Appending random True or False to the end of each line in Python
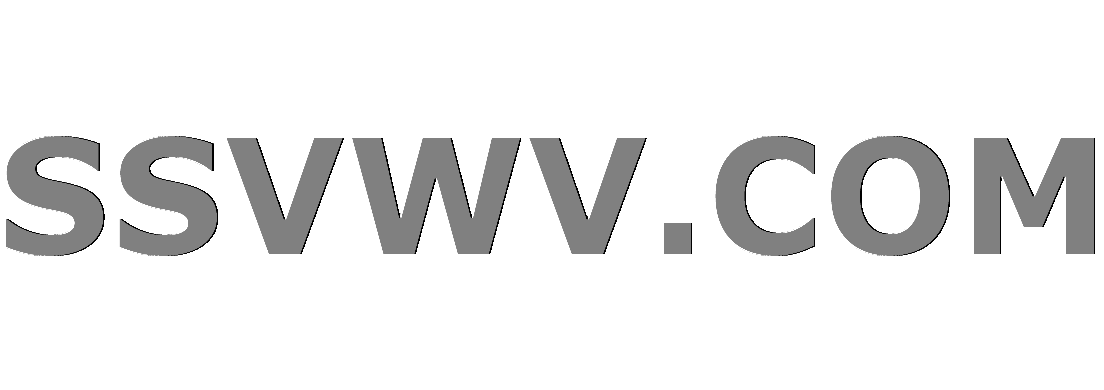
Multi tool use
I have a text file that is organized into lines and columns. Here is a sample of the file
ATOM 1 N MET A 0 7.721 14.287 22.993 1.00 56.77 N
ATOM 2 CA MET A 0 8.721 15.393 22.939 1.00 56.81 C
ATOM 3 C MET A 0 10.044 14.985 23.585 1.00 54.96 C
ATOM 4 O MET A 0 11.059 15.660 23.408 1.00 56.23 O
ATOM 5 CB MET A 0 8.167 16.640 23.642 1.00 59.72 C
ATOM 6 CG MET A 0 7.207 16.349 24.796 1.00 62.33 C
ATOM 7 SD MET A 0 5.772 17.459 24.822 1.00 66.48 S
What I want to do is to add a random true or false to the end of each line, ideally in its own column, something that looks like this
ATOM 1 N MET A 0 7.721 14.287 22.993 1.00 56.77 N True
ATOM 2 CA MET A 0 8.721 15.393 22.939 1.00 56.81 C False
ATOM 3 C MET A 0 10.044 14.985 23.585 1.00 54.96 C False
ATOM 4 O MET A 0 11.059 15.660 23.408 1.00 56.23 O False
ATOM 5 CB MET A 0 8.167 16.640 23.642 1.00 59.72 C True
ATOM 6 CG MET A 0 7.207 16.349 24.796 1.00 62.33 C False
ATOM 7 SD MET A 0 5.772 17.459 24.822 1.00 66.48 S False
Here is the function that I have written to try to accomplish this
def random_TF(pdbFileName): #This function will randomly generate TRUE or FALSE
with open(pdbFileName, 'r') as e:
with open('1fkqTF.txt', 'w') as f:
for i, line in enumerate(e):
line = line.rstrip('n')
line += str(random.choice([True, False]))
e.close()
f.close()
However, when I call the function it returns a blank text file.
python bioinformatics python-3.7
add a comment |
I have a text file that is organized into lines and columns. Here is a sample of the file
ATOM 1 N MET A 0 7.721 14.287 22.993 1.00 56.77 N
ATOM 2 CA MET A 0 8.721 15.393 22.939 1.00 56.81 C
ATOM 3 C MET A 0 10.044 14.985 23.585 1.00 54.96 C
ATOM 4 O MET A 0 11.059 15.660 23.408 1.00 56.23 O
ATOM 5 CB MET A 0 8.167 16.640 23.642 1.00 59.72 C
ATOM 6 CG MET A 0 7.207 16.349 24.796 1.00 62.33 C
ATOM 7 SD MET A 0 5.772 17.459 24.822 1.00 66.48 S
What I want to do is to add a random true or false to the end of each line, ideally in its own column, something that looks like this
ATOM 1 N MET A 0 7.721 14.287 22.993 1.00 56.77 N True
ATOM 2 CA MET A 0 8.721 15.393 22.939 1.00 56.81 C False
ATOM 3 C MET A 0 10.044 14.985 23.585 1.00 54.96 C False
ATOM 4 O MET A 0 11.059 15.660 23.408 1.00 56.23 O False
ATOM 5 CB MET A 0 8.167 16.640 23.642 1.00 59.72 C True
ATOM 6 CG MET A 0 7.207 16.349 24.796 1.00 62.33 C False
ATOM 7 SD MET A 0 5.772 17.459 24.822 1.00 66.48 S False
Here is the function that I have written to try to accomplish this
def random_TF(pdbFileName): #This function will randomly generate TRUE or FALSE
with open(pdbFileName, 'r') as e:
with open('1fkqTF.txt', 'w') as f:
for i, line in enumerate(e):
line = line.rstrip('n')
line += str(random.choice([True, False]))
e.close()
f.close()
However, when I call the function it returns a blank text file.
python bioinformatics python-3.7
Similar (if not duplicate): Add a new column in text file
– narendra-choudhary
Nov 13 '18 at 18:24
add a comment |
I have a text file that is organized into lines and columns. Here is a sample of the file
ATOM 1 N MET A 0 7.721 14.287 22.993 1.00 56.77 N
ATOM 2 CA MET A 0 8.721 15.393 22.939 1.00 56.81 C
ATOM 3 C MET A 0 10.044 14.985 23.585 1.00 54.96 C
ATOM 4 O MET A 0 11.059 15.660 23.408 1.00 56.23 O
ATOM 5 CB MET A 0 8.167 16.640 23.642 1.00 59.72 C
ATOM 6 CG MET A 0 7.207 16.349 24.796 1.00 62.33 C
ATOM 7 SD MET A 0 5.772 17.459 24.822 1.00 66.48 S
What I want to do is to add a random true or false to the end of each line, ideally in its own column, something that looks like this
ATOM 1 N MET A 0 7.721 14.287 22.993 1.00 56.77 N True
ATOM 2 CA MET A 0 8.721 15.393 22.939 1.00 56.81 C False
ATOM 3 C MET A 0 10.044 14.985 23.585 1.00 54.96 C False
ATOM 4 O MET A 0 11.059 15.660 23.408 1.00 56.23 O False
ATOM 5 CB MET A 0 8.167 16.640 23.642 1.00 59.72 C True
ATOM 6 CG MET A 0 7.207 16.349 24.796 1.00 62.33 C False
ATOM 7 SD MET A 0 5.772 17.459 24.822 1.00 66.48 S False
Here is the function that I have written to try to accomplish this
def random_TF(pdbFileName): #This function will randomly generate TRUE or FALSE
with open(pdbFileName, 'r') as e:
with open('1fkqTF.txt', 'w') as f:
for i, line in enumerate(e):
line = line.rstrip('n')
line += str(random.choice([True, False]))
e.close()
f.close()
However, when I call the function it returns a blank text file.
python bioinformatics python-3.7
I have a text file that is organized into lines and columns. Here is a sample of the file
ATOM 1 N MET A 0 7.721 14.287 22.993 1.00 56.77 N
ATOM 2 CA MET A 0 8.721 15.393 22.939 1.00 56.81 C
ATOM 3 C MET A 0 10.044 14.985 23.585 1.00 54.96 C
ATOM 4 O MET A 0 11.059 15.660 23.408 1.00 56.23 O
ATOM 5 CB MET A 0 8.167 16.640 23.642 1.00 59.72 C
ATOM 6 CG MET A 0 7.207 16.349 24.796 1.00 62.33 C
ATOM 7 SD MET A 0 5.772 17.459 24.822 1.00 66.48 S
What I want to do is to add a random true or false to the end of each line, ideally in its own column, something that looks like this
ATOM 1 N MET A 0 7.721 14.287 22.993 1.00 56.77 N True
ATOM 2 CA MET A 0 8.721 15.393 22.939 1.00 56.81 C False
ATOM 3 C MET A 0 10.044 14.985 23.585 1.00 54.96 C False
ATOM 4 O MET A 0 11.059 15.660 23.408 1.00 56.23 O False
ATOM 5 CB MET A 0 8.167 16.640 23.642 1.00 59.72 C True
ATOM 6 CG MET A 0 7.207 16.349 24.796 1.00 62.33 C False
ATOM 7 SD MET A 0 5.772 17.459 24.822 1.00 66.48 S False
Here is the function that I have written to try to accomplish this
def random_TF(pdbFileName): #This function will randomly generate TRUE or FALSE
with open(pdbFileName, 'r') as e:
with open('1fkqTF.txt', 'w') as f:
for i, line in enumerate(e):
line = line.rstrip('n')
line += str(random.choice([True, False]))
e.close()
f.close()
However, when I call the function it returns a blank text file.
python bioinformatics python-3.7
python bioinformatics python-3.7
asked Nov 13 '18 at 18:22
flannel_bioinformaticianflannel_bioinformatician
616
616
Similar (if not duplicate): Add a new column in text file
– narendra-choudhary
Nov 13 '18 at 18:24
add a comment |
Similar (if not duplicate): Add a new column in text file
– narendra-choudhary
Nov 13 '18 at 18:24
Similar (if not duplicate): Add a new column in text file
– narendra-choudhary
Nov 13 '18 at 18:24
Similar (if not duplicate): Add a new column in text file
– narendra-choudhary
Nov 13 '18 at 18:24
add a comment |
2 Answers
2
active
oldest
votes
you forgot f.write(line)
. I've included 'n' and 't' to improve the formatting.
def random_TF(pdbFileName): #This function will randomly generate TRUE or FALSE
with open(pdbFileName, 'r') as e:
with open('text2.txt', 'w') as f:
for i, line in enumerate(e):
line = line.rstrip('n')
line += 't' + str(random.choice([True, False])) + 'n'
f.write(line)
e.close()
f.close()
add a comment |
You aren't actually writing to the file. You need to call:
f.write(line + "n")
after you have updated the line.
Also, you don't need to call e.close()
or f.close()
, that's the advantage of using the context manager with
.
Finally, there is likely a better way to do what you are doing using a proper data manipulation library such as pandas
, but without additional context as to why you are doing this it will be tough to provide much insight.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53287296%2fappending-random-true-or-false-to-the-end-of-each-line-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
you forgot f.write(line)
. I've included 'n' and 't' to improve the formatting.
def random_TF(pdbFileName): #This function will randomly generate TRUE or FALSE
with open(pdbFileName, 'r') as e:
with open('text2.txt', 'w') as f:
for i, line in enumerate(e):
line = line.rstrip('n')
line += 't' + str(random.choice([True, False])) + 'n'
f.write(line)
e.close()
f.close()
add a comment |
you forgot f.write(line)
. I've included 'n' and 't' to improve the formatting.
def random_TF(pdbFileName): #This function will randomly generate TRUE or FALSE
with open(pdbFileName, 'r') as e:
with open('text2.txt', 'w') as f:
for i, line in enumerate(e):
line = line.rstrip('n')
line += 't' + str(random.choice([True, False])) + 'n'
f.write(line)
e.close()
f.close()
add a comment |
you forgot f.write(line)
. I've included 'n' and 't' to improve the formatting.
def random_TF(pdbFileName): #This function will randomly generate TRUE or FALSE
with open(pdbFileName, 'r') as e:
with open('text2.txt', 'w') as f:
for i, line in enumerate(e):
line = line.rstrip('n')
line += 't' + str(random.choice([True, False])) + 'n'
f.write(line)
e.close()
f.close()
you forgot f.write(line)
. I've included 'n' and 't' to improve the formatting.
def random_TF(pdbFileName): #This function will randomly generate TRUE or FALSE
with open(pdbFileName, 'r') as e:
with open('text2.txt', 'w') as f:
for i, line in enumerate(e):
line = line.rstrip('n')
line += 't' + str(random.choice([True, False])) + 'n'
f.write(line)
e.close()
f.close()
answered Nov 13 '18 at 18:45
MaedaMaeda
39957
39957
add a comment |
add a comment |
You aren't actually writing to the file. You need to call:
f.write(line + "n")
after you have updated the line.
Also, you don't need to call e.close()
or f.close()
, that's the advantage of using the context manager with
.
Finally, there is likely a better way to do what you are doing using a proper data manipulation library such as pandas
, but without additional context as to why you are doing this it will be tough to provide much insight.
add a comment |
You aren't actually writing to the file. You need to call:
f.write(line + "n")
after you have updated the line.
Also, you don't need to call e.close()
or f.close()
, that's the advantage of using the context manager with
.
Finally, there is likely a better way to do what you are doing using a proper data manipulation library such as pandas
, but without additional context as to why you are doing this it will be tough to provide much insight.
add a comment |
You aren't actually writing to the file. You need to call:
f.write(line + "n")
after you have updated the line.
Also, you don't need to call e.close()
or f.close()
, that's the advantage of using the context manager with
.
Finally, there is likely a better way to do what you are doing using a proper data manipulation library such as pandas
, but without additional context as to why you are doing this it will be tough to provide much insight.
You aren't actually writing to the file. You need to call:
f.write(line + "n")
after you have updated the line.
Also, you don't need to call e.close()
or f.close()
, that's the advantage of using the context manager with
.
Finally, there is likely a better way to do what you are doing using a proper data manipulation library such as pandas
, but without additional context as to why you are doing this it will be tough to provide much insight.
answered Nov 13 '18 at 18:24
Nick ChapmanNick Chapman
2,2611126
2,2611126
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53287296%2fappending-random-true-or-false-to-the-end-of-each-line-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qRNgq,XTzSX WwhTKLETgRzabb dH0rc lfiU,Ye,cj EiZAzITMQhpw,CNvn,HwcmFLWDC4QEh5nyZ5VUL9Py6i5xO3kik byj
Similar (if not duplicate): Add a new column in text file
– narendra-choudhary
Nov 13 '18 at 18:24