How to find the value inside an array with objects with the most occuring value (deep mode)?
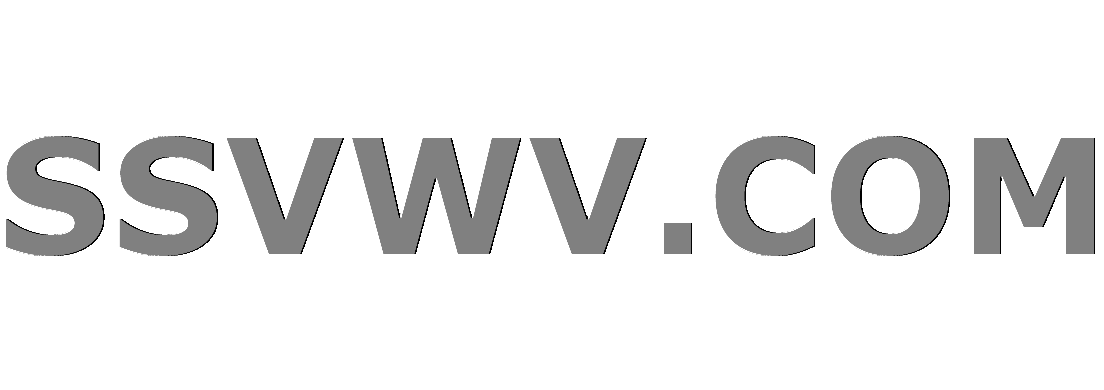
Multi tool use
up vote
0
down vote
favorite
Let's say I have an array with objects like this:
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
How do I find the value based on a key with the most occuring value?
In this example, when passing name
as key that would be Erik
.
Something like this:
const deepMode = (array, key) => {
// Perhaps something with .reduce?
}
And when called, it should return:
deepMode(persons, "name"); // Returns "Erik"
javascript
|
show 2 more comments
up vote
0
down vote
favorite
Let's say I have an array with objects like this:
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
How do I find the value based on a key with the most occuring value?
In this example, when passing name
as key that would be Erik
.
Something like this:
const deepMode = (array, key) => {
// Perhaps something with .reduce?
}
And when called, it should return:
deepMode(persons, "name"); // Returns "Erik"
javascript
1
Exactly what makes the "Bob" object the one with most occurring value?
– amn
Nov 12 at 10:47
1
please explain what should look like your desired output
– Artyom Amiryan
Nov 12 at 10:47
@artyom I explained that, according to this example, when passing name as key, that would be "Erik", I'll update it in the code to make it more clearer
– Fabian Tjoe A On
Nov 12 at 10:48
1
@amn Oh no I'm sorry! It should be Erik. I updated my question!
– Fabian Tjoe A On
Nov 12 at 10:49
so what if key passed for example as 'Jasper' ?
– Artyom Amiryan
Nov 12 at 10:50
|
show 2 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Let's say I have an array with objects like this:
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
How do I find the value based on a key with the most occuring value?
In this example, when passing name
as key that would be Erik
.
Something like this:
const deepMode = (array, key) => {
// Perhaps something with .reduce?
}
And when called, it should return:
deepMode(persons, "name"); // Returns "Erik"
javascript
Let's say I have an array with objects like this:
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
How do I find the value based on a key with the most occuring value?
In this example, when passing name
as key that would be Erik
.
Something like this:
const deepMode = (array, key) => {
// Perhaps something with .reduce?
}
And when called, it should return:
deepMode(persons, "name"); // Returns "Erik"
javascript
javascript
edited Nov 12 at 10:51
asked Nov 12 at 10:45


Fabian Tjoe A On
593721
593721
1
Exactly what makes the "Bob" object the one with most occurring value?
– amn
Nov 12 at 10:47
1
please explain what should look like your desired output
– Artyom Amiryan
Nov 12 at 10:47
@artyom I explained that, according to this example, when passing name as key, that would be "Erik", I'll update it in the code to make it more clearer
– Fabian Tjoe A On
Nov 12 at 10:48
1
@amn Oh no I'm sorry! It should be Erik. I updated my question!
– Fabian Tjoe A On
Nov 12 at 10:49
so what if key passed for example as 'Jasper' ?
– Artyom Amiryan
Nov 12 at 10:50
|
show 2 more comments
1
Exactly what makes the "Bob" object the one with most occurring value?
– amn
Nov 12 at 10:47
1
please explain what should look like your desired output
– Artyom Amiryan
Nov 12 at 10:47
@artyom I explained that, according to this example, when passing name as key, that would be "Erik", I'll update it in the code to make it more clearer
– Fabian Tjoe A On
Nov 12 at 10:48
1
@amn Oh no I'm sorry! It should be Erik. I updated my question!
– Fabian Tjoe A On
Nov 12 at 10:49
so what if key passed for example as 'Jasper' ?
– Artyom Amiryan
Nov 12 at 10:50
1
1
Exactly what makes the "Bob" object the one with most occurring value?
– amn
Nov 12 at 10:47
Exactly what makes the "Bob" object the one with most occurring value?
– amn
Nov 12 at 10:47
1
1
please explain what should look like your desired output
– Artyom Amiryan
Nov 12 at 10:47
please explain what should look like your desired output
– Artyom Amiryan
Nov 12 at 10:47
@artyom I explained that, according to this example, when passing name as key, that would be "Erik", I'll update it in the code to make it more clearer
– Fabian Tjoe A On
Nov 12 at 10:48
@artyom I explained that, according to this example, when passing name as key, that would be "Erik", I'll update it in the code to make it more clearer
– Fabian Tjoe A On
Nov 12 at 10:48
1
1
@amn Oh no I'm sorry! It should be Erik. I updated my question!
– Fabian Tjoe A On
Nov 12 at 10:49
@amn Oh no I'm sorry! It should be Erik. I updated my question!
– Fabian Tjoe A On
Nov 12 at 10:49
so what if key passed for example as 'Jasper' ?
– Artyom Amiryan
Nov 12 at 10:50
so what if key passed for example as 'Jasper' ?
– Artyom Amiryan
Nov 12 at 10:50
|
show 2 more comments
3 Answers
3
active
oldest
votes
up vote
1
down vote
accepted
you can count keys by adding them in object and checking if key exists in object,then increment value, if not then add key into object, after that with Object.keys
get keys of object sort them and get first element which is most occurring
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
const deepMode = (array, key) => {
const obj = {};
array.forEach(v => {
if (obj[v[key]]) {
obj[v[key]] += 1;
} else {
obj[v[key]] = 1;
}
});
return Object.keys(obj).sort((a,b) => obj[b]-obj[a])[0];
}
console.log(deepMode(persons, 'name'));
Cool! This is very readable, I benchmarked it with the other answers and it's just as performant, so I went for this one in the end :)
– Fabian Tjoe A On
Nov 12 at 12:11
@FabianTjoeAOn glad to help, if you chose this one, then please mark as accepted answer
– Artyom Amiryan
Nov 12 at 12:16
add a comment |
up vote
2
down vote
You could take a Map
, count the ocurrences and reduce the key/value pairs for getting the max valaue. Return the key without iterating again.
const
deepMode = (array, key) => Array
.from(array.reduce((m, o) => m.set(o[key], (m.get(o[key]) || 0) + 1), new Map))
.reduce((a, b) => a[1] > b[1] ? a : b)[0],
persons = [{ name: "Erik", age: 45 }, { name: "Bob", age: 37 }, { name: "Erik", age: 28 }, { name: "Jasper", age: 29 }, { name: "Erik", age: 34 }];
console.log(deepMode(persons, 'name'));
add a comment |
up vote
1
down vote
You could reduce into a Map, find the max, then find and return it.
function findMostOccuringKeyValue(arr, key) {
const grouped = arr.reduce((a, b) => a.set(b[key], (a.get(b[key]) || 0) + 1), new Map);
const max = Math.max(...grouped.values());
return [...grouped].find(([k, v]) => v === max)[0];
}
console.log(findMostOccuringKeyValue(persons, 'name'));
<script>
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
</script>
Awesome! Just what I'm looking for. Would you also know how to return the full object instead of just the string?
– Fabian Tjoe A On
Nov 12 at 11:00
What do you mean with full object? The resulting count too? @FabianTjoeAOn
– bambam
Nov 12 at 11:01
Oh no never mind, I thought about returning the full object (like{name: "Erik", age: 45}
, but then all keys of that object must be the same.
– Fabian Tjoe A On
Nov 12 at 11:05
Hm, you could filter the array by the matches maybe,function findMatches(arr, value, key) { return arr.filter(e => e[key] === value); } const n = findMostOccuringKeyValue(persons, 'name'); console.log(findMatches(persons, n, 'name'));
– bambam
Nov 12 at 11:08
Yep, already got it using array.find()
, thanks :)
– Fabian Tjoe A On
Nov 12 at 11:12
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53260512%2fhow-to-find-the-value-inside-an-array-with-objects-with-the-most-occuring-value%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
you can count keys by adding them in object and checking if key exists in object,then increment value, if not then add key into object, after that with Object.keys
get keys of object sort them and get first element which is most occurring
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
const deepMode = (array, key) => {
const obj = {};
array.forEach(v => {
if (obj[v[key]]) {
obj[v[key]] += 1;
} else {
obj[v[key]] = 1;
}
});
return Object.keys(obj).sort((a,b) => obj[b]-obj[a])[0];
}
console.log(deepMode(persons, 'name'));
Cool! This is very readable, I benchmarked it with the other answers and it's just as performant, so I went for this one in the end :)
– Fabian Tjoe A On
Nov 12 at 12:11
@FabianTjoeAOn glad to help, if you chose this one, then please mark as accepted answer
– Artyom Amiryan
Nov 12 at 12:16
add a comment |
up vote
1
down vote
accepted
you can count keys by adding them in object and checking if key exists in object,then increment value, if not then add key into object, after that with Object.keys
get keys of object sort them and get first element which is most occurring
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
const deepMode = (array, key) => {
const obj = {};
array.forEach(v => {
if (obj[v[key]]) {
obj[v[key]] += 1;
} else {
obj[v[key]] = 1;
}
});
return Object.keys(obj).sort((a,b) => obj[b]-obj[a])[0];
}
console.log(deepMode(persons, 'name'));
Cool! This is very readable, I benchmarked it with the other answers and it's just as performant, so I went for this one in the end :)
– Fabian Tjoe A On
Nov 12 at 12:11
@FabianTjoeAOn glad to help, if you chose this one, then please mark as accepted answer
– Artyom Amiryan
Nov 12 at 12:16
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
you can count keys by adding them in object and checking if key exists in object,then increment value, if not then add key into object, after that with Object.keys
get keys of object sort them and get first element which is most occurring
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
const deepMode = (array, key) => {
const obj = {};
array.forEach(v => {
if (obj[v[key]]) {
obj[v[key]] += 1;
} else {
obj[v[key]] = 1;
}
});
return Object.keys(obj).sort((a,b) => obj[b]-obj[a])[0];
}
console.log(deepMode(persons, 'name'));
you can count keys by adding them in object and checking if key exists in object,then increment value, if not then add key into object, after that with Object.keys
get keys of object sort them and get first element which is most occurring
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
const deepMode = (array, key) => {
const obj = {};
array.forEach(v => {
if (obj[v[key]]) {
obj[v[key]] += 1;
} else {
obj[v[key]] = 1;
}
});
return Object.keys(obj).sort((a,b) => obj[b]-obj[a])[0];
}
console.log(deepMode(persons, 'name'));
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
const deepMode = (array, key) => {
const obj = {};
array.forEach(v => {
if (obj[v[key]]) {
obj[v[key]] += 1;
} else {
obj[v[key]] = 1;
}
});
return Object.keys(obj).sort((a,b) => obj[b]-obj[a])[0];
}
console.log(deepMode(persons, 'name'));
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
const deepMode = (array, key) => {
const obj = {};
array.forEach(v => {
if (obj[v[key]]) {
obj[v[key]] += 1;
} else {
obj[v[key]] = 1;
}
});
return Object.keys(obj).sort((a,b) => obj[b]-obj[a])[0];
}
console.log(deepMode(persons, 'name'));
answered Nov 12 at 11:01


Artyom Amiryan
1,725113
1,725113
Cool! This is very readable, I benchmarked it with the other answers and it's just as performant, so I went for this one in the end :)
– Fabian Tjoe A On
Nov 12 at 12:11
@FabianTjoeAOn glad to help, if you chose this one, then please mark as accepted answer
– Artyom Amiryan
Nov 12 at 12:16
add a comment |
Cool! This is very readable, I benchmarked it with the other answers and it's just as performant, so I went for this one in the end :)
– Fabian Tjoe A On
Nov 12 at 12:11
@FabianTjoeAOn glad to help, if you chose this one, then please mark as accepted answer
– Artyom Amiryan
Nov 12 at 12:16
Cool! This is very readable, I benchmarked it with the other answers and it's just as performant, so I went for this one in the end :)
– Fabian Tjoe A On
Nov 12 at 12:11
Cool! This is very readable, I benchmarked it with the other answers and it's just as performant, so I went for this one in the end :)
– Fabian Tjoe A On
Nov 12 at 12:11
@FabianTjoeAOn glad to help, if you chose this one, then please mark as accepted answer
– Artyom Amiryan
Nov 12 at 12:16
@FabianTjoeAOn glad to help, if you chose this one, then please mark as accepted answer
– Artyom Amiryan
Nov 12 at 12:16
add a comment |
up vote
2
down vote
You could take a Map
, count the ocurrences and reduce the key/value pairs for getting the max valaue. Return the key without iterating again.
const
deepMode = (array, key) => Array
.from(array.reduce((m, o) => m.set(o[key], (m.get(o[key]) || 0) + 1), new Map))
.reduce((a, b) => a[1] > b[1] ? a : b)[0],
persons = [{ name: "Erik", age: 45 }, { name: "Bob", age: 37 }, { name: "Erik", age: 28 }, { name: "Jasper", age: 29 }, { name: "Erik", age: 34 }];
console.log(deepMode(persons, 'name'));
add a comment |
up vote
2
down vote
You could take a Map
, count the ocurrences and reduce the key/value pairs for getting the max valaue. Return the key without iterating again.
const
deepMode = (array, key) => Array
.from(array.reduce((m, o) => m.set(o[key], (m.get(o[key]) || 0) + 1), new Map))
.reduce((a, b) => a[1] > b[1] ? a : b)[0],
persons = [{ name: "Erik", age: 45 }, { name: "Bob", age: 37 }, { name: "Erik", age: 28 }, { name: "Jasper", age: 29 }, { name: "Erik", age: 34 }];
console.log(deepMode(persons, 'name'));
add a comment |
up vote
2
down vote
up vote
2
down vote
You could take a Map
, count the ocurrences and reduce the key/value pairs for getting the max valaue. Return the key without iterating again.
const
deepMode = (array, key) => Array
.from(array.reduce((m, o) => m.set(o[key], (m.get(o[key]) || 0) + 1), new Map))
.reduce((a, b) => a[1] > b[1] ? a : b)[0],
persons = [{ name: "Erik", age: 45 }, { name: "Bob", age: 37 }, { name: "Erik", age: 28 }, { name: "Jasper", age: 29 }, { name: "Erik", age: 34 }];
console.log(deepMode(persons, 'name'));
You could take a Map
, count the ocurrences and reduce the key/value pairs for getting the max valaue. Return the key without iterating again.
const
deepMode = (array, key) => Array
.from(array.reduce((m, o) => m.set(o[key], (m.get(o[key]) || 0) + 1), new Map))
.reduce((a, b) => a[1] > b[1] ? a : b)[0],
persons = [{ name: "Erik", age: 45 }, { name: "Bob", age: 37 }, { name: "Erik", age: 28 }, { name: "Jasper", age: 29 }, { name: "Erik", age: 34 }];
console.log(deepMode(persons, 'name'));
const
deepMode = (array, key) => Array
.from(array.reduce((m, o) => m.set(o[key], (m.get(o[key]) || 0) + 1), new Map))
.reduce((a, b) => a[1] > b[1] ? a : b)[0],
persons = [{ name: "Erik", age: 45 }, { name: "Bob", age: 37 }, { name: "Erik", age: 28 }, { name: "Jasper", age: 29 }, { name: "Erik", age: 34 }];
console.log(deepMode(persons, 'name'));
const
deepMode = (array, key) => Array
.from(array.reduce((m, o) => m.set(o[key], (m.get(o[key]) || 0) + 1), new Map))
.reduce((a, b) => a[1] > b[1] ? a : b)[0],
persons = [{ name: "Erik", age: 45 }, { name: "Bob", age: 37 }, { name: "Erik", age: 28 }, { name: "Jasper", age: 29 }, { name: "Erik", age: 34 }];
console.log(deepMode(persons, 'name'));
answered Nov 12 at 11:07


Nina Scholz
174k1387151
174k1387151
add a comment |
add a comment |
up vote
1
down vote
You could reduce into a Map, find the max, then find and return it.
function findMostOccuringKeyValue(arr, key) {
const grouped = arr.reduce((a, b) => a.set(b[key], (a.get(b[key]) || 0) + 1), new Map);
const max = Math.max(...grouped.values());
return [...grouped].find(([k, v]) => v === max)[0];
}
console.log(findMostOccuringKeyValue(persons, 'name'));
<script>
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
</script>
Awesome! Just what I'm looking for. Would you also know how to return the full object instead of just the string?
– Fabian Tjoe A On
Nov 12 at 11:00
What do you mean with full object? The resulting count too? @FabianTjoeAOn
– bambam
Nov 12 at 11:01
Oh no never mind, I thought about returning the full object (like{name: "Erik", age: 45}
, but then all keys of that object must be the same.
– Fabian Tjoe A On
Nov 12 at 11:05
Hm, you could filter the array by the matches maybe,function findMatches(arr, value, key) { return arr.filter(e => e[key] === value); } const n = findMostOccuringKeyValue(persons, 'name'); console.log(findMatches(persons, n, 'name'));
– bambam
Nov 12 at 11:08
Yep, already got it using array.find()
, thanks :)
– Fabian Tjoe A On
Nov 12 at 11:12
add a comment |
up vote
1
down vote
You could reduce into a Map, find the max, then find and return it.
function findMostOccuringKeyValue(arr, key) {
const grouped = arr.reduce((a, b) => a.set(b[key], (a.get(b[key]) || 0) + 1), new Map);
const max = Math.max(...grouped.values());
return [...grouped].find(([k, v]) => v === max)[0];
}
console.log(findMostOccuringKeyValue(persons, 'name'));
<script>
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
</script>
Awesome! Just what I'm looking for. Would you also know how to return the full object instead of just the string?
– Fabian Tjoe A On
Nov 12 at 11:00
What do you mean with full object? The resulting count too? @FabianTjoeAOn
– bambam
Nov 12 at 11:01
Oh no never mind, I thought about returning the full object (like{name: "Erik", age: 45}
, but then all keys of that object must be the same.
– Fabian Tjoe A On
Nov 12 at 11:05
Hm, you could filter the array by the matches maybe,function findMatches(arr, value, key) { return arr.filter(e => e[key] === value); } const n = findMostOccuringKeyValue(persons, 'name'); console.log(findMatches(persons, n, 'name'));
– bambam
Nov 12 at 11:08
Yep, already got it using array.find()
, thanks :)
– Fabian Tjoe A On
Nov 12 at 11:12
add a comment |
up vote
1
down vote
up vote
1
down vote
You could reduce into a Map, find the max, then find and return it.
function findMostOccuringKeyValue(arr, key) {
const grouped = arr.reduce((a, b) => a.set(b[key], (a.get(b[key]) || 0) + 1), new Map);
const max = Math.max(...grouped.values());
return [...grouped].find(([k, v]) => v === max)[0];
}
console.log(findMostOccuringKeyValue(persons, 'name'));
<script>
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
</script>
You could reduce into a Map, find the max, then find and return it.
function findMostOccuringKeyValue(arr, key) {
const grouped = arr.reduce((a, b) => a.set(b[key], (a.get(b[key]) || 0) + 1), new Map);
const max = Math.max(...grouped.values());
return [...grouped].find(([k, v]) => v === max)[0];
}
console.log(findMostOccuringKeyValue(persons, 'name'));
<script>
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
</script>
function findMostOccuringKeyValue(arr, key) {
const grouped = arr.reduce((a, b) => a.set(b[key], (a.get(b[key]) || 0) + 1), new Map);
const max = Math.max(...grouped.values());
return [...grouped].find(([k, v]) => v === max)[0];
}
console.log(findMostOccuringKeyValue(persons, 'name'));
<script>
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
</script>
function findMostOccuringKeyValue(arr, key) {
const grouped = arr.reduce((a, b) => a.set(b[key], (a.get(b[key]) || 0) + 1), new Map);
const max = Math.max(...grouped.values());
return [...grouped].find(([k, v]) => v === max)[0];
}
console.log(findMostOccuringKeyValue(persons, 'name'));
<script>
const persons = [
{
name: "Erik",
age: 45
},
{
name: "Bob",
age: 37
},
{
name: "Erik",
age: 28
},
{
name: "Jasper",
age: 29
},
{
name: "Erik",
age: 34
}
];
</script>
answered Nov 12 at 10:53
bambam
44.3k872114
44.3k872114
Awesome! Just what I'm looking for. Would you also know how to return the full object instead of just the string?
– Fabian Tjoe A On
Nov 12 at 11:00
What do you mean with full object? The resulting count too? @FabianTjoeAOn
– bambam
Nov 12 at 11:01
Oh no never mind, I thought about returning the full object (like{name: "Erik", age: 45}
, but then all keys of that object must be the same.
– Fabian Tjoe A On
Nov 12 at 11:05
Hm, you could filter the array by the matches maybe,function findMatches(arr, value, key) { return arr.filter(e => e[key] === value); } const n = findMostOccuringKeyValue(persons, 'name'); console.log(findMatches(persons, n, 'name'));
– bambam
Nov 12 at 11:08
Yep, already got it using array.find()
, thanks :)
– Fabian Tjoe A On
Nov 12 at 11:12
add a comment |
Awesome! Just what I'm looking for. Would you also know how to return the full object instead of just the string?
– Fabian Tjoe A On
Nov 12 at 11:00
What do you mean with full object? The resulting count too? @FabianTjoeAOn
– bambam
Nov 12 at 11:01
Oh no never mind, I thought about returning the full object (like{name: "Erik", age: 45}
, but then all keys of that object must be the same.
– Fabian Tjoe A On
Nov 12 at 11:05
Hm, you could filter the array by the matches maybe,function findMatches(arr, value, key) { return arr.filter(e => e[key] === value); } const n = findMostOccuringKeyValue(persons, 'name'); console.log(findMatches(persons, n, 'name'));
– bambam
Nov 12 at 11:08
Yep, already got it using array.find()
, thanks :)
– Fabian Tjoe A On
Nov 12 at 11:12
Awesome! Just what I'm looking for. Would you also know how to return the full object instead of just the string?
– Fabian Tjoe A On
Nov 12 at 11:00
Awesome! Just what I'm looking for. Would you also know how to return the full object instead of just the string?
– Fabian Tjoe A On
Nov 12 at 11:00
What do you mean with full object? The resulting count too? @FabianTjoeAOn
– bambam
Nov 12 at 11:01
What do you mean with full object? The resulting count too? @FabianTjoeAOn
– bambam
Nov 12 at 11:01
Oh no never mind, I thought about returning the full object (like
{name: "Erik", age: 45}
, but then all keys of that object must be the same.– Fabian Tjoe A On
Nov 12 at 11:05
Oh no never mind, I thought about returning the full object (like
{name: "Erik", age: 45}
, but then all keys of that object must be the same.– Fabian Tjoe A On
Nov 12 at 11:05
Hm, you could filter the array by the matches maybe,
function findMatches(arr, value, key) { return arr.filter(e => e[key] === value); } const n = findMostOccuringKeyValue(persons, 'name'); console.log(findMatches(persons, n, 'name'));
– bambam
Nov 12 at 11:08
Hm, you could filter the array by the matches maybe,
function findMatches(arr, value, key) { return arr.filter(e => e[key] === value); } const n = findMostOccuringKeyValue(persons, 'name'); console.log(findMatches(persons, n, 'name'));
– bambam
Nov 12 at 11:08
Yep, already got it using array
.find()
, thanks :)– Fabian Tjoe A On
Nov 12 at 11:12
Yep, already got it using array
.find()
, thanks :)– Fabian Tjoe A On
Nov 12 at 11:12
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53260512%2fhow-to-find-the-value-inside-an-array-with-objects-with-the-most-occuring-value%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
wZ0bnPMksI27SB7BxaIax1,sQffAJYVeAjx6zHhU NU5nA1mehFxwtvwD9WMjC,xZe3rPW0U,nMVBO5brUeBXkig je,zyujn
1
Exactly what makes the "Bob" object the one with most occurring value?
– amn
Nov 12 at 10:47
1
please explain what should look like your desired output
– Artyom Amiryan
Nov 12 at 10:47
@artyom I explained that, according to this example, when passing name as key, that would be "Erik", I'll update it in the code to make it more clearer
– Fabian Tjoe A On
Nov 12 at 10:48
1
@amn Oh no I'm sorry! It should be Erik. I updated my question!
– Fabian Tjoe A On
Nov 12 at 10:49
so what if key passed for example as 'Jasper' ?
– Artyom Amiryan
Nov 12 at 10:50