How to displaying a numpy int16 array correctly with tkinter?
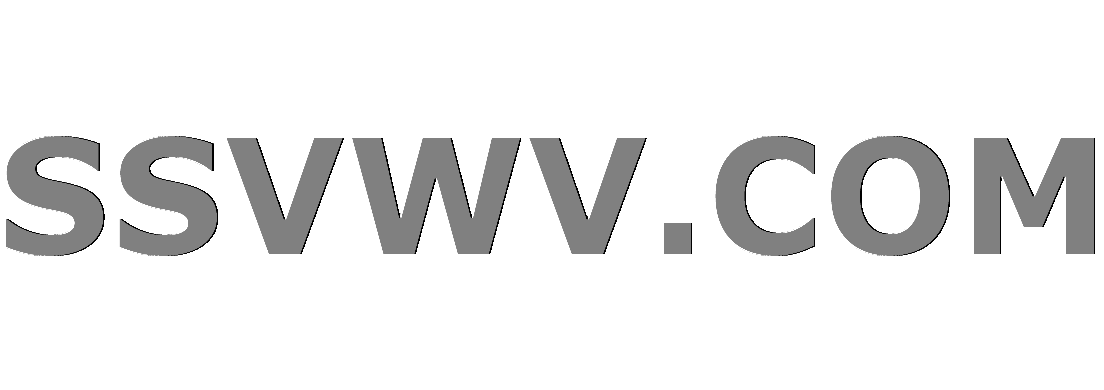
Multi tool use
When loading an image into tkinter, when the image is stored as a numpy array as int16, the image displayed has pixel intensity thresholds for int8, meaning that everything looks awful (all values above 255 -which are plenty- are white). How can I set the min/max values for thresholding on tkinter?
import tkinter as tk
import numpy as np
from PIL import Image, ImageTk
root = tk.Tk()
array = np.reshape(np.array(range(0,200*200), dtype='int32'),(200,200))
img = ImageTk.PhotoImage(image=Image.fromarray(array))
canvas = tk.Canvas(root,width=300,height=300)
canvas.pack()
canvas.create_image(20,20, anchor="nw", image=img)
root.mainloop()
This is how the image looks like:
This does not seem to be a PIL problem, as the 'image' stored in image seems to keep the original values:
image=Image.fromarray(array)
image
python numpy tkinter
|
show 9 more comments
When loading an image into tkinter, when the image is stored as a numpy array as int16, the image displayed has pixel intensity thresholds for int8, meaning that everything looks awful (all values above 255 -which are plenty- are white). How can I set the min/max values for thresholding on tkinter?
import tkinter as tk
import numpy as np
from PIL import Image, ImageTk
root = tk.Tk()
array = np.reshape(np.array(range(0,200*200), dtype='int32'),(200,200))
img = ImageTk.PhotoImage(image=Image.fromarray(array))
canvas = tk.Canvas(root,width=300,height=300)
canvas.pack()
canvas.create_image(20,20, anchor="nw", image=img)
root.mainloop()
This is how the image looks like:
This does not seem to be a PIL problem, as the 'image' stored in image seems to keep the original values:
image=Image.fromarray(array)
image
python numpy tkinter
I don't think the PIL supports 16 bit integer images—at least it's not on the list shown in this documentation.
– martineau
Nov 15 '18 at 1:51
@martineau isn't that really weird? Or am I missing something? How can one display int16 images on tkinter, without depending on PIL?
– hirschme
Nov 15 '18 at 1:59
By converting them to one of the ones it does support, like 8- or 32-bit. Should be easy with numpy...although I'm no expert on using it.
– martineau
Nov 15 '18 at 2:01
int32 also displays an image with completely off threshold values. Apparently it is sticking to int8...
– hirschme
Nov 15 '18 at 2:03
Modify the code in your question so it creates annp.array
array with something in like your image for testing with please.
– martineau
Nov 15 '18 at 2:07
|
show 9 more comments
When loading an image into tkinter, when the image is stored as a numpy array as int16, the image displayed has pixel intensity thresholds for int8, meaning that everything looks awful (all values above 255 -which are plenty- are white). How can I set the min/max values for thresholding on tkinter?
import tkinter as tk
import numpy as np
from PIL import Image, ImageTk
root = tk.Tk()
array = np.reshape(np.array(range(0,200*200), dtype='int32'),(200,200))
img = ImageTk.PhotoImage(image=Image.fromarray(array))
canvas = tk.Canvas(root,width=300,height=300)
canvas.pack()
canvas.create_image(20,20, anchor="nw", image=img)
root.mainloop()
This is how the image looks like:
This does not seem to be a PIL problem, as the 'image' stored in image seems to keep the original values:
image=Image.fromarray(array)
image
python numpy tkinter
When loading an image into tkinter, when the image is stored as a numpy array as int16, the image displayed has pixel intensity thresholds for int8, meaning that everything looks awful (all values above 255 -which are plenty- are white). How can I set the min/max values for thresholding on tkinter?
import tkinter as tk
import numpy as np
from PIL import Image, ImageTk
root = tk.Tk()
array = np.reshape(np.array(range(0,200*200), dtype='int32'),(200,200))
img = ImageTk.PhotoImage(image=Image.fromarray(array))
canvas = tk.Canvas(root,width=300,height=300)
canvas.pack()
canvas.create_image(20,20, anchor="nw", image=img)
root.mainloop()
This is how the image looks like:
This does not seem to be a PIL problem, as the 'image' stored in image seems to keep the original values:
image=Image.fromarray(array)
image
python numpy tkinter
python numpy tkinter
edited Nov 15 '18 at 2:12
hirschme
asked Nov 15 '18 at 0:47
hirschmehirschme
13119
13119
I don't think the PIL supports 16 bit integer images—at least it's not on the list shown in this documentation.
– martineau
Nov 15 '18 at 1:51
@martineau isn't that really weird? Or am I missing something? How can one display int16 images on tkinter, without depending on PIL?
– hirschme
Nov 15 '18 at 1:59
By converting them to one of the ones it does support, like 8- or 32-bit. Should be easy with numpy...although I'm no expert on using it.
– martineau
Nov 15 '18 at 2:01
int32 also displays an image with completely off threshold values. Apparently it is sticking to int8...
– hirschme
Nov 15 '18 at 2:03
Modify the code in your question so it creates annp.array
array with something in like your image for testing with please.
– martineau
Nov 15 '18 at 2:07
|
show 9 more comments
I don't think the PIL supports 16 bit integer images—at least it's not on the list shown in this documentation.
– martineau
Nov 15 '18 at 1:51
@martineau isn't that really weird? Or am I missing something? How can one display int16 images on tkinter, without depending on PIL?
– hirschme
Nov 15 '18 at 1:59
By converting them to one of the ones it does support, like 8- or 32-bit. Should be easy with numpy...although I'm no expert on using it.
– martineau
Nov 15 '18 at 2:01
int32 also displays an image with completely off threshold values. Apparently it is sticking to int8...
– hirschme
Nov 15 '18 at 2:03
Modify the code in your question so it creates annp.array
array with something in like your image for testing with please.
– martineau
Nov 15 '18 at 2:07
I don't think the PIL supports 16 bit integer images—at least it's not on the list shown in this documentation.
– martineau
Nov 15 '18 at 1:51
I don't think the PIL supports 16 bit integer images—at least it's not on the list shown in this documentation.
– martineau
Nov 15 '18 at 1:51
@martineau isn't that really weird? Or am I missing something? How can one display int16 images on tkinter, without depending on PIL?
– hirschme
Nov 15 '18 at 1:59
@martineau isn't that really weird? Or am I missing something? How can one display int16 images on tkinter, without depending on PIL?
– hirschme
Nov 15 '18 at 1:59
By converting them to one of the ones it does support, like 8- or 32-bit. Should be easy with numpy...although I'm no expert on using it.
– martineau
Nov 15 '18 at 2:01
By converting them to one of the ones it does support, like 8- or 32-bit. Should be easy with numpy...although I'm no expert on using it.
– martineau
Nov 15 '18 at 2:01
int32 also displays an image with completely off threshold values. Apparently it is sticking to int8...
– hirschme
Nov 15 '18 at 2:03
int32 also displays an image with completely off threshold values. Apparently it is sticking to int8...
– hirschme
Nov 15 '18 at 2:03
Modify the code in your question so it creates an
np.array
array with something in like your image for testing with please.– martineau
Nov 15 '18 at 2:07
Modify the code in your question so it creates an
np.array
array with something in like your image for testing with please.– martineau
Nov 15 '18 at 2:07
|
show 9 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53310908%2fhow-to-displaying-a-numpy-int16-array-correctly-with-tkinter%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53310908%2fhow-to-displaying-a-numpy-int16-array-correctly-with-tkinter%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VunXAOTug,Tb6Yc6BM5Mzi3nAcYbxJew5vQg25Gol7QusI32Ltfbd T623M7Ucq,iwixXjJ1xkAkiwVbUNECwLIVOWLqF,E7OXnm
I don't think the PIL supports 16 bit integer images—at least it's not on the list shown in this documentation.
– martineau
Nov 15 '18 at 1:51
@martineau isn't that really weird? Or am I missing something? How can one display int16 images on tkinter, without depending on PIL?
– hirschme
Nov 15 '18 at 1:59
By converting them to one of the ones it does support, like 8- or 32-bit. Should be easy with numpy...although I'm no expert on using it.
– martineau
Nov 15 '18 at 2:01
int32 also displays an image with completely off threshold values. Apparently it is sticking to int8...
– hirschme
Nov 15 '18 at 2:03
Modify the code in your question so it creates an
np.array
array with something in like your image for testing with please.– martineau
Nov 15 '18 at 2:07