Get Integer value from NSNumber in NSArray
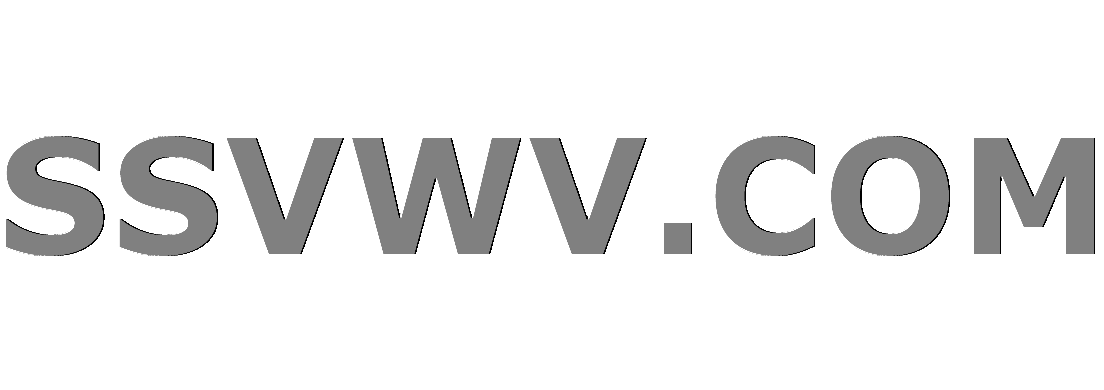
Multi tool use
I have an NSArray with NSNumber objects that have int values:
arrayOfValues = [[[NSArray alloc] initWithObjects: [NSNumber numberWithInt:1], [NSNumber numberWithInt:3], [NSNumber numberWithInt:5], [NSNumber numberWithInt:6], [NSNumber numberWithInt:7], nil] autorelease];
[arrayOfValues retain];
I'm trying to iterate through the array like this:
int currentValue;
for (int i = 0; i < [arrayOfValues count]; i++)
{
currentValue = [(NSNumber *)[arrayOfValues objectAtIndex:i] intValue];
NSLog(@"currentValue: %@", currentValue); // EXE_BAD_ACCESS
}
What am I doing wrong here?
objective-c ios iterator nsarray nsnumber
add a comment |
I have an NSArray with NSNumber objects that have int values:
arrayOfValues = [[[NSArray alloc] initWithObjects: [NSNumber numberWithInt:1], [NSNumber numberWithInt:3], [NSNumber numberWithInt:5], [NSNumber numberWithInt:6], [NSNumber numberWithInt:7], nil] autorelease];
[arrayOfValues retain];
I'm trying to iterate through the array like this:
int currentValue;
for (int i = 0; i < [arrayOfValues count]; i++)
{
currentValue = [(NSNumber *)[arrayOfValues objectAtIndex:i] intValue];
NSLog(@"currentValue: %@", currentValue); // EXE_BAD_ACCESS
}
What am I doing wrong here?
objective-c ios iterator nsarray nsnumber
add a comment |
I have an NSArray with NSNumber objects that have int values:
arrayOfValues = [[[NSArray alloc] initWithObjects: [NSNumber numberWithInt:1], [NSNumber numberWithInt:3], [NSNumber numberWithInt:5], [NSNumber numberWithInt:6], [NSNumber numberWithInt:7], nil] autorelease];
[arrayOfValues retain];
I'm trying to iterate through the array like this:
int currentValue;
for (int i = 0; i < [arrayOfValues count]; i++)
{
currentValue = [(NSNumber *)[arrayOfValues objectAtIndex:i] intValue];
NSLog(@"currentValue: %@", currentValue); // EXE_BAD_ACCESS
}
What am I doing wrong here?
objective-c ios iterator nsarray nsnumber
I have an NSArray with NSNumber objects that have int values:
arrayOfValues = [[[NSArray alloc] initWithObjects: [NSNumber numberWithInt:1], [NSNumber numberWithInt:3], [NSNumber numberWithInt:5], [NSNumber numberWithInt:6], [NSNumber numberWithInt:7], nil] autorelease];
[arrayOfValues retain];
I'm trying to iterate through the array like this:
int currentValue;
for (int i = 0; i < [arrayOfValues count]; i++)
{
currentValue = [(NSNumber *)[arrayOfValues objectAtIndex:i] intValue];
NSLog(@"currentValue: %@", currentValue); // EXE_BAD_ACCESS
}
What am I doing wrong here?
objective-c ios iterator nsarray nsnumber
objective-c ios iterator nsarray nsnumber
edited Nov 12 at 14:15
Jon Schneider
13.1k783118
13.1k783118
asked Aug 1 '12 at 3:48
Phill Pafford
47.5k76239364
47.5k76239364
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
You are using the wrong format specifier. %@ is for objects, but int is not an object. So, you should be doing this:
int currentValue;
for (int i = 0; i < [arrayOfValues count]; i++)
{
currentValue = [(NSNumber *)[arrayOfValues objectAtIndex:i] intValue];
NSLog(@"currentValue: %d", currentValue); // EXE_BAD_ACCESS
}
More information in the docs.
I thought %@ was a wild card so I could pass anything
– Phill Pafford
Aug 1 '12 at 3:56
4
You can pass any object, but like I said, int is not an object.
– sosborn
Aug 1 '12 at 3:57
thanks, this does help with clarification and the error. having a DOH! (slap hand on head) moment
– Phill Pafford
Aug 1 '12 at 3:58
add a comment |
This worked for me when creating an NSArray of enum values I wanted to call (which is useful to know that this is the proper solution for that) I'm using Xcode 6.4.
int currentValue = (int)[(NSNumber *)[arrayOfValues objectAtIndex:i] integerValue];
sosborn's answer will throw a warning since it is still necessary to cast the NSInteger to an int.
Typecasting in ObjC is the bane of my existence. I hope this helps someone!
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f11752317%2fget-integer-value-from-nsnumber-in-nsarray%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You are using the wrong format specifier. %@ is for objects, but int is not an object. So, you should be doing this:
int currentValue;
for (int i = 0; i < [arrayOfValues count]; i++)
{
currentValue = [(NSNumber *)[arrayOfValues objectAtIndex:i] intValue];
NSLog(@"currentValue: %d", currentValue); // EXE_BAD_ACCESS
}
More information in the docs.
I thought %@ was a wild card so I could pass anything
– Phill Pafford
Aug 1 '12 at 3:56
4
You can pass any object, but like I said, int is not an object.
– sosborn
Aug 1 '12 at 3:57
thanks, this does help with clarification and the error. having a DOH! (slap hand on head) moment
– Phill Pafford
Aug 1 '12 at 3:58
add a comment |
You are using the wrong format specifier. %@ is for objects, but int is not an object. So, you should be doing this:
int currentValue;
for (int i = 0; i < [arrayOfValues count]; i++)
{
currentValue = [(NSNumber *)[arrayOfValues objectAtIndex:i] intValue];
NSLog(@"currentValue: %d", currentValue); // EXE_BAD_ACCESS
}
More information in the docs.
I thought %@ was a wild card so I could pass anything
– Phill Pafford
Aug 1 '12 at 3:56
4
You can pass any object, but like I said, int is not an object.
– sosborn
Aug 1 '12 at 3:57
thanks, this does help with clarification and the error. having a DOH! (slap hand on head) moment
– Phill Pafford
Aug 1 '12 at 3:58
add a comment |
You are using the wrong format specifier. %@ is for objects, but int is not an object. So, you should be doing this:
int currentValue;
for (int i = 0; i < [arrayOfValues count]; i++)
{
currentValue = [(NSNumber *)[arrayOfValues objectAtIndex:i] intValue];
NSLog(@"currentValue: %d", currentValue); // EXE_BAD_ACCESS
}
More information in the docs.
You are using the wrong format specifier. %@ is for objects, but int is not an object. So, you should be doing this:
int currentValue;
for (int i = 0; i < [arrayOfValues count]; i++)
{
currentValue = [(NSNumber *)[arrayOfValues objectAtIndex:i] intValue];
NSLog(@"currentValue: %d", currentValue); // EXE_BAD_ACCESS
}
More information in the docs.
answered Aug 1 '12 at 3:54
sosborn
14.1k23543
14.1k23543
I thought %@ was a wild card so I could pass anything
– Phill Pafford
Aug 1 '12 at 3:56
4
You can pass any object, but like I said, int is not an object.
– sosborn
Aug 1 '12 at 3:57
thanks, this does help with clarification and the error. having a DOH! (slap hand on head) moment
– Phill Pafford
Aug 1 '12 at 3:58
add a comment |
I thought %@ was a wild card so I could pass anything
– Phill Pafford
Aug 1 '12 at 3:56
4
You can pass any object, but like I said, int is not an object.
– sosborn
Aug 1 '12 at 3:57
thanks, this does help with clarification and the error. having a DOH! (slap hand on head) moment
– Phill Pafford
Aug 1 '12 at 3:58
I thought %@ was a wild card so I could pass anything
– Phill Pafford
Aug 1 '12 at 3:56
I thought %@ was a wild card so I could pass anything
– Phill Pafford
Aug 1 '12 at 3:56
4
4
You can pass any object, but like I said, int is not an object.
– sosborn
Aug 1 '12 at 3:57
You can pass any object, but like I said, int is not an object.
– sosborn
Aug 1 '12 at 3:57
thanks, this does help with clarification and the error. having a DOH! (slap hand on head) moment
– Phill Pafford
Aug 1 '12 at 3:58
thanks, this does help with clarification and the error. having a DOH! (slap hand on head) moment
– Phill Pafford
Aug 1 '12 at 3:58
add a comment |
This worked for me when creating an NSArray of enum values I wanted to call (which is useful to know that this is the proper solution for that) I'm using Xcode 6.4.
int currentValue = (int)[(NSNumber *)[arrayOfValues objectAtIndex:i] integerValue];
sosborn's answer will throw a warning since it is still necessary to cast the NSInteger to an int.
Typecasting in ObjC is the bane of my existence. I hope this helps someone!
add a comment |
This worked for me when creating an NSArray of enum values I wanted to call (which is useful to know that this is the proper solution for that) I'm using Xcode 6.4.
int currentValue = (int)[(NSNumber *)[arrayOfValues objectAtIndex:i] integerValue];
sosborn's answer will throw a warning since it is still necessary to cast the NSInteger to an int.
Typecasting in ObjC is the bane of my existence. I hope this helps someone!
add a comment |
This worked for me when creating an NSArray of enum values I wanted to call (which is useful to know that this is the proper solution for that) I'm using Xcode 6.4.
int currentValue = (int)[(NSNumber *)[arrayOfValues objectAtIndex:i] integerValue];
sosborn's answer will throw a warning since it is still necessary to cast the NSInteger to an int.
Typecasting in ObjC is the bane of my existence. I hope this helps someone!
This worked for me when creating an NSArray of enum values I wanted to call (which is useful to know that this is the proper solution for that) I'm using Xcode 6.4.
int currentValue = (int)[(NSNumber *)[arrayOfValues objectAtIndex:i] integerValue];
sosborn's answer will throw a warning since it is still necessary to cast the NSInteger to an int.
Typecasting in ObjC is the bane of my existence. I hope this helps someone!
edited Nov 1 '15 at 21:41
answered Aug 7 '15 at 16:27


jungledev
2,1381937
2,1381937
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f11752317%2fget-integer-value-from-nsnumber-in-nsarray%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JPGG n UqKZ,JzYMUz,CWC