Convert Data URI to File then append to FormData
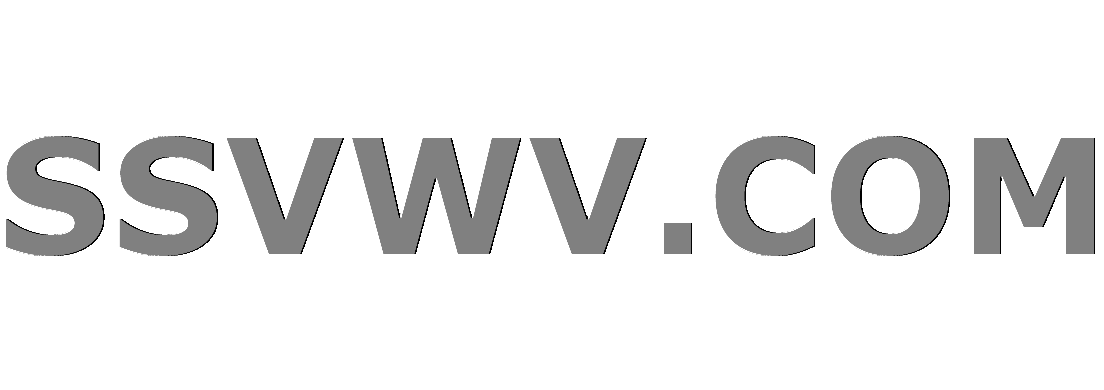
Multi tool use
I've been trying to re-implement an HTML5 image uploader like the one on the Mozilla Hacks site, but that works with WebKit browsers. Part of the task is to extract an image file from the canvas
object and append it to a FormData object for upload.
The issue is that while canvas
has the toDataURL
function to return a representation of the image file, the FormData object only accepts File or Blob objects from the File API.
The Mozilla solution used the following Firefox-only function on canvas
:
var file = canvas.mozGetAsFile("foo.png");
...which isn't available on WebKit browsers. The best solution I could think of is to find some way to convert a Data URI into a File object, which I thought might be part of the File API, but I can't for the life of me find something to do that.
Is it possible? If not, any alternatives?
Thanks.
javascript html5 webkit
add a comment |
I've been trying to re-implement an HTML5 image uploader like the one on the Mozilla Hacks site, but that works with WebKit browsers. Part of the task is to extract an image file from the canvas
object and append it to a FormData object for upload.
The issue is that while canvas
has the toDataURL
function to return a representation of the image file, the FormData object only accepts File or Blob objects from the File API.
The Mozilla solution used the following Firefox-only function on canvas
:
var file = canvas.mozGetAsFile("foo.png");
...which isn't available on WebKit browsers. The best solution I could think of is to find some way to convert a Data URI into a File object, which I thought might be part of the File API, but I can't for the life of me find something to do that.
Is it possible? If not, any alternatives?
Thanks.
javascript html5 webkit
If you want to save the DataURI of an image in server: stackoverflow.com/a/50131281/5466401
– Sibin John Mattappallil
May 2 '18 at 9:49
add a comment |
I've been trying to re-implement an HTML5 image uploader like the one on the Mozilla Hacks site, but that works with WebKit browsers. Part of the task is to extract an image file from the canvas
object and append it to a FormData object for upload.
The issue is that while canvas
has the toDataURL
function to return a representation of the image file, the FormData object only accepts File or Blob objects from the File API.
The Mozilla solution used the following Firefox-only function on canvas
:
var file = canvas.mozGetAsFile("foo.png");
...which isn't available on WebKit browsers. The best solution I could think of is to find some way to convert a Data URI into a File object, which I thought might be part of the File API, but I can't for the life of me find something to do that.
Is it possible? If not, any alternatives?
Thanks.
javascript html5 webkit
I've been trying to re-implement an HTML5 image uploader like the one on the Mozilla Hacks site, but that works with WebKit browsers. Part of the task is to extract an image file from the canvas
object and append it to a FormData object for upload.
The issue is that while canvas
has the toDataURL
function to return a representation of the image file, the FormData object only accepts File or Blob objects from the File API.
The Mozilla solution used the following Firefox-only function on canvas
:
var file = canvas.mozGetAsFile("foo.png");
...which isn't available on WebKit browsers. The best solution I could think of is to find some way to convert a Data URI into a File object, which I thought might be part of the File API, but I can't for the life of me find something to do that.
Is it possible? If not, any alternatives?
Thanks.
javascript html5 webkit
javascript html5 webkit
asked Feb 15 '11 at 0:40
StoiveStoive
8,13642031
8,13642031
If you want to save the DataURI of an image in server: stackoverflow.com/a/50131281/5466401
– Sibin John Mattappallil
May 2 '18 at 9:49
add a comment |
If you want to save the DataURI of an image in server: stackoverflow.com/a/50131281/5466401
– Sibin John Mattappallil
May 2 '18 at 9:49
If you want to save the DataURI of an image in server: stackoverflow.com/a/50131281/5466401
– Sibin John Mattappallil
May 2 '18 at 9:49
If you want to save the DataURI of an image in server: stackoverflow.com/a/50131281/5466401
– Sibin John Mattappallil
May 2 '18 at 9:49
add a comment |
14 Answers
14
active
oldest
votes
After playing around with a few things, I managed to figure this out myself.
First of all, this will convert a dataURI to a Blob:
function dataURItoBlob(dataURI) {
// convert base64/URLEncoded data component to raw binary data held in a string
var byteString;
if (dataURI.split(',')[0].indexOf('base64') >= 0)
byteString = atob(dataURI.split(',')[1]);
else
byteString = unescape(dataURI.split(',')[1]);
// separate out the mime component
var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0];
// write the bytes of the string to a typed array
var ia = new Uint8Array(byteString.length);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return new Blob([ia], {type:mimeString});
}
From there, appending the data to a form such that it will be uploaded as a file is easy:
var dataURL = canvas.toDataURL('image/jpeg', 0.5);
var blob = dataURItoBlob(dataURL);
var fd = new FormData(document.forms[0]);
fd.append("canvasImage", blob);
24
Why does this always happen... You try to solve a problem for hours upon hours with SO searches here and there. Then you post a question. Within an hour you get the answer from another question. Not that I'm complaining... stackoverflow.com/questions/9388412/…
– syaz
Feb 22 '12 at 5:36
1
@mimo - It points to the underlyingArrayBuffer
, which is then written to theBlobBuilder
instance.
– Stoive
Feb 18 '13 at 0:58
2
@stoive In that case why it's not bb.append(ia)?
– Mimo
Feb 18 '13 at 4:13
6
Just use this: github.com/blueimp/JavaScript-Canvas-to-Blob
– TWright
Aug 2 '14 at 3:25
1
@TWright, that's based on this answer.
– Kenny Evitt
Oct 13 '14 at 19:52
|
show 14 more comments
BlobBuilder and ArrayBuffer are now deprecated, here is the top comment's code updated with Blob constructor:
function dataURItoBlob(dataURI) {
var binary = atob(dataURI.split(',')[1]);
var array = ;
for(var i = 0; i < binary.length; i++) {
array.push(binary.charCodeAt(i));
}
return new Blob([new Uint8Array(array)], {type: 'image/jpeg'});
}
2
Just an idea:array=; array.length=binary.length;
...array[i]=bina
... etc. So the array is pre-allocated. It saves a push() having to extend the array each iteration, and we're processing possibly millions of items (=bytes) here, so it matters.
– DDS
Mar 14 '13 at 20:28
5
This doesn't work in Safari.
– William T.
Apr 1 '13 at 23:55
2
Also fails for me on Safari. @WilliamT. 's answer works for Firefox/Safari/Chrome, though.
– ObscureRobot
Apr 10 '13 at 22:29
"binary" is a slightly misleading name, as it is not an array of bits, but an array of bytes.
– Niels Abildgaard
Jun 16 '14 at 8:42
1
"type: 'image/jpeg'" - what if it is a png image OR if you do not know the image extension in advance?
– Jasper
Aug 18 '14 at 10:17
|
show 1 more comment
This one works in iOS and Safari.
You need to use Stoive's ArrayBuffer solution but you can't use BlobBuilder, as vava720 indicates, so here's the mashup of both.
function dataURItoBlob(dataURI) {
var byteString = atob(dataURI.split(',')[1]);
var ab = new ArrayBuffer(byteString.length);
var ia = new Uint8Array(ab);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return new Blob([ab], { type: 'image/jpeg' });
}
11
Great! But you could still keep the mime string dynamic, like in Stoive's solution, I suppose? // separate out the mime component var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0]
– Per Quested Aronsson
Sep 23 '13 at 11:09
What is with the fallback for iOS6 with webkit prefix? How do you handle this?
– confile
Mar 20 '14 at 22:52
add a comment |
Firefox has canvas.toBlob() and canvas.mozGetAsFile() methods.
But other browsers do not.
We can get dataurl from canvas and then convert dataurl to blob object.
Here is my dataURLtoBlob()
function. It's very short.
function dataURLtoBlob(dataurl) {
var arr = dataurl.split(','), mime = arr[0].match(/:(.*?);/)[1],
bstr = atob(arr[1]), n = bstr.length, u8arr = new Uint8Array(n);
while(n--){
u8arr[n] = bstr.charCodeAt(n);
}
return new Blob([u8arr], {type:mime});
}
Use this function with FormData to handle your canvas or dataurl.
For example:
var dataurl = canvas.toDataURL('image/jpeg',0.8);
var blob = dataURLtoBlob(dataurl);
var fd = new FormData();
fd.append("myFile", blob, "thumb.jpg");
Also, you can create a HTMLCanvasElement.prototype.toBlob
method for non gecko engine browser.
if(!HTMLCanvasElement.prototype.toBlob){
HTMLCanvasElement.prototype.toBlob = function(callback, type, encoderOptions){
var dataurl = this.toDataURL(type, encoderOptions);
var bstr = atob(dataurl.split(',')[1]), n = bstr.length, u8arr = new Uint8Array(n);
while(n--){
u8arr[n] = bstr.charCodeAt(n);
}
var blob = new Blob([u8arr], {type: type});
callback.call(this, blob);
};
}
Now canvas.toBlob()
works for all modern browsers not only Firefox.
For example:
canvas.toBlob(
function(blob){
var fd = new FormData();
fd.append("myFile", blob, "thumb.jpg");
//continue do something...
},
'image/jpeg',
0.8
);
1
The polyfill for canvas.toBlob mentioned here is the correct way to handle this issue IMHO.
– Jakob Kruse
Jun 10 '15 at 12:37
1
I would like to emphasize the last thing in this post: "Now canvas.toBlob() works for all modern browsers."
– Eric Simonton
Oct 13 '17 at 13:11
i can't believe that my iphone5 doesn't have the api .toBlob .... i'm crying
– Martian2049
Mar 4 '18 at 23:17
works in IE 11 also for application/pdf !
– Michal.S
Nov 6 '18 at 11:07
add a comment |
Thanks to @Stoive and @vava720 I combined the two in this way, avoiding to use the deprecated BlobBuilder and ArrayBuffer
function dataURItoBlob(dataURI) {
'use strict'
var byteString,
mimestring
if(dataURI.split(',')[0].indexOf('base64') !== -1 ) {
byteString = atob(dataURI.split(',')[1])
} else {
byteString = decodeURI(dataURI.split(',')[1])
}
mimestring = dataURI.split(',')[0].split(':')[1].split(';')[0]
var content = new Array();
for (var i = 0; i < byteString.length; i++) {
content[i] = byteString.charCodeAt(i)
}
return new Blob([new Uint8Array(content)], {type: mimestring});
}
add a comment |
My preferred way is canvas.toBlob()
But anyhow here is yet another way to convert base64 to a blob using fetch ^^,
var url = "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAUAAAAFCAYAAACNbyblAAAAHElEQVQI12P4//8/w38GIAXDIBKE0DHxgljNBAAO9TXL0Y4OHwAAAABJRU5ErkJggg=="
fetch(url)
.then(res => res.blob())
.then(blob => {
var fd = new FormData()
fd.append('image', blob, 'filename')
console.log(blob)
// Upload
// fetch('upload', {method: 'POST', body: fd})
})
what is fetch and how is it relevant?
– Ricardo Freitas
Nov 28 '16 at 17:33
Fetch is a modern ajax method that you can use instead ofXMLHttpRequest
since data url is just a url, You can use ajax to fetch that resource and you got yourself an option to decide if you want it as blob, arraybuffer or text
– Endless
Nov 28 '16 at 17:49
1
@Endless 'fetch()' a local base64 string... a really clever hack!
– Diego ZoracKy
Mar 6 '17 at 21:18
add a comment |
The evolving standard looks to be canvas.toBlob() not canvas.getAsFile() as Mozilla hazarded to guess.
I don't see any browser yet supporting it :(
Thanks for this great thread!
Also, anyone trying the accepted answer should be careful with BlobBuilder as I'm finding support to be limited (and namespaced):
var bb;
try {
bb = new BlobBuilder();
} catch(e) {
try {
bb = new WebKitBlobBuilder();
} catch(e) {
bb = new MozBlobBuilder();
}
}
Were you using another library's polyfill for BlobBuilder?
I was using Chrome with no polyfills, and don't recall coming across namespacing. I eagerly anticipatecanvas.toBlob()
- it seems much more appropriate thangetAsFile
.
– Stoive
Jun 28 '11 at 0:45
1
BlobBuilder
seem to be deprecated in favor ofBlob
– sandstrom
Oct 10 '12 at 12:57
BlobBuilder is deprecated and this pattern is awful. Better would be : window.BlobBuilder = (window.BlobBuilder || window.WebKitBlobBuilder || window.MozBlobBuilder || window.MSBlobBuilder); the nested try catches are really ugly and what happens if none of the builders are available?
– Chris Hanson
Sep 12 '13 at 18:05
How is this awful? If an exception is thrown and 1) BlobBuilder doesn't exist then nothing happens and the next block is executed. 2) If it does exist, but an exception is thrown then it is deprecated and shouldn't be used anyway, so it continues into the next try block. Ideally you would check if Blob is supported first, and even before this check for toBlob support
– TaylorMac
Feb 14 '14 at 19:33
add a comment |
var BlobBuilder = (window.MozBlobBuilder || window.WebKitBlobBuilder || window.BlobBuilder);
can be used without the try catch.
Thankx to check_ca. Great work.
1
This will still throw an error if the browser supports the deprecated BlobBuilder. The browser will use the old method if it supports it, even if it supports the new method. This is not desired, see Chris Bosco's approach below
– TaylorMac
Feb 14 '14 at 19:30
add a comment |
The original answer by Stoive is easily fixable by changing the last line to accommodate Blob:
function dataURItoBlob (dataURI) {
// convert base64 to raw binary data held in a string
// doesn't handle URLEncoded DataURIs
var byteString;
if (dataURI.split(',')[0].indexOf('base64') >= 0)
byteString = atob(dataURI.split(',')[1]);
else
byteString = unescape(dataURI.split(',')[1]);
// separate out the mime component
var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0];
// write the bytes of the string to an ArrayBuffer
var ab = new ArrayBuffer(byteString.length);
var ia = new Uint8Array(ab);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
// write the ArrayBuffer to a blob, and you're done
return new Blob([ab],{type: mimeString});
}
add a comment |
Here is an ES6 version of Stoive's answer:
export class ImageDataConverter {
constructor(dataURI) {
this.dataURI = dataURI;
}
getByteString() {
let byteString;
if (this.dataURI.split(',')[0].indexOf('base64') >= 0) {
byteString = atob(this.dataURI.split(',')[1]);
} else {
byteString = decodeURI(this.dataURI.split(',')[1]);
}
return byteString;
}
getMimeString() {
return this.dataURI.split(',')[0].split(':')[1].split(';')[0];
}
convertToTypedArray() {
let byteString = this.getByteString();
let ia = new Uint8Array(byteString.length);
for (let i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return ia;
}
dataURItoBlob() {
let mimeString = this.getMimeString();
let intArray = this.convertToTypedArray();
return new Blob([intArray], {type: mimeString});
}
}
Usage:
const dataURL = canvas.toDataURL('image/jpeg', 0.5);
const blob = new ImageDataConverter(dataURL).dataURItoBlob();
let fd = new FormData(document.forms[0]);
fd.append("canvasImage", blob);
add a comment |
make it simple :D
function dataURItoBlob(dataURI,mime) {
// convert base64 to raw binary data held in a string
// doesn't handle URLEncoded DataURIs
var byteString = window.atob(dataURI);
// separate out the mime component
// write the bytes of the string to an ArrayBuffer
//var ab = new ArrayBuffer(byteString.length);
var ia = new Uint8Array(byteString.length);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
// write the ArrayBuffer to a blob, and you're done
var blob = new Blob([ia], { type: mime });
return blob;
}
add a comment |
Thanks! @steovi for this solution.
I have added support to ES6 version and changed from unescape to dataURI(unescape is deprecated).
converterDataURItoBlob(dataURI) {
let byteString;
let mimeString;
let ia;
if (dataURI.split(',')[0].indexOf('base64') >= 0) {
byteString = atob(dataURI.split(',')[1]);
} else {
byteString = encodeURI(dataURI.split(',')[1]);
}
// separate out the mime component
mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0];
// write the bytes of the string to a typed array
ia = new Uint8Array(byteString.length);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return new Blob([ia], {type:mimeString});
}
add a comment |
toDataURL gives you a string and you can put that string to a hidden input.
Could you please give an example? I don't wan't to upload a base64 string (which is what doing<input type=hidden value="data:..." />
would do), I want to upload the file data (like what<input type="file" />
does, except you're not allowed to set thevalue
property on these).
– Stoive
Feb 24 '11 at 3:11
This should be a comment rather than an answer. Please elaborate the answer with proper explanation. @Cat Chen
– Lucky
Mar 30 '15 at 8:31
add a comment |
I had exactly the same problem as Ravinder Payal, and I've found the answer. Try this:
var dataURL = canvas.toDataURL("image/jpeg");
var name = "image.jpg";
var parseFile = new Parse.File(name, {base64: dataURL.substring(23)});
2
Are you really suggesting to use Parse.com ? You should mention that your answer require dependencies !
– Pierre Maoui
Jul 7 '16 at 14:44
2
WTF ? Why any one will upload base64 code of image to PARSE server and then download?When we can directly upload base64 on our servers and main thing is that it takes same data to upload base64 string or image file. And if you just want to alow user to download the image , you can use thiswindow.open(canvas.toDataURL("image/jpeg"))
– Ravinder Payal
Jul 19 '16 at 18:52
That is coolest answer I have ever seen, LOL
– Humoyun
Oct 4 '18 at 5:09
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f4998908%2fconvert-data-uri-to-file-then-append-to-formdata%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
14 Answers
14
active
oldest
votes
14 Answers
14
active
oldest
votes
active
oldest
votes
active
oldest
votes
After playing around with a few things, I managed to figure this out myself.
First of all, this will convert a dataURI to a Blob:
function dataURItoBlob(dataURI) {
// convert base64/URLEncoded data component to raw binary data held in a string
var byteString;
if (dataURI.split(',')[0].indexOf('base64') >= 0)
byteString = atob(dataURI.split(',')[1]);
else
byteString = unescape(dataURI.split(',')[1]);
// separate out the mime component
var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0];
// write the bytes of the string to a typed array
var ia = new Uint8Array(byteString.length);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return new Blob([ia], {type:mimeString});
}
From there, appending the data to a form such that it will be uploaded as a file is easy:
var dataURL = canvas.toDataURL('image/jpeg', 0.5);
var blob = dataURItoBlob(dataURL);
var fd = new FormData(document.forms[0]);
fd.append("canvasImage", blob);
24
Why does this always happen... You try to solve a problem for hours upon hours with SO searches here and there. Then you post a question. Within an hour you get the answer from another question. Not that I'm complaining... stackoverflow.com/questions/9388412/…
– syaz
Feb 22 '12 at 5:36
1
@mimo - It points to the underlyingArrayBuffer
, which is then written to theBlobBuilder
instance.
– Stoive
Feb 18 '13 at 0:58
2
@stoive In that case why it's not bb.append(ia)?
– Mimo
Feb 18 '13 at 4:13
6
Just use this: github.com/blueimp/JavaScript-Canvas-to-Blob
– TWright
Aug 2 '14 at 3:25
1
@TWright, that's based on this answer.
– Kenny Evitt
Oct 13 '14 at 19:52
|
show 14 more comments
After playing around with a few things, I managed to figure this out myself.
First of all, this will convert a dataURI to a Blob:
function dataURItoBlob(dataURI) {
// convert base64/URLEncoded data component to raw binary data held in a string
var byteString;
if (dataURI.split(',')[0].indexOf('base64') >= 0)
byteString = atob(dataURI.split(',')[1]);
else
byteString = unescape(dataURI.split(',')[1]);
// separate out the mime component
var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0];
// write the bytes of the string to a typed array
var ia = new Uint8Array(byteString.length);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return new Blob([ia], {type:mimeString});
}
From there, appending the data to a form such that it will be uploaded as a file is easy:
var dataURL = canvas.toDataURL('image/jpeg', 0.5);
var blob = dataURItoBlob(dataURL);
var fd = new FormData(document.forms[0]);
fd.append("canvasImage", blob);
24
Why does this always happen... You try to solve a problem for hours upon hours with SO searches here and there. Then you post a question. Within an hour you get the answer from another question. Not that I'm complaining... stackoverflow.com/questions/9388412/…
– syaz
Feb 22 '12 at 5:36
1
@mimo - It points to the underlyingArrayBuffer
, which is then written to theBlobBuilder
instance.
– Stoive
Feb 18 '13 at 0:58
2
@stoive In that case why it's not bb.append(ia)?
– Mimo
Feb 18 '13 at 4:13
6
Just use this: github.com/blueimp/JavaScript-Canvas-to-Blob
– TWright
Aug 2 '14 at 3:25
1
@TWright, that's based on this answer.
– Kenny Evitt
Oct 13 '14 at 19:52
|
show 14 more comments
After playing around with a few things, I managed to figure this out myself.
First of all, this will convert a dataURI to a Blob:
function dataURItoBlob(dataURI) {
// convert base64/URLEncoded data component to raw binary data held in a string
var byteString;
if (dataURI.split(',')[0].indexOf('base64') >= 0)
byteString = atob(dataURI.split(',')[1]);
else
byteString = unescape(dataURI.split(',')[1]);
// separate out the mime component
var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0];
// write the bytes of the string to a typed array
var ia = new Uint8Array(byteString.length);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return new Blob([ia], {type:mimeString});
}
From there, appending the data to a form such that it will be uploaded as a file is easy:
var dataURL = canvas.toDataURL('image/jpeg', 0.5);
var blob = dataURItoBlob(dataURL);
var fd = new FormData(document.forms[0]);
fd.append("canvasImage", blob);
After playing around with a few things, I managed to figure this out myself.
First of all, this will convert a dataURI to a Blob:
function dataURItoBlob(dataURI) {
// convert base64/URLEncoded data component to raw binary data held in a string
var byteString;
if (dataURI.split(',')[0].indexOf('base64') >= 0)
byteString = atob(dataURI.split(',')[1]);
else
byteString = unescape(dataURI.split(',')[1]);
// separate out the mime component
var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0];
// write the bytes of the string to a typed array
var ia = new Uint8Array(byteString.length);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return new Blob([ia], {type:mimeString});
}
From there, appending the data to a form such that it will be uploaded as a file is easy:
var dataURL = canvas.toDataURL('image/jpeg', 0.5);
var blob = dataURItoBlob(dataURL);
var fd = new FormData(document.forms[0]);
fd.append("canvasImage", blob);
edited Oct 20 '14 at 23:04
answered Feb 24 '11 at 3:50
StoiveStoive
8,13642031
8,13642031
24
Why does this always happen... You try to solve a problem for hours upon hours with SO searches here and there. Then you post a question. Within an hour you get the answer from another question. Not that I'm complaining... stackoverflow.com/questions/9388412/…
– syaz
Feb 22 '12 at 5:36
1
@mimo - It points to the underlyingArrayBuffer
, which is then written to theBlobBuilder
instance.
– Stoive
Feb 18 '13 at 0:58
2
@stoive In that case why it's not bb.append(ia)?
– Mimo
Feb 18 '13 at 4:13
6
Just use this: github.com/blueimp/JavaScript-Canvas-to-Blob
– TWright
Aug 2 '14 at 3:25
1
@TWright, that's based on this answer.
– Kenny Evitt
Oct 13 '14 at 19:52
|
show 14 more comments
24
Why does this always happen... You try to solve a problem for hours upon hours with SO searches here and there. Then you post a question. Within an hour you get the answer from another question. Not that I'm complaining... stackoverflow.com/questions/9388412/…
– syaz
Feb 22 '12 at 5:36
1
@mimo - It points to the underlyingArrayBuffer
, which is then written to theBlobBuilder
instance.
– Stoive
Feb 18 '13 at 0:58
2
@stoive In that case why it's not bb.append(ia)?
– Mimo
Feb 18 '13 at 4:13
6
Just use this: github.com/blueimp/JavaScript-Canvas-to-Blob
– TWright
Aug 2 '14 at 3:25
1
@TWright, that's based on this answer.
– Kenny Evitt
Oct 13 '14 at 19:52
24
24
Why does this always happen... You try to solve a problem for hours upon hours with SO searches here and there. Then you post a question. Within an hour you get the answer from another question. Not that I'm complaining... stackoverflow.com/questions/9388412/…
– syaz
Feb 22 '12 at 5:36
Why does this always happen... You try to solve a problem for hours upon hours with SO searches here and there. Then you post a question. Within an hour you get the answer from another question. Not that I'm complaining... stackoverflow.com/questions/9388412/…
– syaz
Feb 22 '12 at 5:36
1
1
@mimo - It points to the underlying
ArrayBuffer
, which is then written to the BlobBuilder
instance.– Stoive
Feb 18 '13 at 0:58
@mimo - It points to the underlying
ArrayBuffer
, which is then written to the BlobBuilder
instance.– Stoive
Feb 18 '13 at 0:58
2
2
@stoive In that case why it's not bb.append(ia)?
– Mimo
Feb 18 '13 at 4:13
@stoive In that case why it's not bb.append(ia)?
– Mimo
Feb 18 '13 at 4:13
6
6
Just use this: github.com/blueimp/JavaScript-Canvas-to-Blob
– TWright
Aug 2 '14 at 3:25
Just use this: github.com/blueimp/JavaScript-Canvas-to-Blob
– TWright
Aug 2 '14 at 3:25
1
1
@TWright, that's based on this answer.
– Kenny Evitt
Oct 13 '14 at 19:52
@TWright, that's based on this answer.
– Kenny Evitt
Oct 13 '14 at 19:52
|
show 14 more comments
BlobBuilder and ArrayBuffer are now deprecated, here is the top comment's code updated with Blob constructor:
function dataURItoBlob(dataURI) {
var binary = atob(dataURI.split(',')[1]);
var array = ;
for(var i = 0; i < binary.length; i++) {
array.push(binary.charCodeAt(i));
}
return new Blob([new Uint8Array(array)], {type: 'image/jpeg'});
}
2
Just an idea:array=; array.length=binary.length;
...array[i]=bina
... etc. So the array is pre-allocated. It saves a push() having to extend the array each iteration, and we're processing possibly millions of items (=bytes) here, so it matters.
– DDS
Mar 14 '13 at 20:28
5
This doesn't work in Safari.
– William T.
Apr 1 '13 at 23:55
2
Also fails for me on Safari. @WilliamT. 's answer works for Firefox/Safari/Chrome, though.
– ObscureRobot
Apr 10 '13 at 22:29
"binary" is a slightly misleading name, as it is not an array of bits, but an array of bytes.
– Niels Abildgaard
Jun 16 '14 at 8:42
1
"type: 'image/jpeg'" - what if it is a png image OR if you do not know the image extension in advance?
– Jasper
Aug 18 '14 at 10:17
|
show 1 more comment
BlobBuilder and ArrayBuffer are now deprecated, here is the top comment's code updated with Blob constructor:
function dataURItoBlob(dataURI) {
var binary = atob(dataURI.split(',')[1]);
var array = ;
for(var i = 0; i < binary.length; i++) {
array.push(binary.charCodeAt(i));
}
return new Blob([new Uint8Array(array)], {type: 'image/jpeg'});
}
2
Just an idea:array=; array.length=binary.length;
...array[i]=bina
... etc. So the array is pre-allocated. It saves a push() having to extend the array each iteration, and we're processing possibly millions of items (=bytes) here, so it matters.
– DDS
Mar 14 '13 at 20:28
5
This doesn't work in Safari.
– William T.
Apr 1 '13 at 23:55
2
Also fails for me on Safari. @WilliamT. 's answer works for Firefox/Safari/Chrome, though.
– ObscureRobot
Apr 10 '13 at 22:29
"binary" is a slightly misleading name, as it is not an array of bits, but an array of bytes.
– Niels Abildgaard
Jun 16 '14 at 8:42
1
"type: 'image/jpeg'" - what if it is a png image OR if you do not know the image extension in advance?
– Jasper
Aug 18 '14 at 10:17
|
show 1 more comment
BlobBuilder and ArrayBuffer are now deprecated, here is the top comment's code updated with Blob constructor:
function dataURItoBlob(dataURI) {
var binary = atob(dataURI.split(',')[1]);
var array = ;
for(var i = 0; i < binary.length; i++) {
array.push(binary.charCodeAt(i));
}
return new Blob([new Uint8Array(array)], {type: 'image/jpeg'});
}
BlobBuilder and ArrayBuffer are now deprecated, here is the top comment's code updated with Blob constructor:
function dataURItoBlob(dataURI) {
var binary = atob(dataURI.split(',')[1]);
var array = ;
for(var i = 0; i < binary.length; i++) {
array.push(binary.charCodeAt(i));
}
return new Blob([new Uint8Array(array)], {type: 'image/jpeg'});
}
answered Aug 14 '12 at 14:19
vava720vava720
1,381182
1,381182
2
Just an idea:array=; array.length=binary.length;
...array[i]=bina
... etc. So the array is pre-allocated. It saves a push() having to extend the array each iteration, and we're processing possibly millions of items (=bytes) here, so it matters.
– DDS
Mar 14 '13 at 20:28
5
This doesn't work in Safari.
– William T.
Apr 1 '13 at 23:55
2
Also fails for me on Safari. @WilliamT. 's answer works for Firefox/Safari/Chrome, though.
– ObscureRobot
Apr 10 '13 at 22:29
"binary" is a slightly misleading name, as it is not an array of bits, but an array of bytes.
– Niels Abildgaard
Jun 16 '14 at 8:42
1
"type: 'image/jpeg'" - what if it is a png image OR if you do not know the image extension in advance?
– Jasper
Aug 18 '14 at 10:17
|
show 1 more comment
2
Just an idea:array=; array.length=binary.length;
...array[i]=bina
... etc. So the array is pre-allocated. It saves a push() having to extend the array each iteration, and we're processing possibly millions of items (=bytes) here, so it matters.
– DDS
Mar 14 '13 at 20:28
5
This doesn't work in Safari.
– William T.
Apr 1 '13 at 23:55
2
Also fails for me on Safari. @WilliamT. 's answer works for Firefox/Safari/Chrome, though.
– ObscureRobot
Apr 10 '13 at 22:29
"binary" is a slightly misleading name, as it is not an array of bits, but an array of bytes.
– Niels Abildgaard
Jun 16 '14 at 8:42
1
"type: 'image/jpeg'" - what if it is a png image OR if you do not know the image extension in advance?
– Jasper
Aug 18 '14 at 10:17
2
2
Just an idea:
array=; array.length=binary.length;
... array[i]=bina
... etc. So the array is pre-allocated. It saves a push() having to extend the array each iteration, and we're processing possibly millions of items (=bytes) here, so it matters.– DDS
Mar 14 '13 at 20:28
Just an idea:
array=; array.length=binary.length;
... array[i]=bina
... etc. So the array is pre-allocated. It saves a push() having to extend the array each iteration, and we're processing possibly millions of items (=bytes) here, so it matters.– DDS
Mar 14 '13 at 20:28
5
5
This doesn't work in Safari.
– William T.
Apr 1 '13 at 23:55
This doesn't work in Safari.
– William T.
Apr 1 '13 at 23:55
2
2
Also fails for me on Safari. @WilliamT. 's answer works for Firefox/Safari/Chrome, though.
– ObscureRobot
Apr 10 '13 at 22:29
Also fails for me on Safari. @WilliamT. 's answer works for Firefox/Safari/Chrome, though.
– ObscureRobot
Apr 10 '13 at 22:29
"binary" is a slightly misleading name, as it is not an array of bits, but an array of bytes.
– Niels Abildgaard
Jun 16 '14 at 8:42
"binary" is a slightly misleading name, as it is not an array of bits, but an array of bytes.
– Niels Abildgaard
Jun 16 '14 at 8:42
1
1
"type: 'image/jpeg'" - what if it is a png image OR if you do not know the image extension in advance?
– Jasper
Aug 18 '14 at 10:17
"type: 'image/jpeg'" - what if it is a png image OR if you do not know the image extension in advance?
– Jasper
Aug 18 '14 at 10:17
|
show 1 more comment
This one works in iOS and Safari.
You need to use Stoive's ArrayBuffer solution but you can't use BlobBuilder, as vava720 indicates, so here's the mashup of both.
function dataURItoBlob(dataURI) {
var byteString = atob(dataURI.split(',')[1]);
var ab = new ArrayBuffer(byteString.length);
var ia = new Uint8Array(ab);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return new Blob([ab], { type: 'image/jpeg' });
}
11
Great! But you could still keep the mime string dynamic, like in Stoive's solution, I suppose? // separate out the mime component var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0]
– Per Quested Aronsson
Sep 23 '13 at 11:09
What is with the fallback for iOS6 with webkit prefix? How do you handle this?
– confile
Mar 20 '14 at 22:52
add a comment |
This one works in iOS and Safari.
You need to use Stoive's ArrayBuffer solution but you can't use BlobBuilder, as vava720 indicates, so here's the mashup of both.
function dataURItoBlob(dataURI) {
var byteString = atob(dataURI.split(',')[1]);
var ab = new ArrayBuffer(byteString.length);
var ia = new Uint8Array(ab);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return new Blob([ab], { type: 'image/jpeg' });
}
11
Great! But you could still keep the mime string dynamic, like in Stoive's solution, I suppose? // separate out the mime component var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0]
– Per Quested Aronsson
Sep 23 '13 at 11:09
What is with the fallback for iOS6 with webkit prefix? How do you handle this?
– confile
Mar 20 '14 at 22:52
add a comment |
This one works in iOS and Safari.
You need to use Stoive's ArrayBuffer solution but you can't use BlobBuilder, as vava720 indicates, so here's the mashup of both.
function dataURItoBlob(dataURI) {
var byteString = atob(dataURI.split(',')[1]);
var ab = new ArrayBuffer(byteString.length);
var ia = new Uint8Array(ab);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return new Blob([ab], { type: 'image/jpeg' });
}
This one works in iOS and Safari.
You need to use Stoive's ArrayBuffer solution but you can't use BlobBuilder, as vava720 indicates, so here's the mashup of both.
function dataURItoBlob(dataURI) {
var byteString = atob(dataURI.split(',')[1]);
var ab = new ArrayBuffer(byteString.length);
var ia = new Uint8Array(ab);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return new Blob([ab], { type: 'image/jpeg' });
}
answered Apr 2 '13 at 0:18
William T.William T.
9,60134342
9,60134342
11
Great! But you could still keep the mime string dynamic, like in Stoive's solution, I suppose? // separate out the mime component var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0]
– Per Quested Aronsson
Sep 23 '13 at 11:09
What is with the fallback for iOS6 with webkit prefix? How do you handle this?
– confile
Mar 20 '14 at 22:52
add a comment |
11
Great! But you could still keep the mime string dynamic, like in Stoive's solution, I suppose? // separate out the mime component var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0]
– Per Quested Aronsson
Sep 23 '13 at 11:09
What is with the fallback for iOS6 with webkit prefix? How do you handle this?
– confile
Mar 20 '14 at 22:52
11
11
Great! But you could still keep the mime string dynamic, like in Stoive's solution, I suppose? // separate out the mime component var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0]
– Per Quested Aronsson
Sep 23 '13 at 11:09
Great! But you could still keep the mime string dynamic, like in Stoive's solution, I suppose? // separate out the mime component var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0]
– Per Quested Aronsson
Sep 23 '13 at 11:09
What is with the fallback for iOS6 with webkit prefix? How do you handle this?
– confile
Mar 20 '14 at 22:52
What is with the fallback for iOS6 with webkit prefix? How do you handle this?
– confile
Mar 20 '14 at 22:52
add a comment |
Firefox has canvas.toBlob() and canvas.mozGetAsFile() methods.
But other browsers do not.
We can get dataurl from canvas and then convert dataurl to blob object.
Here is my dataURLtoBlob()
function. It's very short.
function dataURLtoBlob(dataurl) {
var arr = dataurl.split(','), mime = arr[0].match(/:(.*?);/)[1],
bstr = atob(arr[1]), n = bstr.length, u8arr = new Uint8Array(n);
while(n--){
u8arr[n] = bstr.charCodeAt(n);
}
return new Blob([u8arr], {type:mime});
}
Use this function with FormData to handle your canvas or dataurl.
For example:
var dataurl = canvas.toDataURL('image/jpeg',0.8);
var blob = dataURLtoBlob(dataurl);
var fd = new FormData();
fd.append("myFile", blob, "thumb.jpg");
Also, you can create a HTMLCanvasElement.prototype.toBlob
method for non gecko engine browser.
if(!HTMLCanvasElement.prototype.toBlob){
HTMLCanvasElement.prototype.toBlob = function(callback, type, encoderOptions){
var dataurl = this.toDataURL(type, encoderOptions);
var bstr = atob(dataurl.split(',')[1]), n = bstr.length, u8arr = new Uint8Array(n);
while(n--){
u8arr[n] = bstr.charCodeAt(n);
}
var blob = new Blob([u8arr], {type: type});
callback.call(this, blob);
};
}
Now canvas.toBlob()
works for all modern browsers not only Firefox.
For example:
canvas.toBlob(
function(blob){
var fd = new FormData();
fd.append("myFile", blob, "thumb.jpg");
//continue do something...
},
'image/jpeg',
0.8
);
1
The polyfill for canvas.toBlob mentioned here is the correct way to handle this issue IMHO.
– Jakob Kruse
Jun 10 '15 at 12:37
1
I would like to emphasize the last thing in this post: "Now canvas.toBlob() works for all modern browsers."
– Eric Simonton
Oct 13 '17 at 13:11
i can't believe that my iphone5 doesn't have the api .toBlob .... i'm crying
– Martian2049
Mar 4 '18 at 23:17
works in IE 11 also for application/pdf !
– Michal.S
Nov 6 '18 at 11:07
add a comment |
Firefox has canvas.toBlob() and canvas.mozGetAsFile() methods.
But other browsers do not.
We can get dataurl from canvas and then convert dataurl to blob object.
Here is my dataURLtoBlob()
function. It's very short.
function dataURLtoBlob(dataurl) {
var arr = dataurl.split(','), mime = arr[0].match(/:(.*?);/)[1],
bstr = atob(arr[1]), n = bstr.length, u8arr = new Uint8Array(n);
while(n--){
u8arr[n] = bstr.charCodeAt(n);
}
return new Blob([u8arr], {type:mime});
}
Use this function with FormData to handle your canvas or dataurl.
For example:
var dataurl = canvas.toDataURL('image/jpeg',0.8);
var blob = dataURLtoBlob(dataurl);
var fd = new FormData();
fd.append("myFile", blob, "thumb.jpg");
Also, you can create a HTMLCanvasElement.prototype.toBlob
method for non gecko engine browser.
if(!HTMLCanvasElement.prototype.toBlob){
HTMLCanvasElement.prototype.toBlob = function(callback, type, encoderOptions){
var dataurl = this.toDataURL(type, encoderOptions);
var bstr = atob(dataurl.split(',')[1]), n = bstr.length, u8arr = new Uint8Array(n);
while(n--){
u8arr[n] = bstr.charCodeAt(n);
}
var blob = new Blob([u8arr], {type: type});
callback.call(this, blob);
};
}
Now canvas.toBlob()
works for all modern browsers not only Firefox.
For example:
canvas.toBlob(
function(blob){
var fd = new FormData();
fd.append("myFile", blob, "thumb.jpg");
//continue do something...
},
'image/jpeg',
0.8
);
1
The polyfill for canvas.toBlob mentioned here is the correct way to handle this issue IMHO.
– Jakob Kruse
Jun 10 '15 at 12:37
1
I would like to emphasize the last thing in this post: "Now canvas.toBlob() works for all modern browsers."
– Eric Simonton
Oct 13 '17 at 13:11
i can't believe that my iphone5 doesn't have the api .toBlob .... i'm crying
– Martian2049
Mar 4 '18 at 23:17
works in IE 11 also for application/pdf !
– Michal.S
Nov 6 '18 at 11:07
add a comment |
Firefox has canvas.toBlob() and canvas.mozGetAsFile() methods.
But other browsers do not.
We can get dataurl from canvas and then convert dataurl to blob object.
Here is my dataURLtoBlob()
function. It's very short.
function dataURLtoBlob(dataurl) {
var arr = dataurl.split(','), mime = arr[0].match(/:(.*?);/)[1],
bstr = atob(arr[1]), n = bstr.length, u8arr = new Uint8Array(n);
while(n--){
u8arr[n] = bstr.charCodeAt(n);
}
return new Blob([u8arr], {type:mime});
}
Use this function with FormData to handle your canvas or dataurl.
For example:
var dataurl = canvas.toDataURL('image/jpeg',0.8);
var blob = dataURLtoBlob(dataurl);
var fd = new FormData();
fd.append("myFile", blob, "thumb.jpg");
Also, you can create a HTMLCanvasElement.prototype.toBlob
method for non gecko engine browser.
if(!HTMLCanvasElement.prototype.toBlob){
HTMLCanvasElement.prototype.toBlob = function(callback, type, encoderOptions){
var dataurl = this.toDataURL(type, encoderOptions);
var bstr = atob(dataurl.split(',')[1]), n = bstr.length, u8arr = new Uint8Array(n);
while(n--){
u8arr[n] = bstr.charCodeAt(n);
}
var blob = new Blob([u8arr], {type: type});
callback.call(this, blob);
};
}
Now canvas.toBlob()
works for all modern browsers not only Firefox.
For example:
canvas.toBlob(
function(blob){
var fd = new FormData();
fd.append("myFile", blob, "thumb.jpg");
//continue do something...
},
'image/jpeg',
0.8
);
Firefox has canvas.toBlob() and canvas.mozGetAsFile() methods.
But other browsers do not.
We can get dataurl from canvas and then convert dataurl to blob object.
Here is my dataURLtoBlob()
function. It's very short.
function dataURLtoBlob(dataurl) {
var arr = dataurl.split(','), mime = arr[0].match(/:(.*?);/)[1],
bstr = atob(arr[1]), n = bstr.length, u8arr = new Uint8Array(n);
while(n--){
u8arr[n] = bstr.charCodeAt(n);
}
return new Blob([u8arr], {type:mime});
}
Use this function with FormData to handle your canvas or dataurl.
For example:
var dataurl = canvas.toDataURL('image/jpeg',0.8);
var blob = dataURLtoBlob(dataurl);
var fd = new FormData();
fd.append("myFile", blob, "thumb.jpg");
Also, you can create a HTMLCanvasElement.prototype.toBlob
method for non gecko engine browser.
if(!HTMLCanvasElement.prototype.toBlob){
HTMLCanvasElement.prototype.toBlob = function(callback, type, encoderOptions){
var dataurl = this.toDataURL(type, encoderOptions);
var bstr = atob(dataurl.split(',')[1]), n = bstr.length, u8arr = new Uint8Array(n);
while(n--){
u8arr[n] = bstr.charCodeAt(n);
}
var blob = new Blob([u8arr], {type: type});
callback.call(this, blob);
};
}
Now canvas.toBlob()
works for all modern browsers not only Firefox.
For example:
canvas.toBlob(
function(blob){
var fd = new FormData();
fd.append("myFile", blob, "thumb.jpg");
//continue do something...
},
'image/jpeg',
0.8
);
edited Nov 14 '18 at 0:12


naXa
13.5k889136
13.5k889136
answered May 26 '15 at 22:51


cuixipingcuixiping
11.1k35270
11.1k35270
1
The polyfill for canvas.toBlob mentioned here is the correct way to handle this issue IMHO.
– Jakob Kruse
Jun 10 '15 at 12:37
1
I would like to emphasize the last thing in this post: "Now canvas.toBlob() works for all modern browsers."
– Eric Simonton
Oct 13 '17 at 13:11
i can't believe that my iphone5 doesn't have the api .toBlob .... i'm crying
– Martian2049
Mar 4 '18 at 23:17
works in IE 11 also for application/pdf !
– Michal.S
Nov 6 '18 at 11:07
add a comment |
1
The polyfill for canvas.toBlob mentioned here is the correct way to handle this issue IMHO.
– Jakob Kruse
Jun 10 '15 at 12:37
1
I would like to emphasize the last thing in this post: "Now canvas.toBlob() works for all modern browsers."
– Eric Simonton
Oct 13 '17 at 13:11
i can't believe that my iphone5 doesn't have the api .toBlob .... i'm crying
– Martian2049
Mar 4 '18 at 23:17
works in IE 11 also for application/pdf !
– Michal.S
Nov 6 '18 at 11:07
1
1
The polyfill for canvas.toBlob mentioned here is the correct way to handle this issue IMHO.
– Jakob Kruse
Jun 10 '15 at 12:37
The polyfill for canvas.toBlob mentioned here is the correct way to handle this issue IMHO.
– Jakob Kruse
Jun 10 '15 at 12:37
1
1
I would like to emphasize the last thing in this post: "Now canvas.toBlob() works for all modern browsers."
– Eric Simonton
Oct 13 '17 at 13:11
I would like to emphasize the last thing in this post: "Now canvas.toBlob() works for all modern browsers."
– Eric Simonton
Oct 13 '17 at 13:11
i can't believe that my iphone5 doesn't have the api .toBlob .... i'm crying
– Martian2049
Mar 4 '18 at 23:17
i can't believe that my iphone5 doesn't have the api .toBlob .... i'm crying
– Martian2049
Mar 4 '18 at 23:17
works in IE 11 also for application/pdf !
– Michal.S
Nov 6 '18 at 11:07
works in IE 11 also for application/pdf !
– Michal.S
Nov 6 '18 at 11:07
add a comment |
Thanks to @Stoive and @vava720 I combined the two in this way, avoiding to use the deprecated BlobBuilder and ArrayBuffer
function dataURItoBlob(dataURI) {
'use strict'
var byteString,
mimestring
if(dataURI.split(',')[0].indexOf('base64') !== -1 ) {
byteString = atob(dataURI.split(',')[1])
} else {
byteString = decodeURI(dataURI.split(',')[1])
}
mimestring = dataURI.split(',')[0].split(':')[1].split(';')[0]
var content = new Array();
for (var i = 0; i < byteString.length; i++) {
content[i] = byteString.charCodeAt(i)
}
return new Blob([new Uint8Array(content)], {type: mimestring});
}
add a comment |
Thanks to @Stoive and @vava720 I combined the two in this way, avoiding to use the deprecated BlobBuilder and ArrayBuffer
function dataURItoBlob(dataURI) {
'use strict'
var byteString,
mimestring
if(dataURI.split(',')[0].indexOf('base64') !== -1 ) {
byteString = atob(dataURI.split(',')[1])
} else {
byteString = decodeURI(dataURI.split(',')[1])
}
mimestring = dataURI.split(',')[0].split(':')[1].split(';')[0]
var content = new Array();
for (var i = 0; i < byteString.length; i++) {
content[i] = byteString.charCodeAt(i)
}
return new Blob([new Uint8Array(content)], {type: mimestring});
}
add a comment |
Thanks to @Stoive and @vava720 I combined the two in this way, avoiding to use the deprecated BlobBuilder and ArrayBuffer
function dataURItoBlob(dataURI) {
'use strict'
var byteString,
mimestring
if(dataURI.split(',')[0].indexOf('base64') !== -1 ) {
byteString = atob(dataURI.split(',')[1])
} else {
byteString = decodeURI(dataURI.split(',')[1])
}
mimestring = dataURI.split(',')[0].split(':')[1].split(';')[0]
var content = new Array();
for (var i = 0; i < byteString.length; i++) {
content[i] = byteString.charCodeAt(i)
}
return new Blob([new Uint8Array(content)], {type: mimestring});
}
Thanks to @Stoive and @vava720 I combined the two in this way, avoiding to use the deprecated BlobBuilder and ArrayBuffer
function dataURItoBlob(dataURI) {
'use strict'
var byteString,
mimestring
if(dataURI.split(',')[0].indexOf('base64') !== -1 ) {
byteString = atob(dataURI.split(',')[1])
} else {
byteString = decodeURI(dataURI.split(',')[1])
}
mimestring = dataURI.split(',')[0].split(':')[1].split(';')[0]
var content = new Array();
for (var i = 0; i < byteString.length; i++) {
content[i] = byteString.charCodeAt(i)
}
return new Blob([new Uint8Array(content)], {type: mimestring});
}
answered Feb 18 '13 at 6:33


MimoMimo
3,81042642
3,81042642
add a comment |
add a comment |
My preferred way is canvas.toBlob()
But anyhow here is yet another way to convert base64 to a blob using fetch ^^,
var url = "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAUAAAAFCAYAAACNbyblAAAAHElEQVQI12P4//8/w38GIAXDIBKE0DHxgljNBAAO9TXL0Y4OHwAAAABJRU5ErkJggg=="
fetch(url)
.then(res => res.blob())
.then(blob => {
var fd = new FormData()
fd.append('image', blob, 'filename')
console.log(blob)
// Upload
// fetch('upload', {method: 'POST', body: fd})
})
what is fetch and how is it relevant?
– Ricardo Freitas
Nov 28 '16 at 17:33
Fetch is a modern ajax method that you can use instead ofXMLHttpRequest
since data url is just a url, You can use ajax to fetch that resource and you got yourself an option to decide if you want it as blob, arraybuffer or text
– Endless
Nov 28 '16 at 17:49
1
@Endless 'fetch()' a local base64 string... a really clever hack!
– Diego ZoracKy
Mar 6 '17 at 21:18
add a comment |
My preferred way is canvas.toBlob()
But anyhow here is yet another way to convert base64 to a blob using fetch ^^,
var url = "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAUAAAAFCAYAAACNbyblAAAAHElEQVQI12P4//8/w38GIAXDIBKE0DHxgljNBAAO9TXL0Y4OHwAAAABJRU5ErkJggg=="
fetch(url)
.then(res => res.blob())
.then(blob => {
var fd = new FormData()
fd.append('image', blob, 'filename')
console.log(blob)
// Upload
// fetch('upload', {method: 'POST', body: fd})
})
what is fetch and how is it relevant?
– Ricardo Freitas
Nov 28 '16 at 17:33
Fetch is a modern ajax method that you can use instead ofXMLHttpRequest
since data url is just a url, You can use ajax to fetch that resource and you got yourself an option to decide if you want it as blob, arraybuffer or text
– Endless
Nov 28 '16 at 17:49
1
@Endless 'fetch()' a local base64 string... a really clever hack!
– Diego ZoracKy
Mar 6 '17 at 21:18
add a comment |
My preferred way is canvas.toBlob()
But anyhow here is yet another way to convert base64 to a blob using fetch ^^,
var url = "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAUAAAAFCAYAAACNbyblAAAAHElEQVQI12P4//8/w38GIAXDIBKE0DHxgljNBAAO9TXL0Y4OHwAAAABJRU5ErkJggg=="
fetch(url)
.then(res => res.blob())
.then(blob => {
var fd = new FormData()
fd.append('image', blob, 'filename')
console.log(blob)
// Upload
// fetch('upload', {method: 'POST', body: fd})
})
My preferred way is canvas.toBlob()
But anyhow here is yet another way to convert base64 to a blob using fetch ^^,
var url = "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAUAAAAFCAYAAACNbyblAAAAHElEQVQI12P4//8/w38GIAXDIBKE0DHxgljNBAAO9TXL0Y4OHwAAAABJRU5ErkJggg=="
fetch(url)
.then(res => res.blob())
.then(blob => {
var fd = new FormData()
fd.append('image', blob, 'filename')
console.log(blob)
// Upload
// fetch('upload', {method: 'POST', body: fd})
})
var url = "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAUAAAAFCAYAAACNbyblAAAAHElEQVQI12P4//8/w38GIAXDIBKE0DHxgljNBAAO9TXL0Y4OHwAAAABJRU5ErkJggg=="
fetch(url)
.then(res => res.blob())
.then(blob => {
var fd = new FormData()
fd.append('image', blob, 'filename')
console.log(blob)
// Upload
// fetch('upload', {method: 'POST', body: fd})
})
var url = "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAUAAAAFCAYAAACNbyblAAAAHElEQVQI12P4//8/w38GIAXDIBKE0DHxgljNBAAO9TXL0Y4OHwAAAABJRU5ErkJggg=="
fetch(url)
.then(res => res.blob())
.then(blob => {
var fd = new FormData()
fd.append('image', blob, 'filename')
console.log(blob)
// Upload
// fetch('upload', {method: 'POST', body: fd})
})
edited Sep 1 '17 at 18:48
answered Oct 19 '16 at 20:38
EndlessEndless
12.2k65070
12.2k65070
what is fetch and how is it relevant?
– Ricardo Freitas
Nov 28 '16 at 17:33
Fetch is a modern ajax method that you can use instead ofXMLHttpRequest
since data url is just a url, You can use ajax to fetch that resource and you got yourself an option to decide if you want it as blob, arraybuffer or text
– Endless
Nov 28 '16 at 17:49
1
@Endless 'fetch()' a local base64 string... a really clever hack!
– Diego ZoracKy
Mar 6 '17 at 21:18
add a comment |
what is fetch and how is it relevant?
– Ricardo Freitas
Nov 28 '16 at 17:33
Fetch is a modern ajax method that you can use instead ofXMLHttpRequest
since data url is just a url, You can use ajax to fetch that resource and you got yourself an option to decide if you want it as blob, arraybuffer or text
– Endless
Nov 28 '16 at 17:49
1
@Endless 'fetch()' a local base64 string... a really clever hack!
– Diego ZoracKy
Mar 6 '17 at 21:18
what is fetch and how is it relevant?
– Ricardo Freitas
Nov 28 '16 at 17:33
what is fetch and how is it relevant?
– Ricardo Freitas
Nov 28 '16 at 17:33
Fetch is a modern ajax method that you can use instead of
XMLHttpRequest
since data url is just a url, You can use ajax to fetch that resource and you got yourself an option to decide if you want it as blob, arraybuffer or text– Endless
Nov 28 '16 at 17:49
Fetch is a modern ajax method that you can use instead of
XMLHttpRequest
since data url is just a url, You can use ajax to fetch that resource and you got yourself an option to decide if you want it as blob, arraybuffer or text– Endless
Nov 28 '16 at 17:49
1
1
@Endless 'fetch()' a local base64 string... a really clever hack!
– Diego ZoracKy
Mar 6 '17 at 21:18
@Endless 'fetch()' a local base64 string... a really clever hack!
– Diego ZoracKy
Mar 6 '17 at 21:18
add a comment |
The evolving standard looks to be canvas.toBlob() not canvas.getAsFile() as Mozilla hazarded to guess.
I don't see any browser yet supporting it :(
Thanks for this great thread!
Also, anyone trying the accepted answer should be careful with BlobBuilder as I'm finding support to be limited (and namespaced):
var bb;
try {
bb = new BlobBuilder();
} catch(e) {
try {
bb = new WebKitBlobBuilder();
} catch(e) {
bb = new MozBlobBuilder();
}
}
Were you using another library's polyfill for BlobBuilder?
I was using Chrome with no polyfills, and don't recall coming across namespacing. I eagerly anticipatecanvas.toBlob()
- it seems much more appropriate thangetAsFile
.
– Stoive
Jun 28 '11 at 0:45
1
BlobBuilder
seem to be deprecated in favor ofBlob
– sandstrom
Oct 10 '12 at 12:57
BlobBuilder is deprecated and this pattern is awful. Better would be : window.BlobBuilder = (window.BlobBuilder || window.WebKitBlobBuilder || window.MozBlobBuilder || window.MSBlobBuilder); the nested try catches are really ugly and what happens if none of the builders are available?
– Chris Hanson
Sep 12 '13 at 18:05
How is this awful? If an exception is thrown and 1) BlobBuilder doesn't exist then nothing happens and the next block is executed. 2) If it does exist, but an exception is thrown then it is deprecated and shouldn't be used anyway, so it continues into the next try block. Ideally you would check if Blob is supported first, and even before this check for toBlob support
– TaylorMac
Feb 14 '14 at 19:33
add a comment |
The evolving standard looks to be canvas.toBlob() not canvas.getAsFile() as Mozilla hazarded to guess.
I don't see any browser yet supporting it :(
Thanks for this great thread!
Also, anyone trying the accepted answer should be careful with BlobBuilder as I'm finding support to be limited (and namespaced):
var bb;
try {
bb = new BlobBuilder();
} catch(e) {
try {
bb = new WebKitBlobBuilder();
} catch(e) {
bb = new MozBlobBuilder();
}
}
Were you using another library's polyfill for BlobBuilder?
I was using Chrome with no polyfills, and don't recall coming across namespacing. I eagerly anticipatecanvas.toBlob()
- it seems much more appropriate thangetAsFile
.
– Stoive
Jun 28 '11 at 0:45
1
BlobBuilder
seem to be deprecated in favor ofBlob
– sandstrom
Oct 10 '12 at 12:57
BlobBuilder is deprecated and this pattern is awful. Better would be : window.BlobBuilder = (window.BlobBuilder || window.WebKitBlobBuilder || window.MozBlobBuilder || window.MSBlobBuilder); the nested try catches are really ugly and what happens if none of the builders are available?
– Chris Hanson
Sep 12 '13 at 18:05
How is this awful? If an exception is thrown and 1) BlobBuilder doesn't exist then nothing happens and the next block is executed. 2) If it does exist, but an exception is thrown then it is deprecated and shouldn't be used anyway, so it continues into the next try block. Ideally you would check if Blob is supported first, and even before this check for toBlob support
– TaylorMac
Feb 14 '14 at 19:33
add a comment |
The evolving standard looks to be canvas.toBlob() not canvas.getAsFile() as Mozilla hazarded to guess.
I don't see any browser yet supporting it :(
Thanks for this great thread!
Also, anyone trying the accepted answer should be careful with BlobBuilder as I'm finding support to be limited (and namespaced):
var bb;
try {
bb = new BlobBuilder();
} catch(e) {
try {
bb = new WebKitBlobBuilder();
} catch(e) {
bb = new MozBlobBuilder();
}
}
Were you using another library's polyfill for BlobBuilder?
The evolving standard looks to be canvas.toBlob() not canvas.getAsFile() as Mozilla hazarded to guess.
I don't see any browser yet supporting it :(
Thanks for this great thread!
Also, anyone trying the accepted answer should be careful with BlobBuilder as I'm finding support to be limited (and namespaced):
var bb;
try {
bb = new BlobBuilder();
} catch(e) {
try {
bb = new WebKitBlobBuilder();
} catch(e) {
bb = new MozBlobBuilder();
}
}
Were you using another library's polyfill for BlobBuilder?
edited Jun 27 '11 at 21:36
answered Jun 27 '11 at 20:39
Chris BoscoChris Bosco
990916
990916
I was using Chrome with no polyfills, and don't recall coming across namespacing. I eagerly anticipatecanvas.toBlob()
- it seems much more appropriate thangetAsFile
.
– Stoive
Jun 28 '11 at 0:45
1
BlobBuilder
seem to be deprecated in favor ofBlob
– sandstrom
Oct 10 '12 at 12:57
BlobBuilder is deprecated and this pattern is awful. Better would be : window.BlobBuilder = (window.BlobBuilder || window.WebKitBlobBuilder || window.MozBlobBuilder || window.MSBlobBuilder); the nested try catches are really ugly and what happens if none of the builders are available?
– Chris Hanson
Sep 12 '13 at 18:05
How is this awful? If an exception is thrown and 1) BlobBuilder doesn't exist then nothing happens and the next block is executed. 2) If it does exist, but an exception is thrown then it is deprecated and shouldn't be used anyway, so it continues into the next try block. Ideally you would check if Blob is supported first, and even before this check for toBlob support
– TaylorMac
Feb 14 '14 at 19:33
add a comment |
I was using Chrome with no polyfills, and don't recall coming across namespacing. I eagerly anticipatecanvas.toBlob()
- it seems much more appropriate thangetAsFile
.
– Stoive
Jun 28 '11 at 0:45
1
BlobBuilder
seem to be deprecated in favor ofBlob
– sandstrom
Oct 10 '12 at 12:57
BlobBuilder is deprecated and this pattern is awful. Better would be : window.BlobBuilder = (window.BlobBuilder || window.WebKitBlobBuilder || window.MozBlobBuilder || window.MSBlobBuilder); the nested try catches are really ugly and what happens if none of the builders are available?
– Chris Hanson
Sep 12 '13 at 18:05
How is this awful? If an exception is thrown and 1) BlobBuilder doesn't exist then nothing happens and the next block is executed. 2) If it does exist, but an exception is thrown then it is deprecated and shouldn't be used anyway, so it continues into the next try block. Ideally you would check if Blob is supported first, and even before this check for toBlob support
– TaylorMac
Feb 14 '14 at 19:33
I was using Chrome with no polyfills, and don't recall coming across namespacing. I eagerly anticipate
canvas.toBlob()
- it seems much more appropriate than getAsFile
.– Stoive
Jun 28 '11 at 0:45
I was using Chrome with no polyfills, and don't recall coming across namespacing. I eagerly anticipate
canvas.toBlob()
- it seems much more appropriate than getAsFile
.– Stoive
Jun 28 '11 at 0:45
1
1
BlobBuilder
seem to be deprecated in favor of Blob
– sandstrom
Oct 10 '12 at 12:57
BlobBuilder
seem to be deprecated in favor of Blob
– sandstrom
Oct 10 '12 at 12:57
BlobBuilder is deprecated and this pattern is awful. Better would be : window.BlobBuilder = (window.BlobBuilder || window.WebKitBlobBuilder || window.MozBlobBuilder || window.MSBlobBuilder); the nested try catches are really ugly and what happens if none of the builders are available?
– Chris Hanson
Sep 12 '13 at 18:05
BlobBuilder is deprecated and this pattern is awful. Better would be : window.BlobBuilder = (window.BlobBuilder || window.WebKitBlobBuilder || window.MozBlobBuilder || window.MSBlobBuilder); the nested try catches are really ugly and what happens if none of the builders are available?
– Chris Hanson
Sep 12 '13 at 18:05
How is this awful? If an exception is thrown and 1) BlobBuilder doesn't exist then nothing happens and the next block is executed. 2) If it does exist, but an exception is thrown then it is deprecated and shouldn't be used anyway, so it continues into the next try block. Ideally you would check if Blob is supported first, and even before this check for toBlob support
– TaylorMac
Feb 14 '14 at 19:33
How is this awful? If an exception is thrown and 1) BlobBuilder doesn't exist then nothing happens and the next block is executed. 2) If it does exist, but an exception is thrown then it is deprecated and shouldn't be used anyway, so it continues into the next try block. Ideally you would check if Blob is supported first, and even before this check for toBlob support
– TaylorMac
Feb 14 '14 at 19:33
add a comment |
var BlobBuilder = (window.MozBlobBuilder || window.WebKitBlobBuilder || window.BlobBuilder);
can be used without the try catch.
Thankx to check_ca. Great work.
1
This will still throw an error if the browser supports the deprecated BlobBuilder. The browser will use the old method if it supports it, even if it supports the new method. This is not desired, see Chris Bosco's approach below
– TaylorMac
Feb 14 '14 at 19:30
add a comment |
var BlobBuilder = (window.MozBlobBuilder || window.WebKitBlobBuilder || window.BlobBuilder);
can be used without the try catch.
Thankx to check_ca. Great work.
1
This will still throw an error if the browser supports the deprecated BlobBuilder. The browser will use the old method if it supports it, even if it supports the new method. This is not desired, see Chris Bosco's approach below
– TaylorMac
Feb 14 '14 at 19:30
add a comment |
var BlobBuilder = (window.MozBlobBuilder || window.WebKitBlobBuilder || window.BlobBuilder);
can be used without the try catch.
Thankx to check_ca. Great work.
var BlobBuilder = (window.MozBlobBuilder || window.WebKitBlobBuilder || window.BlobBuilder);
can be used without the try catch.
Thankx to check_ca. Great work.
edited Jan 29 '12 at 16:32
answered Jan 25 '12 at 20:57
Nafis AhmadNafis Ahmad
1,7051912
1,7051912
1
This will still throw an error if the browser supports the deprecated BlobBuilder. The browser will use the old method if it supports it, even if it supports the new method. This is not desired, see Chris Bosco's approach below
– TaylorMac
Feb 14 '14 at 19:30
add a comment |
1
This will still throw an error if the browser supports the deprecated BlobBuilder. The browser will use the old method if it supports it, even if it supports the new method. This is not desired, see Chris Bosco's approach below
– TaylorMac
Feb 14 '14 at 19:30
1
1
This will still throw an error if the browser supports the deprecated BlobBuilder. The browser will use the old method if it supports it, even if it supports the new method. This is not desired, see Chris Bosco's approach below
– TaylorMac
Feb 14 '14 at 19:30
This will still throw an error if the browser supports the deprecated BlobBuilder. The browser will use the old method if it supports it, even if it supports the new method. This is not desired, see Chris Bosco's approach below
– TaylorMac
Feb 14 '14 at 19:30
add a comment |
The original answer by Stoive is easily fixable by changing the last line to accommodate Blob:
function dataURItoBlob (dataURI) {
// convert base64 to raw binary data held in a string
// doesn't handle URLEncoded DataURIs
var byteString;
if (dataURI.split(',')[0].indexOf('base64') >= 0)
byteString = atob(dataURI.split(',')[1]);
else
byteString = unescape(dataURI.split(',')[1]);
// separate out the mime component
var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0];
// write the bytes of the string to an ArrayBuffer
var ab = new ArrayBuffer(byteString.length);
var ia = new Uint8Array(ab);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
// write the ArrayBuffer to a blob, and you're done
return new Blob([ab],{type: mimeString});
}
add a comment |
The original answer by Stoive is easily fixable by changing the last line to accommodate Blob:
function dataURItoBlob (dataURI) {
// convert base64 to raw binary data held in a string
// doesn't handle URLEncoded DataURIs
var byteString;
if (dataURI.split(',')[0].indexOf('base64') >= 0)
byteString = atob(dataURI.split(',')[1]);
else
byteString = unescape(dataURI.split(',')[1]);
// separate out the mime component
var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0];
// write the bytes of the string to an ArrayBuffer
var ab = new ArrayBuffer(byteString.length);
var ia = new Uint8Array(ab);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
// write the ArrayBuffer to a blob, and you're done
return new Blob([ab],{type: mimeString});
}
add a comment |
The original answer by Stoive is easily fixable by changing the last line to accommodate Blob:
function dataURItoBlob (dataURI) {
// convert base64 to raw binary data held in a string
// doesn't handle URLEncoded DataURIs
var byteString;
if (dataURI.split(',')[0].indexOf('base64') >= 0)
byteString = atob(dataURI.split(',')[1]);
else
byteString = unescape(dataURI.split(',')[1]);
// separate out the mime component
var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0];
// write the bytes of the string to an ArrayBuffer
var ab = new ArrayBuffer(byteString.length);
var ia = new Uint8Array(ab);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
// write the ArrayBuffer to a blob, and you're done
return new Blob([ab],{type: mimeString});
}
The original answer by Stoive is easily fixable by changing the last line to accommodate Blob:
function dataURItoBlob (dataURI) {
// convert base64 to raw binary data held in a string
// doesn't handle URLEncoded DataURIs
var byteString;
if (dataURI.split(',')[0].indexOf('base64') >= 0)
byteString = atob(dataURI.split(',')[1]);
else
byteString = unescape(dataURI.split(',')[1]);
// separate out the mime component
var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0];
// write the bytes of the string to an ArrayBuffer
var ab = new ArrayBuffer(byteString.length);
var ia = new Uint8Array(ab);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
// write the ArrayBuffer to a blob, and you're done
return new Blob([ab],{type: mimeString});
}
answered Jul 16 '13 at 17:14


topkaratopkara
678615
678615
add a comment |
add a comment |
Here is an ES6 version of Stoive's answer:
export class ImageDataConverter {
constructor(dataURI) {
this.dataURI = dataURI;
}
getByteString() {
let byteString;
if (this.dataURI.split(',')[0].indexOf('base64') >= 0) {
byteString = atob(this.dataURI.split(',')[1]);
} else {
byteString = decodeURI(this.dataURI.split(',')[1]);
}
return byteString;
}
getMimeString() {
return this.dataURI.split(',')[0].split(':')[1].split(';')[0];
}
convertToTypedArray() {
let byteString = this.getByteString();
let ia = new Uint8Array(byteString.length);
for (let i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return ia;
}
dataURItoBlob() {
let mimeString = this.getMimeString();
let intArray = this.convertToTypedArray();
return new Blob([intArray], {type: mimeString});
}
}
Usage:
const dataURL = canvas.toDataURL('image/jpeg', 0.5);
const blob = new ImageDataConverter(dataURL).dataURItoBlob();
let fd = new FormData(document.forms[0]);
fd.append("canvasImage", blob);
add a comment |
Here is an ES6 version of Stoive's answer:
export class ImageDataConverter {
constructor(dataURI) {
this.dataURI = dataURI;
}
getByteString() {
let byteString;
if (this.dataURI.split(',')[0].indexOf('base64') >= 0) {
byteString = atob(this.dataURI.split(',')[1]);
} else {
byteString = decodeURI(this.dataURI.split(',')[1]);
}
return byteString;
}
getMimeString() {
return this.dataURI.split(',')[0].split(':')[1].split(';')[0];
}
convertToTypedArray() {
let byteString = this.getByteString();
let ia = new Uint8Array(byteString.length);
for (let i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return ia;
}
dataURItoBlob() {
let mimeString = this.getMimeString();
let intArray = this.convertToTypedArray();
return new Blob([intArray], {type: mimeString});
}
}
Usage:
const dataURL = canvas.toDataURL('image/jpeg', 0.5);
const blob = new ImageDataConverter(dataURL).dataURItoBlob();
let fd = new FormData(document.forms[0]);
fd.append("canvasImage", blob);
add a comment |
Here is an ES6 version of Stoive's answer:
export class ImageDataConverter {
constructor(dataURI) {
this.dataURI = dataURI;
}
getByteString() {
let byteString;
if (this.dataURI.split(',')[0].indexOf('base64') >= 0) {
byteString = atob(this.dataURI.split(',')[1]);
} else {
byteString = decodeURI(this.dataURI.split(',')[1]);
}
return byteString;
}
getMimeString() {
return this.dataURI.split(',')[0].split(':')[1].split(';')[0];
}
convertToTypedArray() {
let byteString = this.getByteString();
let ia = new Uint8Array(byteString.length);
for (let i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return ia;
}
dataURItoBlob() {
let mimeString = this.getMimeString();
let intArray = this.convertToTypedArray();
return new Blob([intArray], {type: mimeString});
}
}
Usage:
const dataURL = canvas.toDataURL('image/jpeg', 0.5);
const blob = new ImageDataConverter(dataURL).dataURItoBlob();
let fd = new FormData(document.forms[0]);
fd.append("canvasImage", blob);
Here is an ES6 version of Stoive's answer:
export class ImageDataConverter {
constructor(dataURI) {
this.dataURI = dataURI;
}
getByteString() {
let byteString;
if (this.dataURI.split(',')[0].indexOf('base64') >= 0) {
byteString = atob(this.dataURI.split(',')[1]);
} else {
byteString = decodeURI(this.dataURI.split(',')[1]);
}
return byteString;
}
getMimeString() {
return this.dataURI.split(',')[0].split(':')[1].split(';')[0];
}
convertToTypedArray() {
let byteString = this.getByteString();
let ia = new Uint8Array(byteString.length);
for (let i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return ia;
}
dataURItoBlob() {
let mimeString = this.getMimeString();
let intArray = this.convertToTypedArray();
return new Blob([intArray], {type: mimeString});
}
}
Usage:
const dataURL = canvas.toDataURL('image/jpeg', 0.5);
const blob = new ImageDataConverter(dataURL).dataURItoBlob();
let fd = new FormData(document.forms[0]);
fd.append("canvasImage", blob);
edited May 23 '17 at 12:26
Community♦
11
11
answered Oct 18 '16 at 17:18
vekerdybvekerdyb
402412
402412
add a comment |
add a comment |
make it simple :D
function dataURItoBlob(dataURI,mime) {
// convert base64 to raw binary data held in a string
// doesn't handle URLEncoded DataURIs
var byteString = window.atob(dataURI);
// separate out the mime component
// write the bytes of the string to an ArrayBuffer
//var ab = new ArrayBuffer(byteString.length);
var ia = new Uint8Array(byteString.length);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
// write the ArrayBuffer to a blob, and you're done
var blob = new Blob([ia], { type: mime });
return blob;
}
add a comment |
make it simple :D
function dataURItoBlob(dataURI,mime) {
// convert base64 to raw binary data held in a string
// doesn't handle URLEncoded DataURIs
var byteString = window.atob(dataURI);
// separate out the mime component
// write the bytes of the string to an ArrayBuffer
//var ab = new ArrayBuffer(byteString.length);
var ia = new Uint8Array(byteString.length);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
// write the ArrayBuffer to a blob, and you're done
var blob = new Blob([ia], { type: mime });
return blob;
}
add a comment |
make it simple :D
function dataURItoBlob(dataURI,mime) {
// convert base64 to raw binary data held in a string
// doesn't handle URLEncoded DataURIs
var byteString = window.atob(dataURI);
// separate out the mime component
// write the bytes of the string to an ArrayBuffer
//var ab = new ArrayBuffer(byteString.length);
var ia = new Uint8Array(byteString.length);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
// write the ArrayBuffer to a blob, and you're done
var blob = new Blob([ia], { type: mime });
return blob;
}
make it simple :D
function dataURItoBlob(dataURI,mime) {
// convert base64 to raw binary data held in a string
// doesn't handle URLEncoded DataURIs
var byteString = window.atob(dataURI);
// separate out the mime component
// write the bytes of the string to an ArrayBuffer
//var ab = new ArrayBuffer(byteString.length);
var ia = new Uint8Array(byteString.length);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
// write the ArrayBuffer to a blob, and you're done
var blob = new Blob([ia], { type: mime });
return blob;
}
answered Sep 7 '14 at 19:16
SendySendy
1614
1614
add a comment |
add a comment |
Thanks! @steovi for this solution.
I have added support to ES6 version and changed from unescape to dataURI(unescape is deprecated).
converterDataURItoBlob(dataURI) {
let byteString;
let mimeString;
let ia;
if (dataURI.split(',')[0].indexOf('base64') >= 0) {
byteString = atob(dataURI.split(',')[1]);
} else {
byteString = encodeURI(dataURI.split(',')[1]);
}
// separate out the mime component
mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0];
// write the bytes of the string to a typed array
ia = new Uint8Array(byteString.length);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return new Blob([ia], {type:mimeString});
}
add a comment |
Thanks! @steovi for this solution.
I have added support to ES6 version and changed from unescape to dataURI(unescape is deprecated).
converterDataURItoBlob(dataURI) {
let byteString;
let mimeString;
let ia;
if (dataURI.split(',')[0].indexOf('base64') >= 0) {
byteString = atob(dataURI.split(',')[1]);
} else {
byteString = encodeURI(dataURI.split(',')[1]);
}
// separate out the mime component
mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0];
// write the bytes of the string to a typed array
ia = new Uint8Array(byteString.length);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return new Blob([ia], {type:mimeString});
}
add a comment |
Thanks! @steovi for this solution.
I have added support to ES6 version and changed from unescape to dataURI(unescape is deprecated).
converterDataURItoBlob(dataURI) {
let byteString;
let mimeString;
let ia;
if (dataURI.split(',')[0].indexOf('base64') >= 0) {
byteString = atob(dataURI.split(',')[1]);
} else {
byteString = encodeURI(dataURI.split(',')[1]);
}
// separate out the mime component
mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0];
// write the bytes of the string to a typed array
ia = new Uint8Array(byteString.length);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return new Blob([ia], {type:mimeString});
}
Thanks! @steovi for this solution.
I have added support to ES6 version and changed from unescape to dataURI(unescape is deprecated).
converterDataURItoBlob(dataURI) {
let byteString;
let mimeString;
let ia;
if (dataURI.split(',')[0].indexOf('base64') >= 0) {
byteString = atob(dataURI.split(',')[1]);
} else {
byteString = encodeURI(dataURI.split(',')[1]);
}
// separate out the mime component
mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0];
// write the bytes of the string to a typed array
ia = new Uint8Array(byteString.length);
for (var i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i);
}
return new Blob([ia], {type:mimeString});
}
edited Apr 9 '18 at 16:12
crabbly
3,3221925
3,3221925
answered Apr 2 '18 at 18:07


wilfredonoyolawilfredonoyola
767
767
add a comment |
add a comment |
toDataURL gives you a string and you can put that string to a hidden input.
Could you please give an example? I don't wan't to upload a base64 string (which is what doing<input type=hidden value="data:..." />
would do), I want to upload the file data (like what<input type="file" />
does, except you're not allowed to set thevalue
property on these).
– Stoive
Feb 24 '11 at 3:11
This should be a comment rather than an answer. Please elaborate the answer with proper explanation. @Cat Chen
– Lucky
Mar 30 '15 at 8:31
add a comment |
toDataURL gives you a string and you can put that string to a hidden input.
Could you please give an example? I don't wan't to upload a base64 string (which is what doing<input type=hidden value="data:..." />
would do), I want to upload the file data (like what<input type="file" />
does, except you're not allowed to set thevalue
property on these).
– Stoive
Feb 24 '11 at 3:11
This should be a comment rather than an answer. Please elaborate the answer with proper explanation. @Cat Chen
– Lucky
Mar 30 '15 at 8:31
add a comment |
toDataURL gives you a string and you can put that string to a hidden input.
toDataURL gives you a string and you can put that string to a hidden input.
answered Feb 23 '11 at 14:19
Cat ChenCat Chen
2,1431210
2,1431210
Could you please give an example? I don't wan't to upload a base64 string (which is what doing<input type=hidden value="data:..." />
would do), I want to upload the file data (like what<input type="file" />
does, except you're not allowed to set thevalue
property on these).
– Stoive
Feb 24 '11 at 3:11
This should be a comment rather than an answer. Please elaborate the answer with proper explanation. @Cat Chen
– Lucky
Mar 30 '15 at 8:31
add a comment |
Could you please give an example? I don't wan't to upload a base64 string (which is what doing<input type=hidden value="data:..." />
would do), I want to upload the file data (like what<input type="file" />
does, except you're not allowed to set thevalue
property on these).
– Stoive
Feb 24 '11 at 3:11
This should be a comment rather than an answer. Please elaborate the answer with proper explanation. @Cat Chen
– Lucky
Mar 30 '15 at 8:31
Could you please give an example? I don't wan't to upload a base64 string (which is what doing
<input type=hidden value="data:..." />
would do), I want to upload the file data (like what <input type="file" />
does, except you're not allowed to set the value
property on these).– Stoive
Feb 24 '11 at 3:11
Could you please give an example? I don't wan't to upload a base64 string (which is what doing
<input type=hidden value="data:..." />
would do), I want to upload the file data (like what <input type="file" />
does, except you're not allowed to set the value
property on these).– Stoive
Feb 24 '11 at 3:11
This should be a comment rather than an answer. Please elaborate the answer with proper explanation. @Cat Chen
– Lucky
Mar 30 '15 at 8:31
This should be a comment rather than an answer. Please elaborate the answer with proper explanation. @Cat Chen
– Lucky
Mar 30 '15 at 8:31
add a comment |
I had exactly the same problem as Ravinder Payal, and I've found the answer. Try this:
var dataURL = canvas.toDataURL("image/jpeg");
var name = "image.jpg";
var parseFile = new Parse.File(name, {base64: dataURL.substring(23)});
2
Are you really suggesting to use Parse.com ? You should mention that your answer require dependencies !
– Pierre Maoui
Jul 7 '16 at 14:44
2
WTF ? Why any one will upload base64 code of image to PARSE server and then download?When we can directly upload base64 on our servers and main thing is that it takes same data to upload base64 string or image file. And if you just want to alow user to download the image , you can use thiswindow.open(canvas.toDataURL("image/jpeg"))
– Ravinder Payal
Jul 19 '16 at 18:52
That is coolest answer I have ever seen, LOL
– Humoyun
Oct 4 '18 at 5:09
add a comment |
I had exactly the same problem as Ravinder Payal, and I've found the answer. Try this:
var dataURL = canvas.toDataURL("image/jpeg");
var name = "image.jpg";
var parseFile = new Parse.File(name, {base64: dataURL.substring(23)});
2
Are you really suggesting to use Parse.com ? You should mention that your answer require dependencies !
– Pierre Maoui
Jul 7 '16 at 14:44
2
WTF ? Why any one will upload base64 code of image to PARSE server and then download?When we can directly upload base64 on our servers and main thing is that it takes same data to upload base64 string or image file. And if you just want to alow user to download the image , you can use thiswindow.open(canvas.toDataURL("image/jpeg"))
– Ravinder Payal
Jul 19 '16 at 18:52
That is coolest answer I have ever seen, LOL
– Humoyun
Oct 4 '18 at 5:09
add a comment |
I had exactly the same problem as Ravinder Payal, and I've found the answer. Try this:
var dataURL = canvas.toDataURL("image/jpeg");
var name = "image.jpg";
var parseFile = new Parse.File(name, {base64: dataURL.substring(23)});
I had exactly the same problem as Ravinder Payal, and I've found the answer. Try this:
var dataURL = canvas.toDataURL("image/jpeg");
var name = "image.jpg";
var parseFile = new Parse.File(name, {base64: dataURL.substring(23)});
edited Aug 30 '14 at 17:28
Spooky
2,53472136
2,53472136
answered Aug 30 '14 at 16:31
fengfeng
332
332
2
Are you really suggesting to use Parse.com ? You should mention that your answer require dependencies !
– Pierre Maoui
Jul 7 '16 at 14:44
2
WTF ? Why any one will upload base64 code of image to PARSE server and then download?When we can directly upload base64 on our servers and main thing is that it takes same data to upload base64 string or image file. And if you just want to alow user to download the image , you can use thiswindow.open(canvas.toDataURL("image/jpeg"))
– Ravinder Payal
Jul 19 '16 at 18:52
That is coolest answer I have ever seen, LOL
– Humoyun
Oct 4 '18 at 5:09
add a comment |
2
Are you really suggesting to use Parse.com ? You should mention that your answer require dependencies !
– Pierre Maoui
Jul 7 '16 at 14:44
2
WTF ? Why any one will upload base64 code of image to PARSE server and then download?When we can directly upload base64 on our servers and main thing is that it takes same data to upload base64 string or image file. And if you just want to alow user to download the image , you can use thiswindow.open(canvas.toDataURL("image/jpeg"))
– Ravinder Payal
Jul 19 '16 at 18:52
That is coolest answer I have ever seen, LOL
– Humoyun
Oct 4 '18 at 5:09
2
2
Are you really suggesting to use Parse.com ? You should mention that your answer require dependencies !
– Pierre Maoui
Jul 7 '16 at 14:44
Are you really suggesting to use Parse.com ? You should mention that your answer require dependencies !
– Pierre Maoui
Jul 7 '16 at 14:44
2
2
WTF ? Why any one will upload base64 code of image to PARSE server and then download?When we can directly upload base64 on our servers and main thing is that it takes same data to upload base64 string or image file. And if you just want to alow user to download the image , you can use this
window.open(canvas.toDataURL("image/jpeg"))
– Ravinder Payal
Jul 19 '16 at 18:52
WTF ? Why any one will upload base64 code of image to PARSE server and then download?When we can directly upload base64 on our servers and main thing is that it takes same data to upload base64 string or image file. And if you just want to alow user to download the image , you can use this
window.open(canvas.toDataURL("image/jpeg"))
– Ravinder Payal
Jul 19 '16 at 18:52
That is coolest answer I have ever seen, LOL
– Humoyun
Oct 4 '18 at 5:09
That is coolest answer I have ever seen, LOL
– Humoyun
Oct 4 '18 at 5:09
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f4998908%2fconvert-data-uri-to-file-then-append-to-formdata%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
aqEZC,tp xC,ur9k,rn jgKGGC0IPd,ZZDr5yZXwS dZ
If you want to save the DataURI of an image in server: stackoverflow.com/a/50131281/5466401
– Sibin John Mattappallil
May 2 '18 at 9:49