Angular2-jwt: intercept requested api route
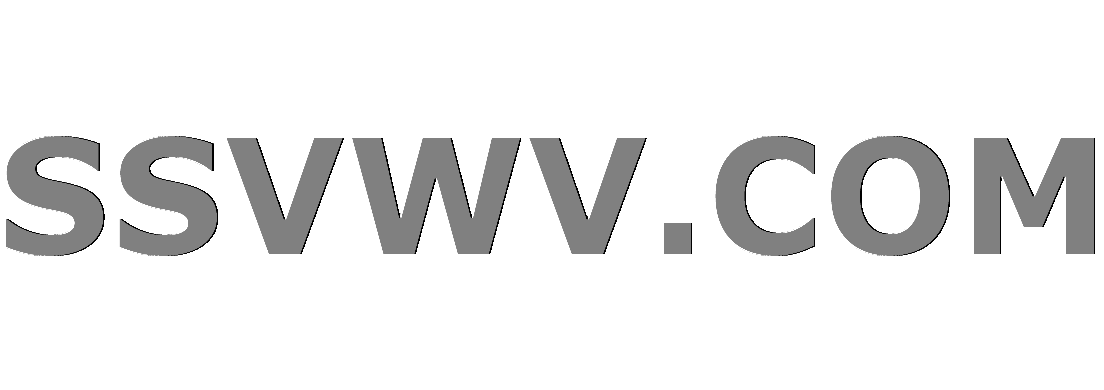
Multi tool use
I'm implementing a jwt refresh mechanism. I'm using auth0/angular2-jwt with Angular 7. When I'm accessing a protected route, I'm sending a request to /api/v1/protected with my access_token
in the Authorization header. When I need to refresh the jwt token, I've got to send a request to /api/v1/auth/refresh with my refresh_token
in the Authorization header.
What I can't figure out is how to select which token I'll send based on the api route.
app.module.ts
import { JwtModule, JWT_OPTIONS } from '@auth0/angular-jwt';
import { HttpClientModule } from '@angular/common/http';
import { TokenService } from './services/token.service';
export function jwtOptionsFactory(tokenService) {
return {
tokenGetter: () => {
return tokenService.getAsyncToken();
},
whitelistedDomains: [
'localhost',
'localhost:80',
'localhost:443',
'localhost:4200',
],
blacklistedRoutes: ,
throwNoTokenError: false,
}
}
...
@NgModule({
...
imports: [
HttpClientModule,
JwtModule.forRoot({
jwtOptionsProvider: {
provide: JWT_OPTIONS,
useFactory: jwtOptionsFactory,
deps: [TokenService]
}
}),
],
...
})
token.service.ts
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class TokenService {
constructor() { }
getAsyncToken(){
// This is the part in question
if (route=='/api/v1/auth/refresh') {
return localStorage.getItem('refresh_token');
}
return localStorage.getItem('access_token');
}
}
My plan B is to blacklist the refresh route in angulat-jwt and write a custom interceptor.
But I'd like to know if there's something I messed. Is this possible to intercept the api call (check the api route) in my token service?
angular angular2-jwt
add a comment |
I'm implementing a jwt refresh mechanism. I'm using auth0/angular2-jwt with Angular 7. When I'm accessing a protected route, I'm sending a request to /api/v1/protected with my access_token
in the Authorization header. When I need to refresh the jwt token, I've got to send a request to /api/v1/auth/refresh with my refresh_token
in the Authorization header.
What I can't figure out is how to select which token I'll send based on the api route.
app.module.ts
import { JwtModule, JWT_OPTIONS } from '@auth0/angular-jwt';
import { HttpClientModule } from '@angular/common/http';
import { TokenService } from './services/token.service';
export function jwtOptionsFactory(tokenService) {
return {
tokenGetter: () => {
return tokenService.getAsyncToken();
},
whitelistedDomains: [
'localhost',
'localhost:80',
'localhost:443',
'localhost:4200',
],
blacklistedRoutes: ,
throwNoTokenError: false,
}
}
...
@NgModule({
...
imports: [
HttpClientModule,
JwtModule.forRoot({
jwtOptionsProvider: {
provide: JWT_OPTIONS,
useFactory: jwtOptionsFactory,
deps: [TokenService]
}
}),
],
...
})
token.service.ts
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class TokenService {
constructor() { }
getAsyncToken(){
// This is the part in question
if (route=='/api/v1/auth/refresh') {
return localStorage.getItem('refresh_token');
}
return localStorage.getItem('access_token');
}
}
My plan B is to blacklist the refresh route in angulat-jwt and write a custom interceptor.
But I'd like to know if there's something I messed. Is this possible to intercept the api call (check the api route) in my token service?
angular angular2-jwt
1
Hope this might give you the right direction itnext.io/…
– Suryan
Nov 13 '18 at 12:24
@Suryan Thanks for the link. Basically an interceptor will do the same thing as JwtModule. I'm thinking, why am I using JwtModule, if I still have to write a custom interceptor? :)
– sr9yar
Nov 13 '18 at 13:23
add a comment |
I'm implementing a jwt refresh mechanism. I'm using auth0/angular2-jwt with Angular 7. When I'm accessing a protected route, I'm sending a request to /api/v1/protected with my access_token
in the Authorization header. When I need to refresh the jwt token, I've got to send a request to /api/v1/auth/refresh with my refresh_token
in the Authorization header.
What I can't figure out is how to select which token I'll send based on the api route.
app.module.ts
import { JwtModule, JWT_OPTIONS } from '@auth0/angular-jwt';
import { HttpClientModule } from '@angular/common/http';
import { TokenService } from './services/token.service';
export function jwtOptionsFactory(tokenService) {
return {
tokenGetter: () => {
return tokenService.getAsyncToken();
},
whitelistedDomains: [
'localhost',
'localhost:80',
'localhost:443',
'localhost:4200',
],
blacklistedRoutes: ,
throwNoTokenError: false,
}
}
...
@NgModule({
...
imports: [
HttpClientModule,
JwtModule.forRoot({
jwtOptionsProvider: {
provide: JWT_OPTIONS,
useFactory: jwtOptionsFactory,
deps: [TokenService]
}
}),
],
...
})
token.service.ts
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class TokenService {
constructor() { }
getAsyncToken(){
// This is the part in question
if (route=='/api/v1/auth/refresh') {
return localStorage.getItem('refresh_token');
}
return localStorage.getItem('access_token');
}
}
My plan B is to blacklist the refresh route in angulat-jwt and write a custom interceptor.
But I'd like to know if there's something I messed. Is this possible to intercept the api call (check the api route) in my token service?
angular angular2-jwt
I'm implementing a jwt refresh mechanism. I'm using auth0/angular2-jwt with Angular 7. When I'm accessing a protected route, I'm sending a request to /api/v1/protected with my access_token
in the Authorization header. When I need to refresh the jwt token, I've got to send a request to /api/v1/auth/refresh with my refresh_token
in the Authorization header.
What I can't figure out is how to select which token I'll send based on the api route.
app.module.ts
import { JwtModule, JWT_OPTIONS } from '@auth0/angular-jwt';
import { HttpClientModule } from '@angular/common/http';
import { TokenService } from './services/token.service';
export function jwtOptionsFactory(tokenService) {
return {
tokenGetter: () => {
return tokenService.getAsyncToken();
},
whitelistedDomains: [
'localhost',
'localhost:80',
'localhost:443',
'localhost:4200',
],
blacklistedRoutes: ,
throwNoTokenError: false,
}
}
...
@NgModule({
...
imports: [
HttpClientModule,
JwtModule.forRoot({
jwtOptionsProvider: {
provide: JWT_OPTIONS,
useFactory: jwtOptionsFactory,
deps: [TokenService]
}
}),
],
...
})
token.service.ts
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class TokenService {
constructor() { }
getAsyncToken(){
// This is the part in question
if (route=='/api/v1/auth/refresh') {
return localStorage.getItem('refresh_token');
}
return localStorage.getItem('access_token');
}
}
My plan B is to blacklist the refresh route in angulat-jwt and write a custom interceptor.
But I'd like to know if there's something I messed. Is this possible to intercept the api call (check the api route) in my token service?
angular angular2-jwt
angular angular2-jwt
asked Nov 13 '18 at 11:28


sr9yarsr9yar
1,4051434
1,4051434
1
Hope this might give you the right direction itnext.io/…
– Suryan
Nov 13 '18 at 12:24
@Suryan Thanks for the link. Basically an interceptor will do the same thing as JwtModule. I'm thinking, why am I using JwtModule, if I still have to write a custom interceptor? :)
– sr9yar
Nov 13 '18 at 13:23
add a comment |
1
Hope this might give you the right direction itnext.io/…
– Suryan
Nov 13 '18 at 12:24
@Suryan Thanks for the link. Basically an interceptor will do the same thing as JwtModule. I'm thinking, why am I using JwtModule, if I still have to write a custom interceptor? :)
– sr9yar
Nov 13 '18 at 13:23
1
1
Hope this might give you the right direction itnext.io/…
– Suryan
Nov 13 '18 at 12:24
Hope this might give you the right direction itnext.io/…
– Suryan
Nov 13 '18 at 12:24
@Suryan Thanks for the link. Basically an interceptor will do the same thing as JwtModule. I'm thinking, why am I using JwtModule, if I still have to write a custom interceptor? :)
– sr9yar
Nov 13 '18 at 13:23
@Suryan Thanks for the link. Basically an interceptor will do the same thing as JwtModule. I'm thinking, why am I using JwtModule, if I still have to write a custom interceptor? :)
– sr9yar
Nov 13 '18 at 13:23
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53280046%2fangular2-jwt-intercept-requested-api-route%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53280046%2fangular2-jwt-intercept-requested-api-route%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
AO FFgmVW,bi,Q01A,UMveWBbNyOcVb2,HleBDQl,7lC,P Fc2phx,rEE7igX,0tKulXH7bGUWigmeQ i7PgPhoKxwD10ys,lzBE12PorCE0,xb
1
Hope this might give you the right direction itnext.io/…
– Suryan
Nov 13 '18 at 12:24
@Suryan Thanks for the link. Basically an interceptor will do the same thing as JwtModule. I'm thinking, why am I using JwtModule, if I still have to write a custom interceptor? :)
– sr9yar
Nov 13 '18 at 13:23