Callback executed too early
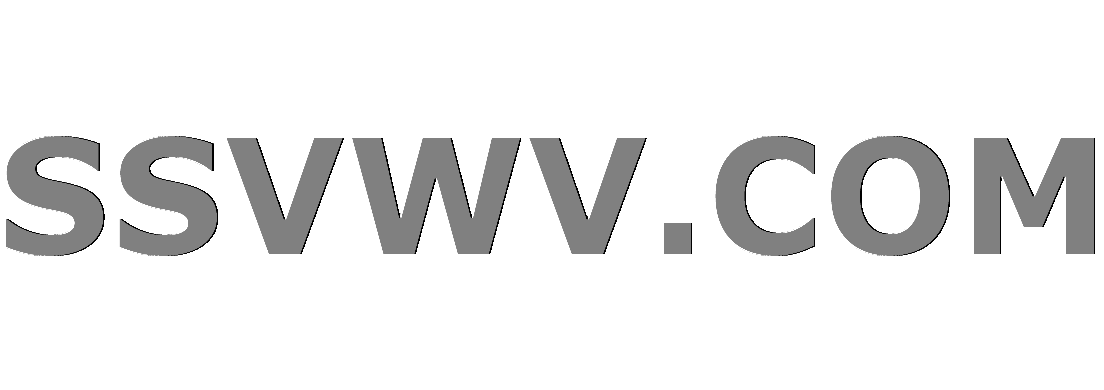
Multi tool use
I'm struggling with an issue I can't get around.
I'm using NodeJS to request data from Rest API´s. For this program I first retrieve an array of data, then I request some details from another endpoint based on an id from the first array.
I use a foreach loop inside the first function call with callback, and then another function call within the foreach loop to get the details.
What I experience is that the second function does callback before the response is retrieved.
I have managed to reproduce the issue with the following example code:
console.log('Program start')
// Executing program
getFirstname(function(person) {
person.forEach(firstname => {
getSurname(firstname.id, function(lastname) {
console.log(`${firstname.value} ${lastname}`)
});
});
})
// Emulating REST API´s
function getFirstname(callback) {
console.log('Returning list of firstnames')
let data = [
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
]
callback(data);
}
function getSurname(id, callback) {
console.log(`Querying for lastname id ${id}`)
let data = [
'Andersen',
'Johsen',
'Falon',
'Alexander',
]
setTimeout(() => {
callback(data[id]);
}, 2000);
}
Here is the result of the program:
Program start
Returning list of firstnames
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
As you can see, there are several records with undefined
value. Also I have added a setTimeout
to emulate that each rest call takes a few seconds. What happens is that all the queries are fired off instantly, then it goes 2 seconds before all the responses return simultaneous.
I would expect this to wait for each second rest call and then return the result.
How can I solve this issue?
Best regards,
Christian
javascript node.js loops foreach callback
add a comment |
I'm struggling with an issue I can't get around.
I'm using NodeJS to request data from Rest API´s. For this program I first retrieve an array of data, then I request some details from another endpoint based on an id from the first array.
I use a foreach loop inside the first function call with callback, and then another function call within the foreach loop to get the details.
What I experience is that the second function does callback before the response is retrieved.
I have managed to reproduce the issue with the following example code:
console.log('Program start')
// Executing program
getFirstname(function(person) {
person.forEach(firstname => {
getSurname(firstname.id, function(lastname) {
console.log(`${firstname.value} ${lastname}`)
});
});
})
// Emulating REST API´s
function getFirstname(callback) {
console.log('Returning list of firstnames')
let data = [
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
]
callback(data);
}
function getSurname(id, callback) {
console.log(`Querying for lastname id ${id}`)
let data = [
'Andersen',
'Johsen',
'Falon',
'Alexander',
]
setTimeout(() => {
callback(data[id]);
}, 2000);
}
Here is the result of the program:
Program start
Returning list of firstnames
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
As you can see, there are several records with undefined
value. Also I have added a setTimeout
to emulate that each rest call takes a few seconds. What happens is that all the queries are fired off instantly, then it goes 2 seconds before all the responses return simultaneous.
I would expect this to wait for each second rest call and then return the result.
How can I solve this issue?
Best regards,
Christian
javascript node.js loops foreach callback
add a comment |
I'm struggling with an issue I can't get around.
I'm using NodeJS to request data from Rest API´s. For this program I first retrieve an array of data, then I request some details from another endpoint based on an id from the first array.
I use a foreach loop inside the first function call with callback, and then another function call within the foreach loop to get the details.
What I experience is that the second function does callback before the response is retrieved.
I have managed to reproduce the issue with the following example code:
console.log('Program start')
// Executing program
getFirstname(function(person) {
person.forEach(firstname => {
getSurname(firstname.id, function(lastname) {
console.log(`${firstname.value} ${lastname}`)
});
});
})
// Emulating REST API´s
function getFirstname(callback) {
console.log('Returning list of firstnames')
let data = [
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
]
callback(data);
}
function getSurname(id, callback) {
console.log(`Querying for lastname id ${id}`)
let data = [
'Andersen',
'Johsen',
'Falon',
'Alexander',
]
setTimeout(() => {
callback(data[id]);
}, 2000);
}
Here is the result of the program:
Program start
Returning list of firstnames
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
As you can see, there are several records with undefined
value. Also I have added a setTimeout
to emulate that each rest call takes a few seconds. What happens is that all the queries are fired off instantly, then it goes 2 seconds before all the responses return simultaneous.
I would expect this to wait for each second rest call and then return the result.
How can I solve this issue?
Best regards,
Christian
javascript node.js loops foreach callback
I'm struggling with an issue I can't get around.
I'm using NodeJS to request data from Rest API´s. For this program I first retrieve an array of data, then I request some details from another endpoint based on an id from the first array.
I use a foreach loop inside the first function call with callback, and then another function call within the foreach loop to get the details.
What I experience is that the second function does callback before the response is retrieved.
I have managed to reproduce the issue with the following example code:
console.log('Program start')
// Executing program
getFirstname(function(person) {
person.forEach(firstname => {
getSurname(firstname.id, function(lastname) {
console.log(`${firstname.value} ${lastname}`)
});
});
})
// Emulating REST API´s
function getFirstname(callback) {
console.log('Returning list of firstnames')
let data = [
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
{id: 0, value: 'John'},
{id: 2, value: 'Andy'},
{id: 3, value: 'Jimmy'},
{id: 4, value: 'Alex'},
]
callback(data);
}
function getSurname(id, callback) {
console.log(`Querying for lastname id ${id}`)
let data = [
'Andersen',
'Johsen',
'Falon',
'Alexander',
]
setTimeout(() => {
callback(data[id]);
}, 2000);
}
Here is the result of the program:
Program start
Returning list of firstnames
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
Querying for lastname id 0
Querying for lastname id 2
Querying for lastname id 3
Querying for lastname id 4
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
John Andersen
Andy Falon
Jimmy Alexander
Alex undefined
As you can see, there are several records with undefined
value. Also I have added a setTimeout
to emulate that each rest call takes a few seconds. What happens is that all the queries are fired off instantly, then it goes 2 seconds before all the responses return simultaneous.
I would expect this to wait for each second rest call and then return the result.
How can I solve this issue?
Best regards,
Christian
javascript node.js loops foreach callback
javascript node.js loops foreach callback
edited Nov 13 at 15:06


mihai
23.3k73968
23.3k73968
asked Nov 12 at 20:21
chranmat
294
294
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
The reason you have undefined
is because your data
array has length of 4
(indices go from 0 to 3) but your id
's range from 0 to 4. Which means that when you reference data[4]
you get undefined
.
Your right. That was actually a typo in the example code, but I have this problem with my real code.
– chranmat
Nov 12 at 20:43
add a comment |
There is nothing wrong with your callbacks. The data, however is incorrect. See below:
function getSurname(id, callback) {
console.log(`Querying for lastname id ${id}`)
let data = [
'Andersen',
'Johsen',
'Falon',
'Alexander',
]
setTimeout(() => {
callback(data[id]);
}, 2000);
}
Everytime Alex
is called, you're passing id 4
for use in your data
array. Remember that array's are 0
based so data[4] = undefined
...
Ref comment on previous answer. I can see I did a typo there and that my example actually works. This does not solve the problem in my real code :(
– chranmat
Nov 12 at 20:47
@chranmat No worries. Can you post your actual code please?
– War10ck
Nov 12 at 20:48
pastebin.com/XpcWUtrv
– chranmat
Nov 12 at 21:06
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53269520%2fcallback-executed-too-early%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The reason you have undefined
is because your data
array has length of 4
(indices go from 0 to 3) but your id
's range from 0 to 4. Which means that when you reference data[4]
you get undefined
.
Your right. That was actually a typo in the example code, but I have this problem with my real code.
– chranmat
Nov 12 at 20:43
add a comment |
The reason you have undefined
is because your data
array has length of 4
(indices go from 0 to 3) but your id
's range from 0 to 4. Which means that when you reference data[4]
you get undefined
.
Your right. That was actually a typo in the example code, but I have this problem with my real code.
– chranmat
Nov 12 at 20:43
add a comment |
The reason you have undefined
is because your data
array has length of 4
(indices go from 0 to 3) but your id
's range from 0 to 4. Which means that when you reference data[4]
you get undefined
.
The reason you have undefined
is because your data
array has length of 4
(indices go from 0 to 3) but your id
's range from 0 to 4. Which means that when you reference data[4]
you get undefined
.
answered Nov 12 at 20:28


ODYN-Kon
2,0841625
2,0841625
Your right. That was actually a typo in the example code, but I have this problem with my real code.
– chranmat
Nov 12 at 20:43
add a comment |
Your right. That was actually a typo in the example code, but I have this problem with my real code.
– chranmat
Nov 12 at 20:43
Your right. That was actually a typo in the example code, but I have this problem with my real code.
– chranmat
Nov 12 at 20:43
Your right. That was actually a typo in the example code, but I have this problem with my real code.
– chranmat
Nov 12 at 20:43
add a comment |
There is nothing wrong with your callbacks. The data, however is incorrect. See below:
function getSurname(id, callback) {
console.log(`Querying for lastname id ${id}`)
let data = [
'Andersen',
'Johsen',
'Falon',
'Alexander',
]
setTimeout(() => {
callback(data[id]);
}, 2000);
}
Everytime Alex
is called, you're passing id 4
for use in your data
array. Remember that array's are 0
based so data[4] = undefined
...
Ref comment on previous answer. I can see I did a typo there and that my example actually works. This does not solve the problem in my real code :(
– chranmat
Nov 12 at 20:47
@chranmat No worries. Can you post your actual code please?
– War10ck
Nov 12 at 20:48
pastebin.com/XpcWUtrv
– chranmat
Nov 12 at 21:06
add a comment |
There is nothing wrong with your callbacks. The data, however is incorrect. See below:
function getSurname(id, callback) {
console.log(`Querying for lastname id ${id}`)
let data = [
'Andersen',
'Johsen',
'Falon',
'Alexander',
]
setTimeout(() => {
callback(data[id]);
}, 2000);
}
Everytime Alex
is called, you're passing id 4
for use in your data
array. Remember that array's are 0
based so data[4] = undefined
...
Ref comment on previous answer. I can see I did a typo there and that my example actually works. This does not solve the problem in my real code :(
– chranmat
Nov 12 at 20:47
@chranmat No worries. Can you post your actual code please?
– War10ck
Nov 12 at 20:48
pastebin.com/XpcWUtrv
– chranmat
Nov 12 at 21:06
add a comment |
There is nothing wrong with your callbacks. The data, however is incorrect. See below:
function getSurname(id, callback) {
console.log(`Querying for lastname id ${id}`)
let data = [
'Andersen',
'Johsen',
'Falon',
'Alexander',
]
setTimeout(() => {
callback(data[id]);
}, 2000);
}
Everytime Alex
is called, you're passing id 4
for use in your data
array. Remember that array's are 0
based so data[4] = undefined
...
There is nothing wrong with your callbacks. The data, however is incorrect. See below:
function getSurname(id, callback) {
console.log(`Querying for lastname id ${id}`)
let data = [
'Andersen',
'Johsen',
'Falon',
'Alexander',
]
setTimeout(() => {
callback(data[id]);
}, 2000);
}
Everytime Alex
is called, you're passing id 4
for use in your data
array. Remember that array's are 0
based so data[4] = undefined
...
answered Nov 12 at 20:28
War10ck
10.1k62945
10.1k62945
Ref comment on previous answer. I can see I did a typo there and that my example actually works. This does not solve the problem in my real code :(
– chranmat
Nov 12 at 20:47
@chranmat No worries. Can you post your actual code please?
– War10ck
Nov 12 at 20:48
pastebin.com/XpcWUtrv
– chranmat
Nov 12 at 21:06
add a comment |
Ref comment on previous answer. I can see I did a typo there and that my example actually works. This does not solve the problem in my real code :(
– chranmat
Nov 12 at 20:47
@chranmat No worries. Can you post your actual code please?
– War10ck
Nov 12 at 20:48
pastebin.com/XpcWUtrv
– chranmat
Nov 12 at 21:06
Ref comment on previous answer. I can see I did a typo there and that my example actually works. This does not solve the problem in my real code :(
– chranmat
Nov 12 at 20:47
Ref comment on previous answer. I can see I did a typo there and that my example actually works. This does not solve the problem in my real code :(
– chranmat
Nov 12 at 20:47
@chranmat No worries. Can you post your actual code please?
– War10ck
Nov 12 at 20:48
@chranmat No worries. Can you post your actual code please?
– War10ck
Nov 12 at 20:48
pastebin.com/XpcWUtrv
– chranmat
Nov 12 at 21:06
pastebin.com/XpcWUtrv
– chranmat
Nov 12 at 21:06
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53269520%2fcallback-executed-too-early%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
A4jTVlzO,2f,zggR wTjQ88YI8HajPy,VBBX005