SQLAlchemy: insert record if certain record and relationship data does not already exist
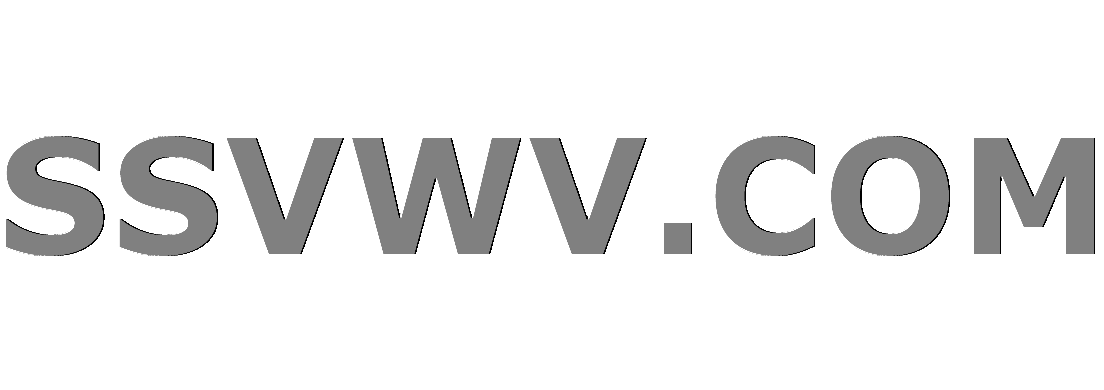
Multi tool use
up vote
1
down vote
favorite
I want to know how to query data from multiple tables with multiple conditions.
My example db has the following tables:
class Location(Base):
__tablename__ = "location"
id = Column('id', Integer, primary_key=True)
location = Column('Location', String)
class Person(Base):
__tablename__ = "person"
id = Column('id', Integer, primary_key=True)
name = Column('Name', String, unique=True)
profession = Column('Profession', String)
location_id = Column(Integer, ForeignKey('location.id'))
location = relationship(Location)
We have in this database a person with a specific location. My goal is to write a query where I can check conditions of the Location
table and the Person
table.
A person with the name Eric lives in Houston. Now I want to know if I already have an Eric from Houston in my database.
The following query doesn't work.
new_location = Location(location='Houston')
obj = Person(name='Eric', profession='Teacher', location=new_location)
if session.query(Person).filter(Person.name == obj.name,
Person.profession == obj.profession,
Person.location_id == obj.location.id).first() == None:
session.add(obj)
session.commit()
print("Insert sucessful")
The problem in my query is the last line where I check the location but I don't know how to solve it. Maybe someone has a working example with the SQLAlchemy method exists()
?
python python-3.x sqlalchemy filtering exists
add a comment |
up vote
1
down vote
favorite
I want to know how to query data from multiple tables with multiple conditions.
My example db has the following tables:
class Location(Base):
__tablename__ = "location"
id = Column('id', Integer, primary_key=True)
location = Column('Location', String)
class Person(Base):
__tablename__ = "person"
id = Column('id', Integer, primary_key=True)
name = Column('Name', String, unique=True)
profession = Column('Profession', String)
location_id = Column(Integer, ForeignKey('location.id'))
location = relationship(Location)
We have in this database a person with a specific location. My goal is to write a query where I can check conditions of the Location
table and the Person
table.
A person with the name Eric lives in Houston. Now I want to know if I already have an Eric from Houston in my database.
The following query doesn't work.
new_location = Location(location='Houston')
obj = Person(name='Eric', profession='Teacher', location=new_location)
if session.query(Person).filter(Person.name == obj.name,
Person.profession == obj.profession,
Person.location_id == obj.location.id).first() == None:
session.add(obj)
session.commit()
print("Insert sucessful")
The problem in my query is the last line where I check the location but I don't know how to solve it. Maybe someone has a working example with the SQLAlchemy method exists()
?
python python-3.x sqlalchemy filtering exists
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I want to know how to query data from multiple tables with multiple conditions.
My example db has the following tables:
class Location(Base):
__tablename__ = "location"
id = Column('id', Integer, primary_key=True)
location = Column('Location', String)
class Person(Base):
__tablename__ = "person"
id = Column('id', Integer, primary_key=True)
name = Column('Name', String, unique=True)
profession = Column('Profession', String)
location_id = Column(Integer, ForeignKey('location.id'))
location = relationship(Location)
We have in this database a person with a specific location. My goal is to write a query where I can check conditions of the Location
table and the Person
table.
A person with the name Eric lives in Houston. Now I want to know if I already have an Eric from Houston in my database.
The following query doesn't work.
new_location = Location(location='Houston')
obj = Person(name='Eric', profession='Teacher', location=new_location)
if session.query(Person).filter(Person.name == obj.name,
Person.profession == obj.profession,
Person.location_id == obj.location.id).first() == None:
session.add(obj)
session.commit()
print("Insert sucessful")
The problem in my query is the last line where I check the location but I don't know how to solve it. Maybe someone has a working example with the SQLAlchemy method exists()
?
python python-3.x sqlalchemy filtering exists
I want to know how to query data from multiple tables with multiple conditions.
My example db has the following tables:
class Location(Base):
__tablename__ = "location"
id = Column('id', Integer, primary_key=True)
location = Column('Location', String)
class Person(Base):
__tablename__ = "person"
id = Column('id', Integer, primary_key=True)
name = Column('Name', String, unique=True)
profession = Column('Profession', String)
location_id = Column(Integer, ForeignKey('location.id'))
location = relationship(Location)
We have in this database a person with a specific location. My goal is to write a query where I can check conditions of the Location
table and the Person
table.
A person with the name Eric lives in Houston. Now I want to know if I already have an Eric from Houston in my database.
The following query doesn't work.
new_location = Location(location='Houston')
obj = Person(name='Eric', profession='Teacher', location=new_location)
if session.query(Person).filter(Person.name == obj.name,
Person.profession == obj.profession,
Person.location_id == obj.location.id).first() == None:
session.add(obj)
session.commit()
print("Insert sucessful")
The problem in my query is the last line where I check the location but I don't know how to solve it. Maybe someone has a working example with the SQLAlchemy method exists()
?
python python-3.x sqlalchemy filtering exists
python python-3.x sqlalchemy filtering exists
edited Nov 12 at 15:08
benvc
3,5921319
3,5921319
asked Nov 12 at 10:51
Neal Mc Beal
297
297
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
You can do something like the following to join Person
and Location
and filter for any record where the name and location are the same as the new person instance you have created. The query will either return the record or None
, so you can use the result in your if
(remember that indentation matters - maybe the code example in your question just copied incorrectly).
new_location = Location(location='Houston')
new_person = Person(name='Eric', profession='Teacher', location=houston)
person_location_exists = session.query(Person).
join(Location).
filter(Person.name == new_person.name).
filter(Location.location == new_location.location).
first()
if person_location_exists:
session.add(new_person)
session.commit()
print("Insert sucessful")
You could use exists()
to accomplish the same thing, but I think the above is a bit simpler.
Hey ty for your answer! I found a very similar solution but your solution is much better :)
– Neal Mc Beal
Nov 15 at 10:44
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53260586%2fsqlalchemy-insert-record-if-certain-record-and-relationship-data-does-not-alrea%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
You can do something like the following to join Person
and Location
and filter for any record where the name and location are the same as the new person instance you have created. The query will either return the record or None
, so you can use the result in your if
(remember that indentation matters - maybe the code example in your question just copied incorrectly).
new_location = Location(location='Houston')
new_person = Person(name='Eric', profession='Teacher', location=houston)
person_location_exists = session.query(Person).
join(Location).
filter(Person.name == new_person.name).
filter(Location.location == new_location.location).
first()
if person_location_exists:
session.add(new_person)
session.commit()
print("Insert sucessful")
You could use exists()
to accomplish the same thing, but I think the above is a bit simpler.
Hey ty for your answer! I found a very similar solution but your solution is much better :)
– Neal Mc Beal
Nov 15 at 10:44
add a comment |
up vote
1
down vote
accepted
You can do something like the following to join Person
and Location
and filter for any record where the name and location are the same as the new person instance you have created. The query will either return the record or None
, so you can use the result in your if
(remember that indentation matters - maybe the code example in your question just copied incorrectly).
new_location = Location(location='Houston')
new_person = Person(name='Eric', profession='Teacher', location=houston)
person_location_exists = session.query(Person).
join(Location).
filter(Person.name == new_person.name).
filter(Location.location == new_location.location).
first()
if person_location_exists:
session.add(new_person)
session.commit()
print("Insert sucessful")
You could use exists()
to accomplish the same thing, but I think the above is a bit simpler.
Hey ty for your answer! I found a very similar solution but your solution is much better :)
– Neal Mc Beal
Nov 15 at 10:44
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
You can do something like the following to join Person
and Location
and filter for any record where the name and location are the same as the new person instance you have created. The query will either return the record or None
, so you can use the result in your if
(remember that indentation matters - maybe the code example in your question just copied incorrectly).
new_location = Location(location='Houston')
new_person = Person(name='Eric', profession='Teacher', location=houston)
person_location_exists = session.query(Person).
join(Location).
filter(Person.name == new_person.name).
filter(Location.location == new_location.location).
first()
if person_location_exists:
session.add(new_person)
session.commit()
print("Insert sucessful")
You could use exists()
to accomplish the same thing, but I think the above is a bit simpler.
You can do something like the following to join Person
and Location
and filter for any record where the name and location are the same as the new person instance you have created. The query will either return the record or None
, so you can use the result in your if
(remember that indentation matters - maybe the code example in your question just copied incorrectly).
new_location = Location(location='Houston')
new_person = Person(name='Eric', profession='Teacher', location=houston)
person_location_exists = session.query(Person).
join(Location).
filter(Person.name == new_person.name).
filter(Location.location == new_location.location).
first()
if person_location_exists:
session.add(new_person)
session.commit()
print("Insert sucessful")
You could use exists()
to accomplish the same thing, but I think the above is a bit simpler.
edited Nov 12 at 15:09
answered Nov 12 at 14:55
benvc
3,5921319
3,5921319
Hey ty for your answer! I found a very similar solution but your solution is much better :)
– Neal Mc Beal
Nov 15 at 10:44
add a comment |
Hey ty for your answer! I found a very similar solution but your solution is much better :)
– Neal Mc Beal
Nov 15 at 10:44
Hey ty for your answer! I found a very similar solution but your solution is much better :)
– Neal Mc Beal
Nov 15 at 10:44
Hey ty for your answer! I found a very similar solution but your solution is much better :)
– Neal Mc Beal
Nov 15 at 10:44
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53260586%2fsqlalchemy-insert-record-if-certain-record-and-relationship-data-does-not-alrea%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vem,hV0O4HV,Wm9J9mD,4o,kOgDvF58VIqVjWHkPpjk 2oN,2