Can I borrow a slice from a custom type?
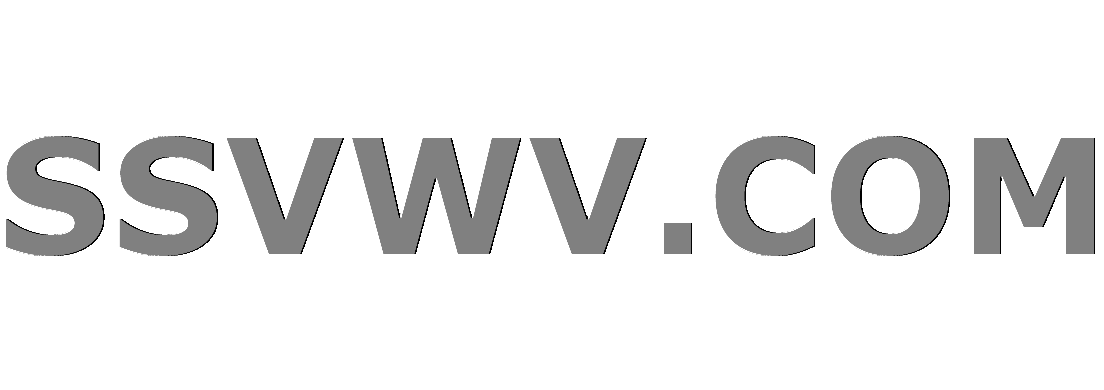
Multi tool use
up vote
0
down vote
favorite
It's possible to borrow a Vec<u32>
into either a &Vec<u32>
or a &[u32]
. I thought this was thanks to either the AsRef
or Borrow
traits. However, I was unable to implement such borrowing on my own custom type. Am I barking up the wrong tree here?
use std::borrow::Borrow;
struct MyArray([u32; 5]);
impl MyArray {
fn new() -> MyArray {
MyArray([42; 5])
}
}
impl AsRef<[u32]> for MyArray {
fn as_ref(&self) -> &[u32] {
&self.0
}
}
impl Borrow<[u32]> for MyArray {
fn borrow(&self) -> &[u32] {
&self.0
}
}
fn main() {
let ma = MyArray::new();
let _: &[u32] = &ma; // compilation failure
}
rust
add a comment |
up vote
0
down vote
favorite
It's possible to borrow a Vec<u32>
into either a &Vec<u32>
or a &[u32]
. I thought this was thanks to either the AsRef
or Borrow
traits. However, I was unable to implement such borrowing on my own custom type. Am I barking up the wrong tree here?
use std::borrow::Borrow;
struct MyArray([u32; 5]);
impl MyArray {
fn new() -> MyArray {
MyArray([42; 5])
}
}
impl AsRef<[u32]> for MyArray {
fn as_ref(&self) -> &[u32] {
&self.0
}
}
impl Borrow<[u32]> for MyArray {
fn borrow(&self) -> &[u32] {
&self.0
}
}
fn main() {
let ma = MyArray::new();
let _: &[u32] = &ma; // compilation failure
}
rust
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
It's possible to borrow a Vec<u32>
into either a &Vec<u32>
or a &[u32]
. I thought this was thanks to either the AsRef
or Borrow
traits. However, I was unable to implement such borrowing on my own custom type. Am I barking up the wrong tree here?
use std::borrow::Borrow;
struct MyArray([u32; 5]);
impl MyArray {
fn new() -> MyArray {
MyArray([42; 5])
}
}
impl AsRef<[u32]> for MyArray {
fn as_ref(&self) -> &[u32] {
&self.0
}
}
impl Borrow<[u32]> for MyArray {
fn borrow(&self) -> &[u32] {
&self.0
}
}
fn main() {
let ma = MyArray::new();
let _: &[u32] = &ma; // compilation failure
}
rust
It's possible to borrow a Vec<u32>
into either a &Vec<u32>
or a &[u32]
. I thought this was thanks to either the AsRef
or Borrow
traits. However, I was unable to implement such borrowing on my own custom type. Am I barking up the wrong tree here?
use std::borrow::Borrow;
struct MyArray([u32; 5]);
impl MyArray {
fn new() -> MyArray {
MyArray([42; 5])
}
}
impl AsRef<[u32]> for MyArray {
fn as_ref(&self) -> &[u32] {
&self.0
}
}
impl Borrow<[u32]> for MyArray {
fn borrow(&self) -> &[u32] {
&self.0
}
}
fn main() {
let ma = MyArray::new();
let _: &[u32] = &ma; // compilation failure
}
rust
rust
edited Nov 11 at 16:50
Shepmaster
145k11274408
145k11274408
asked Nov 11 at 16:37
Michael Snoyman
27.5k23466
27.5k23466
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
3
down vote
accepted
You are looking for std::ops::Deref
:
In addition to being used for explicit dereferencing operations with the (unary)
*
operator in immutable contexts,Deref
is also used implicitly by the compiler in many circumstances. This mechanism is called 'Deref
coercion'. In mutable contexts,DerefMut
is used.
Modified code:
use std::ops::Deref;
struct MyArray([u32; 5]);
impl MyArray {
fn new() -> MyArray {
MyArray([42; 5])
}
}
impl Deref for MyArray {
type Target = [u32];
fn deref(&self) -> &[u32] {
&self.0
}
}
fn main() {
let ma = MyArray::new();
let _: &[u32] = &ma;
}
Related: Is it considered a bad practice to implement Deref for newtypes?.
– Shepmaster
Nov 11 at 16:51
That's exactly what I was missing, thank you!
– Michael Snoyman
Nov 11 at 17:04
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
accepted
You are looking for std::ops::Deref
:
In addition to being used for explicit dereferencing operations with the (unary)
*
operator in immutable contexts,Deref
is also used implicitly by the compiler in many circumstances. This mechanism is called 'Deref
coercion'. In mutable contexts,DerefMut
is used.
Modified code:
use std::ops::Deref;
struct MyArray([u32; 5]);
impl MyArray {
fn new() -> MyArray {
MyArray([42; 5])
}
}
impl Deref for MyArray {
type Target = [u32];
fn deref(&self) -> &[u32] {
&self.0
}
}
fn main() {
let ma = MyArray::new();
let _: &[u32] = &ma;
}
Related: Is it considered a bad practice to implement Deref for newtypes?.
– Shepmaster
Nov 11 at 16:51
That's exactly what I was missing, thank you!
– Michael Snoyman
Nov 11 at 17:04
add a comment |
up vote
3
down vote
accepted
You are looking for std::ops::Deref
:
In addition to being used for explicit dereferencing operations with the (unary)
*
operator in immutable contexts,Deref
is also used implicitly by the compiler in many circumstances. This mechanism is called 'Deref
coercion'. In mutable contexts,DerefMut
is used.
Modified code:
use std::ops::Deref;
struct MyArray([u32; 5]);
impl MyArray {
fn new() -> MyArray {
MyArray([42; 5])
}
}
impl Deref for MyArray {
type Target = [u32];
fn deref(&self) -> &[u32] {
&self.0
}
}
fn main() {
let ma = MyArray::new();
let _: &[u32] = &ma;
}
Related: Is it considered a bad practice to implement Deref for newtypes?.
– Shepmaster
Nov 11 at 16:51
That's exactly what I was missing, thank you!
– Michael Snoyman
Nov 11 at 17:04
add a comment |
up vote
3
down vote
accepted
up vote
3
down vote
accepted
You are looking for std::ops::Deref
:
In addition to being used for explicit dereferencing operations with the (unary)
*
operator in immutable contexts,Deref
is also used implicitly by the compiler in many circumstances. This mechanism is called 'Deref
coercion'. In mutable contexts,DerefMut
is used.
Modified code:
use std::ops::Deref;
struct MyArray([u32; 5]);
impl MyArray {
fn new() -> MyArray {
MyArray([42; 5])
}
}
impl Deref for MyArray {
type Target = [u32];
fn deref(&self) -> &[u32] {
&self.0
}
}
fn main() {
let ma = MyArray::new();
let _: &[u32] = &ma;
}
You are looking for std::ops::Deref
:
In addition to being used for explicit dereferencing operations with the (unary)
*
operator in immutable contexts,Deref
is also used implicitly by the compiler in many circumstances. This mechanism is called 'Deref
coercion'. In mutable contexts,DerefMut
is used.
Modified code:
use std::ops::Deref;
struct MyArray([u32; 5]);
impl MyArray {
fn new() -> MyArray {
MyArray([42; 5])
}
}
impl Deref for MyArray {
type Target = [u32];
fn deref(&self) -> &[u32] {
&self.0
}
}
fn main() {
let ma = MyArray::new();
let _: &[u32] = &ma;
}
edited Nov 11 at 16:52
Shepmaster
145k11274408
145k11274408
answered Nov 11 at 16:50
udoprog
1,557914
1,557914
Related: Is it considered a bad practice to implement Deref for newtypes?.
– Shepmaster
Nov 11 at 16:51
That's exactly what I was missing, thank you!
– Michael Snoyman
Nov 11 at 17:04
add a comment |
Related: Is it considered a bad practice to implement Deref for newtypes?.
– Shepmaster
Nov 11 at 16:51
That's exactly what I was missing, thank you!
– Michael Snoyman
Nov 11 at 17:04
Related: Is it considered a bad practice to implement Deref for newtypes?.
– Shepmaster
Nov 11 at 16:51
Related: Is it considered a bad practice to implement Deref for newtypes?.
– Shepmaster
Nov 11 at 16:51
That's exactly what I was missing, thank you!
– Michael Snoyman
Nov 11 at 17:04
That's exactly what I was missing, thank you!
– Michael Snoyman
Nov 11 at 17:04
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53250856%2fcan-i-borrow-a-slice-from-a-custom-type%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
EPadeq35VzoI ShoEcI1L9GaYXx MySl1ohK74AE2pkSbnHhxyvjjuG0d8OcNJ