Is it possible to print a chart with vue-chartjs?
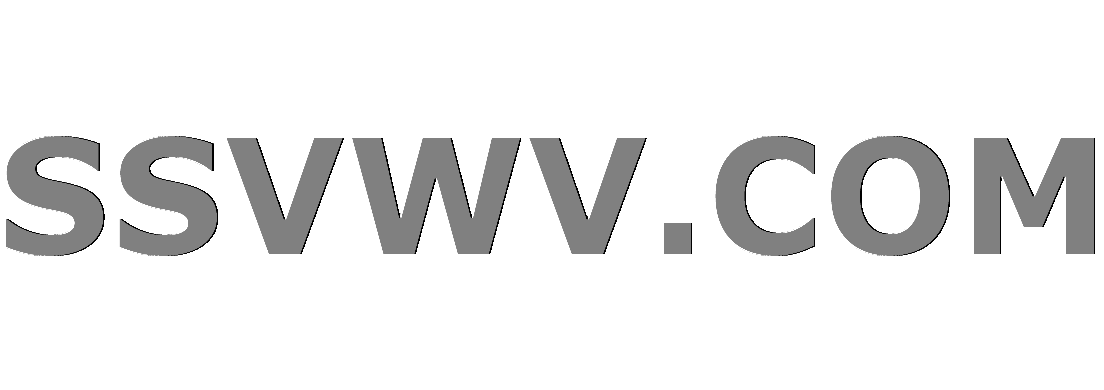
Multi tool use
I am using vue-chartjs to render graphs on a webapp. I know you can print charts if you are using the original library. However I have no idea on how to do it with the vue version of the library.
I have my charts variable on an external charts.js file
import {Bar, mixins } from 'vue-chartjs'
Chart.defaults.global
let chartOptions = Chart.defaults.global;
const { reactiveProp } = mixins
export default {
extends: Bar,
mixins: [reactiveProp],
props: ['options'],
mounted () {
let that = this;
that.chartOptions = {
scales: {
yAxes: [{
ticks: {
suggestedMin: 0,
fontFamily: "'Overpass_Mono', 'Monaco', monospace",
fontColor: "rgba(254, 255, 248, 0.5)"
},
gridLines: {
color: 'rgba(255, 80, 248, 0.08)',
zeroLineColor: 'rgb(168, 119, 181)',
zeroLineWidth: 2
},
}],
xAxes: [{
ticks: {
suggestedMin: 0,
fontColor: "rgb(168, 119, 181)"
},
gridLines: {
color: 'rgba(255, 80, 248, 0.08)',
zeroLineColor: 'transparent',
}
}],
},
legend: {
labels: {
fontColor: 'rgb(168, 119, 181)',
}
}
},
this.renderChart(this.chartData, that.chartOptions)
}
}
Then on my component template I have:
<template>
<div class="report">
<charts v-if="todaySelected"
:chart-id="'total_visits_chart_bar'"
:height="chartsHeight"
:options="chartOptions"
:chart-data="datacollection"
></charts>
<div v-if="todaySelected">
<button @click="printChart(charts)">Print chart</button>
</div>
</template>
<script>
import charts from './chart_0.js'
components: {
charts,
},
data() {
return{
datacollection: {"datasets":[{"label":"Entries Today","data":[15,15,15,0]},{"label":"Currently Inside","data":[2,2,2,0]}],"labels":}
}
}.
methods: {
printChart(charts) {
charts.print();
},
}
</script>
Any help would be appreciated.
vue.js printing chart.js vue-chartjs
add a comment |
I am using vue-chartjs to render graphs on a webapp. I know you can print charts if you are using the original library. However I have no idea on how to do it with the vue version of the library.
I have my charts variable on an external charts.js file
import {Bar, mixins } from 'vue-chartjs'
Chart.defaults.global
let chartOptions = Chart.defaults.global;
const { reactiveProp } = mixins
export default {
extends: Bar,
mixins: [reactiveProp],
props: ['options'],
mounted () {
let that = this;
that.chartOptions = {
scales: {
yAxes: [{
ticks: {
suggestedMin: 0,
fontFamily: "'Overpass_Mono', 'Monaco', monospace",
fontColor: "rgba(254, 255, 248, 0.5)"
},
gridLines: {
color: 'rgba(255, 80, 248, 0.08)',
zeroLineColor: 'rgb(168, 119, 181)',
zeroLineWidth: 2
},
}],
xAxes: [{
ticks: {
suggestedMin: 0,
fontColor: "rgb(168, 119, 181)"
},
gridLines: {
color: 'rgba(255, 80, 248, 0.08)',
zeroLineColor: 'transparent',
}
}],
},
legend: {
labels: {
fontColor: 'rgb(168, 119, 181)',
}
}
},
this.renderChart(this.chartData, that.chartOptions)
}
}
Then on my component template I have:
<template>
<div class="report">
<charts v-if="todaySelected"
:chart-id="'total_visits_chart_bar'"
:height="chartsHeight"
:options="chartOptions"
:chart-data="datacollection"
></charts>
<div v-if="todaySelected">
<button @click="printChart(charts)">Print chart</button>
</div>
</template>
<script>
import charts from './chart_0.js'
components: {
charts,
},
data() {
return{
datacollection: {"datasets":[{"label":"Entries Today","data":[15,15,15,0]},{"label":"Currently Inside","data":[2,2,2,0]}],"labels":}
}
}.
methods: {
printChart(charts) {
charts.print();
},
}
</script>
Any help would be appreciated.
vue.js printing chart.js vue-chartjs
add a comment |
I am using vue-chartjs to render graphs on a webapp. I know you can print charts if you are using the original library. However I have no idea on how to do it with the vue version of the library.
I have my charts variable on an external charts.js file
import {Bar, mixins } from 'vue-chartjs'
Chart.defaults.global
let chartOptions = Chart.defaults.global;
const { reactiveProp } = mixins
export default {
extends: Bar,
mixins: [reactiveProp],
props: ['options'],
mounted () {
let that = this;
that.chartOptions = {
scales: {
yAxes: [{
ticks: {
suggestedMin: 0,
fontFamily: "'Overpass_Mono', 'Monaco', monospace",
fontColor: "rgba(254, 255, 248, 0.5)"
},
gridLines: {
color: 'rgba(255, 80, 248, 0.08)',
zeroLineColor: 'rgb(168, 119, 181)',
zeroLineWidth: 2
},
}],
xAxes: [{
ticks: {
suggestedMin: 0,
fontColor: "rgb(168, 119, 181)"
},
gridLines: {
color: 'rgba(255, 80, 248, 0.08)',
zeroLineColor: 'transparent',
}
}],
},
legend: {
labels: {
fontColor: 'rgb(168, 119, 181)',
}
}
},
this.renderChart(this.chartData, that.chartOptions)
}
}
Then on my component template I have:
<template>
<div class="report">
<charts v-if="todaySelected"
:chart-id="'total_visits_chart_bar'"
:height="chartsHeight"
:options="chartOptions"
:chart-data="datacollection"
></charts>
<div v-if="todaySelected">
<button @click="printChart(charts)">Print chart</button>
</div>
</template>
<script>
import charts from './chart_0.js'
components: {
charts,
},
data() {
return{
datacollection: {"datasets":[{"label":"Entries Today","data":[15,15,15,0]},{"label":"Currently Inside","data":[2,2,2,0]}],"labels":}
}
}.
methods: {
printChart(charts) {
charts.print();
},
}
</script>
Any help would be appreciated.
vue.js printing chart.js vue-chartjs
I am using vue-chartjs to render graphs on a webapp. I know you can print charts if you are using the original library. However I have no idea on how to do it with the vue version of the library.
I have my charts variable on an external charts.js file
import {Bar, mixins } from 'vue-chartjs'
Chart.defaults.global
let chartOptions = Chart.defaults.global;
const { reactiveProp } = mixins
export default {
extends: Bar,
mixins: [reactiveProp],
props: ['options'],
mounted () {
let that = this;
that.chartOptions = {
scales: {
yAxes: [{
ticks: {
suggestedMin: 0,
fontFamily: "'Overpass_Mono', 'Monaco', monospace",
fontColor: "rgba(254, 255, 248, 0.5)"
},
gridLines: {
color: 'rgba(255, 80, 248, 0.08)',
zeroLineColor: 'rgb(168, 119, 181)',
zeroLineWidth: 2
},
}],
xAxes: [{
ticks: {
suggestedMin: 0,
fontColor: "rgb(168, 119, 181)"
},
gridLines: {
color: 'rgba(255, 80, 248, 0.08)',
zeroLineColor: 'transparent',
}
}],
},
legend: {
labels: {
fontColor: 'rgb(168, 119, 181)',
}
}
},
this.renderChart(this.chartData, that.chartOptions)
}
}
Then on my component template I have:
<template>
<div class="report">
<charts v-if="todaySelected"
:chart-id="'total_visits_chart_bar'"
:height="chartsHeight"
:options="chartOptions"
:chart-data="datacollection"
></charts>
<div v-if="todaySelected">
<button @click="printChart(charts)">Print chart</button>
</div>
</template>
<script>
import charts from './chart_0.js'
components: {
charts,
},
data() {
return{
datacollection: {"datasets":[{"label":"Entries Today","data":[15,15,15,0]},{"label":"Currently Inside","data":[2,2,2,0]}],"labels":}
}
}.
methods: {
printChart(charts) {
charts.print();
},
}
</script>
Any help would be appreciated.
vue.js printing chart.js vue-chartjs
vue.js printing chart.js vue-chartjs
edited Nov 13 '18 at 13:28
asked Nov 12 '18 at 21:20


Joao Alves Marrucho
337213
337213
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
vue-chartjs is based on chart.js and not canvas.js, thus it does not have a "build-in" way of printing.
You have to do it with some custom logic and the native javascript printing functions.
You can however grab the canvas element inside your chart component and generate for example an image and then print that image.
It will get a bit tricky, because you only have access to the canvas inside your chart component. So you will need to maybe wait for an event or prop to trigger the toDataURL
call and then emit the image to your parent component where you can print it. If you want to trigger the print in your parent component.
methods: {
print () {
// grab the canvas and generate an image
let image = this.$refs.canvas.toDataURL('image/png')
// Emits an event with the image
this.$emit('chart:print', image)
}
}
In your parent component:
<template>
<your-chart @chart:print="handlePrint"
<template/>
....
...
methods: {
handlePrint(image) {
const win = window.open('', 'Print', 'height=600, width=800')
win.document.write(`<br><img src='${image}' />`)
win.print()
win.close()
}
}
add a comment |
It seems like the library is based on chartjs not canvasjs https://www.chartjs.org/docs/latest/ you might want to look into how to print a window Quick Print HTML5 Canvas, and remember you have access to the canvas element where your graph is drawn:
methods: {
printChart() {
const canvasEle = this.$el.querySelector('canvas');
//now your chart image is on canvasEle
},
}
add a comment |
The answer is: Yes, it is. Your print method in the components' script could be:
methods:{
printChart() {
var canvasEle = document.getElementById('total_visits_chart_bar');
var win = window.open('', 'Print', 'height=600,width=800');
win.document.write("<br><img src='" + canvasEle.toDataURL() + "' />");
setTimeout(function(){ //giving it 200 milliseconds time to load
win.document.close();
win.focus()
win.print();
win.location.reload()
}, 200);
},
}
You can also add this to your component's style:
@media print{
@page {
size: landscape
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53270288%2fis-it-possible-to-print-a-chart-with-vue-chartjs%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
vue-chartjs is based on chart.js and not canvas.js, thus it does not have a "build-in" way of printing.
You have to do it with some custom logic and the native javascript printing functions.
You can however grab the canvas element inside your chart component and generate for example an image and then print that image.
It will get a bit tricky, because you only have access to the canvas inside your chart component. So you will need to maybe wait for an event or prop to trigger the toDataURL
call and then emit the image to your parent component where you can print it. If you want to trigger the print in your parent component.
methods: {
print () {
// grab the canvas and generate an image
let image = this.$refs.canvas.toDataURL('image/png')
// Emits an event with the image
this.$emit('chart:print', image)
}
}
In your parent component:
<template>
<your-chart @chart:print="handlePrint"
<template/>
....
...
methods: {
handlePrint(image) {
const win = window.open('', 'Print', 'height=600, width=800')
win.document.write(`<br><img src='${image}' />`)
win.print()
win.close()
}
}
add a comment |
vue-chartjs is based on chart.js and not canvas.js, thus it does not have a "build-in" way of printing.
You have to do it with some custom logic and the native javascript printing functions.
You can however grab the canvas element inside your chart component and generate for example an image and then print that image.
It will get a bit tricky, because you only have access to the canvas inside your chart component. So you will need to maybe wait for an event or prop to trigger the toDataURL
call and then emit the image to your parent component where you can print it. If you want to trigger the print in your parent component.
methods: {
print () {
// grab the canvas and generate an image
let image = this.$refs.canvas.toDataURL('image/png')
// Emits an event with the image
this.$emit('chart:print', image)
}
}
In your parent component:
<template>
<your-chart @chart:print="handlePrint"
<template/>
....
...
methods: {
handlePrint(image) {
const win = window.open('', 'Print', 'height=600, width=800')
win.document.write(`<br><img src='${image}' />`)
win.print()
win.close()
}
}
add a comment |
vue-chartjs is based on chart.js and not canvas.js, thus it does not have a "build-in" way of printing.
You have to do it with some custom logic and the native javascript printing functions.
You can however grab the canvas element inside your chart component and generate for example an image and then print that image.
It will get a bit tricky, because you only have access to the canvas inside your chart component. So you will need to maybe wait for an event or prop to trigger the toDataURL
call and then emit the image to your parent component where you can print it. If you want to trigger the print in your parent component.
methods: {
print () {
// grab the canvas and generate an image
let image = this.$refs.canvas.toDataURL('image/png')
// Emits an event with the image
this.$emit('chart:print', image)
}
}
In your parent component:
<template>
<your-chart @chart:print="handlePrint"
<template/>
....
...
methods: {
handlePrint(image) {
const win = window.open('', 'Print', 'height=600, width=800')
win.document.write(`<br><img src='${image}' />`)
win.print()
win.close()
}
}
vue-chartjs is based on chart.js and not canvas.js, thus it does not have a "build-in" way of printing.
You have to do it with some custom logic and the native javascript printing functions.
You can however grab the canvas element inside your chart component and generate for example an image and then print that image.
It will get a bit tricky, because you only have access to the canvas inside your chart component. So you will need to maybe wait for an event or prop to trigger the toDataURL
call and then emit the image to your parent component where you can print it. If you want to trigger the print in your parent component.
methods: {
print () {
// grab the canvas and generate an image
let image = this.$refs.canvas.toDataURL('image/png')
// Emits an event with the image
this.$emit('chart:print', image)
}
}
In your parent component:
<template>
<your-chart @chart:print="handlePrint"
<template/>
....
...
methods: {
handlePrint(image) {
const win = window.open('', 'Print', 'height=600, width=800')
win.document.write(`<br><img src='${image}' />`)
win.print()
win.close()
}
}
answered Nov 14 '18 at 9:54


Jakub Juszczak
1,30511127
1,30511127
add a comment |
add a comment |
It seems like the library is based on chartjs not canvasjs https://www.chartjs.org/docs/latest/ you might want to look into how to print a window Quick Print HTML5 Canvas, and remember you have access to the canvas element where your graph is drawn:
methods: {
printChart() {
const canvasEle = this.$el.querySelector('canvas');
//now your chart image is on canvasEle
},
}
add a comment |
It seems like the library is based on chartjs not canvasjs https://www.chartjs.org/docs/latest/ you might want to look into how to print a window Quick Print HTML5 Canvas, and remember you have access to the canvas element where your graph is drawn:
methods: {
printChart() {
const canvasEle = this.$el.querySelector('canvas');
//now your chart image is on canvasEle
},
}
add a comment |
It seems like the library is based on chartjs not canvasjs https://www.chartjs.org/docs/latest/ you might want to look into how to print a window Quick Print HTML5 Canvas, and remember you have access to the canvas element where your graph is drawn:
methods: {
printChart() {
const canvasEle = this.$el.querySelector('canvas');
//now your chart image is on canvasEle
},
}
It seems like the library is based on chartjs not canvasjs https://www.chartjs.org/docs/latest/ you might want to look into how to print a window Quick Print HTML5 Canvas, and remember you have access to the canvas element where your graph is drawn:
methods: {
printChart() {
const canvasEle = this.$el.querySelector('canvas');
//now your chart image is on canvasEle
},
}
answered Nov 12 '18 at 22:47
cal_br_mar
60138
60138
add a comment |
add a comment |
The answer is: Yes, it is. Your print method in the components' script could be:
methods:{
printChart() {
var canvasEle = document.getElementById('total_visits_chart_bar');
var win = window.open('', 'Print', 'height=600,width=800');
win.document.write("<br><img src='" + canvasEle.toDataURL() + "' />");
setTimeout(function(){ //giving it 200 milliseconds time to load
win.document.close();
win.focus()
win.print();
win.location.reload()
}, 200);
},
}
You can also add this to your component's style:
@media print{
@page {
size: landscape
}
}
add a comment |
The answer is: Yes, it is. Your print method in the components' script could be:
methods:{
printChart() {
var canvasEle = document.getElementById('total_visits_chart_bar');
var win = window.open('', 'Print', 'height=600,width=800');
win.document.write("<br><img src='" + canvasEle.toDataURL() + "' />");
setTimeout(function(){ //giving it 200 milliseconds time to load
win.document.close();
win.focus()
win.print();
win.location.reload()
}, 200);
},
}
You can also add this to your component's style:
@media print{
@page {
size: landscape
}
}
add a comment |
The answer is: Yes, it is. Your print method in the components' script could be:
methods:{
printChart() {
var canvasEle = document.getElementById('total_visits_chart_bar');
var win = window.open('', 'Print', 'height=600,width=800');
win.document.write("<br><img src='" + canvasEle.toDataURL() + "' />");
setTimeout(function(){ //giving it 200 milliseconds time to load
win.document.close();
win.focus()
win.print();
win.location.reload()
}, 200);
},
}
You can also add this to your component's style:
@media print{
@page {
size: landscape
}
}
The answer is: Yes, it is. Your print method in the components' script could be:
methods:{
printChart() {
var canvasEle = document.getElementById('total_visits_chart_bar');
var win = window.open('', 'Print', 'height=600,width=800');
win.document.write("<br><img src='" + canvasEle.toDataURL() + "' />");
setTimeout(function(){ //giving it 200 milliseconds time to load
win.document.close();
win.focus()
win.print();
win.location.reload()
}, 200);
},
}
You can also add this to your component's style:
@media print{
@page {
size: landscape
}
}
edited Nov 13 '18 at 13:32
answered Nov 13 '18 at 13:26


Joao Alves Marrucho
337213
337213
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53270288%2fis-it-possible-to-print-a-chart-with-vue-chartjs%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
511LNlgNEUOlAYXXb9,7dI tKk7u0JJRGz5JbCNv3mC3s,4dkfq0w,M72TZh5K3 SrWotk