React componentDidMount fetch api
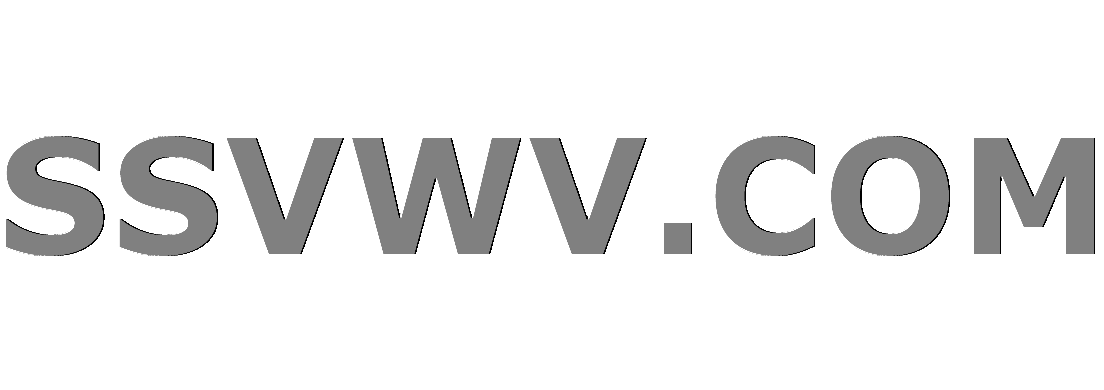
Multi tool use
I am trying to fetch an api inside componentDidMount. The api result will be set to the component's state and the state mapped and passed to a children component.
If I fetch the api using the fetch method inside the componentDidMount everything works fine:
componentDidMount(){
fetch(apiToFetch)
.then((result) => result.json())
.then((result) => result.entries)
.then((entries) => this.setState({ ...this.state, entries }))
.catch((error) => console.log(error));
}
if I use fetch inside a method and then call this method inside componentDidMount nothing is rendered:
componentDidMount() {
this.fetchApiToEntries(GLOBAL_PORTFOLIO_COLLECTION_API);
}
fetchApiToEntries(apiToFetch) {
fetch(apiToFetch)
.then((result) => result.json())
.then((result) => result.entries)
.then((entries) => this.setState({ ...this.state, entries }))
.catch((error) => console.log(error));
}
I cannot understand what I am missing from the lifecycle.
Shouldn't react do the following?
- Init the state
- Render
- Call componentDidMount
- Rerender
Here is my initial component:
export default class Portfolio extends React.Component {
constructor(props) {
super(props);
this.state = {
entries:
}
}
componentDidMount() {
this.fetchApiToEntries(GLOBAL_PORTFOLIO_COLLECTION_API);
}
fetchApiToEntries(apiToFetch) {
fetch(apiToFetch)
.then((result) => result.json())
.then((result) => result.entries)
.then((entries) => {
this.setState({
...this.state,
entries
})
})
.catch((error) => console.log(error));
}
render() {
return (
<Fade bottom>
<div className="Portfolio">
<div className="Portfolio__title"><h4 className="color--gradient text--spacing">WORKS</h4></div>
<OwlCarousel {...options}>
{this.state.entries.map((item) => (
<PortfolioElement item={item} />
))}
</OwlCarousel>
<AnchorLink href='#contact'><Button className="contact-button btn--gradient slider-button Services__button">Let's get in touch</Button></AnchorLink>
</div>
</Fade>
)
}
}
PortfolioElement is the actual component not being rendered.
Any advice?
Thank you.
Edit: both methods are not rerendering the component the right way (...something I didn't expect: I don't know why but if I call them twice in componentDidMount the component will render the right way). I think I am missing something in the state.
I have no error in the console and this is how I set my initial state:
this.state={entries:}
and this is what the actual entries looks like from the console:
entries:
[0:{credits: "..."
description: "..."
featuredImage: {path: "portfolio/01.jpg"}
firstImage: {path: "portfolio/firstimage.jpg"}
secondImage:
slug: "..."
tasks: (5) [{…}, {…}, {…}, {…}, {…}]
title: "..."
_by: "5beae6553362340b040001ee"
_created: 1542123322
_id: "5beaef3a3362340bf70000b4"
_mby: "5beae6553362340b040001ee"
_modified: 1542149308
},
1:{...}
]
My state after the fetch is the same way.
UPDATE I figured out that the the problem is: when the state changes the component is not rerendering the child with the correct props. I called the API in an higher order component passed down the props and added a componentWillUpdate method forcing a state refresh that rerenders the component. Not the ideal solution but I am not figuring out other ways until now. Any advice?
javascript reactjs
add a comment |
I am trying to fetch an api inside componentDidMount. The api result will be set to the component's state and the state mapped and passed to a children component.
If I fetch the api using the fetch method inside the componentDidMount everything works fine:
componentDidMount(){
fetch(apiToFetch)
.then((result) => result.json())
.then((result) => result.entries)
.then((entries) => this.setState({ ...this.state, entries }))
.catch((error) => console.log(error));
}
if I use fetch inside a method and then call this method inside componentDidMount nothing is rendered:
componentDidMount() {
this.fetchApiToEntries(GLOBAL_PORTFOLIO_COLLECTION_API);
}
fetchApiToEntries(apiToFetch) {
fetch(apiToFetch)
.then((result) => result.json())
.then((result) => result.entries)
.then((entries) => this.setState({ ...this.state, entries }))
.catch((error) => console.log(error));
}
I cannot understand what I am missing from the lifecycle.
Shouldn't react do the following?
- Init the state
- Render
- Call componentDidMount
- Rerender
Here is my initial component:
export default class Portfolio extends React.Component {
constructor(props) {
super(props);
this.state = {
entries:
}
}
componentDidMount() {
this.fetchApiToEntries(GLOBAL_PORTFOLIO_COLLECTION_API);
}
fetchApiToEntries(apiToFetch) {
fetch(apiToFetch)
.then((result) => result.json())
.then((result) => result.entries)
.then((entries) => {
this.setState({
...this.state,
entries
})
})
.catch((error) => console.log(error));
}
render() {
return (
<Fade bottom>
<div className="Portfolio">
<div className="Portfolio__title"><h4 className="color--gradient text--spacing">WORKS</h4></div>
<OwlCarousel {...options}>
{this.state.entries.map((item) => (
<PortfolioElement item={item} />
))}
</OwlCarousel>
<AnchorLink href='#contact'><Button className="contact-button btn--gradient slider-button Services__button">Let's get in touch</Button></AnchorLink>
</div>
</Fade>
)
}
}
PortfolioElement is the actual component not being rendered.
Any advice?
Thank you.
Edit: both methods are not rerendering the component the right way (...something I didn't expect: I don't know why but if I call them twice in componentDidMount the component will render the right way). I think I am missing something in the state.
I have no error in the console and this is how I set my initial state:
this.state={entries:}
and this is what the actual entries looks like from the console:
entries:
[0:{credits: "..."
description: "..."
featuredImage: {path: "portfolio/01.jpg"}
firstImage: {path: "portfolio/firstimage.jpg"}
secondImage:
slug: "..."
tasks: (5) [{…}, {…}, {…}, {…}, {…}]
title: "..."
_by: "5beae6553362340b040001ee"
_created: 1542123322
_id: "5beaef3a3362340bf70000b4"
_mby: "5beae6553362340b040001ee"
_modified: 1542149308
},
1:{...}
]
My state after the fetch is the same way.
UPDATE I figured out that the the problem is: when the state changes the component is not rerendering the child with the correct props. I called the API in an higher order component passed down the props and added a componentWillUpdate method forcing a state refresh that rerenders the component. Not the ideal solution but I am not figuring out other ways until now. Any advice?
javascript reactjs
1
I know the method binding in the constructor is missing.
– Filippo Rivolta
Nov 14 '18 at 13:49
3
Shouldn't react do the following? - it should. if I use fetch inside a method and then call this method inside componentDidMount nothing is rendered - this is unlikely. These twocomponentDidMount
snippets should behave exactly the same way, as long asapiToFetch
is the same. Consider providing a way to replicate the problem.
– estus
Nov 14 '18 at 13:54
1
It should work, please check console log for any error or replicate this on codepen so it will be easy for other to debug the issue
– Bheru Lal Lohar
Nov 14 '18 at 14:07
Can you do a log and show us the structure ofresult.entries
? Are you getting error in the console, network or browser?
– Abrar
Nov 14 '18 at 14:08
I get no errors, and I have to edit my question: both methods give the same results. I think I am missing something in my initial state. I will update the question.
– Filippo Rivolta
Nov 14 '18 at 14:21
add a comment |
I am trying to fetch an api inside componentDidMount. The api result will be set to the component's state and the state mapped and passed to a children component.
If I fetch the api using the fetch method inside the componentDidMount everything works fine:
componentDidMount(){
fetch(apiToFetch)
.then((result) => result.json())
.then((result) => result.entries)
.then((entries) => this.setState({ ...this.state, entries }))
.catch((error) => console.log(error));
}
if I use fetch inside a method and then call this method inside componentDidMount nothing is rendered:
componentDidMount() {
this.fetchApiToEntries(GLOBAL_PORTFOLIO_COLLECTION_API);
}
fetchApiToEntries(apiToFetch) {
fetch(apiToFetch)
.then((result) => result.json())
.then((result) => result.entries)
.then((entries) => this.setState({ ...this.state, entries }))
.catch((error) => console.log(error));
}
I cannot understand what I am missing from the lifecycle.
Shouldn't react do the following?
- Init the state
- Render
- Call componentDidMount
- Rerender
Here is my initial component:
export default class Portfolio extends React.Component {
constructor(props) {
super(props);
this.state = {
entries:
}
}
componentDidMount() {
this.fetchApiToEntries(GLOBAL_PORTFOLIO_COLLECTION_API);
}
fetchApiToEntries(apiToFetch) {
fetch(apiToFetch)
.then((result) => result.json())
.then((result) => result.entries)
.then((entries) => {
this.setState({
...this.state,
entries
})
})
.catch((error) => console.log(error));
}
render() {
return (
<Fade bottom>
<div className="Portfolio">
<div className="Portfolio__title"><h4 className="color--gradient text--spacing">WORKS</h4></div>
<OwlCarousel {...options}>
{this.state.entries.map((item) => (
<PortfolioElement item={item} />
))}
</OwlCarousel>
<AnchorLink href='#contact'><Button className="contact-button btn--gradient slider-button Services__button">Let's get in touch</Button></AnchorLink>
</div>
</Fade>
)
}
}
PortfolioElement is the actual component not being rendered.
Any advice?
Thank you.
Edit: both methods are not rerendering the component the right way (...something I didn't expect: I don't know why but if I call them twice in componentDidMount the component will render the right way). I think I am missing something in the state.
I have no error in the console and this is how I set my initial state:
this.state={entries:}
and this is what the actual entries looks like from the console:
entries:
[0:{credits: "..."
description: "..."
featuredImage: {path: "portfolio/01.jpg"}
firstImage: {path: "portfolio/firstimage.jpg"}
secondImage:
slug: "..."
tasks: (5) [{…}, {…}, {…}, {…}, {…}]
title: "..."
_by: "5beae6553362340b040001ee"
_created: 1542123322
_id: "5beaef3a3362340bf70000b4"
_mby: "5beae6553362340b040001ee"
_modified: 1542149308
},
1:{...}
]
My state after the fetch is the same way.
UPDATE I figured out that the the problem is: when the state changes the component is not rerendering the child with the correct props. I called the API in an higher order component passed down the props and added a componentWillUpdate method forcing a state refresh that rerenders the component. Not the ideal solution but I am not figuring out other ways until now. Any advice?
javascript reactjs
I am trying to fetch an api inside componentDidMount. The api result will be set to the component's state and the state mapped and passed to a children component.
If I fetch the api using the fetch method inside the componentDidMount everything works fine:
componentDidMount(){
fetch(apiToFetch)
.then((result) => result.json())
.then((result) => result.entries)
.then((entries) => this.setState({ ...this.state, entries }))
.catch((error) => console.log(error));
}
if I use fetch inside a method and then call this method inside componentDidMount nothing is rendered:
componentDidMount() {
this.fetchApiToEntries(GLOBAL_PORTFOLIO_COLLECTION_API);
}
fetchApiToEntries(apiToFetch) {
fetch(apiToFetch)
.then((result) => result.json())
.then((result) => result.entries)
.then((entries) => this.setState({ ...this.state, entries }))
.catch((error) => console.log(error));
}
I cannot understand what I am missing from the lifecycle.
Shouldn't react do the following?
- Init the state
- Render
- Call componentDidMount
- Rerender
Here is my initial component:
export default class Portfolio extends React.Component {
constructor(props) {
super(props);
this.state = {
entries:
}
}
componentDidMount() {
this.fetchApiToEntries(GLOBAL_PORTFOLIO_COLLECTION_API);
}
fetchApiToEntries(apiToFetch) {
fetch(apiToFetch)
.then((result) => result.json())
.then((result) => result.entries)
.then((entries) => {
this.setState({
...this.state,
entries
})
})
.catch((error) => console.log(error));
}
render() {
return (
<Fade bottom>
<div className="Portfolio">
<div className="Portfolio__title"><h4 className="color--gradient text--spacing">WORKS</h4></div>
<OwlCarousel {...options}>
{this.state.entries.map((item) => (
<PortfolioElement item={item} />
))}
</OwlCarousel>
<AnchorLink href='#contact'><Button className="contact-button btn--gradient slider-button Services__button">Let's get in touch</Button></AnchorLink>
</div>
</Fade>
)
}
}
PortfolioElement is the actual component not being rendered.
Any advice?
Thank you.
Edit: both methods are not rerendering the component the right way (...something I didn't expect: I don't know why but if I call them twice in componentDidMount the component will render the right way). I think I am missing something in the state.
I have no error in the console and this is how I set my initial state:
this.state={entries:}
and this is what the actual entries looks like from the console:
entries:
[0:{credits: "..."
description: "..."
featuredImage: {path: "portfolio/01.jpg"}
firstImage: {path: "portfolio/firstimage.jpg"}
secondImage:
slug: "..."
tasks: (5) [{…}, {…}, {…}, {…}, {…}]
title: "..."
_by: "5beae6553362340b040001ee"
_created: 1542123322
_id: "5beaef3a3362340bf70000b4"
_mby: "5beae6553362340b040001ee"
_modified: 1542149308
},
1:{...}
]
My state after the fetch is the same way.
UPDATE I figured out that the the problem is: when the state changes the component is not rerendering the child with the correct props. I called the API in an higher order component passed down the props and added a componentWillUpdate method forcing a state refresh that rerenders the component. Not the ideal solution but I am not figuring out other ways until now. Any advice?
javascript reactjs
javascript reactjs
edited Nov 14 '18 at 16:30
Filippo Rivolta
asked Nov 14 '18 at 13:46


Filippo RivoltaFilippo Rivolta
165
165
1
I know the method binding in the constructor is missing.
– Filippo Rivolta
Nov 14 '18 at 13:49
3
Shouldn't react do the following? - it should. if I use fetch inside a method and then call this method inside componentDidMount nothing is rendered - this is unlikely. These twocomponentDidMount
snippets should behave exactly the same way, as long asapiToFetch
is the same. Consider providing a way to replicate the problem.
– estus
Nov 14 '18 at 13:54
1
It should work, please check console log for any error or replicate this on codepen so it will be easy for other to debug the issue
– Bheru Lal Lohar
Nov 14 '18 at 14:07
Can you do a log and show us the structure ofresult.entries
? Are you getting error in the console, network or browser?
– Abrar
Nov 14 '18 at 14:08
I get no errors, and I have to edit my question: both methods give the same results. I think I am missing something in my initial state. I will update the question.
– Filippo Rivolta
Nov 14 '18 at 14:21
add a comment |
1
I know the method binding in the constructor is missing.
– Filippo Rivolta
Nov 14 '18 at 13:49
3
Shouldn't react do the following? - it should. if I use fetch inside a method and then call this method inside componentDidMount nothing is rendered - this is unlikely. These twocomponentDidMount
snippets should behave exactly the same way, as long asapiToFetch
is the same. Consider providing a way to replicate the problem.
– estus
Nov 14 '18 at 13:54
1
It should work, please check console log for any error or replicate this on codepen so it will be easy for other to debug the issue
– Bheru Lal Lohar
Nov 14 '18 at 14:07
Can you do a log and show us the structure ofresult.entries
? Are you getting error in the console, network or browser?
– Abrar
Nov 14 '18 at 14:08
I get no errors, and I have to edit my question: both methods give the same results. I think I am missing something in my initial state. I will update the question.
– Filippo Rivolta
Nov 14 '18 at 14:21
1
1
I know the method binding in the constructor is missing.
– Filippo Rivolta
Nov 14 '18 at 13:49
I know the method binding in the constructor is missing.
– Filippo Rivolta
Nov 14 '18 at 13:49
3
3
Shouldn't react do the following? - it should. if I use fetch inside a method and then call this method inside componentDidMount nothing is rendered - this is unlikely. These two
componentDidMount
snippets should behave exactly the same way, as long as apiToFetch
is the same. Consider providing a way to replicate the problem.– estus
Nov 14 '18 at 13:54
Shouldn't react do the following? - it should. if I use fetch inside a method and then call this method inside componentDidMount nothing is rendered - this is unlikely. These two
componentDidMount
snippets should behave exactly the same way, as long as apiToFetch
is the same. Consider providing a way to replicate the problem.– estus
Nov 14 '18 at 13:54
1
1
It should work, please check console log for any error or replicate this on codepen so it will be easy for other to debug the issue
– Bheru Lal Lohar
Nov 14 '18 at 14:07
It should work, please check console log for any error or replicate this on codepen so it will be easy for other to debug the issue
– Bheru Lal Lohar
Nov 14 '18 at 14:07
Can you do a log and show us the structure of
result.entries
? Are you getting error in the console, network or browser?– Abrar
Nov 14 '18 at 14:08
Can you do a log and show us the structure of
result.entries
? Are you getting error in the console, network or browser?– Abrar
Nov 14 '18 at 14:08
I get no errors, and I have to edit my question: both methods give the same results. I think I am missing something in my initial state. I will update the question.
– Filippo Rivolta
Nov 14 '18 at 14:21
I get no errors, and I have to edit my question: both methods give the same results. I think I am missing something in my initial state. I will update the question.
– Filippo Rivolta
Nov 14 '18 at 14:21
add a comment |
2 Answers
2
active
oldest
votes
do you need to bind fetchApiToEntries in the constructor or use fat arrows?
this.fetchApiToEntries = this.fetchApiToEntries.bind(this);
sorry I cant comment not enough rep
2
No, you don't need.
– estus
Nov 14 '18 at 13:52
As stated in my comment, I know it's missing but it is not relevant in this case...
– Filippo Rivolta
Nov 14 '18 at 13:54
break point the bit where its setting the state and check whats in it. as its binding all the ways down ( with the fat arrows) after the fetch and setState will trigger a re-render. my GUESS is this is not the this you want.
– Chris Parsonage
Nov 14 '18 at 14:00
I have edited the question with furter details
– Filippo Rivolta
Nov 14 '18 at 14:36
add a comment |
Idk what your api response is but I tested your code with a fake API and changed
fetchApiToEntries(apiToFetch){}
to Arrow Function (Arrow Function)
fetchApiToEntries = (apiToFetch) => {}
and it's working fine.
Full Example:
export default class Portfolio extends React.Component {
constructor(props) {
super(props);
this.state = {
entries:
}
}
componentDidMount() {
this.fetchApiToEntries('https://jsonplaceholder.typicode.com/posts');
}
fetchApiToEntries = (apiToFetch) => {
fetch(apiToFetch)
.then(result => result.json())
.then((entries) => {
this.setState({
...this.state,
entries
})
})
.catch((error) => console.log(error));
}
render() {
const {entries} = this.state;
console.log(entries);
return (
// Everything you want to render.
)
}
}
Hope it helps you.
1
This code is workable without an arrow, so it's unwanted here.
– estus
Nov 14 '18 at 14:21
Thanks but I have tried the same before, nothing changes, I have posted the api response in the question edit. By the way, mapping the state in a simple <li></li> instead of inside of a children component works fine. Wondering if I have to set the state in an higher order component and pass down the state as props even if I feel it is unnecessary.
– Filippo Rivolta
Nov 14 '18 at 14:48
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53301726%2freact-componentdidmount-fetch-api%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
do you need to bind fetchApiToEntries in the constructor or use fat arrows?
this.fetchApiToEntries = this.fetchApiToEntries.bind(this);
sorry I cant comment not enough rep
2
No, you don't need.
– estus
Nov 14 '18 at 13:52
As stated in my comment, I know it's missing but it is not relevant in this case...
– Filippo Rivolta
Nov 14 '18 at 13:54
break point the bit where its setting the state and check whats in it. as its binding all the ways down ( with the fat arrows) after the fetch and setState will trigger a re-render. my GUESS is this is not the this you want.
– Chris Parsonage
Nov 14 '18 at 14:00
I have edited the question with furter details
– Filippo Rivolta
Nov 14 '18 at 14:36
add a comment |
do you need to bind fetchApiToEntries in the constructor or use fat arrows?
this.fetchApiToEntries = this.fetchApiToEntries.bind(this);
sorry I cant comment not enough rep
2
No, you don't need.
– estus
Nov 14 '18 at 13:52
As stated in my comment, I know it's missing but it is not relevant in this case...
– Filippo Rivolta
Nov 14 '18 at 13:54
break point the bit where its setting the state and check whats in it. as its binding all the ways down ( with the fat arrows) after the fetch and setState will trigger a re-render. my GUESS is this is not the this you want.
– Chris Parsonage
Nov 14 '18 at 14:00
I have edited the question with furter details
– Filippo Rivolta
Nov 14 '18 at 14:36
add a comment |
do you need to bind fetchApiToEntries in the constructor or use fat arrows?
this.fetchApiToEntries = this.fetchApiToEntries.bind(this);
sorry I cant comment not enough rep
do you need to bind fetchApiToEntries in the constructor or use fat arrows?
this.fetchApiToEntries = this.fetchApiToEntries.bind(this);
sorry I cant comment not enough rep
answered Nov 14 '18 at 13:52
Chris ParsonageChris Parsonage
735
735
2
No, you don't need.
– estus
Nov 14 '18 at 13:52
As stated in my comment, I know it's missing but it is not relevant in this case...
– Filippo Rivolta
Nov 14 '18 at 13:54
break point the bit where its setting the state and check whats in it. as its binding all the ways down ( with the fat arrows) after the fetch and setState will trigger a re-render. my GUESS is this is not the this you want.
– Chris Parsonage
Nov 14 '18 at 14:00
I have edited the question with furter details
– Filippo Rivolta
Nov 14 '18 at 14:36
add a comment |
2
No, you don't need.
– estus
Nov 14 '18 at 13:52
As stated in my comment, I know it's missing but it is not relevant in this case...
– Filippo Rivolta
Nov 14 '18 at 13:54
break point the bit where its setting the state and check whats in it. as its binding all the ways down ( with the fat arrows) after the fetch and setState will trigger a re-render. my GUESS is this is not the this you want.
– Chris Parsonage
Nov 14 '18 at 14:00
I have edited the question with furter details
– Filippo Rivolta
Nov 14 '18 at 14:36
2
2
No, you don't need.
– estus
Nov 14 '18 at 13:52
No, you don't need.
– estus
Nov 14 '18 at 13:52
As stated in my comment, I know it's missing but it is not relevant in this case...
– Filippo Rivolta
Nov 14 '18 at 13:54
As stated in my comment, I know it's missing but it is not relevant in this case...
– Filippo Rivolta
Nov 14 '18 at 13:54
break point the bit where its setting the state and check whats in it. as its binding all the ways down ( with the fat arrows) after the fetch and setState will trigger a re-render. my GUESS is this is not the this you want.
– Chris Parsonage
Nov 14 '18 at 14:00
break point the bit where its setting the state and check whats in it. as its binding all the ways down ( with the fat arrows) after the fetch and setState will trigger a re-render. my GUESS is this is not the this you want.
– Chris Parsonage
Nov 14 '18 at 14:00
I have edited the question with furter details
– Filippo Rivolta
Nov 14 '18 at 14:36
I have edited the question with furter details
– Filippo Rivolta
Nov 14 '18 at 14:36
add a comment |
Idk what your api response is but I tested your code with a fake API and changed
fetchApiToEntries(apiToFetch){}
to Arrow Function (Arrow Function)
fetchApiToEntries = (apiToFetch) => {}
and it's working fine.
Full Example:
export default class Portfolio extends React.Component {
constructor(props) {
super(props);
this.state = {
entries:
}
}
componentDidMount() {
this.fetchApiToEntries('https://jsonplaceholder.typicode.com/posts');
}
fetchApiToEntries = (apiToFetch) => {
fetch(apiToFetch)
.then(result => result.json())
.then((entries) => {
this.setState({
...this.state,
entries
})
})
.catch((error) => console.log(error));
}
render() {
const {entries} = this.state;
console.log(entries);
return (
// Everything you want to render.
)
}
}
Hope it helps you.
1
This code is workable without an arrow, so it's unwanted here.
– estus
Nov 14 '18 at 14:21
Thanks but I have tried the same before, nothing changes, I have posted the api response in the question edit. By the way, mapping the state in a simple <li></li> instead of inside of a children component works fine. Wondering if I have to set the state in an higher order component and pass down the state as props even if I feel it is unnecessary.
– Filippo Rivolta
Nov 14 '18 at 14:48
add a comment |
Idk what your api response is but I tested your code with a fake API and changed
fetchApiToEntries(apiToFetch){}
to Arrow Function (Arrow Function)
fetchApiToEntries = (apiToFetch) => {}
and it's working fine.
Full Example:
export default class Portfolio extends React.Component {
constructor(props) {
super(props);
this.state = {
entries:
}
}
componentDidMount() {
this.fetchApiToEntries('https://jsonplaceholder.typicode.com/posts');
}
fetchApiToEntries = (apiToFetch) => {
fetch(apiToFetch)
.then(result => result.json())
.then((entries) => {
this.setState({
...this.state,
entries
})
})
.catch((error) => console.log(error));
}
render() {
const {entries} = this.state;
console.log(entries);
return (
// Everything you want to render.
)
}
}
Hope it helps you.
1
This code is workable without an arrow, so it's unwanted here.
– estus
Nov 14 '18 at 14:21
Thanks but I have tried the same before, nothing changes, I have posted the api response in the question edit. By the way, mapping the state in a simple <li></li> instead of inside of a children component works fine. Wondering if I have to set the state in an higher order component and pass down the state as props even if I feel it is unnecessary.
– Filippo Rivolta
Nov 14 '18 at 14:48
add a comment |
Idk what your api response is but I tested your code with a fake API and changed
fetchApiToEntries(apiToFetch){}
to Arrow Function (Arrow Function)
fetchApiToEntries = (apiToFetch) => {}
and it's working fine.
Full Example:
export default class Portfolio extends React.Component {
constructor(props) {
super(props);
this.state = {
entries:
}
}
componentDidMount() {
this.fetchApiToEntries('https://jsonplaceholder.typicode.com/posts');
}
fetchApiToEntries = (apiToFetch) => {
fetch(apiToFetch)
.then(result => result.json())
.then((entries) => {
this.setState({
...this.state,
entries
})
})
.catch((error) => console.log(error));
}
render() {
const {entries} = this.state;
console.log(entries);
return (
// Everything you want to render.
)
}
}
Hope it helps you.
Idk what your api response is but I tested your code with a fake API and changed
fetchApiToEntries(apiToFetch){}
to Arrow Function (Arrow Function)
fetchApiToEntries = (apiToFetch) => {}
and it's working fine.
Full Example:
export default class Portfolio extends React.Component {
constructor(props) {
super(props);
this.state = {
entries:
}
}
componentDidMount() {
this.fetchApiToEntries('https://jsonplaceholder.typicode.com/posts');
}
fetchApiToEntries = (apiToFetch) => {
fetch(apiToFetch)
.then(result => result.json())
.then((entries) => {
this.setState({
...this.state,
entries
})
})
.catch((error) => console.log(error));
}
render() {
const {entries} = this.state;
console.log(entries);
return (
// Everything you want to render.
)
}
}
Hope it helps you.
answered Nov 14 '18 at 14:16


Majid AmiriMajid Amiri
568
568
1
This code is workable without an arrow, so it's unwanted here.
– estus
Nov 14 '18 at 14:21
Thanks but I have tried the same before, nothing changes, I have posted the api response in the question edit. By the way, mapping the state in a simple <li></li> instead of inside of a children component works fine. Wondering if I have to set the state in an higher order component and pass down the state as props even if I feel it is unnecessary.
– Filippo Rivolta
Nov 14 '18 at 14:48
add a comment |
1
This code is workable without an arrow, so it's unwanted here.
– estus
Nov 14 '18 at 14:21
Thanks but I have tried the same before, nothing changes, I have posted the api response in the question edit. By the way, mapping the state in a simple <li></li> instead of inside of a children component works fine. Wondering if I have to set the state in an higher order component and pass down the state as props even if I feel it is unnecessary.
– Filippo Rivolta
Nov 14 '18 at 14:48
1
1
This code is workable without an arrow, so it's unwanted here.
– estus
Nov 14 '18 at 14:21
This code is workable without an arrow, so it's unwanted here.
– estus
Nov 14 '18 at 14:21
Thanks but I have tried the same before, nothing changes, I have posted the api response in the question edit. By the way, mapping the state in a simple <li></li> instead of inside of a children component works fine. Wondering if I have to set the state in an higher order component and pass down the state as props even if I feel it is unnecessary.
– Filippo Rivolta
Nov 14 '18 at 14:48
Thanks but I have tried the same before, nothing changes, I have posted the api response in the question edit. By the way, mapping the state in a simple <li></li> instead of inside of a children component works fine. Wondering if I have to set the state in an higher order component and pass down the state as props even if I feel it is unnecessary.
– Filippo Rivolta
Nov 14 '18 at 14:48
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53301726%2freact-componentdidmount-fetch-api%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7,QJ,f9CF8 lW,FtW7aZicpmS
1
I know the method binding in the constructor is missing.
– Filippo Rivolta
Nov 14 '18 at 13:49
3
Shouldn't react do the following? - it should. if I use fetch inside a method and then call this method inside componentDidMount nothing is rendered - this is unlikely. These two
componentDidMount
snippets should behave exactly the same way, as long asapiToFetch
is the same. Consider providing a way to replicate the problem.– estus
Nov 14 '18 at 13:54
1
It should work, please check console log for any error or replicate this on codepen so it will be easy for other to debug the issue
– Bheru Lal Lohar
Nov 14 '18 at 14:07
Can you do a log and show us the structure of
result.entries
? Are you getting error in the console, network or browser?– Abrar
Nov 14 '18 at 14:08
I get no errors, and I have to edit my question: both methods give the same results. I think I am missing something in my initial state. I will update the question.
– Filippo Rivolta
Nov 14 '18 at 14:21