React Native & Firebase Search
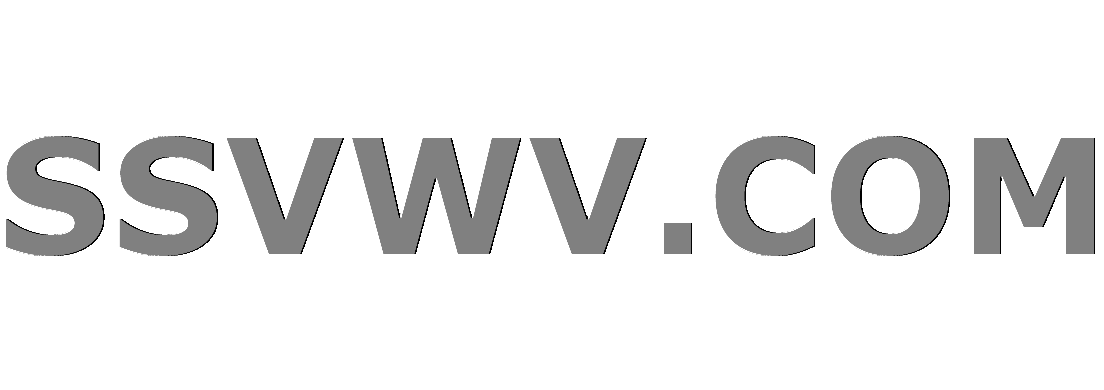
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I am trying to use firebase and perform a search. The trouble I am having is when I type the search text the list data isn't being updated.
Below is the code I have come up with
componentDidMount() {
this.setState({loading: true});
var merchants = new Array;
merchantsRef.once('value').then((snapshot) => {
snapshot.forEach((child) => {
merchants.push({
id: child.val().id,
type: child.val().type,
attributes: {
coming_soon: child.val().attributes.coming_soon,
featured_image_url: child.val().attributes.featured_image_url,
has_locations: child.val().attributes.has_locations,
highlight: child.val().attributes.highlight,
logo_url: child.val().attributes.logo_url,
main_image_url: child.val().attributes.main_image_url,
name: child.val().attributes.name,
offer_active: child.val().attributes.offer_active,
offer_cta_title: child.val().attributes.offer_cta_title,
offer_ends_at: child.val().attributes.offer_ends_at,
offer_starts_at: child.val().attributes.offer_starts_at,
offer_teaser: child.val().attributes.offer_teaser,
offer_terms: child.val().attributes.offer_terms,
offer_text: child.val().attributes.offer_text,
offer_url: child.val().attributes.offer_url,
permalink: child.val().attributes.permalink,
shop_url: child.val().attributes.shop_url,
},
_key: child.key
})
})
this.setState({
data: merchants,
loading: false,
})
// console.log(this.state.data)
}).catch((error) => {
console.log('Error fetching retailer data:', error)
})
}
searchFilterFunction = text => {
merchantsRef.orderByChild('name').startAt(text.toString()).on('value', function(child) {
console.log(child.val());
});
};
_renderEmpty = () => {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<ActivityIndicator />
</View>
)
}
_renderItem = ({item}) => (
<MerchantRow data={item} />
)
_keyExtractor = (item, index) => item._key;
render() {
if (this.state.loading) {
console.log("Loading")
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<ActivityIndicator
animating
size="large"
/>
<Text>Fetching shops...</Text>
</View>
)
}
return (
<View style={styles.container}>
<SearchBar
placeholder='Search for retailers...'
containerStyle={{backgroundColor: Colors.blue, borderTopWidth: 0, borderBottomWidth: 0}}
inputStyle={{backgroundColor: Colors.snow}}
onChangeText={text => this.searchFilterFunction(text)}
autoCorrect={false}
/>
<ScrollView style={styles.container}>
<FlatList
data={this.state.data}
renderItem={this._renderItem.bind(this)}
keyExtractor={this._keyExtractor}
initialNumToRender={9}
ItemSeparatorComponent={this._renderSeparator}
removeClippedSubviews={false}
ListEmptyComponent={this._renderEmpty}
/>
</ScrollView>
</View>
)
}
javascript react-native firebase-realtime-database expo searchbar
add a comment |
I am trying to use firebase and perform a search. The trouble I am having is when I type the search text the list data isn't being updated.
Below is the code I have come up with
componentDidMount() {
this.setState({loading: true});
var merchants = new Array;
merchantsRef.once('value').then((snapshot) => {
snapshot.forEach((child) => {
merchants.push({
id: child.val().id,
type: child.val().type,
attributes: {
coming_soon: child.val().attributes.coming_soon,
featured_image_url: child.val().attributes.featured_image_url,
has_locations: child.val().attributes.has_locations,
highlight: child.val().attributes.highlight,
logo_url: child.val().attributes.logo_url,
main_image_url: child.val().attributes.main_image_url,
name: child.val().attributes.name,
offer_active: child.val().attributes.offer_active,
offer_cta_title: child.val().attributes.offer_cta_title,
offer_ends_at: child.val().attributes.offer_ends_at,
offer_starts_at: child.val().attributes.offer_starts_at,
offer_teaser: child.val().attributes.offer_teaser,
offer_terms: child.val().attributes.offer_terms,
offer_text: child.val().attributes.offer_text,
offer_url: child.val().attributes.offer_url,
permalink: child.val().attributes.permalink,
shop_url: child.val().attributes.shop_url,
},
_key: child.key
})
})
this.setState({
data: merchants,
loading: false,
})
// console.log(this.state.data)
}).catch((error) => {
console.log('Error fetching retailer data:', error)
})
}
searchFilterFunction = text => {
merchantsRef.orderByChild('name').startAt(text.toString()).on('value', function(child) {
console.log(child.val());
});
};
_renderEmpty = () => {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<ActivityIndicator />
</View>
)
}
_renderItem = ({item}) => (
<MerchantRow data={item} />
)
_keyExtractor = (item, index) => item._key;
render() {
if (this.state.loading) {
console.log("Loading")
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<ActivityIndicator
animating
size="large"
/>
<Text>Fetching shops...</Text>
</View>
)
}
return (
<View style={styles.container}>
<SearchBar
placeholder='Search for retailers...'
containerStyle={{backgroundColor: Colors.blue, borderTopWidth: 0, borderBottomWidth: 0}}
inputStyle={{backgroundColor: Colors.snow}}
onChangeText={text => this.searchFilterFunction(text)}
autoCorrect={false}
/>
<ScrollView style={styles.container}>
<FlatList
data={this.state.data}
renderItem={this._renderItem.bind(this)}
keyExtractor={this._keyExtractor}
initialNumToRender={9}
ItemSeparatorComponent={this._renderSeparator}
removeClippedSubviews={false}
ListEmptyComponent={this._renderEmpty}
/>
</ScrollView>
</View>
)
}
javascript react-native firebase-realtime-database expo searchbar
What does yourSearchBar
component look like?
– Tom Davies
Nov 16 '18 at 14:06
@TomDavies its in the code above<SearchBar placeholder='Search for retailers...' containerStyle={{backgroundColor: Colors.blue, borderTopWidth: 0, borderBottomWidth: 0}} inputStyle={{backgroundColor: Colors.snow}} onChangeText={text => this.searchFilterFunction(text)} autoCorrect={false} />
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:07
@Paul'Whippet'McGuane, I can't seem to see where you're setting the data on yoursearchFilterFunction
, is the function currently console.logging the values correctly? If so, it might just be a matter of needing to run `this.setState({ data: child.val() });
– Ariel Salem
Nov 16 '18 at 14:15
@ArielSalem when do that I get setstate is undefined
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:19
add a comment |
I am trying to use firebase and perform a search. The trouble I am having is when I type the search text the list data isn't being updated.
Below is the code I have come up with
componentDidMount() {
this.setState({loading: true});
var merchants = new Array;
merchantsRef.once('value').then((snapshot) => {
snapshot.forEach((child) => {
merchants.push({
id: child.val().id,
type: child.val().type,
attributes: {
coming_soon: child.val().attributes.coming_soon,
featured_image_url: child.val().attributes.featured_image_url,
has_locations: child.val().attributes.has_locations,
highlight: child.val().attributes.highlight,
logo_url: child.val().attributes.logo_url,
main_image_url: child.val().attributes.main_image_url,
name: child.val().attributes.name,
offer_active: child.val().attributes.offer_active,
offer_cta_title: child.val().attributes.offer_cta_title,
offer_ends_at: child.val().attributes.offer_ends_at,
offer_starts_at: child.val().attributes.offer_starts_at,
offer_teaser: child.val().attributes.offer_teaser,
offer_terms: child.val().attributes.offer_terms,
offer_text: child.val().attributes.offer_text,
offer_url: child.val().attributes.offer_url,
permalink: child.val().attributes.permalink,
shop_url: child.val().attributes.shop_url,
},
_key: child.key
})
})
this.setState({
data: merchants,
loading: false,
})
// console.log(this.state.data)
}).catch((error) => {
console.log('Error fetching retailer data:', error)
})
}
searchFilterFunction = text => {
merchantsRef.orderByChild('name').startAt(text.toString()).on('value', function(child) {
console.log(child.val());
});
};
_renderEmpty = () => {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<ActivityIndicator />
</View>
)
}
_renderItem = ({item}) => (
<MerchantRow data={item} />
)
_keyExtractor = (item, index) => item._key;
render() {
if (this.state.loading) {
console.log("Loading")
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<ActivityIndicator
animating
size="large"
/>
<Text>Fetching shops...</Text>
</View>
)
}
return (
<View style={styles.container}>
<SearchBar
placeholder='Search for retailers...'
containerStyle={{backgroundColor: Colors.blue, borderTopWidth: 0, borderBottomWidth: 0}}
inputStyle={{backgroundColor: Colors.snow}}
onChangeText={text => this.searchFilterFunction(text)}
autoCorrect={false}
/>
<ScrollView style={styles.container}>
<FlatList
data={this.state.data}
renderItem={this._renderItem.bind(this)}
keyExtractor={this._keyExtractor}
initialNumToRender={9}
ItemSeparatorComponent={this._renderSeparator}
removeClippedSubviews={false}
ListEmptyComponent={this._renderEmpty}
/>
</ScrollView>
</View>
)
}
javascript react-native firebase-realtime-database expo searchbar
I am trying to use firebase and perform a search. The trouble I am having is when I type the search text the list data isn't being updated.
Below is the code I have come up with
componentDidMount() {
this.setState({loading: true});
var merchants = new Array;
merchantsRef.once('value').then((snapshot) => {
snapshot.forEach((child) => {
merchants.push({
id: child.val().id,
type: child.val().type,
attributes: {
coming_soon: child.val().attributes.coming_soon,
featured_image_url: child.val().attributes.featured_image_url,
has_locations: child.val().attributes.has_locations,
highlight: child.val().attributes.highlight,
logo_url: child.val().attributes.logo_url,
main_image_url: child.val().attributes.main_image_url,
name: child.val().attributes.name,
offer_active: child.val().attributes.offer_active,
offer_cta_title: child.val().attributes.offer_cta_title,
offer_ends_at: child.val().attributes.offer_ends_at,
offer_starts_at: child.val().attributes.offer_starts_at,
offer_teaser: child.val().attributes.offer_teaser,
offer_terms: child.val().attributes.offer_terms,
offer_text: child.val().attributes.offer_text,
offer_url: child.val().attributes.offer_url,
permalink: child.val().attributes.permalink,
shop_url: child.val().attributes.shop_url,
},
_key: child.key
})
})
this.setState({
data: merchants,
loading: false,
})
// console.log(this.state.data)
}).catch((error) => {
console.log('Error fetching retailer data:', error)
})
}
searchFilterFunction = text => {
merchantsRef.orderByChild('name').startAt(text.toString()).on('value', function(child) {
console.log(child.val());
});
};
_renderEmpty = () => {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<ActivityIndicator />
</View>
)
}
_renderItem = ({item}) => (
<MerchantRow data={item} />
)
_keyExtractor = (item, index) => item._key;
render() {
if (this.state.loading) {
console.log("Loading")
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<ActivityIndicator
animating
size="large"
/>
<Text>Fetching shops...</Text>
</View>
)
}
return (
<View style={styles.container}>
<SearchBar
placeholder='Search for retailers...'
containerStyle={{backgroundColor: Colors.blue, borderTopWidth: 0, borderBottomWidth: 0}}
inputStyle={{backgroundColor: Colors.snow}}
onChangeText={text => this.searchFilterFunction(text)}
autoCorrect={false}
/>
<ScrollView style={styles.container}>
<FlatList
data={this.state.data}
renderItem={this._renderItem.bind(this)}
keyExtractor={this._keyExtractor}
initialNumToRender={9}
ItemSeparatorComponent={this._renderSeparator}
removeClippedSubviews={false}
ListEmptyComponent={this._renderEmpty}
/>
</ScrollView>
</View>
)
}
javascript react-native firebase-realtime-database expo searchbar
javascript react-native firebase-realtime-database expo searchbar
edited Nov 16 '18 at 14:04
Frank van Puffelen
245k30389418
245k30389418
asked Nov 16 '18 at 14:00
Paul 'Whippet' McGuanePaul 'Whippet' McGuane
1,56383266
1,56383266
What does yourSearchBar
component look like?
– Tom Davies
Nov 16 '18 at 14:06
@TomDavies its in the code above<SearchBar placeholder='Search for retailers...' containerStyle={{backgroundColor: Colors.blue, borderTopWidth: 0, borderBottomWidth: 0}} inputStyle={{backgroundColor: Colors.snow}} onChangeText={text => this.searchFilterFunction(text)} autoCorrect={false} />
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:07
@Paul'Whippet'McGuane, I can't seem to see where you're setting the data on yoursearchFilterFunction
, is the function currently console.logging the values correctly? If so, it might just be a matter of needing to run `this.setState({ data: child.val() });
– Ariel Salem
Nov 16 '18 at 14:15
@ArielSalem when do that I get setstate is undefined
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:19
add a comment |
What does yourSearchBar
component look like?
– Tom Davies
Nov 16 '18 at 14:06
@TomDavies its in the code above<SearchBar placeholder='Search for retailers...' containerStyle={{backgroundColor: Colors.blue, borderTopWidth: 0, borderBottomWidth: 0}} inputStyle={{backgroundColor: Colors.snow}} onChangeText={text => this.searchFilterFunction(text)} autoCorrect={false} />
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:07
@Paul'Whippet'McGuane, I can't seem to see where you're setting the data on yoursearchFilterFunction
, is the function currently console.logging the values correctly? If so, it might just be a matter of needing to run `this.setState({ data: child.val() });
– Ariel Salem
Nov 16 '18 at 14:15
@ArielSalem when do that I get setstate is undefined
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:19
What does your
SearchBar
component look like?– Tom Davies
Nov 16 '18 at 14:06
What does your
SearchBar
component look like?– Tom Davies
Nov 16 '18 at 14:06
@TomDavies its in the code above
<SearchBar placeholder='Search for retailers...' containerStyle={{backgroundColor: Colors.blue, borderTopWidth: 0, borderBottomWidth: 0}} inputStyle={{backgroundColor: Colors.snow}} onChangeText={text => this.searchFilterFunction(text)} autoCorrect={false} />
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:07
@TomDavies its in the code above
<SearchBar placeholder='Search for retailers...' containerStyle={{backgroundColor: Colors.blue, borderTopWidth: 0, borderBottomWidth: 0}} inputStyle={{backgroundColor: Colors.snow}} onChangeText={text => this.searchFilterFunction(text)} autoCorrect={false} />
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:07
@Paul'Whippet'McGuane, I can't seem to see where you're setting the data on your
searchFilterFunction
, is the function currently console.logging the values correctly? If so, it might just be a matter of needing to run `this.setState({ data: child.val() });– Ariel Salem
Nov 16 '18 at 14:15
@Paul'Whippet'McGuane, I can't seem to see where you're setting the data on your
searchFilterFunction
, is the function currently console.logging the values correctly? If so, it might just be a matter of needing to run `this.setState({ data: child.val() });– Ariel Salem
Nov 16 '18 at 14:15
@ArielSalem when do that I get setstate is undefined
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:19
@ArielSalem when do that I get setstate is undefined
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:19
add a comment |
1 Answer
1
active
oldest
votes
@Paul'Whippet'McGuane, good to know. That's likely because the context of this
isn't defined. In order to maintain the context of the keyword this, you can modify the searchFilterFunction like so:
searchFilterFunction = text => {
const that = this;
merchantsRef.orderByChild('name').startAt(text.toString()).on('value', function(child) {
console.log(child.val());
const val = child.val();
that.setState({ data: val });
});
};
@TomDavies, they do, and changing the existing function call fromfunction(child) { ....
to(child) => { ...
could be a worthy option to look into
– Ariel Salem
Nov 16 '18 at 14:25
that is presenting a new error 'Invariant Violation tried to get frame out of range' which appears to because flatlist is expecting an array?
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:30
@Paul'Whippet'McGuane, what is being console.logged in the searchFilterFunctionchild.val()
?
– Ariel Salem
Nov 16 '18 at 14:33
nopaste.xyz/?096907cc94ea6ad7#EjDbvba6osoojT8fhjv7q2XEv/…
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:38
1
Perfect. So the error was telling you that the data that was being set wasn't the correct type (i.e. expected an array, got an object). In your component did mount, you are creating an array of objects based on the child.val(). I'd recommend you utilize that same logic to set the state of your data since it appears that that is what you need to do
– Ariel Salem
Nov 16 '18 at 14:51
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53339332%2freact-native-firebase-search%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
@Paul'Whippet'McGuane, good to know. That's likely because the context of this
isn't defined. In order to maintain the context of the keyword this, you can modify the searchFilterFunction like so:
searchFilterFunction = text => {
const that = this;
merchantsRef.orderByChild('name').startAt(text.toString()).on('value', function(child) {
console.log(child.val());
const val = child.val();
that.setState({ data: val });
});
};
@TomDavies, they do, and changing the existing function call fromfunction(child) { ....
to(child) => { ...
could be a worthy option to look into
– Ariel Salem
Nov 16 '18 at 14:25
that is presenting a new error 'Invariant Violation tried to get frame out of range' which appears to because flatlist is expecting an array?
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:30
@Paul'Whippet'McGuane, what is being console.logged in the searchFilterFunctionchild.val()
?
– Ariel Salem
Nov 16 '18 at 14:33
nopaste.xyz/?096907cc94ea6ad7#EjDbvba6osoojT8fhjv7q2XEv/…
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:38
1
Perfect. So the error was telling you that the data that was being set wasn't the correct type (i.e. expected an array, got an object). In your component did mount, you are creating an array of objects based on the child.val(). I'd recommend you utilize that same logic to set the state of your data since it appears that that is what you need to do
– Ariel Salem
Nov 16 '18 at 14:51
add a comment |
@Paul'Whippet'McGuane, good to know. That's likely because the context of this
isn't defined. In order to maintain the context of the keyword this, you can modify the searchFilterFunction like so:
searchFilterFunction = text => {
const that = this;
merchantsRef.orderByChild('name').startAt(text.toString()).on('value', function(child) {
console.log(child.val());
const val = child.val();
that.setState({ data: val });
});
};
@TomDavies, they do, and changing the existing function call fromfunction(child) { ....
to(child) => { ...
could be a worthy option to look into
– Ariel Salem
Nov 16 '18 at 14:25
that is presenting a new error 'Invariant Violation tried to get frame out of range' which appears to because flatlist is expecting an array?
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:30
@Paul'Whippet'McGuane, what is being console.logged in the searchFilterFunctionchild.val()
?
– Ariel Salem
Nov 16 '18 at 14:33
nopaste.xyz/?096907cc94ea6ad7#EjDbvba6osoojT8fhjv7q2XEv/…
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:38
1
Perfect. So the error was telling you that the data that was being set wasn't the correct type (i.e. expected an array, got an object). In your component did mount, you are creating an array of objects based on the child.val(). I'd recommend you utilize that same logic to set the state of your data since it appears that that is what you need to do
– Ariel Salem
Nov 16 '18 at 14:51
add a comment |
@Paul'Whippet'McGuane, good to know. That's likely because the context of this
isn't defined. In order to maintain the context of the keyword this, you can modify the searchFilterFunction like so:
searchFilterFunction = text => {
const that = this;
merchantsRef.orderByChild('name').startAt(text.toString()).on('value', function(child) {
console.log(child.val());
const val = child.val();
that.setState({ data: val });
});
};
@Paul'Whippet'McGuane, good to know. That's likely because the context of this
isn't defined. In order to maintain the context of the keyword this, you can modify the searchFilterFunction like so:
searchFilterFunction = text => {
const that = this;
merchantsRef.orderByChild('name').startAt(text.toString()).on('value', function(child) {
console.log(child.val());
const val = child.val();
that.setState({ data: val });
});
};
answered Nov 16 '18 at 14:21


Ariel SalemAriel Salem
21629
21629
@TomDavies, they do, and changing the existing function call fromfunction(child) { ....
to(child) => { ...
could be a worthy option to look into
– Ariel Salem
Nov 16 '18 at 14:25
that is presenting a new error 'Invariant Violation tried to get frame out of range' which appears to because flatlist is expecting an array?
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:30
@Paul'Whippet'McGuane, what is being console.logged in the searchFilterFunctionchild.val()
?
– Ariel Salem
Nov 16 '18 at 14:33
nopaste.xyz/?096907cc94ea6ad7#EjDbvba6osoojT8fhjv7q2XEv/…
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:38
1
Perfect. So the error was telling you that the data that was being set wasn't the correct type (i.e. expected an array, got an object). In your component did mount, you are creating an array of objects based on the child.val(). I'd recommend you utilize that same logic to set the state of your data since it appears that that is what you need to do
– Ariel Salem
Nov 16 '18 at 14:51
add a comment |
@TomDavies, they do, and changing the existing function call fromfunction(child) { ....
to(child) => { ...
could be a worthy option to look into
– Ariel Salem
Nov 16 '18 at 14:25
that is presenting a new error 'Invariant Violation tried to get frame out of range' which appears to because flatlist is expecting an array?
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:30
@Paul'Whippet'McGuane, what is being console.logged in the searchFilterFunctionchild.val()
?
– Ariel Salem
Nov 16 '18 at 14:33
nopaste.xyz/?096907cc94ea6ad7#EjDbvba6osoojT8fhjv7q2XEv/…
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:38
1
Perfect. So the error was telling you that the data that was being set wasn't the correct type (i.e. expected an array, got an object). In your component did mount, you are creating an array of objects based on the child.val(). I'd recommend you utilize that same logic to set the state of your data since it appears that that is what you need to do
– Ariel Salem
Nov 16 '18 at 14:51
@TomDavies, they do, and changing the existing function call from
function(child) { ....
to (child) => { ...
could be a worthy option to look into– Ariel Salem
Nov 16 '18 at 14:25
@TomDavies, they do, and changing the existing function call from
function(child) { ....
to (child) => { ...
could be a worthy option to look into– Ariel Salem
Nov 16 '18 at 14:25
that is presenting a new error 'Invariant Violation tried to get frame out of range' which appears to because flatlist is expecting an array?
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:30
that is presenting a new error 'Invariant Violation tried to get frame out of range' which appears to because flatlist is expecting an array?
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:30
@Paul'Whippet'McGuane, what is being console.logged in the searchFilterFunction
child.val()
?– Ariel Salem
Nov 16 '18 at 14:33
@Paul'Whippet'McGuane, what is being console.logged in the searchFilterFunction
child.val()
?– Ariel Salem
Nov 16 '18 at 14:33
nopaste.xyz/?096907cc94ea6ad7#EjDbvba6osoojT8fhjv7q2XEv/…
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:38
nopaste.xyz/?096907cc94ea6ad7#EjDbvba6osoojT8fhjv7q2XEv/…
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:38
1
1
Perfect. So the error was telling you that the data that was being set wasn't the correct type (i.e. expected an array, got an object). In your component did mount, you are creating an array of objects based on the child.val(). I'd recommend you utilize that same logic to set the state of your data since it appears that that is what you need to do
– Ariel Salem
Nov 16 '18 at 14:51
Perfect. So the error was telling you that the data that was being set wasn't the correct type (i.e. expected an array, got an object). In your component did mount, you are creating an array of objects based on the child.val(). I'd recommend you utilize that same logic to set the state of your data since it appears that that is what you need to do
– Ariel Salem
Nov 16 '18 at 14:51
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53339332%2freact-native-firebase-search%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
cgWvkdR,tam8h,iohY3,zj pOcezcpGJ38ITe4ClHgaUqi7mWQxvgJFaAc FWeSo5DMAha
What does your
SearchBar
component look like?– Tom Davies
Nov 16 '18 at 14:06
@TomDavies its in the code above
<SearchBar placeholder='Search for retailers...' containerStyle={{backgroundColor: Colors.blue, borderTopWidth: 0, borderBottomWidth: 0}} inputStyle={{backgroundColor: Colors.snow}} onChangeText={text => this.searchFilterFunction(text)} autoCorrect={false} />
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:07
@Paul'Whippet'McGuane, I can't seem to see where you're setting the data on your
searchFilterFunction
, is the function currently console.logging the values correctly? If so, it might just be a matter of needing to run `this.setState({ data: child.val() });– Ariel Salem
Nov 16 '18 at 14:15
@ArielSalem when do that I get setstate is undefined
– Paul 'Whippet' McGuane
Nov 16 '18 at 14:19