Does std::optional change signature of the function?
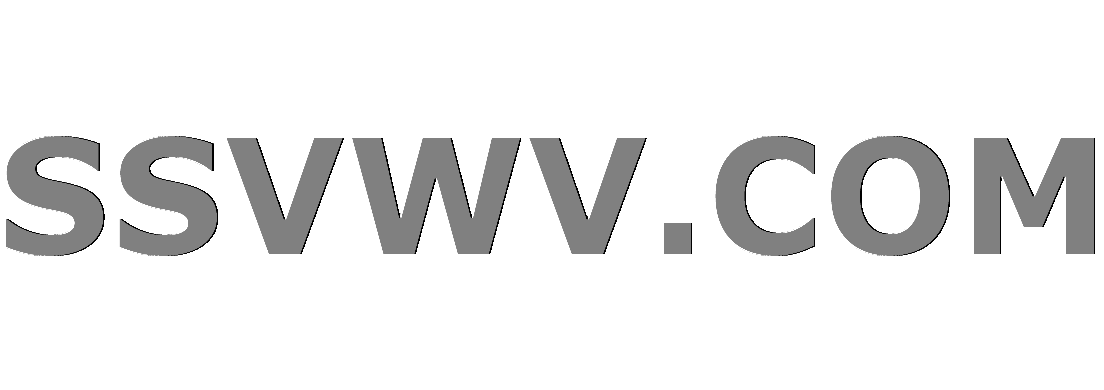
Multi tool use
I need to make an optional argument with a default value in my function. Currently the signature looks something like this:
void func(int a, std::optional<int> b = 10)
and the function behaves in the following way:
func(15, 5); // works
func(15); // works
The question is: If I remove the explicit initialization for the optional argument, like this:
void func(int a, std::optional<int> b)
Then It seems like the signature of the function changes
func(15, 5); // works
func(15); // fails
Which makes me very confused about the purpose of the std::optional
in the first place. What is it good for if not for creating optional arguments?
c++ c++17 stdoptional
add a comment |
I need to make an optional argument with a default value in my function. Currently the signature looks something like this:
void func(int a, std::optional<int> b = 10)
and the function behaves in the following way:
func(15, 5); // works
func(15); // works
The question is: If I remove the explicit initialization for the optional argument, like this:
void func(int a, std::optional<int> b)
Then It seems like the signature of the function changes
func(15, 5); // works
func(15); // fails
Which makes me very confused about the purpose of the std::optional
in the first place. What is it good for if not for creating optional arguments?
c++ c++17 stdoptional
1
std::optional
is a class just like any other so it must be initialized. The only way you can omit an argument is when one has a default argument.
– 0x499602D2
Nov 14 '18 at 0:38
It does not make much sense to have anoptional
with a default value that is not empty as it would only confuse people.
– Phil1970
Nov 14 '18 at 2:51
add a comment |
I need to make an optional argument with a default value in my function. Currently the signature looks something like this:
void func(int a, std::optional<int> b = 10)
and the function behaves in the following way:
func(15, 5); // works
func(15); // works
The question is: If I remove the explicit initialization for the optional argument, like this:
void func(int a, std::optional<int> b)
Then It seems like the signature of the function changes
func(15, 5); // works
func(15); // fails
Which makes me very confused about the purpose of the std::optional
in the first place. What is it good for if not for creating optional arguments?
c++ c++17 stdoptional
I need to make an optional argument with a default value in my function. Currently the signature looks something like this:
void func(int a, std::optional<int> b = 10)
and the function behaves in the following way:
func(15, 5); // works
func(15); // works
The question is: If I remove the explicit initialization for the optional argument, like this:
void func(int a, std::optional<int> b)
Then It seems like the signature of the function changes
func(15, 5); // works
func(15); // fails
Which makes me very confused about the purpose of the std::optional
in the first place. What is it good for if not for creating optional arguments?
c++ c++17 stdoptional
c++ c++17 stdoptional
edited Nov 14 '18 at 1:18
songyuanyao
90.5k11171234
90.5k11171234
asked Nov 14 '18 at 0:34
nikolaevranikolaevra
696
696
1
std::optional
is a class just like any other so it must be initialized. The only way you can omit an argument is when one has a default argument.
– 0x499602D2
Nov 14 '18 at 0:38
It does not make much sense to have anoptional
with a default value that is not empty as it would only confuse people.
– Phil1970
Nov 14 '18 at 2:51
add a comment |
1
std::optional
is a class just like any other so it must be initialized. The only way you can omit an argument is when one has a default argument.
– 0x499602D2
Nov 14 '18 at 0:38
It does not make much sense to have anoptional
with a default value that is not empty as it would only confuse people.
– Phil1970
Nov 14 '18 at 2:51
1
1
std::optional
is a class just like any other so it must be initialized. The only way you can omit an argument is when one has a default argument.– 0x499602D2
Nov 14 '18 at 0:38
std::optional
is a class just like any other so it must be initialized. The only way you can omit an argument is when one has a default argument.– 0x499602D2
Nov 14 '18 at 0:38
It does not make much sense to have an
optional
with a default value that is not empty as it would only confuse people.– Phil1970
Nov 14 '18 at 2:51
It does not make much sense to have an
optional
with a default value that is not empty as it would only confuse people.– Phil1970
Nov 14 '18 at 2:51
add a comment |
3 Answers
3
active
oldest
votes
What is it good for if not for creating optional arguments?
std::optional
is not supposed to be used for optional argument what you expect; which requires default argument as your 1st code sample showed, std::optional
won't change the language syntax.
The class template
std::optional
manages an optional contained value, i.e. a value that may or may not be present.
You can used it like
void func(int a, std::optional<int> b = std::nullopt) {
if (b) {
// if b contains a value
...
} else {
...
}
}
then
func(15, 5); // b will contain a value (i.e. `5`)
func(15); // b doesn't contain a value
Thank you, makes perfect sense to me. Found it really hard to get to the bottom of this from just cpp docs, so had to ask here.
– nikolaevra
Nov 14 '18 at 1:15
add a comment |
std::optional<int>
is still a concrete type despite being "optional" so, unless you have a default value for it in your function specification, you need to supply one.
You seem to be conflating the two definitions of optional here:
- the concrete type allowing you to store an object or lack thereof; and
- the optionality (if that's even a real word) of function arguments.
They are not the same thing.
add a comment |
Another use: optional return values:
// throws if cannot parse
auto parse_int(const std::string& s) -> int;
// returns std::nullopt if it cannot parse
auto try_parse_int(const std::string& s) -> std::optional<int>
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53291485%2fdoes-stdoptional-change-signature-of-the-function%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
What is it good for if not for creating optional arguments?
std::optional
is not supposed to be used for optional argument what you expect; which requires default argument as your 1st code sample showed, std::optional
won't change the language syntax.
The class template
std::optional
manages an optional contained value, i.e. a value that may or may not be present.
You can used it like
void func(int a, std::optional<int> b = std::nullopt) {
if (b) {
// if b contains a value
...
} else {
...
}
}
then
func(15, 5); // b will contain a value (i.e. `5`)
func(15); // b doesn't contain a value
Thank you, makes perfect sense to me. Found it really hard to get to the bottom of this from just cpp docs, so had to ask here.
– nikolaevra
Nov 14 '18 at 1:15
add a comment |
What is it good for if not for creating optional arguments?
std::optional
is not supposed to be used for optional argument what you expect; which requires default argument as your 1st code sample showed, std::optional
won't change the language syntax.
The class template
std::optional
manages an optional contained value, i.e. a value that may or may not be present.
You can used it like
void func(int a, std::optional<int> b = std::nullopt) {
if (b) {
// if b contains a value
...
} else {
...
}
}
then
func(15, 5); // b will contain a value (i.e. `5`)
func(15); // b doesn't contain a value
Thank you, makes perfect sense to me. Found it really hard to get to the bottom of this from just cpp docs, so had to ask here.
– nikolaevra
Nov 14 '18 at 1:15
add a comment |
What is it good for if not for creating optional arguments?
std::optional
is not supposed to be used for optional argument what you expect; which requires default argument as your 1st code sample showed, std::optional
won't change the language syntax.
The class template
std::optional
manages an optional contained value, i.e. a value that may or may not be present.
You can used it like
void func(int a, std::optional<int> b = std::nullopt) {
if (b) {
// if b contains a value
...
} else {
...
}
}
then
func(15, 5); // b will contain a value (i.e. `5`)
func(15); // b doesn't contain a value
What is it good for if not for creating optional arguments?
std::optional
is not supposed to be used for optional argument what you expect; which requires default argument as your 1st code sample showed, std::optional
won't change the language syntax.
The class template
std::optional
manages an optional contained value, i.e. a value that may or may not be present.
You can used it like
void func(int a, std::optional<int> b = std::nullopt) {
if (b) {
// if b contains a value
...
} else {
...
}
}
then
func(15, 5); // b will contain a value (i.e. `5`)
func(15); // b doesn't contain a value
edited Nov 14 '18 at 4:20
Nicol Bolas
285k33472645
285k33472645
answered Nov 14 '18 at 0:42
songyuanyaosongyuanyao
90.5k11171234
90.5k11171234
Thank you, makes perfect sense to me. Found it really hard to get to the bottom of this from just cpp docs, so had to ask here.
– nikolaevra
Nov 14 '18 at 1:15
add a comment |
Thank you, makes perfect sense to me. Found it really hard to get to the bottom of this from just cpp docs, so had to ask here.
– nikolaevra
Nov 14 '18 at 1:15
Thank you, makes perfect sense to me. Found it really hard to get to the bottom of this from just cpp docs, so had to ask here.
– nikolaevra
Nov 14 '18 at 1:15
Thank you, makes perfect sense to me. Found it really hard to get to the bottom of this from just cpp docs, so had to ask here.
– nikolaevra
Nov 14 '18 at 1:15
add a comment |
std::optional<int>
is still a concrete type despite being "optional" so, unless you have a default value for it in your function specification, you need to supply one.
You seem to be conflating the two definitions of optional here:
- the concrete type allowing you to store an object or lack thereof; and
- the optionality (if that's even a real word) of function arguments.
They are not the same thing.
add a comment |
std::optional<int>
is still a concrete type despite being "optional" so, unless you have a default value for it in your function specification, you need to supply one.
You seem to be conflating the two definitions of optional here:
- the concrete type allowing you to store an object or lack thereof; and
- the optionality (if that's even a real word) of function arguments.
They are not the same thing.
add a comment |
std::optional<int>
is still a concrete type despite being "optional" so, unless you have a default value for it in your function specification, you need to supply one.
You seem to be conflating the two definitions of optional here:
- the concrete type allowing you to store an object or lack thereof; and
- the optionality (if that's even a real word) of function arguments.
They are not the same thing.
std::optional<int>
is still a concrete type despite being "optional" so, unless you have a default value for it in your function specification, you need to supply one.
You seem to be conflating the two definitions of optional here:
- the concrete type allowing you to store an object or lack thereof; and
- the optionality (if that's even a real word) of function arguments.
They are not the same thing.
answered Nov 14 '18 at 0:36


paxdiablopaxdiablo
632k17012451669
632k17012451669
add a comment |
add a comment |
Another use: optional return values:
// throws if cannot parse
auto parse_int(const std::string& s) -> int;
// returns std::nullopt if it cannot parse
auto try_parse_int(const std::string& s) -> std::optional<int>
add a comment |
Another use: optional return values:
// throws if cannot parse
auto parse_int(const std::string& s) -> int;
// returns std::nullopt if it cannot parse
auto try_parse_int(const std::string& s) -> std::optional<int>
add a comment |
Another use: optional return values:
// throws if cannot parse
auto parse_int(const std::string& s) -> int;
// returns std::nullopt if it cannot parse
auto try_parse_int(const std::string& s) -> std::optional<int>
Another use: optional return values:
// throws if cannot parse
auto parse_int(const std::string& s) -> int;
// returns std::nullopt if it cannot parse
auto try_parse_int(const std::string& s) -> std::optional<int>
answered Nov 14 '18 at 4:26
bolovbolov
31k670130
31k670130
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53291485%2fdoes-stdoptional-change-signature-of-the-function%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Olj vF7DgUM7T7,kz3rRq TbifpSAqnClxyStnPVfiDv0ju7sQ,bGRLaisNjZv 3grl ngWZqFAb,kEf8sMNqOb7aQ
1
std::optional
is a class just like any other so it must be initialized. The only way you can omit an argument is when one has a default argument.– 0x499602D2
Nov 14 '18 at 0:38
It does not make much sense to have an
optional
with a default value that is not empty as it would only confuse people.– Phil1970
Nov 14 '18 at 2:51