OpenGL application crashes when number of vertexes increases [closed]
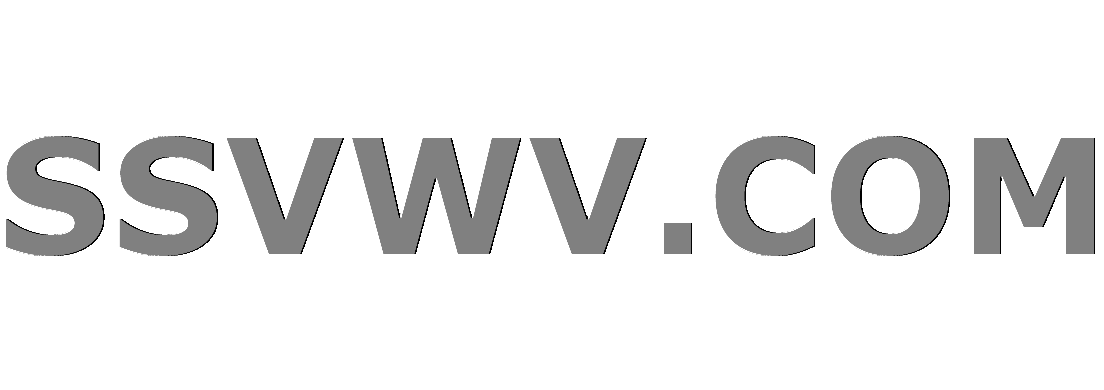
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I'm working on OpenGL application, c++ and Qt5
I wrote basic rendering engine, everything is fine when number of vertexes is relatively small, it plots, pans, zooms, etc. Then I increase number of vertex and application crashes with segmentation fault. On my Linux machine it happens when there are 650000-ish vertexes (the number floats a little, may be +/- 50), on windows laptop it is less. Of course, first thought was about memory overflow, but I got 2Gb GPU memory and 1.6Gb free after I create and fill a buffer with the number of point close to the crash level (memory stats taken from NVX extension, so should be reliable enough).
I made a code some time ago in Python, doing same things, and it worked totally well on the same laptop. Do you have any idea what I do wrong now?
Here are some code snippets:
Vertex shader (simplified as much as possible):
#version 330
uniform mat4 projection;
in vec3 position;
out vec4 a_facecolor;
void main()
{
gl_Position = projection*vec4(position,1);
gl_PointSize = 5;
a_facecolor = vec4(1,0,0,1);
}
Fragment:
in vec4 a_facecolor;
void main(void)
{
gl_FragColor = a_facecolor;
}
Array initialization:
#define N_POINTS 600000
....
QVector3D data[N_POINTS];
for (long i=0; i < N_POINTS; i++){
data[i] = QVector3D((qrand()%100-50)/50.0f*2,
(qrand()%100-50)/50.0f*2,
(qrand()%100-50)/50.0f*1);
}
buffer.create();
buffer.bind();
buffer.allocate(data, sizeof(data));
Rendering:
program.bind();
buffer.bind();
program.setUniformValue("projection", projection*transform);
int vertexLocation = program.attributeLocation("position");
program.enableAttributeArray(vertexLocation);
program.setAttributeBuffer(vertexLocation, GL_FLOAT, 0, 3);
glDrawArrays(GL_LINE_STRIP,0,N_POINTS);
glDrawArrays(GL_POINTS,0,N_POINTS);
c++ qt opengl
closed as off-topic by Nicol Bolas, genpfault, meowgoesthedog, Rabbid76, eyllanesc Nov 16 '18 at 20:11
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Questions seeking debugging help ("why isn't this code working?") must include the desired behavior, a specific problem or error and the shortest code necessary to reproduce it in the question itself. Questions without a clear problem statement are not useful to other readers. See: How to create a Minimal, Complete, and Verifiable example." – Nicol Bolas, genpfault, meowgoesthedog, Rabbid76, eyllanesc
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
I'm working on OpenGL application, c++ and Qt5
I wrote basic rendering engine, everything is fine when number of vertexes is relatively small, it plots, pans, zooms, etc. Then I increase number of vertex and application crashes with segmentation fault. On my Linux machine it happens when there are 650000-ish vertexes (the number floats a little, may be +/- 50), on windows laptop it is less. Of course, first thought was about memory overflow, but I got 2Gb GPU memory and 1.6Gb free after I create and fill a buffer with the number of point close to the crash level (memory stats taken from NVX extension, so should be reliable enough).
I made a code some time ago in Python, doing same things, and it worked totally well on the same laptop. Do you have any idea what I do wrong now?
Here are some code snippets:
Vertex shader (simplified as much as possible):
#version 330
uniform mat4 projection;
in vec3 position;
out vec4 a_facecolor;
void main()
{
gl_Position = projection*vec4(position,1);
gl_PointSize = 5;
a_facecolor = vec4(1,0,0,1);
}
Fragment:
in vec4 a_facecolor;
void main(void)
{
gl_FragColor = a_facecolor;
}
Array initialization:
#define N_POINTS 600000
....
QVector3D data[N_POINTS];
for (long i=0; i < N_POINTS; i++){
data[i] = QVector3D((qrand()%100-50)/50.0f*2,
(qrand()%100-50)/50.0f*2,
(qrand()%100-50)/50.0f*1);
}
buffer.create();
buffer.bind();
buffer.allocate(data, sizeof(data));
Rendering:
program.bind();
buffer.bind();
program.setUniformValue("projection", projection*transform);
int vertexLocation = program.attributeLocation("position");
program.enableAttributeArray(vertexLocation);
program.setAttributeBuffer(vertexLocation, GL_FLOAT, 0, 3);
glDrawArrays(GL_LINE_STRIP,0,N_POINTS);
glDrawArrays(GL_POINTS,0,N_POINTS);
c++ qt opengl
closed as off-topic by Nicol Bolas, genpfault, meowgoesthedog, Rabbid76, eyllanesc Nov 16 '18 at 20:11
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Questions seeking debugging help ("why isn't this code working?") must include the desired behavior, a specific problem or error and the shortest code necessary to reproduce it in the question itself. Questions without a clear problem statement are not useful to other readers. See: How to create a Minimal, Complete, and Verifiable example." – Nicol Bolas, genpfault, meowgoesthedog, Rabbid76, eyllanesc
If this question can be reworded to fit the rules in the help center, please edit the question.
2
Possible issue might be thatdata
is allocated on the stack and large enough to cause a stack overflow. To make sure whether this is the case, please provide a MCVE
– user10605163
Nov 16 '18 at 17:29
Thanks eukaryouta, that was it!
– Василий Синкевич
Nov 16 '18 at 17:42
add a comment |
I'm working on OpenGL application, c++ and Qt5
I wrote basic rendering engine, everything is fine when number of vertexes is relatively small, it plots, pans, zooms, etc. Then I increase number of vertex and application crashes with segmentation fault. On my Linux machine it happens when there are 650000-ish vertexes (the number floats a little, may be +/- 50), on windows laptop it is less. Of course, first thought was about memory overflow, but I got 2Gb GPU memory and 1.6Gb free after I create and fill a buffer with the number of point close to the crash level (memory stats taken from NVX extension, so should be reliable enough).
I made a code some time ago in Python, doing same things, and it worked totally well on the same laptop. Do you have any idea what I do wrong now?
Here are some code snippets:
Vertex shader (simplified as much as possible):
#version 330
uniform mat4 projection;
in vec3 position;
out vec4 a_facecolor;
void main()
{
gl_Position = projection*vec4(position,1);
gl_PointSize = 5;
a_facecolor = vec4(1,0,0,1);
}
Fragment:
in vec4 a_facecolor;
void main(void)
{
gl_FragColor = a_facecolor;
}
Array initialization:
#define N_POINTS 600000
....
QVector3D data[N_POINTS];
for (long i=0; i < N_POINTS; i++){
data[i] = QVector3D((qrand()%100-50)/50.0f*2,
(qrand()%100-50)/50.0f*2,
(qrand()%100-50)/50.0f*1);
}
buffer.create();
buffer.bind();
buffer.allocate(data, sizeof(data));
Rendering:
program.bind();
buffer.bind();
program.setUniformValue("projection", projection*transform);
int vertexLocation = program.attributeLocation("position");
program.enableAttributeArray(vertexLocation);
program.setAttributeBuffer(vertexLocation, GL_FLOAT, 0, 3);
glDrawArrays(GL_LINE_STRIP,0,N_POINTS);
glDrawArrays(GL_POINTS,0,N_POINTS);
c++ qt opengl
I'm working on OpenGL application, c++ and Qt5
I wrote basic rendering engine, everything is fine when number of vertexes is relatively small, it plots, pans, zooms, etc. Then I increase number of vertex and application crashes with segmentation fault. On my Linux machine it happens when there are 650000-ish vertexes (the number floats a little, may be +/- 50), on windows laptop it is less. Of course, first thought was about memory overflow, but I got 2Gb GPU memory and 1.6Gb free after I create and fill a buffer with the number of point close to the crash level (memory stats taken from NVX extension, so should be reliable enough).
I made a code some time ago in Python, doing same things, and it worked totally well on the same laptop. Do you have any idea what I do wrong now?
Here are some code snippets:
Vertex shader (simplified as much as possible):
#version 330
uniform mat4 projection;
in vec3 position;
out vec4 a_facecolor;
void main()
{
gl_Position = projection*vec4(position,1);
gl_PointSize = 5;
a_facecolor = vec4(1,0,0,1);
}
Fragment:
in vec4 a_facecolor;
void main(void)
{
gl_FragColor = a_facecolor;
}
Array initialization:
#define N_POINTS 600000
....
QVector3D data[N_POINTS];
for (long i=0; i < N_POINTS; i++){
data[i] = QVector3D((qrand()%100-50)/50.0f*2,
(qrand()%100-50)/50.0f*2,
(qrand()%100-50)/50.0f*1);
}
buffer.create();
buffer.bind();
buffer.allocate(data, sizeof(data));
Rendering:
program.bind();
buffer.bind();
program.setUniformValue("projection", projection*transform);
int vertexLocation = program.attributeLocation("position");
program.enableAttributeArray(vertexLocation);
program.setAttributeBuffer(vertexLocation, GL_FLOAT, 0, 3);
glDrawArrays(GL_LINE_STRIP,0,N_POINTS);
glDrawArrays(GL_POINTS,0,N_POINTS);
c++ qt opengl
c++ qt opengl
edited Nov 16 '18 at 18:27


genpfault
42.6k954100
42.6k954100
asked Nov 16 '18 at 17:18


Василий СинкевичВасилий Синкевич
61
61
closed as off-topic by Nicol Bolas, genpfault, meowgoesthedog, Rabbid76, eyllanesc Nov 16 '18 at 20:11
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Questions seeking debugging help ("why isn't this code working?") must include the desired behavior, a specific problem or error and the shortest code necessary to reproduce it in the question itself. Questions without a clear problem statement are not useful to other readers. See: How to create a Minimal, Complete, and Verifiable example." – Nicol Bolas, genpfault, meowgoesthedog, Rabbid76, eyllanesc
If this question can be reworded to fit the rules in the help center, please edit the question.
closed as off-topic by Nicol Bolas, genpfault, meowgoesthedog, Rabbid76, eyllanesc Nov 16 '18 at 20:11
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Questions seeking debugging help ("why isn't this code working?") must include the desired behavior, a specific problem or error and the shortest code necessary to reproduce it in the question itself. Questions without a clear problem statement are not useful to other readers. See: How to create a Minimal, Complete, and Verifiable example." – Nicol Bolas, genpfault, meowgoesthedog, Rabbid76, eyllanesc
If this question can be reworded to fit the rules in the help center, please edit the question.
2
Possible issue might be thatdata
is allocated on the stack and large enough to cause a stack overflow. To make sure whether this is the case, please provide a MCVE
– user10605163
Nov 16 '18 at 17:29
Thanks eukaryouta, that was it!
– Василий Синкевич
Nov 16 '18 at 17:42
add a comment |
2
Possible issue might be thatdata
is allocated on the stack and large enough to cause a stack overflow. To make sure whether this is the case, please provide a MCVE
– user10605163
Nov 16 '18 at 17:29
Thanks eukaryouta, that was it!
– Василий Синкевич
Nov 16 '18 at 17:42
2
2
Possible issue might be that
data
is allocated on the stack and large enough to cause a stack overflow. To make sure whether this is the case, please provide a MCVE– user10605163
Nov 16 '18 at 17:29
Possible issue might be that
data
is allocated on the stack and large enough to cause a stack overflow. To make sure whether this is the case, please provide a MCVE– user10605163
Nov 16 '18 at 17:29
Thanks eukaryouta, that was it!
– Василий Синкевич
Nov 16 '18 at 17:42
Thanks eukaryouta, that was it!
– Василий Синкевич
Nov 16 '18 at 17:42
add a comment |
1 Answer
1
active
oldest
votes
Based on answer to comment:
data
is an array allocated on the stack. If its size becomes larger than the maximal stack size allowed, which is typically much smaller than the total available memory, the program will crash with a stack overflow.
In order to use heap allocation, try:
std::vector<QVector3D> data(N_POINTS);
and then:
buffer.allocate(data.data(), sizeof(QVector3D)*data.size());
3
"I am vaguely guessing here from the snippets:" Please do not guess. If the question is missing information, then flag for closure.
– Nicol Bolas
Nov 16 '18 at 17:27
@NicolBolas I have adjusted the answer based on the discussion in the question comments. Is this ok or should the question still be flagged?
– user10605163
Nov 16 '18 at 17:53
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Based on answer to comment:
data
is an array allocated on the stack. If its size becomes larger than the maximal stack size allowed, which is typically much smaller than the total available memory, the program will crash with a stack overflow.
In order to use heap allocation, try:
std::vector<QVector3D> data(N_POINTS);
and then:
buffer.allocate(data.data(), sizeof(QVector3D)*data.size());
3
"I am vaguely guessing here from the snippets:" Please do not guess. If the question is missing information, then flag for closure.
– Nicol Bolas
Nov 16 '18 at 17:27
@NicolBolas I have adjusted the answer based on the discussion in the question comments. Is this ok or should the question still be flagged?
– user10605163
Nov 16 '18 at 17:53
add a comment |
Based on answer to comment:
data
is an array allocated on the stack. If its size becomes larger than the maximal stack size allowed, which is typically much smaller than the total available memory, the program will crash with a stack overflow.
In order to use heap allocation, try:
std::vector<QVector3D> data(N_POINTS);
and then:
buffer.allocate(data.data(), sizeof(QVector3D)*data.size());
3
"I am vaguely guessing here from the snippets:" Please do not guess. If the question is missing information, then flag for closure.
– Nicol Bolas
Nov 16 '18 at 17:27
@NicolBolas I have adjusted the answer based on the discussion in the question comments. Is this ok or should the question still be flagged?
– user10605163
Nov 16 '18 at 17:53
add a comment |
Based on answer to comment:
data
is an array allocated on the stack. If its size becomes larger than the maximal stack size allowed, which is typically much smaller than the total available memory, the program will crash with a stack overflow.
In order to use heap allocation, try:
std::vector<QVector3D> data(N_POINTS);
and then:
buffer.allocate(data.data(), sizeof(QVector3D)*data.size());
Based on answer to comment:
data
is an array allocated on the stack. If its size becomes larger than the maximal stack size allowed, which is typically much smaller than the total available memory, the program will crash with a stack overflow.
In order to use heap allocation, try:
std::vector<QVector3D> data(N_POINTS);
and then:
buffer.allocate(data.data(), sizeof(QVector3D)*data.size());
edited Nov 16 '18 at 19:03
answered Nov 16 '18 at 17:23
user10605163user10605163
2,868624
2,868624
3
"I am vaguely guessing here from the snippets:" Please do not guess. If the question is missing information, then flag for closure.
– Nicol Bolas
Nov 16 '18 at 17:27
@NicolBolas I have adjusted the answer based on the discussion in the question comments. Is this ok or should the question still be flagged?
– user10605163
Nov 16 '18 at 17:53
add a comment |
3
"I am vaguely guessing here from the snippets:" Please do not guess. If the question is missing information, then flag for closure.
– Nicol Bolas
Nov 16 '18 at 17:27
@NicolBolas I have adjusted the answer based on the discussion in the question comments. Is this ok or should the question still be flagged?
– user10605163
Nov 16 '18 at 17:53
3
3
"I am vaguely guessing here from the snippets:" Please do not guess. If the question is missing information, then flag for closure.
– Nicol Bolas
Nov 16 '18 at 17:27
"I am vaguely guessing here from the snippets:" Please do not guess. If the question is missing information, then flag for closure.
– Nicol Bolas
Nov 16 '18 at 17:27
@NicolBolas I have adjusted the answer based on the discussion in the question comments. Is this ok or should the question still be flagged?
– user10605163
Nov 16 '18 at 17:53
@NicolBolas I have adjusted the answer based on the discussion in the question comments. Is this ok or should the question still be flagged?
– user10605163
Nov 16 '18 at 17:53
add a comment |
mi8xZuTZq1,5jtRJuYQ3mD4e,x,KcZ
2
Possible issue might be that
data
is allocated on the stack and large enough to cause a stack overflow. To make sure whether this is the case, please provide a MCVE– user10605163
Nov 16 '18 at 17:29
Thanks eukaryouta, that was it!
– Василий Синкевич
Nov 16 '18 at 17:42