C# How to stop a Timer after 10 min
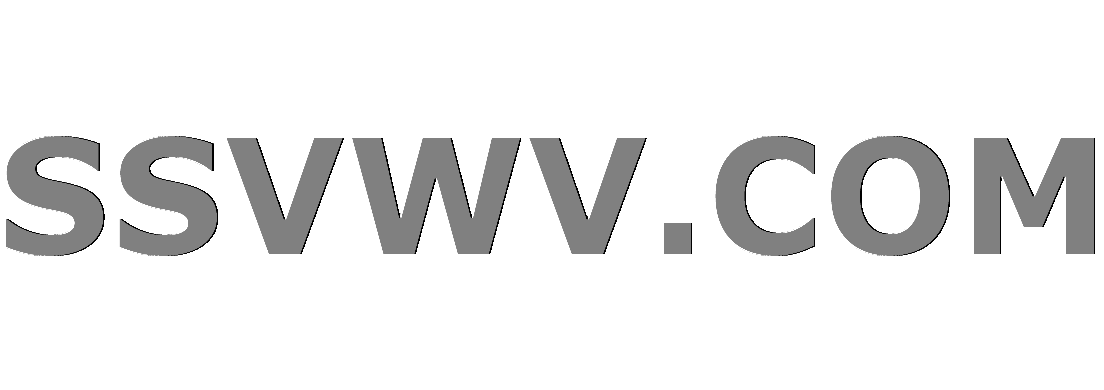
Multi tool use
I tried to write some values after every 10 seconds for 10 minutes in a Text file.
My code looks like this.
private void button12_Click(object sender, RoutedEventArgs e)
{
DispatcherTimer tPOI = new DispatcherTimer();
tPOI.Tick += new System.EventHandler(dispatcherTimer_TickPOI);
tPOI.Interval = new TimeSpan(0, 0, 10);
tPOI.Start();
MessageBox.Show("recording POI");
}
private void dispatcherTimer_TickPOI(object sender, EventArgs e)
{
using (StreamWriter file = new StreamWriter(@textBox36.Text, true))
{
file.WriteLine("pos POI");
file.WriteLine(textBox40.Text);
file.WriteLine(textBox41.Text);
file.WriteLine(textBox42.Text);
file.WriteLine(textBox46.Text);
}
}
But now i don't know how to stop my Timer after 10 minutes. Does anyone have a hint for me?
c# timer
add a comment |
I tried to write some values after every 10 seconds for 10 minutes in a Text file.
My code looks like this.
private void button12_Click(object sender, RoutedEventArgs e)
{
DispatcherTimer tPOI = new DispatcherTimer();
tPOI.Tick += new System.EventHandler(dispatcherTimer_TickPOI);
tPOI.Interval = new TimeSpan(0, 0, 10);
tPOI.Start();
MessageBox.Show("recording POI");
}
private void dispatcherTimer_TickPOI(object sender, EventArgs e)
{
using (StreamWriter file = new StreamWriter(@textBox36.Text, true))
{
file.WriteLine("pos POI");
file.WriteLine(textBox40.Text);
file.WriteLine(textBox41.Text);
file.WriteLine(textBox42.Text);
file.WriteLine(textBox46.Text);
}
}
But now i don't know how to stop my Timer after 10 minutes. Does anyone have a hint for me?
c# timer
6
Call the stop method?
– rory.ap
Nov 14 '18 at 12:28
Use Quartz.NET
– BWA
Nov 14 '18 at 12:29
either you count the amount ofdispatcherTimer_TickPOI
calls: 6 per minute for 10 minutes = 60 times. Or you have a second timer which fires after 10 Minutes and stops the first one
– Mong Zhu
Nov 14 '18 at 12:30
add a comment |
I tried to write some values after every 10 seconds for 10 minutes in a Text file.
My code looks like this.
private void button12_Click(object sender, RoutedEventArgs e)
{
DispatcherTimer tPOI = new DispatcherTimer();
tPOI.Tick += new System.EventHandler(dispatcherTimer_TickPOI);
tPOI.Interval = new TimeSpan(0, 0, 10);
tPOI.Start();
MessageBox.Show("recording POI");
}
private void dispatcherTimer_TickPOI(object sender, EventArgs e)
{
using (StreamWriter file = new StreamWriter(@textBox36.Text, true))
{
file.WriteLine("pos POI");
file.WriteLine(textBox40.Text);
file.WriteLine(textBox41.Text);
file.WriteLine(textBox42.Text);
file.WriteLine(textBox46.Text);
}
}
But now i don't know how to stop my Timer after 10 minutes. Does anyone have a hint for me?
c# timer
I tried to write some values after every 10 seconds for 10 minutes in a Text file.
My code looks like this.
private void button12_Click(object sender, RoutedEventArgs e)
{
DispatcherTimer tPOI = new DispatcherTimer();
tPOI.Tick += new System.EventHandler(dispatcherTimer_TickPOI);
tPOI.Interval = new TimeSpan(0, 0, 10);
tPOI.Start();
MessageBox.Show("recording POI");
}
private void dispatcherTimer_TickPOI(object sender, EventArgs e)
{
using (StreamWriter file = new StreamWriter(@textBox36.Text, true))
{
file.WriteLine("pos POI");
file.WriteLine(textBox40.Text);
file.WriteLine(textBox41.Text);
file.WriteLine(textBox42.Text);
file.WriteLine(textBox46.Text);
}
}
But now i don't know how to stop my Timer after 10 minutes. Does anyone have a hint for me?
c# timer
c# timer
edited Nov 14 '18 at 12:57
Vikas Sonichya
183
183
asked Nov 14 '18 at 12:27


Walter NazarenusWalter Nazarenus
65
65
6
Call the stop method?
– rory.ap
Nov 14 '18 at 12:28
Use Quartz.NET
– BWA
Nov 14 '18 at 12:29
either you count the amount ofdispatcherTimer_TickPOI
calls: 6 per minute for 10 minutes = 60 times. Or you have a second timer which fires after 10 Minutes and stops the first one
– Mong Zhu
Nov 14 '18 at 12:30
add a comment |
6
Call the stop method?
– rory.ap
Nov 14 '18 at 12:28
Use Quartz.NET
– BWA
Nov 14 '18 at 12:29
either you count the amount ofdispatcherTimer_TickPOI
calls: 6 per minute for 10 minutes = 60 times. Or you have a second timer which fires after 10 Minutes and stops the first one
– Mong Zhu
Nov 14 '18 at 12:30
6
6
Call the stop method?
– rory.ap
Nov 14 '18 at 12:28
Call the stop method?
– rory.ap
Nov 14 '18 at 12:28
Use Quartz.NET
– BWA
Nov 14 '18 at 12:29
Use Quartz.NET
– BWA
Nov 14 '18 at 12:29
either you count the amount of
dispatcherTimer_TickPOI
calls: 6 per minute for 10 minutes = 60 times. Or you have a second timer which fires after 10 Minutes and stops the first one– Mong Zhu
Nov 14 '18 at 12:30
either you count the amount of
dispatcherTimer_TickPOI
calls: 6 per minute for 10 minutes = 60 times. Or you have a second timer which fires after 10 Minutes and stops the first one– Mong Zhu
Nov 14 '18 at 12:30
add a comment |
1 Answer
1
active
oldest
votes
You can do something like this. Track the StartTime
and check if it crossed 10 mins.
private DateTime _startTime; //track the start time
private DispatcherTimer tPOI;
private void button12_Click(object sender, RoutedEventArgs e)
{
tPOI = new DispatcherTimer();
tPOI.Tick += new System.EventHandler(dispatcherTimer_TickPOI);
tPOI.Interval = new TimeSpan(0, 0, 10);
_startTime = DateTime.Now; //assign start time when it actually starts
tPOI.Start();
MessageBox.Show("recording POI");
}
private void dispatcherTimer_TickPOI(object sender, EventArgs e)
{
if (_startTime.AddMinutes(10) < DateTime.Now) //check if it has crossed 10 mins
{
tPOI.Stop();
}
else
{
using(StreamWriter file = new StreamWriter(@textBox36.Text, true))
{
file.WriteLine("pos POI");
file.WriteLine(textBox40.Text);
file.WriteLine(textBox41.Text);
file.WriteLine(textBox42.Text);
file.WriteLine(textBox46.Text);
}
}
}
Shouldn't that be_startTime.AddMinutes(10) <= DateTime.Now
?
– Fildor
Nov 14 '18 at 12:39
Ahh! Yes, it should be<
as it should also write on 10th minute.
– mbharanidharan88
Nov 14 '18 at 12:42
1
It probably doesn't matter much in this case due to the small number of invocations (60), but using the pattern above, storing a "stop time" equal to Now+10 minutes in the "Click" method prevents having to add 10 minutes each time you check. Also, in general I would use a count to 60 instead, if it's important that the timer fires exactly "10*60 seconds/10 seconds" times, as suggested above.
– Jesper Matthiesen
Nov 14 '18 at 12:50
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53300249%2fc-sharp-how-to-stop-a-timer-after-10-min%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can do something like this. Track the StartTime
and check if it crossed 10 mins.
private DateTime _startTime; //track the start time
private DispatcherTimer tPOI;
private void button12_Click(object sender, RoutedEventArgs e)
{
tPOI = new DispatcherTimer();
tPOI.Tick += new System.EventHandler(dispatcherTimer_TickPOI);
tPOI.Interval = new TimeSpan(0, 0, 10);
_startTime = DateTime.Now; //assign start time when it actually starts
tPOI.Start();
MessageBox.Show("recording POI");
}
private void dispatcherTimer_TickPOI(object sender, EventArgs e)
{
if (_startTime.AddMinutes(10) < DateTime.Now) //check if it has crossed 10 mins
{
tPOI.Stop();
}
else
{
using(StreamWriter file = new StreamWriter(@textBox36.Text, true))
{
file.WriteLine("pos POI");
file.WriteLine(textBox40.Text);
file.WriteLine(textBox41.Text);
file.WriteLine(textBox42.Text);
file.WriteLine(textBox46.Text);
}
}
}
Shouldn't that be_startTime.AddMinutes(10) <= DateTime.Now
?
– Fildor
Nov 14 '18 at 12:39
Ahh! Yes, it should be<
as it should also write on 10th minute.
– mbharanidharan88
Nov 14 '18 at 12:42
1
It probably doesn't matter much in this case due to the small number of invocations (60), but using the pattern above, storing a "stop time" equal to Now+10 minutes in the "Click" method prevents having to add 10 minutes each time you check. Also, in general I would use a count to 60 instead, if it's important that the timer fires exactly "10*60 seconds/10 seconds" times, as suggested above.
– Jesper Matthiesen
Nov 14 '18 at 12:50
add a comment |
You can do something like this. Track the StartTime
and check if it crossed 10 mins.
private DateTime _startTime; //track the start time
private DispatcherTimer tPOI;
private void button12_Click(object sender, RoutedEventArgs e)
{
tPOI = new DispatcherTimer();
tPOI.Tick += new System.EventHandler(dispatcherTimer_TickPOI);
tPOI.Interval = new TimeSpan(0, 0, 10);
_startTime = DateTime.Now; //assign start time when it actually starts
tPOI.Start();
MessageBox.Show("recording POI");
}
private void dispatcherTimer_TickPOI(object sender, EventArgs e)
{
if (_startTime.AddMinutes(10) < DateTime.Now) //check if it has crossed 10 mins
{
tPOI.Stop();
}
else
{
using(StreamWriter file = new StreamWriter(@textBox36.Text, true))
{
file.WriteLine("pos POI");
file.WriteLine(textBox40.Text);
file.WriteLine(textBox41.Text);
file.WriteLine(textBox42.Text);
file.WriteLine(textBox46.Text);
}
}
}
Shouldn't that be_startTime.AddMinutes(10) <= DateTime.Now
?
– Fildor
Nov 14 '18 at 12:39
Ahh! Yes, it should be<
as it should also write on 10th minute.
– mbharanidharan88
Nov 14 '18 at 12:42
1
It probably doesn't matter much in this case due to the small number of invocations (60), but using the pattern above, storing a "stop time" equal to Now+10 minutes in the "Click" method prevents having to add 10 minutes each time you check. Also, in general I would use a count to 60 instead, if it's important that the timer fires exactly "10*60 seconds/10 seconds" times, as suggested above.
– Jesper Matthiesen
Nov 14 '18 at 12:50
add a comment |
You can do something like this. Track the StartTime
and check if it crossed 10 mins.
private DateTime _startTime; //track the start time
private DispatcherTimer tPOI;
private void button12_Click(object sender, RoutedEventArgs e)
{
tPOI = new DispatcherTimer();
tPOI.Tick += new System.EventHandler(dispatcherTimer_TickPOI);
tPOI.Interval = new TimeSpan(0, 0, 10);
_startTime = DateTime.Now; //assign start time when it actually starts
tPOI.Start();
MessageBox.Show("recording POI");
}
private void dispatcherTimer_TickPOI(object sender, EventArgs e)
{
if (_startTime.AddMinutes(10) < DateTime.Now) //check if it has crossed 10 mins
{
tPOI.Stop();
}
else
{
using(StreamWriter file = new StreamWriter(@textBox36.Text, true))
{
file.WriteLine("pos POI");
file.WriteLine(textBox40.Text);
file.WriteLine(textBox41.Text);
file.WriteLine(textBox42.Text);
file.WriteLine(textBox46.Text);
}
}
}
You can do something like this. Track the StartTime
and check if it crossed 10 mins.
private DateTime _startTime; //track the start time
private DispatcherTimer tPOI;
private void button12_Click(object sender, RoutedEventArgs e)
{
tPOI = new DispatcherTimer();
tPOI.Tick += new System.EventHandler(dispatcherTimer_TickPOI);
tPOI.Interval = new TimeSpan(0, 0, 10);
_startTime = DateTime.Now; //assign start time when it actually starts
tPOI.Start();
MessageBox.Show("recording POI");
}
private void dispatcherTimer_TickPOI(object sender, EventArgs e)
{
if (_startTime.AddMinutes(10) < DateTime.Now) //check if it has crossed 10 mins
{
tPOI.Stop();
}
else
{
using(StreamWriter file = new StreamWriter(@textBox36.Text, true))
{
file.WriteLine("pos POI");
file.WriteLine(textBox40.Text);
file.WriteLine(textBox41.Text);
file.WriteLine(textBox42.Text);
file.WriteLine(textBox46.Text);
}
}
}
edited Nov 14 '18 at 12:42
answered Nov 14 '18 at 12:33
mbharanidharan88mbharanidharan88
4,04442455
4,04442455
Shouldn't that be_startTime.AddMinutes(10) <= DateTime.Now
?
– Fildor
Nov 14 '18 at 12:39
Ahh! Yes, it should be<
as it should also write on 10th minute.
– mbharanidharan88
Nov 14 '18 at 12:42
1
It probably doesn't matter much in this case due to the small number of invocations (60), but using the pattern above, storing a "stop time" equal to Now+10 minutes in the "Click" method prevents having to add 10 minutes each time you check. Also, in general I would use a count to 60 instead, if it's important that the timer fires exactly "10*60 seconds/10 seconds" times, as suggested above.
– Jesper Matthiesen
Nov 14 '18 at 12:50
add a comment |
Shouldn't that be_startTime.AddMinutes(10) <= DateTime.Now
?
– Fildor
Nov 14 '18 at 12:39
Ahh! Yes, it should be<
as it should also write on 10th minute.
– mbharanidharan88
Nov 14 '18 at 12:42
1
It probably doesn't matter much in this case due to the small number of invocations (60), but using the pattern above, storing a "stop time" equal to Now+10 minutes in the "Click" method prevents having to add 10 minutes each time you check. Also, in general I would use a count to 60 instead, if it's important that the timer fires exactly "10*60 seconds/10 seconds" times, as suggested above.
– Jesper Matthiesen
Nov 14 '18 at 12:50
Shouldn't that be
_startTime.AddMinutes(10) <= DateTime.Now
?– Fildor
Nov 14 '18 at 12:39
Shouldn't that be
_startTime.AddMinutes(10) <= DateTime.Now
?– Fildor
Nov 14 '18 at 12:39
Ahh! Yes, it should be
<
as it should also write on 10th minute.– mbharanidharan88
Nov 14 '18 at 12:42
Ahh! Yes, it should be
<
as it should also write on 10th minute.– mbharanidharan88
Nov 14 '18 at 12:42
1
1
It probably doesn't matter much in this case due to the small number of invocations (60), but using the pattern above, storing a "stop time" equal to Now+10 minutes in the "Click" method prevents having to add 10 minutes each time you check. Also, in general I would use a count to 60 instead, if it's important that the timer fires exactly "10*60 seconds/10 seconds" times, as suggested above.
– Jesper Matthiesen
Nov 14 '18 at 12:50
It probably doesn't matter much in this case due to the small number of invocations (60), but using the pattern above, storing a "stop time" equal to Now+10 minutes in the "Click" method prevents having to add 10 minutes each time you check. Also, in general I would use a count to 60 instead, if it's important that the timer fires exactly "10*60 seconds/10 seconds" times, as suggested above.
– Jesper Matthiesen
Nov 14 '18 at 12:50
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53300249%2fc-sharp-how-to-stop-a-timer-after-10-min%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
cd,MtKWh43qOTVZEMRZpX7ebzQccfaglm8e8 eksH Uuf3CgskJJ1Rqr t,kjySvlg,Brkp0iS0n
6
Call the stop method?
– rory.ap
Nov 14 '18 at 12:28
Use Quartz.NET
– BWA
Nov 14 '18 at 12:29
either you count the amount of
dispatcherTimer_TickPOI
calls: 6 per minute for 10 minutes = 60 times. Or you have a second timer which fires after 10 Minutes and stops the first one– Mong Zhu
Nov 14 '18 at 12:30