Java InputStream Crypting
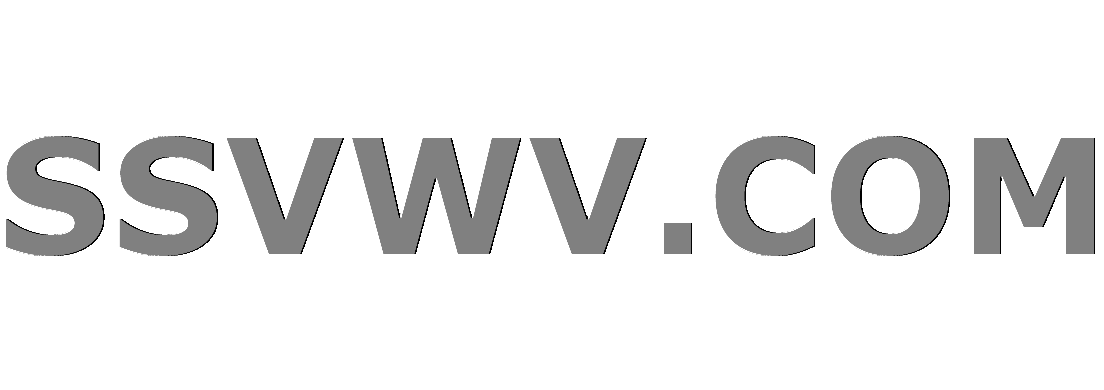
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I'm making a simple project where a client encrypt a file with JCE (DES) and sends it to the server with sockets. The server receives it and decrypts it.It works perfectly till the part where the server has to decrypt the file.Indeed the CipherInputStream which should return the decrypted plain text results null but the FileInputStream that I use in the function is ok so I don't really know what the problem may be.
Client:
import jdk.internal.util.xml.impl.Input;
import javax.crypto.*;
import javax.crypto.spec.DESKeySpec;
import java.io.*;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
import java.security.spec.InvalidKeySpecException;
import java.util.Scanner;
import java.net.*;
import java.nio.file.Files;
import java.nio.file.Path;
public class Main {
static private int porta = 81;
static private String hostname = "localhost";
public static void main(String args) {
OutputStream outputStream = null;
Socket socket = null;
boolean bIsConnected = false;
System.out.println("Inserisci il percorso del file che vuoi criptare");
Scanner in = new Scanner(System.in);
String path = in.nextLine(); //percorso da usare
System.out.println("Inserisci la chiave di criptazione");
String key = in.nextLine(); //Chiede la chiave di decriptazione (8 caratteri)
File plaintext = new File(path);
File encrypted = new File("Criptato.txt"); //salva il file nella cartella locale del progetto
try {
Encrypt(key, plaintext, encrypted);
System.out.println("Crittazione completata");
} catch (InvalidKeyException | NoSuchAlgorithmException | InvalidKeySpecException | NoSuchPaddingException | IOException e) {
e.printStackTrace();
}
while (!bIsConnected) { //crittato il file aspetta che l'utente si colleghi al portale del server per mandare il file
try {
socket = new Socket(hostname, porta);
bIsConnected = true;
} catch (SocketException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
byte bytes = new byte[16 * 1024];
try {
InputStream inp = new FileInputStream(encrypted);//converte il file in uno stream
OutputStream outp = socket.getOutputStream();
int count;
while ((count = inp.read(bytes)) > 0) {
outp.write(bytes, 0, count); //scrive sulla socket del server il contenuto del file byte per byte
}
} //si collega all'output stream della socket
catch (IOException e) {
}
}
private static void write(InputStream in, OutputStream out) throws IOException {
byte buffer = new byte[64];
int numOfBytesRead;
while ((numOfBytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, numOfBytesRead);
}
out.close();
in.close();
}
public static void Encrypt(String key, File in, File out)
throws InvalidKeyException, NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeySpecException, IOException {
FileInputStream fis = new FileInputStream(in);
FileOutputStream fos = new FileOutputStream(out);
DESKeySpec desKeySpec = new DESKeySpec(key.getBytes());
SecretKeyFactory skf = SecretKeyFactory.getInstance("DES");
SecretKey secretKey = skf.generateSecret(desKeySpec);
Cipher cipher = Cipher.getInstance("DES/ECB/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, secretKey, SecureRandom.getInstance("SHA1PRNG"));
CipherInputStream cis = new CipherInputStream(fis, cipher);
write(cis, fos);
}
}
Server:
import javax.crypto.*;
import javax.crypto.spec.DESKeySpec;
import java.io.*;
import java.nio.file.Paths;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
import java.security.spec.InvalidKeySpecException;
import java.util.Scanner;
import java.net.*;
import java.nio.file.Files;
public class Main {
static private int portNumber = 81;
public static void main(String args) {
File criptato = new File("CriptatoServer.txt");
File decriptato = new File("Decrittato.txt");
System.out.println("Server running");
// Listening in entrata
ServerSocket socketServer;
try {
socketServer = new ServerSocket(portNumber);
} catch (IOException e) {
System.out.println("Impossibile ascoltare sulla porta.");
e.printStackTrace();
return;
}
while (true) {
Socket socket = null;
try {
socket = socketServer.accept();
} catch (IOException e) {
System.out.println("Impossibile accettare client.");
e.printStackTrace();
return;
}
System.out.println("Ricevuto client socket!");
InputStream in = null;
OutputStream out = null;
try {
in = socket.getInputStream(); //prende ciò che arriva dal client
} catch (IOException ex) {
System.out.println("Can't get socket input stream. ");
}
try {
out = new FileOutputStream(criptato); //scrive nel file criptato (sta copiando dal client)
} catch (FileNotFoundException ex) {
System.out.println("File not found. ");
}
byte bytes = new byte[16 * 1024];
int count;
try {
while ((count = in.read(bytes)) > 0) {
out.write(bytes, 0, count);
}
}catch(IOException e){System.out.println("AHIAIAI");}
System.out.println("Inserisci la chiave per decriptare il file e leggere il messaggio");
Scanner ins = new Scanner(System.in);
String key = ins.nextLine(); //chiave
try {
Decrypt(key, criptato, decriptato);
System.out.println("Decrittazione completata");
} catch (InvalidKeyException | NoSuchAlgorithmException | InvalidKeySpecException | NoSuchPaddingException | IOException e) {
e.printStackTrace();
}
}
}
private static void write(InputStream in, OutputStream out) throws IOException {
byte buffer = new byte[64];
int numOfBytesRead;
while ((numOfBytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, numOfBytesRead);
}
out.close();
in.close();
}
public static void Decrypt(String key, File in, File out)
throws InvalidKeyException, NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeySpecException, IOException {
FileInputStream fis = new FileInputStream(in); //seems ok
FileOutputStream fos = new FileOutputStream(out);
DESKeySpec desKeySpec = new DESKeySpec(key.getBytes());
SecretKeyFactory skf = SecretKeyFactory.getInstance("DES");
SecretKey secretKey = skf.generateSecret(desKeySpec);
Cipher cipher = Cipher.getInstance("DES/ECB/PKCS5Padding");
cipher.init(Cipher.DECRYPT_MODE, secretKey, SecureRandom.getInstance("SHA1PRNG"));
CipherInputStream cis = new CipherInputStream(fis, cipher); //returns null
write(cis, fos);
}
public static String getFileContent(
FileInputStream fis,
String encoding ) throws IOException
{
try( BufferedReader br =
new BufferedReader( new InputStreamReader(fis, encoding )))
{
StringBuilder sb = new StringBuilder();
String line;
while(( line = br.readLine()) != null ) {
sb.append( line );
sb.append( 'n' );
}
return sb.toString();
}
}
}
I know it's a bit messy but I'm still working on it.
java sockets encryption cryptography jce
add a comment |
I'm making a simple project where a client encrypt a file with JCE (DES) and sends it to the server with sockets. The server receives it and decrypts it.It works perfectly till the part where the server has to decrypt the file.Indeed the CipherInputStream which should return the decrypted plain text results null but the FileInputStream that I use in the function is ok so I don't really know what the problem may be.
Client:
import jdk.internal.util.xml.impl.Input;
import javax.crypto.*;
import javax.crypto.spec.DESKeySpec;
import java.io.*;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
import java.security.spec.InvalidKeySpecException;
import java.util.Scanner;
import java.net.*;
import java.nio.file.Files;
import java.nio.file.Path;
public class Main {
static private int porta = 81;
static private String hostname = "localhost";
public static void main(String args) {
OutputStream outputStream = null;
Socket socket = null;
boolean bIsConnected = false;
System.out.println("Inserisci il percorso del file che vuoi criptare");
Scanner in = new Scanner(System.in);
String path = in.nextLine(); //percorso da usare
System.out.println("Inserisci la chiave di criptazione");
String key = in.nextLine(); //Chiede la chiave di decriptazione (8 caratteri)
File plaintext = new File(path);
File encrypted = new File("Criptato.txt"); //salva il file nella cartella locale del progetto
try {
Encrypt(key, plaintext, encrypted);
System.out.println("Crittazione completata");
} catch (InvalidKeyException | NoSuchAlgorithmException | InvalidKeySpecException | NoSuchPaddingException | IOException e) {
e.printStackTrace();
}
while (!bIsConnected) { //crittato il file aspetta che l'utente si colleghi al portale del server per mandare il file
try {
socket = new Socket(hostname, porta);
bIsConnected = true;
} catch (SocketException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
byte bytes = new byte[16 * 1024];
try {
InputStream inp = new FileInputStream(encrypted);//converte il file in uno stream
OutputStream outp = socket.getOutputStream();
int count;
while ((count = inp.read(bytes)) > 0) {
outp.write(bytes, 0, count); //scrive sulla socket del server il contenuto del file byte per byte
}
} //si collega all'output stream della socket
catch (IOException e) {
}
}
private static void write(InputStream in, OutputStream out) throws IOException {
byte buffer = new byte[64];
int numOfBytesRead;
while ((numOfBytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, numOfBytesRead);
}
out.close();
in.close();
}
public static void Encrypt(String key, File in, File out)
throws InvalidKeyException, NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeySpecException, IOException {
FileInputStream fis = new FileInputStream(in);
FileOutputStream fos = new FileOutputStream(out);
DESKeySpec desKeySpec = new DESKeySpec(key.getBytes());
SecretKeyFactory skf = SecretKeyFactory.getInstance("DES");
SecretKey secretKey = skf.generateSecret(desKeySpec);
Cipher cipher = Cipher.getInstance("DES/ECB/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, secretKey, SecureRandom.getInstance("SHA1PRNG"));
CipherInputStream cis = new CipherInputStream(fis, cipher);
write(cis, fos);
}
}
Server:
import javax.crypto.*;
import javax.crypto.spec.DESKeySpec;
import java.io.*;
import java.nio.file.Paths;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
import java.security.spec.InvalidKeySpecException;
import java.util.Scanner;
import java.net.*;
import java.nio.file.Files;
public class Main {
static private int portNumber = 81;
public static void main(String args) {
File criptato = new File("CriptatoServer.txt");
File decriptato = new File("Decrittato.txt");
System.out.println("Server running");
// Listening in entrata
ServerSocket socketServer;
try {
socketServer = new ServerSocket(portNumber);
} catch (IOException e) {
System.out.println("Impossibile ascoltare sulla porta.");
e.printStackTrace();
return;
}
while (true) {
Socket socket = null;
try {
socket = socketServer.accept();
} catch (IOException e) {
System.out.println("Impossibile accettare client.");
e.printStackTrace();
return;
}
System.out.println("Ricevuto client socket!");
InputStream in = null;
OutputStream out = null;
try {
in = socket.getInputStream(); //prende ciò che arriva dal client
} catch (IOException ex) {
System.out.println("Can't get socket input stream. ");
}
try {
out = new FileOutputStream(criptato); //scrive nel file criptato (sta copiando dal client)
} catch (FileNotFoundException ex) {
System.out.println("File not found. ");
}
byte bytes = new byte[16 * 1024];
int count;
try {
while ((count = in.read(bytes)) > 0) {
out.write(bytes, 0, count);
}
}catch(IOException e){System.out.println("AHIAIAI");}
System.out.println("Inserisci la chiave per decriptare il file e leggere il messaggio");
Scanner ins = new Scanner(System.in);
String key = ins.nextLine(); //chiave
try {
Decrypt(key, criptato, decriptato);
System.out.println("Decrittazione completata");
} catch (InvalidKeyException | NoSuchAlgorithmException | InvalidKeySpecException | NoSuchPaddingException | IOException e) {
e.printStackTrace();
}
}
}
private static void write(InputStream in, OutputStream out) throws IOException {
byte buffer = new byte[64];
int numOfBytesRead;
while ((numOfBytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, numOfBytesRead);
}
out.close();
in.close();
}
public static void Decrypt(String key, File in, File out)
throws InvalidKeyException, NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeySpecException, IOException {
FileInputStream fis = new FileInputStream(in); //seems ok
FileOutputStream fos = new FileOutputStream(out);
DESKeySpec desKeySpec = new DESKeySpec(key.getBytes());
SecretKeyFactory skf = SecretKeyFactory.getInstance("DES");
SecretKey secretKey = skf.generateSecret(desKeySpec);
Cipher cipher = Cipher.getInstance("DES/ECB/PKCS5Padding");
cipher.init(Cipher.DECRYPT_MODE, secretKey, SecureRandom.getInstance("SHA1PRNG"));
CipherInputStream cis = new CipherInputStream(fis, cipher); //returns null
write(cis, fos);
}
public static String getFileContent(
FileInputStream fis,
String encoding ) throws IOException
{
try( BufferedReader br =
new BufferedReader( new InputStreamReader(fis, encoding )))
{
StringBuilder sb = new StringBuilder();
String line;
while(( line = br.readLine()) != null ) {
sb.append( line );
sb.append( 'n' );
}
return sb.toString();
}
}
}
I know it's a bit messy but I'm still working on it.
java sockets encryption cryptography jce
Why would you use an insecure cipher like DES in an insecure mode of operation like ECB?
– TheGreatContini
Nov 16 '18 at 18:46
Initial observation: you often don't close your Input or Output streams.
– James K Polk
Nov 16 '18 at 20:40
Yep, you need to close the client'soutp
after you've written all the data. Otherwise the server is just blocking on it's socket read, waiting for more data.
– James K Polk
Nov 16 '18 at 21:03
@TheGreatContini it's an assignment.If it was me I'd have used AES and CBC
– Fiorenzo
Nov 17 '18 at 20:20
Read about try with resources. Note that not all exceptions need to be handled directly, it is possible to rethrow or to wrap them in aRuntimeException
. Using CBC over a channel is insecure as well, as it is commonly susceptible to padding oracle attacks.
– Maarten Bodewes
Nov 29 '18 at 4:15
add a comment |
I'm making a simple project where a client encrypt a file with JCE (DES) and sends it to the server with sockets. The server receives it and decrypts it.It works perfectly till the part where the server has to decrypt the file.Indeed the CipherInputStream which should return the decrypted plain text results null but the FileInputStream that I use in the function is ok so I don't really know what the problem may be.
Client:
import jdk.internal.util.xml.impl.Input;
import javax.crypto.*;
import javax.crypto.spec.DESKeySpec;
import java.io.*;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
import java.security.spec.InvalidKeySpecException;
import java.util.Scanner;
import java.net.*;
import java.nio.file.Files;
import java.nio.file.Path;
public class Main {
static private int porta = 81;
static private String hostname = "localhost";
public static void main(String args) {
OutputStream outputStream = null;
Socket socket = null;
boolean bIsConnected = false;
System.out.println("Inserisci il percorso del file che vuoi criptare");
Scanner in = new Scanner(System.in);
String path = in.nextLine(); //percorso da usare
System.out.println("Inserisci la chiave di criptazione");
String key = in.nextLine(); //Chiede la chiave di decriptazione (8 caratteri)
File plaintext = new File(path);
File encrypted = new File("Criptato.txt"); //salva il file nella cartella locale del progetto
try {
Encrypt(key, plaintext, encrypted);
System.out.println("Crittazione completata");
} catch (InvalidKeyException | NoSuchAlgorithmException | InvalidKeySpecException | NoSuchPaddingException | IOException e) {
e.printStackTrace();
}
while (!bIsConnected) { //crittato il file aspetta che l'utente si colleghi al portale del server per mandare il file
try {
socket = new Socket(hostname, porta);
bIsConnected = true;
} catch (SocketException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
byte bytes = new byte[16 * 1024];
try {
InputStream inp = new FileInputStream(encrypted);//converte il file in uno stream
OutputStream outp = socket.getOutputStream();
int count;
while ((count = inp.read(bytes)) > 0) {
outp.write(bytes, 0, count); //scrive sulla socket del server il contenuto del file byte per byte
}
} //si collega all'output stream della socket
catch (IOException e) {
}
}
private static void write(InputStream in, OutputStream out) throws IOException {
byte buffer = new byte[64];
int numOfBytesRead;
while ((numOfBytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, numOfBytesRead);
}
out.close();
in.close();
}
public static void Encrypt(String key, File in, File out)
throws InvalidKeyException, NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeySpecException, IOException {
FileInputStream fis = new FileInputStream(in);
FileOutputStream fos = new FileOutputStream(out);
DESKeySpec desKeySpec = new DESKeySpec(key.getBytes());
SecretKeyFactory skf = SecretKeyFactory.getInstance("DES");
SecretKey secretKey = skf.generateSecret(desKeySpec);
Cipher cipher = Cipher.getInstance("DES/ECB/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, secretKey, SecureRandom.getInstance("SHA1PRNG"));
CipherInputStream cis = new CipherInputStream(fis, cipher);
write(cis, fos);
}
}
Server:
import javax.crypto.*;
import javax.crypto.spec.DESKeySpec;
import java.io.*;
import java.nio.file.Paths;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
import java.security.spec.InvalidKeySpecException;
import java.util.Scanner;
import java.net.*;
import java.nio.file.Files;
public class Main {
static private int portNumber = 81;
public static void main(String args) {
File criptato = new File("CriptatoServer.txt");
File decriptato = new File("Decrittato.txt");
System.out.println("Server running");
// Listening in entrata
ServerSocket socketServer;
try {
socketServer = new ServerSocket(portNumber);
} catch (IOException e) {
System.out.println("Impossibile ascoltare sulla porta.");
e.printStackTrace();
return;
}
while (true) {
Socket socket = null;
try {
socket = socketServer.accept();
} catch (IOException e) {
System.out.println("Impossibile accettare client.");
e.printStackTrace();
return;
}
System.out.println("Ricevuto client socket!");
InputStream in = null;
OutputStream out = null;
try {
in = socket.getInputStream(); //prende ciò che arriva dal client
} catch (IOException ex) {
System.out.println("Can't get socket input stream. ");
}
try {
out = new FileOutputStream(criptato); //scrive nel file criptato (sta copiando dal client)
} catch (FileNotFoundException ex) {
System.out.println("File not found. ");
}
byte bytes = new byte[16 * 1024];
int count;
try {
while ((count = in.read(bytes)) > 0) {
out.write(bytes, 0, count);
}
}catch(IOException e){System.out.println("AHIAIAI");}
System.out.println("Inserisci la chiave per decriptare il file e leggere il messaggio");
Scanner ins = new Scanner(System.in);
String key = ins.nextLine(); //chiave
try {
Decrypt(key, criptato, decriptato);
System.out.println("Decrittazione completata");
} catch (InvalidKeyException | NoSuchAlgorithmException | InvalidKeySpecException | NoSuchPaddingException | IOException e) {
e.printStackTrace();
}
}
}
private static void write(InputStream in, OutputStream out) throws IOException {
byte buffer = new byte[64];
int numOfBytesRead;
while ((numOfBytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, numOfBytesRead);
}
out.close();
in.close();
}
public static void Decrypt(String key, File in, File out)
throws InvalidKeyException, NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeySpecException, IOException {
FileInputStream fis = new FileInputStream(in); //seems ok
FileOutputStream fos = new FileOutputStream(out);
DESKeySpec desKeySpec = new DESKeySpec(key.getBytes());
SecretKeyFactory skf = SecretKeyFactory.getInstance("DES");
SecretKey secretKey = skf.generateSecret(desKeySpec);
Cipher cipher = Cipher.getInstance("DES/ECB/PKCS5Padding");
cipher.init(Cipher.DECRYPT_MODE, secretKey, SecureRandom.getInstance("SHA1PRNG"));
CipherInputStream cis = new CipherInputStream(fis, cipher); //returns null
write(cis, fos);
}
public static String getFileContent(
FileInputStream fis,
String encoding ) throws IOException
{
try( BufferedReader br =
new BufferedReader( new InputStreamReader(fis, encoding )))
{
StringBuilder sb = new StringBuilder();
String line;
while(( line = br.readLine()) != null ) {
sb.append( line );
sb.append( 'n' );
}
return sb.toString();
}
}
}
I know it's a bit messy but I'm still working on it.
java sockets encryption cryptography jce
I'm making a simple project where a client encrypt a file with JCE (DES) and sends it to the server with sockets. The server receives it and decrypts it.It works perfectly till the part where the server has to decrypt the file.Indeed the CipherInputStream which should return the decrypted plain text results null but the FileInputStream that I use in the function is ok so I don't really know what the problem may be.
Client:
import jdk.internal.util.xml.impl.Input;
import javax.crypto.*;
import javax.crypto.spec.DESKeySpec;
import java.io.*;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
import java.security.spec.InvalidKeySpecException;
import java.util.Scanner;
import java.net.*;
import java.nio.file.Files;
import java.nio.file.Path;
public class Main {
static private int porta = 81;
static private String hostname = "localhost";
public static void main(String args) {
OutputStream outputStream = null;
Socket socket = null;
boolean bIsConnected = false;
System.out.println("Inserisci il percorso del file che vuoi criptare");
Scanner in = new Scanner(System.in);
String path = in.nextLine(); //percorso da usare
System.out.println("Inserisci la chiave di criptazione");
String key = in.nextLine(); //Chiede la chiave di decriptazione (8 caratteri)
File plaintext = new File(path);
File encrypted = new File("Criptato.txt"); //salva il file nella cartella locale del progetto
try {
Encrypt(key, plaintext, encrypted);
System.out.println("Crittazione completata");
} catch (InvalidKeyException | NoSuchAlgorithmException | InvalidKeySpecException | NoSuchPaddingException | IOException e) {
e.printStackTrace();
}
while (!bIsConnected) { //crittato il file aspetta che l'utente si colleghi al portale del server per mandare il file
try {
socket = new Socket(hostname, porta);
bIsConnected = true;
} catch (SocketException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
byte bytes = new byte[16 * 1024];
try {
InputStream inp = new FileInputStream(encrypted);//converte il file in uno stream
OutputStream outp = socket.getOutputStream();
int count;
while ((count = inp.read(bytes)) > 0) {
outp.write(bytes, 0, count); //scrive sulla socket del server il contenuto del file byte per byte
}
} //si collega all'output stream della socket
catch (IOException e) {
}
}
private static void write(InputStream in, OutputStream out) throws IOException {
byte buffer = new byte[64];
int numOfBytesRead;
while ((numOfBytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, numOfBytesRead);
}
out.close();
in.close();
}
public static void Encrypt(String key, File in, File out)
throws InvalidKeyException, NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeySpecException, IOException {
FileInputStream fis = new FileInputStream(in);
FileOutputStream fos = new FileOutputStream(out);
DESKeySpec desKeySpec = new DESKeySpec(key.getBytes());
SecretKeyFactory skf = SecretKeyFactory.getInstance("DES");
SecretKey secretKey = skf.generateSecret(desKeySpec);
Cipher cipher = Cipher.getInstance("DES/ECB/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, secretKey, SecureRandom.getInstance("SHA1PRNG"));
CipherInputStream cis = new CipherInputStream(fis, cipher);
write(cis, fos);
}
}
Server:
import javax.crypto.*;
import javax.crypto.spec.DESKeySpec;
import java.io.*;
import java.nio.file.Paths;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
import java.security.spec.InvalidKeySpecException;
import java.util.Scanner;
import java.net.*;
import java.nio.file.Files;
public class Main {
static private int portNumber = 81;
public static void main(String args) {
File criptato = new File("CriptatoServer.txt");
File decriptato = new File("Decrittato.txt");
System.out.println("Server running");
// Listening in entrata
ServerSocket socketServer;
try {
socketServer = new ServerSocket(portNumber);
} catch (IOException e) {
System.out.println("Impossibile ascoltare sulla porta.");
e.printStackTrace();
return;
}
while (true) {
Socket socket = null;
try {
socket = socketServer.accept();
} catch (IOException e) {
System.out.println("Impossibile accettare client.");
e.printStackTrace();
return;
}
System.out.println("Ricevuto client socket!");
InputStream in = null;
OutputStream out = null;
try {
in = socket.getInputStream(); //prende ciò che arriva dal client
} catch (IOException ex) {
System.out.println("Can't get socket input stream. ");
}
try {
out = new FileOutputStream(criptato); //scrive nel file criptato (sta copiando dal client)
} catch (FileNotFoundException ex) {
System.out.println("File not found. ");
}
byte bytes = new byte[16 * 1024];
int count;
try {
while ((count = in.read(bytes)) > 0) {
out.write(bytes, 0, count);
}
}catch(IOException e){System.out.println("AHIAIAI");}
System.out.println("Inserisci la chiave per decriptare il file e leggere il messaggio");
Scanner ins = new Scanner(System.in);
String key = ins.nextLine(); //chiave
try {
Decrypt(key, criptato, decriptato);
System.out.println("Decrittazione completata");
} catch (InvalidKeyException | NoSuchAlgorithmException | InvalidKeySpecException | NoSuchPaddingException | IOException e) {
e.printStackTrace();
}
}
}
private static void write(InputStream in, OutputStream out) throws IOException {
byte buffer = new byte[64];
int numOfBytesRead;
while ((numOfBytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, numOfBytesRead);
}
out.close();
in.close();
}
public static void Decrypt(String key, File in, File out)
throws InvalidKeyException, NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeySpecException, IOException {
FileInputStream fis = new FileInputStream(in); //seems ok
FileOutputStream fos = new FileOutputStream(out);
DESKeySpec desKeySpec = new DESKeySpec(key.getBytes());
SecretKeyFactory skf = SecretKeyFactory.getInstance("DES");
SecretKey secretKey = skf.generateSecret(desKeySpec);
Cipher cipher = Cipher.getInstance("DES/ECB/PKCS5Padding");
cipher.init(Cipher.DECRYPT_MODE, secretKey, SecureRandom.getInstance("SHA1PRNG"));
CipherInputStream cis = new CipherInputStream(fis, cipher); //returns null
write(cis, fos);
}
public static String getFileContent(
FileInputStream fis,
String encoding ) throws IOException
{
try( BufferedReader br =
new BufferedReader( new InputStreamReader(fis, encoding )))
{
StringBuilder sb = new StringBuilder();
String line;
while(( line = br.readLine()) != null ) {
sb.append( line );
sb.append( 'n' );
}
return sb.toString();
}
}
}
I know it's a bit messy but I'm still working on it.
java sockets encryption cryptography jce
java sockets encryption cryptography jce
asked Nov 16 '18 at 18:39
FiorenzoFiorenzo
82
82
Why would you use an insecure cipher like DES in an insecure mode of operation like ECB?
– TheGreatContini
Nov 16 '18 at 18:46
Initial observation: you often don't close your Input or Output streams.
– James K Polk
Nov 16 '18 at 20:40
Yep, you need to close the client'soutp
after you've written all the data. Otherwise the server is just blocking on it's socket read, waiting for more data.
– James K Polk
Nov 16 '18 at 21:03
@TheGreatContini it's an assignment.If it was me I'd have used AES and CBC
– Fiorenzo
Nov 17 '18 at 20:20
Read about try with resources. Note that not all exceptions need to be handled directly, it is possible to rethrow or to wrap them in aRuntimeException
. Using CBC over a channel is insecure as well, as it is commonly susceptible to padding oracle attacks.
– Maarten Bodewes
Nov 29 '18 at 4:15
add a comment |
Why would you use an insecure cipher like DES in an insecure mode of operation like ECB?
– TheGreatContini
Nov 16 '18 at 18:46
Initial observation: you often don't close your Input or Output streams.
– James K Polk
Nov 16 '18 at 20:40
Yep, you need to close the client'soutp
after you've written all the data. Otherwise the server is just blocking on it's socket read, waiting for more data.
– James K Polk
Nov 16 '18 at 21:03
@TheGreatContini it's an assignment.If it was me I'd have used AES and CBC
– Fiorenzo
Nov 17 '18 at 20:20
Read about try with resources. Note that not all exceptions need to be handled directly, it is possible to rethrow or to wrap them in aRuntimeException
. Using CBC over a channel is insecure as well, as it is commonly susceptible to padding oracle attacks.
– Maarten Bodewes
Nov 29 '18 at 4:15
Why would you use an insecure cipher like DES in an insecure mode of operation like ECB?
– TheGreatContini
Nov 16 '18 at 18:46
Why would you use an insecure cipher like DES in an insecure mode of operation like ECB?
– TheGreatContini
Nov 16 '18 at 18:46
Initial observation: you often don't close your Input or Output streams.
– James K Polk
Nov 16 '18 at 20:40
Initial observation: you often don't close your Input or Output streams.
– James K Polk
Nov 16 '18 at 20:40
Yep, you need to close the client's
outp
after you've written all the data. Otherwise the server is just blocking on it's socket read, waiting for more data.– James K Polk
Nov 16 '18 at 21:03
Yep, you need to close the client's
outp
after you've written all the data. Otherwise the server is just blocking on it's socket read, waiting for more data.– James K Polk
Nov 16 '18 at 21:03
@TheGreatContini it's an assignment.If it was me I'd have used AES and CBC
– Fiorenzo
Nov 17 '18 at 20:20
@TheGreatContini it's an assignment.If it was me I'd have used AES and CBC
– Fiorenzo
Nov 17 '18 at 20:20
Read about try with resources. Note that not all exceptions need to be handled directly, it is possible to rethrow or to wrap them in a
RuntimeException
. Using CBC over a channel is insecure as well, as it is commonly susceptible to padding oracle attacks.– Maarten Bodewes
Nov 29 '18 at 4:15
Read about try with resources. Note that not all exceptions need to be handled directly, it is possible to rethrow or to wrap them in a
RuntimeException
. Using CBC over a channel is insecure as well, as it is commonly susceptible to padding oracle attacks.– Maarten Bodewes
Nov 29 '18 at 4:15
add a comment |
1 Answer
1
active
oldest
votes
You have neglected to close the output side of the client's socket after you finished writing to it. Thus the connection is left open, and the server side simply blocks in its read loop waiting for more input.
Your exception handling is so complicated it actually obscures the logic of your code. On the client side you can do something like:
InputStream inp = new FileInputStream(encrypted);//converte il file in uno stream
OutputStream outp = socket.getOutputStream();
int count;
while ((count = inp.read(bytes)) > 0) {
outp.write(bytes, 0, count); //scrive sulla socket del server il contenuto del file byte per byte
}
outp.close();
socket.close();
yes you are right.Anyway the problem was that the file I crypted was EMPTY.When I tried to fill it with random words the program finally started working and that was quite embarassing...
– Fiorenzo
Nov 17 '18 at 20:16
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53343624%2fjava-inputstream-crypting%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You have neglected to close the output side of the client's socket after you finished writing to it. Thus the connection is left open, and the server side simply blocks in its read loop waiting for more input.
Your exception handling is so complicated it actually obscures the logic of your code. On the client side you can do something like:
InputStream inp = new FileInputStream(encrypted);//converte il file in uno stream
OutputStream outp = socket.getOutputStream();
int count;
while ((count = inp.read(bytes)) > 0) {
outp.write(bytes, 0, count); //scrive sulla socket del server il contenuto del file byte per byte
}
outp.close();
socket.close();
yes you are right.Anyway the problem was that the file I crypted was EMPTY.When I tried to fill it with random words the program finally started working and that was quite embarassing...
– Fiorenzo
Nov 17 '18 at 20:16
add a comment |
You have neglected to close the output side of the client's socket after you finished writing to it. Thus the connection is left open, and the server side simply blocks in its read loop waiting for more input.
Your exception handling is so complicated it actually obscures the logic of your code. On the client side you can do something like:
InputStream inp = new FileInputStream(encrypted);//converte il file in uno stream
OutputStream outp = socket.getOutputStream();
int count;
while ((count = inp.read(bytes)) > 0) {
outp.write(bytes, 0, count); //scrive sulla socket del server il contenuto del file byte per byte
}
outp.close();
socket.close();
yes you are right.Anyway the problem was that the file I crypted was EMPTY.When I tried to fill it with random words the program finally started working and that was quite embarassing...
– Fiorenzo
Nov 17 '18 at 20:16
add a comment |
You have neglected to close the output side of the client's socket after you finished writing to it. Thus the connection is left open, and the server side simply blocks in its read loop waiting for more input.
Your exception handling is so complicated it actually obscures the logic of your code. On the client side you can do something like:
InputStream inp = new FileInputStream(encrypted);//converte il file in uno stream
OutputStream outp = socket.getOutputStream();
int count;
while ((count = inp.read(bytes)) > 0) {
outp.write(bytes, 0, count); //scrive sulla socket del server il contenuto del file byte per byte
}
outp.close();
socket.close();
You have neglected to close the output side of the client's socket after you finished writing to it. Thus the connection is left open, and the server side simply blocks in its read loop waiting for more input.
Your exception handling is so complicated it actually obscures the logic of your code. On the client side you can do something like:
InputStream inp = new FileInputStream(encrypted);//converte il file in uno stream
OutputStream outp = socket.getOutputStream();
int count;
while ((count = inp.read(bytes)) > 0) {
outp.write(bytes, 0, count); //scrive sulla socket del server il contenuto del file byte per byte
}
outp.close();
socket.close();
answered Nov 16 '18 at 21:10


James K PolkJames K Polk
30.6k116997
30.6k116997
yes you are right.Anyway the problem was that the file I crypted was EMPTY.When I tried to fill it with random words the program finally started working and that was quite embarassing...
– Fiorenzo
Nov 17 '18 at 20:16
add a comment |
yes you are right.Anyway the problem was that the file I crypted was EMPTY.When I tried to fill it with random words the program finally started working and that was quite embarassing...
– Fiorenzo
Nov 17 '18 at 20:16
yes you are right.Anyway the problem was that the file I crypted was EMPTY.When I tried to fill it with random words the program finally started working and that was quite embarassing...
– Fiorenzo
Nov 17 '18 at 20:16
yes you are right.Anyway the problem was that the file I crypted was EMPTY.When I tried to fill it with random words the program finally started working and that was quite embarassing...
– Fiorenzo
Nov 17 '18 at 20:16
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53343624%2fjava-inputstream-crypting%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
k0ABKmEGVzcuUkY MR0U,KTj,Vfnb6Vtv,5MYMJtpmfmjWlqN3r2,D,y1LFkArdpf Mah,3,5qG3 PC
Why would you use an insecure cipher like DES in an insecure mode of operation like ECB?
– TheGreatContini
Nov 16 '18 at 18:46
Initial observation: you often don't close your Input or Output streams.
– James K Polk
Nov 16 '18 at 20:40
Yep, you need to close the client's
outp
after you've written all the data. Otherwise the server is just blocking on it's socket read, waiting for more data.– James K Polk
Nov 16 '18 at 21:03
@TheGreatContini it's an assignment.If it was me I'd have used AES and CBC
– Fiorenzo
Nov 17 '18 at 20:20
Read about try with resources. Note that not all exceptions need to be handled directly, it is possible to rethrow or to wrap them in a
RuntimeException
. Using CBC over a channel is insecure as well, as it is commonly susceptible to padding oracle attacks.– Maarten Bodewes
Nov 29 '18 at 4:15