Spring JPA using SQLite : Filed *repository* required a bean named 'entityManagerFactory' that could not be...
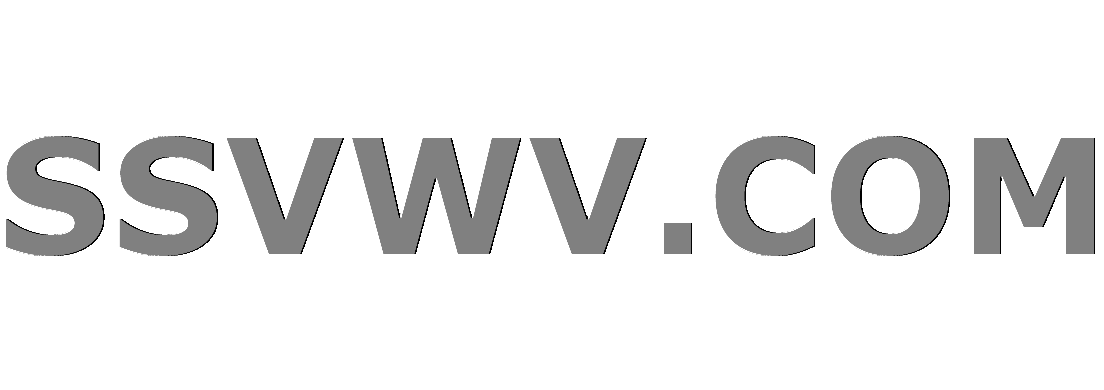
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I am relatively new in using Spring JPA, and I've spent a couple of hours trying to solve this problem. I am aware this question has been asked many times, and I have tried many of their solutions to no avail (most likely due to my own experience, I found myself lost many times in trying out their solutions). I have deleted my .m2 repository folder as some suggested, I made sure to place the SQLite dialect dependency in my pom.xml, and I have even tried making a configuration class where I would create this bean manually (but as mentioned before I got so lost in what was going on that I decided to delete it after it failed to run again). What I am trying to do is connect my SQLite database to my Spring JPA project (it seems that not many people use SQLite for their Spring JPA projects as I have failed to find a clear tutorial/documentation in doing so). Here are the relevant files:
Application Main
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@EnableJpaRepositories
public class myApplication {
public static void main(String args) {
SpringApplication.run(myApplication.class, args);
}
}
My Repository
import org.springframework.data.repository.JpaRepository;
import java.util.List;
public interface UserRepository extends JpaRepository<User, Integer> {
List<User> getUsers();
}
My Entity
@Entity
@Table(name = "User")
public class User {
@Id
@GeneratedValue(strategy=GenerationType.AUTO)
private int user_id;
@Column(name="user_name")
private String user_name;
@Column(name="user_password")
private String user_password;
protected User() {}
public User(String user_name, String user_password) {
this.user_name = user_name;
this.user_password = user_password;
}
public int getUser_id() {
return user_id;
}
public void setUser_id(int user_id) {
this.user_id = user_id;
}
public String getUser_name() {
return user_name;
}
public void setUser_name(String user_name) {
this.user_name = user_name;
}
public String getUser_password() {
return user_password;
}
public void setUser_password(String user_password) {
this.user_password = user_password;
}
@Override
public String toString() {
return String.format("User[id=%d, user_name='%s']", user_id, user_name);
}
}
My Service
@Service
public class myService {
@Autowired
UserRepository userRepository;
public List<User> getUsers() {
return userRepository.getUsers();
}
}
Application Properties
spring.datasource.url= jdbc:sqlite:memory:database/myDB
spring.datasource.driver-class-name=org.sqlite.JDBC
spring.datasource.username = ""
spring.datasource.password = ""
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.SQLiteDialect
spring.jpa.generate-ddl=true
POM
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/org.xerial/sqlite-jdbc -->
<dependency>
<groupId>org.xerial</groupId>
<artifactId>sqlite-jdbc</artifactId>
<version>3.23.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.hibernate.dialect/sqlite-dialect -->
<dependency>
<groupId>org.hibernate.dialect</groupId>
<artifactId>sqlite-dialect</artifactId>
<version>0.1.0</version>
</dependency>
As stated in the title, the problem appears to be that a bean named entityManagerFactory
can not be found:
Field userRepository in com.project.myService required a bean named 'entityManagerFactory' that could not be found.
I thought Spring can automatically detect the beans needed for your project and automatically produces them for you? Many tutorials that use MySQL and Spring's embedded database seem to never encounter this issue. Is this mainly an issue in using SQLite with Spring JPA? I'd really appreciate anyone's guidance in configuring SQLite to work with Spring JPA, thank you!
spring sqlite spring-boot spring-data-jpa spring-jdbc
add a comment |
I am relatively new in using Spring JPA, and I've spent a couple of hours trying to solve this problem. I am aware this question has been asked many times, and I have tried many of their solutions to no avail (most likely due to my own experience, I found myself lost many times in trying out their solutions). I have deleted my .m2 repository folder as some suggested, I made sure to place the SQLite dialect dependency in my pom.xml, and I have even tried making a configuration class where I would create this bean manually (but as mentioned before I got so lost in what was going on that I decided to delete it after it failed to run again). What I am trying to do is connect my SQLite database to my Spring JPA project (it seems that not many people use SQLite for their Spring JPA projects as I have failed to find a clear tutorial/documentation in doing so). Here are the relevant files:
Application Main
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@EnableJpaRepositories
public class myApplication {
public static void main(String args) {
SpringApplication.run(myApplication.class, args);
}
}
My Repository
import org.springframework.data.repository.JpaRepository;
import java.util.List;
public interface UserRepository extends JpaRepository<User, Integer> {
List<User> getUsers();
}
My Entity
@Entity
@Table(name = "User")
public class User {
@Id
@GeneratedValue(strategy=GenerationType.AUTO)
private int user_id;
@Column(name="user_name")
private String user_name;
@Column(name="user_password")
private String user_password;
protected User() {}
public User(String user_name, String user_password) {
this.user_name = user_name;
this.user_password = user_password;
}
public int getUser_id() {
return user_id;
}
public void setUser_id(int user_id) {
this.user_id = user_id;
}
public String getUser_name() {
return user_name;
}
public void setUser_name(String user_name) {
this.user_name = user_name;
}
public String getUser_password() {
return user_password;
}
public void setUser_password(String user_password) {
this.user_password = user_password;
}
@Override
public String toString() {
return String.format("User[id=%d, user_name='%s']", user_id, user_name);
}
}
My Service
@Service
public class myService {
@Autowired
UserRepository userRepository;
public List<User> getUsers() {
return userRepository.getUsers();
}
}
Application Properties
spring.datasource.url= jdbc:sqlite:memory:database/myDB
spring.datasource.driver-class-name=org.sqlite.JDBC
spring.datasource.username = ""
spring.datasource.password = ""
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.SQLiteDialect
spring.jpa.generate-ddl=true
POM
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/org.xerial/sqlite-jdbc -->
<dependency>
<groupId>org.xerial</groupId>
<artifactId>sqlite-jdbc</artifactId>
<version>3.23.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.hibernate.dialect/sqlite-dialect -->
<dependency>
<groupId>org.hibernate.dialect</groupId>
<artifactId>sqlite-dialect</artifactId>
<version>0.1.0</version>
</dependency>
As stated in the title, the problem appears to be that a bean named entityManagerFactory
can not be found:
Field userRepository in com.project.myService required a bean named 'entityManagerFactory' that could not be found.
I thought Spring can automatically detect the beans needed for your project and automatically produces them for you? Many tutorials that use MySQL and Spring's embedded database seem to never encounter this issue. Is this mainly an issue in using SQLite with Spring JPA? I'd really appreciate anyone's guidance in configuring SQLite to work with Spring JPA, thank you!
spring sqlite spring-boot spring-data-jpa spring-jdbc
add a comment |
I am relatively new in using Spring JPA, and I've spent a couple of hours trying to solve this problem. I am aware this question has been asked many times, and I have tried many of their solutions to no avail (most likely due to my own experience, I found myself lost many times in trying out their solutions). I have deleted my .m2 repository folder as some suggested, I made sure to place the SQLite dialect dependency in my pom.xml, and I have even tried making a configuration class where I would create this bean manually (but as mentioned before I got so lost in what was going on that I decided to delete it after it failed to run again). What I am trying to do is connect my SQLite database to my Spring JPA project (it seems that not many people use SQLite for their Spring JPA projects as I have failed to find a clear tutorial/documentation in doing so). Here are the relevant files:
Application Main
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@EnableJpaRepositories
public class myApplication {
public static void main(String args) {
SpringApplication.run(myApplication.class, args);
}
}
My Repository
import org.springframework.data.repository.JpaRepository;
import java.util.List;
public interface UserRepository extends JpaRepository<User, Integer> {
List<User> getUsers();
}
My Entity
@Entity
@Table(name = "User")
public class User {
@Id
@GeneratedValue(strategy=GenerationType.AUTO)
private int user_id;
@Column(name="user_name")
private String user_name;
@Column(name="user_password")
private String user_password;
protected User() {}
public User(String user_name, String user_password) {
this.user_name = user_name;
this.user_password = user_password;
}
public int getUser_id() {
return user_id;
}
public void setUser_id(int user_id) {
this.user_id = user_id;
}
public String getUser_name() {
return user_name;
}
public void setUser_name(String user_name) {
this.user_name = user_name;
}
public String getUser_password() {
return user_password;
}
public void setUser_password(String user_password) {
this.user_password = user_password;
}
@Override
public String toString() {
return String.format("User[id=%d, user_name='%s']", user_id, user_name);
}
}
My Service
@Service
public class myService {
@Autowired
UserRepository userRepository;
public List<User> getUsers() {
return userRepository.getUsers();
}
}
Application Properties
spring.datasource.url= jdbc:sqlite:memory:database/myDB
spring.datasource.driver-class-name=org.sqlite.JDBC
spring.datasource.username = ""
spring.datasource.password = ""
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.SQLiteDialect
spring.jpa.generate-ddl=true
POM
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/org.xerial/sqlite-jdbc -->
<dependency>
<groupId>org.xerial</groupId>
<artifactId>sqlite-jdbc</artifactId>
<version>3.23.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.hibernate.dialect/sqlite-dialect -->
<dependency>
<groupId>org.hibernate.dialect</groupId>
<artifactId>sqlite-dialect</artifactId>
<version>0.1.0</version>
</dependency>
As stated in the title, the problem appears to be that a bean named entityManagerFactory
can not be found:
Field userRepository in com.project.myService required a bean named 'entityManagerFactory' that could not be found.
I thought Spring can automatically detect the beans needed for your project and automatically produces them for you? Many tutorials that use MySQL and Spring's embedded database seem to never encounter this issue. Is this mainly an issue in using SQLite with Spring JPA? I'd really appreciate anyone's guidance in configuring SQLite to work with Spring JPA, thank you!
spring sqlite spring-boot spring-data-jpa spring-jdbc
I am relatively new in using Spring JPA, and I've spent a couple of hours trying to solve this problem. I am aware this question has been asked many times, and I have tried many of their solutions to no avail (most likely due to my own experience, I found myself lost many times in trying out their solutions). I have deleted my .m2 repository folder as some suggested, I made sure to place the SQLite dialect dependency in my pom.xml, and I have even tried making a configuration class where I would create this bean manually (but as mentioned before I got so lost in what was going on that I decided to delete it after it failed to run again). What I am trying to do is connect my SQLite database to my Spring JPA project (it seems that not many people use SQLite for their Spring JPA projects as I have failed to find a clear tutorial/documentation in doing so). Here are the relevant files:
Application Main
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@EnableJpaRepositories
public class myApplication {
public static void main(String args) {
SpringApplication.run(myApplication.class, args);
}
}
My Repository
import org.springframework.data.repository.JpaRepository;
import java.util.List;
public interface UserRepository extends JpaRepository<User, Integer> {
List<User> getUsers();
}
My Entity
@Entity
@Table(name = "User")
public class User {
@Id
@GeneratedValue(strategy=GenerationType.AUTO)
private int user_id;
@Column(name="user_name")
private String user_name;
@Column(name="user_password")
private String user_password;
protected User() {}
public User(String user_name, String user_password) {
this.user_name = user_name;
this.user_password = user_password;
}
public int getUser_id() {
return user_id;
}
public void setUser_id(int user_id) {
this.user_id = user_id;
}
public String getUser_name() {
return user_name;
}
public void setUser_name(String user_name) {
this.user_name = user_name;
}
public String getUser_password() {
return user_password;
}
public void setUser_password(String user_password) {
this.user_password = user_password;
}
@Override
public String toString() {
return String.format("User[id=%d, user_name='%s']", user_id, user_name);
}
}
My Service
@Service
public class myService {
@Autowired
UserRepository userRepository;
public List<User> getUsers() {
return userRepository.getUsers();
}
}
Application Properties
spring.datasource.url= jdbc:sqlite:memory:database/myDB
spring.datasource.driver-class-name=org.sqlite.JDBC
spring.datasource.username = ""
spring.datasource.password = ""
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.SQLiteDialect
spring.jpa.generate-ddl=true
POM
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/org.xerial/sqlite-jdbc -->
<dependency>
<groupId>org.xerial</groupId>
<artifactId>sqlite-jdbc</artifactId>
<version>3.23.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.hibernate.dialect/sqlite-dialect -->
<dependency>
<groupId>org.hibernate.dialect</groupId>
<artifactId>sqlite-dialect</artifactId>
<version>0.1.0</version>
</dependency>
As stated in the title, the problem appears to be that a bean named entityManagerFactory
can not be found:
Field userRepository in com.project.myService required a bean named 'entityManagerFactory' that could not be found.
I thought Spring can automatically detect the beans needed for your project and automatically produces them for you? Many tutorials that use MySQL and Spring's embedded database seem to never encounter this issue. Is this mainly an issue in using SQLite with Spring JPA? I'd really appreciate anyone's guidance in configuring SQLite to work with Spring JPA, thank you!
spring sqlite spring-boot spring-data-jpa spring-jdbc
spring sqlite spring-boot spring-data-jpa spring-jdbc
asked Nov 16 '18 at 17:33
Michael ValentineMichael Valentine
53
53
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53342774%2fspring-jpa-using-sqlite-filed-repository-required-a-bean-named-entitymanage%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53342774%2fspring-jpa-using-sqlite-filed-repository-required-a-bean-named-entitymanage%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
FktgVHXcAiyl,y TdEuDF,81AyhWHvl9c4jlcfbvkJjS0VehSKzu,Rkx,Gt1,I 17KvcOi7gGdW,6Vd 8