Does OpenJ9 support sun.misc.Contended annotation?
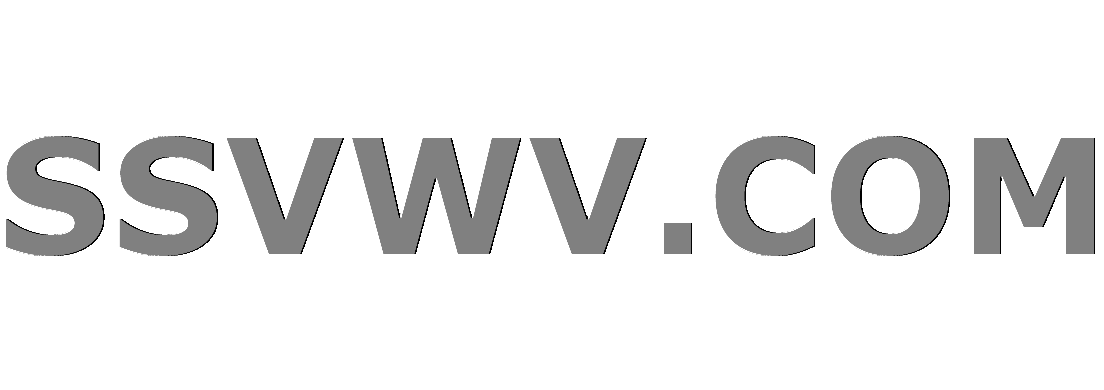
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I've created a program to check if @sun.misc.Contended
is in effect. The idea is that when @Contended
is in effect, the field offsets in annotated class will be larger.
I can see the expected difference in offsets on OpenJDK, if I specify the -XX:-RestrictContended
flag. I do not see any difference on OpenJ9 11 (jdk-11.0.1+13, Eclipse OpenJ9 VM-11.0.1) through.
The OpenJDK output is
readOnly: 12
writeOnly: 16
----
readOnly: 12
writeOnly: 144
The OpenJ9 output is
readOnly: 8
writeOnly: 12
----
readOnly: 8
writeOnly: 12
The program is
import sun.misc.Unsafe;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
public class Main {
public static class Baseline {
int readOnly;
int writeOnly;
}
public static class Contended {
int readOnly;
@sun.misc.Contended
int writeOnly;
}
public static void main(String args) throws Exception {
Baseline b = new Baseline();
Contended s = new Contended();
printOffsets(b);
System.out.println("----");
printOffsets(s);
}
// https://blog.hazelcast.com/using-sun-misc-unsafe-in-java-9/
@SuppressWarnings("restriction")
private static Unsafe getUnsafe() throws NoSuchFieldException, IllegalAccessException {
Field singleoneInstanceField = Unsafe.class.getDeclaredField("theUnsafe");
singleoneInstanceField.setAccessible(true);
return (Unsafe) singleoneInstanceField.get(null);
}
// http://mishadoff.com/blog/java-magic-part-4-sun-dot-misc-dot-unsafe/
public static void printOffsets(Object o) throws Exception {
Unsafe u = getUnsafe();
Class c = o.getClass();
for (Field f : c.getDeclaredFields()) {
if ((f.getModifiers() & Modifier.STATIC) == 0) {
printOffset(u, f);
}
}
}
public static void printOffset(Unsafe u, Field f) {
long offset = u.objectFieldOffset(f);
System.out.println(f.getName() + ": " + offset);
}
}
java ibm-jvm openj9
add a comment |
I've created a program to check if @sun.misc.Contended
is in effect. The idea is that when @Contended
is in effect, the field offsets in annotated class will be larger.
I can see the expected difference in offsets on OpenJDK, if I specify the -XX:-RestrictContended
flag. I do not see any difference on OpenJ9 11 (jdk-11.0.1+13, Eclipse OpenJ9 VM-11.0.1) through.
The OpenJDK output is
readOnly: 12
writeOnly: 16
----
readOnly: 12
writeOnly: 144
The OpenJ9 output is
readOnly: 8
writeOnly: 12
----
readOnly: 8
writeOnly: 12
The program is
import sun.misc.Unsafe;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
public class Main {
public static class Baseline {
int readOnly;
int writeOnly;
}
public static class Contended {
int readOnly;
@sun.misc.Contended
int writeOnly;
}
public static void main(String args) throws Exception {
Baseline b = new Baseline();
Contended s = new Contended();
printOffsets(b);
System.out.println("----");
printOffsets(s);
}
// https://blog.hazelcast.com/using-sun-misc-unsafe-in-java-9/
@SuppressWarnings("restriction")
private static Unsafe getUnsafe() throws NoSuchFieldException, IllegalAccessException {
Field singleoneInstanceField = Unsafe.class.getDeclaredField("theUnsafe");
singleoneInstanceField.setAccessible(true);
return (Unsafe) singleoneInstanceField.get(null);
}
// http://mishadoff.com/blog/java-magic-part-4-sun-dot-misc-dot-unsafe/
public static void printOffsets(Object o) throws Exception {
Unsafe u = getUnsafe();
Class c = o.getClass();
for (Field f : c.getDeclaredFields()) {
if ((f.getModifiers() & Modifier.STATIC) == 0) {
printOffset(u, f);
}
}
}
public static void printOffset(Unsafe u, Field f) {
long offset = u.objectFieldOffset(f);
System.out.println(f.getName() + ": " + offset);
}
}
java ibm-jvm openj9
helpful discussion on OpenJ9 chat openj9.slack.com/archives/C862YFGL9/p1542383521060200
– user7610
Nov 16 '18 at 16:46
feature request github.com/eclipse/openj9/issues/3716
– user7610
Nov 16 '18 at 19:50
add a comment |
I've created a program to check if @sun.misc.Contended
is in effect. The idea is that when @Contended
is in effect, the field offsets in annotated class will be larger.
I can see the expected difference in offsets on OpenJDK, if I specify the -XX:-RestrictContended
flag. I do not see any difference on OpenJ9 11 (jdk-11.0.1+13, Eclipse OpenJ9 VM-11.0.1) through.
The OpenJDK output is
readOnly: 12
writeOnly: 16
----
readOnly: 12
writeOnly: 144
The OpenJ9 output is
readOnly: 8
writeOnly: 12
----
readOnly: 8
writeOnly: 12
The program is
import sun.misc.Unsafe;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
public class Main {
public static class Baseline {
int readOnly;
int writeOnly;
}
public static class Contended {
int readOnly;
@sun.misc.Contended
int writeOnly;
}
public static void main(String args) throws Exception {
Baseline b = new Baseline();
Contended s = new Contended();
printOffsets(b);
System.out.println("----");
printOffsets(s);
}
// https://blog.hazelcast.com/using-sun-misc-unsafe-in-java-9/
@SuppressWarnings("restriction")
private static Unsafe getUnsafe() throws NoSuchFieldException, IllegalAccessException {
Field singleoneInstanceField = Unsafe.class.getDeclaredField("theUnsafe");
singleoneInstanceField.setAccessible(true);
return (Unsafe) singleoneInstanceField.get(null);
}
// http://mishadoff.com/blog/java-magic-part-4-sun-dot-misc-dot-unsafe/
public static void printOffsets(Object o) throws Exception {
Unsafe u = getUnsafe();
Class c = o.getClass();
for (Field f : c.getDeclaredFields()) {
if ((f.getModifiers() & Modifier.STATIC) == 0) {
printOffset(u, f);
}
}
}
public static void printOffset(Unsafe u, Field f) {
long offset = u.objectFieldOffset(f);
System.out.println(f.getName() + ": " + offset);
}
}
java ibm-jvm openj9
I've created a program to check if @sun.misc.Contended
is in effect. The idea is that when @Contended
is in effect, the field offsets in annotated class will be larger.
I can see the expected difference in offsets on OpenJDK, if I specify the -XX:-RestrictContended
flag. I do not see any difference on OpenJ9 11 (jdk-11.0.1+13, Eclipse OpenJ9 VM-11.0.1) through.
The OpenJDK output is
readOnly: 12
writeOnly: 16
----
readOnly: 12
writeOnly: 144
The OpenJ9 output is
readOnly: 8
writeOnly: 12
----
readOnly: 8
writeOnly: 12
The program is
import sun.misc.Unsafe;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
public class Main {
public static class Baseline {
int readOnly;
int writeOnly;
}
public static class Contended {
int readOnly;
@sun.misc.Contended
int writeOnly;
}
public static void main(String args) throws Exception {
Baseline b = new Baseline();
Contended s = new Contended();
printOffsets(b);
System.out.println("----");
printOffsets(s);
}
// https://blog.hazelcast.com/using-sun-misc-unsafe-in-java-9/
@SuppressWarnings("restriction")
private static Unsafe getUnsafe() throws NoSuchFieldException, IllegalAccessException {
Field singleoneInstanceField = Unsafe.class.getDeclaredField("theUnsafe");
singleoneInstanceField.setAccessible(true);
return (Unsafe) singleoneInstanceField.get(null);
}
// http://mishadoff.com/blog/java-magic-part-4-sun-dot-misc-dot-unsafe/
public static void printOffsets(Object o) throws Exception {
Unsafe u = getUnsafe();
Class c = o.getClass();
for (Field f : c.getDeclaredFields()) {
if ((f.getModifiers() & Modifier.STATIC) == 0) {
printOffset(u, f);
}
}
}
public static void printOffset(Unsafe u, Field f) {
long offset = u.objectFieldOffset(f);
System.out.println(f.getName() + ": " + offset);
}
}
java ibm-jvm openj9
java ibm-jvm openj9
asked Nov 16 '18 at 15:48


user7610user7610
8,97446178
8,97446178
helpful discussion on OpenJ9 chat openj9.slack.com/archives/C862YFGL9/p1542383521060200
– user7610
Nov 16 '18 at 16:46
feature request github.com/eclipse/openj9/issues/3716
– user7610
Nov 16 '18 at 19:50
add a comment |
helpful discussion on OpenJ9 chat openj9.slack.com/archives/C862YFGL9/p1542383521060200
– user7610
Nov 16 '18 at 16:46
feature request github.com/eclipse/openj9/issues/3716
– user7610
Nov 16 '18 at 19:50
helpful discussion on OpenJ9 chat openj9.slack.com/archives/C862YFGL9/p1542383521060200
– user7610
Nov 16 '18 at 16:46
helpful discussion on OpenJ9 chat openj9.slack.com/archives/C862YFGL9/p1542383521060200
– user7610
Nov 16 '18 at 16:46
feature request github.com/eclipse/openj9/issues/3716
– user7610
Nov 16 '18 at 19:50
feature request github.com/eclipse/openj9/issues/3716
– user7610
Nov 16 '18 at 19:50
add a comment |
1 Answer
1
active
oldest
votes
At present, OpenJ9 supports @Contended
at the class level only.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53341202%2fdoes-openj9-support-sun-misc-contended-annotation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
At present, OpenJ9 supports @Contended
at the class level only.
add a comment |
At present, OpenJ9 supports @Contended
at the class level only.
add a comment |
At present, OpenJ9 supports @Contended
at the class level only.
At present, OpenJ9 supports @Contended
at the class level only.
answered Nov 16 '18 at 16:44


Peter BainPeter Bain
361
361
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53341202%2fdoes-openj9-support-sun-misc-contended-annotation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Mz,16Na1NB
helpful discussion on OpenJ9 chat openj9.slack.com/archives/C862YFGL9/p1542383521060200
– user7610
Nov 16 '18 at 16:46
feature request github.com/eclipse/openj9/issues/3716
– user7610
Nov 16 '18 at 19:50