Recursive data on MySQL 5.5
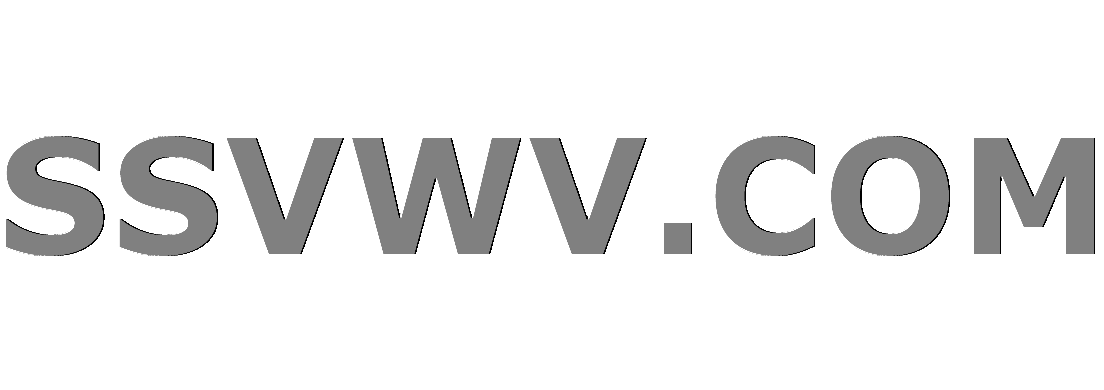
Multi tool use
I have a table structure (that cannot be changed) like this:
CREATE TABLE people(id INT PRIMARY KEY, name VARCHAR(150));
CREATE TABLE positions(id INT PRIMARY KEY, name VARCHAR(100));
CREATE TABLE positionAssignments(id INT PRIMARY KEY, fkPosition INT,
fkAssignedPerson INT, fkAssignedPosition INT, startDate DATETIME,
endDate DATETIME,
FOREIGN KEY(fkAssignedPerson) REFERENCES people(id),
FOREIGN KEY(fkAssignedPosition REFERENCES positions(id));
Being in positionAssignments
:
fkPosition
is which position is assigned to the person, or another position.
fkAssignedPerson
the person assigned to the position.
fkAssignedPosition
the position assigned to another.
My problem is that I need to obtain all positions of a person. As this structure can be recursive, I need create a SQL that returns up to 5 levels depth. Data can be as follows:
people positions
| id | name | | id | name |
+----+---------+ +----+-------------+
| 1 | Alice | | 10 | Position 1 |
+----+---------+ +----+-------------+
| 2 | Bob | | 20 | Position 2 |
+----+---------+ +----+-------------+
| 3 | Charlie | | 30 | Position 3 |
+----+---------+ +----+-------------+
| 4 | Daniel | | 40 | Position 4 |
+----+---------+ +----+-------------+
| 50 | Position 5 |
+----+-------------+
| 60 | Position 6 |
+----+-------------+
positionAssignments
| id | fkPosition | fkAssignedPerson | fkAssignedPosition| startDate | endDate |
+----+------------+------------------+-------------------+------------+---------+
| 1 | 10 | 1 | null | 2018-01-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 2 | 20 | 2 | null | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 3 | 20 | null | 30 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 4 | 30 | 3 | null | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 5 | 40 | null | 20 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 6 | 50 | null | 40 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 7 | 60 | null | 50 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 8 | 60 | null | 10 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 9 | 60 | 4 | null | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
Whis this structure, Alice belongs to Position 1, Bob belongs to Position 2 and Charlie to Position 2 and 3.
My question: I've done a SQL obtaining all positions of a person using UNION
like this:
SELECT pe.id, pa1.fkPosition, pa1.id, pa1.startDate, pa1.endDate
FROM people pe
INNER JOIN positionAssignments pa1
ON pe.id = pa1.fkAssignedPerson
UNION
SELECT pe.id, pa2.fkPosition, pa2.id, pa2.startDate, pa2.endDate
FROM people pe
INNER JOIN positionAssignments pa1
ON pe.id = pa1.fkAssignedPerson
INNER JOIN positionAssignments pa2
ON pa1.fkPosition = pa2.fkAssignedPosition
-- And so on...
Is there any better way to do it, having in mind that I use MySQL 5.5 and I'd want to store this in a view (that should use merge algorithm)?
A desired output would be:
Expected Result
| idPerson | idPosition | idAssignment | startDate | endDate |
+----------+------------+--------------+------------+---------+
| 1 | 10 | 1 | 2018-01-01 | null |
+----------+------------+--------------+------------+---------+
| 1 | 60 | 8 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 2 | 20 | 2 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 2 | 40 | 5 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 2 | 50 | 6 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 2 | 60 | 7 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 20 | 3 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 30 | 4 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 40 | 5 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 50 | 6 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 60 | 7 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 4 | 60 | 9 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
EDIT: Added new test data
mysql
|
show 7 more comments
I have a table structure (that cannot be changed) like this:
CREATE TABLE people(id INT PRIMARY KEY, name VARCHAR(150));
CREATE TABLE positions(id INT PRIMARY KEY, name VARCHAR(100));
CREATE TABLE positionAssignments(id INT PRIMARY KEY, fkPosition INT,
fkAssignedPerson INT, fkAssignedPosition INT, startDate DATETIME,
endDate DATETIME,
FOREIGN KEY(fkAssignedPerson) REFERENCES people(id),
FOREIGN KEY(fkAssignedPosition REFERENCES positions(id));
Being in positionAssignments
:
fkPosition
is which position is assigned to the person, or another position.
fkAssignedPerson
the person assigned to the position.
fkAssignedPosition
the position assigned to another.
My problem is that I need to obtain all positions of a person. As this structure can be recursive, I need create a SQL that returns up to 5 levels depth. Data can be as follows:
people positions
| id | name | | id | name |
+----+---------+ +----+-------------+
| 1 | Alice | | 10 | Position 1 |
+----+---------+ +----+-------------+
| 2 | Bob | | 20 | Position 2 |
+----+---------+ +----+-------------+
| 3 | Charlie | | 30 | Position 3 |
+----+---------+ +----+-------------+
| 4 | Daniel | | 40 | Position 4 |
+----+---------+ +----+-------------+
| 50 | Position 5 |
+----+-------------+
| 60 | Position 6 |
+----+-------------+
positionAssignments
| id | fkPosition | fkAssignedPerson | fkAssignedPosition| startDate | endDate |
+----+------------+------------------+-------------------+------------+---------+
| 1 | 10 | 1 | null | 2018-01-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 2 | 20 | 2 | null | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 3 | 20 | null | 30 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 4 | 30 | 3 | null | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 5 | 40 | null | 20 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 6 | 50 | null | 40 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 7 | 60 | null | 50 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 8 | 60 | null | 10 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 9 | 60 | 4 | null | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
Whis this structure, Alice belongs to Position 1, Bob belongs to Position 2 and Charlie to Position 2 and 3.
My question: I've done a SQL obtaining all positions of a person using UNION
like this:
SELECT pe.id, pa1.fkPosition, pa1.id, pa1.startDate, pa1.endDate
FROM people pe
INNER JOIN positionAssignments pa1
ON pe.id = pa1.fkAssignedPerson
UNION
SELECT pe.id, pa2.fkPosition, pa2.id, pa2.startDate, pa2.endDate
FROM people pe
INNER JOIN positionAssignments pa1
ON pe.id = pa1.fkAssignedPerson
INNER JOIN positionAssignments pa2
ON pa1.fkPosition = pa2.fkAssignedPosition
-- And so on...
Is there any better way to do it, having in mind that I use MySQL 5.5 and I'd want to store this in a view (that should use merge algorithm)?
A desired output would be:
Expected Result
| idPerson | idPosition | idAssignment | startDate | endDate |
+----------+------------+--------------+------------+---------+
| 1 | 10 | 1 | 2018-01-01 | null |
+----------+------------+--------------+------------+---------+
| 1 | 60 | 8 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 2 | 20 | 2 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 2 | 40 | 5 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 2 | 50 | 6 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 2 | 60 | 7 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 20 | 3 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 30 | 4 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 40 | 5 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 50 | 6 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 60 | 7 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 4 | 60 | 9 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
EDIT: Added new test data
mysql
Your second statement lacks a FROM; does it even work?
– Caius Jard
Nov 15 '18 at 9:44
@CaiusJard An error doing copy-paste
– Shirkam
Nov 15 '18 at 9:52
1
Have you seen stackoverflow.com/questions/20215744/…
– Caius Jard
Nov 15 '18 at 9:55
@CaiusJard Yes, but I think answers aren't applicable to this question.
– Shirkam
Nov 15 '18 at 10:07
Sorry, i just picked on the recursion element, but having re-reviewed this I can't see how it's recursive from the data shown
– Caius Jard
Nov 15 '18 at 10:18
|
show 7 more comments
I have a table structure (that cannot be changed) like this:
CREATE TABLE people(id INT PRIMARY KEY, name VARCHAR(150));
CREATE TABLE positions(id INT PRIMARY KEY, name VARCHAR(100));
CREATE TABLE positionAssignments(id INT PRIMARY KEY, fkPosition INT,
fkAssignedPerson INT, fkAssignedPosition INT, startDate DATETIME,
endDate DATETIME,
FOREIGN KEY(fkAssignedPerson) REFERENCES people(id),
FOREIGN KEY(fkAssignedPosition REFERENCES positions(id));
Being in positionAssignments
:
fkPosition
is which position is assigned to the person, or another position.
fkAssignedPerson
the person assigned to the position.
fkAssignedPosition
the position assigned to another.
My problem is that I need to obtain all positions of a person. As this structure can be recursive, I need create a SQL that returns up to 5 levels depth. Data can be as follows:
people positions
| id | name | | id | name |
+----+---------+ +----+-------------+
| 1 | Alice | | 10 | Position 1 |
+----+---------+ +----+-------------+
| 2 | Bob | | 20 | Position 2 |
+----+---------+ +----+-------------+
| 3 | Charlie | | 30 | Position 3 |
+----+---------+ +----+-------------+
| 4 | Daniel | | 40 | Position 4 |
+----+---------+ +----+-------------+
| 50 | Position 5 |
+----+-------------+
| 60 | Position 6 |
+----+-------------+
positionAssignments
| id | fkPosition | fkAssignedPerson | fkAssignedPosition| startDate | endDate |
+----+------------+------------------+-------------------+------------+---------+
| 1 | 10 | 1 | null | 2018-01-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 2 | 20 | 2 | null | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 3 | 20 | null | 30 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 4 | 30 | 3 | null | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 5 | 40 | null | 20 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 6 | 50 | null | 40 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 7 | 60 | null | 50 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 8 | 60 | null | 10 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 9 | 60 | 4 | null | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
Whis this structure, Alice belongs to Position 1, Bob belongs to Position 2 and Charlie to Position 2 and 3.
My question: I've done a SQL obtaining all positions of a person using UNION
like this:
SELECT pe.id, pa1.fkPosition, pa1.id, pa1.startDate, pa1.endDate
FROM people pe
INNER JOIN positionAssignments pa1
ON pe.id = pa1.fkAssignedPerson
UNION
SELECT pe.id, pa2.fkPosition, pa2.id, pa2.startDate, pa2.endDate
FROM people pe
INNER JOIN positionAssignments pa1
ON pe.id = pa1.fkAssignedPerson
INNER JOIN positionAssignments pa2
ON pa1.fkPosition = pa2.fkAssignedPosition
-- And so on...
Is there any better way to do it, having in mind that I use MySQL 5.5 and I'd want to store this in a view (that should use merge algorithm)?
A desired output would be:
Expected Result
| idPerson | idPosition | idAssignment | startDate | endDate |
+----------+------------+--------------+------------+---------+
| 1 | 10 | 1 | 2018-01-01 | null |
+----------+------------+--------------+------------+---------+
| 1 | 60 | 8 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 2 | 20 | 2 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 2 | 40 | 5 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 2 | 50 | 6 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 2 | 60 | 7 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 20 | 3 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 30 | 4 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 40 | 5 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 50 | 6 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 60 | 7 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 4 | 60 | 9 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
EDIT: Added new test data
mysql
I have a table structure (that cannot be changed) like this:
CREATE TABLE people(id INT PRIMARY KEY, name VARCHAR(150));
CREATE TABLE positions(id INT PRIMARY KEY, name VARCHAR(100));
CREATE TABLE positionAssignments(id INT PRIMARY KEY, fkPosition INT,
fkAssignedPerson INT, fkAssignedPosition INT, startDate DATETIME,
endDate DATETIME,
FOREIGN KEY(fkAssignedPerson) REFERENCES people(id),
FOREIGN KEY(fkAssignedPosition REFERENCES positions(id));
Being in positionAssignments
:
fkPosition
is which position is assigned to the person, or another position.
fkAssignedPerson
the person assigned to the position.
fkAssignedPosition
the position assigned to another.
My problem is that I need to obtain all positions of a person. As this structure can be recursive, I need create a SQL that returns up to 5 levels depth. Data can be as follows:
people positions
| id | name | | id | name |
+----+---------+ +----+-------------+
| 1 | Alice | | 10 | Position 1 |
+----+---------+ +----+-------------+
| 2 | Bob | | 20 | Position 2 |
+----+---------+ +----+-------------+
| 3 | Charlie | | 30 | Position 3 |
+----+---------+ +----+-------------+
| 4 | Daniel | | 40 | Position 4 |
+----+---------+ +----+-------------+
| 50 | Position 5 |
+----+-------------+
| 60 | Position 6 |
+----+-------------+
positionAssignments
| id | fkPosition | fkAssignedPerson | fkAssignedPosition| startDate | endDate |
+----+------------+------------------+-------------------+------------+---------+
| 1 | 10 | 1 | null | 2018-01-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 2 | 20 | 2 | null | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 3 | 20 | null | 30 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 4 | 30 | 3 | null | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 5 | 40 | null | 20 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 6 | 50 | null | 40 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 7 | 60 | null | 50 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 8 | 60 | null | 10 | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
| 9 | 60 | 4 | null | 2018-02-01 | null |
+----+------------+------------------+-------------------+------------+---------+
Whis this structure, Alice belongs to Position 1, Bob belongs to Position 2 and Charlie to Position 2 and 3.
My question: I've done a SQL obtaining all positions of a person using UNION
like this:
SELECT pe.id, pa1.fkPosition, pa1.id, pa1.startDate, pa1.endDate
FROM people pe
INNER JOIN positionAssignments pa1
ON pe.id = pa1.fkAssignedPerson
UNION
SELECT pe.id, pa2.fkPosition, pa2.id, pa2.startDate, pa2.endDate
FROM people pe
INNER JOIN positionAssignments pa1
ON pe.id = pa1.fkAssignedPerson
INNER JOIN positionAssignments pa2
ON pa1.fkPosition = pa2.fkAssignedPosition
-- And so on...
Is there any better way to do it, having in mind that I use MySQL 5.5 and I'd want to store this in a view (that should use merge algorithm)?
A desired output would be:
Expected Result
| idPerson | idPosition | idAssignment | startDate | endDate |
+----------+------------+--------------+------------+---------+
| 1 | 10 | 1 | 2018-01-01 | null |
+----------+------------+--------------+------------+---------+
| 1 | 60 | 8 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 2 | 20 | 2 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 2 | 40 | 5 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 2 | 50 | 6 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 2 | 60 | 7 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 20 | 3 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 30 | 4 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 40 | 5 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 50 | 6 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 3 | 60 | 7 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
| 4 | 60 | 9 | 2018-02-01 | null |
+----------+------------+--------------+------------+---------+
EDIT: Added new test data
mysql
mysql
edited Nov 15 '18 at 17:08
Shirkam
asked Nov 15 '18 at 9:42
ShirkamShirkam
625516
625516
Your second statement lacks a FROM; does it even work?
– Caius Jard
Nov 15 '18 at 9:44
@CaiusJard An error doing copy-paste
– Shirkam
Nov 15 '18 at 9:52
1
Have you seen stackoverflow.com/questions/20215744/…
– Caius Jard
Nov 15 '18 at 9:55
@CaiusJard Yes, but I think answers aren't applicable to this question.
– Shirkam
Nov 15 '18 at 10:07
Sorry, i just picked on the recursion element, but having re-reviewed this I can't see how it's recursive from the data shown
– Caius Jard
Nov 15 '18 at 10:18
|
show 7 more comments
Your second statement lacks a FROM; does it even work?
– Caius Jard
Nov 15 '18 at 9:44
@CaiusJard An error doing copy-paste
– Shirkam
Nov 15 '18 at 9:52
1
Have you seen stackoverflow.com/questions/20215744/…
– Caius Jard
Nov 15 '18 at 9:55
@CaiusJard Yes, but I think answers aren't applicable to this question.
– Shirkam
Nov 15 '18 at 10:07
Sorry, i just picked on the recursion element, but having re-reviewed this I can't see how it's recursive from the data shown
– Caius Jard
Nov 15 '18 at 10:18
Your second statement lacks a FROM; does it even work?
– Caius Jard
Nov 15 '18 at 9:44
Your second statement lacks a FROM; does it even work?
– Caius Jard
Nov 15 '18 at 9:44
@CaiusJard An error doing copy-paste
– Shirkam
Nov 15 '18 at 9:52
@CaiusJard An error doing copy-paste
– Shirkam
Nov 15 '18 at 9:52
1
1
Have you seen stackoverflow.com/questions/20215744/…
– Caius Jard
Nov 15 '18 at 9:55
Have you seen stackoverflow.com/questions/20215744/…
– Caius Jard
Nov 15 '18 at 9:55
@CaiusJard Yes, but I think answers aren't applicable to this question.
– Shirkam
Nov 15 '18 at 10:07
@CaiusJard Yes, but I think answers aren't applicable to this question.
– Shirkam
Nov 15 '18 at 10:07
Sorry, i just picked on the recursion element, but having re-reviewed this I can't see how it's recursive from the data shown
– Caius Jard
Nov 15 '18 at 10:18
Sorry, i just picked on the recursion element, but having re-reviewed this I can't see how it's recursive from the data shown
– Caius Jard
Nov 15 '18 at 10:18
|
show 7 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53316474%2frecursive-data-on-mysql-5-5%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53316474%2frecursive-data-on-mysql-5-5%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
IOj,l2J3W00F,icW4CiR MXbT 0we 3,Ds 7JcLi,wMAzEwKrrVOPD0 zPRGmu2rJp8Eo
Your second statement lacks a FROM; does it even work?
– Caius Jard
Nov 15 '18 at 9:44
@CaiusJard An error doing copy-paste
– Shirkam
Nov 15 '18 at 9:52
1
Have you seen stackoverflow.com/questions/20215744/…
– Caius Jard
Nov 15 '18 at 9:55
@CaiusJard Yes, but I think answers aren't applicable to this question.
– Shirkam
Nov 15 '18 at 10:07
Sorry, i just picked on the recursion element, but having re-reviewed this I can't see how it's recursive from the data shown
– Caius Jard
Nov 15 '18 at 10:18