How to test that a JavaScript object has only specific fields/properties
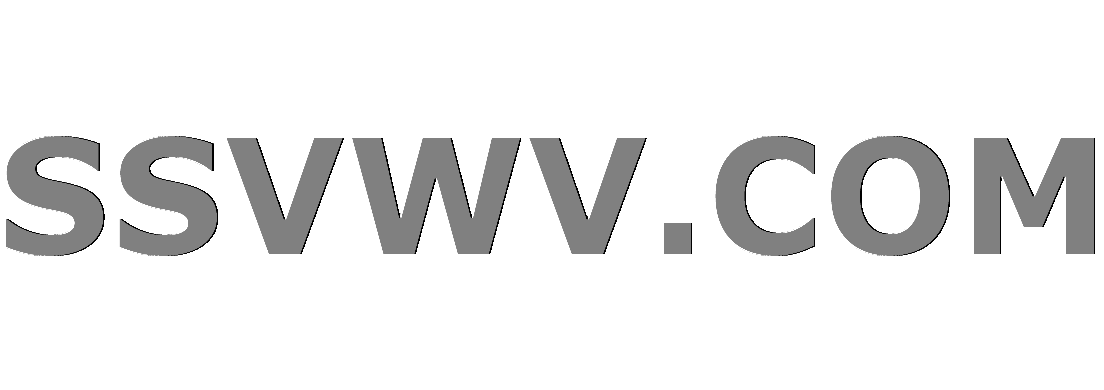
Multi tool use
up vote
0
down vote
favorite
I have this function which tests that an object has certain fields
validateServerResponseStructure(res: any) {
let isTypeCorrect: boolean = (
res.result != undefined) && (res['additional-info'] != undefined
);
return isTypeCorrect;
}
Is there a way to test that res
has only result
and additional-info
properties and nothing else?
javascript typescript
add a comment |
up vote
0
down vote
favorite
I have this function which tests that an object has certain fields
validateServerResponseStructure(res: any) {
let isTypeCorrect: boolean = (
res.result != undefined) && (res['additional-info'] != undefined
);
return isTypeCorrect;
}
Is there a way to test that res
has only result
and additional-info
properties and nothing else?
javascript typescript
You should have usedin
operator instead: eg:'result' in res
– zerkms
Nov 11 at 7:09
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have this function which tests that an object has certain fields
validateServerResponseStructure(res: any) {
let isTypeCorrect: boolean = (
res.result != undefined) && (res['additional-info'] != undefined
);
return isTypeCorrect;
}
Is there a way to test that res
has only result
and additional-info
properties and nothing else?
javascript typescript
I have this function which tests that an object has certain fields
validateServerResponseStructure(res: any) {
let isTypeCorrect: boolean = (
res.result != undefined) && (res['additional-info'] != undefined
);
return isTypeCorrect;
}
Is there a way to test that res
has only result
and additional-info
properties and nothing else?
javascript typescript
javascript typescript
edited Nov 11 at 7:17
Roy Scheffers
2,11281625
2,11281625
asked Nov 11 at 6:56
Manu Chadha
2,61611235
2,61611235
You should have usedin
operator instead: eg:'result' in res
– zerkms
Nov 11 at 7:09
add a comment |
You should have usedin
operator instead: eg:'result' in res
– zerkms
Nov 11 at 7:09
You should have used
in
operator instead: eg: 'result' in res
– zerkms
Nov 11 at 7:09
You should have used
in
operator instead: eg: 'result' in res
– zerkms
Nov 11 at 7:09
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
Just check that the length of its keys
is exactly two. Also, probably best to use strict equality comparison, if you want to ensure that the properties are not undefined
:
validateServerResponseStructure(res: any) {
let isTypeCorrect: boolean = (
res.result !== undefined
&& res['additional-info'] !== undefined
&& Object.keys(res).length === 2
);
return isTypeCorrect;
}
In the unusual case that you may have properties that contain the undefined
value, and you want to permit such values, check the length of the keys, and that both result
and additional-info
are included in the keys:
const keys = Object.keys(res);
let isTypeCorrect: boolean = (
['result', 'additional-info'].every(key => keys.includes(key))
&& keys.length === 2
)
You say " best to use strict equality", but you don't use it. You should usetypeof res.result !== "undefined" && typeof res['additional-info'] !== "undefined"
– Tsahi Asher
Nov 11 at 7:08
res.result !== undefined
does not check if a property exists, but only if its value is notundefined
.{ foo: undefined }
--- here the.foo
property would exist yet it'sundefined
.
– zerkms
Nov 11 at 7:08
@TsahiAsher "Strict equality" refers to using===
and!==
rather than==
and!=
. Pretty sure addingtypeof
only adds a bit of syntax noise
– CertainPerformance
Nov 11 at 7:17
@zerkms - I don't understand your comment.
– Manu Chadha
Nov 11 at 16:52
@ManuChadha you asked "How to test that a JavaScript object has only specific fields/properties " --- you don't check if an object has a particular property with!== undefined
. Equality toundefined
has nothing to do with property presence. To check if property presents you need to usein
orObject.keys
as in this answer
– zerkms
Nov 11 at 22:37
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
Just check that the length of its keys
is exactly two. Also, probably best to use strict equality comparison, if you want to ensure that the properties are not undefined
:
validateServerResponseStructure(res: any) {
let isTypeCorrect: boolean = (
res.result !== undefined
&& res['additional-info'] !== undefined
&& Object.keys(res).length === 2
);
return isTypeCorrect;
}
In the unusual case that you may have properties that contain the undefined
value, and you want to permit such values, check the length of the keys, and that both result
and additional-info
are included in the keys:
const keys = Object.keys(res);
let isTypeCorrect: boolean = (
['result', 'additional-info'].every(key => keys.includes(key))
&& keys.length === 2
)
You say " best to use strict equality", but you don't use it. You should usetypeof res.result !== "undefined" && typeof res['additional-info'] !== "undefined"
– Tsahi Asher
Nov 11 at 7:08
res.result !== undefined
does not check if a property exists, but only if its value is notundefined
.{ foo: undefined }
--- here the.foo
property would exist yet it'sundefined
.
– zerkms
Nov 11 at 7:08
@TsahiAsher "Strict equality" refers to using===
and!==
rather than==
and!=
. Pretty sure addingtypeof
only adds a bit of syntax noise
– CertainPerformance
Nov 11 at 7:17
@zerkms - I don't understand your comment.
– Manu Chadha
Nov 11 at 16:52
@ManuChadha you asked "How to test that a JavaScript object has only specific fields/properties " --- you don't check if an object has a particular property with!== undefined
. Equality toundefined
has nothing to do with property presence. To check if property presents you need to usein
orObject.keys
as in this answer
– zerkms
Nov 11 at 22:37
add a comment |
up vote
2
down vote
accepted
Just check that the length of its keys
is exactly two. Also, probably best to use strict equality comparison, if you want to ensure that the properties are not undefined
:
validateServerResponseStructure(res: any) {
let isTypeCorrect: boolean = (
res.result !== undefined
&& res['additional-info'] !== undefined
&& Object.keys(res).length === 2
);
return isTypeCorrect;
}
In the unusual case that you may have properties that contain the undefined
value, and you want to permit such values, check the length of the keys, and that both result
and additional-info
are included in the keys:
const keys = Object.keys(res);
let isTypeCorrect: boolean = (
['result', 'additional-info'].every(key => keys.includes(key))
&& keys.length === 2
)
You say " best to use strict equality", but you don't use it. You should usetypeof res.result !== "undefined" && typeof res['additional-info'] !== "undefined"
– Tsahi Asher
Nov 11 at 7:08
res.result !== undefined
does not check if a property exists, but only if its value is notundefined
.{ foo: undefined }
--- here the.foo
property would exist yet it'sundefined
.
– zerkms
Nov 11 at 7:08
@TsahiAsher "Strict equality" refers to using===
and!==
rather than==
and!=
. Pretty sure addingtypeof
only adds a bit of syntax noise
– CertainPerformance
Nov 11 at 7:17
@zerkms - I don't understand your comment.
– Manu Chadha
Nov 11 at 16:52
@ManuChadha you asked "How to test that a JavaScript object has only specific fields/properties " --- you don't check if an object has a particular property with!== undefined
. Equality toundefined
has nothing to do with property presence. To check if property presents you need to usein
orObject.keys
as in this answer
– zerkms
Nov 11 at 22:37
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
Just check that the length of its keys
is exactly two. Also, probably best to use strict equality comparison, if you want to ensure that the properties are not undefined
:
validateServerResponseStructure(res: any) {
let isTypeCorrect: boolean = (
res.result !== undefined
&& res['additional-info'] !== undefined
&& Object.keys(res).length === 2
);
return isTypeCorrect;
}
In the unusual case that you may have properties that contain the undefined
value, and you want to permit such values, check the length of the keys, and that both result
and additional-info
are included in the keys:
const keys = Object.keys(res);
let isTypeCorrect: boolean = (
['result', 'additional-info'].every(key => keys.includes(key))
&& keys.length === 2
)
Just check that the length of its keys
is exactly two. Also, probably best to use strict equality comparison, if you want to ensure that the properties are not undefined
:
validateServerResponseStructure(res: any) {
let isTypeCorrect: boolean = (
res.result !== undefined
&& res['additional-info'] !== undefined
&& Object.keys(res).length === 2
);
return isTypeCorrect;
}
In the unusual case that you may have properties that contain the undefined
value, and you want to permit such values, check the length of the keys, and that both result
and additional-info
are included in the keys:
const keys = Object.keys(res);
let isTypeCorrect: boolean = (
['result', 'additional-info'].every(key => keys.includes(key))
&& keys.length === 2
)
edited Nov 11 at 7:13
answered Nov 11 at 6:58
CertainPerformance
67.3k143353
67.3k143353
You say " best to use strict equality", but you don't use it. You should usetypeof res.result !== "undefined" && typeof res['additional-info'] !== "undefined"
– Tsahi Asher
Nov 11 at 7:08
res.result !== undefined
does not check if a property exists, but only if its value is notundefined
.{ foo: undefined }
--- here the.foo
property would exist yet it'sundefined
.
– zerkms
Nov 11 at 7:08
@TsahiAsher "Strict equality" refers to using===
and!==
rather than==
and!=
. Pretty sure addingtypeof
only adds a bit of syntax noise
– CertainPerformance
Nov 11 at 7:17
@zerkms - I don't understand your comment.
– Manu Chadha
Nov 11 at 16:52
@ManuChadha you asked "How to test that a JavaScript object has only specific fields/properties " --- you don't check if an object has a particular property with!== undefined
. Equality toundefined
has nothing to do with property presence. To check if property presents you need to usein
orObject.keys
as in this answer
– zerkms
Nov 11 at 22:37
add a comment |
You say " best to use strict equality", but you don't use it. You should usetypeof res.result !== "undefined" && typeof res['additional-info'] !== "undefined"
– Tsahi Asher
Nov 11 at 7:08
res.result !== undefined
does not check if a property exists, but only if its value is notundefined
.{ foo: undefined }
--- here the.foo
property would exist yet it'sundefined
.
– zerkms
Nov 11 at 7:08
@TsahiAsher "Strict equality" refers to using===
and!==
rather than==
and!=
. Pretty sure addingtypeof
only adds a bit of syntax noise
– CertainPerformance
Nov 11 at 7:17
@zerkms - I don't understand your comment.
– Manu Chadha
Nov 11 at 16:52
@ManuChadha you asked "How to test that a JavaScript object has only specific fields/properties " --- you don't check if an object has a particular property with!== undefined
. Equality toundefined
has nothing to do with property presence. To check if property presents you need to usein
orObject.keys
as in this answer
– zerkms
Nov 11 at 22:37
You say " best to use strict equality", but you don't use it. You should use
typeof res.result !== "undefined" && typeof res['additional-info'] !== "undefined"
– Tsahi Asher
Nov 11 at 7:08
You say " best to use strict equality", but you don't use it. You should use
typeof res.result !== "undefined" && typeof res['additional-info'] !== "undefined"
– Tsahi Asher
Nov 11 at 7:08
res.result !== undefined
does not check if a property exists, but only if its value is not undefined
. { foo: undefined }
--- here the .foo
property would exist yet it's undefined
.– zerkms
Nov 11 at 7:08
res.result !== undefined
does not check if a property exists, but only if its value is not undefined
. { foo: undefined }
--- here the .foo
property would exist yet it's undefined
.– zerkms
Nov 11 at 7:08
@TsahiAsher "Strict equality" refers to using
===
and !==
rather than ==
and !=
. Pretty sure adding typeof
only adds a bit of syntax noise– CertainPerformance
Nov 11 at 7:17
@TsahiAsher "Strict equality" refers to using
===
and !==
rather than ==
and !=
. Pretty sure adding typeof
only adds a bit of syntax noise– CertainPerformance
Nov 11 at 7:17
@zerkms - I don't understand your comment.
– Manu Chadha
Nov 11 at 16:52
@zerkms - I don't understand your comment.
– Manu Chadha
Nov 11 at 16:52
@ManuChadha you asked "How to test that a JavaScript object has only specific fields/properties " --- you don't check if an object has a particular property with
!== undefined
. Equality to undefined
has nothing to do with property presence. To check if property presents you need to use in
or Object.keys
as in this answer– zerkms
Nov 11 at 22:37
@ManuChadha you asked "How to test that a JavaScript object has only specific fields/properties " --- you don't check if an object has a particular property with
!== undefined
. Equality to undefined
has nothing to do with property presence. To check if property presents you need to use in
or Object.keys
as in this answer– zerkms
Nov 11 at 22:37
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53246514%2fhow-to-test-that-a-javascript-object-has-only-specific-fields-properties%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Jn aVlY2G6GdJ,4rwnT0QhJB2,hCCnhOLWPEyBksk vL9niIvaqF6wFl
You should have used
in
operator instead: eg:'result' in res
– zerkms
Nov 11 at 7:09