How to create a new pages using classes in Tkinter?
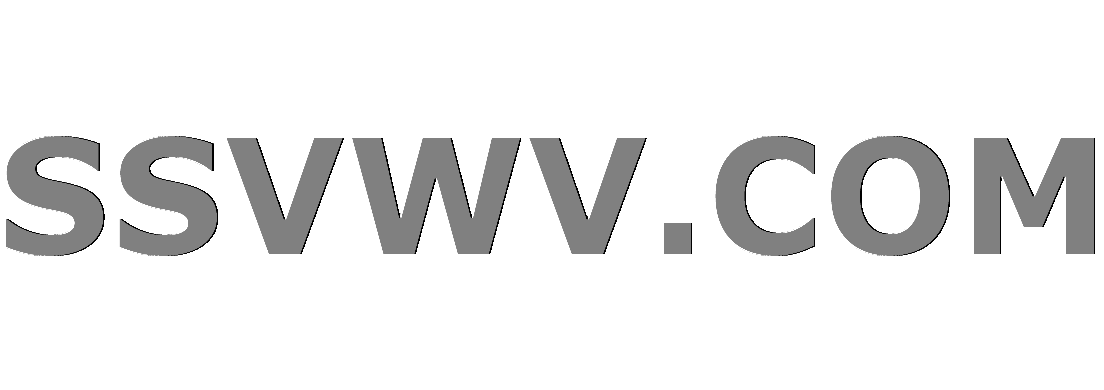
Multi tool use
up vote
1
down vote
favorite
I have attempted to create a tkinter GUI which can overlap each page Page 1, Page 2, Page 3
. I am struggling in two places:
Using the class rather than the method so I have commented them out, but what I have tried at is putting the class in brackets within the command i.e. command=(mainPage)
but this gives the error:
builtins.NameError: name 'mainPage' is not defined
Second place I am struggling is not being able to overlap each class.
My code is as follows:
from tkinter import *
class testOverlap(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.root = root
self.topButtons()
self.pageOne()
self.pageTwo()
self.pageThree()
def topButtons(self):
self.firstPage = Button(self.root, text="Go to Page 1", background="WHITE", height = 2, width = 16, command= self.pageOne())
self.firstPage.place(x=0, y=0)
self.secondPage = Button(self.root, text="Go to Page 2", background="WHITE",height = 2, width = 16, command= self.pageTwo())
self.secondPage.place(x=121, y=0)
self.thirdPage = Button(self.root, text="Go to Page 3", background="WHITE",height = 2, width = 17, command= self.pageThree())
self.thirdPage.place(x=242, y=0)
#class mainPage(Frame):
def pageOne(self):
self.Label1 = Label(self.root, text=" First Page ", height = 20, width = 52, background="Green")
self.Label1.place(x=0, y=40)
#class middlePage(Frame):
def pageTwo(self):
self.Label2 = Label(self.root, text=" Second Page ", height = 20, width = 52, background="Blue")
self.Label2.place(x=0, y=40)
#class finalPage(Frame):
def pageThree(self):
self.Label3 = Label(self.root, text=" Third Page ", height = 20, width = 52, background="Red")
self.Label3.place(x=0, y=40)
def main():
root = Tk()
root.title("Tk")
root.geometry('370x340')
app = testOverlap(root)
root.mainloop()
if __name__ == '__main__':
main()
python python-3.x class user-interface tkinter
add a comment |
up vote
1
down vote
favorite
I have attempted to create a tkinter GUI which can overlap each page Page 1, Page 2, Page 3
. I am struggling in two places:
Using the class rather than the method so I have commented them out, but what I have tried at is putting the class in brackets within the command i.e. command=(mainPage)
but this gives the error:
builtins.NameError: name 'mainPage' is not defined
Second place I am struggling is not being able to overlap each class.
My code is as follows:
from tkinter import *
class testOverlap(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.root = root
self.topButtons()
self.pageOne()
self.pageTwo()
self.pageThree()
def topButtons(self):
self.firstPage = Button(self.root, text="Go to Page 1", background="WHITE", height = 2, width = 16, command= self.pageOne())
self.firstPage.place(x=0, y=0)
self.secondPage = Button(self.root, text="Go to Page 2", background="WHITE",height = 2, width = 16, command= self.pageTwo())
self.secondPage.place(x=121, y=0)
self.thirdPage = Button(self.root, text="Go to Page 3", background="WHITE",height = 2, width = 17, command= self.pageThree())
self.thirdPage.place(x=242, y=0)
#class mainPage(Frame):
def pageOne(self):
self.Label1 = Label(self.root, text=" First Page ", height = 20, width = 52, background="Green")
self.Label1.place(x=0, y=40)
#class middlePage(Frame):
def pageTwo(self):
self.Label2 = Label(self.root, text=" Second Page ", height = 20, width = 52, background="Blue")
self.Label2.place(x=0, y=40)
#class finalPage(Frame):
def pageThree(self):
self.Label3 = Label(self.root, text=" Third Page ", height = 20, width = 52, background="Red")
self.Label3.place(x=0, y=40)
def main():
root = Tk()
root.title("Tk")
root.geometry('370x340')
app = testOverlap(root)
root.mainloop()
if __name__ == '__main__':
main()
python python-3.x class user-interface tkinter
"Using the class rather than the method so I have commented them out" - this makes no sense. If they are commented out, you are not using classes. Is the problem you're solving using classes or not? If not, I recommend removing the commented code because it's very confusing as written.
– Bryan Oakley
Nov 7 at 21:35
I commented them out because if i left it in it would be classed as a non-functional code but i will try re-iterate this in the future.
– pythonewbie
Nov 7 at 21:52
The problem is, removing the class completely changes the nature of the code under the class statements.
– Bryan Oakley
Nov 7 at 22:27
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have attempted to create a tkinter GUI which can overlap each page Page 1, Page 2, Page 3
. I am struggling in two places:
Using the class rather than the method so I have commented them out, but what I have tried at is putting the class in brackets within the command i.e. command=(mainPage)
but this gives the error:
builtins.NameError: name 'mainPage' is not defined
Second place I am struggling is not being able to overlap each class.
My code is as follows:
from tkinter import *
class testOverlap(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.root = root
self.topButtons()
self.pageOne()
self.pageTwo()
self.pageThree()
def topButtons(self):
self.firstPage = Button(self.root, text="Go to Page 1", background="WHITE", height = 2, width = 16, command= self.pageOne())
self.firstPage.place(x=0, y=0)
self.secondPage = Button(self.root, text="Go to Page 2", background="WHITE",height = 2, width = 16, command= self.pageTwo())
self.secondPage.place(x=121, y=0)
self.thirdPage = Button(self.root, text="Go to Page 3", background="WHITE",height = 2, width = 17, command= self.pageThree())
self.thirdPage.place(x=242, y=0)
#class mainPage(Frame):
def pageOne(self):
self.Label1 = Label(self.root, text=" First Page ", height = 20, width = 52, background="Green")
self.Label1.place(x=0, y=40)
#class middlePage(Frame):
def pageTwo(self):
self.Label2 = Label(self.root, text=" Second Page ", height = 20, width = 52, background="Blue")
self.Label2.place(x=0, y=40)
#class finalPage(Frame):
def pageThree(self):
self.Label3 = Label(self.root, text=" Third Page ", height = 20, width = 52, background="Red")
self.Label3.place(x=0, y=40)
def main():
root = Tk()
root.title("Tk")
root.geometry('370x340')
app = testOverlap(root)
root.mainloop()
if __name__ == '__main__':
main()
python python-3.x class user-interface tkinter
I have attempted to create a tkinter GUI which can overlap each page Page 1, Page 2, Page 3
. I am struggling in two places:
Using the class rather than the method so I have commented them out, but what I have tried at is putting the class in brackets within the command i.e. command=(mainPage)
but this gives the error:
builtins.NameError: name 'mainPage' is not defined
Second place I am struggling is not being able to overlap each class.
My code is as follows:
from tkinter import *
class testOverlap(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.root = root
self.topButtons()
self.pageOne()
self.pageTwo()
self.pageThree()
def topButtons(self):
self.firstPage = Button(self.root, text="Go to Page 1", background="WHITE", height = 2, width = 16, command= self.pageOne())
self.firstPage.place(x=0, y=0)
self.secondPage = Button(self.root, text="Go to Page 2", background="WHITE",height = 2, width = 16, command= self.pageTwo())
self.secondPage.place(x=121, y=0)
self.thirdPage = Button(self.root, text="Go to Page 3", background="WHITE",height = 2, width = 17, command= self.pageThree())
self.thirdPage.place(x=242, y=0)
#class mainPage(Frame):
def pageOne(self):
self.Label1 = Label(self.root, text=" First Page ", height = 20, width = 52, background="Green")
self.Label1.place(x=0, y=40)
#class middlePage(Frame):
def pageTwo(self):
self.Label2 = Label(self.root, text=" Second Page ", height = 20, width = 52, background="Blue")
self.Label2.place(x=0, y=40)
#class finalPage(Frame):
def pageThree(self):
self.Label3 = Label(self.root, text=" Third Page ", height = 20, width = 52, background="Red")
self.Label3.place(x=0, y=40)
def main():
root = Tk()
root.title("Tk")
root.geometry('370x340')
app = testOverlap(root)
root.mainloop()
if __name__ == '__main__':
main()
python python-3.x class user-interface tkinter
python python-3.x class user-interface tkinter
edited Nov 7 at 21:21


martineau
64.8k887175
64.8k887175
asked Nov 7 at 21:16
pythonewbie
165
165
"Using the class rather than the method so I have commented them out" - this makes no sense. If they are commented out, you are not using classes. Is the problem you're solving using classes or not? If not, I recommend removing the commented code because it's very confusing as written.
– Bryan Oakley
Nov 7 at 21:35
I commented them out because if i left it in it would be classed as a non-functional code but i will try re-iterate this in the future.
– pythonewbie
Nov 7 at 21:52
The problem is, removing the class completely changes the nature of the code under the class statements.
– Bryan Oakley
Nov 7 at 22:27
add a comment |
"Using the class rather than the method so I have commented them out" - this makes no sense. If they are commented out, you are not using classes. Is the problem you're solving using classes or not? If not, I recommend removing the commented code because it's very confusing as written.
– Bryan Oakley
Nov 7 at 21:35
I commented them out because if i left it in it would be classed as a non-functional code but i will try re-iterate this in the future.
– pythonewbie
Nov 7 at 21:52
The problem is, removing the class completely changes the nature of the code under the class statements.
– Bryan Oakley
Nov 7 at 22:27
"Using the class rather than the method so I have commented them out" - this makes no sense. If they are commented out, you are not using classes. Is the problem you're solving using classes or not? If not, I recommend removing the commented code because it's very confusing as written.
– Bryan Oakley
Nov 7 at 21:35
"Using the class rather than the method so I have commented them out" - this makes no sense. If they are commented out, you are not using classes. Is the problem you're solving using classes or not? If not, I recommend removing the commented code because it's very confusing as written.
– Bryan Oakley
Nov 7 at 21:35
I commented them out because if i left it in it would be classed as a non-functional code but i will try re-iterate this in the future.
– pythonewbie
Nov 7 at 21:52
I commented them out because if i left it in it would be classed as a non-functional code but i will try re-iterate this in the future.
– pythonewbie
Nov 7 at 21:52
The problem is, removing the class completely changes the nature of the code under the class statements.
– Bryan Oakley
Nov 7 at 22:27
The problem is, removing the class completely changes the nature of the code under the class statements.
– Bryan Oakley
Nov 7 at 22:27
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
Creating a Frame (page) and raising it (bringing it to the top to view it) are 2 completely different operations. To create it you need to use a layout like grid
on the class instance, and to raise it you need to use the tkraise
method of the instance. We usually do this by dedicating a class to do nothing but handle all the pages. Try this:
from tkinter import *
class Controller(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.pageOne = mainPage(self)
self.pageOne.grid(row=0, column=0, sticky='nsew')
self.pageTwo = middlePage(self)
self.pageTwo.grid(row=0, column=0, sticky='nsew')
self.pageThree = finalPage(self)
self.pageThree.grid(row=0, column=0, sticky='nsew')
self.menu = testOverlap(self)
self.menu.grid(row=0, column=0, sticky='nsew')
self.menu.tkraise() # show the testOverlap Frame now
class testOverlap(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.topButtons()
def topButtons(self):
self.firstPage = Button(self, text="Go to Page 1", background="WHITE", height = 2, width = 16, command= self.master.pageOne.tkraise)
self.firstPage.grid(row=0, column=0)
self.secondPage = Button(self, text="Go to Page 2", background="WHITE",height = 2, width = 16, command= self.master.pageTwo.tkraise)
self.secondPage.grid(row=0, column=1)
self.thirdPage = Button(self, text="Go to Page 3", background="WHITE",height = 2, width = 17, command= self.master.pageThree.tkraise)
self.thirdPage.grid(row=0, column=2)
class mainPage(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.Label1 = Label(self, text=" First Page ", height = 20, width = 52, background="Green")
self.Label1.grid()
class middlePage(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.Label2 = Label(self, text=" Second Page ", height = 20, width = 52, background="Blue")
self.Label2.grid()
class finalPage(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.Label3 = Label(self, text=" Third Page ", height = 20, width = 52, background="Red")
self.Label3.grid()
def main():
root = Tk()
root.title("Tk")
root.geometry('370x340')
app = Controller(root)
app.pack(expand=True, fill=BOTH)
root.mainloop()
if __name__ == '__main__':
main()
Note also I removed your use of place
. I recommend you stay away from place if you can, use grid
or pack
instead. Using place requires specifying the exact size in a lot of other places, and grid or pack can handle that for you.
when would you suggest i could use place, could i read on these bugs if you have a link? I am new to python, but this was great help i shall study this code now and compare it to my question.
– pythonewbie
Nov 7 at 21:54
I don't think it's fair to sayplace
causes weird bugs.place
is entirely deterministic and isn't buggy. The only problems it causes are that it requires you to do a lot more work if you want your UI to be responsive and easy to maintain.
– Bryan Oakley
Nov 7 at 22:02
place
should only be used when a floating widget is needed that partially covers up other widgets. It requires that the user defines ALL dimensions, so forgetting one makes the whole thing not work (which is what happens if you replace grid with place in the code above) or it makes things appear off screen with new fonts / versions of python / computers. For now forget that place exists and let tkinter do the hard work.
– Novel
Nov 7 at 22:03
No, that's not necessarily true that place is only for floating widgets.place
is actually quite good when you want to stack widgets on top of each other. it has other uses as well.place
is actually quite powerful and flexible. I don't recommend it for most work, and I especially don't recommend it for beginners, but it's definitely good for more things than just floating widgets.
– Bryan Oakley
Nov 7 at 22:04
@BryanOakley I edited out the buggy comment, I agree that implied something wrong with tkinter not the user. But I disagree with your other comment; I have yet to find any other use for place that can't be solved more easily and neater with grid. (Mind floating widgets is a very important role; I'm not advocating for never using place.)
– Novel
Nov 7 at 22:16
|
show 4 more comments
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
Creating a Frame (page) and raising it (bringing it to the top to view it) are 2 completely different operations. To create it you need to use a layout like grid
on the class instance, and to raise it you need to use the tkraise
method of the instance. We usually do this by dedicating a class to do nothing but handle all the pages. Try this:
from tkinter import *
class Controller(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.pageOne = mainPage(self)
self.pageOne.grid(row=0, column=0, sticky='nsew')
self.pageTwo = middlePage(self)
self.pageTwo.grid(row=0, column=0, sticky='nsew')
self.pageThree = finalPage(self)
self.pageThree.grid(row=0, column=0, sticky='nsew')
self.menu = testOverlap(self)
self.menu.grid(row=0, column=0, sticky='nsew')
self.menu.tkraise() # show the testOverlap Frame now
class testOverlap(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.topButtons()
def topButtons(self):
self.firstPage = Button(self, text="Go to Page 1", background="WHITE", height = 2, width = 16, command= self.master.pageOne.tkraise)
self.firstPage.grid(row=0, column=0)
self.secondPage = Button(self, text="Go to Page 2", background="WHITE",height = 2, width = 16, command= self.master.pageTwo.tkraise)
self.secondPage.grid(row=0, column=1)
self.thirdPage = Button(self, text="Go to Page 3", background="WHITE",height = 2, width = 17, command= self.master.pageThree.tkraise)
self.thirdPage.grid(row=0, column=2)
class mainPage(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.Label1 = Label(self, text=" First Page ", height = 20, width = 52, background="Green")
self.Label1.grid()
class middlePage(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.Label2 = Label(self, text=" Second Page ", height = 20, width = 52, background="Blue")
self.Label2.grid()
class finalPage(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.Label3 = Label(self, text=" Third Page ", height = 20, width = 52, background="Red")
self.Label3.grid()
def main():
root = Tk()
root.title("Tk")
root.geometry('370x340')
app = Controller(root)
app.pack(expand=True, fill=BOTH)
root.mainloop()
if __name__ == '__main__':
main()
Note also I removed your use of place
. I recommend you stay away from place if you can, use grid
or pack
instead. Using place requires specifying the exact size in a lot of other places, and grid or pack can handle that for you.
when would you suggest i could use place, could i read on these bugs if you have a link? I am new to python, but this was great help i shall study this code now and compare it to my question.
– pythonewbie
Nov 7 at 21:54
I don't think it's fair to sayplace
causes weird bugs.place
is entirely deterministic and isn't buggy. The only problems it causes are that it requires you to do a lot more work if you want your UI to be responsive and easy to maintain.
– Bryan Oakley
Nov 7 at 22:02
place
should only be used when a floating widget is needed that partially covers up other widgets. It requires that the user defines ALL dimensions, so forgetting one makes the whole thing not work (which is what happens if you replace grid with place in the code above) or it makes things appear off screen with new fonts / versions of python / computers. For now forget that place exists and let tkinter do the hard work.
– Novel
Nov 7 at 22:03
No, that's not necessarily true that place is only for floating widgets.place
is actually quite good when you want to stack widgets on top of each other. it has other uses as well.place
is actually quite powerful and flexible. I don't recommend it for most work, and I especially don't recommend it for beginners, but it's definitely good for more things than just floating widgets.
– Bryan Oakley
Nov 7 at 22:04
@BryanOakley I edited out the buggy comment, I agree that implied something wrong with tkinter not the user. But I disagree with your other comment; I have yet to find any other use for place that can't be solved more easily and neater with grid. (Mind floating widgets is a very important role; I'm not advocating for never using place.)
– Novel
Nov 7 at 22:16
|
show 4 more comments
up vote
2
down vote
accepted
Creating a Frame (page) and raising it (bringing it to the top to view it) are 2 completely different operations. To create it you need to use a layout like grid
on the class instance, and to raise it you need to use the tkraise
method of the instance. We usually do this by dedicating a class to do nothing but handle all the pages. Try this:
from tkinter import *
class Controller(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.pageOne = mainPage(self)
self.pageOne.grid(row=0, column=0, sticky='nsew')
self.pageTwo = middlePage(self)
self.pageTwo.grid(row=0, column=0, sticky='nsew')
self.pageThree = finalPage(self)
self.pageThree.grid(row=0, column=0, sticky='nsew')
self.menu = testOverlap(self)
self.menu.grid(row=0, column=0, sticky='nsew')
self.menu.tkraise() # show the testOverlap Frame now
class testOverlap(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.topButtons()
def topButtons(self):
self.firstPage = Button(self, text="Go to Page 1", background="WHITE", height = 2, width = 16, command= self.master.pageOne.tkraise)
self.firstPage.grid(row=0, column=0)
self.secondPage = Button(self, text="Go to Page 2", background="WHITE",height = 2, width = 16, command= self.master.pageTwo.tkraise)
self.secondPage.grid(row=0, column=1)
self.thirdPage = Button(self, text="Go to Page 3", background="WHITE",height = 2, width = 17, command= self.master.pageThree.tkraise)
self.thirdPage.grid(row=0, column=2)
class mainPage(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.Label1 = Label(self, text=" First Page ", height = 20, width = 52, background="Green")
self.Label1.grid()
class middlePage(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.Label2 = Label(self, text=" Second Page ", height = 20, width = 52, background="Blue")
self.Label2.grid()
class finalPage(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.Label3 = Label(self, text=" Third Page ", height = 20, width = 52, background="Red")
self.Label3.grid()
def main():
root = Tk()
root.title("Tk")
root.geometry('370x340')
app = Controller(root)
app.pack(expand=True, fill=BOTH)
root.mainloop()
if __name__ == '__main__':
main()
Note also I removed your use of place
. I recommend you stay away from place if you can, use grid
or pack
instead. Using place requires specifying the exact size in a lot of other places, and grid or pack can handle that for you.
when would you suggest i could use place, could i read on these bugs if you have a link? I am new to python, but this was great help i shall study this code now and compare it to my question.
– pythonewbie
Nov 7 at 21:54
I don't think it's fair to sayplace
causes weird bugs.place
is entirely deterministic and isn't buggy. The only problems it causes are that it requires you to do a lot more work if you want your UI to be responsive and easy to maintain.
– Bryan Oakley
Nov 7 at 22:02
place
should only be used when a floating widget is needed that partially covers up other widgets. It requires that the user defines ALL dimensions, so forgetting one makes the whole thing not work (which is what happens if you replace grid with place in the code above) or it makes things appear off screen with new fonts / versions of python / computers. For now forget that place exists and let tkinter do the hard work.
– Novel
Nov 7 at 22:03
No, that's not necessarily true that place is only for floating widgets.place
is actually quite good when you want to stack widgets on top of each other. it has other uses as well.place
is actually quite powerful and flexible. I don't recommend it for most work, and I especially don't recommend it for beginners, but it's definitely good for more things than just floating widgets.
– Bryan Oakley
Nov 7 at 22:04
@BryanOakley I edited out the buggy comment, I agree that implied something wrong with tkinter not the user. But I disagree with your other comment; I have yet to find any other use for place that can't be solved more easily and neater with grid. (Mind floating widgets is a very important role; I'm not advocating for never using place.)
– Novel
Nov 7 at 22:16
|
show 4 more comments
up vote
2
down vote
accepted
up vote
2
down vote
accepted
Creating a Frame (page) and raising it (bringing it to the top to view it) are 2 completely different operations. To create it you need to use a layout like grid
on the class instance, and to raise it you need to use the tkraise
method of the instance. We usually do this by dedicating a class to do nothing but handle all the pages. Try this:
from tkinter import *
class Controller(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.pageOne = mainPage(self)
self.pageOne.grid(row=0, column=0, sticky='nsew')
self.pageTwo = middlePage(self)
self.pageTwo.grid(row=0, column=0, sticky='nsew')
self.pageThree = finalPage(self)
self.pageThree.grid(row=0, column=0, sticky='nsew')
self.menu = testOverlap(self)
self.menu.grid(row=0, column=0, sticky='nsew')
self.menu.tkraise() # show the testOverlap Frame now
class testOverlap(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.topButtons()
def topButtons(self):
self.firstPage = Button(self, text="Go to Page 1", background="WHITE", height = 2, width = 16, command= self.master.pageOne.tkraise)
self.firstPage.grid(row=0, column=0)
self.secondPage = Button(self, text="Go to Page 2", background="WHITE",height = 2, width = 16, command= self.master.pageTwo.tkraise)
self.secondPage.grid(row=0, column=1)
self.thirdPage = Button(self, text="Go to Page 3", background="WHITE",height = 2, width = 17, command= self.master.pageThree.tkraise)
self.thirdPage.grid(row=0, column=2)
class mainPage(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.Label1 = Label(self, text=" First Page ", height = 20, width = 52, background="Green")
self.Label1.grid()
class middlePage(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.Label2 = Label(self, text=" Second Page ", height = 20, width = 52, background="Blue")
self.Label2.grid()
class finalPage(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.Label3 = Label(self, text=" Third Page ", height = 20, width = 52, background="Red")
self.Label3.grid()
def main():
root = Tk()
root.title("Tk")
root.geometry('370x340')
app = Controller(root)
app.pack(expand=True, fill=BOTH)
root.mainloop()
if __name__ == '__main__':
main()
Note also I removed your use of place
. I recommend you stay away from place if you can, use grid
or pack
instead. Using place requires specifying the exact size in a lot of other places, and grid or pack can handle that for you.
Creating a Frame (page) and raising it (bringing it to the top to view it) are 2 completely different operations. To create it you need to use a layout like grid
on the class instance, and to raise it you need to use the tkraise
method of the instance. We usually do this by dedicating a class to do nothing but handle all the pages. Try this:
from tkinter import *
class Controller(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.pageOne = mainPage(self)
self.pageOne.grid(row=0, column=0, sticky='nsew')
self.pageTwo = middlePage(self)
self.pageTwo.grid(row=0, column=0, sticky='nsew')
self.pageThree = finalPage(self)
self.pageThree.grid(row=0, column=0, sticky='nsew')
self.menu = testOverlap(self)
self.menu.grid(row=0, column=0, sticky='nsew')
self.menu.tkraise() # show the testOverlap Frame now
class testOverlap(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.topButtons()
def topButtons(self):
self.firstPage = Button(self, text="Go to Page 1", background="WHITE", height = 2, width = 16, command= self.master.pageOne.tkraise)
self.firstPage.grid(row=0, column=0)
self.secondPage = Button(self, text="Go to Page 2", background="WHITE",height = 2, width = 16, command= self.master.pageTwo.tkraise)
self.secondPage.grid(row=0, column=1)
self.thirdPage = Button(self, text="Go to Page 3", background="WHITE",height = 2, width = 17, command= self.master.pageThree.tkraise)
self.thirdPage.grid(row=0, column=2)
class mainPage(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.Label1 = Label(self, text=" First Page ", height = 20, width = 52, background="Green")
self.Label1.grid()
class middlePage(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.Label2 = Label(self, text=" Second Page ", height = 20, width = 52, background="Blue")
self.Label2.grid()
class finalPage(Frame):
def __init__(self, root):
Frame.__init__(self, root)
self.Label3 = Label(self, text=" Third Page ", height = 20, width = 52, background="Red")
self.Label3.grid()
def main():
root = Tk()
root.title("Tk")
root.geometry('370x340')
app = Controller(root)
app.pack(expand=True, fill=BOTH)
root.mainloop()
if __name__ == '__main__':
main()
Note also I removed your use of place
. I recommend you stay away from place if you can, use grid
or pack
instead. Using place requires specifying the exact size in a lot of other places, and grid or pack can handle that for you.
edited Nov 11 at 6:45
answered Nov 7 at 21:44
Novel
7,1801922
7,1801922
when would you suggest i could use place, could i read on these bugs if you have a link? I am new to python, but this was great help i shall study this code now and compare it to my question.
– pythonewbie
Nov 7 at 21:54
I don't think it's fair to sayplace
causes weird bugs.place
is entirely deterministic and isn't buggy. The only problems it causes are that it requires you to do a lot more work if you want your UI to be responsive and easy to maintain.
– Bryan Oakley
Nov 7 at 22:02
place
should only be used when a floating widget is needed that partially covers up other widgets. It requires that the user defines ALL dimensions, so forgetting one makes the whole thing not work (which is what happens if you replace grid with place in the code above) or it makes things appear off screen with new fonts / versions of python / computers. For now forget that place exists and let tkinter do the hard work.
– Novel
Nov 7 at 22:03
No, that's not necessarily true that place is only for floating widgets.place
is actually quite good when you want to stack widgets on top of each other. it has other uses as well.place
is actually quite powerful and flexible. I don't recommend it for most work, and I especially don't recommend it for beginners, but it's definitely good for more things than just floating widgets.
– Bryan Oakley
Nov 7 at 22:04
@BryanOakley I edited out the buggy comment, I agree that implied something wrong with tkinter not the user. But I disagree with your other comment; I have yet to find any other use for place that can't be solved more easily and neater with grid. (Mind floating widgets is a very important role; I'm not advocating for never using place.)
– Novel
Nov 7 at 22:16
|
show 4 more comments
when would you suggest i could use place, could i read on these bugs if you have a link? I am new to python, but this was great help i shall study this code now and compare it to my question.
– pythonewbie
Nov 7 at 21:54
I don't think it's fair to sayplace
causes weird bugs.place
is entirely deterministic and isn't buggy. The only problems it causes are that it requires you to do a lot more work if you want your UI to be responsive and easy to maintain.
– Bryan Oakley
Nov 7 at 22:02
place
should only be used when a floating widget is needed that partially covers up other widgets. It requires that the user defines ALL dimensions, so forgetting one makes the whole thing not work (which is what happens if you replace grid with place in the code above) or it makes things appear off screen with new fonts / versions of python / computers. For now forget that place exists and let tkinter do the hard work.
– Novel
Nov 7 at 22:03
No, that's not necessarily true that place is only for floating widgets.place
is actually quite good when you want to stack widgets on top of each other. it has other uses as well.place
is actually quite powerful and flexible. I don't recommend it for most work, and I especially don't recommend it for beginners, but it's definitely good for more things than just floating widgets.
– Bryan Oakley
Nov 7 at 22:04
@BryanOakley I edited out the buggy comment, I agree that implied something wrong with tkinter not the user. But I disagree with your other comment; I have yet to find any other use for place that can't be solved more easily and neater with grid. (Mind floating widgets is a very important role; I'm not advocating for never using place.)
– Novel
Nov 7 at 22:16
when would you suggest i could use place, could i read on these bugs if you have a link? I am new to python, but this was great help i shall study this code now and compare it to my question.
– pythonewbie
Nov 7 at 21:54
when would you suggest i could use place, could i read on these bugs if you have a link? I am new to python, but this was great help i shall study this code now and compare it to my question.
– pythonewbie
Nov 7 at 21:54
I don't think it's fair to say
place
causes weird bugs. place
is entirely deterministic and isn't buggy. The only problems it causes are that it requires you to do a lot more work if you want your UI to be responsive and easy to maintain.– Bryan Oakley
Nov 7 at 22:02
I don't think it's fair to say
place
causes weird bugs. place
is entirely deterministic and isn't buggy. The only problems it causes are that it requires you to do a lot more work if you want your UI to be responsive and easy to maintain.– Bryan Oakley
Nov 7 at 22:02
place
should only be used when a floating widget is needed that partially covers up other widgets. It requires that the user defines ALL dimensions, so forgetting one makes the whole thing not work (which is what happens if you replace grid with place in the code above) or it makes things appear off screen with new fonts / versions of python / computers. For now forget that place exists and let tkinter do the hard work.– Novel
Nov 7 at 22:03
place
should only be used when a floating widget is needed that partially covers up other widgets. It requires that the user defines ALL dimensions, so forgetting one makes the whole thing not work (which is what happens if you replace grid with place in the code above) or it makes things appear off screen with new fonts / versions of python / computers. For now forget that place exists and let tkinter do the hard work.– Novel
Nov 7 at 22:03
No, that's not necessarily true that place is only for floating widgets.
place
is actually quite good when you want to stack widgets on top of each other. it has other uses as well. place
is actually quite powerful and flexible. I don't recommend it for most work, and I especially don't recommend it for beginners, but it's definitely good for more things than just floating widgets.– Bryan Oakley
Nov 7 at 22:04
No, that's not necessarily true that place is only for floating widgets.
place
is actually quite good when you want to stack widgets on top of each other. it has other uses as well. place
is actually quite powerful and flexible. I don't recommend it for most work, and I especially don't recommend it for beginners, but it's definitely good for more things than just floating widgets.– Bryan Oakley
Nov 7 at 22:04
@BryanOakley I edited out the buggy comment, I agree that implied something wrong with tkinter not the user. But I disagree with your other comment; I have yet to find any other use for place that can't be solved more easily and neater with grid. (Mind floating widgets is a very important role; I'm not advocating for never using place.)
– Novel
Nov 7 at 22:16
@BryanOakley I edited out the buggy comment, I agree that implied something wrong with tkinter not the user. But I disagree with your other comment; I have yet to find any other use for place that can't be solved more easily and neater with grid. (Mind floating widgets is a very important role; I'm not advocating for never using place.)
– Novel
Nov 7 at 22:16
|
show 4 more comments
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53197938%2fhow-to-create-a-new-pages-using-classes-in-tkinter%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zJRHQc
"Using the class rather than the method so I have commented them out" - this makes no sense. If they are commented out, you are not using classes. Is the problem you're solving using classes or not? If not, I recommend removing the commented code because it's very confusing as written.
– Bryan Oakley
Nov 7 at 21:35
I commented them out because if i left it in it would be classed as a non-functional code but i will try re-iterate this in the future.
– pythonewbie
Nov 7 at 21:52
The problem is, removing the class completely changes the nature of the code under the class statements.
– Bryan Oakley
Nov 7 at 22:27