Postfix Calculator Java -cannot resolve or is not a field
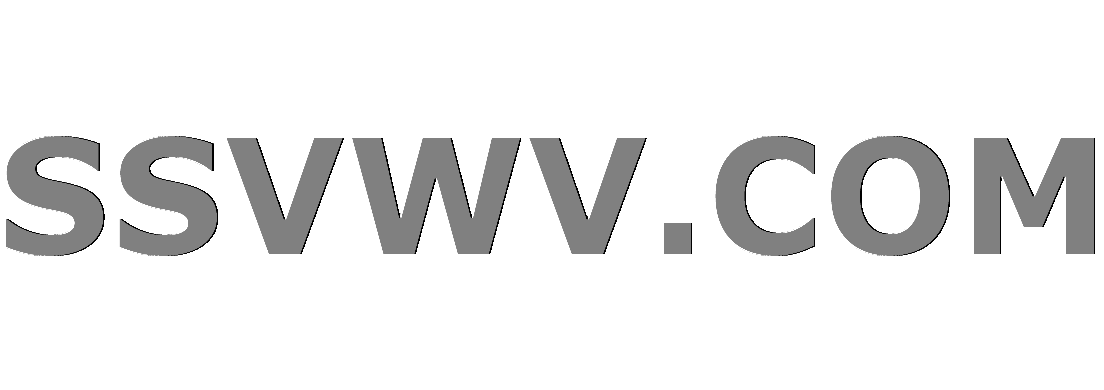
Multi tool use
I am encountering this error in the postfix calculator: the integerOperand cannot be resolved or is not a field. Below I have shown the main code, and the code from the IntegerOperand class file. How can I fix this? I am trying to call the add function from the IntegerOperand class.
public class IntegerOperand implements CalculatorOperand<IntegerOperand> {
BigInteger value;
IntegerOperand (BigInteger value) {
this.value = value;
}
public IntegerOperand add (IntegerOperand that) {
return new IntegerOperand(this.value.add(that.value));
}
public IntegerOperand subtract (IntegerOperand that) {
return new IntegerOperand(this.value.subtract(that.value));
}
public IntegerOperand multiply (IntegerOperand that) {
return new IntegerOperand(this.value.multiply(that.value));
}
public String toString () {
return value.toString();
}
}
public void operation (OperationType operation) {
T t1;
T t2;
if(stack.isEmpty())
{
t2= stack.pop();
t1= stack.pop();
stack.push(t1.IntegerOperand.add(t2));
}
}
java list stack
add a comment |
I am encountering this error in the postfix calculator: the integerOperand cannot be resolved or is not a field. Below I have shown the main code, and the code from the IntegerOperand class file. How can I fix this? I am trying to call the add function from the IntegerOperand class.
public class IntegerOperand implements CalculatorOperand<IntegerOperand> {
BigInteger value;
IntegerOperand (BigInteger value) {
this.value = value;
}
public IntegerOperand add (IntegerOperand that) {
return new IntegerOperand(this.value.add(that.value));
}
public IntegerOperand subtract (IntegerOperand that) {
return new IntegerOperand(this.value.subtract(that.value));
}
public IntegerOperand multiply (IntegerOperand that) {
return new IntegerOperand(this.value.multiply(that.value));
}
public String toString () {
return value.toString();
}
}
public void operation (OperationType operation) {
T t1;
T t2;
if(stack.isEmpty())
{
t2= stack.pop();
t1= stack.pop();
stack.push(t1.IntegerOperand.add(t2));
}
}
java list stack
add a comment |
I am encountering this error in the postfix calculator: the integerOperand cannot be resolved or is not a field. Below I have shown the main code, and the code from the IntegerOperand class file. How can I fix this? I am trying to call the add function from the IntegerOperand class.
public class IntegerOperand implements CalculatorOperand<IntegerOperand> {
BigInteger value;
IntegerOperand (BigInteger value) {
this.value = value;
}
public IntegerOperand add (IntegerOperand that) {
return new IntegerOperand(this.value.add(that.value));
}
public IntegerOperand subtract (IntegerOperand that) {
return new IntegerOperand(this.value.subtract(that.value));
}
public IntegerOperand multiply (IntegerOperand that) {
return new IntegerOperand(this.value.multiply(that.value));
}
public String toString () {
return value.toString();
}
}
public void operation (OperationType operation) {
T t1;
T t2;
if(stack.isEmpty())
{
t2= stack.pop();
t1= stack.pop();
stack.push(t1.IntegerOperand.add(t2));
}
}
java list stack
I am encountering this error in the postfix calculator: the integerOperand cannot be resolved or is not a field. Below I have shown the main code, and the code from the IntegerOperand class file. How can I fix this? I am trying to call the add function from the IntegerOperand class.
public class IntegerOperand implements CalculatorOperand<IntegerOperand> {
BigInteger value;
IntegerOperand (BigInteger value) {
this.value = value;
}
public IntegerOperand add (IntegerOperand that) {
return new IntegerOperand(this.value.add(that.value));
}
public IntegerOperand subtract (IntegerOperand that) {
return new IntegerOperand(this.value.subtract(that.value));
}
public IntegerOperand multiply (IntegerOperand that) {
return new IntegerOperand(this.value.multiply(that.value));
}
public String toString () {
return value.toString();
}
}
public void operation (OperationType operation) {
T t1;
T t2;
if(stack.isEmpty())
{
t2= stack.pop();
t1= stack.pop();
stack.push(t1.IntegerOperand.add(t2));
}
}
java list stack
java list stack
edited Nov 13 at 13:21
Stephan
333211
333211
asked Nov 12 at 13:25


MunchiesOats
61
61
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
The main issue is that you are not calling the function correctly.
// You don't need the class name
//stack.push(t1.IntegerOperand.add(t2));
stack.push(t1.add(t2));
Second, you check if the stack is empty, then if it is, you try to pop
from it. But you should check if the stack is not empty: if (!stack.isEmpty())
. But since you then do 2 calls to pop
you should instead check if there are at least 2 items in the stack.
if (stack.size() >= 2) {
t2 = stack.pop();
t1 = stack.pop();
stack.push(t1.add(t2));
}
Thank you! However, the function has to allow for stacks with less than 2 items in the stack, and the code cannot modify the stack if it contains less than 2 items. What should I consider in this case?
– MunchiesOats
Nov 12 at 14:13
@ChloePupaiboon This code will not modify the stack if it has less than 2 items. For stacks with one item, you could add anelse
to handle that case.
– Johnny Mopp
Nov 12 at 14:21
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53263155%2fpostfix-calculator-java-cannot-resolve-or-is-not-a-field%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The main issue is that you are not calling the function correctly.
// You don't need the class name
//stack.push(t1.IntegerOperand.add(t2));
stack.push(t1.add(t2));
Second, you check if the stack is empty, then if it is, you try to pop
from it. But you should check if the stack is not empty: if (!stack.isEmpty())
. But since you then do 2 calls to pop
you should instead check if there are at least 2 items in the stack.
if (stack.size() >= 2) {
t2 = stack.pop();
t1 = stack.pop();
stack.push(t1.add(t2));
}
Thank you! However, the function has to allow for stacks with less than 2 items in the stack, and the code cannot modify the stack if it contains less than 2 items. What should I consider in this case?
– MunchiesOats
Nov 12 at 14:13
@ChloePupaiboon This code will not modify the stack if it has less than 2 items. For stacks with one item, you could add anelse
to handle that case.
– Johnny Mopp
Nov 12 at 14:21
add a comment |
The main issue is that you are not calling the function correctly.
// You don't need the class name
//stack.push(t1.IntegerOperand.add(t2));
stack.push(t1.add(t2));
Second, you check if the stack is empty, then if it is, you try to pop
from it. But you should check if the stack is not empty: if (!stack.isEmpty())
. But since you then do 2 calls to pop
you should instead check if there are at least 2 items in the stack.
if (stack.size() >= 2) {
t2 = stack.pop();
t1 = stack.pop();
stack.push(t1.add(t2));
}
Thank you! However, the function has to allow for stacks with less than 2 items in the stack, and the code cannot modify the stack if it contains less than 2 items. What should I consider in this case?
– MunchiesOats
Nov 12 at 14:13
@ChloePupaiboon This code will not modify the stack if it has less than 2 items. For stacks with one item, you could add anelse
to handle that case.
– Johnny Mopp
Nov 12 at 14:21
add a comment |
The main issue is that you are not calling the function correctly.
// You don't need the class name
//stack.push(t1.IntegerOperand.add(t2));
stack.push(t1.add(t2));
Second, you check if the stack is empty, then if it is, you try to pop
from it. But you should check if the stack is not empty: if (!stack.isEmpty())
. But since you then do 2 calls to pop
you should instead check if there are at least 2 items in the stack.
if (stack.size() >= 2) {
t2 = stack.pop();
t1 = stack.pop();
stack.push(t1.add(t2));
}
The main issue is that you are not calling the function correctly.
// You don't need the class name
//stack.push(t1.IntegerOperand.add(t2));
stack.push(t1.add(t2));
Second, you check if the stack is empty, then if it is, you try to pop
from it. But you should check if the stack is not empty: if (!stack.isEmpty())
. But since you then do 2 calls to pop
you should instead check if there are at least 2 items in the stack.
if (stack.size() >= 2) {
t2 = stack.pop();
t1 = stack.pop();
stack.push(t1.add(t2));
}
answered Nov 12 at 13:42


Johnny Mopp
6,77722344
6,77722344
Thank you! However, the function has to allow for stacks with less than 2 items in the stack, and the code cannot modify the stack if it contains less than 2 items. What should I consider in this case?
– MunchiesOats
Nov 12 at 14:13
@ChloePupaiboon This code will not modify the stack if it has less than 2 items. For stacks with one item, you could add anelse
to handle that case.
– Johnny Mopp
Nov 12 at 14:21
add a comment |
Thank you! However, the function has to allow for stacks with less than 2 items in the stack, and the code cannot modify the stack if it contains less than 2 items. What should I consider in this case?
– MunchiesOats
Nov 12 at 14:13
@ChloePupaiboon This code will not modify the stack if it has less than 2 items. For stacks with one item, you could add anelse
to handle that case.
– Johnny Mopp
Nov 12 at 14:21
Thank you! However, the function has to allow for stacks with less than 2 items in the stack, and the code cannot modify the stack if it contains less than 2 items. What should I consider in this case?
– MunchiesOats
Nov 12 at 14:13
Thank you! However, the function has to allow for stacks with less than 2 items in the stack, and the code cannot modify the stack if it contains less than 2 items. What should I consider in this case?
– MunchiesOats
Nov 12 at 14:13
@ChloePupaiboon This code will not modify the stack if it has less than 2 items. For stacks with one item, you could add an
else
to handle that case.– Johnny Mopp
Nov 12 at 14:21
@ChloePupaiboon This code will not modify the stack if it has less than 2 items. For stacks with one item, you could add an
else
to handle that case.– Johnny Mopp
Nov 12 at 14:21
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53263155%2fpostfix-calculator-java-cannot-resolve-or-is-not-a-field%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
K SgnarkJXLMcMOqZA obpQXnBlnYvxWREKv 5XF2BZG6p fov