Call function of specific type in Go
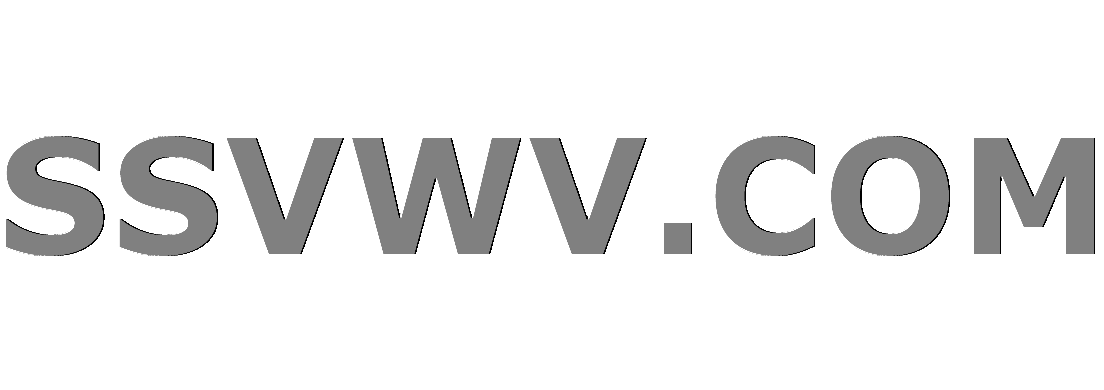
Multi tool use
I'm a complete Go newbie, so sorry for the question in advance.
I'm trying to work with a so-defined interface to connect to a message broker:
// Broker is an interface used for asynchronous messaging.
type Broker interface {
Options() Options
Address() string
Connect() error
Disconnect() error
Init(...Option) error
Publish(string, *Message, ...PublishOption) error
Subscribe(string, Handler, ...SubscribeOption) (Subscriber, error)
String() string
}
// Handler is used to process messages via a subscription of a topic.
// The handler is passed a publication interface which contains the
// message and optional Ack method to acknowledge receipt of the message.
type Handler func(Publication) error
// Publication is given to a subscription handler for processing
type Publication interface {
Topic() string
Message() *Message
Ack() error
}
I'm trying to use the Subscribe
-function to subscribe to a channel and thats the point where I'm struggeling right now.
My current approach is the following one:
natsBroker.Subscribe(
"QueueName",
func(p broker.Publication) {
fmt.Printf(p.Message)
},
)
The error output is cannot use func literal (type func(broker.Publication)) as type broker.Handler in argument to natsBroker.Subscribe
.
But how do I ensure that the function type actually is a broker.Handler
?
Thx for your time in advance!
Update
In case anybody is interested, the error return type was missing which caused the error, so it should look similar to that:
natsBroker.Subscribe(
"QueueName",
broker.Handler(func(p broker.Publication) error {
fmt.Printf(p.Topic())
return nil
}),
)
go go-micro
add a comment |
I'm a complete Go newbie, so sorry for the question in advance.
I'm trying to work with a so-defined interface to connect to a message broker:
// Broker is an interface used for asynchronous messaging.
type Broker interface {
Options() Options
Address() string
Connect() error
Disconnect() error
Init(...Option) error
Publish(string, *Message, ...PublishOption) error
Subscribe(string, Handler, ...SubscribeOption) (Subscriber, error)
String() string
}
// Handler is used to process messages via a subscription of a topic.
// The handler is passed a publication interface which contains the
// message and optional Ack method to acknowledge receipt of the message.
type Handler func(Publication) error
// Publication is given to a subscription handler for processing
type Publication interface {
Topic() string
Message() *Message
Ack() error
}
I'm trying to use the Subscribe
-function to subscribe to a channel and thats the point where I'm struggeling right now.
My current approach is the following one:
natsBroker.Subscribe(
"QueueName",
func(p broker.Publication) {
fmt.Printf(p.Message)
},
)
The error output is cannot use func literal (type func(broker.Publication)) as type broker.Handler in argument to natsBroker.Subscribe
.
But how do I ensure that the function type actually is a broker.Handler
?
Thx for your time in advance!
Update
In case anybody is interested, the error return type was missing which caused the error, so it should look similar to that:
natsBroker.Subscribe(
"QueueName",
broker.Handler(func(p broker.Publication) error {
fmt.Printf(p.Topic())
return nil
}),
)
go go-micro
add a comment |
I'm a complete Go newbie, so sorry for the question in advance.
I'm trying to work with a so-defined interface to connect to a message broker:
// Broker is an interface used for asynchronous messaging.
type Broker interface {
Options() Options
Address() string
Connect() error
Disconnect() error
Init(...Option) error
Publish(string, *Message, ...PublishOption) error
Subscribe(string, Handler, ...SubscribeOption) (Subscriber, error)
String() string
}
// Handler is used to process messages via a subscription of a topic.
// The handler is passed a publication interface which contains the
// message and optional Ack method to acknowledge receipt of the message.
type Handler func(Publication) error
// Publication is given to a subscription handler for processing
type Publication interface {
Topic() string
Message() *Message
Ack() error
}
I'm trying to use the Subscribe
-function to subscribe to a channel and thats the point where I'm struggeling right now.
My current approach is the following one:
natsBroker.Subscribe(
"QueueName",
func(p broker.Publication) {
fmt.Printf(p.Message)
},
)
The error output is cannot use func literal (type func(broker.Publication)) as type broker.Handler in argument to natsBroker.Subscribe
.
But how do I ensure that the function type actually is a broker.Handler
?
Thx for your time in advance!
Update
In case anybody is interested, the error return type was missing which caused the error, so it should look similar to that:
natsBroker.Subscribe(
"QueueName",
broker.Handler(func(p broker.Publication) error {
fmt.Printf(p.Topic())
return nil
}),
)
go go-micro
I'm a complete Go newbie, so sorry for the question in advance.
I'm trying to work with a so-defined interface to connect to a message broker:
// Broker is an interface used for asynchronous messaging.
type Broker interface {
Options() Options
Address() string
Connect() error
Disconnect() error
Init(...Option) error
Publish(string, *Message, ...PublishOption) error
Subscribe(string, Handler, ...SubscribeOption) (Subscriber, error)
String() string
}
// Handler is used to process messages via a subscription of a topic.
// The handler is passed a publication interface which contains the
// message and optional Ack method to acknowledge receipt of the message.
type Handler func(Publication) error
// Publication is given to a subscription handler for processing
type Publication interface {
Topic() string
Message() *Message
Ack() error
}
I'm trying to use the Subscribe
-function to subscribe to a channel and thats the point where I'm struggeling right now.
My current approach is the following one:
natsBroker.Subscribe(
"QueueName",
func(p broker.Publication) {
fmt.Printf(p.Message)
},
)
The error output is cannot use func literal (type func(broker.Publication)) as type broker.Handler in argument to natsBroker.Subscribe
.
But how do I ensure that the function type actually is a broker.Handler
?
Thx for your time in advance!
Update
In case anybody is interested, the error return type was missing which caused the error, so it should look similar to that:
natsBroker.Subscribe(
"QueueName",
broker.Handler(func(p broker.Publication) error {
fmt.Printf(p.Topic())
return nil
}),
)
go go-micro
go go-micro
edited Nov 14 '18 at 19:47
patreu22
asked Nov 14 '18 at 19:11


patreu22patreu22
183317
183317
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
As the error indicates, the parameter and what you're passing don't match:
type Handler func(Publication) error
func(p broker.Publication)
You have no return value. If you add a return value (even if you always return nil
), it will work fine.
Thanks Adrian, as you can see I already updated my question, because that was the critical point :)
– patreu22
Nov 14 '18 at 19:49
add a comment |
If your signature of your anonymous function matched that of the handler type declaration (Adrian correctly points out you're missing the error return), you should be able to just do a type conversion:
package main
import "fmt"
type Handler func(int) error
var a Handler
func main() {
a = Handler(func(i int) error {
return nil
})
fmt.Println(isHandler(a))
}
func isHandler(h Handler) bool {
return true
}
Since the the compiler knows at compiler-time that the types match, there's no need to do additional checking, like you might in the case of, say, a type assertion.
Thanks Keith, I was able to make it work :) I updated my answer for anyone interested in the concrete use-case
– patreu22
Nov 14 '18 at 19:46
1
"the signature of your anonymous function matches that of the handler type declaration" No it doesn't. The anonymous function has no return, the parameter takes a function that returnserror
.
– Adrian
Nov 14 '18 at 19:47
Oh you're right @Adrian, I missed that. I have updated the answer, thanks.
– Keith
Nov 14 '18 at 20:44
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53307233%2fcall-function-of-specific-type-in-go%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
As the error indicates, the parameter and what you're passing don't match:
type Handler func(Publication) error
func(p broker.Publication)
You have no return value. If you add a return value (even if you always return nil
), it will work fine.
Thanks Adrian, as you can see I already updated my question, because that was the critical point :)
– patreu22
Nov 14 '18 at 19:49
add a comment |
As the error indicates, the parameter and what you're passing don't match:
type Handler func(Publication) error
func(p broker.Publication)
You have no return value. If you add a return value (even if you always return nil
), it will work fine.
Thanks Adrian, as you can see I already updated my question, because that was the critical point :)
– patreu22
Nov 14 '18 at 19:49
add a comment |
As the error indicates, the parameter and what you're passing don't match:
type Handler func(Publication) error
func(p broker.Publication)
You have no return value. If you add a return value (even if you always return nil
), it will work fine.
As the error indicates, the parameter and what you're passing don't match:
type Handler func(Publication) error
func(p broker.Publication)
You have no return value. If you add a return value (even if you always return nil
), it will work fine.
answered Nov 14 '18 at 19:48
AdrianAdrian
18.7k33647
18.7k33647
Thanks Adrian, as you can see I already updated my question, because that was the critical point :)
– patreu22
Nov 14 '18 at 19:49
add a comment |
Thanks Adrian, as you can see I already updated my question, because that was the critical point :)
– patreu22
Nov 14 '18 at 19:49
Thanks Adrian, as you can see I already updated my question, because that was the critical point :)
– patreu22
Nov 14 '18 at 19:49
Thanks Adrian, as you can see I already updated my question, because that was the critical point :)
– patreu22
Nov 14 '18 at 19:49
add a comment |
If your signature of your anonymous function matched that of the handler type declaration (Adrian correctly points out you're missing the error return), you should be able to just do a type conversion:
package main
import "fmt"
type Handler func(int) error
var a Handler
func main() {
a = Handler(func(i int) error {
return nil
})
fmt.Println(isHandler(a))
}
func isHandler(h Handler) bool {
return true
}
Since the the compiler knows at compiler-time that the types match, there's no need to do additional checking, like you might in the case of, say, a type assertion.
Thanks Keith, I was able to make it work :) I updated my answer for anyone interested in the concrete use-case
– patreu22
Nov 14 '18 at 19:46
1
"the signature of your anonymous function matches that of the handler type declaration" No it doesn't. The anonymous function has no return, the parameter takes a function that returnserror
.
– Adrian
Nov 14 '18 at 19:47
Oh you're right @Adrian, I missed that. I have updated the answer, thanks.
– Keith
Nov 14 '18 at 20:44
add a comment |
If your signature of your anonymous function matched that of the handler type declaration (Adrian correctly points out you're missing the error return), you should be able to just do a type conversion:
package main
import "fmt"
type Handler func(int) error
var a Handler
func main() {
a = Handler(func(i int) error {
return nil
})
fmt.Println(isHandler(a))
}
func isHandler(h Handler) bool {
return true
}
Since the the compiler knows at compiler-time that the types match, there's no need to do additional checking, like you might in the case of, say, a type assertion.
Thanks Keith, I was able to make it work :) I updated my answer for anyone interested in the concrete use-case
– patreu22
Nov 14 '18 at 19:46
1
"the signature of your anonymous function matches that of the handler type declaration" No it doesn't. The anonymous function has no return, the parameter takes a function that returnserror
.
– Adrian
Nov 14 '18 at 19:47
Oh you're right @Adrian, I missed that. I have updated the answer, thanks.
– Keith
Nov 14 '18 at 20:44
add a comment |
If your signature of your anonymous function matched that of the handler type declaration (Adrian correctly points out you're missing the error return), you should be able to just do a type conversion:
package main
import "fmt"
type Handler func(int) error
var a Handler
func main() {
a = Handler(func(i int) error {
return nil
})
fmt.Println(isHandler(a))
}
func isHandler(h Handler) bool {
return true
}
Since the the compiler knows at compiler-time that the types match, there's no need to do additional checking, like you might in the case of, say, a type assertion.
If your signature of your anonymous function matched that of the handler type declaration (Adrian correctly points out you're missing the error return), you should be able to just do a type conversion:
package main
import "fmt"
type Handler func(int) error
var a Handler
func main() {
a = Handler(func(i int) error {
return nil
})
fmt.Println(isHandler(a))
}
func isHandler(h Handler) bool {
return true
}
Since the the compiler knows at compiler-time that the types match, there's no need to do additional checking, like you might in the case of, say, a type assertion.
edited Nov 14 '18 at 20:41
answered Nov 14 '18 at 19:21
KeithKeith
5415
5415
Thanks Keith, I was able to make it work :) I updated my answer for anyone interested in the concrete use-case
– patreu22
Nov 14 '18 at 19:46
1
"the signature of your anonymous function matches that of the handler type declaration" No it doesn't. The anonymous function has no return, the parameter takes a function that returnserror
.
– Adrian
Nov 14 '18 at 19:47
Oh you're right @Adrian, I missed that. I have updated the answer, thanks.
– Keith
Nov 14 '18 at 20:44
add a comment |
Thanks Keith, I was able to make it work :) I updated my answer for anyone interested in the concrete use-case
– patreu22
Nov 14 '18 at 19:46
1
"the signature of your anonymous function matches that of the handler type declaration" No it doesn't. The anonymous function has no return, the parameter takes a function that returnserror
.
– Adrian
Nov 14 '18 at 19:47
Oh you're right @Adrian, I missed that. I have updated the answer, thanks.
– Keith
Nov 14 '18 at 20:44
Thanks Keith, I was able to make it work :) I updated my answer for anyone interested in the concrete use-case
– patreu22
Nov 14 '18 at 19:46
Thanks Keith, I was able to make it work :) I updated my answer for anyone interested in the concrete use-case
– patreu22
Nov 14 '18 at 19:46
1
1
"the signature of your anonymous function matches that of the handler type declaration" No it doesn't. The anonymous function has no return, the parameter takes a function that returns
error
.– Adrian
Nov 14 '18 at 19:47
"the signature of your anonymous function matches that of the handler type declaration" No it doesn't. The anonymous function has no return, the parameter takes a function that returns
error
.– Adrian
Nov 14 '18 at 19:47
Oh you're right @Adrian, I missed that. I have updated the answer, thanks.
– Keith
Nov 14 '18 at 20:44
Oh you're right @Adrian, I missed that. I have updated the answer, thanks.
– Keith
Nov 14 '18 at 20:44
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53307233%2fcall-function-of-specific-type-in-go%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6H65ugdJXqrQZdsY16O HsQB1WUgn,WFEAus,oWZT4SkSITXfdB S0TF