Find and remove elements from List<Dictionary>
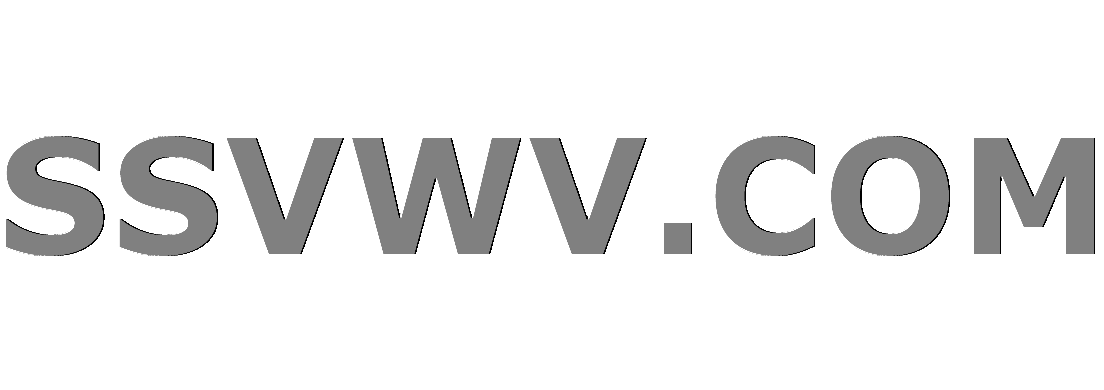
Multi tool use
I'm currently working on a C# 4.7.2 application. I'm about to write an extension method for a custom type and I'm struggling with my LINQ query unfortunately.
I need filter a List<Dictionary<string, object>>
to find the elements of Dictionary<string, object>
in the list with a certain key and remove it from my list. Furthermore, a list entry can be null.
A list entry (dictionary) can look like this, there can be several elements with value key A, i need to remove all actually:
Key | Value
"MyId" : "A",
"Width" : 100,
"Length" : 50
Very simple structure. The tricky thing is to find the dictionary elements in the list. My extension method looks like that:
public static List<Dictionary<string, object>> RemoveItem(this List<Dictionary<string, object> items, string value)
{
var itemToRemove = items.FirstOrDefault(x => x.ContainsKey("MyId")).Values.Contains(value);
items.Remove(itemToRemove);
return items;
}
Unfortunately this LINQ query does not work correctly.
Do you know how to solve this issue?
Thank you very much!!
c# .net linq dictionary
add a comment |
I'm currently working on a C# 4.7.2 application. I'm about to write an extension method for a custom type and I'm struggling with my LINQ query unfortunately.
I need filter a List<Dictionary<string, object>>
to find the elements of Dictionary<string, object>
in the list with a certain key and remove it from my list. Furthermore, a list entry can be null.
A list entry (dictionary) can look like this, there can be several elements with value key A, i need to remove all actually:
Key | Value
"MyId" : "A",
"Width" : 100,
"Length" : 50
Very simple structure. The tricky thing is to find the dictionary elements in the list. My extension method looks like that:
public static List<Dictionary<string, object>> RemoveItem(this List<Dictionary<string, object> items, string value)
{
var itemToRemove = items.FirstOrDefault(x => x.ContainsKey("MyId")).Values.Contains(value);
items.Remove(itemToRemove);
return items;
}
Unfortunately this LINQ query does not work correctly.
Do you know how to solve this issue?
Thank you very much!!
c# .net linq dictionary
3
Unfortunately this LINQ query does not work correctly. What is happening with it?
– Jay Gould
Nov 15 '18 at 9:12
"Does not work" has never been a suiteable problem description on a programming forum. Please tell us what exactly is happening or not happening.
– Christopher
Nov 15 '18 at 9:13
Why you need the value?
– Rango
Nov 15 '18 at 9:13
Hi sorry, actually I need to remove all dictionary items from the list, that have the key MyId and it's value A, let's say...
– timhorton42
Nov 15 '18 at 9:14
Please have a look at this: stackoverflow.com/a/24549702/4329813
– popsiporkkanaa
Nov 15 '18 at 9:15
add a comment |
I'm currently working on a C# 4.7.2 application. I'm about to write an extension method for a custom type and I'm struggling with my LINQ query unfortunately.
I need filter a List<Dictionary<string, object>>
to find the elements of Dictionary<string, object>
in the list with a certain key and remove it from my list. Furthermore, a list entry can be null.
A list entry (dictionary) can look like this, there can be several elements with value key A, i need to remove all actually:
Key | Value
"MyId" : "A",
"Width" : 100,
"Length" : 50
Very simple structure. The tricky thing is to find the dictionary elements in the list. My extension method looks like that:
public static List<Dictionary<string, object>> RemoveItem(this List<Dictionary<string, object> items, string value)
{
var itemToRemove = items.FirstOrDefault(x => x.ContainsKey("MyId")).Values.Contains(value);
items.Remove(itemToRemove);
return items;
}
Unfortunately this LINQ query does not work correctly.
Do you know how to solve this issue?
Thank you very much!!
c# .net linq dictionary
I'm currently working on a C# 4.7.2 application. I'm about to write an extension method for a custom type and I'm struggling with my LINQ query unfortunately.
I need filter a List<Dictionary<string, object>>
to find the elements of Dictionary<string, object>
in the list with a certain key and remove it from my list. Furthermore, a list entry can be null.
A list entry (dictionary) can look like this, there can be several elements with value key A, i need to remove all actually:
Key | Value
"MyId" : "A",
"Width" : 100,
"Length" : 50
Very simple structure. The tricky thing is to find the dictionary elements in the list. My extension method looks like that:
public static List<Dictionary<string, object>> RemoveItem(this List<Dictionary<string, object> items, string value)
{
var itemToRemove = items.FirstOrDefault(x => x.ContainsKey("MyId")).Values.Contains(value);
items.Remove(itemToRemove);
return items;
}
Unfortunately this LINQ query does not work correctly.
Do you know how to solve this issue?
Thank you very much!!
c# .net linq dictionary
c# .net linq dictionary
edited Nov 15 '18 at 9:35
timhorton42
asked Nov 15 '18 at 9:08
timhorton42timhorton42
617
617
3
Unfortunately this LINQ query does not work correctly. What is happening with it?
– Jay Gould
Nov 15 '18 at 9:12
"Does not work" has never been a suiteable problem description on a programming forum. Please tell us what exactly is happening or not happening.
– Christopher
Nov 15 '18 at 9:13
Why you need the value?
– Rango
Nov 15 '18 at 9:13
Hi sorry, actually I need to remove all dictionary items from the list, that have the key MyId and it's value A, let's say...
– timhorton42
Nov 15 '18 at 9:14
Please have a look at this: stackoverflow.com/a/24549702/4329813
– popsiporkkanaa
Nov 15 '18 at 9:15
add a comment |
3
Unfortunately this LINQ query does not work correctly. What is happening with it?
– Jay Gould
Nov 15 '18 at 9:12
"Does not work" has never been a suiteable problem description on a programming forum. Please tell us what exactly is happening or not happening.
– Christopher
Nov 15 '18 at 9:13
Why you need the value?
– Rango
Nov 15 '18 at 9:13
Hi sorry, actually I need to remove all dictionary items from the list, that have the key MyId and it's value A, let's say...
– timhorton42
Nov 15 '18 at 9:14
Please have a look at this: stackoverflow.com/a/24549702/4329813
– popsiporkkanaa
Nov 15 '18 at 9:15
3
3
Unfortunately this LINQ query does not work correctly. What is happening with it?
– Jay Gould
Nov 15 '18 at 9:12
Unfortunately this LINQ query does not work correctly. What is happening with it?
– Jay Gould
Nov 15 '18 at 9:12
"Does not work" has never been a suiteable problem description on a programming forum. Please tell us what exactly is happening or not happening.
– Christopher
Nov 15 '18 at 9:13
"Does not work" has never been a suiteable problem description on a programming forum. Please tell us what exactly is happening or not happening.
– Christopher
Nov 15 '18 at 9:13
Why you need the value?
– Rango
Nov 15 '18 at 9:13
Why you need the value?
– Rango
Nov 15 '18 at 9:13
Hi sorry, actually I need to remove all dictionary items from the list, that have the key MyId and it's value A, let's say...
– timhorton42
Nov 15 '18 at 9:14
Hi sorry, actually I need to remove all dictionary items from the list, that have the key MyId and it's value A, let's say...
– timhorton42
Nov 15 '18 at 9:14
Please have a look at this: stackoverflow.com/a/24549702/4329813
– popsiporkkanaa
Nov 15 '18 at 9:15
Please have a look at this: stackoverflow.com/a/24549702/4329813
– popsiporkkanaa
Nov 15 '18 at 9:15
add a comment |
5 Answers
5
active
oldest
votes
You want to remove all with a key and a value? You don't even need LINQ:
public static int RemoveItems(this List<Dictionary<string, object>> dictionaryList, string value)
{
int removed = dictionaryList
.RemoveAll(dict => dict.TryGetValue("MyId", out object val) && value.Equals(val));
return removed;
}
1
thanks for this helpful response! i added a null checker and it works very well!
– timhorton42
Nov 15 '18 at 9:59
add a comment |
You could use the method RemoveAll
of the list. Then you give in a predicate that checks the dictionary (which is a collection of KeyValuePair<TKey, TValue>
):
items.RemoveAll(dict => dict.Any(kv => kv.Key == "MyId" && ( kv.Value as string ) == "A"));
Or as suggested by Tim Schmelter:
items.RemoveAll(dict => dict.TryGetValue("MyId", out object value) && (value as string) == "A");
2
I would not enumerate the dictionary to find keys, useTryGetValue
– Rango
Nov 15 '18 at 9:24
Depends on the size of the dictionary, but fair enough
– SynerCoder
Nov 15 '18 at 9:25
well, if you use a dictionary then mostly because retrieving a value by using its key is very fast(O(1)
).
– Rango
Nov 15 '18 at 9:29
1
@TimSchmelter better? ^^
– SynerCoder
Nov 15 '18 at 9:35
add a comment |
You would need to do something like:
itemsToRemove = items.Where(x => x.ContainsKey("MyId") && x["MyId"].ToString() == value);
add a comment |
From your description, you seem to want the first dictionary containing the key with the value from the parameter value
. That would be items.FirstOrDefault(x => x.ContainsKey(value))
What you are doing is getting dictionary containing one predefined key "myId"
and then going through the objects inside the dictionary and comparing their values with your value
parameter, which is not what you described you want.
If you expect more dictionaries to contain the given key,and you want to remove all of them, you should use list.RemoveAll(dict => dict.ContainsKey(value))
The value should also match
– Rango
Nov 15 '18 at 9:31
add a comment |
public static List<Dictionary<string, object>> RemoveItem(this List<Dictionary<string, object>> items, string value, string key)
{
foreach (var item in items)
{
if(item.ContainsKey(key) && item[key] == value)
{
item.Remove(key);
}
}
return items;
}
This gonna work just fine.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53315839%2ffind-and-remove-elements-from-listdictionarystring-object%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
You want to remove all with a key and a value? You don't even need LINQ:
public static int RemoveItems(this List<Dictionary<string, object>> dictionaryList, string value)
{
int removed = dictionaryList
.RemoveAll(dict => dict.TryGetValue("MyId", out object val) && value.Equals(val));
return removed;
}
1
thanks for this helpful response! i added a null checker and it works very well!
– timhorton42
Nov 15 '18 at 9:59
add a comment |
You want to remove all with a key and a value? You don't even need LINQ:
public static int RemoveItems(this List<Dictionary<string, object>> dictionaryList, string value)
{
int removed = dictionaryList
.RemoveAll(dict => dict.TryGetValue("MyId", out object val) && value.Equals(val));
return removed;
}
1
thanks for this helpful response! i added a null checker and it works very well!
– timhorton42
Nov 15 '18 at 9:59
add a comment |
You want to remove all with a key and a value? You don't even need LINQ:
public static int RemoveItems(this List<Dictionary<string, object>> dictionaryList, string value)
{
int removed = dictionaryList
.RemoveAll(dict => dict.TryGetValue("MyId", out object val) && value.Equals(val));
return removed;
}
You want to remove all with a key and a value? You don't even need LINQ:
public static int RemoveItems(this List<Dictionary<string, object>> dictionaryList, string value)
{
int removed = dictionaryList
.RemoveAll(dict => dict.TryGetValue("MyId", out object val) && value.Equals(val));
return removed;
}
edited Nov 15 '18 at 9:22
answered Nov 15 '18 at 9:14


RangoRango
365k46469729
365k46469729
1
thanks for this helpful response! i added a null checker and it works very well!
– timhorton42
Nov 15 '18 at 9:59
add a comment |
1
thanks for this helpful response! i added a null checker and it works very well!
– timhorton42
Nov 15 '18 at 9:59
1
1
thanks for this helpful response! i added a null checker and it works very well!
– timhorton42
Nov 15 '18 at 9:59
thanks for this helpful response! i added a null checker and it works very well!
– timhorton42
Nov 15 '18 at 9:59
add a comment |
You could use the method RemoveAll
of the list. Then you give in a predicate that checks the dictionary (which is a collection of KeyValuePair<TKey, TValue>
):
items.RemoveAll(dict => dict.Any(kv => kv.Key == "MyId" && ( kv.Value as string ) == "A"));
Or as suggested by Tim Schmelter:
items.RemoveAll(dict => dict.TryGetValue("MyId", out object value) && (value as string) == "A");
2
I would not enumerate the dictionary to find keys, useTryGetValue
– Rango
Nov 15 '18 at 9:24
Depends on the size of the dictionary, but fair enough
– SynerCoder
Nov 15 '18 at 9:25
well, if you use a dictionary then mostly because retrieving a value by using its key is very fast(O(1)
).
– Rango
Nov 15 '18 at 9:29
1
@TimSchmelter better? ^^
– SynerCoder
Nov 15 '18 at 9:35
add a comment |
You could use the method RemoveAll
of the list. Then you give in a predicate that checks the dictionary (which is a collection of KeyValuePair<TKey, TValue>
):
items.RemoveAll(dict => dict.Any(kv => kv.Key == "MyId" && ( kv.Value as string ) == "A"));
Or as suggested by Tim Schmelter:
items.RemoveAll(dict => dict.TryGetValue("MyId", out object value) && (value as string) == "A");
2
I would not enumerate the dictionary to find keys, useTryGetValue
– Rango
Nov 15 '18 at 9:24
Depends on the size of the dictionary, but fair enough
– SynerCoder
Nov 15 '18 at 9:25
well, if you use a dictionary then mostly because retrieving a value by using its key is very fast(O(1)
).
– Rango
Nov 15 '18 at 9:29
1
@TimSchmelter better? ^^
– SynerCoder
Nov 15 '18 at 9:35
add a comment |
You could use the method RemoveAll
of the list. Then you give in a predicate that checks the dictionary (which is a collection of KeyValuePair<TKey, TValue>
):
items.RemoveAll(dict => dict.Any(kv => kv.Key == "MyId" && ( kv.Value as string ) == "A"));
Or as suggested by Tim Schmelter:
items.RemoveAll(dict => dict.TryGetValue("MyId", out object value) && (value as string) == "A");
You could use the method RemoveAll
of the list. Then you give in a predicate that checks the dictionary (which is a collection of KeyValuePair<TKey, TValue>
):
items.RemoveAll(dict => dict.Any(kv => kv.Key == "MyId" && ( kv.Value as string ) == "A"));
Or as suggested by Tim Schmelter:
items.RemoveAll(dict => dict.TryGetValue("MyId", out object value) && (value as string) == "A");
edited Nov 15 '18 at 9:34
answered Nov 15 '18 at 9:19
SynerCoderSynerCoder
10.2k44173
10.2k44173
2
I would not enumerate the dictionary to find keys, useTryGetValue
– Rango
Nov 15 '18 at 9:24
Depends on the size of the dictionary, but fair enough
– SynerCoder
Nov 15 '18 at 9:25
well, if you use a dictionary then mostly because retrieving a value by using its key is very fast(O(1)
).
– Rango
Nov 15 '18 at 9:29
1
@TimSchmelter better? ^^
– SynerCoder
Nov 15 '18 at 9:35
add a comment |
2
I would not enumerate the dictionary to find keys, useTryGetValue
– Rango
Nov 15 '18 at 9:24
Depends on the size of the dictionary, but fair enough
– SynerCoder
Nov 15 '18 at 9:25
well, if you use a dictionary then mostly because retrieving a value by using its key is very fast(O(1)
).
– Rango
Nov 15 '18 at 9:29
1
@TimSchmelter better? ^^
– SynerCoder
Nov 15 '18 at 9:35
2
2
I would not enumerate the dictionary to find keys, use
TryGetValue
– Rango
Nov 15 '18 at 9:24
I would not enumerate the dictionary to find keys, use
TryGetValue
– Rango
Nov 15 '18 at 9:24
Depends on the size of the dictionary, but fair enough
– SynerCoder
Nov 15 '18 at 9:25
Depends on the size of the dictionary, but fair enough
– SynerCoder
Nov 15 '18 at 9:25
well, if you use a dictionary then mostly because retrieving a value by using its key is very fast(
O(1)
).– Rango
Nov 15 '18 at 9:29
well, if you use a dictionary then mostly because retrieving a value by using its key is very fast(
O(1)
).– Rango
Nov 15 '18 at 9:29
1
1
@TimSchmelter better? ^^
– SynerCoder
Nov 15 '18 at 9:35
@TimSchmelter better? ^^
– SynerCoder
Nov 15 '18 at 9:35
add a comment |
You would need to do something like:
itemsToRemove = items.Where(x => x.ContainsKey("MyId") && x["MyId"].ToString() == value);
add a comment |
You would need to do something like:
itemsToRemove = items.Where(x => x.ContainsKey("MyId") && x["MyId"].ToString() == value);
add a comment |
You would need to do something like:
itemsToRemove = items.Where(x => x.ContainsKey("MyId") && x["MyId"].ToString() == value);
You would need to do something like:
itemsToRemove = items.Where(x => x.ContainsKey("MyId") && x["MyId"].ToString() == value);
answered Nov 15 '18 at 9:17


GabituGabitu
1514
1514
add a comment |
add a comment |
From your description, you seem to want the first dictionary containing the key with the value from the parameter value
. That would be items.FirstOrDefault(x => x.ContainsKey(value))
What you are doing is getting dictionary containing one predefined key "myId"
and then going through the objects inside the dictionary and comparing their values with your value
parameter, which is not what you described you want.
If you expect more dictionaries to contain the given key,and you want to remove all of them, you should use list.RemoveAll(dict => dict.ContainsKey(value))
The value should also match
– Rango
Nov 15 '18 at 9:31
add a comment |
From your description, you seem to want the first dictionary containing the key with the value from the parameter value
. That would be items.FirstOrDefault(x => x.ContainsKey(value))
What you are doing is getting dictionary containing one predefined key "myId"
and then going through the objects inside the dictionary and comparing their values with your value
parameter, which is not what you described you want.
If you expect more dictionaries to contain the given key,and you want to remove all of them, you should use list.RemoveAll(dict => dict.ContainsKey(value))
The value should also match
– Rango
Nov 15 '18 at 9:31
add a comment |
From your description, you seem to want the first dictionary containing the key with the value from the parameter value
. That would be items.FirstOrDefault(x => x.ContainsKey(value))
What you are doing is getting dictionary containing one predefined key "myId"
and then going through the objects inside the dictionary and comparing their values with your value
parameter, which is not what you described you want.
If you expect more dictionaries to contain the given key,and you want to remove all of them, you should use list.RemoveAll(dict => dict.ContainsKey(value))
From your description, you seem to want the first dictionary containing the key with the value from the parameter value
. That would be items.FirstOrDefault(x => x.ContainsKey(value))
What you are doing is getting dictionary containing one predefined key "myId"
and then going through the objects inside the dictionary and comparing their values with your value
parameter, which is not what you described you want.
If you expect more dictionaries to contain the given key,and you want to remove all of them, you should use list.RemoveAll(dict => dict.ContainsKey(value))
answered Nov 15 '18 at 9:18
MadKarelMadKarel
812
812
The value should also match
– Rango
Nov 15 '18 at 9:31
add a comment |
The value should also match
– Rango
Nov 15 '18 at 9:31
The value should also match
– Rango
Nov 15 '18 at 9:31
The value should also match
– Rango
Nov 15 '18 at 9:31
add a comment |
public static List<Dictionary<string, object>> RemoveItem(this List<Dictionary<string, object>> items, string value, string key)
{
foreach (var item in items)
{
if(item.ContainsKey(key) && item[key] == value)
{
item.Remove(key);
}
}
return items;
}
This gonna work just fine.
add a comment |
public static List<Dictionary<string, object>> RemoveItem(this List<Dictionary<string, object>> items, string value, string key)
{
foreach (var item in items)
{
if(item.ContainsKey(key) && item[key] == value)
{
item.Remove(key);
}
}
return items;
}
This gonna work just fine.
add a comment |
public static List<Dictionary<string, object>> RemoveItem(this List<Dictionary<string, object>> items, string value, string key)
{
foreach (var item in items)
{
if(item.ContainsKey(key) && item[key] == value)
{
item.Remove(key);
}
}
return items;
}
This gonna work just fine.
public static List<Dictionary<string, object>> RemoveItem(this List<Dictionary<string, object>> items, string value, string key)
{
foreach (var item in items)
{
if(item.ContainsKey(key) && item[key] == value)
{
item.Remove(key);
}
}
return items;
}
This gonna work just fine.
edited Nov 15 '18 at 10:04
answered Nov 15 '18 at 9:33
Aditya JaiswalAditya Jaiswal
12
12
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53315839%2ffind-and-remove-elements-from-listdictionarystring-object%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
AZ5TpV59nrgYHGB,Gu dqtQWToEcospKWJE
3
Unfortunately this LINQ query does not work correctly. What is happening with it?
– Jay Gould
Nov 15 '18 at 9:12
"Does not work" has never been a suiteable problem description on a programming forum. Please tell us what exactly is happening or not happening.
– Christopher
Nov 15 '18 at 9:13
Why you need the value?
– Rango
Nov 15 '18 at 9:13
Hi sorry, actually I need to remove all dictionary items from the list, that have the key MyId and it's value A, let's say...
– timhorton42
Nov 15 '18 at 9:14
Please have a look at this: stackoverflow.com/a/24549702/4329813
– popsiporkkanaa
Nov 15 '18 at 9:15