Locker room question generalized to n people - repeating error
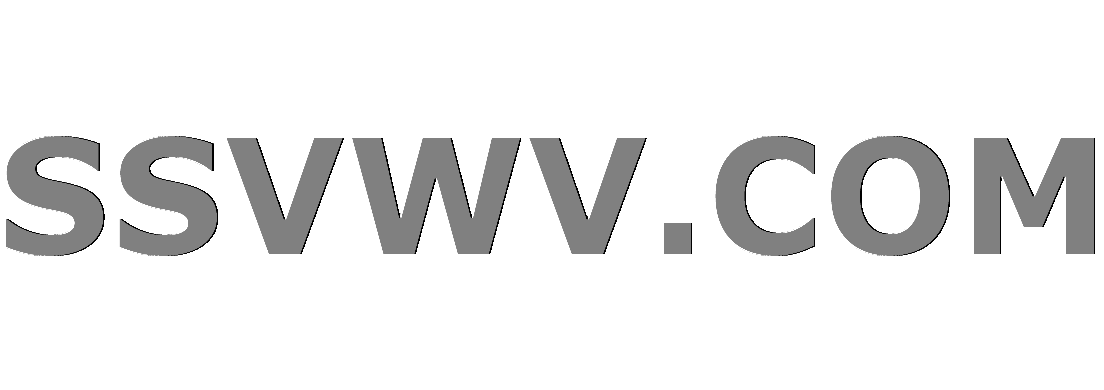
Multi tool use
up vote
-2
down vote
favorite
I have the following code:
import java.util.Scanner;
import java.util.Arrays;
public class Program11 {
public static void main(String args) {
Scanner input = new Scanner(System.in);
System.out.print("Number of lockers:");
int number = input.nextInt();
System.out.print("Show stages [y/n]?");
String show = input.next();
if(show.equals("y"))
{
for (char lockers : getStages(number))
{
for (char c : lockers)
{
System.out.print("" + c);
}
System.out.println();
}
}
}
public static char getStages(int n){
char lockers = new char[n];
char arrayLockers = new char[n];
for (int i = 0; i < n; i++) {
lockers[i] = 'O';
}
for (int i = 0; i<1;i++){
arrayLockers[i] = lockers;
}
for(int i = 2; i<=n; i++){
for(int z = 1; z<n; z++){
for (int w = i-1; w <= n; w += i){
lockers[w] = 'X';
}
arrayLockers[z] = lockers;
}
}
return arrayLockers;
}
}
The output is for n = 10:
> run Program11
Number of lockers: 10
Show stages [y/n]? y
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
>
The locker room problem goes as follows:
There are n students and n lockers. The first student opens all the lockers. The second student closes lockers 2,4,6,8,.... the third student closes lockers 3,6,9,12,... This patterns repeats itself until all n students have gone.
My task:
I am supposed to show the lockers at each stage for a given n. 'X' represents closed and 'O' represents open. I am supposed to use arrays only. Clearly this is repeating the same array over and over again which is incorrect. I used the method getStages to return a multi-dimensional array "arrayLockers" to store each stage.
Could anyone tell me where I have gone wrong?
Expected output is:
OOOOOOOOOO
OXOXOXOXOX
OXXXOXXXXX
OXXXOXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
java arrays multidimensional-array
|
show 4 more comments
up vote
-2
down vote
favorite
I have the following code:
import java.util.Scanner;
import java.util.Arrays;
public class Program11 {
public static void main(String args) {
Scanner input = new Scanner(System.in);
System.out.print("Number of lockers:");
int number = input.nextInt();
System.out.print("Show stages [y/n]?");
String show = input.next();
if(show.equals("y"))
{
for (char lockers : getStages(number))
{
for (char c : lockers)
{
System.out.print("" + c);
}
System.out.println();
}
}
}
public static char getStages(int n){
char lockers = new char[n];
char arrayLockers = new char[n];
for (int i = 0; i < n; i++) {
lockers[i] = 'O';
}
for (int i = 0; i<1;i++){
arrayLockers[i] = lockers;
}
for(int i = 2; i<=n; i++){
for(int z = 1; z<n; z++){
for (int w = i-1; w <= n; w += i){
lockers[w] = 'X';
}
arrayLockers[z] = lockers;
}
}
return arrayLockers;
}
}
The output is for n = 10:
> run Program11
Number of lockers: 10
Show stages [y/n]? y
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
>
The locker room problem goes as follows:
There are n students and n lockers. The first student opens all the lockers. The second student closes lockers 2,4,6,8,.... the third student closes lockers 3,6,9,12,... This patterns repeats itself until all n students have gone.
My task:
I am supposed to show the lockers at each stage for a given n. 'X' represents closed and 'O' represents open. I am supposed to use arrays only. Clearly this is repeating the same array over and over again which is incorrect. I used the method getStages to return a multi-dimensional array "arrayLockers" to store each stage.
Could anyone tell me where I have gone wrong?
Expected output is:
OOOOOOOOOO
OXOXOXOXOX
OXXXOXXXXX
OXXXOXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
java arrays multidimensional-array
arrayLockers[z] = lockers;
Are you assigning one array to another here? Instead, useSystem.arraycopy()
function.
– kiner_shah
Nov 11 at 7:00
Correct, this is where i make my multi-dimensional array.
– the boy 88
Nov 11 at 7:03
1
that is not the expected output but thank you for trying.
– the boy 88
Nov 11 at 7:36
1
Then what is the expected outcome? Is there a pattern/algorithm or should it be random which ones are opened?
– Joakim Danielson
Nov 11 at 7:39
1
Thanks @kiner_shah, that is correct. I will compare my code to see where my logic went wrong.
– the boy 88
Nov 11 at 7:44
|
show 4 more comments
up vote
-2
down vote
favorite
up vote
-2
down vote
favorite
I have the following code:
import java.util.Scanner;
import java.util.Arrays;
public class Program11 {
public static void main(String args) {
Scanner input = new Scanner(System.in);
System.out.print("Number of lockers:");
int number = input.nextInt();
System.out.print("Show stages [y/n]?");
String show = input.next();
if(show.equals("y"))
{
for (char lockers : getStages(number))
{
for (char c : lockers)
{
System.out.print("" + c);
}
System.out.println();
}
}
}
public static char getStages(int n){
char lockers = new char[n];
char arrayLockers = new char[n];
for (int i = 0; i < n; i++) {
lockers[i] = 'O';
}
for (int i = 0; i<1;i++){
arrayLockers[i] = lockers;
}
for(int i = 2; i<=n; i++){
for(int z = 1; z<n; z++){
for (int w = i-1; w <= n; w += i){
lockers[w] = 'X';
}
arrayLockers[z] = lockers;
}
}
return arrayLockers;
}
}
The output is for n = 10:
> run Program11
Number of lockers: 10
Show stages [y/n]? y
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
>
The locker room problem goes as follows:
There are n students and n lockers. The first student opens all the lockers. The second student closes lockers 2,4,6,8,.... the third student closes lockers 3,6,9,12,... This patterns repeats itself until all n students have gone.
My task:
I am supposed to show the lockers at each stage for a given n. 'X' represents closed and 'O' represents open. I am supposed to use arrays only. Clearly this is repeating the same array over and over again which is incorrect. I used the method getStages to return a multi-dimensional array "arrayLockers" to store each stage.
Could anyone tell me where I have gone wrong?
Expected output is:
OOOOOOOOOO
OXOXOXOXOX
OXXXOXXXXX
OXXXOXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
java arrays multidimensional-array
I have the following code:
import java.util.Scanner;
import java.util.Arrays;
public class Program11 {
public static void main(String args) {
Scanner input = new Scanner(System.in);
System.out.print("Number of lockers:");
int number = input.nextInt();
System.out.print("Show stages [y/n]?");
String show = input.next();
if(show.equals("y"))
{
for (char lockers : getStages(number))
{
for (char c : lockers)
{
System.out.print("" + c);
}
System.out.println();
}
}
}
public static char getStages(int n){
char lockers = new char[n];
char arrayLockers = new char[n];
for (int i = 0; i < n; i++) {
lockers[i] = 'O';
}
for (int i = 0; i<1;i++){
arrayLockers[i] = lockers;
}
for(int i = 2; i<=n; i++){
for(int z = 1; z<n; z++){
for (int w = i-1; w <= n; w += i){
lockers[w] = 'X';
}
arrayLockers[z] = lockers;
}
}
return arrayLockers;
}
}
The output is for n = 10:
> run Program11
Number of lockers: 10
Show stages [y/n]? y
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
>
The locker room problem goes as follows:
There are n students and n lockers. The first student opens all the lockers. The second student closes lockers 2,4,6,8,.... the third student closes lockers 3,6,9,12,... This patterns repeats itself until all n students have gone.
My task:
I am supposed to show the lockers at each stage for a given n. 'X' represents closed and 'O' represents open. I am supposed to use arrays only. Clearly this is repeating the same array over and over again which is incorrect. I used the method getStages to return a multi-dimensional array "arrayLockers" to store each stage.
Could anyone tell me where I have gone wrong?
Expected output is:
OOOOOOOOOO
OXOXOXOXOX
OXXXOXXXXX
OXXXOXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
OXXXXXXXXX
java arrays multidimensional-array
java arrays multidimensional-array
edited Nov 11 at 8:23
Deadpool
3,1112324
3,1112324
asked Nov 11 at 6:55
the boy 88
255
255
arrayLockers[z] = lockers;
Are you assigning one array to another here? Instead, useSystem.arraycopy()
function.
– kiner_shah
Nov 11 at 7:00
Correct, this is where i make my multi-dimensional array.
– the boy 88
Nov 11 at 7:03
1
that is not the expected output but thank you for trying.
– the boy 88
Nov 11 at 7:36
1
Then what is the expected outcome? Is there a pattern/algorithm or should it be random which ones are opened?
– Joakim Danielson
Nov 11 at 7:39
1
Thanks @kiner_shah, that is correct. I will compare my code to see where my logic went wrong.
– the boy 88
Nov 11 at 7:44
|
show 4 more comments
arrayLockers[z] = lockers;
Are you assigning one array to another here? Instead, useSystem.arraycopy()
function.
– kiner_shah
Nov 11 at 7:00
Correct, this is where i make my multi-dimensional array.
– the boy 88
Nov 11 at 7:03
1
that is not the expected output but thank you for trying.
– the boy 88
Nov 11 at 7:36
1
Then what is the expected outcome? Is there a pattern/algorithm or should it be random which ones are opened?
– Joakim Danielson
Nov 11 at 7:39
1
Thanks @kiner_shah, that is correct. I will compare my code to see where my logic went wrong.
– the boy 88
Nov 11 at 7:44
arrayLockers[z] = lockers;
Are you assigning one array to another here? Instead, use System.arraycopy()
function.– kiner_shah
Nov 11 at 7:00
arrayLockers[z] = lockers;
Are you assigning one array to another here? Instead, use System.arraycopy()
function.– kiner_shah
Nov 11 at 7:00
Correct, this is where i make my multi-dimensional array.
– the boy 88
Nov 11 at 7:03
Correct, this is where i make my multi-dimensional array.
– the boy 88
Nov 11 at 7:03
1
1
that is not the expected output but thank you for trying.
– the boy 88
Nov 11 at 7:36
that is not the expected output but thank you for trying.
– the boy 88
Nov 11 at 7:36
1
1
Then what is the expected outcome? Is there a pattern/algorithm or should it be random which ones are opened?
– Joakim Danielson
Nov 11 at 7:39
Then what is the expected outcome? Is there a pattern/algorithm or should it be random which ones are opened?
– Joakim Danielson
Nov 11 at 7:39
1
1
Thanks @kiner_shah, that is correct. I will compare my code to see where my logic went wrong.
– the boy 88
Nov 11 at 7:44
Thanks @kiner_shah, that is correct. I will compare my code to see where my logic went wrong.
– the boy 88
Nov 11 at 7:44
|
show 4 more comments
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
There were some problems with your code. Firstly, you were not allocating memory to arrayLockers
before copying. Secondly, you were complicating the logic by using three for
loops (nested). It could be done with just two nested loops. Also, you were using array assignment which I am not sure whether it works; so I changed it with System.arraycopy()
which I use often (or you can do it manually using a for
loop).
import java.util.*;
import java.lang.*;
import java.io.*;
class Program11 {
public static void main(String args) {
Scanner input = new Scanner(System.in);
System.out.print("Number of lockers:n");
int number = input.nextInt();
System.out.print("Show stages [y/n]?n");
String show = input.next();
if(show.equals("y"))
{
for (char lockers : getStages(number))
{
for (char c : lockers)
{
System.out.print("" + c);
}
System.out.println();
}
}
}
public static char getStages(int n){
char lockers = new char[n];
char arrayLockers = new char[n];
for (int i = 0; i < n; i++) {
lockers[i] = 'O';
}
arrayLockers[0] = new char[lockers.length];
System.arraycopy(lockers, 0, arrayLockers[0], 0, lockers.length);
int cnt = 2;
for (int i = 1; i < n; i++) {
for (int j = i; j < n; j += cnt) {
lockers[j] = 'X';
}
arrayLockers[i] = new char[lockers.length];
System.arraycopy(lockers, 0, arrayLockers[i], 0, lockers.length);
cnt++;
}
return arrayLockers;
}
}
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
There were some problems with your code. Firstly, you were not allocating memory to arrayLockers
before copying. Secondly, you were complicating the logic by using three for
loops (nested). It could be done with just two nested loops. Also, you were using array assignment which I am not sure whether it works; so I changed it with System.arraycopy()
which I use often (or you can do it manually using a for
loop).
import java.util.*;
import java.lang.*;
import java.io.*;
class Program11 {
public static void main(String args) {
Scanner input = new Scanner(System.in);
System.out.print("Number of lockers:n");
int number = input.nextInt();
System.out.print("Show stages [y/n]?n");
String show = input.next();
if(show.equals("y"))
{
for (char lockers : getStages(number))
{
for (char c : lockers)
{
System.out.print("" + c);
}
System.out.println();
}
}
}
public static char getStages(int n){
char lockers = new char[n];
char arrayLockers = new char[n];
for (int i = 0; i < n; i++) {
lockers[i] = 'O';
}
arrayLockers[0] = new char[lockers.length];
System.arraycopy(lockers, 0, arrayLockers[0], 0, lockers.length);
int cnt = 2;
for (int i = 1; i < n; i++) {
for (int j = i; j < n; j += cnt) {
lockers[j] = 'X';
}
arrayLockers[i] = new char[lockers.length];
System.arraycopy(lockers, 0, arrayLockers[i], 0, lockers.length);
cnt++;
}
return arrayLockers;
}
}
add a comment |
up vote
0
down vote
accepted
There were some problems with your code. Firstly, you were not allocating memory to arrayLockers
before copying. Secondly, you were complicating the logic by using three for
loops (nested). It could be done with just two nested loops. Also, you were using array assignment which I am not sure whether it works; so I changed it with System.arraycopy()
which I use often (or you can do it manually using a for
loop).
import java.util.*;
import java.lang.*;
import java.io.*;
class Program11 {
public static void main(String args) {
Scanner input = new Scanner(System.in);
System.out.print("Number of lockers:n");
int number = input.nextInt();
System.out.print("Show stages [y/n]?n");
String show = input.next();
if(show.equals("y"))
{
for (char lockers : getStages(number))
{
for (char c : lockers)
{
System.out.print("" + c);
}
System.out.println();
}
}
}
public static char getStages(int n){
char lockers = new char[n];
char arrayLockers = new char[n];
for (int i = 0; i < n; i++) {
lockers[i] = 'O';
}
arrayLockers[0] = new char[lockers.length];
System.arraycopy(lockers, 0, arrayLockers[0], 0, lockers.length);
int cnt = 2;
for (int i = 1; i < n; i++) {
for (int j = i; j < n; j += cnt) {
lockers[j] = 'X';
}
arrayLockers[i] = new char[lockers.length];
System.arraycopy(lockers, 0, arrayLockers[i], 0, lockers.length);
cnt++;
}
return arrayLockers;
}
}
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
There were some problems with your code. Firstly, you were not allocating memory to arrayLockers
before copying. Secondly, you were complicating the logic by using three for
loops (nested). It could be done with just two nested loops. Also, you were using array assignment which I am not sure whether it works; so I changed it with System.arraycopy()
which I use often (or you can do it manually using a for
loop).
import java.util.*;
import java.lang.*;
import java.io.*;
class Program11 {
public static void main(String args) {
Scanner input = new Scanner(System.in);
System.out.print("Number of lockers:n");
int number = input.nextInt();
System.out.print("Show stages [y/n]?n");
String show = input.next();
if(show.equals("y"))
{
for (char lockers : getStages(number))
{
for (char c : lockers)
{
System.out.print("" + c);
}
System.out.println();
}
}
}
public static char getStages(int n){
char lockers = new char[n];
char arrayLockers = new char[n];
for (int i = 0; i < n; i++) {
lockers[i] = 'O';
}
arrayLockers[0] = new char[lockers.length];
System.arraycopy(lockers, 0, arrayLockers[0], 0, lockers.length);
int cnt = 2;
for (int i = 1; i < n; i++) {
for (int j = i; j < n; j += cnt) {
lockers[j] = 'X';
}
arrayLockers[i] = new char[lockers.length];
System.arraycopy(lockers, 0, arrayLockers[i], 0, lockers.length);
cnt++;
}
return arrayLockers;
}
}
There were some problems with your code. Firstly, you were not allocating memory to arrayLockers
before copying. Secondly, you were complicating the logic by using three for
loops (nested). It could be done with just two nested loops. Also, you were using array assignment which I am not sure whether it works; so I changed it with System.arraycopy()
which I use often (or you can do it manually using a for
loop).
import java.util.*;
import java.lang.*;
import java.io.*;
class Program11 {
public static void main(String args) {
Scanner input = new Scanner(System.in);
System.out.print("Number of lockers:n");
int number = input.nextInt();
System.out.print("Show stages [y/n]?n");
String show = input.next();
if(show.equals("y"))
{
for (char lockers : getStages(number))
{
for (char c : lockers)
{
System.out.print("" + c);
}
System.out.println();
}
}
}
public static char getStages(int n){
char lockers = new char[n];
char arrayLockers = new char[n];
for (int i = 0; i < n; i++) {
lockers[i] = 'O';
}
arrayLockers[0] = new char[lockers.length];
System.arraycopy(lockers, 0, arrayLockers[0], 0, lockers.length);
int cnt = 2;
for (int i = 1; i < n; i++) {
for (int j = i; j < n; j += cnt) {
lockers[j] = 'X';
}
arrayLockers[i] = new char[lockers.length];
System.arraycopy(lockers, 0, arrayLockers[i], 0, lockers.length);
cnt++;
}
return arrayLockers;
}
}
answered Nov 11 at 7:47
kiner_shah
1,13321323
1,13321323
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53246507%2flocker-room-question-generalized-to-n-people-repeating-error%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
deFNZ ZYtBmP3y1z,4,bn,pJ5AuV48sc6kkWnt86unRJf7ov 3UVz38FcjPpjGBQ Fc3
arrayLockers[z] = lockers;
Are you assigning one array to another here? Instead, useSystem.arraycopy()
function.– kiner_shah
Nov 11 at 7:00
Correct, this is where i make my multi-dimensional array.
– the boy 88
Nov 11 at 7:03
1
that is not the expected output but thank you for trying.
– the boy 88
Nov 11 at 7:36
1
Then what is the expected outcome? Is there a pattern/algorithm or should it be random which ones are opened?
– Joakim Danielson
Nov 11 at 7:39
1
Thanks @kiner_shah, that is correct. I will compare my code to see where my logic went wrong.
– the boy 88
Nov 11 at 7:44