Why is my recursive function returning this pointer in a weird format?
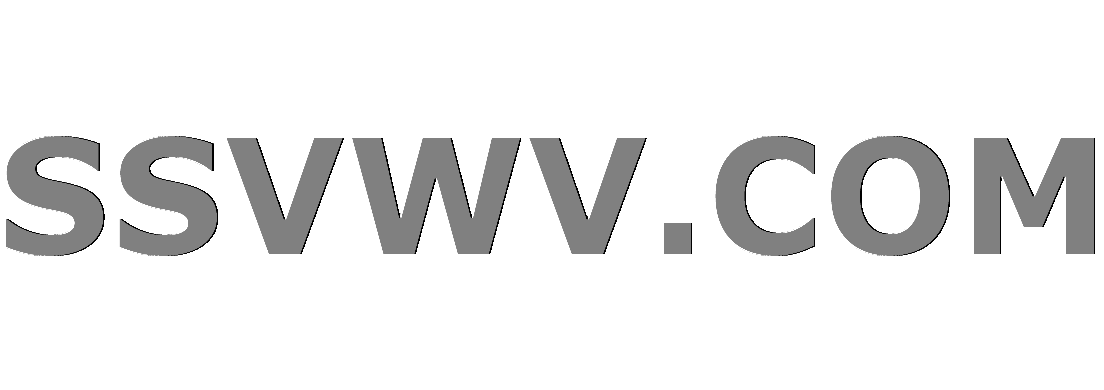
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
So I've made this function to recover the closest number from what I've searched in an AVL Tree (like, if the numbers are 1 4 5 6 and if I search 5, it'll return 4).
I store this number in the variable 'closer' and check if the node I'm comparing is the last node in the tree. If I print closer
in the auxiliary function it prints the exact number I wanted, but when I return it to the main function it's non-sense.
void searchCloser(AVL_TREE* tree, void* search) {
if (AVL_Retrieve(tree,search)!= NULL)
{
NODE* node=tree->root;
while (node->left)
node=node->left;
void* closer=node->dataPtr; //First Element of Tree
node=tree->root;
while (node->right)
node=node->right;
void* end=node; //End of Tree
closer=_searchCloser(tree->root,search,closer,end);
printf("--%d",*(int*)closer);
} else
printf("Nao Encontrado");
}
void _searchCloser(NODE* root, void* search, void* closer, void*end) {
if (root->left)
_searchCloser(root->left,search,closer,end);
if(compare(search,root->dataPtr)==1 && compare(search,closer)==1) //Compare returns 1 for A bigger then B
closer=root->dataPtr;
if (root->right)
_searchCloser(root->right,search,closer,end);
if (end==root)
return(closer);
}
c avl-tree
|
show 5 more comments
So I've made this function to recover the closest number from what I've searched in an AVL Tree (like, if the numbers are 1 4 5 6 and if I search 5, it'll return 4).
I store this number in the variable 'closer' and check if the node I'm comparing is the last node in the tree. If I print closer
in the auxiliary function it prints the exact number I wanted, but when I return it to the main function it's non-sense.
void searchCloser(AVL_TREE* tree, void* search) {
if (AVL_Retrieve(tree,search)!= NULL)
{
NODE* node=tree->root;
while (node->left)
node=node->left;
void* closer=node->dataPtr; //First Element of Tree
node=tree->root;
while (node->right)
node=node->right;
void* end=node; //End of Tree
closer=_searchCloser(tree->root,search,closer,end);
printf("--%d",*(int*)closer);
} else
printf("Nao Encontrado");
}
void _searchCloser(NODE* root, void* search, void* closer, void*end) {
if (root->left)
_searchCloser(root->left,search,closer,end);
if(compare(search,root->dataPtr)==1 && compare(search,closer)==1) //Compare returns 1 for A bigger then B
closer=root->dataPtr;
if (root->right)
_searchCloser(root->right,search,closer,end);
if (end==root)
return(closer);
}
c avl-tree
you're returning the value that represents the address thatcloser
holds, not the value it points to. usereturn *closer;
instead
– Tau
Nov 16 '18 at 17:44
4
The return type of_searchCloser
is declared to bevoid
. How can itreturn
anything?
– Fred Larson
Nov 16 '18 at 17:45
... so how does the above code even compile?
– John Bollinger
Nov 16 '18 at 17:47
2
Save time. Enable compiler warnings.
– chux
Nov 16 '18 at 18:04
1
"when i print it in the main function" --> that code is not posted. To best answer this, please provide a Minimal, Complete, and Verifiable example. And change_searchCloser()
from returningvoid
to something. With "use void* the compile warn against conflicting types and does not compile." then post the corresponding code and exact warning.
– chux
Nov 16 '18 at 18:33
|
show 5 more comments
So I've made this function to recover the closest number from what I've searched in an AVL Tree (like, if the numbers are 1 4 5 6 and if I search 5, it'll return 4).
I store this number in the variable 'closer' and check if the node I'm comparing is the last node in the tree. If I print closer
in the auxiliary function it prints the exact number I wanted, but when I return it to the main function it's non-sense.
void searchCloser(AVL_TREE* tree, void* search) {
if (AVL_Retrieve(tree,search)!= NULL)
{
NODE* node=tree->root;
while (node->left)
node=node->left;
void* closer=node->dataPtr; //First Element of Tree
node=tree->root;
while (node->right)
node=node->right;
void* end=node; //End of Tree
closer=_searchCloser(tree->root,search,closer,end);
printf("--%d",*(int*)closer);
} else
printf("Nao Encontrado");
}
void _searchCloser(NODE* root, void* search, void* closer, void*end) {
if (root->left)
_searchCloser(root->left,search,closer,end);
if(compare(search,root->dataPtr)==1 && compare(search,closer)==1) //Compare returns 1 for A bigger then B
closer=root->dataPtr;
if (root->right)
_searchCloser(root->right,search,closer,end);
if (end==root)
return(closer);
}
c avl-tree
So I've made this function to recover the closest number from what I've searched in an AVL Tree (like, if the numbers are 1 4 5 6 and if I search 5, it'll return 4).
I store this number in the variable 'closer' and check if the node I'm comparing is the last node in the tree. If I print closer
in the auxiliary function it prints the exact number I wanted, but when I return it to the main function it's non-sense.
void searchCloser(AVL_TREE* tree, void* search) {
if (AVL_Retrieve(tree,search)!= NULL)
{
NODE* node=tree->root;
while (node->left)
node=node->left;
void* closer=node->dataPtr; //First Element of Tree
node=tree->root;
while (node->right)
node=node->right;
void* end=node; //End of Tree
closer=_searchCloser(tree->root,search,closer,end);
printf("--%d",*(int*)closer);
} else
printf("Nao Encontrado");
}
void _searchCloser(NODE* root, void* search, void* closer, void*end) {
if (root->left)
_searchCloser(root->left,search,closer,end);
if(compare(search,root->dataPtr)==1 && compare(search,closer)==1) //Compare returns 1 for A bigger then B
closer=root->dataPtr;
if (root->right)
_searchCloser(root->right,search,closer,end);
if (end==root)
return(closer);
}
c avl-tree
c avl-tree
edited Nov 16 '18 at 17:45


Jonny Henly
3,51042040
3,51042040
asked Nov 16 '18 at 17:40
Matheus DiasMatheus Dias
122
122
you're returning the value that represents the address thatcloser
holds, not the value it points to. usereturn *closer;
instead
– Tau
Nov 16 '18 at 17:44
4
The return type of_searchCloser
is declared to bevoid
. How can itreturn
anything?
– Fred Larson
Nov 16 '18 at 17:45
... so how does the above code even compile?
– John Bollinger
Nov 16 '18 at 17:47
2
Save time. Enable compiler warnings.
– chux
Nov 16 '18 at 18:04
1
"when i print it in the main function" --> that code is not posted. To best answer this, please provide a Minimal, Complete, and Verifiable example. And change_searchCloser()
from returningvoid
to something. With "use void* the compile warn against conflicting types and does not compile." then post the corresponding code and exact warning.
– chux
Nov 16 '18 at 18:33
|
show 5 more comments
you're returning the value that represents the address thatcloser
holds, not the value it points to. usereturn *closer;
instead
– Tau
Nov 16 '18 at 17:44
4
The return type of_searchCloser
is declared to bevoid
. How can itreturn
anything?
– Fred Larson
Nov 16 '18 at 17:45
... so how does the above code even compile?
– John Bollinger
Nov 16 '18 at 17:47
2
Save time. Enable compiler warnings.
– chux
Nov 16 '18 at 18:04
1
"when i print it in the main function" --> that code is not posted. To best answer this, please provide a Minimal, Complete, and Verifiable example. And change_searchCloser()
from returningvoid
to something. With "use void* the compile warn against conflicting types and does not compile." then post the corresponding code and exact warning.
– chux
Nov 16 '18 at 18:33
you're returning the value that represents the address that
closer
holds, not the value it points to. use return *closer;
instead– Tau
Nov 16 '18 at 17:44
you're returning the value that represents the address that
closer
holds, not the value it points to. use return *closer;
instead– Tau
Nov 16 '18 at 17:44
4
4
The return type of
_searchCloser
is declared to be void
. How can it return
anything?– Fred Larson
Nov 16 '18 at 17:45
The return type of
_searchCloser
is declared to be void
. How can it return
anything?– Fred Larson
Nov 16 '18 at 17:45
... so how does the above code even compile?
– John Bollinger
Nov 16 '18 at 17:47
... so how does the above code even compile?
– John Bollinger
Nov 16 '18 at 17:47
2
2
Save time. Enable compiler warnings.
– chux
Nov 16 '18 at 18:04
Save time. Enable compiler warnings.
– chux
Nov 16 '18 at 18:04
1
1
"when i print it in the main function" --> that code is not posted. To best answer this, please provide a Minimal, Complete, and Verifiable example. And change
_searchCloser()
from returning void
to something. With "use void* the compile warn against conflicting types and does not compile." then post the corresponding code and exact warning.– chux
Nov 16 '18 at 18:33
"when i print it in the main function" --> that code is not posted. To best answer this, please provide a Minimal, Complete, and Verifiable example. And change
_searchCloser()
from returning void
to something. With "use void* the compile warn against conflicting types and does not compile." then post the corresponding code and exact warning.– chux
Nov 16 '18 at 18:33
|
show 5 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53342855%2fwhy-is-my-recursive-function-returning-this-pointer-in-a-weird-format%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53342855%2fwhy-is-my-recursive-function-returning-this-pointer-in-a-weird-format%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
P3ET iXtM3EXcvj8IaAwER,a97GwQQ,q1MtIgdVRZ h HSB,sYW3uJNAKUOTvy
you're returning the value that represents the address that
closer
holds, not the value it points to. usereturn *closer;
instead– Tau
Nov 16 '18 at 17:44
4
The return type of
_searchCloser
is declared to bevoid
. How can itreturn
anything?– Fred Larson
Nov 16 '18 at 17:45
... so how does the above code even compile?
– John Bollinger
Nov 16 '18 at 17:47
2
Save time. Enable compiler warnings.
– chux
Nov 16 '18 at 18:04
1
"when i print it in the main function" --> that code is not posted. To best answer this, please provide a Minimal, Complete, and Verifiable example. And change
_searchCloser()
from returningvoid
to something. With "use void* the compile warn against conflicting types and does not compile." then post the corresponding code and exact warning.– chux
Nov 16 '18 at 18:33