Passing custom props to router component in react-router v4
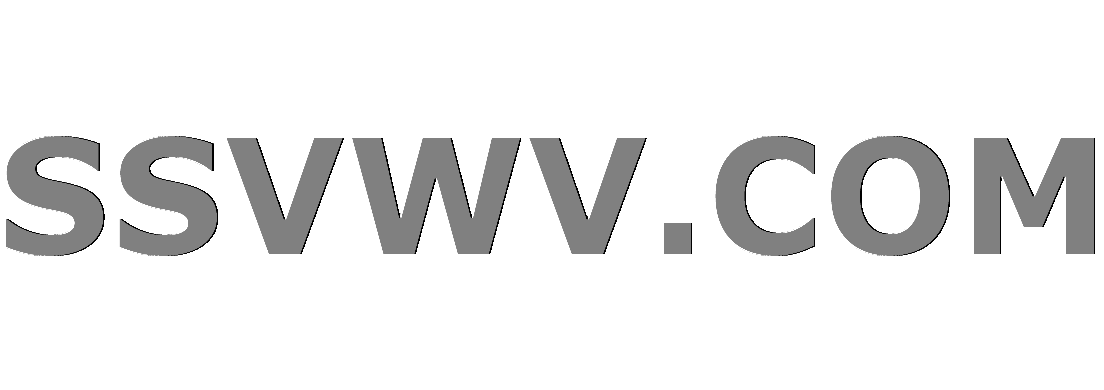
Multi tool use
I'm using React Router to create a multi page app. My main component is <App/>
and it renders all of the routing to to child components. I'm trying to pass props via the route, and based on some research I did, the most common way for child components to tap into props passed down is via the this.props.route
object that they inherit. However, this object is undefined for me. On my render()
function in the child component, I console.log(this.props)
and am return an object that looks like this
{match: Object, location: Object, history: Object, staticContext: undefined}
Doesn't look like the props I expected at all. Here is my code in detail.
Parent Component (I'm trying to pass the word "hi" down as a prop called "test" in all of my child components):
import { BrowserRouter as Router, HashRouter, Route, Switch } from 'react-router-dom';
import Link from 'react-router';
import React from 'react';
import Home from './Home.jsx';
import Nav from './Nav.jsx';
import Progress from './Progress.jsx';
import Test from './Test.jsx';
export default class App extends React.Component {
constructor() {
super();
this._fetchPuzzle = this._fetchPuzzle.bind(this);
}
render() {
return (
<Router>
<div>
<Nav />
<Switch>
<Route path="/" exact test="hi" component={Home} />
<Route path="/progress" test="hi" component={Progress} />
<Route path="/test" test="hi" component={Test} />
<Route render={() => <p>Page not found!</p>} />
</Switch>
</div>
</Router>
);
}
}
Child:
import React from 'react';
const CodeMirror = require('react-codemirror');
import { Link } from 'react-router-dom';
require('codemirror/mode/javascript/javascript')
require('codemirror/mode/xml/xml');
require('codemirror/mode/markdown/markdown');
export default class Home extends React.Component {
constructor(props) {
super(props);
console.log(props)
}
render() {
const options = {
lineNumbers: true,
theme: 'abcdef'
// mode: this.state.mode
};
console.log(this.props)
return (
<div>
<h1>First page bro</h1>
<CodeMirror value='code lol' onChange={()=>'do something'} options={options} />
</div>);
}
}
I'm pretty new to React so my apologies if I'm missing something obvious.
Thanks!
javascript reactjs react-router react-router-v4
|
show 1 more comment
I'm using React Router to create a multi page app. My main component is <App/>
and it renders all of the routing to to child components. I'm trying to pass props via the route, and based on some research I did, the most common way for child components to tap into props passed down is via the this.props.route
object that they inherit. However, this object is undefined for me. On my render()
function in the child component, I console.log(this.props)
and am return an object that looks like this
{match: Object, location: Object, history: Object, staticContext: undefined}
Doesn't look like the props I expected at all. Here is my code in detail.
Parent Component (I'm trying to pass the word "hi" down as a prop called "test" in all of my child components):
import { BrowserRouter as Router, HashRouter, Route, Switch } from 'react-router-dom';
import Link from 'react-router';
import React from 'react';
import Home from './Home.jsx';
import Nav from './Nav.jsx';
import Progress from './Progress.jsx';
import Test from './Test.jsx';
export default class App extends React.Component {
constructor() {
super();
this._fetchPuzzle = this._fetchPuzzle.bind(this);
}
render() {
return (
<Router>
<div>
<Nav />
<Switch>
<Route path="/" exact test="hi" component={Home} />
<Route path="/progress" test="hi" component={Progress} />
<Route path="/test" test="hi" component={Test} />
<Route render={() => <p>Page not found!</p>} />
</Switch>
</div>
</Router>
);
}
}
Child:
import React from 'react';
const CodeMirror = require('react-codemirror');
import { Link } from 'react-router-dom';
require('codemirror/mode/javascript/javascript')
require('codemirror/mode/xml/xml');
require('codemirror/mode/markdown/markdown');
export default class Home extends React.Component {
constructor(props) {
super(props);
console.log(props)
}
render() {
const options = {
lineNumbers: true,
theme: 'abcdef'
// mode: this.state.mode
};
console.log(this.props)
return (
<div>
<h1>First page bro</h1>
<CodeMirror value='code lol' onChange={()=>'do something'} options={options} />
</div>);
}
}
I'm pretty new to React so my apologies if I'm missing something obvious.
Thanks!
javascript reactjs react-router react-router-v4
1
What information do you want to extract from theroute
object? in react-router v4 you should be able to retrieve the same info from eithermatch
orlocation
– Anthony Kong
May 30 '17 at 7:00
@AnthonyKong I'm trying to extract the "test" property.<Route path="/" exact test="hi" component={Home} />
. I was under the impression that the rendered<Home/>
component would have access to an object that held this value. I looked into thematch
andlocation
objects and didn't find my prop.
– Mark Romano
May 30 '17 at 7:04
1
how aboutmatch.params.test
?
– Anthony Kong
May 30 '17 at 7:05
2
Don't know why that value is not present, but alternative of that is, pass that value like this:<Route path="/test" render={(props) => <Test {...props} test="hi"/>} />
and access that bythis.props.test
.
– Mayank Shukla
May 30 '17 at 7:11
1
@MayankShukla Thank you. This delivered. Odd that the value wasn't present using the other technique.
– Mark Romano
May 30 '17 at 7:18
|
show 1 more comment
I'm using React Router to create a multi page app. My main component is <App/>
and it renders all of the routing to to child components. I'm trying to pass props via the route, and based on some research I did, the most common way for child components to tap into props passed down is via the this.props.route
object that they inherit. However, this object is undefined for me. On my render()
function in the child component, I console.log(this.props)
and am return an object that looks like this
{match: Object, location: Object, history: Object, staticContext: undefined}
Doesn't look like the props I expected at all. Here is my code in detail.
Parent Component (I'm trying to pass the word "hi" down as a prop called "test" in all of my child components):
import { BrowserRouter as Router, HashRouter, Route, Switch } from 'react-router-dom';
import Link from 'react-router';
import React from 'react';
import Home from './Home.jsx';
import Nav from './Nav.jsx';
import Progress from './Progress.jsx';
import Test from './Test.jsx';
export default class App extends React.Component {
constructor() {
super();
this._fetchPuzzle = this._fetchPuzzle.bind(this);
}
render() {
return (
<Router>
<div>
<Nav />
<Switch>
<Route path="/" exact test="hi" component={Home} />
<Route path="/progress" test="hi" component={Progress} />
<Route path="/test" test="hi" component={Test} />
<Route render={() => <p>Page not found!</p>} />
</Switch>
</div>
</Router>
);
}
}
Child:
import React from 'react';
const CodeMirror = require('react-codemirror');
import { Link } from 'react-router-dom';
require('codemirror/mode/javascript/javascript')
require('codemirror/mode/xml/xml');
require('codemirror/mode/markdown/markdown');
export default class Home extends React.Component {
constructor(props) {
super(props);
console.log(props)
}
render() {
const options = {
lineNumbers: true,
theme: 'abcdef'
// mode: this.state.mode
};
console.log(this.props)
return (
<div>
<h1>First page bro</h1>
<CodeMirror value='code lol' onChange={()=>'do something'} options={options} />
</div>);
}
}
I'm pretty new to React so my apologies if I'm missing something obvious.
Thanks!
javascript reactjs react-router react-router-v4
I'm using React Router to create a multi page app. My main component is <App/>
and it renders all of the routing to to child components. I'm trying to pass props via the route, and based on some research I did, the most common way for child components to tap into props passed down is via the this.props.route
object that they inherit. However, this object is undefined for me. On my render()
function in the child component, I console.log(this.props)
and am return an object that looks like this
{match: Object, location: Object, history: Object, staticContext: undefined}
Doesn't look like the props I expected at all. Here is my code in detail.
Parent Component (I'm trying to pass the word "hi" down as a prop called "test" in all of my child components):
import { BrowserRouter as Router, HashRouter, Route, Switch } from 'react-router-dom';
import Link from 'react-router';
import React from 'react';
import Home from './Home.jsx';
import Nav from './Nav.jsx';
import Progress from './Progress.jsx';
import Test from './Test.jsx';
export default class App extends React.Component {
constructor() {
super();
this._fetchPuzzle = this._fetchPuzzle.bind(this);
}
render() {
return (
<Router>
<div>
<Nav />
<Switch>
<Route path="/" exact test="hi" component={Home} />
<Route path="/progress" test="hi" component={Progress} />
<Route path="/test" test="hi" component={Test} />
<Route render={() => <p>Page not found!</p>} />
</Switch>
</div>
</Router>
);
}
}
Child:
import React from 'react';
const CodeMirror = require('react-codemirror');
import { Link } from 'react-router-dom';
require('codemirror/mode/javascript/javascript')
require('codemirror/mode/xml/xml');
require('codemirror/mode/markdown/markdown');
export default class Home extends React.Component {
constructor(props) {
super(props);
console.log(props)
}
render() {
const options = {
lineNumbers: true,
theme: 'abcdef'
// mode: this.state.mode
};
console.log(this.props)
return (
<div>
<h1>First page bro</h1>
<CodeMirror value='code lol' onChange={()=>'do something'} options={options} />
</div>);
}
}
I'm pretty new to React so my apologies if I'm missing something obvious.
Thanks!
javascript reactjs react-router react-router-v4
javascript reactjs react-router react-router-v4
edited Nov 3 '17 at 9:18


Shubham Khatri
83.6k15102141
83.6k15102141
asked May 30 '17 at 6:51
Mark RomanoMark Romano
3442420
3442420
1
What information do you want to extract from theroute
object? in react-router v4 you should be able to retrieve the same info from eithermatch
orlocation
– Anthony Kong
May 30 '17 at 7:00
@AnthonyKong I'm trying to extract the "test" property.<Route path="/" exact test="hi" component={Home} />
. I was under the impression that the rendered<Home/>
component would have access to an object that held this value. I looked into thematch
andlocation
objects and didn't find my prop.
– Mark Romano
May 30 '17 at 7:04
1
how aboutmatch.params.test
?
– Anthony Kong
May 30 '17 at 7:05
2
Don't know why that value is not present, but alternative of that is, pass that value like this:<Route path="/test" render={(props) => <Test {...props} test="hi"/>} />
and access that bythis.props.test
.
– Mayank Shukla
May 30 '17 at 7:11
1
@MayankShukla Thank you. This delivered. Odd that the value wasn't present using the other technique.
– Mark Romano
May 30 '17 at 7:18
|
show 1 more comment
1
What information do you want to extract from theroute
object? in react-router v4 you should be able to retrieve the same info from eithermatch
orlocation
– Anthony Kong
May 30 '17 at 7:00
@AnthonyKong I'm trying to extract the "test" property.<Route path="/" exact test="hi" component={Home} />
. I was under the impression that the rendered<Home/>
component would have access to an object that held this value. I looked into thematch
andlocation
objects and didn't find my prop.
– Mark Romano
May 30 '17 at 7:04
1
how aboutmatch.params.test
?
– Anthony Kong
May 30 '17 at 7:05
2
Don't know why that value is not present, but alternative of that is, pass that value like this:<Route path="/test" render={(props) => <Test {...props} test="hi"/>} />
and access that bythis.props.test
.
– Mayank Shukla
May 30 '17 at 7:11
1
@MayankShukla Thank you. This delivered. Odd that the value wasn't present using the other technique.
– Mark Romano
May 30 '17 at 7:18
1
1
What information do you want to extract from the
route
object? in react-router v4 you should be able to retrieve the same info from either match
or location
– Anthony Kong
May 30 '17 at 7:00
What information do you want to extract from the
route
object? in react-router v4 you should be able to retrieve the same info from either match
or location
– Anthony Kong
May 30 '17 at 7:00
@AnthonyKong I'm trying to extract the "test" property.
<Route path="/" exact test="hi" component={Home} />
. I was under the impression that the rendered <Home/>
component would have access to an object that held this value. I looked into the match
and location
objects and didn't find my prop.– Mark Romano
May 30 '17 at 7:04
@AnthonyKong I'm trying to extract the "test" property.
<Route path="/" exact test="hi" component={Home} />
. I was under the impression that the rendered <Home/>
component would have access to an object that held this value. I looked into the match
and location
objects and didn't find my prop.– Mark Romano
May 30 '17 at 7:04
1
1
how about
match.params.test
?– Anthony Kong
May 30 '17 at 7:05
how about
match.params.test
?– Anthony Kong
May 30 '17 at 7:05
2
2
Don't know why that value is not present, but alternative of that is, pass that value like this:
<Route path="/test" render={(props) => <Test {...props} test="hi"/>} />
and access that by this.props.test
.– Mayank Shukla
May 30 '17 at 7:11
Don't know why that value is not present, but alternative of that is, pass that value like this:
<Route path="/test" render={(props) => <Test {...props} test="hi"/>} />
and access that by this.props.test
.– Mayank Shukla
May 30 '17 at 7:11
1
1
@MayankShukla Thank you. This delivered. Odd that the value wasn't present using the other technique.
– Mark Romano
May 30 '17 at 7:18
@MayankShukla Thank you. This delivered. Odd that the value wasn't present using the other technique.
– Mark Romano
May 30 '17 at 7:18
|
show 1 more comment
1 Answer
1
active
oldest
votes
You can pass props to the component by making use of the render
prop to the Route
and thus inlining your component definition. According to the DOCS:
This allows for convenient inline rendering and wrapping without the
undesired remounting explained above.Instead of having a new React
element created for you using thecomponent
prop, you can pass in a
function to be called when thelocation
matches. The render prop
receives all the same route props as the component render prop
So you can pass the prop to component like
<Route path="/" exact render={(props) => (<Home test="hi" {...props}/>)} />
and then you can access it like
this.props.test
in your Home
component
P.S. Also make sure that you are passing
{...props}
so that the
default router props likelocation, history, match etc
are also getting passed on to theHome
component
otherwise the only prop that is getting passed down to it istest
.
This worked thank you. Odd that the method I tried in my question above didn't work.
– Mark Romano
May 30 '17 at 7:19
1
Yeah I had done that analysis in my local setup before answering this, took me some time in that
– Shubham Khatri
May 30 '17 at 7:20
is this the recommended approach? It looks like a quick fix?
– pczern
Aug 27 '17 at 20:50
you should add the props parameter to the function you pass in and use it in the component 'Home' with {...props} otherwise you would lose the standard props react router v4 passes in
– pczern
Aug 27 '17 at 20:59
@speedDeveloper, I don't think its a quick fix, and the router props will be passed if you pass it to the component inrender()
. I missed that part in the answer. However I updated it to include the same
– Shubham Khatri
Aug 28 '17 at 5:25
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f44255415%2fpassing-custom-props-to-router-component-in-react-router-v4%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can pass props to the component by making use of the render
prop to the Route
and thus inlining your component definition. According to the DOCS:
This allows for convenient inline rendering and wrapping without the
undesired remounting explained above.Instead of having a new React
element created for you using thecomponent
prop, you can pass in a
function to be called when thelocation
matches. The render prop
receives all the same route props as the component render prop
So you can pass the prop to component like
<Route path="/" exact render={(props) => (<Home test="hi" {...props}/>)} />
and then you can access it like
this.props.test
in your Home
component
P.S. Also make sure that you are passing
{...props}
so that the
default router props likelocation, history, match etc
are also getting passed on to theHome
component
otherwise the only prop that is getting passed down to it istest
.
This worked thank you. Odd that the method I tried in my question above didn't work.
– Mark Romano
May 30 '17 at 7:19
1
Yeah I had done that analysis in my local setup before answering this, took me some time in that
– Shubham Khatri
May 30 '17 at 7:20
is this the recommended approach? It looks like a quick fix?
– pczern
Aug 27 '17 at 20:50
you should add the props parameter to the function you pass in and use it in the component 'Home' with {...props} otherwise you would lose the standard props react router v4 passes in
– pczern
Aug 27 '17 at 20:59
@speedDeveloper, I don't think its a quick fix, and the router props will be passed if you pass it to the component inrender()
. I missed that part in the answer. However I updated it to include the same
– Shubham Khatri
Aug 28 '17 at 5:25
add a comment |
You can pass props to the component by making use of the render
prop to the Route
and thus inlining your component definition. According to the DOCS:
This allows for convenient inline rendering and wrapping without the
undesired remounting explained above.Instead of having a new React
element created for you using thecomponent
prop, you can pass in a
function to be called when thelocation
matches. The render prop
receives all the same route props as the component render prop
So you can pass the prop to component like
<Route path="/" exact render={(props) => (<Home test="hi" {...props}/>)} />
and then you can access it like
this.props.test
in your Home
component
P.S. Also make sure that you are passing
{...props}
so that the
default router props likelocation, history, match etc
are also getting passed on to theHome
component
otherwise the only prop that is getting passed down to it istest
.
This worked thank you. Odd that the method I tried in my question above didn't work.
– Mark Romano
May 30 '17 at 7:19
1
Yeah I had done that analysis in my local setup before answering this, took me some time in that
– Shubham Khatri
May 30 '17 at 7:20
is this the recommended approach? It looks like a quick fix?
– pczern
Aug 27 '17 at 20:50
you should add the props parameter to the function you pass in and use it in the component 'Home' with {...props} otherwise you would lose the standard props react router v4 passes in
– pczern
Aug 27 '17 at 20:59
@speedDeveloper, I don't think its a quick fix, and the router props will be passed if you pass it to the component inrender()
. I missed that part in the answer. However I updated it to include the same
– Shubham Khatri
Aug 28 '17 at 5:25
add a comment |
You can pass props to the component by making use of the render
prop to the Route
and thus inlining your component definition. According to the DOCS:
This allows for convenient inline rendering and wrapping without the
undesired remounting explained above.Instead of having a new React
element created for you using thecomponent
prop, you can pass in a
function to be called when thelocation
matches. The render prop
receives all the same route props as the component render prop
So you can pass the prop to component like
<Route path="/" exact render={(props) => (<Home test="hi" {...props}/>)} />
and then you can access it like
this.props.test
in your Home
component
P.S. Also make sure that you are passing
{...props}
so that the
default router props likelocation, history, match etc
are also getting passed on to theHome
component
otherwise the only prop that is getting passed down to it istest
.
You can pass props to the component by making use of the render
prop to the Route
and thus inlining your component definition. According to the DOCS:
This allows for convenient inline rendering and wrapping without the
undesired remounting explained above.Instead of having a new React
element created for you using thecomponent
prop, you can pass in a
function to be called when thelocation
matches. The render prop
receives all the same route props as the component render prop
So you can pass the prop to component like
<Route path="/" exact render={(props) => (<Home test="hi" {...props}/>)} />
and then you can access it like
this.props.test
in your Home
component
P.S. Also make sure that you are passing
{...props}
so that the
default router props likelocation, history, match etc
are also getting passed on to theHome
component
otherwise the only prop that is getting passed down to it istest
.
edited Nov 13 '17 at 18:33
answered May 30 '17 at 7:15


Shubham KhatriShubham Khatri
83.6k15102141
83.6k15102141
This worked thank you. Odd that the method I tried in my question above didn't work.
– Mark Romano
May 30 '17 at 7:19
1
Yeah I had done that analysis in my local setup before answering this, took me some time in that
– Shubham Khatri
May 30 '17 at 7:20
is this the recommended approach? It looks like a quick fix?
– pczern
Aug 27 '17 at 20:50
you should add the props parameter to the function you pass in and use it in the component 'Home' with {...props} otherwise you would lose the standard props react router v4 passes in
– pczern
Aug 27 '17 at 20:59
@speedDeveloper, I don't think its a quick fix, and the router props will be passed if you pass it to the component inrender()
. I missed that part in the answer. However I updated it to include the same
– Shubham Khatri
Aug 28 '17 at 5:25
add a comment |
This worked thank you. Odd that the method I tried in my question above didn't work.
– Mark Romano
May 30 '17 at 7:19
1
Yeah I had done that analysis in my local setup before answering this, took me some time in that
– Shubham Khatri
May 30 '17 at 7:20
is this the recommended approach? It looks like a quick fix?
– pczern
Aug 27 '17 at 20:50
you should add the props parameter to the function you pass in and use it in the component 'Home' with {...props} otherwise you would lose the standard props react router v4 passes in
– pczern
Aug 27 '17 at 20:59
@speedDeveloper, I don't think its a quick fix, and the router props will be passed if you pass it to the component inrender()
. I missed that part in the answer. However I updated it to include the same
– Shubham Khatri
Aug 28 '17 at 5:25
This worked thank you. Odd that the method I tried in my question above didn't work.
– Mark Romano
May 30 '17 at 7:19
This worked thank you. Odd that the method I tried in my question above didn't work.
– Mark Romano
May 30 '17 at 7:19
1
1
Yeah I had done that analysis in my local setup before answering this, took me some time in that
– Shubham Khatri
May 30 '17 at 7:20
Yeah I had done that analysis in my local setup before answering this, took me some time in that
– Shubham Khatri
May 30 '17 at 7:20
is this the recommended approach? It looks like a quick fix?
– pczern
Aug 27 '17 at 20:50
is this the recommended approach? It looks like a quick fix?
– pczern
Aug 27 '17 at 20:50
you should add the props parameter to the function you pass in and use it in the component 'Home' with {...props} otherwise you would lose the standard props react router v4 passes in
– pczern
Aug 27 '17 at 20:59
you should add the props parameter to the function you pass in and use it in the component 'Home' with {...props} otherwise you would lose the standard props react router v4 passes in
– pczern
Aug 27 '17 at 20:59
@speedDeveloper, I don't think its a quick fix, and the router props will be passed if you pass it to the component in
render()
. I missed that part in the answer. However I updated it to include the same– Shubham Khatri
Aug 28 '17 at 5:25
@speedDeveloper, I don't think its a quick fix, and the router props will be passed if you pass it to the component in
render()
. I missed that part in the answer. However I updated it to include the same– Shubham Khatri
Aug 28 '17 at 5:25
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f44255415%2fpassing-custom-props-to-router-component-in-react-router-v4%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VP4rI695LH1UC mg xdm fPUpVNXU keYhd,XfkUM8L7 5
1
What information do you want to extract from the
route
object? in react-router v4 you should be able to retrieve the same info from eithermatch
orlocation
– Anthony Kong
May 30 '17 at 7:00
@AnthonyKong I'm trying to extract the "test" property.
<Route path="/" exact test="hi" component={Home} />
. I was under the impression that the rendered<Home/>
component would have access to an object that held this value. I looked into thematch
andlocation
objects and didn't find my prop.– Mark Romano
May 30 '17 at 7:04
1
how about
match.params.test
?– Anthony Kong
May 30 '17 at 7:05
2
Don't know why that value is not present, but alternative of that is, pass that value like this:
<Route path="/test" render={(props) => <Test {...props} test="hi"/>} />
and access that bythis.props.test
.– Mayank Shukla
May 30 '17 at 7:11
1
@MayankShukla Thank you. This delivered. Odd that the value wasn't present using the other technique.
– Mark Romano
May 30 '17 at 7:18