PHP - Loop cURL with increase id of URL
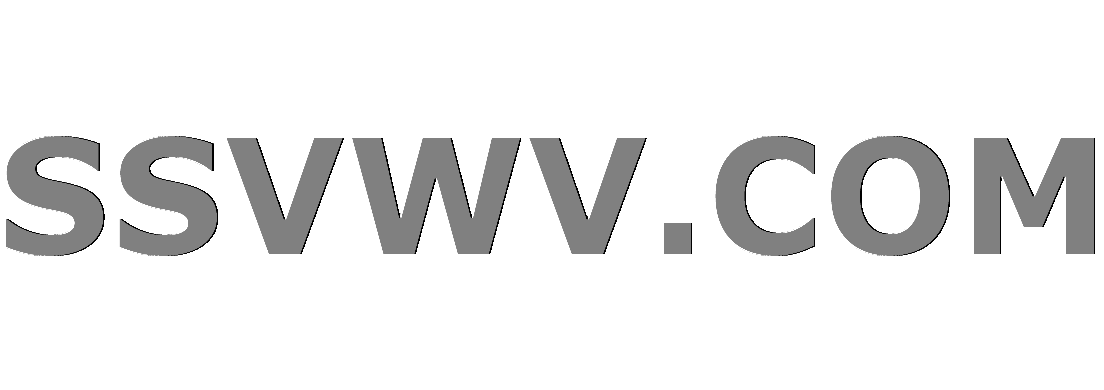
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
with the following code a name with an ID (each name in the gnd can be addressed by an ID) is received via the GND interface. This works.
Now i want to get many names at the same time with a cURL loop. The ID of the URL must always be increased by one and the cURL request must loop. How can I do this?
With this Code i receive for example names from the GND database
<?php
header('Content-type: text/html; charset=utf-8');
$User_Agent = 'Mozilla/5.0 (Windows NT 6.1; rv:60.0) Gecko/20100101 Firefox/60.0';
$url = "http://hub.culturegraph.org/entityfacts/118600001";
$request_headers = ;
$request_headers = 'Accept: application/json';
$request_headers = 'charset=utf-8';
$request_headers = 'Content-Type: application/json; charset=utf-8';
$request_headers = 'Accept-Encoding: gzip, deflate, identity';
$request_headers = 'Accept-Language: de,en-US;q=0.7,en;q=0.3';
for ($i = 1; $i <= 10; $i++)
{
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_USERAGENT, $User_Agent);
curl_setopt($ch, CURLOPT_HTTPHEADER, $request_headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_ENCODING, "");
$result = curl_exec($ch);
$code = curl_getinfo($ch, CURLINFO_HTTP_CODE);
}
curl_close($ch);
$data = json_decode($result, true);
if ($code == 200) {
$data = json_decode($result, true);
echo 'Erfolg';
} else {
$error = $data['Error'];
echo 'Beim anfordern der GND-Daten ist ein Fehler aufgetreten: Fehlercode ' . $error;
echo ' <a href="PHP_skript_login.php">Zurueck</a><br />';
}
var_dump($data['preferredName']);
Result for URL with ID 118600001 = Gerhardt von Reutern
But how must the code be adapted, so that also the names of the URL's 118600002, 118600003 and so on are output? So as often as it is specified. Be it 100 or 1000 times.
php api loops curl
add a comment |
with the following code a name with an ID (each name in the gnd can be addressed by an ID) is received via the GND interface. This works.
Now i want to get many names at the same time with a cURL loop. The ID of the URL must always be increased by one and the cURL request must loop. How can I do this?
With this Code i receive for example names from the GND database
<?php
header('Content-type: text/html; charset=utf-8');
$User_Agent = 'Mozilla/5.0 (Windows NT 6.1; rv:60.0) Gecko/20100101 Firefox/60.0';
$url = "http://hub.culturegraph.org/entityfacts/118600001";
$request_headers = ;
$request_headers = 'Accept: application/json';
$request_headers = 'charset=utf-8';
$request_headers = 'Content-Type: application/json; charset=utf-8';
$request_headers = 'Accept-Encoding: gzip, deflate, identity';
$request_headers = 'Accept-Language: de,en-US;q=0.7,en;q=0.3';
for ($i = 1; $i <= 10; $i++)
{
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_USERAGENT, $User_Agent);
curl_setopt($ch, CURLOPT_HTTPHEADER, $request_headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_ENCODING, "");
$result = curl_exec($ch);
$code = curl_getinfo($ch, CURLINFO_HTTP_CODE);
}
curl_close($ch);
$data = json_decode($result, true);
if ($code == 200) {
$data = json_decode($result, true);
echo 'Erfolg';
} else {
$error = $data['Error'];
echo 'Beim anfordern der GND-Daten ist ein Fehler aufgetreten: Fehlercode ' . $error;
echo ' <a href="PHP_skript_login.php">Zurueck</a><br />';
}
var_dump($data['preferredName']);
Result for URL with ID 118600001 = Gerhardt von Reutern
But how must the code be adapted, so that also the names of the URL's 118600002, 118600003 and so on are output? So as often as it is specified. Be it 100 or 1000 times.
php api loops curl
you create 10 curl handles and only close 1 of them. that's a resource leak and waste of cpu and ram. you can actually keep re-using the same curl handle and just change CURLOPT_URL~
– hanshenrik
Nov 16 '18 at 23:18
add a comment |
with the following code a name with an ID (each name in the gnd can be addressed by an ID) is received via the GND interface. This works.
Now i want to get many names at the same time with a cURL loop. The ID of the URL must always be increased by one and the cURL request must loop. How can I do this?
With this Code i receive for example names from the GND database
<?php
header('Content-type: text/html; charset=utf-8');
$User_Agent = 'Mozilla/5.0 (Windows NT 6.1; rv:60.0) Gecko/20100101 Firefox/60.0';
$url = "http://hub.culturegraph.org/entityfacts/118600001";
$request_headers = ;
$request_headers = 'Accept: application/json';
$request_headers = 'charset=utf-8';
$request_headers = 'Content-Type: application/json; charset=utf-8';
$request_headers = 'Accept-Encoding: gzip, deflate, identity';
$request_headers = 'Accept-Language: de,en-US;q=0.7,en;q=0.3';
for ($i = 1; $i <= 10; $i++)
{
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_USERAGENT, $User_Agent);
curl_setopt($ch, CURLOPT_HTTPHEADER, $request_headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_ENCODING, "");
$result = curl_exec($ch);
$code = curl_getinfo($ch, CURLINFO_HTTP_CODE);
}
curl_close($ch);
$data = json_decode($result, true);
if ($code == 200) {
$data = json_decode($result, true);
echo 'Erfolg';
} else {
$error = $data['Error'];
echo 'Beim anfordern der GND-Daten ist ein Fehler aufgetreten: Fehlercode ' . $error;
echo ' <a href="PHP_skript_login.php">Zurueck</a><br />';
}
var_dump($data['preferredName']);
Result for URL with ID 118600001 = Gerhardt von Reutern
But how must the code be adapted, so that also the names of the URL's 118600002, 118600003 and so on are output? So as often as it is specified. Be it 100 or 1000 times.
php api loops curl
with the following code a name with an ID (each name in the gnd can be addressed by an ID) is received via the GND interface. This works.
Now i want to get many names at the same time with a cURL loop. The ID of the URL must always be increased by one and the cURL request must loop. How can I do this?
With this Code i receive for example names from the GND database
<?php
header('Content-type: text/html; charset=utf-8');
$User_Agent = 'Mozilla/5.0 (Windows NT 6.1; rv:60.0) Gecko/20100101 Firefox/60.0';
$url = "http://hub.culturegraph.org/entityfacts/118600001";
$request_headers = ;
$request_headers = 'Accept: application/json';
$request_headers = 'charset=utf-8';
$request_headers = 'Content-Type: application/json; charset=utf-8';
$request_headers = 'Accept-Encoding: gzip, deflate, identity';
$request_headers = 'Accept-Language: de,en-US;q=0.7,en;q=0.3';
for ($i = 1; $i <= 10; $i++)
{
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_USERAGENT, $User_Agent);
curl_setopt($ch, CURLOPT_HTTPHEADER, $request_headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_ENCODING, "");
$result = curl_exec($ch);
$code = curl_getinfo($ch, CURLINFO_HTTP_CODE);
}
curl_close($ch);
$data = json_decode($result, true);
if ($code == 200) {
$data = json_decode($result, true);
echo 'Erfolg';
} else {
$error = $data['Error'];
echo 'Beim anfordern der GND-Daten ist ein Fehler aufgetreten: Fehlercode ' . $error;
echo ' <a href="PHP_skript_login.php">Zurueck</a><br />';
}
var_dump($data['preferredName']);
Result for URL with ID 118600001 = Gerhardt von Reutern
But how must the code be adapted, so that also the names of the URL's 118600002, 118600003 and so on are output? So as often as it is specified. Be it 100 or 1000 times.
php api loops curl
php api loops curl
asked Nov 16 '18 at 14:00
SIBSIB
189
189
you create 10 curl handles and only close 1 of them. that's a resource leak and waste of cpu and ram. you can actually keep re-using the same curl handle and just change CURLOPT_URL~
– hanshenrik
Nov 16 '18 at 23:18
add a comment |
you create 10 curl handles and only close 1 of them. that's a resource leak and waste of cpu and ram. you can actually keep re-using the same curl handle and just change CURLOPT_URL~
– hanshenrik
Nov 16 '18 at 23:18
you create 10 curl handles and only close 1 of them. that's a resource leak and waste of cpu and ram. you can actually keep re-using the same curl handle and just change CURLOPT_URL~
– hanshenrik
Nov 16 '18 at 23:18
you create 10 curl handles and only close 1 of them. that's a resource leak and waste of cpu and ram. you can actually keep re-using the same curl handle and just change CURLOPT_URL~
– hanshenrik
Nov 16 '18 at 23:18
add a comment |
1 Answer
1
active
oldest
votes
change your $url variable to something like $base_url which is the url without the ID
$base_url = "http://hub.culturegraph.org/entityfacts/";
Then in your for loop you do:
$ch = curl_init($base_url . (118600001 + $i));
this rule can be removed, this is not necessary:
curl_setopt($ch, CURLOPT_URL, $url);
You should also handle the response inside the for loop, else you will only see the last person's name, after an incredibly long load time.
Thanks that works. Do you have any idea how to ingorate empty ID's to avoid error messages? Or can you skip empty ID's?
– SIB
Nov 16 '18 at 14:18
You check on the error yourself, just don't throw an error.
– Bert Bijn
Nov 16 '18 at 16:20
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53339325%2fphp-loop-curl-with-increase-id-of-url%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
change your $url variable to something like $base_url which is the url without the ID
$base_url = "http://hub.culturegraph.org/entityfacts/";
Then in your for loop you do:
$ch = curl_init($base_url . (118600001 + $i));
this rule can be removed, this is not necessary:
curl_setopt($ch, CURLOPT_URL, $url);
You should also handle the response inside the for loop, else you will only see the last person's name, after an incredibly long load time.
Thanks that works. Do you have any idea how to ingorate empty ID's to avoid error messages? Or can you skip empty ID's?
– SIB
Nov 16 '18 at 14:18
You check on the error yourself, just don't throw an error.
– Bert Bijn
Nov 16 '18 at 16:20
add a comment |
change your $url variable to something like $base_url which is the url without the ID
$base_url = "http://hub.culturegraph.org/entityfacts/";
Then in your for loop you do:
$ch = curl_init($base_url . (118600001 + $i));
this rule can be removed, this is not necessary:
curl_setopt($ch, CURLOPT_URL, $url);
You should also handle the response inside the for loop, else you will only see the last person's name, after an incredibly long load time.
Thanks that works. Do you have any idea how to ingorate empty ID's to avoid error messages? Or can you skip empty ID's?
– SIB
Nov 16 '18 at 14:18
You check on the error yourself, just don't throw an error.
– Bert Bijn
Nov 16 '18 at 16:20
add a comment |
change your $url variable to something like $base_url which is the url without the ID
$base_url = "http://hub.culturegraph.org/entityfacts/";
Then in your for loop you do:
$ch = curl_init($base_url . (118600001 + $i));
this rule can be removed, this is not necessary:
curl_setopt($ch, CURLOPT_URL, $url);
You should also handle the response inside the for loop, else you will only see the last person's name, after an incredibly long load time.
change your $url variable to something like $base_url which is the url without the ID
$base_url = "http://hub.culturegraph.org/entityfacts/";
Then in your for loop you do:
$ch = curl_init($base_url . (118600001 + $i));
this rule can be removed, this is not necessary:
curl_setopt($ch, CURLOPT_URL, $url);
You should also handle the response inside the for loop, else you will only see the last person's name, after an incredibly long load time.
answered Nov 16 '18 at 14:05
Bert BijnBert Bijn
1529
1529
Thanks that works. Do you have any idea how to ingorate empty ID's to avoid error messages? Or can you skip empty ID's?
– SIB
Nov 16 '18 at 14:18
You check on the error yourself, just don't throw an error.
– Bert Bijn
Nov 16 '18 at 16:20
add a comment |
Thanks that works. Do you have any idea how to ingorate empty ID's to avoid error messages? Or can you skip empty ID's?
– SIB
Nov 16 '18 at 14:18
You check on the error yourself, just don't throw an error.
– Bert Bijn
Nov 16 '18 at 16:20
Thanks that works. Do you have any idea how to ingorate empty ID's to avoid error messages? Or can you skip empty ID's?
– SIB
Nov 16 '18 at 14:18
Thanks that works. Do you have any idea how to ingorate empty ID's to avoid error messages? Or can you skip empty ID's?
– SIB
Nov 16 '18 at 14:18
You check on the error yourself, just don't throw an error.
– Bert Bijn
Nov 16 '18 at 16:20
You check on the error yourself, just don't throw an error.
– Bert Bijn
Nov 16 '18 at 16:20
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53339325%2fphp-loop-curl-with-increase-id-of-url%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
m,jfLQz Dh K18hAV,rFJYRljDX84lZLe,Cl S2hD8,FUjk1 UX3JLLgL3BkYR5,sCk3
you create 10 curl handles and only close 1 of them. that's a resource leak and waste of cpu and ram. you can actually keep re-using the same curl handle and just change CURLOPT_URL~
– hanshenrik
Nov 16 '18 at 23:18