PyOpenGl Validation failure
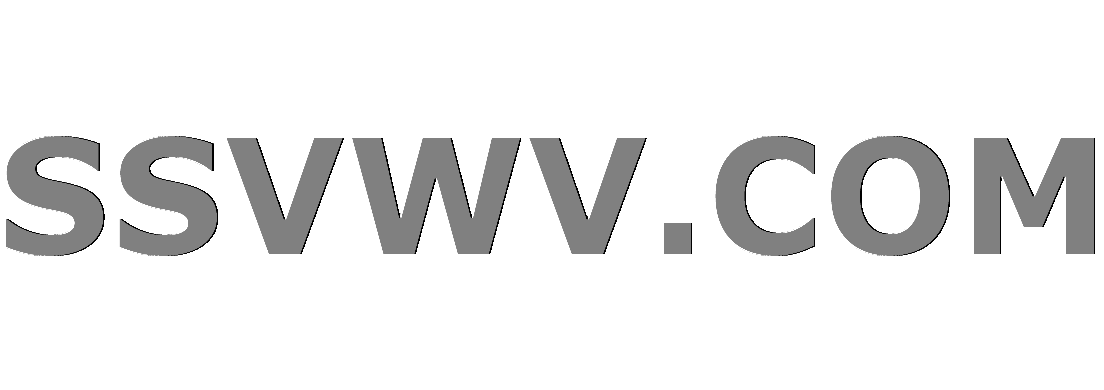
Multi tool use
I am trying to create a simple scene with pyopengl, but I keep getting runtime error. I am using glfw for displaying opengl scene.
I am using python because I would like to include opengl in some other python projects.
I am on a macOS Mojave (10.14), Python 3.7
Error:
Traceback (most recent call last):
File "main.py", line 109, in <module>
main()
File "main.py", line 64, in main
shader = OpenGL.GL.shaders.compileProgram(s.vertex(), s.fragment())
File "slib/python3.7/site-packages/OpenGL/GL/shaders.py", line 196, in
compileProgram
program.check_validate()
File "lib/python3.7/site-packages/OpenGL/GL/shaders.py", line 108, in
check_validate
glGetProgramInfoLog( self ),
RuntimeError: Validation failure (0): b'Validation Failed: No vertex
array object bound.n'
Code:
import glfw
from OpenGL.GL import *
import OpenGL.GL.shaders
import numpy as np
def trikotnik():
trikotnik = np.array([
-0.5, -0.5, 0.0,
0.5, -0.5, 0.0,
0.0, 0.5, 0.0
], dtype=np.float32)
return trikotnik
class Shaders:
def __init__(self):
pass
def vertex(self):
v = """
#version 330
in vec4 position;
void main() {
gl_Position = position;
}
"""
return OpenGL.GL.shaders.compileShader(v, GL_VERTEX_SHADER)
def fragment(self):
f = """
#version 330
out vec4 fragColor;
void main() {
fragColor = vec4( 0, 1, 0, 1 );
}
"""
return OpenGL.GL.shaders.compileShader(f, GL_FRAGMENT_SHADER)
def main():
# Initialize the library
if not glfw.init():
return
glfw.window_hint(glfw.CONTEXT_VERSION_MAJOR, 3)
glfw.window_hint(glfw.CONTEXT_VERSION_MINOR, 2)
glfw.window_hint(glfw.OPENGL_PROFILE, glfw.OPENGL_CORE_PROFILE)
glfw.window_hint(glfw.OPENGL_FORWARD_COMPAT, GL_TRUE)
# Create a windowed mode window and its OpenGL context
window = glfw.create_window(640, 480, "Hello World", None, None)
if not window:
glfw.terminate()
return
# Make the window's context current
glfw.make_context_current(window)
# Creating shaders
s = Shaders()
shader = OpenGL.GL.shaders.compileProgram(s.vertex(), s.fragment())
# Creating vertex buffer object on gpu
VBO = glGenBuffers(1)
glBindBuffer(GL_ARRAY_BUFFER, VBO)
# Load data on array buffer...32 = size in bytes 9x4
# GL_STATIC_DRAW: The vertex data will be uploaded once and drawn many times.
glBufferData(GL_ARRAY_BUFFER, 32, trikotnik(), GL_STATIC_DRAW)
glBindBuffer(GL_ARRAY_BUFFER, VBO)
position = glGetAttribLocation(shader, "position")
glVertexAttribPointer(
position, # attribute 0. No particular reason for 0, but must match the layout in the shader.
3, # size
GL_FLOAT, # type
GL_FALSE, # normalized
0, # stride
None # array buffer offset
)
glEnableVertexAttribArray(position)
glUseProgram(shader)
glClearColor(0, 107, 179, 1.0)
# Loop until the user closes the window
while not glfw.window_should_close(window):
# Render here, e.g. using pyOpenGL
# Clear color buffer
glClear(GL_COLOR_BUFFER_BIT)
# Draw
glDrawArrays(GL_TRIANGLES, 0, 3)
# Swap front and back buffers
glfw.swap_buffers(window)
# Poll for and process events
glfw.poll_events()
glfw.terminate()
if __name__ == "__main__":
main()
I found this in source code:
validate (keyword only) -- if False, suppress automatic
validation against current GL state. In advanced usage
the validation can produce spurious errors. Note: this
function is not really intended for advanced usage,
if you're finding yourself specifying this flag you
likely should be using your own shader management code.
I try to set it to False but I am still getting an error.
python opengl glfw pyopengl
add a comment |
I am trying to create a simple scene with pyopengl, but I keep getting runtime error. I am using glfw for displaying opengl scene.
I am using python because I would like to include opengl in some other python projects.
I am on a macOS Mojave (10.14), Python 3.7
Error:
Traceback (most recent call last):
File "main.py", line 109, in <module>
main()
File "main.py", line 64, in main
shader = OpenGL.GL.shaders.compileProgram(s.vertex(), s.fragment())
File "slib/python3.7/site-packages/OpenGL/GL/shaders.py", line 196, in
compileProgram
program.check_validate()
File "lib/python3.7/site-packages/OpenGL/GL/shaders.py", line 108, in
check_validate
glGetProgramInfoLog( self ),
RuntimeError: Validation failure (0): b'Validation Failed: No vertex
array object bound.n'
Code:
import glfw
from OpenGL.GL import *
import OpenGL.GL.shaders
import numpy as np
def trikotnik():
trikotnik = np.array([
-0.5, -0.5, 0.0,
0.5, -0.5, 0.0,
0.0, 0.5, 0.0
], dtype=np.float32)
return trikotnik
class Shaders:
def __init__(self):
pass
def vertex(self):
v = """
#version 330
in vec4 position;
void main() {
gl_Position = position;
}
"""
return OpenGL.GL.shaders.compileShader(v, GL_VERTEX_SHADER)
def fragment(self):
f = """
#version 330
out vec4 fragColor;
void main() {
fragColor = vec4( 0, 1, 0, 1 );
}
"""
return OpenGL.GL.shaders.compileShader(f, GL_FRAGMENT_SHADER)
def main():
# Initialize the library
if not glfw.init():
return
glfw.window_hint(glfw.CONTEXT_VERSION_MAJOR, 3)
glfw.window_hint(glfw.CONTEXT_VERSION_MINOR, 2)
glfw.window_hint(glfw.OPENGL_PROFILE, glfw.OPENGL_CORE_PROFILE)
glfw.window_hint(glfw.OPENGL_FORWARD_COMPAT, GL_TRUE)
# Create a windowed mode window and its OpenGL context
window = glfw.create_window(640, 480, "Hello World", None, None)
if not window:
glfw.terminate()
return
# Make the window's context current
glfw.make_context_current(window)
# Creating shaders
s = Shaders()
shader = OpenGL.GL.shaders.compileProgram(s.vertex(), s.fragment())
# Creating vertex buffer object on gpu
VBO = glGenBuffers(1)
glBindBuffer(GL_ARRAY_BUFFER, VBO)
# Load data on array buffer...32 = size in bytes 9x4
# GL_STATIC_DRAW: The vertex data will be uploaded once and drawn many times.
glBufferData(GL_ARRAY_BUFFER, 32, trikotnik(), GL_STATIC_DRAW)
glBindBuffer(GL_ARRAY_BUFFER, VBO)
position = glGetAttribLocation(shader, "position")
glVertexAttribPointer(
position, # attribute 0. No particular reason for 0, but must match the layout in the shader.
3, # size
GL_FLOAT, # type
GL_FALSE, # normalized
0, # stride
None # array buffer offset
)
glEnableVertexAttribArray(position)
glUseProgram(shader)
glClearColor(0, 107, 179, 1.0)
# Loop until the user closes the window
while not glfw.window_should_close(window):
# Render here, e.g. using pyOpenGL
# Clear color buffer
glClear(GL_COLOR_BUFFER_BIT)
# Draw
glDrawArrays(GL_TRIANGLES, 0, 3)
# Swap front and back buffers
glfw.swap_buffers(window)
# Poll for and process events
glfw.poll_events()
glfw.terminate()
if __name__ == "__main__":
main()
I found this in source code:
validate (keyword only) -- if False, suppress automatic
validation against current GL state. In advanced usage
the validation can produce spurious errors. Note: this
function is not really intended for advanced usage,
if you're finding yourself specifying this flag you
likely should be using your own shader management code.
I try to set it to False but I am still getting an error.
python opengl glfw pyopengl
1
Either you use a Vertex Array Object or you switch to a compatibility profile contextglfw.window_hint(glfw.OPENGL_PROFILE, glfw.OPENGL_COMPAT_PROFILE)
. In core profile you must have a named VAO. it is not optional.
– Rabbid76
Nov 13 '18 at 10:36
add a comment |
I am trying to create a simple scene with pyopengl, but I keep getting runtime error. I am using glfw for displaying opengl scene.
I am using python because I would like to include opengl in some other python projects.
I am on a macOS Mojave (10.14), Python 3.7
Error:
Traceback (most recent call last):
File "main.py", line 109, in <module>
main()
File "main.py", line 64, in main
shader = OpenGL.GL.shaders.compileProgram(s.vertex(), s.fragment())
File "slib/python3.7/site-packages/OpenGL/GL/shaders.py", line 196, in
compileProgram
program.check_validate()
File "lib/python3.7/site-packages/OpenGL/GL/shaders.py", line 108, in
check_validate
glGetProgramInfoLog( self ),
RuntimeError: Validation failure (0): b'Validation Failed: No vertex
array object bound.n'
Code:
import glfw
from OpenGL.GL import *
import OpenGL.GL.shaders
import numpy as np
def trikotnik():
trikotnik = np.array([
-0.5, -0.5, 0.0,
0.5, -0.5, 0.0,
0.0, 0.5, 0.0
], dtype=np.float32)
return trikotnik
class Shaders:
def __init__(self):
pass
def vertex(self):
v = """
#version 330
in vec4 position;
void main() {
gl_Position = position;
}
"""
return OpenGL.GL.shaders.compileShader(v, GL_VERTEX_SHADER)
def fragment(self):
f = """
#version 330
out vec4 fragColor;
void main() {
fragColor = vec4( 0, 1, 0, 1 );
}
"""
return OpenGL.GL.shaders.compileShader(f, GL_FRAGMENT_SHADER)
def main():
# Initialize the library
if not glfw.init():
return
glfw.window_hint(glfw.CONTEXT_VERSION_MAJOR, 3)
glfw.window_hint(glfw.CONTEXT_VERSION_MINOR, 2)
glfw.window_hint(glfw.OPENGL_PROFILE, glfw.OPENGL_CORE_PROFILE)
glfw.window_hint(glfw.OPENGL_FORWARD_COMPAT, GL_TRUE)
# Create a windowed mode window and its OpenGL context
window = glfw.create_window(640, 480, "Hello World", None, None)
if not window:
glfw.terminate()
return
# Make the window's context current
glfw.make_context_current(window)
# Creating shaders
s = Shaders()
shader = OpenGL.GL.shaders.compileProgram(s.vertex(), s.fragment())
# Creating vertex buffer object on gpu
VBO = glGenBuffers(1)
glBindBuffer(GL_ARRAY_BUFFER, VBO)
# Load data on array buffer...32 = size in bytes 9x4
# GL_STATIC_DRAW: The vertex data will be uploaded once and drawn many times.
glBufferData(GL_ARRAY_BUFFER, 32, trikotnik(), GL_STATIC_DRAW)
glBindBuffer(GL_ARRAY_BUFFER, VBO)
position = glGetAttribLocation(shader, "position")
glVertexAttribPointer(
position, # attribute 0. No particular reason for 0, but must match the layout in the shader.
3, # size
GL_FLOAT, # type
GL_FALSE, # normalized
0, # stride
None # array buffer offset
)
glEnableVertexAttribArray(position)
glUseProgram(shader)
glClearColor(0, 107, 179, 1.0)
# Loop until the user closes the window
while not glfw.window_should_close(window):
# Render here, e.g. using pyOpenGL
# Clear color buffer
glClear(GL_COLOR_BUFFER_BIT)
# Draw
glDrawArrays(GL_TRIANGLES, 0, 3)
# Swap front and back buffers
glfw.swap_buffers(window)
# Poll for and process events
glfw.poll_events()
glfw.terminate()
if __name__ == "__main__":
main()
I found this in source code:
validate (keyword only) -- if False, suppress automatic
validation against current GL state. In advanced usage
the validation can produce spurious errors. Note: this
function is not really intended for advanced usage,
if you're finding yourself specifying this flag you
likely should be using your own shader management code.
I try to set it to False but I am still getting an error.
python opengl glfw pyopengl
I am trying to create a simple scene with pyopengl, but I keep getting runtime error. I am using glfw for displaying opengl scene.
I am using python because I would like to include opengl in some other python projects.
I am on a macOS Mojave (10.14), Python 3.7
Error:
Traceback (most recent call last):
File "main.py", line 109, in <module>
main()
File "main.py", line 64, in main
shader = OpenGL.GL.shaders.compileProgram(s.vertex(), s.fragment())
File "slib/python3.7/site-packages/OpenGL/GL/shaders.py", line 196, in
compileProgram
program.check_validate()
File "lib/python3.7/site-packages/OpenGL/GL/shaders.py", line 108, in
check_validate
glGetProgramInfoLog( self ),
RuntimeError: Validation failure (0): b'Validation Failed: No vertex
array object bound.n'
Code:
import glfw
from OpenGL.GL import *
import OpenGL.GL.shaders
import numpy as np
def trikotnik():
trikotnik = np.array([
-0.5, -0.5, 0.0,
0.5, -0.5, 0.0,
0.0, 0.5, 0.0
], dtype=np.float32)
return trikotnik
class Shaders:
def __init__(self):
pass
def vertex(self):
v = """
#version 330
in vec4 position;
void main() {
gl_Position = position;
}
"""
return OpenGL.GL.shaders.compileShader(v, GL_VERTEX_SHADER)
def fragment(self):
f = """
#version 330
out vec4 fragColor;
void main() {
fragColor = vec4( 0, 1, 0, 1 );
}
"""
return OpenGL.GL.shaders.compileShader(f, GL_FRAGMENT_SHADER)
def main():
# Initialize the library
if not glfw.init():
return
glfw.window_hint(glfw.CONTEXT_VERSION_MAJOR, 3)
glfw.window_hint(glfw.CONTEXT_VERSION_MINOR, 2)
glfw.window_hint(glfw.OPENGL_PROFILE, glfw.OPENGL_CORE_PROFILE)
glfw.window_hint(glfw.OPENGL_FORWARD_COMPAT, GL_TRUE)
# Create a windowed mode window and its OpenGL context
window = glfw.create_window(640, 480, "Hello World", None, None)
if not window:
glfw.terminate()
return
# Make the window's context current
glfw.make_context_current(window)
# Creating shaders
s = Shaders()
shader = OpenGL.GL.shaders.compileProgram(s.vertex(), s.fragment())
# Creating vertex buffer object on gpu
VBO = glGenBuffers(1)
glBindBuffer(GL_ARRAY_BUFFER, VBO)
# Load data on array buffer...32 = size in bytes 9x4
# GL_STATIC_DRAW: The vertex data will be uploaded once and drawn many times.
glBufferData(GL_ARRAY_BUFFER, 32, trikotnik(), GL_STATIC_DRAW)
glBindBuffer(GL_ARRAY_BUFFER, VBO)
position = glGetAttribLocation(shader, "position")
glVertexAttribPointer(
position, # attribute 0. No particular reason for 0, but must match the layout in the shader.
3, # size
GL_FLOAT, # type
GL_FALSE, # normalized
0, # stride
None # array buffer offset
)
glEnableVertexAttribArray(position)
glUseProgram(shader)
glClearColor(0, 107, 179, 1.0)
# Loop until the user closes the window
while not glfw.window_should_close(window):
# Render here, e.g. using pyOpenGL
# Clear color buffer
glClear(GL_COLOR_BUFFER_BIT)
# Draw
glDrawArrays(GL_TRIANGLES, 0, 3)
# Swap front and back buffers
glfw.swap_buffers(window)
# Poll for and process events
glfw.poll_events()
glfw.terminate()
if __name__ == "__main__":
main()
I found this in source code:
validate (keyword only) -- if False, suppress automatic
validation against current GL state. In advanced usage
the validation can produce spurious errors. Note: this
function is not really intended for advanced usage,
if you're finding yourself specifying this flag you
likely should be using your own shader management code.
I try to set it to False but I am still getting an error.
python opengl glfw pyopengl
python opengl glfw pyopengl
asked Nov 13 '18 at 10:32
MihaMiha
132
132
1
Either you use a Vertex Array Object or you switch to a compatibility profile contextglfw.window_hint(glfw.OPENGL_PROFILE, glfw.OPENGL_COMPAT_PROFILE)
. In core profile you must have a named VAO. it is not optional.
– Rabbid76
Nov 13 '18 at 10:36
add a comment |
1
Either you use a Vertex Array Object or you switch to a compatibility profile contextglfw.window_hint(glfw.OPENGL_PROFILE, glfw.OPENGL_COMPAT_PROFILE)
. In core profile you must have a named VAO. it is not optional.
– Rabbid76
Nov 13 '18 at 10:36
1
1
Either you use a Vertex Array Object or you switch to a compatibility profile context
glfw.window_hint(glfw.OPENGL_PROFILE, glfw.OPENGL_COMPAT_PROFILE)
. In core profile you must have a named VAO. it is not optional.– Rabbid76
Nov 13 '18 at 10:36
Either you use a Vertex Array Object or you switch to a compatibility profile context
glfw.window_hint(glfw.OPENGL_PROFILE, glfw.OPENGL_COMPAT_PROFILE)
. In core profile you must have a named VAO. it is not optional.– Rabbid76
Nov 13 '18 at 10:36
add a comment |
1 Answer
1
active
oldest
votes
Either you use a Vertex Array Object or you switch to a compatibility profile context glfw.window_hint(glfw.OPENGL_PROFILE, glfw.OPENGL_COMPAT_PROFILE)
. In core profile you must have a named VAO, it is not optional.
Create and bind a VAO before specifying the array of vertex array data:
VAO = glGenVertexArrays(1)
glBindVertexArray(VAO)
glVertexAttribPointer(
position, # attribute 0. No particular reason for 0, but must match the layout in the shader.
3, # size
GL_FLOAT, # type
GL_FALSE, # normalized
0, # stride
None # array buffer offset
)
glEnableVertexAttribArray(position)
Thank you. Using VAO worked. I believe that I need to use core profile on mac.
– Miha
Nov 14 '18 at 9:34
@Miha You're welcome. It is state of the art to use core profile and vertex array objects.
– Rabbid76
Nov 14 '18 at 9:44
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53279015%2fpyopengl-validation-failure%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Either you use a Vertex Array Object or you switch to a compatibility profile context glfw.window_hint(glfw.OPENGL_PROFILE, glfw.OPENGL_COMPAT_PROFILE)
. In core profile you must have a named VAO, it is not optional.
Create and bind a VAO before specifying the array of vertex array data:
VAO = glGenVertexArrays(1)
glBindVertexArray(VAO)
glVertexAttribPointer(
position, # attribute 0. No particular reason for 0, but must match the layout in the shader.
3, # size
GL_FLOAT, # type
GL_FALSE, # normalized
0, # stride
None # array buffer offset
)
glEnableVertexAttribArray(position)
Thank you. Using VAO worked. I believe that I need to use core profile on mac.
– Miha
Nov 14 '18 at 9:34
@Miha You're welcome. It is state of the art to use core profile and vertex array objects.
– Rabbid76
Nov 14 '18 at 9:44
add a comment |
Either you use a Vertex Array Object or you switch to a compatibility profile context glfw.window_hint(glfw.OPENGL_PROFILE, glfw.OPENGL_COMPAT_PROFILE)
. In core profile you must have a named VAO, it is not optional.
Create and bind a VAO before specifying the array of vertex array data:
VAO = glGenVertexArrays(1)
glBindVertexArray(VAO)
glVertexAttribPointer(
position, # attribute 0. No particular reason for 0, but must match the layout in the shader.
3, # size
GL_FLOAT, # type
GL_FALSE, # normalized
0, # stride
None # array buffer offset
)
glEnableVertexAttribArray(position)
Thank you. Using VAO worked. I believe that I need to use core profile on mac.
– Miha
Nov 14 '18 at 9:34
@Miha You're welcome. It is state of the art to use core profile and vertex array objects.
– Rabbid76
Nov 14 '18 at 9:44
add a comment |
Either you use a Vertex Array Object or you switch to a compatibility profile context glfw.window_hint(glfw.OPENGL_PROFILE, glfw.OPENGL_COMPAT_PROFILE)
. In core profile you must have a named VAO, it is not optional.
Create and bind a VAO before specifying the array of vertex array data:
VAO = glGenVertexArrays(1)
glBindVertexArray(VAO)
glVertexAttribPointer(
position, # attribute 0. No particular reason for 0, but must match the layout in the shader.
3, # size
GL_FLOAT, # type
GL_FALSE, # normalized
0, # stride
None # array buffer offset
)
glEnableVertexAttribArray(position)
Either you use a Vertex Array Object or you switch to a compatibility profile context glfw.window_hint(glfw.OPENGL_PROFILE, glfw.OPENGL_COMPAT_PROFILE)
. In core profile you must have a named VAO, it is not optional.
Create and bind a VAO before specifying the array of vertex array data:
VAO = glGenVertexArrays(1)
glBindVertexArray(VAO)
glVertexAttribPointer(
position, # attribute 0. No particular reason for 0, but must match the layout in the shader.
3, # size
GL_FLOAT, # type
GL_FALSE, # normalized
0, # stride
None # array buffer offset
)
glEnableVertexAttribArray(position)
answered Nov 13 '18 at 10:40


Rabbid76Rabbid76
34.2k113145
34.2k113145
Thank you. Using VAO worked. I believe that I need to use core profile on mac.
– Miha
Nov 14 '18 at 9:34
@Miha You're welcome. It is state of the art to use core profile and vertex array objects.
– Rabbid76
Nov 14 '18 at 9:44
add a comment |
Thank you. Using VAO worked. I believe that I need to use core profile on mac.
– Miha
Nov 14 '18 at 9:34
@Miha You're welcome. It is state of the art to use core profile and vertex array objects.
– Rabbid76
Nov 14 '18 at 9:44
Thank you. Using VAO worked. I believe that I need to use core profile on mac.
– Miha
Nov 14 '18 at 9:34
Thank you. Using VAO worked. I believe that I need to use core profile on mac.
– Miha
Nov 14 '18 at 9:34
@Miha You're welcome. It is state of the art to use core profile and vertex array objects.
– Rabbid76
Nov 14 '18 at 9:44
@Miha You're welcome. It is state of the art to use core profile and vertex array objects.
– Rabbid76
Nov 14 '18 at 9:44
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53279015%2fpyopengl-validation-failure%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
X,VJNO9vLY,5XBra,QYn6K0 D,Ldgl,JA5sMHpEtKghZNC,zzN21IDWDUzWkFWlP6d4KKjK
1
Either you use a Vertex Array Object or you switch to a compatibility profile context
glfw.window_hint(glfw.OPENGL_PROFILE, glfw.OPENGL_COMPAT_PROFILE)
. In core profile you must have a named VAO. it is not optional.– Rabbid76
Nov 13 '18 at 10:36