Is there a way to combine these two if statements?
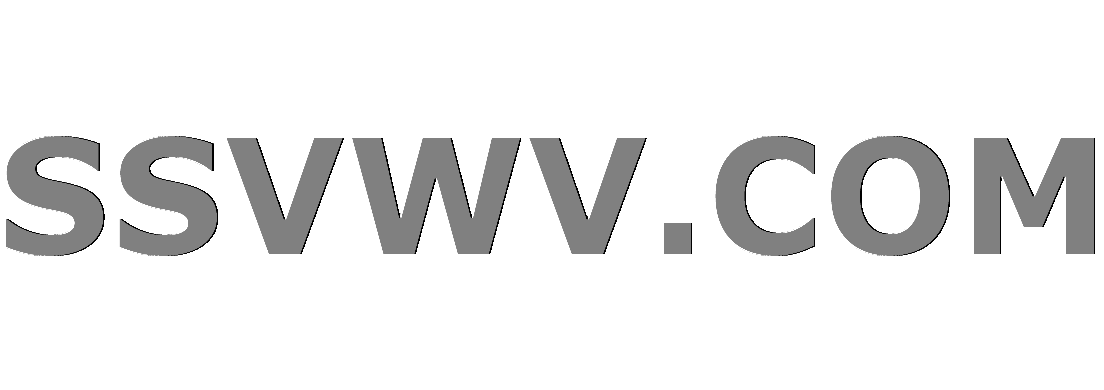
Multi tool use
I was working on a personal assignment where the user types their birthday, and then it displays the user's astrological sign and a description of the sign. But since all astrological signs are multiple months, I have split the if statements into multiple if statements, one for each month, for example
if (birthMonth == 5 && (21<=birthDay && birthDay <=31)) {
JFrame frame = new JFrame();
String symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
symbol += bday;
JLabel label = new JLabel(symbol, new ImageIcon("gemini.jpg"), JLabel.CENTER);
label.setVerticalTextPosition(SwingConstants.TOP);
frame.add(label);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
else if (birthMonth == 6 && (1<=birthDay && birthDay <=21)) {
JFrame frame = new JFrame();
String symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
symbol += bday;
JLabel label = new JLabel(symbol, new ImageIcon("gemini.jpg"), JLabel.CENTER);
label.setVerticalTextPosition(SwingConstants.TOP);
frame.add(label);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
I was wondering if there is a way to combine the if statements, while keeping the code working the way it currently is. Many thanks in advance!
java if-statement jframe
add a comment |
I was working on a personal assignment where the user types their birthday, and then it displays the user's astrological sign and a description of the sign. But since all astrological signs are multiple months, I have split the if statements into multiple if statements, one for each month, for example
if (birthMonth == 5 && (21<=birthDay && birthDay <=31)) {
JFrame frame = new JFrame();
String symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
symbol += bday;
JLabel label = new JLabel(symbol, new ImageIcon("gemini.jpg"), JLabel.CENTER);
label.setVerticalTextPosition(SwingConstants.TOP);
frame.add(label);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
else if (birthMonth == 6 && (1<=birthDay && birthDay <=21)) {
JFrame frame = new JFrame();
String symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
symbol += bday;
JLabel label = new JLabel(symbol, new ImageIcon("gemini.jpg"), JLabel.CENTER);
label.setVerticalTextPosition(SwingConstants.TOP);
frame.add(label);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
I was wondering if there is a way to combine the if statements, while keeping the code working the way it currently is. Many thanks in advance!
java if-statement jframe
1
WHy dont you use || ? like this: (birthMonth == 5 && (21<=birthDay && birthDay <=31) || (birthMonth == 6 && (1<=birthDay && birthDay <=21)))
– Saulo Aires
Nov 9 '18 at 15:08
2
Just combine them with an or. Seems simple enough.
– OH GOD SPIDERS
Nov 9 '18 at 15:09
add a comment |
I was working on a personal assignment where the user types their birthday, and then it displays the user's astrological sign and a description of the sign. But since all astrological signs are multiple months, I have split the if statements into multiple if statements, one for each month, for example
if (birthMonth == 5 && (21<=birthDay && birthDay <=31)) {
JFrame frame = new JFrame();
String symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
symbol += bday;
JLabel label = new JLabel(symbol, new ImageIcon("gemini.jpg"), JLabel.CENTER);
label.setVerticalTextPosition(SwingConstants.TOP);
frame.add(label);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
else if (birthMonth == 6 && (1<=birthDay && birthDay <=21)) {
JFrame frame = new JFrame();
String symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
symbol += bday;
JLabel label = new JLabel(symbol, new ImageIcon("gemini.jpg"), JLabel.CENTER);
label.setVerticalTextPosition(SwingConstants.TOP);
frame.add(label);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
I was wondering if there is a way to combine the if statements, while keeping the code working the way it currently is. Many thanks in advance!
java if-statement jframe
I was working on a personal assignment where the user types their birthday, and then it displays the user's astrological sign and a description of the sign. But since all astrological signs are multiple months, I have split the if statements into multiple if statements, one for each month, for example
if (birthMonth == 5 && (21<=birthDay && birthDay <=31)) {
JFrame frame = new JFrame();
String symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
symbol += bday;
JLabel label = new JLabel(symbol, new ImageIcon("gemini.jpg"), JLabel.CENTER);
label.setVerticalTextPosition(SwingConstants.TOP);
frame.add(label);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
else if (birthMonth == 6 && (1<=birthDay && birthDay <=21)) {
JFrame frame = new JFrame();
String symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
symbol += bday;
JLabel label = new JLabel(symbol, new ImageIcon("gemini.jpg"), JLabel.CENTER);
label.setVerticalTextPosition(SwingConstants.TOP);
frame.add(label);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
I was wondering if there is a way to combine the if statements, while keeping the code working the way it currently is. Many thanks in advance!
java if-statement jframe
java if-statement jframe
edited Nov 13 '18 at 11:23


E_net4 wishes happy holidays
11.9k63468
11.9k63468
asked Nov 9 '18 at 15:06


Alex PetersAlex Peters
14
14
1
WHy dont you use || ? like this: (birthMonth == 5 && (21<=birthDay && birthDay <=31) || (birthMonth == 6 && (1<=birthDay && birthDay <=21)))
– Saulo Aires
Nov 9 '18 at 15:08
2
Just combine them with an or. Seems simple enough.
– OH GOD SPIDERS
Nov 9 '18 at 15:09
add a comment |
1
WHy dont you use || ? like this: (birthMonth == 5 && (21<=birthDay && birthDay <=31) || (birthMonth == 6 && (1<=birthDay && birthDay <=21)))
– Saulo Aires
Nov 9 '18 at 15:08
2
Just combine them with an or. Seems simple enough.
– OH GOD SPIDERS
Nov 9 '18 at 15:09
1
1
WHy dont you use || ? like this: (birthMonth == 5 && (21<=birthDay && birthDay <=31) || (birthMonth == 6 && (1<=birthDay && birthDay <=21)))
– Saulo Aires
Nov 9 '18 at 15:08
WHy dont you use || ? like this: (birthMonth == 5 && (21<=birthDay && birthDay <=31) || (birthMonth == 6 && (1<=birthDay && birthDay <=21)))
– Saulo Aires
Nov 9 '18 at 15:08
2
2
Just combine them with an or. Seems simple enough.
– OH GOD SPIDERS
Nov 9 '18 at 15:09
Just combine them with an or. Seems simple enough.
– OH GOD SPIDERS
Nov 9 '18 at 15:09
add a comment |
2 Answers
2
active
oldest
votes
Both logic and behavior are same so you don't have to use two condition to write your logic you can use below code as a single way.
if (birthMonth == 5 && (21<=birthDay && birthDay <=31) || birthMonth == 6 && (1<=birthDay && birthDay <=21)) {
JFrame frame = new JFrame();
String symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
symbol += bday;
JLabel label = new JLabel(symbol, new ImageIcon("gemini.jpg"), JLabel.CENTER);
label.setVerticalTextPosition(SwingConstants.TOP);
frame.add(label);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
Most of those parentheses are redundant.(birthMonth == 5 && 21<=birthDay && birthDay <=31 || birthMonth == 6 && 1<=birthDay && birthDay <=21)
would work identically.
– khelwood
Nov 9 '18 at 15:16
Yes, but i have included bracket to better understand the code implementation.
– GauravRai1512
Nov 9 '18 at 15:17
@GauravRai1512 Do you know how to add a new line to the string symbol? n doesn't appear to work in JFrame.
– Alex Peters
Nov 9 '18 at 15:21
let me know where you want to use new line do you have any code for reference?
– GauravRai1512
Nov 9 '18 at 15:25
@GauravRai1512 In this lineString symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
– Alex Peters
Nov 9 '18 at 15:27
|
show 4 more comments
Why don't you use the or operator || ? like this:
(birthMonth == 5 && (21<=birthDay && birthDay <=31) || (birthMonth == 6 & (1<=birthDay && birthDay <=21)))
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53228283%2fis-there-a-way-to-combine-these-two-if-statements%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Both logic and behavior are same so you don't have to use two condition to write your logic you can use below code as a single way.
if (birthMonth == 5 && (21<=birthDay && birthDay <=31) || birthMonth == 6 && (1<=birthDay && birthDay <=21)) {
JFrame frame = new JFrame();
String symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
symbol += bday;
JLabel label = new JLabel(symbol, new ImageIcon("gemini.jpg"), JLabel.CENTER);
label.setVerticalTextPosition(SwingConstants.TOP);
frame.add(label);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
Most of those parentheses are redundant.(birthMonth == 5 && 21<=birthDay && birthDay <=31 || birthMonth == 6 && 1<=birthDay && birthDay <=21)
would work identically.
– khelwood
Nov 9 '18 at 15:16
Yes, but i have included bracket to better understand the code implementation.
– GauravRai1512
Nov 9 '18 at 15:17
@GauravRai1512 Do you know how to add a new line to the string symbol? n doesn't appear to work in JFrame.
– Alex Peters
Nov 9 '18 at 15:21
let me know where you want to use new line do you have any code for reference?
– GauravRai1512
Nov 9 '18 at 15:25
@GauravRai1512 In this lineString symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
– Alex Peters
Nov 9 '18 at 15:27
|
show 4 more comments
Both logic and behavior are same so you don't have to use two condition to write your logic you can use below code as a single way.
if (birthMonth == 5 && (21<=birthDay && birthDay <=31) || birthMonth == 6 && (1<=birthDay && birthDay <=21)) {
JFrame frame = new JFrame();
String symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
symbol += bday;
JLabel label = new JLabel(symbol, new ImageIcon("gemini.jpg"), JLabel.CENTER);
label.setVerticalTextPosition(SwingConstants.TOP);
frame.add(label);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
Most of those parentheses are redundant.(birthMonth == 5 && 21<=birthDay && birthDay <=31 || birthMonth == 6 && 1<=birthDay && birthDay <=21)
would work identically.
– khelwood
Nov 9 '18 at 15:16
Yes, but i have included bracket to better understand the code implementation.
– GauravRai1512
Nov 9 '18 at 15:17
@GauravRai1512 Do you know how to add a new line to the string symbol? n doesn't appear to work in JFrame.
– Alex Peters
Nov 9 '18 at 15:21
let me know where you want to use new line do you have any code for reference?
– GauravRai1512
Nov 9 '18 at 15:25
@GauravRai1512 In this lineString symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
– Alex Peters
Nov 9 '18 at 15:27
|
show 4 more comments
Both logic and behavior are same so you don't have to use two condition to write your logic you can use below code as a single way.
if (birthMonth == 5 && (21<=birthDay && birthDay <=31) || birthMonth == 6 && (1<=birthDay && birthDay <=21)) {
JFrame frame = new JFrame();
String symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
symbol += bday;
JLabel label = new JLabel(symbol, new ImageIcon("gemini.jpg"), JLabel.CENTER);
label.setVerticalTextPosition(SwingConstants.TOP);
frame.add(label);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
Both logic and behavior are same so you don't have to use two condition to write your logic you can use below code as a single way.
if (birthMonth == 5 && (21<=birthDay && birthDay <=31) || birthMonth == 6 && (1<=birthDay && birthDay <=21)) {
JFrame frame = new JFrame();
String symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
symbol += bday;
JLabel label = new JLabel(symbol, new ImageIcon("gemini.jpg"), JLabel.CENTER);
label.setVerticalTextPosition(SwingConstants.TOP);
frame.add(label);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
edited Nov 9 '18 at 15:16
answered Nov 9 '18 at 15:14
GauravRai1512GauravRai1512
58811
58811
Most of those parentheses are redundant.(birthMonth == 5 && 21<=birthDay && birthDay <=31 || birthMonth == 6 && 1<=birthDay && birthDay <=21)
would work identically.
– khelwood
Nov 9 '18 at 15:16
Yes, but i have included bracket to better understand the code implementation.
– GauravRai1512
Nov 9 '18 at 15:17
@GauravRai1512 Do you know how to add a new line to the string symbol? n doesn't appear to work in JFrame.
– Alex Peters
Nov 9 '18 at 15:21
let me know where you want to use new line do you have any code for reference?
– GauravRai1512
Nov 9 '18 at 15:25
@GauravRai1512 In this lineString symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
– Alex Peters
Nov 9 '18 at 15:27
|
show 4 more comments
Most of those parentheses are redundant.(birthMonth == 5 && 21<=birthDay && birthDay <=31 || birthMonth == 6 && 1<=birthDay && birthDay <=21)
would work identically.
– khelwood
Nov 9 '18 at 15:16
Yes, but i have included bracket to better understand the code implementation.
– GauravRai1512
Nov 9 '18 at 15:17
@GauravRai1512 Do you know how to add a new line to the string symbol? n doesn't appear to work in JFrame.
– Alex Peters
Nov 9 '18 at 15:21
let me know where you want to use new line do you have any code for reference?
– GauravRai1512
Nov 9 '18 at 15:25
@GauravRai1512 In this lineString symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
– Alex Peters
Nov 9 '18 at 15:27
Most of those parentheses are redundant.
(birthMonth == 5 && 21<=birthDay && birthDay <=31 || birthMonth == 6 && 1<=birthDay && birthDay <=21)
would work identically.– khelwood
Nov 9 '18 at 15:16
Most of those parentheses are redundant.
(birthMonth == 5 && 21<=birthDay && birthDay <=31 || birthMonth == 6 && 1<=birthDay && birthDay <=21)
would work identically.– khelwood
Nov 9 '18 at 15:16
Yes, but i have included bracket to better understand the code implementation.
– GauravRai1512
Nov 9 '18 at 15:17
Yes, but i have included bracket to better understand the code implementation.
– GauravRai1512
Nov 9 '18 at 15:17
@GauravRai1512 Do you know how to add a new line to the string symbol? n doesn't appear to work in JFrame.
– Alex Peters
Nov 9 '18 at 15:21
@GauravRai1512 Do you know how to add a new line to the string symbol? n doesn't appear to work in JFrame.
– Alex Peters
Nov 9 '18 at 15:21
let me know where you want to use new line do you have any code for reference?
– GauravRai1512
Nov 9 '18 at 15:25
let me know where you want to use new line do you have any code for reference?
– GauravRai1512
Nov 9 '18 at 15:25
@GauravRai1512 In this line
String symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
– Alex Peters
Nov 9 '18 at 15:27
@GauravRai1512 In this line
String symbol = "Your sign is Gemini. You are cerebral, chatty, love learning and education, charming, and adventurous. ";
– Alex Peters
Nov 9 '18 at 15:27
|
show 4 more comments
Why don't you use the or operator || ? like this:
(birthMonth == 5 && (21<=birthDay && birthDay <=31) || (birthMonth == 6 & (1<=birthDay && birthDay <=21)))
add a comment |
Why don't you use the or operator || ? like this:
(birthMonth == 5 && (21<=birthDay && birthDay <=31) || (birthMonth == 6 & (1<=birthDay && birthDay <=21)))
add a comment |
Why don't you use the or operator || ? like this:
(birthMonth == 5 && (21<=birthDay && birthDay <=31) || (birthMonth == 6 & (1<=birthDay && birthDay <=21)))
Why don't you use the or operator || ? like this:
(birthMonth == 5 && (21<=birthDay && birthDay <=31) || (birthMonth == 6 & (1<=birthDay && birthDay <=21)))
edited Nov 9 '18 at 17:09
rileyjsumner
1851115
1851115
answered Nov 9 '18 at 15:15


Saulo AiresSaulo Aires
805
805
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53228283%2fis-there-a-way-to-combine-these-two-if-statements%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
I1jUpkeBTfjvGo2Yezen2vnJsxY92,f1,H,Q,v,l,J48vQAOkb n6IZV9ksCWWGTucZDBL3Kd 1
1
WHy dont you use || ? like this: (birthMonth == 5 && (21<=birthDay && birthDay <=31) || (birthMonth == 6 && (1<=birthDay && birthDay <=21)))
– Saulo Aires
Nov 9 '18 at 15:08
2
Just combine them with an or. Seems simple enough.
– OH GOD SPIDERS
Nov 9 '18 at 15:09