for anything else, its not necessary to declare it. The lambda syntax is
lambda <arguments> : <return value>
So this lambda takes a pair, and returns the second element in it. You can save a lambda
and use it like a regular function if you wish.
>>> square = lambda x: x**2 # takes x, returns x squared
>>> square(3)
9
>>> square(6)
36
Is there a way to make it so in prints out Vertically?Like- John 3 Bobba 4 Luke 6 Matthew 13
– user2365677
Jul 13 '13 at 17:28
@user2365677 yes I just added that
– Ryan Haining
Jul 13 '13 at 17:29
Just for information sake, ".sort(key=lambda s: s[1])" i know of the .sort, what does lambda mean? It does work, its what i wanted and thank you :)
– user2365677
Jul 13 '13 at 17:31
@user2365677 I've added a quick explanation oflambda
but it can be a strange thing to understand
– Ryan Haining
Jul 13 '13 at 17:38
AH, thank you very Much :)
– user2365677
Jul 13 '13 at 17:40
|
show 1 more comment
you can use the .split(',')
method to split a line into its separate parts, then use int()
to convert the score to a number. The .sort()
method sorts a list in place, and the key
tells it what to sort by.
scores =
with open("scores.txt") as f:
for line in f:
name, score = line.split(',')
score = int(score)
scores.append((name, score))
scores.sort(key=lambda s: s[1])
for name, score in scores:
print(name, score)
This will give you a list of tuples containing (name, score) pairs in sorted order. If you want to print them out with a comma in between them (to keep it consistent) change the print to print(name, score, sep=', ')
The reading of the input file can also be expressed as one (big) line
with open("scores.txt") as f:
scores = [(name, int(score)) for name, score in (line.split(',') for line in f)]
A brief explanation of the key=
:
a lambda function is an anonymous function, that is, a function without a name. You generally use these when you need a function only for a small operation. .sort
has an optional key
keyword argument that takes a function and uses the return of that function in sorting the objects.
So this lambda
could also be written as
def ret_score(pair):
return pair[1]
And you could then write .sort(key=ret_score)
but since we dont really need that function for anything else, its not necessary to declare it. The lambda syntax is
lambda <arguments> : <return value>
So this lambda takes a pair, and returns the second element in it. You can save a lambda
and use it like a regular function if you wish.
>>> square = lambda x: x**2 # takes x, returns x squared
>>> square(3)
9
>>> square(6)
36
Is there a way to make it so in prints out Vertically?Like- John 3 Bobba 4 Luke 6 Matthew 13
– user2365677
Jul 13 '13 at 17:28
@user2365677 yes I just added that
– Ryan Haining
Jul 13 '13 at 17:29
Just for information sake, ".sort(key=lambda s: s[1])" i know of the .sort, what does lambda mean? It does work, its what i wanted and thank you :)
– user2365677
Jul 13 '13 at 17:31
@user2365677 I've added a quick explanation oflambda
but it can be a strange thing to understand
– Ryan Haining
Jul 13 '13 at 17:38
AH, thank you very Much :)
– user2365677
Jul 13 '13 at 17:40
|
show 1 more comment
you can use the .split(',')
method to split a line into its separate parts, then use int()
to convert the score to a number. The .sort()
method sorts a list in place, and the key
tells it what to sort by.
scores =
with open("scores.txt") as f:
for line in f:
name, score = line.split(',')
score = int(score)
scores.append((name, score))
scores.sort(key=lambda s: s[1])
for name, score in scores:
print(name, score)
This will give you a list of tuples containing (name, score) pairs in sorted order. If you want to print them out with a comma in between them (to keep it consistent) change the print to print(name, score, sep=', ')
The reading of the input file can also be expressed as one (big) line
with open("scores.txt") as f:
scores = [(name, int(score)) for name, score in (line.split(',') for line in f)]
A brief explanation of the key=
:
a lambda function is an anonymous function, that is, a function without a name. You generally use these when you need a function only for a small operation. .sort
has an optional key
keyword argument that takes a function and uses the return of that function in sorting the objects.
So this lambda
could also be written as
def ret_score(pair):
return pair[1]
And you could then write .sort(key=ret_score)
but since we dont really need that function for anything else, its not necessary to declare it. The lambda syntax is
lambda <arguments> : <return value>
So this lambda takes a pair, and returns the second element in it. You can save a lambda
and use it like a regular function if you wish.
>>> square = lambda x: x**2 # takes x, returns x squared
>>> square(3)
9
>>> square(6)
36
you can use the .split(',')
method to split a line into its separate parts, then use int()
to convert the score to a number. The .sort()
method sorts a list in place, and the key
tells it what to sort by.
scores =
with open("scores.txt") as f:
for line in f:
name, score = line.split(',')
score = int(score)
scores.append((name, score))
scores.sort(key=lambda s: s[1])
for name, score in scores:
print(name, score)
This will give you a list of tuples containing (name, score) pairs in sorted order. If you want to print them out with a comma in between them (to keep it consistent) change the print to print(name, score, sep=', ')
The reading of the input file can also be expressed as one (big) line
with open("scores.txt") as f:
scores = [(name, int(score)) for name, score in (line.split(',') for line in f)]
A brief explanation of the key=
:
a lambda function is an anonymous function, that is, a function without a name. You generally use these when you need a function only for a small operation. .sort
has an optional key
keyword argument that takes a function and uses the return of that function in sorting the objects.
So this lambda
could also be written as
def ret_score(pair):
return pair[1]
And you could then write .sort(key=ret_score)
but since we dont really need that function for anything else, its not necessary to declare it. The lambda syntax is
lambda <arguments> : <return value>
So this lambda takes a pair, and returns the second element in it. You can save a lambda
and use it like a regular function if you wish.
>>> square = lambda x: x**2 # takes x, returns x squared
>>> square(3)
9
>>> square(6)
36
edited Jul 13 '13 at 17:45
answered Jul 13 '13 at 17:21


Ryan HainingRyan Haining
21.6k870122
21.6k870122
Is there a way to make it so in prints out Vertically?Like- John 3 Bobba 4 Luke 6 Matthew 13
– user2365677
Jul 13 '13 at 17:28
@user2365677 yes I just added that
– Ryan Haining
Jul 13 '13 at 17:29
Just for information sake, ".sort(key=lambda s: s[1])" i know of the .sort, what does lambda mean? It does work, its what i wanted and thank you :)
– user2365677
Jul 13 '13 at 17:31
@user2365677 I've added a quick explanation oflambda
but it can be a strange thing to understand
– Ryan Haining
Jul 13 '13 at 17:38
AH, thank you very Much :)
– user2365677
Jul 13 '13 at 17:40
|
show 1 more comment
Is there a way to make it so in prints out Vertically?Like- John 3 Bobba 4 Luke 6 Matthew 13
– user2365677
Jul 13 '13 at 17:28
@user2365677 yes I just added that
– Ryan Haining
Jul 13 '13 at 17:29
Just for information sake, ".sort(key=lambda s: s[1])" i know of the .sort, what does lambda mean? It does work, its what i wanted and thank you :)
– user2365677
Jul 13 '13 at 17:31
@user2365677 I've added a quick explanation oflambda
but it can be a strange thing to understand
– Ryan Haining
Jul 13 '13 at 17:38
AH, thank you very Much :)
– user2365677
Jul 13 '13 at 17:40
Is there a way to make it so in prints out Vertically?Like- John 3 Bobba 4 Luke 6 Matthew 13
– user2365677
Jul 13 '13 at 17:28
Is there a way to make it so in prints out Vertically?Like- John 3 Bobba 4 Luke 6 Matthew 13
– user2365677
Jul 13 '13 at 17:28
@user2365677 yes I just added that
– Ryan Haining
Jul 13 '13 at 17:29
@user2365677 yes I just added that
– Ryan Haining
Jul 13 '13 at 17:29
Just for information sake, ".sort(key=lambda s: s[1])" i know of the .sort, what does lambda mean? It does work, its what i wanted and thank you :)
– user2365677
Jul 13 '13 at 17:31
Just for information sake, ".sort(key=lambda s: s[1])" i know of the .sort, what does lambda mean? It does work, its what i wanted and thank you :)
– user2365677
Jul 13 '13 at 17:31
@user2365677 I've added a quick explanation of
lambda
but it can be a strange thing to understand– Ryan Haining
Jul 13 '13 at 17:38
@user2365677 I've added a quick explanation of
lambda
but it can be a strange thing to understand– Ryan Haining
Jul 13 '13 at 17:38
AH, thank you very Much :)
– user2365677
Jul 13 '13 at 17:40
AH, thank you very Much :)
– user2365677
Jul 13 '13 at 17:40
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f17632428%2fsorting-names-by-their-high-scores%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
p1V,1tAo,Iqd5tywI3iNp7vPSK82WB67yHkSmmI5uvZpesjzHViYo0PF SHHziTn0PJ6ZrTjBdc3O,A8A