d3 Cannot read property 'classed' of undefined
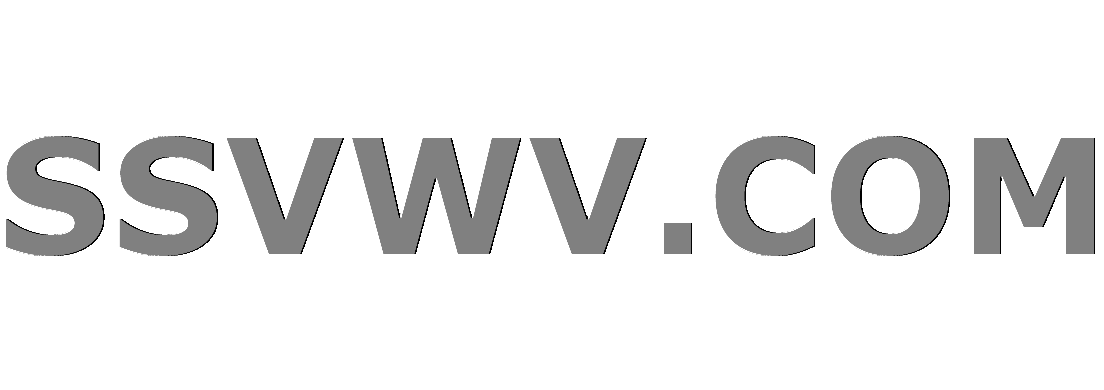
Multi tool use
Trying to highlight current path line by grabbing pathID of a current path and assigning it a class .highlight that makes stroke-width: 2px
.
console.log shows current pathID but it doesn't want to assign a class. Please help. What am I doing wrong here?
Codepen
//Generate group (main)
let groups = svg.selectAll("g")
.data(dataset)
.enter()
.append("g")
.on("mouseover", function(d) {
d3.select(this)
.call(revealCityName)
})
.on("mouseout", hideCityName)
//Path (path #, path line)
groups
.append("path").classed("line", true)
.attr("id", function(d) {
console.log(d.country)
if (d && d.length != 0) {
return d.country.replace(/ |,|./g, '_');
}
})
.attr("d", d => line(d.emissions))
.classed("unfocused", true)
.style("stroke-width", d =>
d.country === "China" ? 1 : 0.2
)
.on("mouseover", highlightPath);
//Text (contries on the left y axis)
groups
.append("text")
.datum(function(d) {
return { country: d.country, value: d.emissions[d.emissions.length - 1]};
})
.attr("transform", function(d) {
if (d.value) {
return "translate(" + xScale(d.value.year) + "," + yScale(d.value.amount) + ")";
} else {
return null;
}
})
.attr("x", 3)
.attr("dy", 4)
.style("font-size", 10)
.style("font-family", "Abril Fatface")
.text(d => d.country)
.classed("hidden", function(d) {
if (d.value && d.value.amount > 700000) {
return false
} else {
return true;
}
})
And a function where I'm trying to assign a class:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
let currentId = d3.select(this).select("path#" + pathID)
console.log("Current ID: ",currentId)
.classed("highlight", true);
}
javascript arrays object d3.js
add a comment |
Trying to highlight current path line by grabbing pathID of a current path and assigning it a class .highlight that makes stroke-width: 2px
.
console.log shows current pathID but it doesn't want to assign a class. Please help. What am I doing wrong here?
Codepen
//Generate group (main)
let groups = svg.selectAll("g")
.data(dataset)
.enter()
.append("g")
.on("mouseover", function(d) {
d3.select(this)
.call(revealCityName)
})
.on("mouseout", hideCityName)
//Path (path #, path line)
groups
.append("path").classed("line", true)
.attr("id", function(d) {
console.log(d.country)
if (d && d.length != 0) {
return d.country.replace(/ |,|./g, '_');
}
})
.attr("d", d => line(d.emissions))
.classed("unfocused", true)
.style("stroke-width", d =>
d.country === "China" ? 1 : 0.2
)
.on("mouseover", highlightPath);
//Text (contries on the left y axis)
groups
.append("text")
.datum(function(d) {
return { country: d.country, value: d.emissions[d.emissions.length - 1]};
})
.attr("transform", function(d) {
if (d.value) {
return "translate(" + xScale(d.value.year) + "," + yScale(d.value.amount) + ")";
} else {
return null;
}
})
.attr("x", 3)
.attr("dy", 4)
.style("font-size", 10)
.style("font-family", "Abril Fatface")
.text(d => d.country)
.classed("hidden", function(d) {
if (d.value && d.value.amount > 700000) {
return false
} else {
return true;
}
})
And a function where I'm trying to assign a class:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
let currentId = d3.select(this).select("path#" + pathID)
console.log("Current ID: ",currentId)
.classed("highlight", true);
}
javascript arrays object d3.js
add a comment |
Trying to highlight current path line by grabbing pathID of a current path and assigning it a class .highlight that makes stroke-width: 2px
.
console.log shows current pathID but it doesn't want to assign a class. Please help. What am I doing wrong here?
Codepen
//Generate group (main)
let groups = svg.selectAll("g")
.data(dataset)
.enter()
.append("g")
.on("mouseover", function(d) {
d3.select(this)
.call(revealCityName)
})
.on("mouseout", hideCityName)
//Path (path #, path line)
groups
.append("path").classed("line", true)
.attr("id", function(d) {
console.log(d.country)
if (d && d.length != 0) {
return d.country.replace(/ |,|./g, '_');
}
})
.attr("d", d => line(d.emissions))
.classed("unfocused", true)
.style("stroke-width", d =>
d.country === "China" ? 1 : 0.2
)
.on("mouseover", highlightPath);
//Text (contries on the left y axis)
groups
.append("text")
.datum(function(d) {
return { country: d.country, value: d.emissions[d.emissions.length - 1]};
})
.attr("transform", function(d) {
if (d.value) {
return "translate(" + xScale(d.value.year) + "," + yScale(d.value.amount) + ")";
} else {
return null;
}
})
.attr("x", 3)
.attr("dy", 4)
.style("font-size", 10)
.style("font-family", "Abril Fatface")
.text(d => d.country)
.classed("hidden", function(d) {
if (d.value && d.value.amount > 700000) {
return false
} else {
return true;
}
})
And a function where I'm trying to assign a class:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
let currentId = d3.select(this).select("path#" + pathID)
console.log("Current ID: ",currentId)
.classed("highlight", true);
}
javascript arrays object d3.js
Trying to highlight current path line by grabbing pathID of a current path and assigning it a class .highlight that makes stroke-width: 2px
.
console.log shows current pathID but it doesn't want to assign a class. Please help. What am I doing wrong here?
Codepen
//Generate group (main)
let groups = svg.selectAll("g")
.data(dataset)
.enter()
.append("g")
.on("mouseover", function(d) {
d3.select(this)
.call(revealCityName)
})
.on("mouseout", hideCityName)
//Path (path #, path line)
groups
.append("path").classed("line", true)
.attr("id", function(d) {
console.log(d.country)
if (d && d.length != 0) {
return d.country.replace(/ |,|./g, '_');
}
})
.attr("d", d => line(d.emissions))
.classed("unfocused", true)
.style("stroke-width", d =>
d.country === "China" ? 1 : 0.2
)
.on("mouseover", highlightPath);
//Text (contries on the left y axis)
groups
.append("text")
.datum(function(d) {
return { country: d.country, value: d.emissions[d.emissions.length - 1]};
})
.attr("transform", function(d) {
if (d.value) {
return "translate(" + xScale(d.value.year) + "," + yScale(d.value.amount) + ")";
} else {
return null;
}
})
.attr("x", 3)
.attr("dy", 4)
.style("font-size", 10)
.style("font-family", "Abril Fatface")
.text(d => d.country)
.classed("hidden", function(d) {
if (d.value && d.value.amount > 700000) {
return false
} else {
return true;
}
})
And a function where I'm trying to assign a class:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
let currentId = d3.select(this).select("path#" + pathID)
console.log("Current ID: ",currentId)
.classed("highlight", true);
}
javascript arrays object d3.js
javascript arrays object d3.js
asked Nov 13 '18 at 18:23


Edgar KiljakEdgar Kiljak
372112
372112
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
console.log
is splitting code. Here is the error:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
let currentId = d3.select(this).select("path#" + pathID)
console.log("Current ID: ",currentId) /* <<<<<<<<< HERE (line 218 in your codepen) */
.classed("highlight", true);
}
Should be:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
let currentId = d3.select(this).classed('highlight', true) // thanks to @shashank
console.log("Current ID: ", currentId)
}
Updated demo: https://codepen.io/anon/pen/qQRJXp
UPDATED after @Shashank comment
highlightPath
is amouseover
event bound to the path which results in
d3.select(this)
to be the path itself and so you won't need any
.select(path#pathID)
in this case but just
d3.select(this).classed('highlighted', true)
Good catch but updated codepen still isn't properly applying highlight class.
– H.P.
Nov 13 '18 at 19:13
1
This question title is d3 Cannot read property 'classed' of undefined - I answered on this. Please avoid asking multiple questions in one article.
– qiAlex
Nov 13 '18 at 20:05
1
@EdgarKiljak This is the correct answer to your question. If you comment out theconsole.log()
or move the line as suggested by @qiAlex's answer the codepen works just fine.
– altocumulus
Nov 13 '18 at 20:14
1
Sorry to interrupt but I see that it doesn't work and here's why:highlightPath
is a mouseover event bound to thepath
which results ind3.select(this)
to be thepath
itself and so you won't need any.select(path#pathID)
in this case but justd3.select(this).classed('highlighted', true)
should work.
– Shashank
Nov 13 '18 at 20:41
1
@EdgarKiljak Again, this is a different question. It's about the specificity of CSS selectors. In your case the classhighlight
is in fact set on the path. You won't see any change, though, because it is trumped by the more specificpath.line
selector and thestyle
attribute on the element itself.
– altocumulus
Nov 13 '18 at 23:32
|
show 5 more comments
Try this:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
d3.select("path#" + pathID)
.attr("class", "highlight");
}
Thanks but it doesn't work
– Edgar Kiljak
Nov 13 '18 at 19:57
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53287312%2fd3-cannot-read-property-classed-of-undefined%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
console.log
is splitting code. Here is the error:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
let currentId = d3.select(this).select("path#" + pathID)
console.log("Current ID: ",currentId) /* <<<<<<<<< HERE (line 218 in your codepen) */
.classed("highlight", true);
}
Should be:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
let currentId = d3.select(this).classed('highlight', true) // thanks to @shashank
console.log("Current ID: ", currentId)
}
Updated demo: https://codepen.io/anon/pen/qQRJXp
UPDATED after @Shashank comment
highlightPath
is amouseover
event bound to the path which results in
d3.select(this)
to be the path itself and so you won't need any
.select(path#pathID)
in this case but just
d3.select(this).classed('highlighted', true)
Good catch but updated codepen still isn't properly applying highlight class.
– H.P.
Nov 13 '18 at 19:13
1
This question title is d3 Cannot read property 'classed' of undefined - I answered on this. Please avoid asking multiple questions in one article.
– qiAlex
Nov 13 '18 at 20:05
1
@EdgarKiljak This is the correct answer to your question. If you comment out theconsole.log()
or move the line as suggested by @qiAlex's answer the codepen works just fine.
– altocumulus
Nov 13 '18 at 20:14
1
Sorry to interrupt but I see that it doesn't work and here's why:highlightPath
is a mouseover event bound to thepath
which results ind3.select(this)
to be thepath
itself and so you won't need any.select(path#pathID)
in this case but justd3.select(this).classed('highlighted', true)
should work.
– Shashank
Nov 13 '18 at 20:41
1
@EdgarKiljak Again, this is a different question. It's about the specificity of CSS selectors. In your case the classhighlight
is in fact set on the path. You won't see any change, though, because it is trumped by the more specificpath.line
selector and thestyle
attribute on the element itself.
– altocumulus
Nov 13 '18 at 23:32
|
show 5 more comments
console.log
is splitting code. Here is the error:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
let currentId = d3.select(this).select("path#" + pathID)
console.log("Current ID: ",currentId) /* <<<<<<<<< HERE (line 218 in your codepen) */
.classed("highlight", true);
}
Should be:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
let currentId = d3.select(this).classed('highlight', true) // thanks to @shashank
console.log("Current ID: ", currentId)
}
Updated demo: https://codepen.io/anon/pen/qQRJXp
UPDATED after @Shashank comment
highlightPath
is amouseover
event bound to the path which results in
d3.select(this)
to be the path itself and so you won't need any
.select(path#pathID)
in this case but just
d3.select(this).classed('highlighted', true)
Good catch but updated codepen still isn't properly applying highlight class.
– H.P.
Nov 13 '18 at 19:13
1
This question title is d3 Cannot read property 'classed' of undefined - I answered on this. Please avoid asking multiple questions in one article.
– qiAlex
Nov 13 '18 at 20:05
1
@EdgarKiljak This is the correct answer to your question. If you comment out theconsole.log()
or move the line as suggested by @qiAlex's answer the codepen works just fine.
– altocumulus
Nov 13 '18 at 20:14
1
Sorry to interrupt but I see that it doesn't work and here's why:highlightPath
is a mouseover event bound to thepath
which results ind3.select(this)
to be thepath
itself and so you won't need any.select(path#pathID)
in this case but justd3.select(this).classed('highlighted', true)
should work.
– Shashank
Nov 13 '18 at 20:41
1
@EdgarKiljak Again, this is a different question. It's about the specificity of CSS selectors. In your case the classhighlight
is in fact set on the path. You won't see any change, though, because it is trumped by the more specificpath.line
selector and thestyle
attribute on the element itself.
– altocumulus
Nov 13 '18 at 23:32
|
show 5 more comments
console.log
is splitting code. Here is the error:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
let currentId = d3.select(this).select("path#" + pathID)
console.log("Current ID: ",currentId) /* <<<<<<<<< HERE (line 218 in your codepen) */
.classed("highlight", true);
}
Should be:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
let currentId = d3.select(this).classed('highlight', true) // thanks to @shashank
console.log("Current ID: ", currentId)
}
Updated demo: https://codepen.io/anon/pen/qQRJXp
UPDATED after @Shashank comment
highlightPath
is amouseover
event bound to the path which results in
d3.select(this)
to be the path itself and so you won't need any
.select(path#pathID)
in this case but just
d3.select(this).classed('highlighted', true)
console.log
is splitting code. Here is the error:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
let currentId = d3.select(this).select("path#" + pathID)
console.log("Current ID: ",currentId) /* <<<<<<<<< HERE (line 218 in your codepen) */
.classed("highlight", true);
}
Should be:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
let currentId = d3.select(this).classed('highlight', true) // thanks to @shashank
console.log("Current ID: ", currentId)
}
Updated demo: https://codepen.io/anon/pen/qQRJXp
UPDATED after @Shashank comment
highlightPath
is amouseover
event bound to the path which results in
d3.select(this)
to be the path itself and so you won't need any
.select(path#pathID)
in this case but just
d3.select(this).classed('highlighted', true)
edited Nov 13 '18 at 21:26
answered Nov 13 '18 at 18:29


qiAlexqiAlex
2,0261724
2,0261724
Good catch but updated codepen still isn't properly applying highlight class.
– H.P.
Nov 13 '18 at 19:13
1
This question title is d3 Cannot read property 'classed' of undefined - I answered on this. Please avoid asking multiple questions in one article.
– qiAlex
Nov 13 '18 at 20:05
1
@EdgarKiljak This is the correct answer to your question. If you comment out theconsole.log()
or move the line as suggested by @qiAlex's answer the codepen works just fine.
– altocumulus
Nov 13 '18 at 20:14
1
Sorry to interrupt but I see that it doesn't work and here's why:highlightPath
is a mouseover event bound to thepath
which results ind3.select(this)
to be thepath
itself and so you won't need any.select(path#pathID)
in this case but justd3.select(this).classed('highlighted', true)
should work.
– Shashank
Nov 13 '18 at 20:41
1
@EdgarKiljak Again, this is a different question. It's about the specificity of CSS selectors. In your case the classhighlight
is in fact set on the path. You won't see any change, though, because it is trumped by the more specificpath.line
selector and thestyle
attribute on the element itself.
– altocumulus
Nov 13 '18 at 23:32
|
show 5 more comments
Good catch but updated codepen still isn't properly applying highlight class.
– H.P.
Nov 13 '18 at 19:13
1
This question title is d3 Cannot read property 'classed' of undefined - I answered on this. Please avoid asking multiple questions in one article.
– qiAlex
Nov 13 '18 at 20:05
1
@EdgarKiljak This is the correct answer to your question. If you comment out theconsole.log()
or move the line as suggested by @qiAlex's answer the codepen works just fine.
– altocumulus
Nov 13 '18 at 20:14
1
Sorry to interrupt but I see that it doesn't work and here's why:highlightPath
is a mouseover event bound to thepath
which results ind3.select(this)
to be thepath
itself and so you won't need any.select(path#pathID)
in this case but justd3.select(this).classed('highlighted', true)
should work.
– Shashank
Nov 13 '18 at 20:41
1
@EdgarKiljak Again, this is a different question. It's about the specificity of CSS selectors. In your case the classhighlight
is in fact set on the path. You won't see any change, though, because it is trumped by the more specificpath.line
selector and thestyle
attribute on the element itself.
– altocumulus
Nov 13 '18 at 23:32
Good catch but updated codepen still isn't properly applying highlight class.
– H.P.
Nov 13 '18 at 19:13
Good catch but updated codepen still isn't properly applying highlight class.
– H.P.
Nov 13 '18 at 19:13
1
1
This question title is d3 Cannot read property 'classed' of undefined - I answered on this. Please avoid asking multiple questions in one article.
– qiAlex
Nov 13 '18 at 20:05
This question title is d3 Cannot read property 'classed' of undefined - I answered on this. Please avoid asking multiple questions in one article.
– qiAlex
Nov 13 '18 at 20:05
1
1
@EdgarKiljak This is the correct answer to your question. If you comment out the
console.log()
or move the line as suggested by @qiAlex's answer the codepen works just fine.– altocumulus
Nov 13 '18 at 20:14
@EdgarKiljak This is the correct answer to your question. If you comment out the
console.log()
or move the line as suggested by @qiAlex's answer the codepen works just fine.– altocumulus
Nov 13 '18 at 20:14
1
1
Sorry to interrupt but I see that it doesn't work and here's why:
highlightPath
is a mouseover event bound to the path
which results in d3.select(this)
to be the path
itself and so you won't need any .select(path#pathID)
in this case but just d3.select(this).classed('highlighted', true)
should work.– Shashank
Nov 13 '18 at 20:41
Sorry to interrupt but I see that it doesn't work and here's why:
highlightPath
is a mouseover event bound to the path
which results in d3.select(this)
to be the path
itself and so you won't need any .select(path#pathID)
in this case but just d3.select(this).classed('highlighted', true)
should work.– Shashank
Nov 13 '18 at 20:41
1
1
@EdgarKiljak Again, this is a different question. It's about the specificity of CSS selectors. In your case the class
highlight
is in fact set on the path. You won't see any change, though, because it is trumped by the more specific path.line
selector and the style
attribute on the element itself.– altocumulus
Nov 13 '18 at 23:32
@EdgarKiljak Again, this is a different question. It's about the specificity of CSS selectors. In your case the class
highlight
is in fact set on the path. You won't see any change, though, because it is trumped by the more specific path.line
selector and the style
attribute on the element itself.– altocumulus
Nov 13 '18 at 23:32
|
show 5 more comments
Try this:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
d3.select("path#" + pathID)
.attr("class", "highlight");
}
Thanks but it doesn't work
– Edgar Kiljak
Nov 13 '18 at 19:57
add a comment |
Try this:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
d3.select("path#" + pathID)
.attr("class", "highlight");
}
Thanks but it doesn't work
– Edgar Kiljak
Nov 13 '18 at 19:57
add a comment |
Try this:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
d3.select("path#" + pathID)
.attr("class", "highlight");
}
Try this:
function highlightPath(d) {
let pathID = d3.select(this).attr("id");
console.log(pathID);
d3.select("path#" + pathID)
.attr("class", "highlight");
}
edited Nov 13 '18 at 19:11
answered Nov 13 '18 at 18:49
H.P.H.P.
565
565
Thanks but it doesn't work
– Edgar Kiljak
Nov 13 '18 at 19:57
add a comment |
Thanks but it doesn't work
– Edgar Kiljak
Nov 13 '18 at 19:57
Thanks but it doesn't work
– Edgar Kiljak
Nov 13 '18 at 19:57
Thanks but it doesn't work
– Edgar Kiljak
Nov 13 '18 at 19:57
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53287312%2fd3-cannot-read-property-classed-of-undefined%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ihAvhD