Create Bootstrap collapsible accordion table rows that display dynamic content from flask sqlalchemy...
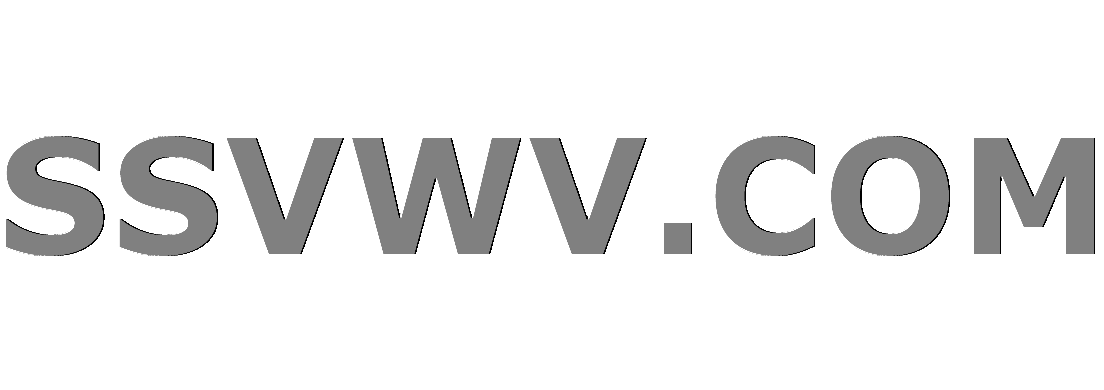
Multi tool use
Consider the following table which iterates data from an sqlite
database using flask
and sqlalchemy
.
Assume for this example that the data is a list of invoices and clicking on each row opens a collapsible bootstrap accordion
whith further information for the clicked invoice.
<table class="table table-hover" data-toggle="table">
<thead>
<tr>
<th>Date</th>
<th>Invoice</th>
</tr>
</thead>
<tbody>
<tr data-toggle="collapse" data-target="#accordion" class="clickable">
{% for inv in invoices %}
<td>
{{ inv.number }}
</td>
</tr>
<tr>
<td>
<div id="accordion" class="collapse">
{{ inv.data }}
</div>
</td>
</tr>
{% endfor %}
</body>
</table>
The problem here is that only the first row is clickable and clicking on it opens all the rows instead of just a single row whereas we would like to be able to click on each row and reveal the data for that specific row exclusively.
I think the problem is do to with the data-target="#accordion" tag which targets the iteration of the collapsed data placeholder instead of the specific placeholder itself.
You can see an example here Twitter Bootstrap Use collapse.js on table cells [Almost Done] and here http://jsfiddle.net/whytheday/2Dj7Y/11/ but again the content is static and not dynamic.
The solution would be to have a "dynamic" data-target tag which matches the target id but I have no idea how to do that.
python-3.x flask sqlite3 bootstrap-4 flask-sqlalchemy
add a comment |
Consider the following table which iterates data from an sqlite
database using flask
and sqlalchemy
.
Assume for this example that the data is a list of invoices and clicking on each row opens a collapsible bootstrap accordion
whith further information for the clicked invoice.
<table class="table table-hover" data-toggle="table">
<thead>
<tr>
<th>Date</th>
<th>Invoice</th>
</tr>
</thead>
<tbody>
<tr data-toggle="collapse" data-target="#accordion" class="clickable">
{% for inv in invoices %}
<td>
{{ inv.number }}
</td>
</tr>
<tr>
<td>
<div id="accordion" class="collapse">
{{ inv.data }}
</div>
</td>
</tr>
{% endfor %}
</body>
</table>
The problem here is that only the first row is clickable and clicking on it opens all the rows instead of just a single row whereas we would like to be able to click on each row and reveal the data for that specific row exclusively.
I think the problem is do to with the data-target="#accordion" tag which targets the iteration of the collapsed data placeholder instead of the specific placeholder itself.
You can see an example here Twitter Bootstrap Use collapse.js on table cells [Almost Done] and here http://jsfiddle.net/whytheday/2Dj7Y/11/ but again the content is static and not dynamic.
The solution would be to have a "dynamic" data-target tag which matches the target id but I have no idea how to do that.
python-3.x flask sqlite3 bootstrap-4 flask-sqlalchemy
add a comment |
Consider the following table which iterates data from an sqlite
database using flask
and sqlalchemy
.
Assume for this example that the data is a list of invoices and clicking on each row opens a collapsible bootstrap accordion
whith further information for the clicked invoice.
<table class="table table-hover" data-toggle="table">
<thead>
<tr>
<th>Date</th>
<th>Invoice</th>
</tr>
</thead>
<tbody>
<tr data-toggle="collapse" data-target="#accordion" class="clickable">
{% for inv in invoices %}
<td>
{{ inv.number }}
</td>
</tr>
<tr>
<td>
<div id="accordion" class="collapse">
{{ inv.data }}
</div>
</td>
</tr>
{% endfor %}
</body>
</table>
The problem here is that only the first row is clickable and clicking on it opens all the rows instead of just a single row whereas we would like to be able to click on each row and reveal the data for that specific row exclusively.
I think the problem is do to with the data-target="#accordion" tag which targets the iteration of the collapsed data placeholder instead of the specific placeholder itself.
You can see an example here Twitter Bootstrap Use collapse.js on table cells [Almost Done] and here http://jsfiddle.net/whytheday/2Dj7Y/11/ but again the content is static and not dynamic.
The solution would be to have a "dynamic" data-target tag which matches the target id but I have no idea how to do that.
python-3.x flask sqlite3 bootstrap-4 flask-sqlalchemy
Consider the following table which iterates data from an sqlite
database using flask
and sqlalchemy
.
Assume for this example that the data is a list of invoices and clicking on each row opens a collapsible bootstrap accordion
whith further information for the clicked invoice.
<table class="table table-hover" data-toggle="table">
<thead>
<tr>
<th>Date</th>
<th>Invoice</th>
</tr>
</thead>
<tbody>
<tr data-toggle="collapse" data-target="#accordion" class="clickable">
{% for inv in invoices %}
<td>
{{ inv.number }}
</td>
</tr>
<tr>
<td>
<div id="accordion" class="collapse">
{{ inv.data }}
</div>
</td>
</tr>
{% endfor %}
</body>
</table>
The problem here is that only the first row is clickable and clicking on it opens all the rows instead of just a single row whereas we would like to be able to click on each row and reveal the data for that specific row exclusively.
I think the problem is do to with the data-target="#accordion" tag which targets the iteration of the collapsed data placeholder instead of the specific placeholder itself.
You can see an example here Twitter Bootstrap Use collapse.js on table cells [Almost Done] and here http://jsfiddle.net/whytheday/2Dj7Y/11/ but again the content is static and not dynamic.
The solution would be to have a "dynamic" data-target tag which matches the target id but I have no idea how to do that.
python-3.x flask sqlite3 bootstrap-4 flask-sqlalchemy
python-3.x flask sqlite3 bootstrap-4 flask-sqlalchemy
edited Nov 15 '18 at 12:47
Alex B
asked Nov 15 '18 at 10:57
Alex BAlex B
16513
16513
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
To accomplish this task, you have to insert your tr tag into the jinja loop, then add a dynamic data-target to your tr tag and a dynamic id to all your collapsible bootstrap accordions
; So each tr tag will point to the corresponding accordion. Here's what the code should look like:
<tbody>
{% for inv in invoices %}
<tr data-toggle="collapse" data-target="#{{inv.number}}" class="clickable">
<td>
{{ inv.number }}
</td>
</tr>
<tr id="{{inv.number}}" class="no-border collapse">
<td>
<div>
{{ inv.data }}
</div>
</td>
</tr>
{% endfor %}
</tbody>
The idea here is that, since the invoice number is unique, you will have accordions with unique ids. Thus each attribute data-target of your tr tags (generated dynamically them too), will point to the corresponding accordion.
Extras just in case:
you will notice that I added the class no-border
to the second tr block. This is for the case where you would not want to have a border from Bootstrap tables... here is the corresponding css:
<style type="text/css">
.table>tbody>tr.no-border>td{
border-top: none;
}
</style>
Unfortunately that makes no difference. I tried moving the tag around myself too to see if it would help but it doesn't. I think the problem is do to with thedata-target="#accordion"
tag which targets the iteration of the collapsed data placeholder instead of the specific placeholder itself. The solution would be to have a "dynamic"data-target
tag which matches the targetid
but I have no idea how to do that.
– Alex B
Nov 15 '18 at 12:38
Adding unique ids is a step in the right direction since only one row collapses now but it immediately closes itself on its own without clicking on it again so doesn't stay open to show the data.
– Alex B
Nov 15 '18 at 16:38
the open close issue was an unrelated issue with some conflicting .js
– Alex B
Nov 15 '18 at 17:01
1
I also move thechild
id and class to<tr>
instead of<div>
so that it applies to the entire row and not just the cell which makes more sense.
– Alex B
Nov 15 '18 at 17:38
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53317916%2fcreate-bootstrap-collapsible-accordion-table-rows-that-display-dynamic-content-f%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
To accomplish this task, you have to insert your tr tag into the jinja loop, then add a dynamic data-target to your tr tag and a dynamic id to all your collapsible bootstrap accordions
; So each tr tag will point to the corresponding accordion. Here's what the code should look like:
<tbody>
{% for inv in invoices %}
<tr data-toggle="collapse" data-target="#{{inv.number}}" class="clickable">
<td>
{{ inv.number }}
</td>
</tr>
<tr id="{{inv.number}}" class="no-border collapse">
<td>
<div>
{{ inv.data }}
</div>
</td>
</tr>
{% endfor %}
</tbody>
The idea here is that, since the invoice number is unique, you will have accordions with unique ids. Thus each attribute data-target of your tr tags (generated dynamically them too), will point to the corresponding accordion.
Extras just in case:
you will notice that I added the class no-border
to the second tr block. This is for the case where you would not want to have a border from Bootstrap tables... here is the corresponding css:
<style type="text/css">
.table>tbody>tr.no-border>td{
border-top: none;
}
</style>
Unfortunately that makes no difference. I tried moving the tag around myself too to see if it would help but it doesn't. I think the problem is do to with thedata-target="#accordion"
tag which targets the iteration of the collapsed data placeholder instead of the specific placeholder itself. The solution would be to have a "dynamic"data-target
tag which matches the targetid
but I have no idea how to do that.
– Alex B
Nov 15 '18 at 12:38
Adding unique ids is a step in the right direction since only one row collapses now but it immediately closes itself on its own without clicking on it again so doesn't stay open to show the data.
– Alex B
Nov 15 '18 at 16:38
the open close issue was an unrelated issue with some conflicting .js
– Alex B
Nov 15 '18 at 17:01
1
I also move thechild
id and class to<tr>
instead of<div>
so that it applies to the entire row and not just the cell which makes more sense.
– Alex B
Nov 15 '18 at 17:38
add a comment |
To accomplish this task, you have to insert your tr tag into the jinja loop, then add a dynamic data-target to your tr tag and a dynamic id to all your collapsible bootstrap accordions
; So each tr tag will point to the corresponding accordion. Here's what the code should look like:
<tbody>
{% for inv in invoices %}
<tr data-toggle="collapse" data-target="#{{inv.number}}" class="clickable">
<td>
{{ inv.number }}
</td>
</tr>
<tr id="{{inv.number}}" class="no-border collapse">
<td>
<div>
{{ inv.data }}
</div>
</td>
</tr>
{% endfor %}
</tbody>
The idea here is that, since the invoice number is unique, you will have accordions with unique ids. Thus each attribute data-target of your tr tags (generated dynamically them too), will point to the corresponding accordion.
Extras just in case:
you will notice that I added the class no-border
to the second tr block. This is for the case where you would not want to have a border from Bootstrap tables... here is the corresponding css:
<style type="text/css">
.table>tbody>tr.no-border>td{
border-top: none;
}
</style>
Unfortunately that makes no difference. I tried moving the tag around myself too to see if it would help but it doesn't. I think the problem is do to with thedata-target="#accordion"
tag which targets the iteration of the collapsed data placeholder instead of the specific placeholder itself. The solution would be to have a "dynamic"data-target
tag which matches the targetid
but I have no idea how to do that.
– Alex B
Nov 15 '18 at 12:38
Adding unique ids is a step in the right direction since only one row collapses now but it immediately closes itself on its own without clicking on it again so doesn't stay open to show the data.
– Alex B
Nov 15 '18 at 16:38
the open close issue was an unrelated issue with some conflicting .js
– Alex B
Nov 15 '18 at 17:01
1
I also move thechild
id and class to<tr>
instead of<div>
so that it applies to the entire row and not just the cell which makes more sense.
– Alex B
Nov 15 '18 at 17:38
add a comment |
To accomplish this task, you have to insert your tr tag into the jinja loop, then add a dynamic data-target to your tr tag and a dynamic id to all your collapsible bootstrap accordions
; So each tr tag will point to the corresponding accordion. Here's what the code should look like:
<tbody>
{% for inv in invoices %}
<tr data-toggle="collapse" data-target="#{{inv.number}}" class="clickable">
<td>
{{ inv.number }}
</td>
</tr>
<tr id="{{inv.number}}" class="no-border collapse">
<td>
<div>
{{ inv.data }}
</div>
</td>
</tr>
{% endfor %}
</tbody>
The idea here is that, since the invoice number is unique, you will have accordions with unique ids. Thus each attribute data-target of your tr tags (generated dynamically them too), will point to the corresponding accordion.
Extras just in case:
you will notice that I added the class no-border
to the second tr block. This is for the case where you would not want to have a border from Bootstrap tables... here is the corresponding css:
<style type="text/css">
.table>tbody>tr.no-border>td{
border-top: none;
}
</style>
To accomplish this task, you have to insert your tr tag into the jinja loop, then add a dynamic data-target to your tr tag and a dynamic id to all your collapsible bootstrap accordions
; So each tr tag will point to the corresponding accordion. Here's what the code should look like:
<tbody>
{% for inv in invoices %}
<tr data-toggle="collapse" data-target="#{{inv.number}}" class="clickable">
<td>
{{ inv.number }}
</td>
</tr>
<tr id="{{inv.number}}" class="no-border collapse">
<td>
<div>
{{ inv.data }}
</div>
</td>
</tr>
{% endfor %}
</tbody>
The idea here is that, since the invoice number is unique, you will have accordions with unique ids. Thus each attribute data-target of your tr tags (generated dynamically them too), will point to the corresponding accordion.
Extras just in case:
you will notice that I added the class no-border
to the second tr block. This is for the case where you would not want to have a border from Bootstrap tables... here is the corresponding css:
<style type="text/css">
.table>tbody>tr.no-border>td{
border-top: none;
}
</style>
edited Nov 15 '18 at 19:28
Alex B
16513
16513
answered Nov 15 '18 at 12:11
TobinTobin
40118
40118
Unfortunately that makes no difference. I tried moving the tag around myself too to see if it would help but it doesn't. I think the problem is do to with thedata-target="#accordion"
tag which targets the iteration of the collapsed data placeholder instead of the specific placeholder itself. The solution would be to have a "dynamic"data-target
tag which matches the targetid
but I have no idea how to do that.
– Alex B
Nov 15 '18 at 12:38
Adding unique ids is a step in the right direction since only one row collapses now but it immediately closes itself on its own without clicking on it again so doesn't stay open to show the data.
– Alex B
Nov 15 '18 at 16:38
the open close issue was an unrelated issue with some conflicting .js
– Alex B
Nov 15 '18 at 17:01
1
I also move thechild
id and class to<tr>
instead of<div>
so that it applies to the entire row and not just the cell which makes more sense.
– Alex B
Nov 15 '18 at 17:38
add a comment |
Unfortunately that makes no difference. I tried moving the tag around myself too to see if it would help but it doesn't. I think the problem is do to with thedata-target="#accordion"
tag which targets the iteration of the collapsed data placeholder instead of the specific placeholder itself. The solution would be to have a "dynamic"data-target
tag which matches the targetid
but I have no idea how to do that.
– Alex B
Nov 15 '18 at 12:38
Adding unique ids is a step in the right direction since only one row collapses now but it immediately closes itself on its own without clicking on it again so doesn't stay open to show the data.
– Alex B
Nov 15 '18 at 16:38
the open close issue was an unrelated issue with some conflicting .js
– Alex B
Nov 15 '18 at 17:01
1
I also move thechild
id and class to<tr>
instead of<div>
so that it applies to the entire row and not just the cell which makes more sense.
– Alex B
Nov 15 '18 at 17:38
Unfortunately that makes no difference. I tried moving the tag around myself too to see if it would help but it doesn't. I think the problem is do to with the
data-target="#accordion"
tag which targets the iteration of the collapsed data placeholder instead of the specific placeholder itself. The solution would be to have a "dynamic" data-target
tag which matches the target id
but I have no idea how to do that.– Alex B
Nov 15 '18 at 12:38
Unfortunately that makes no difference. I tried moving the tag around myself too to see if it would help but it doesn't. I think the problem is do to with the
data-target="#accordion"
tag which targets the iteration of the collapsed data placeholder instead of the specific placeholder itself. The solution would be to have a "dynamic" data-target
tag which matches the target id
but I have no idea how to do that.– Alex B
Nov 15 '18 at 12:38
Adding unique ids is a step in the right direction since only one row collapses now but it immediately closes itself on its own without clicking on it again so doesn't stay open to show the data.
– Alex B
Nov 15 '18 at 16:38
Adding unique ids is a step in the right direction since only one row collapses now but it immediately closes itself on its own without clicking on it again so doesn't stay open to show the data.
– Alex B
Nov 15 '18 at 16:38
the open close issue was an unrelated issue with some conflicting .js
– Alex B
Nov 15 '18 at 17:01
the open close issue was an unrelated issue with some conflicting .js
– Alex B
Nov 15 '18 at 17:01
1
1
I also move the
child
id and class to <tr>
instead of <div>
so that it applies to the entire row and not just the cell which makes more sense.– Alex B
Nov 15 '18 at 17:38
I also move the
child
id and class to <tr>
instead of <div>
so that it applies to the entire row and not just the cell which makes more sense.– Alex B
Nov 15 '18 at 17:38
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53317916%2fcreate-bootstrap-collapsible-accordion-table-rows-that-display-dynamic-content-f%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LQKRZ ry fyHwzYyDDFfyzj OYstM2HaoT,1uMt1Fqgo6bKzOl9Fm,c8CQybCuQw xXGpZ7A8L,In0PagT5FtdNC 5AaU7W3FiSs70