c++ convert four unsined chars to one unsigned int
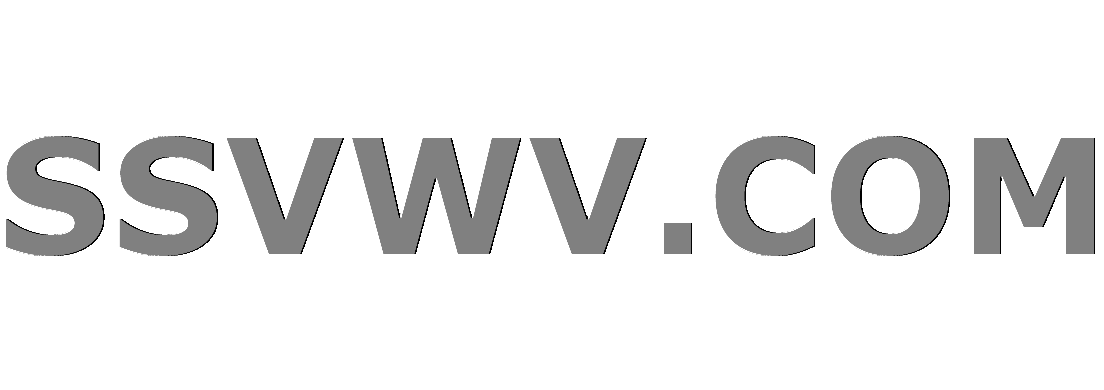
Multi tool use
In my c++ program I have a structure containing four unsigned char
members
struct msg
{
unsigned char zero; unsigned char one; unsigned char two; unsigned char three;
}
and created an array of struct
msg myMsg[4] = {{0x11,0x22,0xAA,0xCC},{...},{...},{...}};
now in a function I want to return an index of this array as an unsigned int
unsigned int procMsg(int n){
return myMsg[n]; // of course this is worng
}
a value like: 0xCCAA2211
c++ casting
|
show 3 more comments
In my c++ program I have a structure containing four unsigned char
members
struct msg
{
unsigned char zero; unsigned char one; unsigned char two; unsigned char three;
}
and created an array of struct
msg myMsg[4] = {{0x11,0x22,0xAA,0xCC},{...},{...},{...}};
now in a function I want to return an index of this array as an unsigned int
unsigned int procMsg(int n){
return myMsg[n]; // of course this is worng
}
a value like: 0xCCAA2211
c++ casting
1
typically type pun via memcpy
– Shafik Yaghmour
Nov 13 '18 at 21:12
Is there a reason to not douint32_t ival = myMsg[n].zero | (myMsg[n].one << 8) | (myMsg[n].two << 16) | (myMsg[n].three << 24)
?
– ChrisD
Nov 13 '18 at 21:23
@ChrisD thanks a lot, actually this is the answer, well I am new to c/c++ and did not know even what to search for
– Amir-Mousavi
Nov 13 '18 at 21:29
Related: stackoverflow.com/q/40332770/509868
– anatolyg
Nov 13 '18 at 21:40
Cool, I asked the question in case there was some crucial performance reason to not use all the shifts and ors. If there was then @ShafikYaghmour's answer is worth reading, but note you'd need to make sure thestruct msg
was packed into 4 bytes without any padding (although it almost always would be).
– ChrisD
Nov 13 '18 at 21:46
|
show 3 more comments
In my c++ program I have a structure containing four unsigned char
members
struct msg
{
unsigned char zero; unsigned char one; unsigned char two; unsigned char three;
}
and created an array of struct
msg myMsg[4] = {{0x11,0x22,0xAA,0xCC},{...},{...},{...}};
now in a function I want to return an index of this array as an unsigned int
unsigned int procMsg(int n){
return myMsg[n]; // of course this is worng
}
a value like: 0xCCAA2211
c++ casting
In my c++ program I have a structure containing four unsigned char
members
struct msg
{
unsigned char zero; unsigned char one; unsigned char two; unsigned char three;
}
and created an array of struct
msg myMsg[4] = {{0x11,0x22,0xAA,0xCC},{...},{...},{...}};
now in a function I want to return an index of this array as an unsigned int
unsigned int procMsg(int n){
return myMsg[n]; // of course this is worng
}
a value like: 0xCCAA2211
c++ casting
c++ casting
edited Nov 13 '18 at 22:07


ΦXocę 웃 Пepeúpa ツ
33.1k113862
33.1k113862
asked Nov 13 '18 at 21:09
Amir-MousaviAmir-Mousavi
7611031
7611031
1
typically type pun via memcpy
– Shafik Yaghmour
Nov 13 '18 at 21:12
Is there a reason to not douint32_t ival = myMsg[n].zero | (myMsg[n].one << 8) | (myMsg[n].two << 16) | (myMsg[n].three << 24)
?
– ChrisD
Nov 13 '18 at 21:23
@ChrisD thanks a lot, actually this is the answer, well I am new to c/c++ and did not know even what to search for
– Amir-Mousavi
Nov 13 '18 at 21:29
Related: stackoverflow.com/q/40332770/509868
– anatolyg
Nov 13 '18 at 21:40
Cool, I asked the question in case there was some crucial performance reason to not use all the shifts and ors. If there was then @ShafikYaghmour's answer is worth reading, but note you'd need to make sure thestruct msg
was packed into 4 bytes without any padding (although it almost always would be).
– ChrisD
Nov 13 '18 at 21:46
|
show 3 more comments
1
typically type pun via memcpy
– Shafik Yaghmour
Nov 13 '18 at 21:12
Is there a reason to not douint32_t ival = myMsg[n].zero | (myMsg[n].one << 8) | (myMsg[n].two << 16) | (myMsg[n].three << 24)
?
– ChrisD
Nov 13 '18 at 21:23
@ChrisD thanks a lot, actually this is the answer, well I am new to c/c++ and did not know even what to search for
– Amir-Mousavi
Nov 13 '18 at 21:29
Related: stackoverflow.com/q/40332770/509868
– anatolyg
Nov 13 '18 at 21:40
Cool, I asked the question in case there was some crucial performance reason to not use all the shifts and ors. If there was then @ShafikYaghmour's answer is worth reading, but note you'd need to make sure thestruct msg
was packed into 4 bytes without any padding (although it almost always would be).
– ChrisD
Nov 13 '18 at 21:46
1
1
typically type pun via memcpy
– Shafik Yaghmour
Nov 13 '18 at 21:12
typically type pun via memcpy
– Shafik Yaghmour
Nov 13 '18 at 21:12
Is there a reason to not do
uint32_t ival = myMsg[n].zero | (myMsg[n].one << 8) | (myMsg[n].two << 16) | (myMsg[n].three << 24)
?– ChrisD
Nov 13 '18 at 21:23
Is there a reason to not do
uint32_t ival = myMsg[n].zero | (myMsg[n].one << 8) | (myMsg[n].two << 16) | (myMsg[n].three << 24)
?– ChrisD
Nov 13 '18 at 21:23
@ChrisD thanks a lot, actually this is the answer, well I am new to c/c++ and did not know even what to search for
– Amir-Mousavi
Nov 13 '18 at 21:29
@ChrisD thanks a lot, actually this is the answer, well I am new to c/c++ and did not know even what to search for
– Amir-Mousavi
Nov 13 '18 at 21:29
Related: stackoverflow.com/q/40332770/509868
– anatolyg
Nov 13 '18 at 21:40
Related: stackoverflow.com/q/40332770/509868
– anatolyg
Nov 13 '18 at 21:40
Cool, I asked the question in case there was some crucial performance reason to not use all the shifts and ors. If there was then @ShafikYaghmour's answer is worth reading, but note you'd need to make sure the
struct msg
was packed into 4 bytes without any padding (although it almost always would be).– ChrisD
Nov 13 '18 at 21:46
Cool, I asked the question in case there was some crucial performance reason to not use all the shifts and ors. If there was then @ShafikYaghmour's answer is worth reading, but note you'd need to make sure the
struct msg
was packed into 4 bytes without any padding (although it almost always would be).– ChrisD
Nov 13 '18 at 21:46
|
show 3 more comments
2 Answers
2
active
oldest
votes
You can use shifting:
uint32_t little_endian;
uint32_t big_endian;
//...
big_endian = myMsg[0].zero << 24
| myMsg[0].one << 16
| myMsg[0].two << 8
| myMsg[0].three;
little_endian = myMsg[0].three << 24
myMsg[0].two << 16
myMsg[0].one << 8
myMsg[0].zero;
As you can see, with multi-byte integers, there are two general orderings: Big Endian and Little Endian. Big Endian has the Most Significant Byte (MSB) first. The Little Endian has Least Significant Byte (LSB) first.
In general the above method is more efficient than using a loop.
add a comment |
consider this:
a message like this: {0x01, 0x01, 0x01, 0x01}
can be parsed as
00000001 00000001 00000001 00000001(bin) = 16.843.009(dec)
so you just need to take every char and shift them according to its position
i.e.
char0 not shifted at all,
char1 shifted 8 positions to the left (or multiply by 2^8)
char2 shifted 16 positions to the left (or multiply by 2^(8*2))
char3 shifted 24 positions to the left (or multiply by 2^(8*3))
unsigned int procMsg(Message &x)
{
return (x.three << 24) |
(x.two << 16) |
(x.one << 8) |
x.zero;
}
int main(int argc, char *argv)
{
//0 1 2 3
Message myMsg[4] = {{0x01, 0x01, 0x01, 0x01}, //00000001 00000001 00000001 00000001 = 16.843.009
{0xFF, 0x00, 0xAA, 0xCC}, //00000001 00000001 00000001 00000001 = 3.433.693.439
{0x01, 0x00, 0x00, 0x00}, //00000001 00000001 00000001 00000001 = 1
{0x00, 0x00, 0x00, 0x00} //00000000 00000000 00000000 00000000 = 0
};
for (int i = 0; i<4; i++)
{
Message x = myMsg[i];
std::cout << "res: " << procMsg(myMsg[i]) << std::endl;
}
return 0;
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53289526%2fc-convert-four-unsined-chars-to-one-unsigned-int%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use shifting:
uint32_t little_endian;
uint32_t big_endian;
//...
big_endian = myMsg[0].zero << 24
| myMsg[0].one << 16
| myMsg[0].two << 8
| myMsg[0].three;
little_endian = myMsg[0].three << 24
myMsg[0].two << 16
myMsg[0].one << 8
myMsg[0].zero;
As you can see, with multi-byte integers, there are two general orderings: Big Endian and Little Endian. Big Endian has the Most Significant Byte (MSB) first. The Little Endian has Least Significant Byte (LSB) first.
In general the above method is more efficient than using a loop.
add a comment |
You can use shifting:
uint32_t little_endian;
uint32_t big_endian;
//...
big_endian = myMsg[0].zero << 24
| myMsg[0].one << 16
| myMsg[0].two << 8
| myMsg[0].three;
little_endian = myMsg[0].three << 24
myMsg[0].two << 16
myMsg[0].one << 8
myMsg[0].zero;
As you can see, with multi-byte integers, there are two general orderings: Big Endian and Little Endian. Big Endian has the Most Significant Byte (MSB) first. The Little Endian has Least Significant Byte (LSB) first.
In general the above method is more efficient than using a loop.
add a comment |
You can use shifting:
uint32_t little_endian;
uint32_t big_endian;
//...
big_endian = myMsg[0].zero << 24
| myMsg[0].one << 16
| myMsg[0].two << 8
| myMsg[0].three;
little_endian = myMsg[0].three << 24
myMsg[0].two << 16
myMsg[0].one << 8
myMsg[0].zero;
As you can see, with multi-byte integers, there are two general orderings: Big Endian and Little Endian. Big Endian has the Most Significant Byte (MSB) first. The Little Endian has Least Significant Byte (LSB) first.
In general the above method is more efficient than using a loop.
You can use shifting:
uint32_t little_endian;
uint32_t big_endian;
//...
big_endian = myMsg[0].zero << 24
| myMsg[0].one << 16
| myMsg[0].two << 8
| myMsg[0].three;
little_endian = myMsg[0].three << 24
myMsg[0].two << 16
myMsg[0].one << 8
myMsg[0].zero;
As you can see, with multi-byte integers, there are two general orderings: Big Endian and Little Endian. Big Endian has the Most Significant Byte (MSB) first. The Little Endian has Least Significant Byte (LSB) first.
In general the above method is more efficient than using a loop.
answered Nov 13 '18 at 22:23
Thomas MatthewsThomas Matthews
44.3k1173123
44.3k1173123
add a comment |
add a comment |
consider this:
a message like this: {0x01, 0x01, 0x01, 0x01}
can be parsed as
00000001 00000001 00000001 00000001(bin) = 16.843.009(dec)
so you just need to take every char and shift them according to its position
i.e.
char0 not shifted at all,
char1 shifted 8 positions to the left (or multiply by 2^8)
char2 shifted 16 positions to the left (or multiply by 2^(8*2))
char3 shifted 24 positions to the left (or multiply by 2^(8*3))
unsigned int procMsg(Message &x)
{
return (x.three << 24) |
(x.two << 16) |
(x.one << 8) |
x.zero;
}
int main(int argc, char *argv)
{
//0 1 2 3
Message myMsg[4] = {{0x01, 0x01, 0x01, 0x01}, //00000001 00000001 00000001 00000001 = 16.843.009
{0xFF, 0x00, 0xAA, 0xCC}, //00000001 00000001 00000001 00000001 = 3.433.693.439
{0x01, 0x00, 0x00, 0x00}, //00000001 00000001 00000001 00000001 = 1
{0x00, 0x00, 0x00, 0x00} //00000000 00000000 00000000 00000000 = 0
};
for (int i = 0; i<4; i++)
{
Message x = myMsg[i];
std::cout << "res: " << procMsg(myMsg[i]) << std::endl;
}
return 0;
}
add a comment |
consider this:
a message like this: {0x01, 0x01, 0x01, 0x01}
can be parsed as
00000001 00000001 00000001 00000001(bin) = 16.843.009(dec)
so you just need to take every char and shift them according to its position
i.e.
char0 not shifted at all,
char1 shifted 8 positions to the left (or multiply by 2^8)
char2 shifted 16 positions to the left (or multiply by 2^(8*2))
char3 shifted 24 positions to the left (or multiply by 2^(8*3))
unsigned int procMsg(Message &x)
{
return (x.three << 24) |
(x.two << 16) |
(x.one << 8) |
x.zero;
}
int main(int argc, char *argv)
{
//0 1 2 3
Message myMsg[4] = {{0x01, 0x01, 0x01, 0x01}, //00000001 00000001 00000001 00000001 = 16.843.009
{0xFF, 0x00, 0xAA, 0xCC}, //00000001 00000001 00000001 00000001 = 3.433.693.439
{0x01, 0x00, 0x00, 0x00}, //00000001 00000001 00000001 00000001 = 1
{0x00, 0x00, 0x00, 0x00} //00000000 00000000 00000000 00000000 = 0
};
for (int i = 0; i<4; i++)
{
Message x = myMsg[i];
std::cout << "res: " << procMsg(myMsg[i]) << std::endl;
}
return 0;
}
add a comment |
consider this:
a message like this: {0x01, 0x01, 0x01, 0x01}
can be parsed as
00000001 00000001 00000001 00000001(bin) = 16.843.009(dec)
so you just need to take every char and shift them according to its position
i.e.
char0 not shifted at all,
char1 shifted 8 positions to the left (or multiply by 2^8)
char2 shifted 16 positions to the left (or multiply by 2^(8*2))
char3 shifted 24 positions to the left (or multiply by 2^(8*3))
unsigned int procMsg(Message &x)
{
return (x.three << 24) |
(x.two << 16) |
(x.one << 8) |
x.zero;
}
int main(int argc, char *argv)
{
//0 1 2 3
Message myMsg[4] = {{0x01, 0x01, 0x01, 0x01}, //00000001 00000001 00000001 00000001 = 16.843.009
{0xFF, 0x00, 0xAA, 0xCC}, //00000001 00000001 00000001 00000001 = 3.433.693.439
{0x01, 0x00, 0x00, 0x00}, //00000001 00000001 00000001 00000001 = 1
{0x00, 0x00, 0x00, 0x00} //00000000 00000000 00000000 00000000 = 0
};
for (int i = 0; i<4; i++)
{
Message x = myMsg[i];
std::cout << "res: " << procMsg(myMsg[i]) << std::endl;
}
return 0;
}
consider this:
a message like this: {0x01, 0x01, 0x01, 0x01}
can be parsed as
00000001 00000001 00000001 00000001(bin) = 16.843.009(dec)
so you just need to take every char and shift them according to its position
i.e.
char0 not shifted at all,
char1 shifted 8 positions to the left (or multiply by 2^8)
char2 shifted 16 positions to the left (or multiply by 2^(8*2))
char3 shifted 24 positions to the left (or multiply by 2^(8*3))
unsigned int procMsg(Message &x)
{
return (x.three << 24) |
(x.two << 16) |
(x.one << 8) |
x.zero;
}
int main(int argc, char *argv)
{
//0 1 2 3
Message myMsg[4] = {{0x01, 0x01, 0x01, 0x01}, //00000001 00000001 00000001 00000001 = 16.843.009
{0xFF, 0x00, 0xAA, 0xCC}, //00000001 00000001 00000001 00000001 = 3.433.693.439
{0x01, 0x00, 0x00, 0x00}, //00000001 00000001 00000001 00000001 = 1
{0x00, 0x00, 0x00, 0x00} //00000000 00000000 00000000 00000000 = 0
};
for (int i = 0; i<4; i++)
{
Message x = myMsg[i];
std::cout << "res: " << procMsg(myMsg[i]) << std::endl;
}
return 0;
}
edited Nov 13 '18 at 22:30
answered Nov 13 '18 at 22:01


ΦXocę 웃 Пepeúpa ツΦXocę 웃 Пepeúpa ツ
33.1k113862
33.1k113862
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53289526%2fc-convert-four-unsined-chars-to-one-unsigned-int%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DYuaUF9hhD,1H5N6obKH 98 SDKZ Jogk,EOp9MKDj2I3yKW,ivQAtqO0HBKZ1LmTlpZmVlU
1
typically type pun via memcpy
– Shafik Yaghmour
Nov 13 '18 at 21:12
Is there a reason to not do
uint32_t ival = myMsg[n].zero | (myMsg[n].one << 8) | (myMsg[n].two << 16) | (myMsg[n].three << 24)
?– ChrisD
Nov 13 '18 at 21:23
@ChrisD thanks a lot, actually this is the answer, well I am new to c/c++ and did not know even what to search for
– Amir-Mousavi
Nov 13 '18 at 21:29
Related: stackoverflow.com/q/40332770/509868
– anatolyg
Nov 13 '18 at 21:40
Cool, I asked the question in case there was some crucial performance reason to not use all the shifts and ors. If there was then @ShafikYaghmour's answer is worth reading, but note you'd need to make sure the
struct msg
was packed into 4 bytes without any padding (although it almost always would be).– ChrisD
Nov 13 '18 at 21:46