Unable to use Spring @Cacheable and @EnableCaching
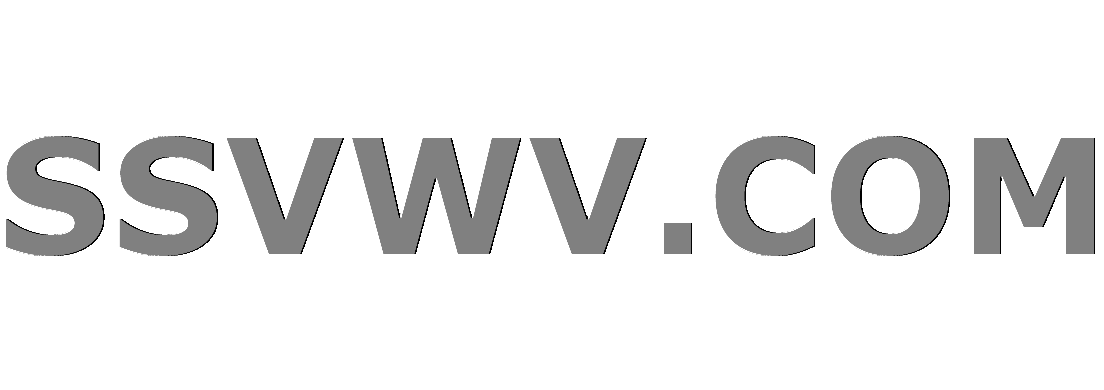
Multi tool use
I'm trying to replace my old:
@Component
public interface MyEntityRepository extends JpaRepository<MyEntity, Integer> {
@QueryHints({@QueryHint(name = CACHEABLE, value = "true")})
MyEntity findByName(String name);
}
by this:
@Component
public interface MyEntityRepository extends JpaRepository<MyEntity, Integer> {
@Cacheable(value = "entities")
MyEntity findByName(String name);
}
Because I want to use advanced caching features like no caching of null values, etc.
To do so, I followed Spring tutorial https://spring.io/guides/gs/caching/
If I don't annotate my Application.java, caching simply doesn't work.
But if I add @EnableCaching
and a CacheManager bean:
package my.application.config;
@EnableWebMvc
@ComponentScan(basePackages = {"my.application"})
@Configuration
@EnableCaching
public class Application extends WebMvcConfigurerAdapter {
@Bean
public CacheManager cacheManager() {
return new ConcurrentMapCacheManager("entities");
}
// ...
}
I get the following error at startup:
java.lang.IllegalStateException: No CacheResolver specified, and no bean of type CacheManager found. Register a CacheManager bean or remove the @EnableCaching annotation from your configuration
I get the same error if I replace My CacheManager bean by a CacheResolver bean like:
@Bean
public CacheResolver cacheResolver() {
return new SimpleCacheResolver(new ConcurrentMapCacheManager("entities"));
}
Do I miss something ?
java spring caching spring-data
add a comment |
I'm trying to replace my old:
@Component
public interface MyEntityRepository extends JpaRepository<MyEntity, Integer> {
@QueryHints({@QueryHint(name = CACHEABLE, value = "true")})
MyEntity findByName(String name);
}
by this:
@Component
public interface MyEntityRepository extends JpaRepository<MyEntity, Integer> {
@Cacheable(value = "entities")
MyEntity findByName(String name);
}
Because I want to use advanced caching features like no caching of null values, etc.
To do so, I followed Spring tutorial https://spring.io/guides/gs/caching/
If I don't annotate my Application.java, caching simply doesn't work.
But if I add @EnableCaching
and a CacheManager bean:
package my.application.config;
@EnableWebMvc
@ComponentScan(basePackages = {"my.application"})
@Configuration
@EnableCaching
public class Application extends WebMvcConfigurerAdapter {
@Bean
public CacheManager cacheManager() {
return new ConcurrentMapCacheManager("entities");
}
// ...
}
I get the following error at startup:
java.lang.IllegalStateException: No CacheResolver specified, and no bean of type CacheManager found. Register a CacheManager bean or remove the @EnableCaching annotation from your configuration
I get the same error if I replace My CacheManager bean by a CacheResolver bean like:
@Bean
public CacheResolver cacheResolver() {
return new SimpleCacheResolver(new ConcurrentMapCacheManager("entities"));
}
Do I miss something ?
java spring caching spring-data
Implement theCacheConfigurer
interface. and implement the methods. A bean of that type is needed to properly configure caching.
– M. Deinum
Sep 1 '15 at 6:55
1
Try to name your cacheManager with the name (entities
) you using in the@cacheable
annotation.
– herau
Sep 2 '15 at 7:13
@M. Deinum yesterday What is thisCacheConfigurer
interface ? It's in no documentation.
– Pleymor
Sep 2 '15 at 7:52
Yes it is. Check the tip/hint here.
– M. Deinum
Sep 2 '15 at 8:00
And are you really following that tutorial or are you "following" that tutorial. You have a web application the tutorial doesn't. The tutorial assumes Spring Boot you aren't... So those are quite different things.
– M. Deinum
Sep 2 '15 at 8:04
add a comment |
I'm trying to replace my old:
@Component
public interface MyEntityRepository extends JpaRepository<MyEntity, Integer> {
@QueryHints({@QueryHint(name = CACHEABLE, value = "true")})
MyEntity findByName(String name);
}
by this:
@Component
public interface MyEntityRepository extends JpaRepository<MyEntity, Integer> {
@Cacheable(value = "entities")
MyEntity findByName(String name);
}
Because I want to use advanced caching features like no caching of null values, etc.
To do so, I followed Spring tutorial https://spring.io/guides/gs/caching/
If I don't annotate my Application.java, caching simply doesn't work.
But if I add @EnableCaching
and a CacheManager bean:
package my.application.config;
@EnableWebMvc
@ComponentScan(basePackages = {"my.application"})
@Configuration
@EnableCaching
public class Application extends WebMvcConfigurerAdapter {
@Bean
public CacheManager cacheManager() {
return new ConcurrentMapCacheManager("entities");
}
// ...
}
I get the following error at startup:
java.lang.IllegalStateException: No CacheResolver specified, and no bean of type CacheManager found. Register a CacheManager bean or remove the @EnableCaching annotation from your configuration
I get the same error if I replace My CacheManager bean by a CacheResolver bean like:
@Bean
public CacheResolver cacheResolver() {
return new SimpleCacheResolver(new ConcurrentMapCacheManager("entities"));
}
Do I miss something ?
java spring caching spring-data
I'm trying to replace my old:
@Component
public interface MyEntityRepository extends JpaRepository<MyEntity, Integer> {
@QueryHints({@QueryHint(name = CACHEABLE, value = "true")})
MyEntity findByName(String name);
}
by this:
@Component
public interface MyEntityRepository extends JpaRepository<MyEntity, Integer> {
@Cacheable(value = "entities")
MyEntity findByName(String name);
}
Because I want to use advanced caching features like no caching of null values, etc.
To do so, I followed Spring tutorial https://spring.io/guides/gs/caching/
If I don't annotate my Application.java, caching simply doesn't work.
But if I add @EnableCaching
and a CacheManager bean:
package my.application.config;
@EnableWebMvc
@ComponentScan(basePackages = {"my.application"})
@Configuration
@EnableCaching
public class Application extends WebMvcConfigurerAdapter {
@Bean
public CacheManager cacheManager() {
return new ConcurrentMapCacheManager("entities");
}
// ...
}
I get the following error at startup:
java.lang.IllegalStateException: No CacheResolver specified, and no bean of type CacheManager found. Register a CacheManager bean or remove the @EnableCaching annotation from your configuration
I get the same error if I replace My CacheManager bean by a CacheResolver bean like:
@Bean
public CacheResolver cacheResolver() {
return new SimpleCacheResolver(new ConcurrentMapCacheManager("entities"));
}
Do I miss something ?
java spring caching spring-data
java spring caching spring-data
asked Sep 1 '15 at 6:23


PleymorPleymor
7991726
7991726
Implement theCacheConfigurer
interface. and implement the methods. A bean of that type is needed to properly configure caching.
– M. Deinum
Sep 1 '15 at 6:55
1
Try to name your cacheManager with the name (entities
) you using in the@cacheable
annotation.
– herau
Sep 2 '15 at 7:13
@M. Deinum yesterday What is thisCacheConfigurer
interface ? It's in no documentation.
– Pleymor
Sep 2 '15 at 7:52
Yes it is. Check the tip/hint here.
– M. Deinum
Sep 2 '15 at 8:00
And are you really following that tutorial or are you "following" that tutorial. You have a web application the tutorial doesn't. The tutorial assumes Spring Boot you aren't... So those are quite different things.
– M. Deinum
Sep 2 '15 at 8:04
add a comment |
Implement theCacheConfigurer
interface. and implement the methods. A bean of that type is needed to properly configure caching.
– M. Deinum
Sep 1 '15 at 6:55
1
Try to name your cacheManager with the name (entities
) you using in the@cacheable
annotation.
– herau
Sep 2 '15 at 7:13
@M. Deinum yesterday What is thisCacheConfigurer
interface ? It's in no documentation.
– Pleymor
Sep 2 '15 at 7:52
Yes it is. Check the tip/hint here.
– M. Deinum
Sep 2 '15 at 8:00
And are you really following that tutorial or are you "following" that tutorial. You have a web application the tutorial doesn't. The tutorial assumes Spring Boot you aren't... So those are quite different things.
– M. Deinum
Sep 2 '15 at 8:04
Implement the
CacheConfigurer
interface. and implement the methods. A bean of that type is needed to properly configure caching.– M. Deinum
Sep 1 '15 at 6:55
Implement the
CacheConfigurer
interface. and implement the methods. A bean of that type is needed to properly configure caching.– M. Deinum
Sep 1 '15 at 6:55
1
1
Try to name your cacheManager with the name (
entities
) you using in the @cacheable
annotation.– herau
Sep 2 '15 at 7:13
Try to name your cacheManager with the name (
entities
) you using in the @cacheable
annotation.– herau
Sep 2 '15 at 7:13
@M. Deinum yesterday What is this
CacheConfigurer
interface ? It's in no documentation.– Pleymor
Sep 2 '15 at 7:52
@M. Deinum yesterday What is this
CacheConfigurer
interface ? It's in no documentation.– Pleymor
Sep 2 '15 at 7:52
Yes it is. Check the tip/hint here.
– M. Deinum
Sep 2 '15 at 8:00
Yes it is. Check the tip/hint here.
– M. Deinum
Sep 2 '15 at 8:00
And are you really following that tutorial or are you "following" that tutorial. You have a web application the tutorial doesn't. The tutorial assumes Spring Boot you aren't... So those are quite different things.
– M. Deinum
Sep 2 '15 at 8:04
And are you really following that tutorial or are you "following" that tutorial. You have a web application the tutorial doesn't. The tutorial assumes Spring Boot you aren't... So those are quite different things.
– M. Deinum
Sep 2 '15 at 8:04
add a comment |
2 Answers
2
active
oldest
votes
@herau You were right I had to name the bean !
The problem was that there were another bean "cacheManager", so finally, I didn't annotate Application, and created a configuration as:
@EnableCaching
@Configuration
public class CacheConf{
@Bean(name = "springCM")
public CacheManager cacheManager() {
return new ConcurrentMapCacheManager("entities");
}
}
in MyEntityRepository
:
@Cacheable(value = "entities", cacheManager = "springCM")
MyEntity findByName(String name);
add a comment |
In my case the Spring Boot library was old, and there was no way to easily upgrade it. So I used EHCache 2 version, and it worked in my application. Here is a project I found useful to refer to: https://github.com/TechPrimers/spring-ehcache-example/blob/master/src/main/resources/ehcache.xml
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f32324832%2funable-to-use-spring-cacheable-and-enablecaching%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
@herau You were right I had to name the bean !
The problem was that there were another bean "cacheManager", so finally, I didn't annotate Application, and created a configuration as:
@EnableCaching
@Configuration
public class CacheConf{
@Bean(name = "springCM")
public CacheManager cacheManager() {
return new ConcurrentMapCacheManager("entities");
}
}
in MyEntityRepository
:
@Cacheable(value = "entities", cacheManager = "springCM")
MyEntity findByName(String name);
add a comment |
@herau You were right I had to name the bean !
The problem was that there were another bean "cacheManager", so finally, I didn't annotate Application, and created a configuration as:
@EnableCaching
@Configuration
public class CacheConf{
@Bean(name = "springCM")
public CacheManager cacheManager() {
return new ConcurrentMapCacheManager("entities");
}
}
in MyEntityRepository
:
@Cacheable(value = "entities", cacheManager = "springCM")
MyEntity findByName(String name);
add a comment |
@herau You were right I had to name the bean !
The problem was that there were another bean "cacheManager", so finally, I didn't annotate Application, and created a configuration as:
@EnableCaching
@Configuration
public class CacheConf{
@Bean(name = "springCM")
public CacheManager cacheManager() {
return new ConcurrentMapCacheManager("entities");
}
}
in MyEntityRepository
:
@Cacheable(value = "entities", cacheManager = "springCM")
MyEntity findByName(String name);
@herau You were right I had to name the bean !
The problem was that there were another bean "cacheManager", so finally, I didn't annotate Application, and created a configuration as:
@EnableCaching
@Configuration
public class CacheConf{
@Bean(name = "springCM")
public CacheManager cacheManager() {
return new ConcurrentMapCacheManager("entities");
}
}
in MyEntityRepository
:
@Cacheable(value = "entities", cacheManager = "springCM")
MyEntity findByName(String name);
edited Mar 2 '16 at 15:01
answered Sep 2 '15 at 8:24


PleymorPleymor
7991726
7991726
add a comment |
add a comment |
In my case the Spring Boot library was old, and there was no way to easily upgrade it. So I used EHCache 2 version, and it worked in my application. Here is a project I found useful to refer to: https://github.com/TechPrimers/spring-ehcache-example/blob/master/src/main/resources/ehcache.xml
add a comment |
In my case the Spring Boot library was old, and there was no way to easily upgrade it. So I used EHCache 2 version, and it worked in my application. Here is a project I found useful to refer to: https://github.com/TechPrimers/spring-ehcache-example/blob/master/src/main/resources/ehcache.xml
add a comment |
In my case the Spring Boot library was old, and there was no way to easily upgrade it. So I used EHCache 2 version, and it worked in my application. Here is a project I found useful to refer to: https://github.com/TechPrimers/spring-ehcache-example/blob/master/src/main/resources/ehcache.xml
In my case the Spring Boot library was old, and there was no way to easily upgrade it. So I used EHCache 2 version, and it worked in my application. Here is a project I found useful to refer to: https://github.com/TechPrimers/spring-ehcache-example/blob/master/src/main/resources/ehcache.xml
answered Nov 15 '18 at 0:41
AliyaAliya
9051015
9051015
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f32324832%2funable-to-use-spring-cacheable-and-enablecaching%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
y8VeG9A5Sc 5,7eTD2V FjdaBHGt7WFNH6KTKbFdMlgCnFpCpydIUDuVa,r17Te,fsA g2,mQ
Implement the
CacheConfigurer
interface. and implement the methods. A bean of that type is needed to properly configure caching.– M. Deinum
Sep 1 '15 at 6:55
1
Try to name your cacheManager with the name (
entities
) you using in the@cacheable
annotation.– herau
Sep 2 '15 at 7:13
@M. Deinum yesterday What is this
CacheConfigurer
interface ? It's in no documentation.– Pleymor
Sep 2 '15 at 7:52
Yes it is. Check the tip/hint here.
– M. Deinum
Sep 2 '15 at 8:00
And are you really following that tutorial or are you "following" that tutorial. You have a web application the tutorial doesn't. The tutorial assumes Spring Boot you aren't... So those are quite different things.
– M. Deinum
Sep 2 '15 at 8:04