What's a clean way to get how long a future takes to resolve?
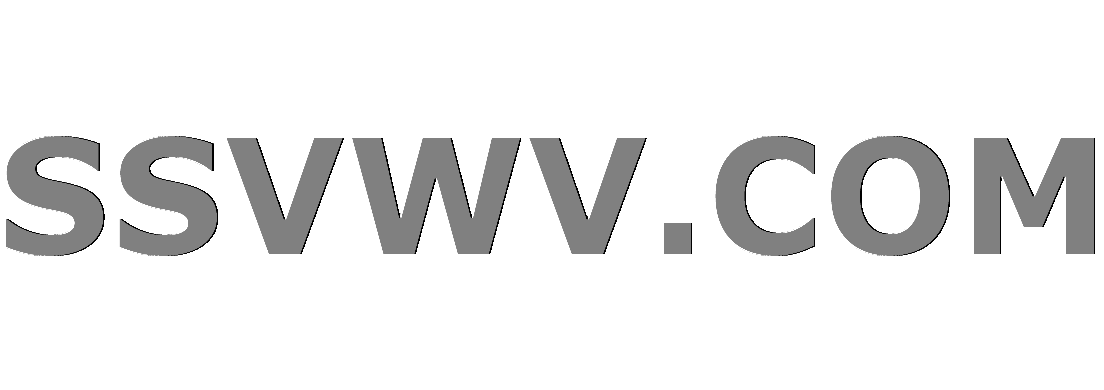
Multi tool use
I'm working with Tokio doing some UDP stuff.
I want to record the amount of time my UDP probe future takes to resolve. I came up with the following function, time_future()
, to wrap a future and give me the result and a duration. The function seems very naive and I think Rust has the power to express the concept much more cleanly.
My working code (Playground):
extern crate futures; // 0.1.25
extern crate tokio; // 0.1.11
use std::time::{Duration, Instant};
use futures::future::{lazy, ok};
use futures::Future;
use tokio::runtime::current_thread::Runtime;
use tokio::timer::Delay;
struct TimedFutureResult<T, E> {
elapsed: Duration,
result: Result<T, E>,
}
impl<T, E> TimedFutureResult<T, E> {
pub fn elapsed_ms(&self) -> i64 {
return (self.elapsed.as_secs() * 1000 + (self.elapsed.subsec_nanos() / 1000000) as u64)
as i64;
}
}
fn time_future<F: Future>(f: F) -> impl Future<Item = TimedFutureResult<F::Item, F::Error>> {
lazy(|| {
let start = Instant::now();
f.then(move |result| {
ok::<TimedFutureResult<F::Item, F::Error>, ()>(TimedFutureResult {
elapsed: start.elapsed(),
result: result,
})
})
})
}
fn main() {
let when = Instant::now() + Duration::from_millis(100);
let f = time_future(Delay::new(when)).then(|r| match r {
Ok(r) => {
println!("resolved in {}ms", r.elapsed_ms());
r.result
}
_ => unreachable!(),
});
let mut runtime = Runtime::new().unwrap();
runtime.block_on(f).unwrap();
}
How can I improve this and make it more idiomatic? Can I somehow get the interface to work similarly to inspect()
or then()
?
Delay::new(when)
.timed(|res, elapsed| println!("{}ms!", elapsed))
.and_then(...);
I tried creating a Timed
trait and implementing it for Future
but I didn't feel at all confident in how I was going about it. The types just really threw me for a loop.
Am I at least barking up the right tree?
rust traits timing rust-tokio
add a comment |
I'm working with Tokio doing some UDP stuff.
I want to record the amount of time my UDP probe future takes to resolve. I came up with the following function, time_future()
, to wrap a future and give me the result and a duration. The function seems very naive and I think Rust has the power to express the concept much more cleanly.
My working code (Playground):
extern crate futures; // 0.1.25
extern crate tokio; // 0.1.11
use std::time::{Duration, Instant};
use futures::future::{lazy, ok};
use futures::Future;
use tokio::runtime::current_thread::Runtime;
use tokio::timer::Delay;
struct TimedFutureResult<T, E> {
elapsed: Duration,
result: Result<T, E>,
}
impl<T, E> TimedFutureResult<T, E> {
pub fn elapsed_ms(&self) -> i64 {
return (self.elapsed.as_secs() * 1000 + (self.elapsed.subsec_nanos() / 1000000) as u64)
as i64;
}
}
fn time_future<F: Future>(f: F) -> impl Future<Item = TimedFutureResult<F::Item, F::Error>> {
lazy(|| {
let start = Instant::now();
f.then(move |result| {
ok::<TimedFutureResult<F::Item, F::Error>, ()>(TimedFutureResult {
elapsed: start.elapsed(),
result: result,
})
})
})
}
fn main() {
let when = Instant::now() + Duration::from_millis(100);
let f = time_future(Delay::new(when)).then(|r| match r {
Ok(r) => {
println!("resolved in {}ms", r.elapsed_ms());
r.result
}
_ => unreachable!(),
});
let mut runtime = Runtime::new().unwrap();
runtime.block_on(f).unwrap();
}
How can I improve this and make it more idiomatic? Can I somehow get the interface to work similarly to inspect()
or then()
?
Delay::new(when)
.timed(|res, elapsed| println!("{}ms!", elapsed))
.and_then(...);
I tried creating a Timed
trait and implementing it for Future
but I didn't feel at all confident in how I was going about it. The types just really threw me for a loop.
Am I at least barking up the right tree?
rust traits timing rust-tokio
Questions asking us to recommend or find a book, tool, software library, tutorial or other off-site resource are off-topic for Stack Overflow — I've removed your sentence pertaining to that. Besides that, this is a top-notch first question; it's clear, concise, provides a code example, etc. Nicely done!
– Shepmaster
Nov 14 '18 at 2:33
add a comment |
I'm working with Tokio doing some UDP stuff.
I want to record the amount of time my UDP probe future takes to resolve. I came up with the following function, time_future()
, to wrap a future and give me the result and a duration. The function seems very naive and I think Rust has the power to express the concept much more cleanly.
My working code (Playground):
extern crate futures; // 0.1.25
extern crate tokio; // 0.1.11
use std::time::{Duration, Instant};
use futures::future::{lazy, ok};
use futures::Future;
use tokio::runtime::current_thread::Runtime;
use tokio::timer::Delay;
struct TimedFutureResult<T, E> {
elapsed: Duration,
result: Result<T, E>,
}
impl<T, E> TimedFutureResult<T, E> {
pub fn elapsed_ms(&self) -> i64 {
return (self.elapsed.as_secs() * 1000 + (self.elapsed.subsec_nanos() / 1000000) as u64)
as i64;
}
}
fn time_future<F: Future>(f: F) -> impl Future<Item = TimedFutureResult<F::Item, F::Error>> {
lazy(|| {
let start = Instant::now();
f.then(move |result| {
ok::<TimedFutureResult<F::Item, F::Error>, ()>(TimedFutureResult {
elapsed: start.elapsed(),
result: result,
})
})
})
}
fn main() {
let when = Instant::now() + Duration::from_millis(100);
let f = time_future(Delay::new(when)).then(|r| match r {
Ok(r) => {
println!("resolved in {}ms", r.elapsed_ms());
r.result
}
_ => unreachable!(),
});
let mut runtime = Runtime::new().unwrap();
runtime.block_on(f).unwrap();
}
How can I improve this and make it more idiomatic? Can I somehow get the interface to work similarly to inspect()
or then()
?
Delay::new(when)
.timed(|res, elapsed| println!("{}ms!", elapsed))
.and_then(...);
I tried creating a Timed
trait and implementing it for Future
but I didn't feel at all confident in how I was going about it. The types just really threw me for a loop.
Am I at least barking up the right tree?
rust traits timing rust-tokio
I'm working with Tokio doing some UDP stuff.
I want to record the amount of time my UDP probe future takes to resolve. I came up with the following function, time_future()
, to wrap a future and give me the result and a duration. The function seems very naive and I think Rust has the power to express the concept much more cleanly.
My working code (Playground):
extern crate futures; // 0.1.25
extern crate tokio; // 0.1.11
use std::time::{Duration, Instant};
use futures::future::{lazy, ok};
use futures::Future;
use tokio::runtime::current_thread::Runtime;
use tokio::timer::Delay;
struct TimedFutureResult<T, E> {
elapsed: Duration,
result: Result<T, E>,
}
impl<T, E> TimedFutureResult<T, E> {
pub fn elapsed_ms(&self) -> i64 {
return (self.elapsed.as_secs() * 1000 + (self.elapsed.subsec_nanos() / 1000000) as u64)
as i64;
}
}
fn time_future<F: Future>(f: F) -> impl Future<Item = TimedFutureResult<F::Item, F::Error>> {
lazy(|| {
let start = Instant::now();
f.then(move |result| {
ok::<TimedFutureResult<F::Item, F::Error>, ()>(TimedFutureResult {
elapsed: start.elapsed(),
result: result,
})
})
})
}
fn main() {
let when = Instant::now() + Duration::from_millis(100);
let f = time_future(Delay::new(when)).then(|r| match r {
Ok(r) => {
println!("resolved in {}ms", r.elapsed_ms());
r.result
}
_ => unreachable!(),
});
let mut runtime = Runtime::new().unwrap();
runtime.block_on(f).unwrap();
}
How can I improve this and make it more idiomatic? Can I somehow get the interface to work similarly to inspect()
or then()
?
Delay::new(when)
.timed(|res, elapsed| println!("{}ms!", elapsed))
.and_then(...);
I tried creating a Timed
trait and implementing it for Future
but I didn't feel at all confident in how I was going about it. The types just really threw me for a loop.
Am I at least barking up the right tree?
rust traits timing rust-tokio
rust traits timing rust-tokio
edited Nov 14 '18 at 2:32
Shepmaster
151k14296434
151k14296434
asked Nov 14 '18 at 0:42
user2460955user2460955
233
233
Questions asking us to recommend or find a book, tool, software library, tutorial or other off-site resource are off-topic for Stack Overflow — I've removed your sentence pertaining to that. Besides that, this is a top-notch first question; it's clear, concise, provides a code example, etc. Nicely done!
– Shepmaster
Nov 14 '18 at 2:33
add a comment |
Questions asking us to recommend or find a book, tool, software library, tutorial or other off-site resource are off-topic for Stack Overflow — I've removed your sentence pertaining to that. Besides that, this is a top-notch first question; it's clear, concise, provides a code example, etc. Nicely done!
– Shepmaster
Nov 14 '18 at 2:33
Questions asking us to recommend or find a book, tool, software library, tutorial or other off-site resource are off-topic for Stack Overflow — I've removed your sentence pertaining to that. Besides that, this is a top-notch first question; it's clear, concise, provides a code example, etc. Nicely done!
– Shepmaster
Nov 14 '18 at 2:33
Questions asking us to recommend or find a book, tool, software library, tutorial or other off-site resource are off-topic for Stack Overflow — I've removed your sentence pertaining to that. Besides that, this is a top-notch first question; it's clear, concise, provides a code example, etc. Nicely done!
– Shepmaster
Nov 14 '18 at 2:33
add a comment |
1 Answer
1
active
oldest
votes
The act of writing the future is easy enough, and adding a chainable method is the same technique as that shown in How can I add new methods to Iterator?.
The only really tricky aspect is deciding when the time starts — is it when the future is created or when it is first polled?
I chose to use when it's first polled, as that seems more useful:
extern crate futures; // 0.1.25
extern crate tokio; // 0.1.11
use std::time::{Duration, Instant};
use futures::{try_ready, Async, Future, Poll};
use tokio::{runtime::current_thread::Runtime, timer::Delay};
struct Timed<Fut, F>
where
Fut: Future,
F: FnMut(&Fut::Item, Duration),
{
inner: Fut,
f: F,
start: Option<Instant>,
}
impl<Fut, F> Future for Timed<Fut, F>
where
Fut: Future,
F: FnMut(&Fut::Item, Duration),
{
type Item = Fut::Item;
type Error = Fut::Error;
fn poll(&mut self) -> Poll<Self::Item, Self::Error> {
let start = self.start.get_or_insert_with(Instant::now);
let v = try_ready!(self.inner.poll());
let elapsed = start.elapsed();
(self.f)(&v, elapsed);
Ok(Async::Ready(v))
}
}
trait TimedExt: Sized + Future {
fn timed<F>(self, f: F) -> Timed<Self, F>
where
F: FnMut(&Self::Item, Duration),
{
Timed {
inner: self,
f,
start: None,
}
}
}
impl<F: Future> TimedExt for F {}
fn main() {
let when = Instant::now() + Duration::from_millis(100);
let f = Delay::new(when).timed(|res, elapsed| println!("{:?} elapsed, got {:?}", elapsed, res));
let mut runtime = Runtime::new().unwrap();
runtime.block_on(f).unwrap();
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53291554%2fwhats-a-clean-way-to-get-how-long-a-future-takes-to-resolve%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The act of writing the future is easy enough, and adding a chainable method is the same technique as that shown in How can I add new methods to Iterator?.
The only really tricky aspect is deciding when the time starts — is it when the future is created or when it is first polled?
I chose to use when it's first polled, as that seems more useful:
extern crate futures; // 0.1.25
extern crate tokio; // 0.1.11
use std::time::{Duration, Instant};
use futures::{try_ready, Async, Future, Poll};
use tokio::{runtime::current_thread::Runtime, timer::Delay};
struct Timed<Fut, F>
where
Fut: Future,
F: FnMut(&Fut::Item, Duration),
{
inner: Fut,
f: F,
start: Option<Instant>,
}
impl<Fut, F> Future for Timed<Fut, F>
where
Fut: Future,
F: FnMut(&Fut::Item, Duration),
{
type Item = Fut::Item;
type Error = Fut::Error;
fn poll(&mut self) -> Poll<Self::Item, Self::Error> {
let start = self.start.get_or_insert_with(Instant::now);
let v = try_ready!(self.inner.poll());
let elapsed = start.elapsed();
(self.f)(&v, elapsed);
Ok(Async::Ready(v))
}
}
trait TimedExt: Sized + Future {
fn timed<F>(self, f: F) -> Timed<Self, F>
where
F: FnMut(&Self::Item, Duration),
{
Timed {
inner: self,
f,
start: None,
}
}
}
impl<F: Future> TimedExt for F {}
fn main() {
let when = Instant::now() + Duration::from_millis(100);
let f = Delay::new(when).timed(|res, elapsed| println!("{:?} elapsed, got {:?}", elapsed, res));
let mut runtime = Runtime::new().unwrap();
runtime.block_on(f).unwrap();
}
add a comment |
The act of writing the future is easy enough, and adding a chainable method is the same technique as that shown in How can I add new methods to Iterator?.
The only really tricky aspect is deciding when the time starts — is it when the future is created or when it is first polled?
I chose to use when it's first polled, as that seems more useful:
extern crate futures; // 0.1.25
extern crate tokio; // 0.1.11
use std::time::{Duration, Instant};
use futures::{try_ready, Async, Future, Poll};
use tokio::{runtime::current_thread::Runtime, timer::Delay};
struct Timed<Fut, F>
where
Fut: Future,
F: FnMut(&Fut::Item, Duration),
{
inner: Fut,
f: F,
start: Option<Instant>,
}
impl<Fut, F> Future for Timed<Fut, F>
where
Fut: Future,
F: FnMut(&Fut::Item, Duration),
{
type Item = Fut::Item;
type Error = Fut::Error;
fn poll(&mut self) -> Poll<Self::Item, Self::Error> {
let start = self.start.get_or_insert_with(Instant::now);
let v = try_ready!(self.inner.poll());
let elapsed = start.elapsed();
(self.f)(&v, elapsed);
Ok(Async::Ready(v))
}
}
trait TimedExt: Sized + Future {
fn timed<F>(self, f: F) -> Timed<Self, F>
where
F: FnMut(&Self::Item, Duration),
{
Timed {
inner: self,
f,
start: None,
}
}
}
impl<F: Future> TimedExt for F {}
fn main() {
let when = Instant::now() + Duration::from_millis(100);
let f = Delay::new(when).timed(|res, elapsed| println!("{:?} elapsed, got {:?}", elapsed, res));
let mut runtime = Runtime::new().unwrap();
runtime.block_on(f).unwrap();
}
add a comment |
The act of writing the future is easy enough, and adding a chainable method is the same technique as that shown in How can I add new methods to Iterator?.
The only really tricky aspect is deciding when the time starts — is it when the future is created or when it is first polled?
I chose to use when it's first polled, as that seems more useful:
extern crate futures; // 0.1.25
extern crate tokio; // 0.1.11
use std::time::{Duration, Instant};
use futures::{try_ready, Async, Future, Poll};
use tokio::{runtime::current_thread::Runtime, timer::Delay};
struct Timed<Fut, F>
where
Fut: Future,
F: FnMut(&Fut::Item, Duration),
{
inner: Fut,
f: F,
start: Option<Instant>,
}
impl<Fut, F> Future for Timed<Fut, F>
where
Fut: Future,
F: FnMut(&Fut::Item, Duration),
{
type Item = Fut::Item;
type Error = Fut::Error;
fn poll(&mut self) -> Poll<Self::Item, Self::Error> {
let start = self.start.get_or_insert_with(Instant::now);
let v = try_ready!(self.inner.poll());
let elapsed = start.elapsed();
(self.f)(&v, elapsed);
Ok(Async::Ready(v))
}
}
trait TimedExt: Sized + Future {
fn timed<F>(self, f: F) -> Timed<Self, F>
where
F: FnMut(&Self::Item, Duration),
{
Timed {
inner: self,
f,
start: None,
}
}
}
impl<F: Future> TimedExt for F {}
fn main() {
let when = Instant::now() + Duration::from_millis(100);
let f = Delay::new(when).timed(|res, elapsed| println!("{:?} elapsed, got {:?}", elapsed, res));
let mut runtime = Runtime::new().unwrap();
runtime.block_on(f).unwrap();
}
The act of writing the future is easy enough, and adding a chainable method is the same technique as that shown in How can I add new methods to Iterator?.
The only really tricky aspect is deciding when the time starts — is it when the future is created or when it is first polled?
I chose to use when it's first polled, as that seems more useful:
extern crate futures; // 0.1.25
extern crate tokio; // 0.1.11
use std::time::{Duration, Instant};
use futures::{try_ready, Async, Future, Poll};
use tokio::{runtime::current_thread::Runtime, timer::Delay};
struct Timed<Fut, F>
where
Fut: Future,
F: FnMut(&Fut::Item, Duration),
{
inner: Fut,
f: F,
start: Option<Instant>,
}
impl<Fut, F> Future for Timed<Fut, F>
where
Fut: Future,
F: FnMut(&Fut::Item, Duration),
{
type Item = Fut::Item;
type Error = Fut::Error;
fn poll(&mut self) -> Poll<Self::Item, Self::Error> {
let start = self.start.get_or_insert_with(Instant::now);
let v = try_ready!(self.inner.poll());
let elapsed = start.elapsed();
(self.f)(&v, elapsed);
Ok(Async::Ready(v))
}
}
trait TimedExt: Sized + Future {
fn timed<F>(self, f: F) -> Timed<Self, F>
where
F: FnMut(&Self::Item, Duration),
{
Timed {
inner: self,
f,
start: None,
}
}
}
impl<F: Future> TimedExt for F {}
fn main() {
let when = Instant::now() + Duration::from_millis(100);
let f = Delay::new(when).timed(|res, elapsed| println!("{:?} elapsed, got {:?}", elapsed, res));
let mut runtime = Runtime::new().unwrap();
runtime.block_on(f).unwrap();
}
answered Nov 14 '18 at 3:12
ShepmasterShepmaster
151k14296434
151k14296434
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53291554%2fwhats-a-clean-way-to-get-how-long-a-future-takes-to-resolve%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
E,JHK U1904gs e2SA1,fU0 I13vsL3,a C9Xp 7HPk MlxhFT6M0jQUVEqxLi4kvA,4JNCb,DrrQkZ,phK0ufd vnU2,hA6d84bTo0hzqbM,0yT
Questions asking us to recommend or find a book, tool, software library, tutorial or other off-site resource are off-topic for Stack Overflow — I've removed your sentence pertaining to that. Besides that, this is a top-notch first question; it's clear, concise, provides a code example, etc. Nicely done!
– Shepmaster
Nov 14 '18 at 2:33