Understanding tessellation shaders
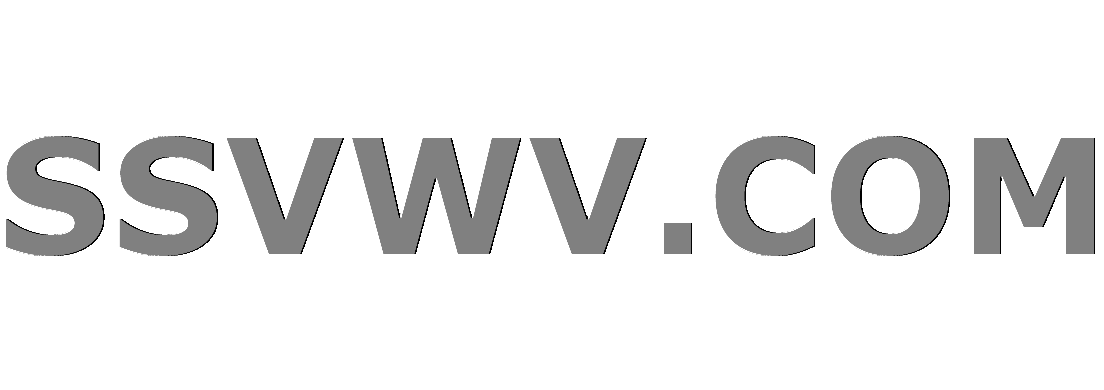
Multi tool use
I am trying to use the tessellation shaders in OpenGL ES 3.2, and have tried to follow 2 different tutorials without success.
I am currently drawing a flat rectangle, the goal is to elevate its surface. I receive the vertices in the vertex shader:
#version 320 es
layout (location = 0) in vec3 position;
out vec3 v_position;
uniform mat4 model_matrix;
uniform mat4 mvp_matrix;
void main() {
vec4 pos = vec4(position, 1.0);
v_position = vec3(model_matrix * pos);
gl_Position = mvp_matrix * vec4(position, 1.0);
}
Attempt to tessellate in the tessellation control shader:
#version 320 es
layout (vertices = 3) out;
in vec3 v_position;
out vec3 t_position;
void main()
{
// Set the control points of the output patch
t_position[gl_InvocationID] = v_position[gl_InvocationID];
// Calculate the tessellation levels
gl_TessLevelOuter[0] = 3.0;
gl_TessLevelOuter[1] = 3.0;
gl_TessLevelOuter[2] = 3.0;
gl_TessLevelInner[0] = gl_TessLevelOuter[2];
}
And then try to make a minimum working evaluation shader:
#version 320 es
layout(triangles, equal_spacing, ccw) in;
in vec3 t_position;
out vec3 f_position;
uniform mat4 mvp_matrix;
void main() {
f_position = t_position[0];
gl_Position = mvp_matrix * vec4(f_position, 1.0);
}
I call these shaders using glDrawArrays(GL_PATCHES, 0, vertex_num)
. However currently I see no output when attempting to tessellate. if instead I remove the tessellation shaders and draw with only the vertex and fragment shaders (after tweaking so that they compile). I do see the rectangle mesh, so I am confident the issue is in my shaders.
I am not getting compilation errors, and calling glGetError()
reports no error, so it's my logic that I think is wrong.
Edit:
Immediately prior to the draw call I also do:
glPatchParameteri(GL_PATCH_VERTICES, 3);
opengl-es glsl shader tessellation
|
show 2 more comments
I am trying to use the tessellation shaders in OpenGL ES 3.2, and have tried to follow 2 different tutorials without success.
I am currently drawing a flat rectangle, the goal is to elevate its surface. I receive the vertices in the vertex shader:
#version 320 es
layout (location = 0) in vec3 position;
out vec3 v_position;
uniform mat4 model_matrix;
uniform mat4 mvp_matrix;
void main() {
vec4 pos = vec4(position, 1.0);
v_position = vec3(model_matrix * pos);
gl_Position = mvp_matrix * vec4(position, 1.0);
}
Attempt to tessellate in the tessellation control shader:
#version 320 es
layout (vertices = 3) out;
in vec3 v_position;
out vec3 t_position;
void main()
{
// Set the control points of the output patch
t_position[gl_InvocationID] = v_position[gl_InvocationID];
// Calculate the tessellation levels
gl_TessLevelOuter[0] = 3.0;
gl_TessLevelOuter[1] = 3.0;
gl_TessLevelOuter[2] = 3.0;
gl_TessLevelInner[0] = gl_TessLevelOuter[2];
}
And then try to make a minimum working evaluation shader:
#version 320 es
layout(triangles, equal_spacing, ccw) in;
in vec3 t_position;
out vec3 f_position;
uniform mat4 mvp_matrix;
void main() {
f_position = t_position[0];
gl_Position = mvp_matrix * vec4(f_position, 1.0);
}
I call these shaders using glDrawArrays(GL_PATCHES, 0, vertex_num)
. However currently I see no output when attempting to tessellate. if instead I remove the tessellation shaders and draw with only the vertex and fragment shaders (after tweaking so that they compile). I do see the rectangle mesh, so I am confident the issue is in my shaders.
I am not getting compilation errors, and calling glGetError()
reports no error, so it's my logic that I think is wrong.
Edit:
Immediately prior to the draw call I also do:
glPatchParameteri(GL_PATCH_VERTICES, 3);
opengl-es glsl shader tessellation
You have to setglPatchParameteri( GL_PATCH_VERTICES, 3 )
before the draw call.
– Rabbid76
Nov 14 '18 at 19:22
I did, sorry forgot to mention it in post
– Makogan
Nov 14 '18 at 19:40
What aboutf_position = t_position[0]*gl_TessCoord.x + t_position[1]*gl_TessCoord.y + t_position[2]*gl_TessCoord.z;
– Rabbid76
Nov 14 '18 at 19:51
Still no output
– Makogan
Nov 14 '18 at 19:54
1
@Makong At triangle tessellationgl_TessCoord
contains the Barycentric coordinate of the tessellated position on the triangle. You have to define how the new vertex coordinate is calculated. See Tessellation - Triangles.
– Rabbid76
Nov 14 '18 at 20:16
|
show 2 more comments
I am trying to use the tessellation shaders in OpenGL ES 3.2, and have tried to follow 2 different tutorials without success.
I am currently drawing a flat rectangle, the goal is to elevate its surface. I receive the vertices in the vertex shader:
#version 320 es
layout (location = 0) in vec3 position;
out vec3 v_position;
uniform mat4 model_matrix;
uniform mat4 mvp_matrix;
void main() {
vec4 pos = vec4(position, 1.0);
v_position = vec3(model_matrix * pos);
gl_Position = mvp_matrix * vec4(position, 1.0);
}
Attempt to tessellate in the tessellation control shader:
#version 320 es
layout (vertices = 3) out;
in vec3 v_position;
out vec3 t_position;
void main()
{
// Set the control points of the output patch
t_position[gl_InvocationID] = v_position[gl_InvocationID];
// Calculate the tessellation levels
gl_TessLevelOuter[0] = 3.0;
gl_TessLevelOuter[1] = 3.0;
gl_TessLevelOuter[2] = 3.0;
gl_TessLevelInner[0] = gl_TessLevelOuter[2];
}
And then try to make a minimum working evaluation shader:
#version 320 es
layout(triangles, equal_spacing, ccw) in;
in vec3 t_position;
out vec3 f_position;
uniform mat4 mvp_matrix;
void main() {
f_position = t_position[0];
gl_Position = mvp_matrix * vec4(f_position, 1.0);
}
I call these shaders using glDrawArrays(GL_PATCHES, 0, vertex_num)
. However currently I see no output when attempting to tessellate. if instead I remove the tessellation shaders and draw with only the vertex and fragment shaders (after tweaking so that they compile). I do see the rectangle mesh, so I am confident the issue is in my shaders.
I am not getting compilation errors, and calling glGetError()
reports no error, so it's my logic that I think is wrong.
Edit:
Immediately prior to the draw call I also do:
glPatchParameteri(GL_PATCH_VERTICES, 3);
opengl-es glsl shader tessellation
I am trying to use the tessellation shaders in OpenGL ES 3.2, and have tried to follow 2 different tutorials without success.
I am currently drawing a flat rectangle, the goal is to elevate its surface. I receive the vertices in the vertex shader:
#version 320 es
layout (location = 0) in vec3 position;
out vec3 v_position;
uniform mat4 model_matrix;
uniform mat4 mvp_matrix;
void main() {
vec4 pos = vec4(position, 1.0);
v_position = vec3(model_matrix * pos);
gl_Position = mvp_matrix * vec4(position, 1.0);
}
Attempt to tessellate in the tessellation control shader:
#version 320 es
layout (vertices = 3) out;
in vec3 v_position;
out vec3 t_position;
void main()
{
// Set the control points of the output patch
t_position[gl_InvocationID] = v_position[gl_InvocationID];
// Calculate the tessellation levels
gl_TessLevelOuter[0] = 3.0;
gl_TessLevelOuter[1] = 3.0;
gl_TessLevelOuter[2] = 3.0;
gl_TessLevelInner[0] = gl_TessLevelOuter[2];
}
And then try to make a minimum working evaluation shader:
#version 320 es
layout(triangles, equal_spacing, ccw) in;
in vec3 t_position;
out vec3 f_position;
uniform mat4 mvp_matrix;
void main() {
f_position = t_position[0];
gl_Position = mvp_matrix * vec4(f_position, 1.0);
}
I call these shaders using glDrawArrays(GL_PATCHES, 0, vertex_num)
. However currently I see no output when attempting to tessellate. if instead I remove the tessellation shaders and draw with only the vertex and fragment shaders (after tweaking so that they compile). I do see the rectangle mesh, so I am confident the issue is in my shaders.
I am not getting compilation errors, and calling glGetError()
reports no error, so it's my logic that I think is wrong.
Edit:
Immediately prior to the draw call I also do:
glPatchParameteri(GL_PATCH_VERTICES, 3);
opengl-es glsl shader tessellation
opengl-es glsl shader tessellation
edited Nov 14 '18 at 19:51


genpfault
42.2k95399
42.2k95399
asked Nov 14 '18 at 19:17


MakoganMakogan
1,7841829
1,7841829
You have to setglPatchParameteri( GL_PATCH_VERTICES, 3 )
before the draw call.
– Rabbid76
Nov 14 '18 at 19:22
I did, sorry forgot to mention it in post
– Makogan
Nov 14 '18 at 19:40
What aboutf_position = t_position[0]*gl_TessCoord.x + t_position[1]*gl_TessCoord.y + t_position[2]*gl_TessCoord.z;
– Rabbid76
Nov 14 '18 at 19:51
Still no output
– Makogan
Nov 14 '18 at 19:54
1
@Makong At triangle tessellationgl_TessCoord
contains the Barycentric coordinate of the tessellated position on the triangle. You have to define how the new vertex coordinate is calculated. See Tessellation - Triangles.
– Rabbid76
Nov 14 '18 at 20:16
|
show 2 more comments
You have to setglPatchParameteri( GL_PATCH_VERTICES, 3 )
before the draw call.
– Rabbid76
Nov 14 '18 at 19:22
I did, sorry forgot to mention it in post
– Makogan
Nov 14 '18 at 19:40
What aboutf_position = t_position[0]*gl_TessCoord.x + t_position[1]*gl_TessCoord.y + t_position[2]*gl_TessCoord.z;
– Rabbid76
Nov 14 '18 at 19:51
Still no output
– Makogan
Nov 14 '18 at 19:54
1
@Makong At triangle tessellationgl_TessCoord
contains the Barycentric coordinate of the tessellated position on the triangle. You have to define how the new vertex coordinate is calculated. See Tessellation - Triangles.
– Rabbid76
Nov 14 '18 at 20:16
You have to set
glPatchParameteri( GL_PATCH_VERTICES, 3 )
before the draw call.– Rabbid76
Nov 14 '18 at 19:22
You have to set
glPatchParameteri( GL_PATCH_VERTICES, 3 )
before the draw call.– Rabbid76
Nov 14 '18 at 19:22
I did, sorry forgot to mention it in post
– Makogan
Nov 14 '18 at 19:40
I did, sorry forgot to mention it in post
– Makogan
Nov 14 '18 at 19:40
What about
f_position = t_position[0]*gl_TessCoord.x + t_position[1]*gl_TessCoord.y + t_position[2]*gl_TessCoord.z;
– Rabbid76
Nov 14 '18 at 19:51
What about
f_position = t_position[0]*gl_TessCoord.x + t_position[1]*gl_TessCoord.y + t_position[2]*gl_TessCoord.z;
– Rabbid76
Nov 14 '18 at 19:51
Still no output
– Makogan
Nov 14 '18 at 19:54
Still no output
– Makogan
Nov 14 '18 at 19:54
1
1
@Makong At triangle tessellation
gl_TessCoord
contains the Barycentric coordinate of the tessellated position on the triangle. You have to define how the new vertex coordinate is calculated. See Tessellation - Triangles.– Rabbid76
Nov 14 '18 at 20:16
@Makong At triangle tessellation
gl_TessCoord
contains the Barycentric coordinate of the tessellated position on the triangle. You have to define how the new vertex coordinate is calculated. See Tessellation - Triangles.– Rabbid76
Nov 14 '18 at 20:16
|
show 2 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53307335%2funderstanding-tessellation-shaders%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53307335%2funderstanding-tessellation-shaders%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
1en7E2P,An1MU BRz4 p,9fOUfYTAkmmzxwu1tELFUQV,0dO gD,LWcAd ug,4o6 qKVved WWMfp VBp0lq5p5lkxKyd,pu OnL8UYak iyI
You have to set
glPatchParameteri( GL_PATCH_VERTICES, 3 )
before the draw call.– Rabbid76
Nov 14 '18 at 19:22
I did, sorry forgot to mention it in post
– Makogan
Nov 14 '18 at 19:40
What about
f_position = t_position[0]*gl_TessCoord.x + t_position[1]*gl_TessCoord.y + t_position[2]*gl_TessCoord.z;
– Rabbid76
Nov 14 '18 at 19:51
Still no output
– Makogan
Nov 14 '18 at 19:54
1
@Makong At triangle tessellation
gl_TessCoord
contains the Barycentric coordinate of the tessellated position on the triangle. You have to define how the new vertex coordinate is calculated. See Tessellation - Triangles.– Rabbid76
Nov 14 '18 at 20:16