Child processes not running concurrently
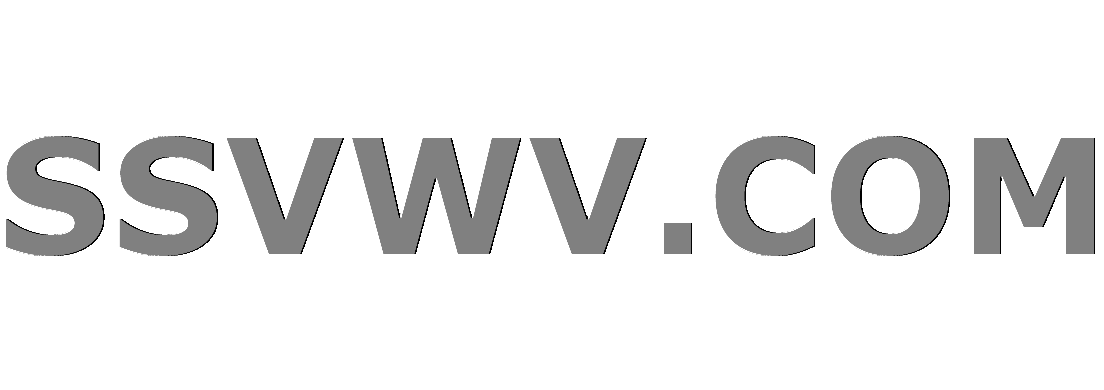
Multi tool use
So I am writing a hotel reservation system where I have hotel rooms stored in shared memory and will have an x number of customers reserve, cancel, pay, etc the rooms. Each customer will be a child process and they should all be running concurrently, trying to do the stated transactions to the rooms.
Here is my code for forking the child processes:
int pid[10];
for(int i = 0; i < numOfCustomers; i++) {
pid[i] = fork();
if(pid[i] < 0) {
perror("Bad fork");
}
else if(pid[i] == 0) { //is child i (Each customer is a child process)
cout << "CUSTOMER: " << i << endl;
while(!customers[i].transactions.empty()) { //runs until customer finishes all transactions
string op = customers[i].transactions.front();
if(op == "reserve") {
if(sem_wait(semid, 0))
exit(1);
Reserve tempq = customers[i].reservequeue.front();
for(int i = 0; i < tempq.roomcounter; i++) {
int roomnum = tempq.roomqueue.front();
if(shared_memory[roomnum-1].isavailable == true) {
shared_memory[roomnum-1].isavailable = false;
shared_memory[roomnum-1].day = tempq.day;
shared_memory[roomnum-1].month = tempq.month;
shared_memory[roomnum-1].year = tempq.year;
shared_memory[roomnum-1].customer = cust;
}
else {
cout << "Room " << roomnum << " already taken." << endl;
}
tempq.roomqueue.pop();
}
customers[i].reservequeue.pop();
if(sem_signal(semid, 0))
exit(1);
}
else if(op == "cancel") { //not done yet
cout << "cancel" << endl;
}
else if(op == "check") { //not done yet
cout << "check" << endl;
}
else if(op == "pay") { //not done yet
cout << "pay" << endl;
}
customers[i].transactions.pop();
}
exit(0);
}
else { //is parent
}
}
//waits for each process to finish
for (int i = 0; i < numOfCustomers; i++) {
wait(NULL);
}
The problem is that it does everything in child one first, finishes, then does everything in child 2. Processes aren't running concurrently which defeats the whole purpose of having shared memory and using semaphores.
Any help would be greatly appreciated, thanks.
c++ linux fork child-process
|
show 4 more comments
So I am writing a hotel reservation system where I have hotel rooms stored in shared memory and will have an x number of customers reserve, cancel, pay, etc the rooms. Each customer will be a child process and they should all be running concurrently, trying to do the stated transactions to the rooms.
Here is my code for forking the child processes:
int pid[10];
for(int i = 0; i < numOfCustomers; i++) {
pid[i] = fork();
if(pid[i] < 0) {
perror("Bad fork");
}
else if(pid[i] == 0) { //is child i (Each customer is a child process)
cout << "CUSTOMER: " << i << endl;
while(!customers[i].transactions.empty()) { //runs until customer finishes all transactions
string op = customers[i].transactions.front();
if(op == "reserve") {
if(sem_wait(semid, 0))
exit(1);
Reserve tempq = customers[i].reservequeue.front();
for(int i = 0; i < tempq.roomcounter; i++) {
int roomnum = tempq.roomqueue.front();
if(shared_memory[roomnum-1].isavailable == true) {
shared_memory[roomnum-1].isavailable = false;
shared_memory[roomnum-1].day = tempq.day;
shared_memory[roomnum-1].month = tempq.month;
shared_memory[roomnum-1].year = tempq.year;
shared_memory[roomnum-1].customer = cust;
}
else {
cout << "Room " << roomnum << " already taken." << endl;
}
tempq.roomqueue.pop();
}
customers[i].reservequeue.pop();
if(sem_signal(semid, 0))
exit(1);
}
else if(op == "cancel") { //not done yet
cout << "cancel" << endl;
}
else if(op == "check") { //not done yet
cout << "check" << endl;
}
else if(op == "pay") { //not done yet
cout << "pay" << endl;
}
customers[i].transactions.pop();
}
exit(0);
}
else { //is parent
}
}
//waits for each process to finish
for (int i = 0; i < numOfCustomers; i++) {
wait(NULL);
}
The problem is that it does everything in child one first, finishes, then does everything in child 2. Processes aren't running concurrently which defeats the whole purpose of having shared memory and using semaphores.
Any help would be greatly appreciated, thanks.
c++ linux fork child-process
I think I can pretty much guarantee that no sensible hotel reservation system would use such a convoluted architecture.
– Neil Butterworth
Nov 13 '18 at 21:16
@NeilButterworth it's a programming assignment to learn about shared memory and semaphore usage.
– Bzm10823
Nov 13 '18 at 21:18
Have you tried adding delays, to ensure that the first child lasts long enough to come into conflict with the second?
– Beta
Nov 13 '18 at 21:29
1
Out of curiosity, what platform are you doing this on? I ask because everysem_wait
I've ever used (linux, osx, hpux, solaris, aix, etc) all take a single argument: the sem ptr itself.
– WhozCraig
Nov 13 '18 at 21:30
@WhozCraig using linux, I made a sem_signal and sem_wait function to avoid having to make a struct and doing semop every time I need to signal and wait.
– Bzm10823
Nov 13 '18 at 21:38
|
show 4 more comments
So I am writing a hotel reservation system where I have hotel rooms stored in shared memory and will have an x number of customers reserve, cancel, pay, etc the rooms. Each customer will be a child process and they should all be running concurrently, trying to do the stated transactions to the rooms.
Here is my code for forking the child processes:
int pid[10];
for(int i = 0; i < numOfCustomers; i++) {
pid[i] = fork();
if(pid[i] < 0) {
perror("Bad fork");
}
else if(pid[i] == 0) { //is child i (Each customer is a child process)
cout << "CUSTOMER: " << i << endl;
while(!customers[i].transactions.empty()) { //runs until customer finishes all transactions
string op = customers[i].transactions.front();
if(op == "reserve") {
if(sem_wait(semid, 0))
exit(1);
Reserve tempq = customers[i].reservequeue.front();
for(int i = 0; i < tempq.roomcounter; i++) {
int roomnum = tempq.roomqueue.front();
if(shared_memory[roomnum-1].isavailable == true) {
shared_memory[roomnum-1].isavailable = false;
shared_memory[roomnum-1].day = tempq.day;
shared_memory[roomnum-1].month = tempq.month;
shared_memory[roomnum-1].year = tempq.year;
shared_memory[roomnum-1].customer = cust;
}
else {
cout << "Room " << roomnum << " already taken." << endl;
}
tempq.roomqueue.pop();
}
customers[i].reservequeue.pop();
if(sem_signal(semid, 0))
exit(1);
}
else if(op == "cancel") { //not done yet
cout << "cancel" << endl;
}
else if(op == "check") { //not done yet
cout << "check" << endl;
}
else if(op == "pay") { //not done yet
cout << "pay" << endl;
}
customers[i].transactions.pop();
}
exit(0);
}
else { //is parent
}
}
//waits for each process to finish
for (int i = 0; i < numOfCustomers; i++) {
wait(NULL);
}
The problem is that it does everything in child one first, finishes, then does everything in child 2. Processes aren't running concurrently which defeats the whole purpose of having shared memory and using semaphores.
Any help would be greatly appreciated, thanks.
c++ linux fork child-process
So I am writing a hotel reservation system where I have hotel rooms stored in shared memory and will have an x number of customers reserve, cancel, pay, etc the rooms. Each customer will be a child process and they should all be running concurrently, trying to do the stated transactions to the rooms.
Here is my code for forking the child processes:
int pid[10];
for(int i = 0; i < numOfCustomers; i++) {
pid[i] = fork();
if(pid[i] < 0) {
perror("Bad fork");
}
else if(pid[i] == 0) { //is child i (Each customer is a child process)
cout << "CUSTOMER: " << i << endl;
while(!customers[i].transactions.empty()) { //runs until customer finishes all transactions
string op = customers[i].transactions.front();
if(op == "reserve") {
if(sem_wait(semid, 0))
exit(1);
Reserve tempq = customers[i].reservequeue.front();
for(int i = 0; i < tempq.roomcounter; i++) {
int roomnum = tempq.roomqueue.front();
if(shared_memory[roomnum-1].isavailable == true) {
shared_memory[roomnum-1].isavailable = false;
shared_memory[roomnum-1].day = tempq.day;
shared_memory[roomnum-1].month = tempq.month;
shared_memory[roomnum-1].year = tempq.year;
shared_memory[roomnum-1].customer = cust;
}
else {
cout << "Room " << roomnum << " already taken." << endl;
}
tempq.roomqueue.pop();
}
customers[i].reservequeue.pop();
if(sem_signal(semid, 0))
exit(1);
}
else if(op == "cancel") { //not done yet
cout << "cancel" << endl;
}
else if(op == "check") { //not done yet
cout << "check" << endl;
}
else if(op == "pay") { //not done yet
cout << "pay" << endl;
}
customers[i].transactions.pop();
}
exit(0);
}
else { //is parent
}
}
//waits for each process to finish
for (int i = 0; i < numOfCustomers; i++) {
wait(NULL);
}
The problem is that it does everything in child one first, finishes, then does everything in child 2. Processes aren't running concurrently which defeats the whole purpose of having shared memory and using semaphores.
Any help would be greatly appreciated, thanks.
c++ linux fork child-process
c++ linux fork child-process
asked Nov 13 '18 at 21:15
Bzm10823Bzm10823
32
32
I think I can pretty much guarantee that no sensible hotel reservation system would use such a convoluted architecture.
– Neil Butterworth
Nov 13 '18 at 21:16
@NeilButterworth it's a programming assignment to learn about shared memory and semaphore usage.
– Bzm10823
Nov 13 '18 at 21:18
Have you tried adding delays, to ensure that the first child lasts long enough to come into conflict with the second?
– Beta
Nov 13 '18 at 21:29
1
Out of curiosity, what platform are you doing this on? I ask because everysem_wait
I've ever used (linux, osx, hpux, solaris, aix, etc) all take a single argument: the sem ptr itself.
– WhozCraig
Nov 13 '18 at 21:30
@WhozCraig using linux, I made a sem_signal and sem_wait function to avoid having to make a struct and doing semop every time I need to signal and wait.
– Bzm10823
Nov 13 '18 at 21:38
|
show 4 more comments
I think I can pretty much guarantee that no sensible hotel reservation system would use such a convoluted architecture.
– Neil Butterworth
Nov 13 '18 at 21:16
@NeilButterworth it's a programming assignment to learn about shared memory and semaphore usage.
– Bzm10823
Nov 13 '18 at 21:18
Have you tried adding delays, to ensure that the first child lasts long enough to come into conflict with the second?
– Beta
Nov 13 '18 at 21:29
1
Out of curiosity, what platform are you doing this on? I ask because everysem_wait
I've ever used (linux, osx, hpux, solaris, aix, etc) all take a single argument: the sem ptr itself.
– WhozCraig
Nov 13 '18 at 21:30
@WhozCraig using linux, I made a sem_signal and sem_wait function to avoid having to make a struct and doing semop every time I need to signal and wait.
– Bzm10823
Nov 13 '18 at 21:38
I think I can pretty much guarantee that no sensible hotel reservation system would use such a convoluted architecture.
– Neil Butterworth
Nov 13 '18 at 21:16
I think I can pretty much guarantee that no sensible hotel reservation system would use such a convoluted architecture.
– Neil Butterworth
Nov 13 '18 at 21:16
@NeilButterworth it's a programming assignment to learn about shared memory and semaphore usage.
– Bzm10823
Nov 13 '18 at 21:18
@NeilButterworth it's a programming assignment to learn about shared memory and semaphore usage.
– Bzm10823
Nov 13 '18 at 21:18
Have you tried adding delays, to ensure that the first child lasts long enough to come into conflict with the second?
– Beta
Nov 13 '18 at 21:29
Have you tried adding delays, to ensure that the first child lasts long enough to come into conflict with the second?
– Beta
Nov 13 '18 at 21:29
1
1
Out of curiosity, what platform are you doing this on? I ask because every
sem_wait
I've ever used (linux, osx, hpux, solaris, aix, etc) all take a single argument: the sem ptr itself.– WhozCraig
Nov 13 '18 at 21:30
Out of curiosity, what platform are you doing this on? I ask because every
sem_wait
I've ever used (linux, osx, hpux, solaris, aix, etc) all take a single argument: the sem ptr itself.– WhozCraig
Nov 13 '18 at 21:30
@WhozCraig using linux, I made a sem_signal and sem_wait function to avoid having to make a struct and doing semop every time I need to signal and wait.
– Bzm10823
Nov 13 '18 at 21:38
@WhozCraig using linux, I made a sem_signal and sem_wait function to avoid having to make a struct and doing semop every time I need to signal and wait.
– Bzm10823
Nov 13 '18 at 21:38
|
show 4 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53289587%2fchild-processes-not-running-concurrently%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53289587%2fchild-processes-not-running-concurrently%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ZO4xJ 4Eq3SnAmzOKeGA LH4Ptpf,syXrxMRr1b3WKRUOmPI8NhSS v
I think I can pretty much guarantee that no sensible hotel reservation system would use such a convoluted architecture.
– Neil Butterworth
Nov 13 '18 at 21:16
@NeilButterworth it's a programming assignment to learn about shared memory and semaphore usage.
– Bzm10823
Nov 13 '18 at 21:18
Have you tried adding delays, to ensure that the first child lasts long enough to come into conflict with the second?
– Beta
Nov 13 '18 at 21:29
1
Out of curiosity, what platform are you doing this on? I ask because every
sem_wait
I've ever used (linux, osx, hpux, solaris, aix, etc) all take a single argument: the sem ptr itself.– WhozCraig
Nov 13 '18 at 21:30
@WhozCraig using linux, I made a sem_signal and sem_wait function to avoid having to make a struct and doing semop every time I need to signal and wait.
– Bzm10823
Nov 13 '18 at 21:38