Join Two Array based on Index and Index Value using Linq Query Expression Syntax
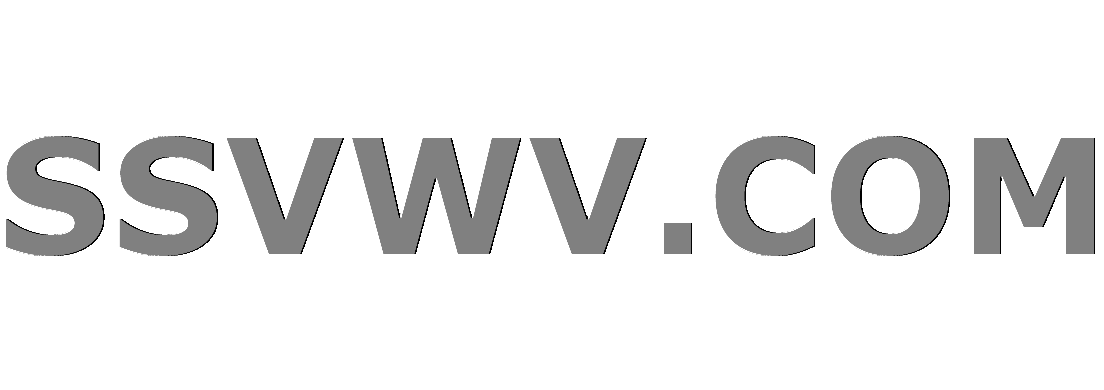
Multi tool use
I want to join two arrays with one array containing index value and a second array containing index position.
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine"};
int nums = new { 2, 32, 70 };
The output I want:
2 Two
32 Three-Two
70 Seven-Zero
I tried below query
var strValuList = (from d in nums
from p in numStrings.Select((value, index) => new { value = value, index = index })
where p.index == d
select new { num = d, p.value}).ToList();
but it returns only
2 Two
c# linq
|
show 5 more comments
I want to join two arrays with one array containing index value and a second array containing index position.
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine"};
int nums = new { 2, 32, 70 };
The output I want:
2 Two
32 Three-Two
70 Seven-Zero
I tried below query
var strValuList = (from d in nums
from p in numStrings.Select((value, index) => new { value = value, index = index })
where p.index == d
select new { num = d, p.value}).ToList();
but it returns only
2 Two
c# linq
So.... what did you try? Looks like an assignment. Just try yourself, else you won't learn anything. We can make up the most beautiful linq queries/string joins that solves this. But are you able to read/understand them?
– J. van Langen
Nov 15 '18 at 10:59
List<string> strValuList = (from d in nums from p in numStrings.Select((value, index) => new { value = value, index = index }) where p.index == d select p.value).ToList();
– Hemendr
Nov 15 '18 at 11:02
You might update your question instead of commenting ;-)
– J. van Langen
Nov 15 '18 at 11:03
1
Doing this in one query is more complicated than necessary. Hint:do { yield return numStrings[n % 10]; n /= 10; } while (n != 0)
. (.ToString
and going over each character is another approach, though it might be considered "cheating":nums.Select(n => ("" + n).Select(c => numStrings[c - '0']))
.)
– Jeroen Mostert
Nov 15 '18 at 11:10
1
The loop you'd do to extract the digits from a number can certainly be written in a LINQ query, and you can join that up with the digit array. It's just not particularly sane to do it this way.
– Jeroen Mostert
Nov 15 '18 at 11:30
|
show 5 more comments
I want to join two arrays with one array containing index value and a second array containing index position.
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine"};
int nums = new { 2, 32, 70 };
The output I want:
2 Two
32 Three-Two
70 Seven-Zero
I tried below query
var strValuList = (from d in nums
from p in numStrings.Select((value, index) => new { value = value, index = index })
where p.index == d
select new { num = d, p.value}).ToList();
but it returns only
2 Two
c# linq
I want to join two arrays with one array containing index value and a second array containing index position.
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine"};
int nums = new { 2, 32, 70 };
The output I want:
2 Two
32 Three-Two
70 Seven-Zero
I tried below query
var strValuList = (from d in nums
from p in numStrings.Select((value, index) => new { value = value, index = index })
where p.index == d
select new { num = d, p.value}).ToList();
but it returns only
2 Two
c# linq
c# linq
edited Nov 15 '18 at 11:06
Hemendr
asked Nov 15 '18 at 10:57
HemendrHemendr
18218
18218
So.... what did you try? Looks like an assignment. Just try yourself, else you won't learn anything. We can make up the most beautiful linq queries/string joins that solves this. But are you able to read/understand them?
– J. van Langen
Nov 15 '18 at 10:59
List<string> strValuList = (from d in nums from p in numStrings.Select((value, index) => new { value = value, index = index }) where p.index == d select p.value).ToList();
– Hemendr
Nov 15 '18 at 11:02
You might update your question instead of commenting ;-)
– J. van Langen
Nov 15 '18 at 11:03
1
Doing this in one query is more complicated than necessary. Hint:do { yield return numStrings[n % 10]; n /= 10; } while (n != 0)
. (.ToString
and going over each character is another approach, though it might be considered "cheating":nums.Select(n => ("" + n).Select(c => numStrings[c - '0']))
.)
– Jeroen Mostert
Nov 15 '18 at 11:10
1
The loop you'd do to extract the digits from a number can certainly be written in a LINQ query, and you can join that up with the digit array. It's just not particularly sane to do it this way.
– Jeroen Mostert
Nov 15 '18 at 11:30
|
show 5 more comments
So.... what did you try? Looks like an assignment. Just try yourself, else you won't learn anything. We can make up the most beautiful linq queries/string joins that solves this. But are you able to read/understand them?
– J. van Langen
Nov 15 '18 at 10:59
List<string> strValuList = (from d in nums from p in numStrings.Select((value, index) => new { value = value, index = index }) where p.index == d select p.value).ToList();
– Hemendr
Nov 15 '18 at 11:02
You might update your question instead of commenting ;-)
– J. van Langen
Nov 15 '18 at 11:03
1
Doing this in one query is more complicated than necessary. Hint:do { yield return numStrings[n % 10]; n /= 10; } while (n != 0)
. (.ToString
and going over each character is another approach, though it might be considered "cheating":nums.Select(n => ("" + n).Select(c => numStrings[c - '0']))
.)
– Jeroen Mostert
Nov 15 '18 at 11:10
1
The loop you'd do to extract the digits from a number can certainly be written in a LINQ query, and you can join that up with the digit array. It's just not particularly sane to do it this way.
– Jeroen Mostert
Nov 15 '18 at 11:30
So.... what did you try? Looks like an assignment. Just try yourself, else you won't learn anything. We can make up the most beautiful linq queries/string joins that solves this. But are you able to read/understand them?
– J. van Langen
Nov 15 '18 at 10:59
So.... what did you try? Looks like an assignment. Just try yourself, else you won't learn anything. We can make up the most beautiful linq queries/string joins that solves this. But are you able to read/understand them?
– J. van Langen
Nov 15 '18 at 10:59
List<string> strValuList = (from d in nums from p in numStrings.Select((value, index) => new { value = value, index = index }) where p.index == d select p.value).ToList();
– Hemendr
Nov 15 '18 at 11:02
List<string> strValuList = (from d in nums from p in numStrings.Select((value, index) => new { value = value, index = index }) where p.index == d select p.value).ToList();
– Hemendr
Nov 15 '18 at 11:02
You might update your question instead of commenting ;-)
– J. van Langen
Nov 15 '18 at 11:03
You might update your question instead of commenting ;-)
– J. van Langen
Nov 15 '18 at 11:03
1
1
Doing this in one query is more complicated than necessary. Hint:
do { yield return numStrings[n % 10]; n /= 10; } while (n != 0)
. (.ToString
and going over each character is another approach, though it might be considered "cheating": nums.Select(n => ("" + n).Select(c => numStrings[c - '0']))
.)– Jeroen Mostert
Nov 15 '18 at 11:10
Doing this in one query is more complicated than necessary. Hint:
do { yield return numStrings[n % 10]; n /= 10; } while (n != 0)
. (.ToString
and going over each character is another approach, though it might be considered "cheating": nums.Select(n => ("" + n).Select(c => numStrings[c - '0']))
.)– Jeroen Mostert
Nov 15 '18 at 11:10
1
1
The loop you'd do to extract the digits from a number can certainly be written in a LINQ query, and you can join that up with the digit array. It's just not particularly sane to do it this way.
– Jeroen Mostert
Nov 15 '18 at 11:30
The loop you'd do to extract the digits from a number can certainly be written in a LINQ query, and you can join that up with the digit array. It's just not particularly sane to do it this way.
– Jeroen Mostert
Nov 15 '18 at 11:30
|
show 5 more comments
4 Answers
4
active
oldest
votes
Try it yourself first, then you can look at answer:
Hint:
IEnumerable<int> SplitNum(int num)
{
while (num > 0)
{
yield return num % 10;
num /= 10;
}
}
Answer:
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine" };
int nums = new { 2, 32, 70 };
(Hover mouse on yellow part for the answer [I will remove spoiler part after some time])
string result = nums.Select(n => string.Join("-", SplitNum(n).Reverse().Select(i => numStrings[i]))).ToArray();
DotNetFiddle example
It's nice but complicated to understand. I upvote it as I've learned from your answer.
– Dmitry Stepanov
Nov 15 '18 at 12:19
@DmitryS Well you can always usestring/ToString()
s,char
s andint.Parse
to get desired result, but I felt unnatural (maybe it will feel less unnatural if you use$"{num}"
instead ofnum.ToString()
, but anyway too muchstring->char->string->int
conversion) to do that much actions for a simple math task - to get separate digits from number. For the linq part, it is not too complicated, OP just need to read carefully and understand each part of that expression.
– SeM
Nov 15 '18 at 12:26
add a comment |
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine"};
int nums = new { 2, 32, 70 };
var dict = new Dictionary<int, string>();
foreach(var n in nums)
{
var str = string.Join("-", n.ToString().Select(s => numStrings[Convert.ToInt32(s.ToString())]));
dict[n] = str;
}
Why you needif (n < 10)
part?
– SeM
Nov 15 '18 at 11:38
Damn it, you're right -)). I will edit the answer
– Dmitry Stepanov
Nov 15 '18 at 11:40
add a comment |
I solve it without much complexity. Thanks, @Jeroen Mostert for your advice
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine" };
int nums = new { 2, 32, 70 };
(from d in nums
select new
{
num = d,
parts = d.ToString().Select(o=> Convert.ToInt32(o.ToString())),
parts2 = d.ToString().Select(o => numStrings[Convert.ToInt32(o.ToString())]),
parts3 = string.Join("-",d.ToString().Select(o => numStrings[Convert.ToInt32(o.ToString())]).ToArray())
}).Dump();
Output in LinqPad:
You do realizedict
is semantically equal tonumStrings
, right?dict[x] == numStrings[x]
for allx
between0
and9
, inclusive.
– Jeroen Mostert
Nov 15 '18 at 12:23
But given what you really want to be looking up is thechar
s from the string then perhaps a dictionary with keytypechar
would have been better and saved a bunch of converting chars to ints...
– Chris
Nov 15 '18 at 12:28
add a comment |
This is one of the many ways to do it:
void Main()
{
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine" };
int nums = new { 2, 32, 70 };
Func<int, string> numToWord = (x) => {
List<string> words = new List<string>();
do {
words.Add( numStrings[x%10] );
x /= 10;
} while (x > 0);
var w = words.Reverse<string>();
return string.Join("-",w);
};
var result = nums.Select(n => numToWord(n));
foreach (var element in result)
{
Console.WriteLine(element);
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53317918%2fjoin-two-array-based-on-index-and-index-value-using-linq-query-expression-syntax%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
Try it yourself first, then you can look at answer:
Hint:
IEnumerable<int> SplitNum(int num)
{
while (num > 0)
{
yield return num % 10;
num /= 10;
}
}
Answer:
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine" };
int nums = new { 2, 32, 70 };
(Hover mouse on yellow part for the answer [I will remove spoiler part after some time])
string result = nums.Select(n => string.Join("-", SplitNum(n).Reverse().Select(i => numStrings[i]))).ToArray();
DotNetFiddle example
It's nice but complicated to understand. I upvote it as I've learned from your answer.
– Dmitry Stepanov
Nov 15 '18 at 12:19
@DmitryS Well you can always usestring/ToString()
s,char
s andint.Parse
to get desired result, but I felt unnatural (maybe it will feel less unnatural if you use$"{num}"
instead ofnum.ToString()
, but anyway too muchstring->char->string->int
conversion) to do that much actions for a simple math task - to get separate digits from number. For the linq part, it is not too complicated, OP just need to read carefully and understand each part of that expression.
– SeM
Nov 15 '18 at 12:26
add a comment |
Try it yourself first, then you can look at answer:
Hint:
IEnumerable<int> SplitNum(int num)
{
while (num > 0)
{
yield return num % 10;
num /= 10;
}
}
Answer:
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine" };
int nums = new { 2, 32, 70 };
(Hover mouse on yellow part for the answer [I will remove spoiler part after some time])
string result = nums.Select(n => string.Join("-", SplitNum(n).Reverse().Select(i => numStrings[i]))).ToArray();
DotNetFiddle example
It's nice but complicated to understand. I upvote it as I've learned from your answer.
– Dmitry Stepanov
Nov 15 '18 at 12:19
@DmitryS Well you can always usestring/ToString()
s,char
s andint.Parse
to get desired result, but I felt unnatural (maybe it will feel less unnatural if you use$"{num}"
instead ofnum.ToString()
, but anyway too muchstring->char->string->int
conversion) to do that much actions for a simple math task - to get separate digits from number. For the linq part, it is not too complicated, OP just need to read carefully and understand each part of that expression.
– SeM
Nov 15 '18 at 12:26
add a comment |
Try it yourself first, then you can look at answer:
Hint:
IEnumerable<int> SplitNum(int num)
{
while (num > 0)
{
yield return num % 10;
num /= 10;
}
}
Answer:
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine" };
int nums = new { 2, 32, 70 };
(Hover mouse on yellow part for the answer [I will remove spoiler part after some time])
string result = nums.Select(n => string.Join("-", SplitNum(n).Reverse().Select(i => numStrings[i]))).ToArray();
DotNetFiddle example
Try it yourself first, then you can look at answer:
Hint:
IEnumerable<int> SplitNum(int num)
{
while (num > 0)
{
yield return num % 10;
num /= 10;
}
}
Answer:
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine" };
int nums = new { 2, 32, 70 };
(Hover mouse on yellow part for the answer [I will remove spoiler part after some time])
string result = nums.Select(n => string.Join("-", SplitNum(n).Reverse().Select(i => numStrings[i]))).ToArray();
DotNetFiddle example
edited Nov 15 '18 at 12:29
answered Nov 15 '18 at 11:52


SeMSeM
4,62011630
4,62011630
It's nice but complicated to understand. I upvote it as I've learned from your answer.
– Dmitry Stepanov
Nov 15 '18 at 12:19
@DmitryS Well you can always usestring/ToString()
s,char
s andint.Parse
to get desired result, but I felt unnatural (maybe it will feel less unnatural if you use$"{num}"
instead ofnum.ToString()
, but anyway too muchstring->char->string->int
conversion) to do that much actions for a simple math task - to get separate digits from number. For the linq part, it is not too complicated, OP just need to read carefully and understand each part of that expression.
– SeM
Nov 15 '18 at 12:26
add a comment |
It's nice but complicated to understand. I upvote it as I've learned from your answer.
– Dmitry Stepanov
Nov 15 '18 at 12:19
@DmitryS Well you can always usestring/ToString()
s,char
s andint.Parse
to get desired result, but I felt unnatural (maybe it will feel less unnatural if you use$"{num}"
instead ofnum.ToString()
, but anyway too muchstring->char->string->int
conversion) to do that much actions for a simple math task - to get separate digits from number. For the linq part, it is not too complicated, OP just need to read carefully and understand each part of that expression.
– SeM
Nov 15 '18 at 12:26
It's nice but complicated to understand. I upvote it as I've learned from your answer.
– Dmitry Stepanov
Nov 15 '18 at 12:19
It's nice but complicated to understand. I upvote it as I've learned from your answer.
– Dmitry Stepanov
Nov 15 '18 at 12:19
@DmitryS Well you can always use
string/ToString()
s, char
s and int.Parse
to get desired result, but I felt unnatural (maybe it will feel less unnatural if you use $"{num}"
instead of num.ToString()
, but anyway too much string->char->string->int
conversion) to do that much actions for a simple math task - to get separate digits from number. For the linq part, it is not too complicated, OP just need to read carefully and understand each part of that expression.– SeM
Nov 15 '18 at 12:26
@DmitryS Well you can always use
string/ToString()
s, char
s and int.Parse
to get desired result, but I felt unnatural (maybe it will feel less unnatural if you use $"{num}"
instead of num.ToString()
, but anyway too much string->char->string->int
conversion) to do that much actions for a simple math task - to get separate digits from number. For the linq part, it is not too complicated, OP just need to read carefully and understand each part of that expression.– SeM
Nov 15 '18 at 12:26
add a comment |
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine"};
int nums = new { 2, 32, 70 };
var dict = new Dictionary<int, string>();
foreach(var n in nums)
{
var str = string.Join("-", n.ToString().Select(s => numStrings[Convert.ToInt32(s.ToString())]));
dict[n] = str;
}
Why you needif (n < 10)
part?
– SeM
Nov 15 '18 at 11:38
Damn it, you're right -)). I will edit the answer
– Dmitry Stepanov
Nov 15 '18 at 11:40
add a comment |
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine"};
int nums = new { 2, 32, 70 };
var dict = new Dictionary<int, string>();
foreach(var n in nums)
{
var str = string.Join("-", n.ToString().Select(s => numStrings[Convert.ToInt32(s.ToString())]));
dict[n] = str;
}
Why you needif (n < 10)
part?
– SeM
Nov 15 '18 at 11:38
Damn it, you're right -)). I will edit the answer
– Dmitry Stepanov
Nov 15 '18 at 11:40
add a comment |
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine"};
int nums = new { 2, 32, 70 };
var dict = new Dictionary<int, string>();
foreach(var n in nums)
{
var str = string.Join("-", n.ToString().Select(s => numStrings[Convert.ToInt32(s.ToString())]));
dict[n] = str;
}
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine"};
int nums = new { 2, 32, 70 };
var dict = new Dictionary<int, string>();
foreach(var n in nums)
{
var str = string.Join("-", n.ToString().Select(s => numStrings[Convert.ToInt32(s.ToString())]));
dict[n] = str;
}
edited Nov 15 '18 at 11:40
answered Nov 15 '18 at 11:36


Dmitry StepanovDmitry Stepanov
1,110720
1,110720
Why you needif (n < 10)
part?
– SeM
Nov 15 '18 at 11:38
Damn it, you're right -)). I will edit the answer
– Dmitry Stepanov
Nov 15 '18 at 11:40
add a comment |
Why you needif (n < 10)
part?
– SeM
Nov 15 '18 at 11:38
Damn it, you're right -)). I will edit the answer
– Dmitry Stepanov
Nov 15 '18 at 11:40
Why you need
if (n < 10)
part?– SeM
Nov 15 '18 at 11:38
Why you need
if (n < 10)
part?– SeM
Nov 15 '18 at 11:38
Damn it, you're right -)). I will edit the answer
– Dmitry Stepanov
Nov 15 '18 at 11:40
Damn it, you're right -)). I will edit the answer
– Dmitry Stepanov
Nov 15 '18 at 11:40
add a comment |
I solve it without much complexity. Thanks, @Jeroen Mostert for your advice
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine" };
int nums = new { 2, 32, 70 };
(from d in nums
select new
{
num = d,
parts = d.ToString().Select(o=> Convert.ToInt32(o.ToString())),
parts2 = d.ToString().Select(o => numStrings[Convert.ToInt32(o.ToString())]),
parts3 = string.Join("-",d.ToString().Select(o => numStrings[Convert.ToInt32(o.ToString())]).ToArray())
}).Dump();
Output in LinqPad:
You do realizedict
is semantically equal tonumStrings
, right?dict[x] == numStrings[x]
for allx
between0
and9
, inclusive.
– Jeroen Mostert
Nov 15 '18 at 12:23
But given what you really want to be looking up is thechar
s from the string then perhaps a dictionary with keytypechar
would have been better and saved a bunch of converting chars to ints...
– Chris
Nov 15 '18 at 12:28
add a comment |
I solve it without much complexity. Thanks, @Jeroen Mostert for your advice
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine" };
int nums = new { 2, 32, 70 };
(from d in nums
select new
{
num = d,
parts = d.ToString().Select(o=> Convert.ToInt32(o.ToString())),
parts2 = d.ToString().Select(o => numStrings[Convert.ToInt32(o.ToString())]),
parts3 = string.Join("-",d.ToString().Select(o => numStrings[Convert.ToInt32(o.ToString())]).ToArray())
}).Dump();
Output in LinqPad:
You do realizedict
is semantically equal tonumStrings
, right?dict[x] == numStrings[x]
for allx
between0
and9
, inclusive.
– Jeroen Mostert
Nov 15 '18 at 12:23
But given what you really want to be looking up is thechar
s from the string then perhaps a dictionary with keytypechar
would have been better and saved a bunch of converting chars to ints...
– Chris
Nov 15 '18 at 12:28
add a comment |
I solve it without much complexity. Thanks, @Jeroen Mostert for your advice
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine" };
int nums = new { 2, 32, 70 };
(from d in nums
select new
{
num = d,
parts = d.ToString().Select(o=> Convert.ToInt32(o.ToString())),
parts2 = d.ToString().Select(o => numStrings[Convert.ToInt32(o.ToString())]),
parts3 = string.Join("-",d.ToString().Select(o => numStrings[Convert.ToInt32(o.ToString())]).ToArray())
}).Dump();
Output in LinqPad:
I solve it without much complexity. Thanks, @Jeroen Mostert for your advice
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine" };
int nums = new { 2, 32, 70 };
(from d in nums
select new
{
num = d,
parts = d.ToString().Select(o=> Convert.ToInt32(o.ToString())),
parts2 = d.ToString().Select(o => numStrings[Convert.ToInt32(o.ToString())]),
parts3 = string.Join("-",d.ToString().Select(o => numStrings[Convert.ToInt32(o.ToString())]).ToArray())
}).Dump();
Output in LinqPad:
edited Nov 15 '18 at 13:15
answered Nov 15 '18 at 12:18
HemendrHemendr
18218
18218
You do realizedict
is semantically equal tonumStrings
, right?dict[x] == numStrings[x]
for allx
between0
and9
, inclusive.
– Jeroen Mostert
Nov 15 '18 at 12:23
But given what you really want to be looking up is thechar
s from the string then perhaps a dictionary with keytypechar
would have been better and saved a bunch of converting chars to ints...
– Chris
Nov 15 '18 at 12:28
add a comment |
You do realizedict
is semantically equal tonumStrings
, right?dict[x] == numStrings[x]
for allx
between0
and9
, inclusive.
– Jeroen Mostert
Nov 15 '18 at 12:23
But given what you really want to be looking up is thechar
s from the string then perhaps a dictionary with keytypechar
would have been better and saved a bunch of converting chars to ints...
– Chris
Nov 15 '18 at 12:28
You do realize
dict
is semantically equal to numStrings
, right? dict[x] == numStrings[x]
for all x
between 0
and 9
, inclusive.– Jeroen Mostert
Nov 15 '18 at 12:23
You do realize
dict
is semantically equal to numStrings
, right? dict[x] == numStrings[x]
for all x
between 0
and 9
, inclusive.– Jeroen Mostert
Nov 15 '18 at 12:23
But given what you really want to be looking up is the
char
s from the string then perhaps a dictionary with keytype char
would have been better and saved a bunch of converting chars to ints...– Chris
Nov 15 '18 at 12:28
But given what you really want to be looking up is the
char
s from the string then perhaps a dictionary with keytype char
would have been better and saved a bunch of converting chars to ints...– Chris
Nov 15 '18 at 12:28
add a comment |
This is one of the many ways to do it:
void Main()
{
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine" };
int nums = new { 2, 32, 70 };
Func<int, string> numToWord = (x) => {
List<string> words = new List<string>();
do {
words.Add( numStrings[x%10] );
x /= 10;
} while (x > 0);
var w = words.Reverse<string>();
return string.Join("-",w);
};
var result = nums.Select(n => numToWord(n));
foreach (var element in result)
{
Console.WriteLine(element);
}
}
add a comment |
This is one of the many ways to do it:
void Main()
{
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine" };
int nums = new { 2, 32, 70 };
Func<int, string> numToWord = (x) => {
List<string> words = new List<string>();
do {
words.Add( numStrings[x%10] );
x /= 10;
} while (x > 0);
var w = words.Reverse<string>();
return string.Join("-",w);
};
var result = nums.Select(n => numToWord(n));
foreach (var element in result)
{
Console.WriteLine(element);
}
}
add a comment |
This is one of the many ways to do it:
void Main()
{
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine" };
int nums = new { 2, 32, 70 };
Func<int, string> numToWord = (x) => {
List<string> words = new List<string>();
do {
words.Add( numStrings[x%10] );
x /= 10;
} while (x > 0);
var w = words.Reverse<string>();
return string.Join("-",w);
};
var result = nums.Select(n => numToWord(n));
foreach (var element in result)
{
Console.WriteLine(element);
}
}
This is one of the many ways to do it:
void Main()
{
string numStrings = new { "Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine" };
int nums = new { 2, 32, 70 };
Func<int, string> numToWord = (x) => {
List<string> words = new List<string>();
do {
words.Add( numStrings[x%10] );
x /= 10;
} while (x > 0);
var w = words.Reverse<string>();
return string.Join("-",w);
};
var result = nums.Select(n => numToWord(n));
foreach (var element in result)
{
Console.WriteLine(element);
}
}
answered Nov 15 '18 at 13:59
Cetin BasozCetin Basoz
11k11528
11k11528
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53317918%2fjoin-two-array-based-on-index-and-index-value-using-linq-query-expression-syntax%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
EOXX 3PUU,38ijH1uLVOzn4f SdJSJ,ssxAGb38BvP7IDYYNq,yEUR,zv u XXuJGAZKtcna1danSARxl1vP
So.... what did you try? Looks like an assignment. Just try yourself, else you won't learn anything. We can make up the most beautiful linq queries/string joins that solves this. But are you able to read/understand them?
– J. van Langen
Nov 15 '18 at 10:59
List<string> strValuList = (from d in nums from p in numStrings.Select((value, index) => new { value = value, index = index }) where p.index == d select p.value).ToList();
– Hemendr
Nov 15 '18 at 11:02
You might update your question instead of commenting ;-)
– J. van Langen
Nov 15 '18 at 11:03
1
Doing this in one query is more complicated than necessary. Hint:
do { yield return numStrings[n % 10]; n /= 10; } while (n != 0)
. (.ToString
and going over each character is another approach, though it might be considered "cheating":nums.Select(n => ("" + n).Select(c => numStrings[c - '0']))
.)– Jeroen Mostert
Nov 15 '18 at 11:10
1
The loop you'd do to extract the digits from a number can certainly be written in a LINQ query, and you can join that up with the digit array. It's just not particularly sane to do it this way.
– Jeroen Mostert
Nov 15 '18 at 11:30