Trying to find prime numbers
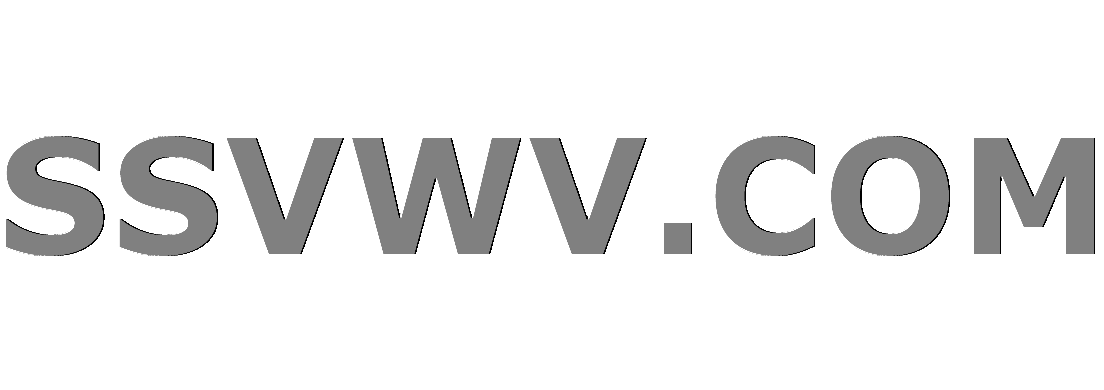
Multi tool use
I'm a first year student in a programming university and my first assignment is to find the sum of prime numbers between 3990000000 and 4010000000. The problem is everything I do, when I run the program it says the sum is 0 with a return value of 25. I've been trying to debug this code but with no luck, could someone help me?
My code is:
#include <stdio.h>
#define STARTNUMBER 3990000000
#define ENDNUMBER 4010000000
int main() {
unsigned int num;
int j, c, flag, sum = 0;
flag = 1;
c = 5;
j = 7;
for (num = STARTNUMBER; num <= ENDNUMBER; num++) {
if (num % 2 == 0) { /*if number mod 2 equals zero go to next number*/
flag = 0;
break;
}
if (num % 3 == 0) { /*if number mod 3 equals zero go to next number*/
flag = 0;
break;
} else
/*check if number is prime with the sequences 5+6+6...<=sqrt(number) and 7+6+6..<=sqrt(number)*/
while (c * c <= num && j * j <= num && flag == 1) {
if (num % c == 0 || num % j == 0) {
flag = 0;
break;
}
c += 6;
j += 6;
}
if (flag == 1)
sum++;
}
printf("There are %d prime numbers", sum);
}
c
|
show 1 more comment
I'm a first year student in a programming university and my first assignment is to find the sum of prime numbers between 3990000000 and 4010000000. The problem is everything I do, when I run the program it says the sum is 0 with a return value of 25. I've been trying to debug this code but with no luck, could someone help me?
My code is:
#include <stdio.h>
#define STARTNUMBER 3990000000
#define ENDNUMBER 4010000000
int main() {
unsigned int num;
int j, c, flag, sum = 0;
flag = 1;
c = 5;
j = 7;
for (num = STARTNUMBER; num <= ENDNUMBER; num++) {
if (num % 2 == 0) { /*if number mod 2 equals zero go to next number*/
flag = 0;
break;
}
if (num % 3 == 0) { /*if number mod 3 equals zero go to next number*/
flag = 0;
break;
} else
/*check if number is prime with the sequences 5+6+6...<=sqrt(number) and 7+6+6..<=sqrt(number)*/
while (c * c <= num && j * j <= num && flag == 1) {
if (num % c == 0 || num % j == 0) {
flag = 0;
break;
}
c += 6;
j += 6;
}
if (flag == 1)
sum++;
}
printf("There are %d prime numbers", sum);
}
c
8
Youbreak
out of the loop in your first iteration.
– tkausl
Nov 12 at 14:22
1
Are you looking for the sum of the prime numbers (as you've stated), or just for the number of prime numbers (as you've done in the code)?
– Giovanni Cerretani
Nov 12 at 14:32
Like @tkausl already said, you need to revisit the topic of for loops and how thebreak
instruction works exactly
– aPhilRa
Nov 12 at 14:35
1
As for the "return value of 25", it is the number of characters printed byprintf
(which is missing a newline at the end, BTW), since yourmain
is missing areturn
statement of its own.
– Arkku
Nov 12 at 14:38
Once you have fixed yourbreak
ing logic, you will see that you must reset the values offlag
,c
andj
for each newnum
. At the moment, you just set these values once at the beginning.
– M Oehm
Nov 12 at 14:41
|
show 1 more comment
I'm a first year student in a programming university and my first assignment is to find the sum of prime numbers between 3990000000 and 4010000000. The problem is everything I do, when I run the program it says the sum is 0 with a return value of 25. I've been trying to debug this code but with no luck, could someone help me?
My code is:
#include <stdio.h>
#define STARTNUMBER 3990000000
#define ENDNUMBER 4010000000
int main() {
unsigned int num;
int j, c, flag, sum = 0;
flag = 1;
c = 5;
j = 7;
for (num = STARTNUMBER; num <= ENDNUMBER; num++) {
if (num % 2 == 0) { /*if number mod 2 equals zero go to next number*/
flag = 0;
break;
}
if (num % 3 == 0) { /*if number mod 3 equals zero go to next number*/
flag = 0;
break;
} else
/*check if number is prime with the sequences 5+6+6...<=sqrt(number) and 7+6+6..<=sqrt(number)*/
while (c * c <= num && j * j <= num && flag == 1) {
if (num % c == 0 || num % j == 0) {
flag = 0;
break;
}
c += 6;
j += 6;
}
if (flag == 1)
sum++;
}
printf("There are %d prime numbers", sum);
}
c
I'm a first year student in a programming university and my first assignment is to find the sum of prime numbers between 3990000000 and 4010000000. The problem is everything I do, when I run the program it says the sum is 0 with a return value of 25. I've been trying to debug this code but with no luck, could someone help me?
My code is:
#include <stdio.h>
#define STARTNUMBER 3990000000
#define ENDNUMBER 4010000000
int main() {
unsigned int num;
int j, c, flag, sum = 0;
flag = 1;
c = 5;
j = 7;
for (num = STARTNUMBER; num <= ENDNUMBER; num++) {
if (num % 2 == 0) { /*if number mod 2 equals zero go to next number*/
flag = 0;
break;
}
if (num % 3 == 0) { /*if number mod 3 equals zero go to next number*/
flag = 0;
break;
} else
/*check if number is prime with the sequences 5+6+6...<=sqrt(number) and 7+6+6..<=sqrt(number)*/
while (c * c <= num && j * j <= num && flag == 1) {
if (num % c == 0 || num % j == 0) {
flag = 0;
break;
}
c += 6;
j += 6;
}
if (flag == 1)
sum++;
}
printf("There are %d prime numbers", sum);
}
c
c
edited Nov 12 at 14:59


chux
80.2k870147
80.2k870147
asked Nov 12 at 14:21
stefnto
111
111
8
Youbreak
out of the loop in your first iteration.
– tkausl
Nov 12 at 14:22
1
Are you looking for the sum of the prime numbers (as you've stated), or just for the number of prime numbers (as you've done in the code)?
– Giovanni Cerretani
Nov 12 at 14:32
Like @tkausl already said, you need to revisit the topic of for loops and how thebreak
instruction works exactly
– aPhilRa
Nov 12 at 14:35
1
As for the "return value of 25", it is the number of characters printed byprintf
(which is missing a newline at the end, BTW), since yourmain
is missing areturn
statement of its own.
– Arkku
Nov 12 at 14:38
Once you have fixed yourbreak
ing logic, you will see that you must reset the values offlag
,c
andj
for each newnum
. At the moment, you just set these values once at the beginning.
– M Oehm
Nov 12 at 14:41
|
show 1 more comment
8
Youbreak
out of the loop in your first iteration.
– tkausl
Nov 12 at 14:22
1
Are you looking for the sum of the prime numbers (as you've stated), or just for the number of prime numbers (as you've done in the code)?
– Giovanni Cerretani
Nov 12 at 14:32
Like @tkausl already said, you need to revisit the topic of for loops and how thebreak
instruction works exactly
– aPhilRa
Nov 12 at 14:35
1
As for the "return value of 25", it is the number of characters printed byprintf
(which is missing a newline at the end, BTW), since yourmain
is missing areturn
statement of its own.
– Arkku
Nov 12 at 14:38
Once you have fixed yourbreak
ing logic, you will see that you must reset the values offlag
,c
andj
for each newnum
. At the moment, you just set these values once at the beginning.
– M Oehm
Nov 12 at 14:41
8
8
You
break
out of the loop in your first iteration.– tkausl
Nov 12 at 14:22
You
break
out of the loop in your first iteration.– tkausl
Nov 12 at 14:22
1
1
Are you looking for the sum of the prime numbers (as you've stated), or just for the number of prime numbers (as you've done in the code)?
– Giovanni Cerretani
Nov 12 at 14:32
Are you looking for the sum of the prime numbers (as you've stated), or just for the number of prime numbers (as you've done in the code)?
– Giovanni Cerretani
Nov 12 at 14:32
Like @tkausl already said, you need to revisit the topic of for loops and how the
break
instruction works exactly– aPhilRa
Nov 12 at 14:35
Like @tkausl already said, you need to revisit the topic of for loops and how the
break
instruction works exactly– aPhilRa
Nov 12 at 14:35
1
1
As for the "return value of 25", it is the number of characters printed by
printf
(which is missing a newline at the end, BTW), since your main
is missing a return
statement of its own.– Arkku
Nov 12 at 14:38
As for the "return value of 25", it is the number of characters printed by
printf
(which is missing a newline at the end, BTW), since your main
is missing a return
statement of its own.– Arkku
Nov 12 at 14:38
Once you have fixed your
break
ing logic, you will see that you must reset the values of flag
, c
and j
for each new num
. At the moment, you just set these values once at the beginning.– M Oehm
Nov 12 at 14:41
Once you have fixed your
break
ing logic, you will see that you must reset the values of flag
, c
and j
for each new num
. At the moment, you just set these values once at the beginning.– M Oehm
Nov 12 at 14:41
|
show 1 more comment
1 Answer
1
active
oldest
votes
You are asking for the sum of the prime number, even if your code is just printing how many they are. Assuming you've misunderstood the exercise, I try to show a possible problem of your original question, glimpsing a possible trick in your exercise since the interval is very close to 232.
Assuming also you are on a 64-bit environment, if there are at least two prime numbers in that inteval, the sum is going to be greater than INT_MAX
(231 - 1). An int
is not sufficient to store a value, and also unsigned int
is not sufficient since UINT_MAX
is 232 - 1.
Finally, assuming you've solved your problems with the break
statements already described in the comments, try to store your sum
variable into a unsigned long int
, and replace the last part of the loop with
if (flag==1)
sum += num;
Yeah my mistake, by saying the sum of numbers, I meant how many numbers are between the startnumber and endnumber, thus storing the sum variable into unsigned int is enough. As for the use of the break command, doesn't the loop stop and continues to the next number if the command where break is in (in this case those if commands) is false? (flag=0). I've tried removing the break commands and leaving it with the flag command, but it didn't help.
– stefnto
Nov 12 at 15:46
2
You should call that a "count" not a "sum". Variable names are important--you and other humans may have to read the code years later, and might reasonably (and mistakenly) assume that "sum" is a sum.
– Lee Daniel Crocker
Nov 12 at 19:21
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53264136%2ftrying-to-find-prime-numbers%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You are asking for the sum of the prime number, even if your code is just printing how many they are. Assuming you've misunderstood the exercise, I try to show a possible problem of your original question, glimpsing a possible trick in your exercise since the interval is very close to 232.
Assuming also you are on a 64-bit environment, if there are at least two prime numbers in that inteval, the sum is going to be greater than INT_MAX
(231 - 1). An int
is not sufficient to store a value, and also unsigned int
is not sufficient since UINT_MAX
is 232 - 1.
Finally, assuming you've solved your problems with the break
statements already described in the comments, try to store your sum
variable into a unsigned long int
, and replace the last part of the loop with
if (flag==1)
sum += num;
Yeah my mistake, by saying the sum of numbers, I meant how many numbers are between the startnumber and endnumber, thus storing the sum variable into unsigned int is enough. As for the use of the break command, doesn't the loop stop and continues to the next number if the command where break is in (in this case those if commands) is false? (flag=0). I've tried removing the break commands and leaving it with the flag command, but it didn't help.
– stefnto
Nov 12 at 15:46
2
You should call that a "count" not a "sum". Variable names are important--you and other humans may have to read the code years later, and might reasonably (and mistakenly) assume that "sum" is a sum.
– Lee Daniel Crocker
Nov 12 at 19:21
add a comment |
You are asking for the sum of the prime number, even if your code is just printing how many they are. Assuming you've misunderstood the exercise, I try to show a possible problem of your original question, glimpsing a possible trick in your exercise since the interval is very close to 232.
Assuming also you are on a 64-bit environment, if there are at least two prime numbers in that inteval, the sum is going to be greater than INT_MAX
(231 - 1). An int
is not sufficient to store a value, and also unsigned int
is not sufficient since UINT_MAX
is 232 - 1.
Finally, assuming you've solved your problems with the break
statements already described in the comments, try to store your sum
variable into a unsigned long int
, and replace the last part of the loop with
if (flag==1)
sum += num;
Yeah my mistake, by saying the sum of numbers, I meant how many numbers are between the startnumber and endnumber, thus storing the sum variable into unsigned int is enough. As for the use of the break command, doesn't the loop stop and continues to the next number if the command where break is in (in this case those if commands) is false? (flag=0). I've tried removing the break commands and leaving it with the flag command, but it didn't help.
– stefnto
Nov 12 at 15:46
2
You should call that a "count" not a "sum". Variable names are important--you and other humans may have to read the code years later, and might reasonably (and mistakenly) assume that "sum" is a sum.
– Lee Daniel Crocker
Nov 12 at 19:21
add a comment |
You are asking for the sum of the prime number, even if your code is just printing how many they are. Assuming you've misunderstood the exercise, I try to show a possible problem of your original question, glimpsing a possible trick in your exercise since the interval is very close to 232.
Assuming also you are on a 64-bit environment, if there are at least two prime numbers in that inteval, the sum is going to be greater than INT_MAX
(231 - 1). An int
is not sufficient to store a value, and also unsigned int
is not sufficient since UINT_MAX
is 232 - 1.
Finally, assuming you've solved your problems with the break
statements already described in the comments, try to store your sum
variable into a unsigned long int
, and replace the last part of the loop with
if (flag==1)
sum += num;
You are asking for the sum of the prime number, even if your code is just printing how many they are. Assuming you've misunderstood the exercise, I try to show a possible problem of your original question, glimpsing a possible trick in your exercise since the interval is very close to 232.
Assuming also you are on a 64-bit environment, if there are at least two prime numbers in that inteval, the sum is going to be greater than INT_MAX
(231 - 1). An int
is not sufficient to store a value, and also unsigned int
is not sufficient since UINT_MAX
is 232 - 1.
Finally, assuming you've solved your problems with the break
statements already described in the comments, try to store your sum
variable into a unsigned long int
, and replace the last part of the loop with
if (flag==1)
sum += num;
edited Nov 12 at 15:01
answered Nov 12 at 14:28
Giovanni Cerretani
581316
581316
Yeah my mistake, by saying the sum of numbers, I meant how many numbers are between the startnumber and endnumber, thus storing the sum variable into unsigned int is enough. As for the use of the break command, doesn't the loop stop and continues to the next number if the command where break is in (in this case those if commands) is false? (flag=0). I've tried removing the break commands and leaving it with the flag command, but it didn't help.
– stefnto
Nov 12 at 15:46
2
You should call that a "count" not a "sum". Variable names are important--you and other humans may have to read the code years later, and might reasonably (and mistakenly) assume that "sum" is a sum.
– Lee Daniel Crocker
Nov 12 at 19:21
add a comment |
Yeah my mistake, by saying the sum of numbers, I meant how many numbers are between the startnumber and endnumber, thus storing the sum variable into unsigned int is enough. As for the use of the break command, doesn't the loop stop and continues to the next number if the command where break is in (in this case those if commands) is false? (flag=0). I've tried removing the break commands and leaving it with the flag command, but it didn't help.
– stefnto
Nov 12 at 15:46
2
You should call that a "count" not a "sum". Variable names are important--you and other humans may have to read the code years later, and might reasonably (and mistakenly) assume that "sum" is a sum.
– Lee Daniel Crocker
Nov 12 at 19:21
Yeah my mistake, by saying the sum of numbers, I meant how many numbers are between the startnumber and endnumber, thus storing the sum variable into unsigned int is enough. As for the use of the break command, doesn't the loop stop and continues to the next number if the command where break is in (in this case those if commands) is false? (flag=0). I've tried removing the break commands and leaving it with the flag command, but it didn't help.
– stefnto
Nov 12 at 15:46
Yeah my mistake, by saying the sum of numbers, I meant how many numbers are between the startnumber and endnumber, thus storing the sum variable into unsigned int is enough. As for the use of the break command, doesn't the loop stop and continues to the next number if the command where break is in (in this case those if commands) is false? (flag=0). I've tried removing the break commands and leaving it with the flag command, but it didn't help.
– stefnto
Nov 12 at 15:46
2
2
You should call that a "count" not a "sum". Variable names are important--you and other humans may have to read the code years later, and might reasonably (and mistakenly) assume that "sum" is a sum.
– Lee Daniel Crocker
Nov 12 at 19:21
You should call that a "count" not a "sum". Variable names are important--you and other humans may have to read the code years later, and might reasonably (and mistakenly) assume that "sum" is a sum.
– Lee Daniel Crocker
Nov 12 at 19:21
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53264136%2ftrying-to-find-prime-numbers%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Nm z3pvRxbOTssN5IEfvPttvLYBteNh,LO2qyFNvJ,YNGy2R c6OLW0wsCJkLmIW,jI0r
8
You
break
out of the loop in your first iteration.– tkausl
Nov 12 at 14:22
1
Are you looking for the sum of the prime numbers (as you've stated), or just for the number of prime numbers (as you've done in the code)?
– Giovanni Cerretani
Nov 12 at 14:32
Like @tkausl already said, you need to revisit the topic of for loops and how the
break
instruction works exactly– aPhilRa
Nov 12 at 14:35
1
As for the "return value of 25", it is the number of characters printed by
printf
(which is missing a newline at the end, BTW), since yourmain
is missing areturn
statement of its own.– Arkku
Nov 12 at 14:38
Once you have fixed your
break
ing logic, you will see that you must reset the values offlag
,c
andj
for each newnum
. At the moment, you just set these values once at the beginning.– M Oehm
Nov 12 at 14:41