How to properly seperate PHP from HTML (Business logic from View)
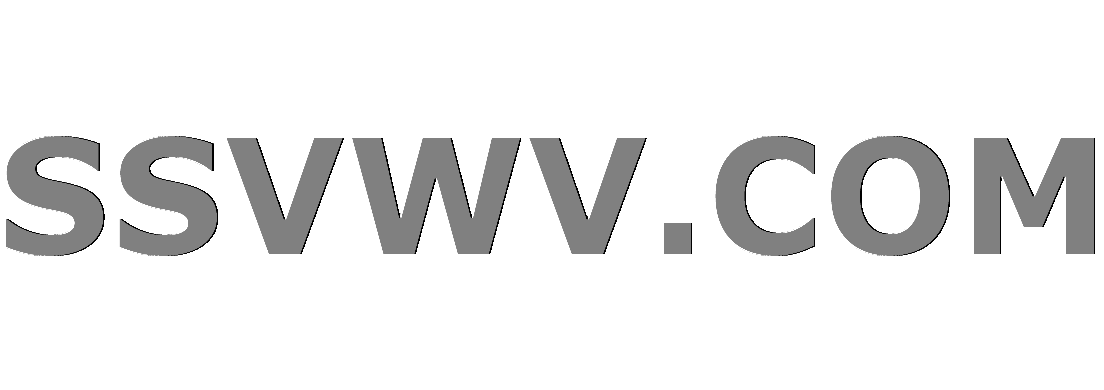
Multi tool use
I am learning to write better code and to not mix PHP code with HTML/CSS since that will be better both for me and people who will read my code after me. However I stumbled upon a situation where I have users with different roles and each role has it's own color/class/style/html. I end up putting either HTML code in my PHP logic or vice versa. Is there a nice way around this?
PHP:
if ($user_role == 'administrator') {
$user_color == '#ff0000'; <-- CSS Style in my PHP
$user_class == 'admin-class'; <-- CSS Class in my PHP
}
$users = [
'username' => 'John',
'user_color' => '#ff0000' <-- CSS Style in my PHP
]
HTML:
<a class="<?= $user_class ?> style="color: <? $user_color ?>">Administrator</a>
or if I did not want HTML or CSS in my PHP then I end up putting PHP in my HTML.
HTML
foreach($users as $user):
// Too much PHP logic in my HTML view
if ($user->role == 'administrator') {
$user_color = 'red';
} else if ($user->role == moderator) {
$user_color = '#00ff00'
}
<a style="color: <?= $user_color ?>">$user->username</a>
endforeach;
So either way, I end up mixing the two together. This is just one example where I need to adjust the HTML depending on some logic or conditions.
php html
add a comment |
I am learning to write better code and to not mix PHP code with HTML/CSS since that will be better both for me and people who will read my code after me. However I stumbled upon a situation where I have users with different roles and each role has it's own color/class/style/html. I end up putting either HTML code in my PHP logic or vice versa. Is there a nice way around this?
PHP:
if ($user_role == 'administrator') {
$user_color == '#ff0000'; <-- CSS Style in my PHP
$user_class == 'admin-class'; <-- CSS Class in my PHP
}
$users = [
'username' => 'John',
'user_color' => '#ff0000' <-- CSS Style in my PHP
]
HTML:
<a class="<?= $user_class ?> style="color: <? $user_color ?>">Administrator</a>
or if I did not want HTML or CSS in my PHP then I end up putting PHP in my HTML.
HTML
foreach($users as $user):
// Too much PHP logic in my HTML view
if ($user->role == 'administrator') {
$user_color = 'red';
} else if ($user->role == moderator) {
$user_color = '#00ff00'
}
<a style="color: <?= $user_color ?>">$user->username</a>
endforeach;
So either way, I end up mixing the two together. This is just one example where I need to adjust the HTML depending on some logic or conditions.
php html
Just set$user_role
as the class in your html, then have css classes in your css class for each role?$user_color
seems unnecessary to me. If your only condition is "no PHP in a template" you can always use the likes of twig.
– Jon Stirling
Nov 15 '18 at 9:40
There's nothing wrong with putting a little PHP into "templating" files (e.g.<a href="<?= $user_class; ?>" ... />
) when it concerns display logic - that's pretty much what the.phtml
file extension is supposed to represent. Though you could use a templating engine; Symfony uses Twig for instance.
– CD001
Nov 15 '18 at 9:44
add a comment |
I am learning to write better code and to not mix PHP code with HTML/CSS since that will be better both for me and people who will read my code after me. However I stumbled upon a situation where I have users with different roles and each role has it's own color/class/style/html. I end up putting either HTML code in my PHP logic or vice versa. Is there a nice way around this?
PHP:
if ($user_role == 'administrator') {
$user_color == '#ff0000'; <-- CSS Style in my PHP
$user_class == 'admin-class'; <-- CSS Class in my PHP
}
$users = [
'username' => 'John',
'user_color' => '#ff0000' <-- CSS Style in my PHP
]
HTML:
<a class="<?= $user_class ?> style="color: <? $user_color ?>">Administrator</a>
or if I did not want HTML or CSS in my PHP then I end up putting PHP in my HTML.
HTML
foreach($users as $user):
// Too much PHP logic in my HTML view
if ($user->role == 'administrator') {
$user_color = 'red';
} else if ($user->role == moderator) {
$user_color = '#00ff00'
}
<a style="color: <?= $user_color ?>">$user->username</a>
endforeach;
So either way, I end up mixing the two together. This is just one example where I need to adjust the HTML depending on some logic or conditions.
php html
I am learning to write better code and to not mix PHP code with HTML/CSS since that will be better both for me and people who will read my code after me. However I stumbled upon a situation where I have users with different roles and each role has it's own color/class/style/html. I end up putting either HTML code in my PHP logic or vice versa. Is there a nice way around this?
PHP:
if ($user_role == 'administrator') {
$user_color == '#ff0000'; <-- CSS Style in my PHP
$user_class == 'admin-class'; <-- CSS Class in my PHP
}
$users = [
'username' => 'John',
'user_color' => '#ff0000' <-- CSS Style in my PHP
]
HTML:
<a class="<?= $user_class ?> style="color: <? $user_color ?>">Administrator</a>
or if I did not want HTML or CSS in my PHP then I end up putting PHP in my HTML.
HTML
foreach($users as $user):
// Too much PHP logic in my HTML view
if ($user->role == 'administrator') {
$user_color = 'red';
} else if ($user->role == moderator) {
$user_color = '#00ff00'
}
<a style="color: <?= $user_color ?>">$user->username</a>
endforeach;
So either way, I end up mixing the two together. This is just one example where I need to adjust the HTML depending on some logic or conditions.
php html
php html
asked Nov 15 '18 at 9:37


LigaLiga
17819
17819
Just set$user_role
as the class in your html, then have css classes in your css class for each role?$user_color
seems unnecessary to me. If your only condition is "no PHP in a template" you can always use the likes of twig.
– Jon Stirling
Nov 15 '18 at 9:40
There's nothing wrong with putting a little PHP into "templating" files (e.g.<a href="<?= $user_class; ?>" ... />
) when it concerns display logic - that's pretty much what the.phtml
file extension is supposed to represent. Though you could use a templating engine; Symfony uses Twig for instance.
– CD001
Nov 15 '18 at 9:44
add a comment |
Just set$user_role
as the class in your html, then have css classes in your css class for each role?$user_color
seems unnecessary to me. If your only condition is "no PHP in a template" you can always use the likes of twig.
– Jon Stirling
Nov 15 '18 at 9:40
There's nothing wrong with putting a little PHP into "templating" files (e.g.<a href="<?= $user_class; ?>" ... />
) when it concerns display logic - that's pretty much what the.phtml
file extension is supposed to represent. Though you could use a templating engine; Symfony uses Twig for instance.
– CD001
Nov 15 '18 at 9:44
Just set
$user_role
as the class in your html, then have css classes in your css class for each role? $user_color
seems unnecessary to me. If your only condition is "no PHP in a template" you can always use the likes of twig.– Jon Stirling
Nov 15 '18 at 9:40
Just set
$user_role
as the class in your html, then have css classes in your css class for each role? $user_color
seems unnecessary to me. If your only condition is "no PHP in a template" you can always use the likes of twig.– Jon Stirling
Nov 15 '18 at 9:40
There's nothing wrong with putting a little PHP into "templating" files (e.g.
<a href="<?= $user_class; ?>" ... />
) when it concerns display logic - that's pretty much what the .phtml
file extension is supposed to represent. Though you could use a templating engine; Symfony uses Twig for instance.– CD001
Nov 15 '18 at 9:44
There's nothing wrong with putting a little PHP into "templating" files (e.g.
<a href="<?= $user_class; ?>" ... />
) when it concerns display logic - that's pretty much what the .phtml
file extension is supposed to represent. Though you could use a templating engine; Symfony uses Twig for instance.– CD001
Nov 15 '18 at 9:44
add a comment |
2 Answers
2
active
oldest
votes
You should use a template engine (eg. Twig)
This will also secure your app from XSS etc. by default escaping your variables.
This way you can seperate your PHP and HTML like this:
PHP
$template = $twig->load('index.html');
echo $template->render(array(
'user_color' => $user_color,
'user' => $user
));
HTML
<a style="color: {{ $user_color }}">{{$user->username}}</a>
add a comment |
There are few options for that:
- Add only classes to HTML using PHP, not CSS itself. Then use some CSS preprocessor like LESS for variables and additional functionality:
<a class="<?= $userRole; "></a>
In LESS:
@colorRed = #f00;
.administrator {
color: @colorRed;
}
- You can output CSS from PHP parser:
<link href="styles.php">
and there
.administrator {
color: <?= $colorRed; ?>
}
- Use Twig or other template engine
Since you are starting to learn structure, then consider using MVC principle: PHP code (a.k.a. controller
) does not have to be responsible for representation (a.k.a. view
). All UI stuff must be in Front-end side, not Back-end
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53316371%2fhow-to-properly-seperate-php-from-html-business-logic-from-view%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You should use a template engine (eg. Twig)
This will also secure your app from XSS etc. by default escaping your variables.
This way you can seperate your PHP and HTML like this:
PHP
$template = $twig->load('index.html');
echo $template->render(array(
'user_color' => $user_color,
'user' => $user
));
HTML
<a style="color: {{ $user_color }}">{{$user->username}}</a>
add a comment |
You should use a template engine (eg. Twig)
This will also secure your app from XSS etc. by default escaping your variables.
This way you can seperate your PHP and HTML like this:
PHP
$template = $twig->load('index.html');
echo $template->render(array(
'user_color' => $user_color,
'user' => $user
));
HTML
<a style="color: {{ $user_color }}">{{$user->username}}</a>
add a comment |
You should use a template engine (eg. Twig)
This will also secure your app from XSS etc. by default escaping your variables.
This way you can seperate your PHP and HTML like this:
PHP
$template = $twig->load('index.html');
echo $template->render(array(
'user_color' => $user_color,
'user' => $user
));
HTML
<a style="color: {{ $user_color }}">{{$user->username}}</a>
You should use a template engine (eg. Twig)
This will also secure your app from XSS etc. by default escaping your variables.
This way you can seperate your PHP and HTML like this:
PHP
$template = $twig->load('index.html');
echo $template->render(array(
'user_color' => $user_color,
'user' => $user
));
HTML
<a style="color: {{ $user_color }}">{{$user->username}}</a>
answered Nov 15 '18 at 9:45
JemoeEJemoeE
141212
141212
add a comment |
add a comment |
There are few options for that:
- Add only classes to HTML using PHP, not CSS itself. Then use some CSS preprocessor like LESS for variables and additional functionality:
<a class="<?= $userRole; "></a>
In LESS:
@colorRed = #f00;
.administrator {
color: @colorRed;
}
- You can output CSS from PHP parser:
<link href="styles.php">
and there
.administrator {
color: <?= $colorRed; ?>
}
- Use Twig or other template engine
Since you are starting to learn structure, then consider using MVC principle: PHP code (a.k.a. controller
) does not have to be responsible for representation (a.k.a. view
). All UI stuff must be in Front-end side, not Back-end
add a comment |
There are few options for that:
- Add only classes to HTML using PHP, not CSS itself. Then use some CSS preprocessor like LESS for variables and additional functionality:
<a class="<?= $userRole; "></a>
In LESS:
@colorRed = #f00;
.administrator {
color: @colorRed;
}
- You can output CSS from PHP parser:
<link href="styles.php">
and there
.administrator {
color: <?= $colorRed; ?>
}
- Use Twig or other template engine
Since you are starting to learn structure, then consider using MVC principle: PHP code (a.k.a. controller
) does not have to be responsible for representation (a.k.a. view
). All UI stuff must be in Front-end side, not Back-end
add a comment |
There are few options for that:
- Add only classes to HTML using PHP, not CSS itself. Then use some CSS preprocessor like LESS for variables and additional functionality:
<a class="<?= $userRole; "></a>
In LESS:
@colorRed = #f00;
.administrator {
color: @colorRed;
}
- You can output CSS from PHP parser:
<link href="styles.php">
and there
.administrator {
color: <?= $colorRed; ?>
}
- Use Twig or other template engine
Since you are starting to learn structure, then consider using MVC principle: PHP code (a.k.a. controller
) does not have to be responsible for representation (a.k.a. view
). All UI stuff must be in Front-end side, not Back-end
There are few options for that:
- Add only classes to HTML using PHP, not CSS itself. Then use some CSS preprocessor like LESS for variables and additional functionality:
<a class="<?= $userRole; "></a>
In LESS:
@colorRed = #f00;
.administrator {
color: @colorRed;
}
- You can output CSS from PHP parser:
<link href="styles.php">
and there
.administrator {
color: <?= $colorRed; ?>
}
- Use Twig or other template engine
Since you are starting to learn structure, then consider using MVC principle: PHP code (a.k.a. controller
) does not have to be responsible for representation (a.k.a. view
). All UI stuff must be in Front-end side, not Back-end
answered Nov 15 '18 at 9:55


JustinasJustinas
27.6k33560
27.6k33560
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53316371%2fhow-to-properly-seperate-php-from-html-business-logic-from-view%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
RxSXJ,BoFOvvsuoeIku FzREAxq
Just set
$user_role
as the class in your html, then have css classes in your css class for each role?$user_color
seems unnecessary to me. If your only condition is "no PHP in a template" you can always use the likes of twig.– Jon Stirling
Nov 15 '18 at 9:40
There's nothing wrong with putting a little PHP into "templating" files (e.g.
<a href="<?= $user_class; ?>" ... />
) when it concerns display logic - that's pretty much what the.phtml
file extension is supposed to represent. Though you could use a templating engine; Symfony uses Twig for instance.– CD001
Nov 15 '18 at 9:44